In this MATLAB tutorial, you'll quickly learn how to create a simple linear plot using the `plot` function to visualize data.
x = 0:0.1:10; % Create a vector from 0 to 10 with an increment of 0.1
y = sin(x); % Compute the sine of each element in x
plot(x, y); % Plot y versus x
title('Sine Wave'); % Add a title to the plot
xlabel('X-axis'); % Label the x-axis
ylabel('Y-axis'); % Label the y-axis
Introduction to MATLAB
What is MATLAB?
MATLAB, short for “Matrix Laboratory”, is a high-level programming language and environment that is primarily used for numerical computing, data analysis, and algorithm development. Originally created in the late 1970s by Cleve Moler, MATLAB has evolved into a powerful tool widely employed in engineering, scientific research, and various industries.
Why Learn MATLAB?
Learning MATLAB is invaluable for anyone in the fields of engineering and data science. Its capabilities allow users to solve complex mathematical problems, handle large data sets, and develop algorithms quickly and efficiently. From signal processing to machine learning, MATLAB finds application in numerous real-world problems, making it a significant skill to have in the job market.
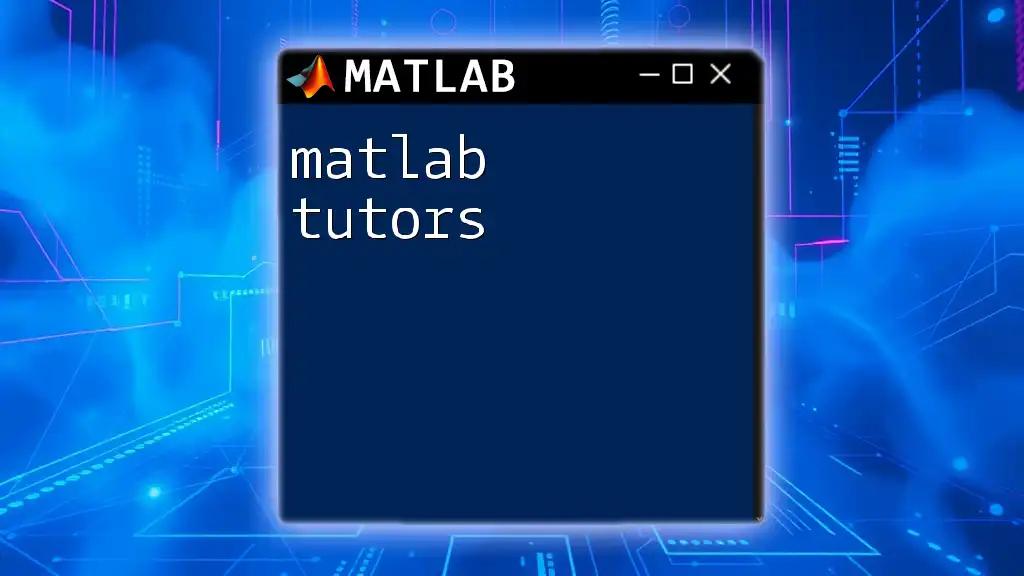
Setting Up Your MATLAB Environment
Installing MATLAB
Before diving into the commands, you need to ensure you have MATLAB installed on your computer. Always check the system requirements to ensure compatibility and follow these steps for installation:
- Download the latest version from the official MathWorks website.
- Follow the installation prompts, which include selecting your installation type (e.g., typical, custom).
- Activate MATLAB with the license provided, whether it be a student version, professional license, or trial.
Navigating the MATLAB Interface
Upon launching MATLAB, you will encounter several key components of the interface:
- Command Window: Where users enter commands directly.
- Editor: Allows for the creation and editing of scripts and functions.
- Workspace: Displays all the variables created during your session.
- Command History: Logs all commands executed for easy reference.
Familiarizing yourself with these components will enhance your productivity while using MATLAB.
Customizing Your Workspace
MATLAB allows users to customize their workspace according to personal preferences. You can set options such as colors, fonts, and the overall layout. Customization not only helps improve visibility but also creates a more comfortable working environment.
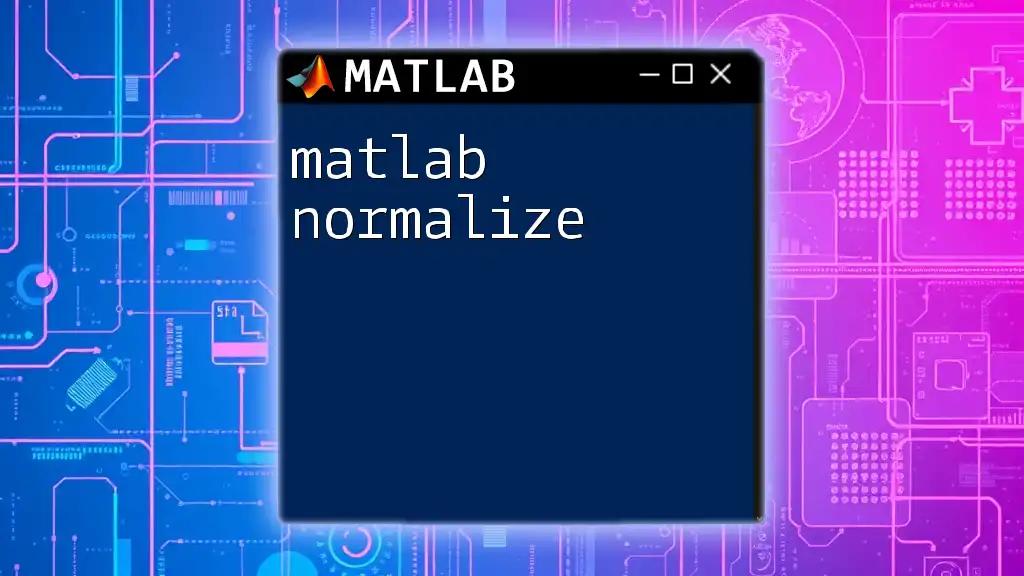
Basic MATLAB Commands
Getting Started with Commands
In MATLAB, commands are entered into the Command Window. To execute a command, simply type it and press Enter. Here are a few fundamental commands that every beginner should know:
- `clc`: Clears the command window.
- `clear`: Clears all variables from the workspace.
- `close all`: Closes all figure windows.
Writing Your First Script
To create a script, open the Editor, where you can write multiple lines of code. Here’s an example of writing a simple MATLAB script that adds two numbers together:
% Simple addition
a = 5;
b = 10;
sum = a + b;
disp(sum);
This script assigns values to `a` and `b`, computes their sum, and then displays the result in the Command Window.

Working with Variables and Data Types
Defining Variables
Variables in MATLAB are created using the assignment operator (`=`). It’s crucial to follow consistent naming conventions, such as starting variable names with a letter and avoiding spaces.
Common Data Types in MATLAB
MATLAB supports various data types, including:
- Scalars: Single numeric values.
- Arrays: Collections of numbers arranged in a linear format.
- Matrices: Two-dimensional arrays, which are crucial in MATLAB.
Here’s an example of creating a simple vector (1x5 array):
% Creating a vector
vector = [1, 2, 3, 4, 5];
Data Type Conversion
MATLAB provides built-in functions to convert between data types. For instance, you can convert an integer to a double (floating-point value) using the `double` function as follows:
myInt = int8(10);
myDouble = double(myInt);
Understanding how to manipulate and convert data types is essential for data handling in MATLAB.
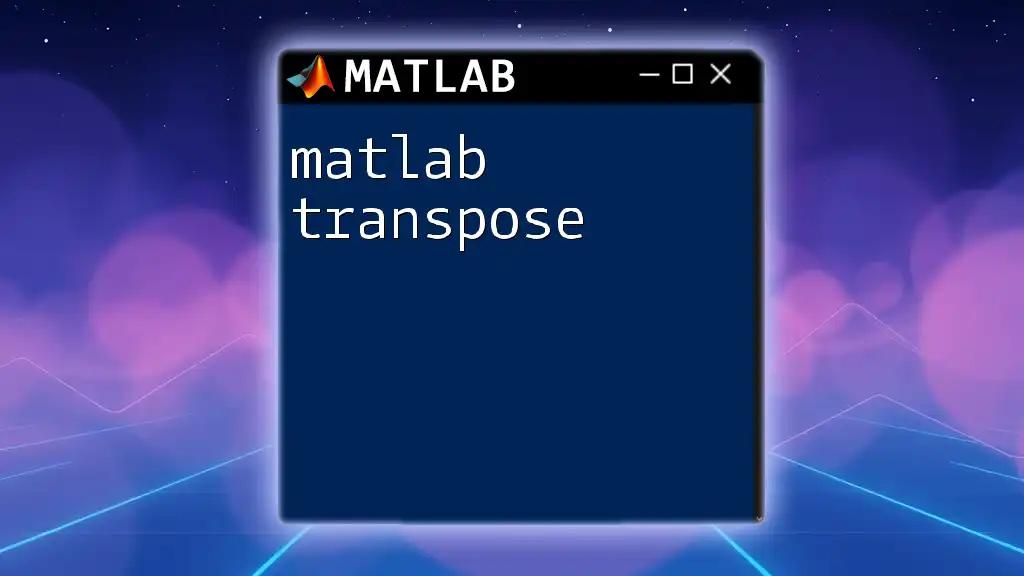
Working with Matrices and Arrays
Introduction to Matrices
Matrices are a cornerstone of MATLAB. They can be created and manipulated with ease. Here’s how you can define a simple 2x2 matrix:
% Creating a 2x2 matrix
A = [1, 2; 3, 4];
Matrix Operations
MATLAB excels at performing operations on matrices, allowing you to execute mathematical calculations seamlessly. You can perform addition, subtraction, and multiplication using typical mathematical syntax:
B = [5, 6; 7, 8];
C = A + B; % Matrix addition
D = A * B; % Matrix multiplication
Understanding matrix operations is pivotal because many mathematical and statistical operations in MATLAB revolve around matrix computations.
Accessing and Modifying Matrix Elements
You can access or modify elements in a matrix using indexing. For example, to access the element in the first row and second column of matrix `A`, you would use:
element = A(1, 2); % Accessing the element in the first row and second column
This powerful feature allows for fine control over data manipulation within matrices.
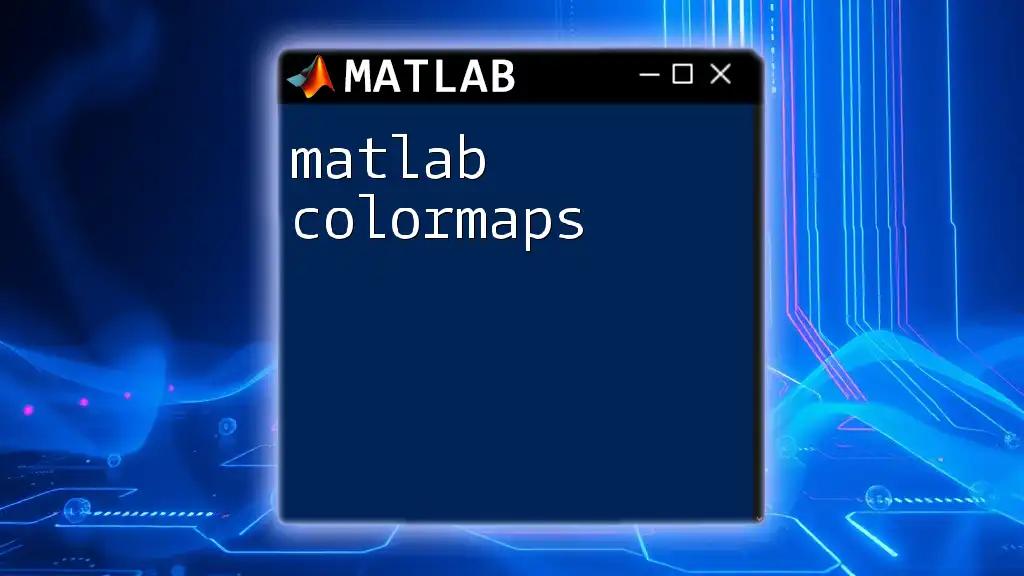
Control Flow and Loops
If-Else Statements
Control flow statements help to make decisions in your code. The structure for an if-else statement is straightforward. For instance:
if a > b
disp('a is greater than b');
else
disp('a is not greater than b');
end
This fundamental structure is essential for any programming language, enabling you to build logical operations.
For and While Loops
Loops allow for repeated execution of code blocks. Here’s how you can create both a for loop and a while loop:
% For loop example
for i = 1:5
disp(i); % Displays numbers from 1 to 5
end
% While loop example
count = 1;
while count <= 5
disp(count);
count = count + 1; % Increment the counter
end
Understanding loops is central to efficiently iterating over datasets or performing repetitive tasks.
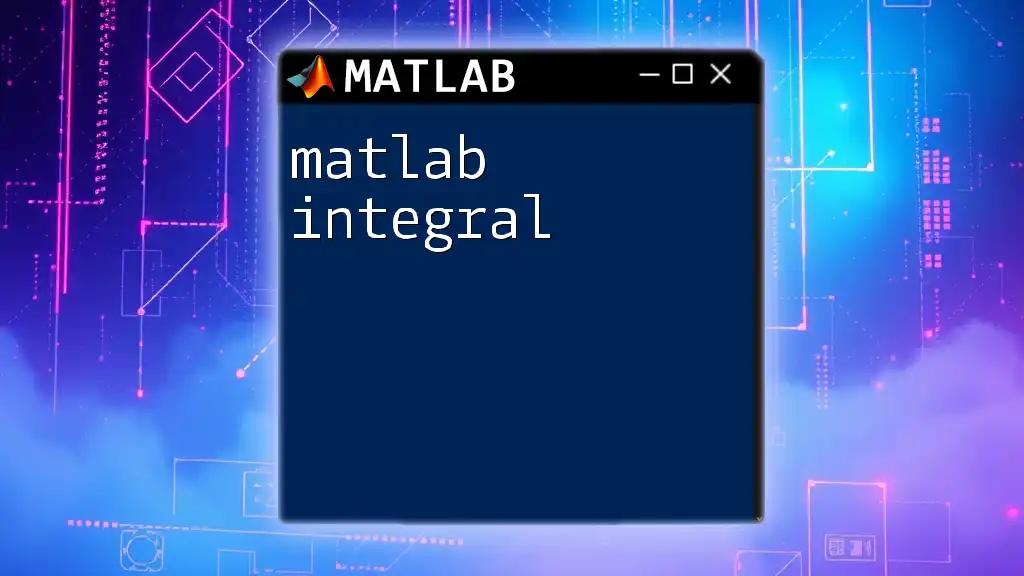
Functions in MATLAB
Creating Functions
Functions are self-contained blocks of code that perform specific tasks. To define a function, use the following syntax:
function result = addNumbers(x, y)
result = x + y; % Function to add two numbers
end
Functions promote code reusability and help in organizing your scripts effectively.
Using Built-in Functions
MATLAB is rich in built-in functions that perform various operations, including mathematical calculations, statistical analysis, and data manipulation. Some commonly used functions include `sum`, `mean`, `max`, and `min`.
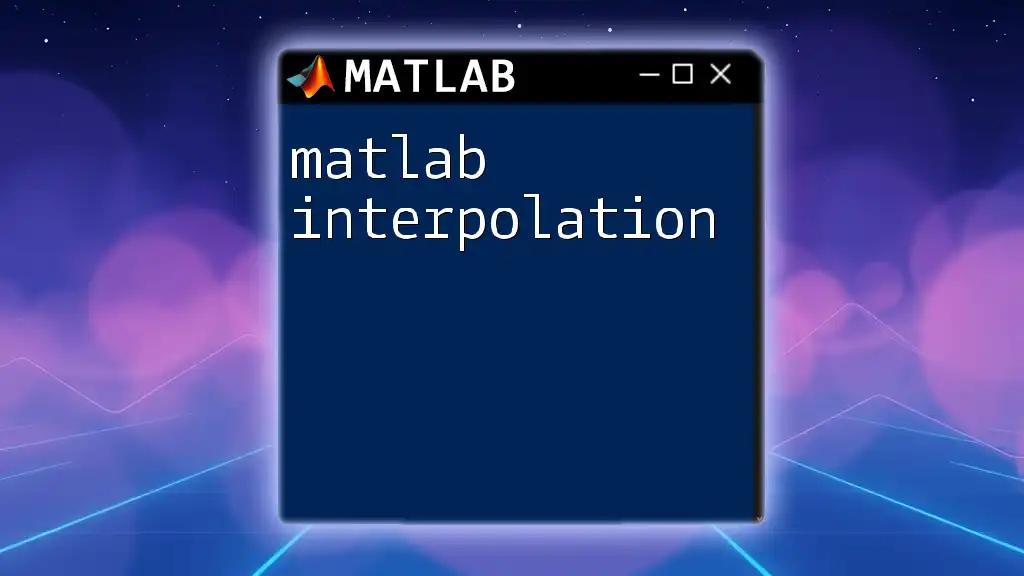
Data Visualization in MATLAB
Introduction to Plotting
Visualizing data is crucial for analysis. MATLAB makes it easy to create plots. For example, to create a simple sine wave plot, you might use:
x = 0:0.1:10; % X values
y = sin(x); % Y values
plot(x, y); % Creating a plot
This command generates a graph of the sine function, providing insight into the data's behavior visually.
Customizing Plots
MATLAB allows you to customize your plots extensively. You can add titles, labels, and legends to make your graphs more informative:
plot(x, y);
title('Sine Wave');
xlabel('X values');
ylabel('Y values');
legend('sin(x)');
These features enhance the clarity and professionalism of your visual presentations.
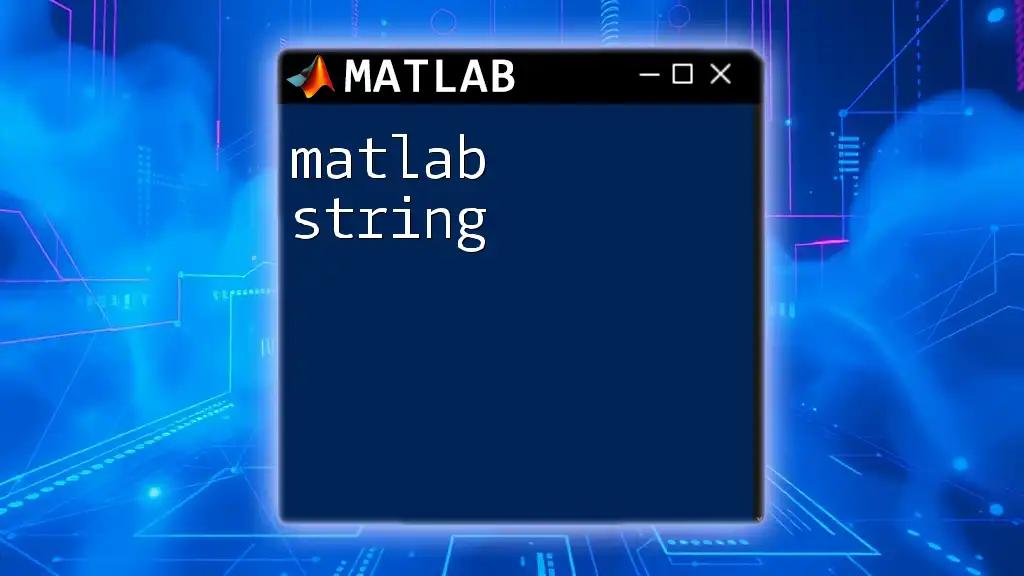
Importing and Exporting Data
Reading Data from Files
MATLAB can process data from various file formats. To read data from a CSV file, use:
data = readtable('data.csv');
This command reads tabular data into a MATLAB table, allowing for easy manipulation and analysis.
Exporting Data
You can save processed results back to files using functions such as `writetable`:
writetable(data, 'output.csv');
Being able to import and export data efficiently is crucial for integrating MATLAB into data workflows.
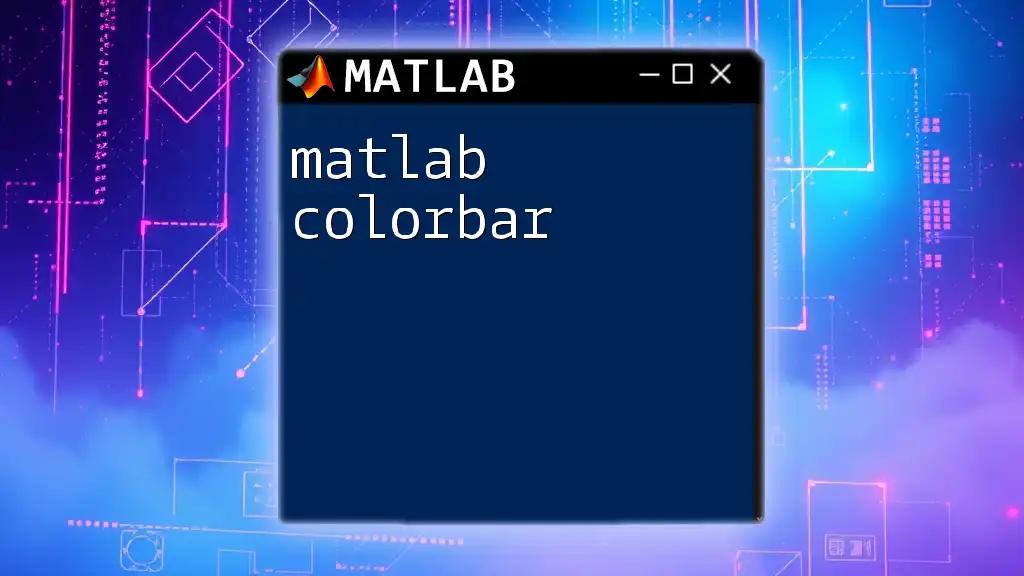
Conclusion
In this comprehensive MATLAB tutorial, we explored the fundamental components of MATLAB—from basic commands and data types to advanced features such as functions, plotting, and data management. Each of these elements is essential for anyone looking to utilize MATLAB effectively.
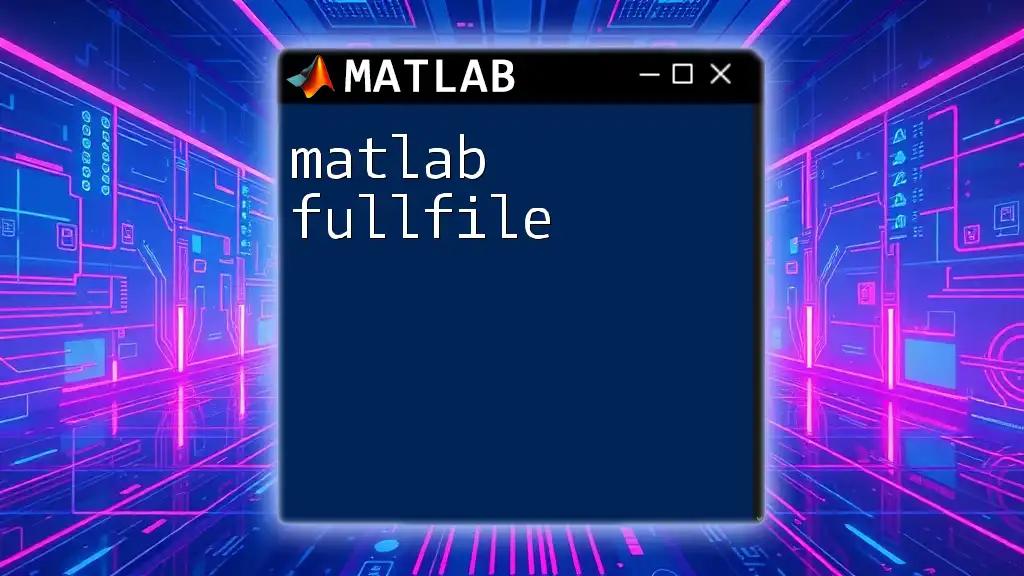
Additional Resources
To further enhance your learning, explore online courses, recommended books, and connect with the MATLAB community through forums where you can get support and share knowledge.
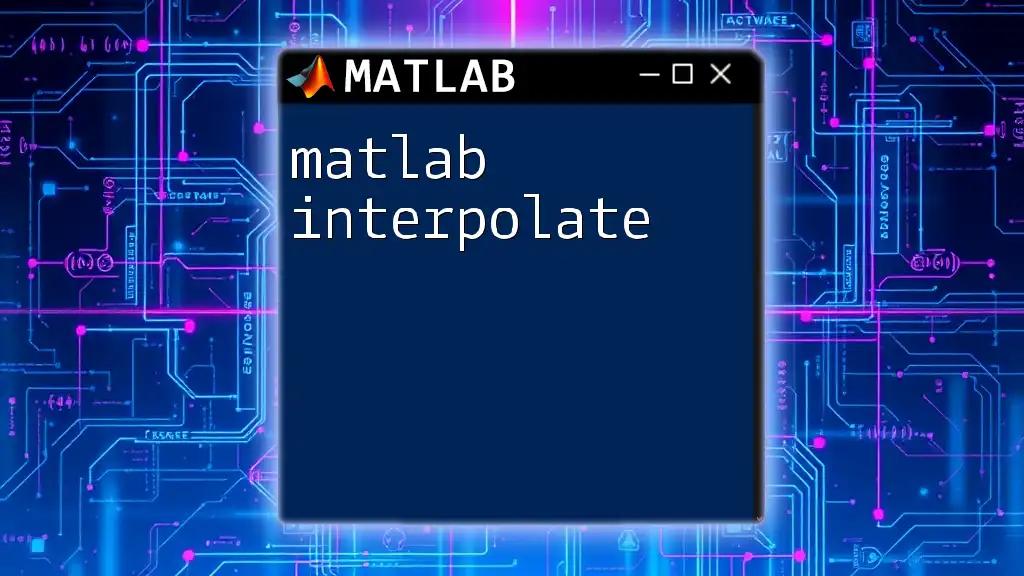
Call to Action
Join Our MATLAB Course
For a hands-on experience and structured learning, consider enrolling in our MATLAB course. Start mastering MATLAB today and unlock its potential for your projects!