The moving average in MATLAB is used to smooth data by averaging a specified number of data points, helping to identify trends over time.
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; % Sample data
windowSize = 3; % Define the window size for the moving average
movingAvg = movmean(data, windowSize); % Calculate the moving average
disp(movingAvg); % Display the results
What is a Moving Average?
Understanding Moving Averages
A moving average is a statistical calculation used to analyze data points by creating averages of various subsets of a dataset. Its primary purpose is to smooth out short-term fluctuations and highlight longer-term trends or cycles.
There are several types of moving averages commonly used:
-
Simple Moving Average (SMA): This is the straightforward average of a set number of data points. It's calculated by adding together values in a fixed window and dividing by the number of points in that window.
-
Exponential Moving Average (EMA): Unlike SMA, EMA gives more weight to recent data points, which makes it more sensitive to recent price movements. This characteristic makes it more responsive in rapidly changing markets.
-
Weighted Moving Average (WMA): Similar to EMA, WMA assigns different weights to each data point, giving more significance to certain points based on predetermined criteria.
Why Use Moving Averages?
Moving averages play a critical role in data analysis. They are widely used in various fields, including finance for stock price analysis, engineering for signal processing, and many other disciplines where time series data is prevalent. Using moving averages helps identify trends, mitigate noise in the data, and forecast future values.
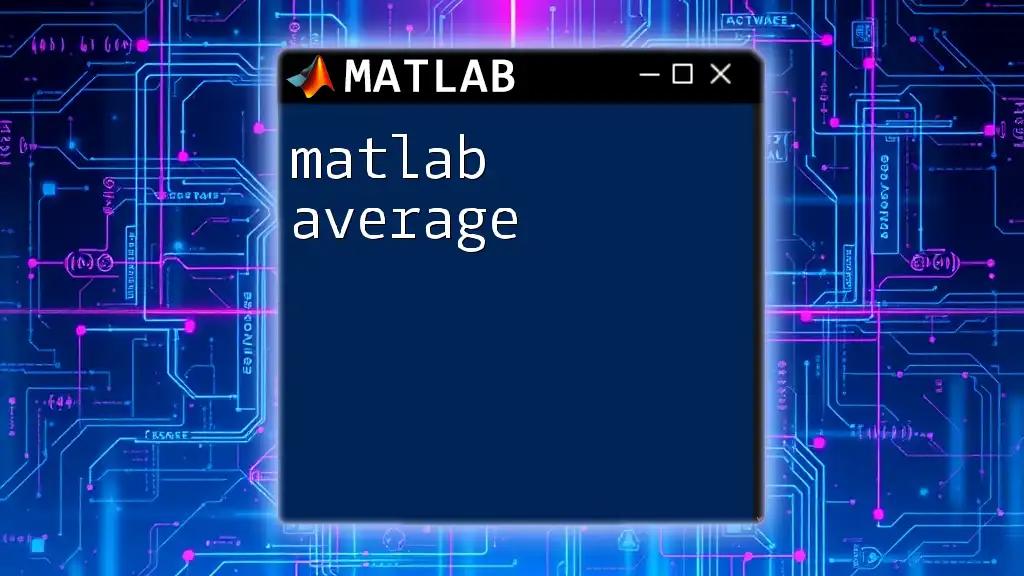
Setting Up MATLAB for Moving Averages
Installing MATLAB
To start using MATLAB for calculating moving averages, you first need to ensure it is installed on your system. The installation process is straightforward:
- Download the MATLAB installer from the official MathWorks website.
- Follow the on-screen instructions to install the software.
- Make sure your system meets the necessary requirements, which usually include a compatible operating system and sufficient RAM.
Importing Required Toolboxes
Before diving into moving average calculations, ensure you have the required toolboxes. You may need the Statistics and Machine Learning Toolbox or any other specialized toolboxes related to your needs. To check if these toolboxes are installed, use the command:
ver
If needed toolboxes are absent, you can install them from the Add-Ons section in MATLAB.
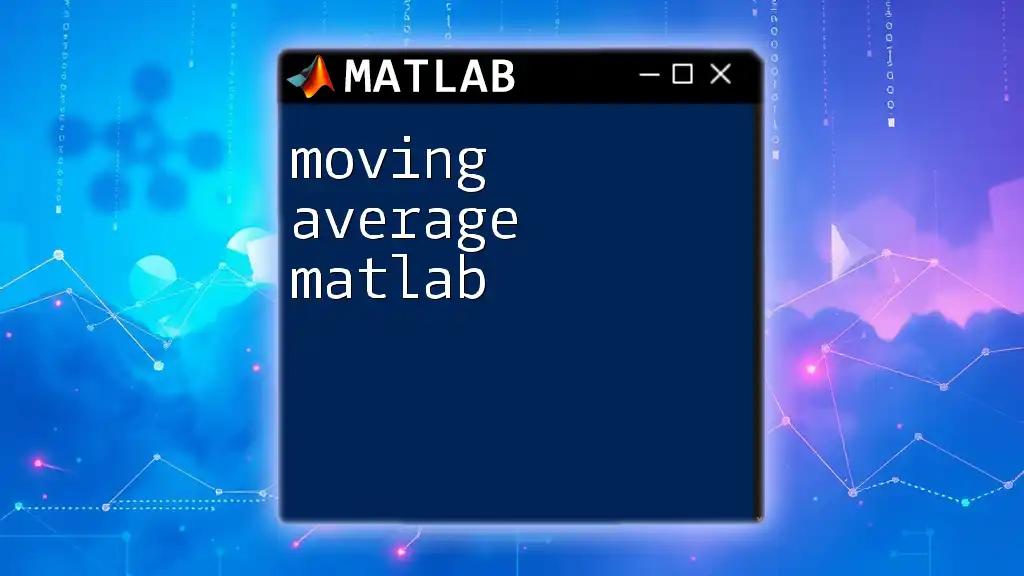
Simple Moving Average (SMA)
Definition and Formula
The Simple Moving Average (SMA) is a sequentially calculated average of a set number of data points in a time series. Its formula can be represented as:
\[ \text{SMA} = \frac{1}{N} \sum_{i=0}^{N-1} x[i] \]
where \(N\) is the number of periods.
While easy to compute, the SMA has its limitations, particularly regarding sensitivity to price movements; it lags behind real-time data.
How to Calculate SMA in MATLAB
In MATLAB, you can easily compute the SMA using the built-in `movmean` function. Here’s an example:
data = [3, 5, 2, 8, 7, 10, 12, 6, 5];
windowSize = 3;
smaResult = movmean(data, windowSize);
disp(smaResult);
In this snippet:
- `data` is an array of numerical values.
- `windowSize` defines the look-back period for averaging.
- The output `smaResult` will contain the calculated SMA based on the specified window size.
Visualizing SMA
To visualize the computed SMA against the original dataset, you can use MATLAB's plotting functions. Here’s how you can create a simple plot:
figure;
plot(data, 'o-'); hold on;
plot(smaResult, 'r-', 'LineWidth', 2);
legend('Original Data', 'SMA');
title('Simple Moving Average');
xlabel('Time');
ylabel('Value');
grid on;
This code plots the original data points and overlays the calculated SMA, making it easy to observe the trend.
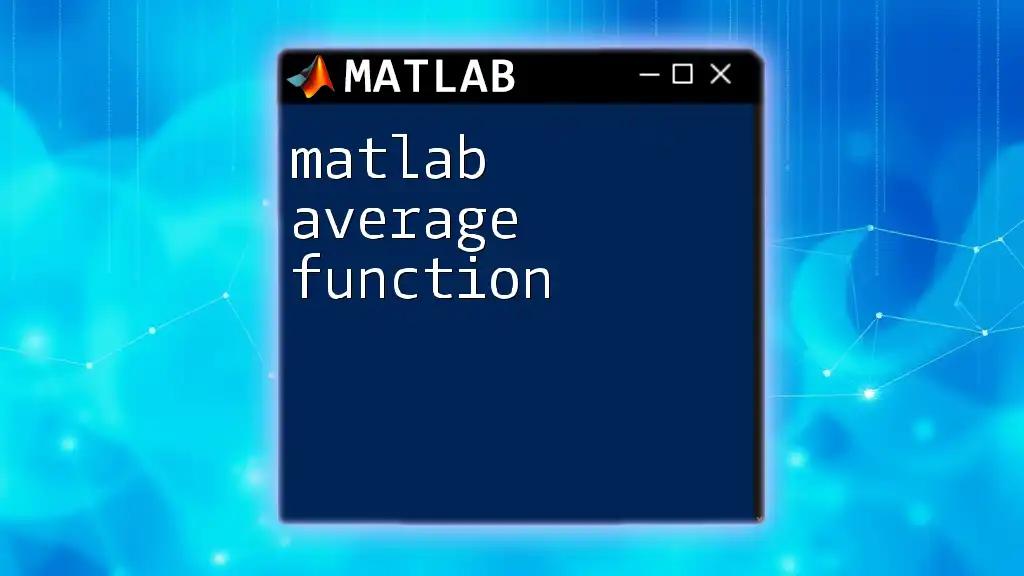
Exponential Moving Average (EMA)
Definition and Formula
The Exponential Moving Average (EMA) calculates the average of a dataset while placing more significance on recent data points. The formula for the EMA is:
\[ \text{EMA} = \alpha \cdot x + (1 - \alpha) \cdot \text{EMA}_{\text{prev}} \]
where \( \alpha \) is the smoothing factor, often calculated as \( \frac{2}{N+1} \) and \(x\) represents the current data point.
How to Calculate EMA in MATLAB
To compute the EMA in MATLAB, you can use a loop or vectorized calculations. Here’s a simple implementation using a for loop:
alpha = 0.1; % Smoothing factor
emaResult = zeros(size(data));
emaResult(1) = data(1); % Initializing first value
for i = 2:length(data)
emaResult(i) = alpha * data(i) + (1 - alpha) * emaResult(i - 1);
end
disp(emaResult);
In this code:
- The first value of the EMA is initialized as the first data point.
- For the remaining values, the EMA is recalculated using the current data point and the previous EMA.
Visualizing EMA
Comparing EMA with the SMA can provide insights into the movement characteristics. Here’s how to visualize the EMA:
figure;
plot(data, 'o-', 'DisplayName', 'Original Data'); hold on;
plot(emaResult, 'g-', 'LineWidth', 2, 'DisplayName', 'EMA');
legend show;
title('Exponential Moving Average');
xlabel('Time');
ylabel('Value');
grid on;
This visualization will allow you to see how EMA reacts to changes in the data compared to SMA.
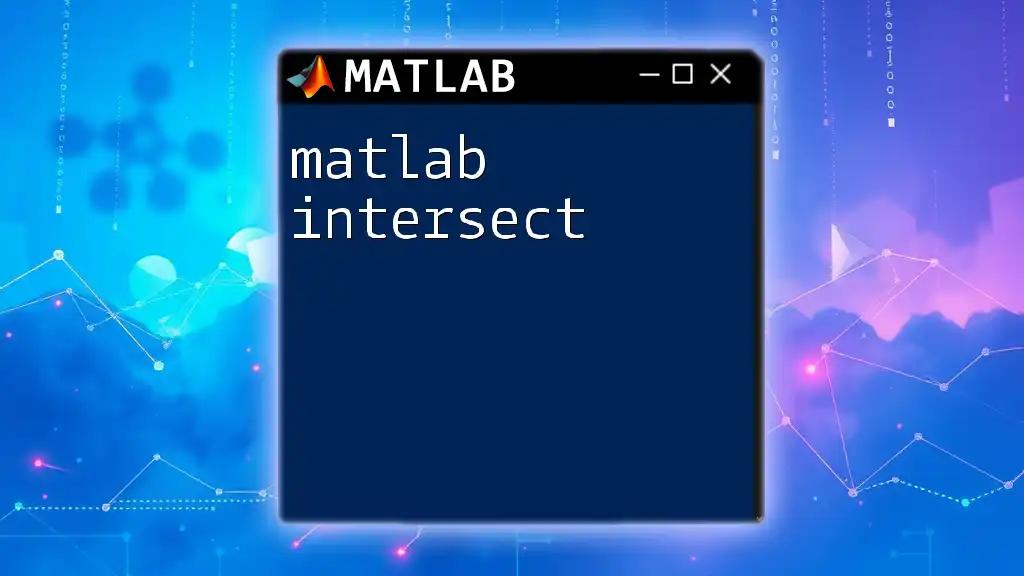
Weighted Moving Average (WMA)
Definition and Formula
The Weighted Moving Average (WMA) is similar to the SMA but assigns different weights to data points, making it a more tailored approach for analyses where certain data points are more significant.
How to Calculate WMA in MATLAB
To compute the WMA in MATLAB, you can use the following code snippet that uses custom weights:
weights = [0.2, 0.3, 0.5]; % Custom weights for the last three data points
wmaResult = zeros(size(data));
for i = 1:length(data)-length(weights)+1
wmaResult(i + length(weights) - 1) = sum(data(i:i + length(weights) - 1) .* weights);
end
disp(wmaResult);
In this example:
- Custom weights are assigned to the last three data points.
- The WMA is computed by taking the weighted average of these points.
Visualizing WMA
To visualize how the WMA compares against SMA and EMA, use the following code:
figure;
plot(data, 'o-', 'DisplayName', 'Original Data'); hold on;
plot(wmaResult, 'b-', 'LineWidth', 2, 'DisplayName', 'WMA');
legend show;
title('Weighted Moving Average');
xlabel('Time');
ylabel('Value');
grid on;
This plot will illustrate how WMA follows the trend differently from other moving averages due to the weight distribution.
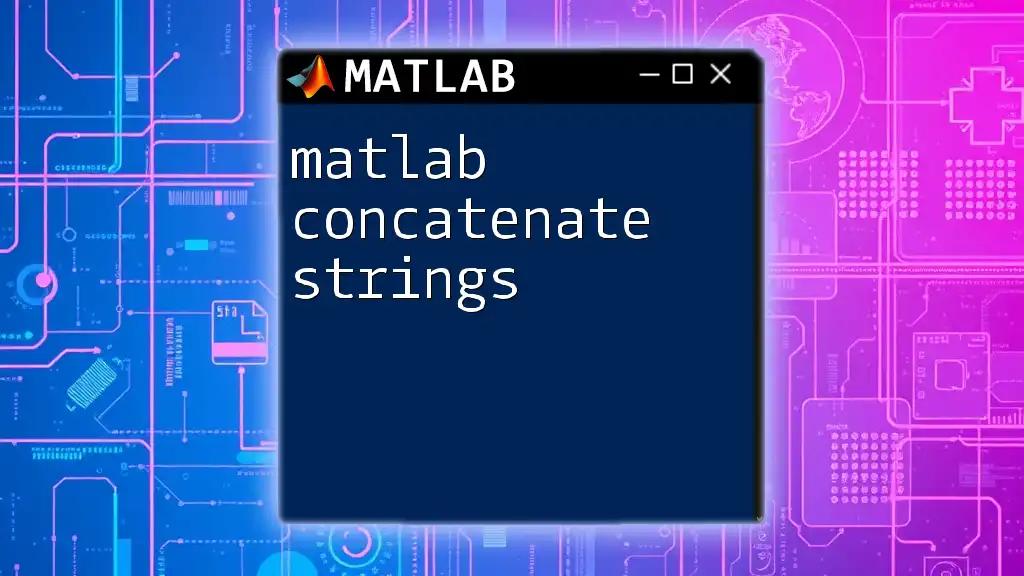
Applications of Moving Averages
In Finance
In the finance sector, moving averages are extensively used for technical analysis. Traders often utilize moving averages to observe market trends. For instance, moving averages can help identify bullish and bearish trends by comparing short-term and long-term moving averages, often referred to as the "crossover strategy."
In Engineering
Moving averages find critical applications in engineering, particularly in signal processing. They help filter out noise from signals, improve the performance of control systems, and allow engineers to make more precise measurements in monitoring systems.
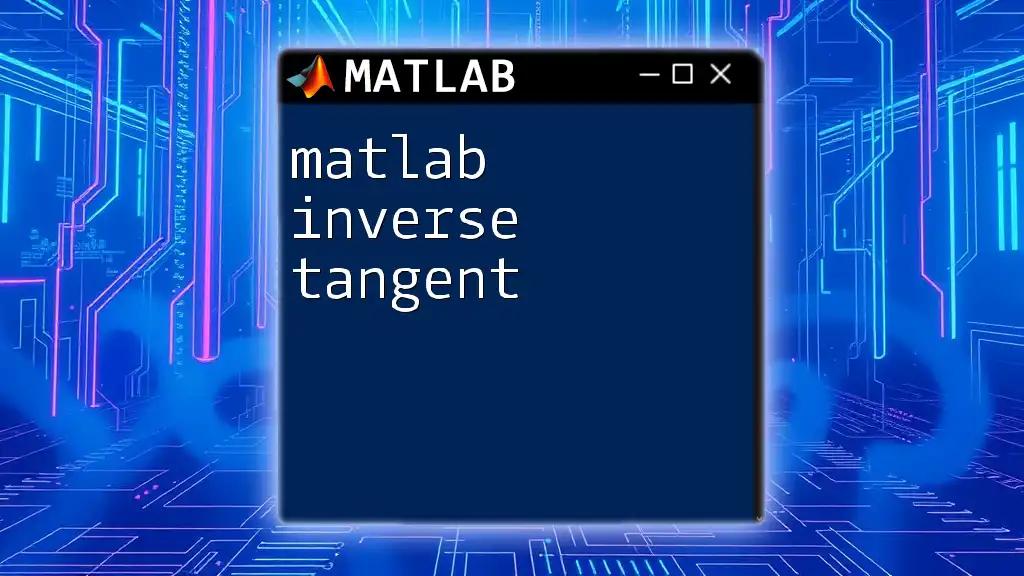
Conclusion
In this extensive guide, we've explored the concept of MATLAB moving average, covering the Simple Moving Average (SMA), Exponential Moving Average (EMA), and Weighted Moving Average (WMA). Each method has unique features that serve various analytical needs. By utilizing MATLAB's robust functions, you can enhance your data analysis skill set and apply these techniques effectively in your projects. Experiment with the provided examples, and consider diving deeper into MATLAB's documentation and resources to further enhance your proficiency in using moving averages.