The MATLAB colon operator (:) is a versatile tool used to create vectors, select array elements, and define ranges, allowing for concise manipulation of data.
Here’s an example of using the colon operator to create a vector of even numbers from 2 to 10:
evenNumbers = 2:2:10; % Creates a vector [2, 4, 6, 8, 10]
What is the MATLAB Colon Operator?
The MATLAB colon operator is a powerful and versatile tool that simplifies the creation of vectors, matrix slicing, and indexing within MATLAB. It is a fundamental part of MATLAB programming that helps in generating sequences and accessing array elements efficiently. Mastering the colon operator enables users to write cleaner, more readable code while enhancing their productivity in numerical computations.
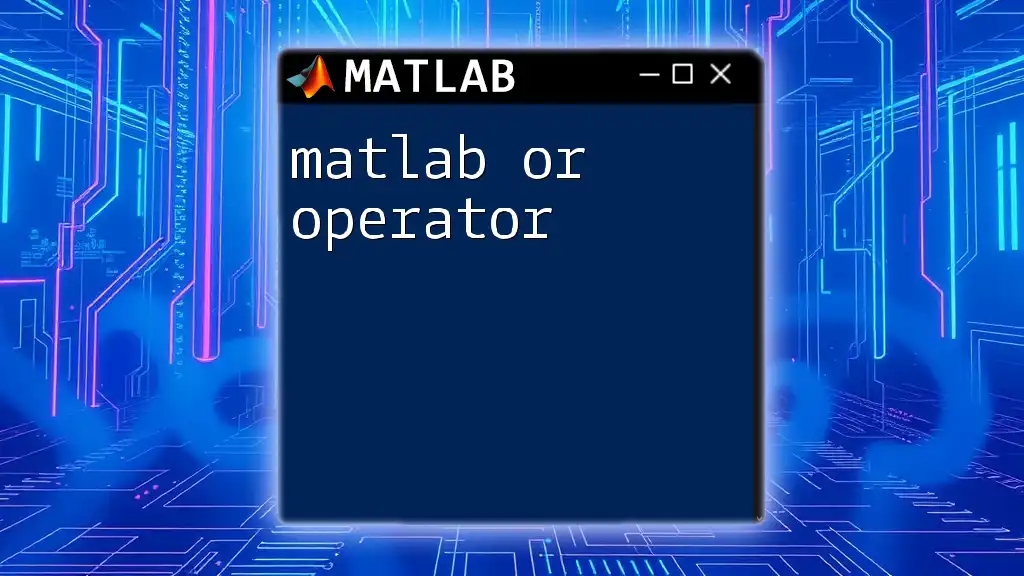
Understanding the Syntax of the Colon Operator
Basic Syntax
The basic structure of the MATLAB colon operator follows the pattern:
start:increment:end
Where:
- start is the first value in the sequence.
- increment specifies the difference between consecutive values (optional).
- end is the last value in the sequence.
For instance, if you utilize the command `1:5`, MATLAB returns the vector:
1 2 3 4 5
This simple syntax generates a regularly spaced sequence of numbers.
Different Variations of the Colon Operator
Basic Ranges
Using just the start and end values creates a sequence from the starting number to the ending number. For example, `1:10` produces:
1 2 3 4 5 6 7 8 9 10
With Increment
When you specify an increment, it alters the step size. For example:
1:2:10
This will yield the result:
1 3 5 7 9
Here, MATLAB starts at 1, increments by 2, and stops before exceeding 10.
Negative Ranges
The colon operator can also be used to create descending sequences. For example, the command `10:-2:0` results in:
10 8 6 4 2 0
This is particularly useful when you want to iterate in reverse order in loops or generate sequences in a descending manner.
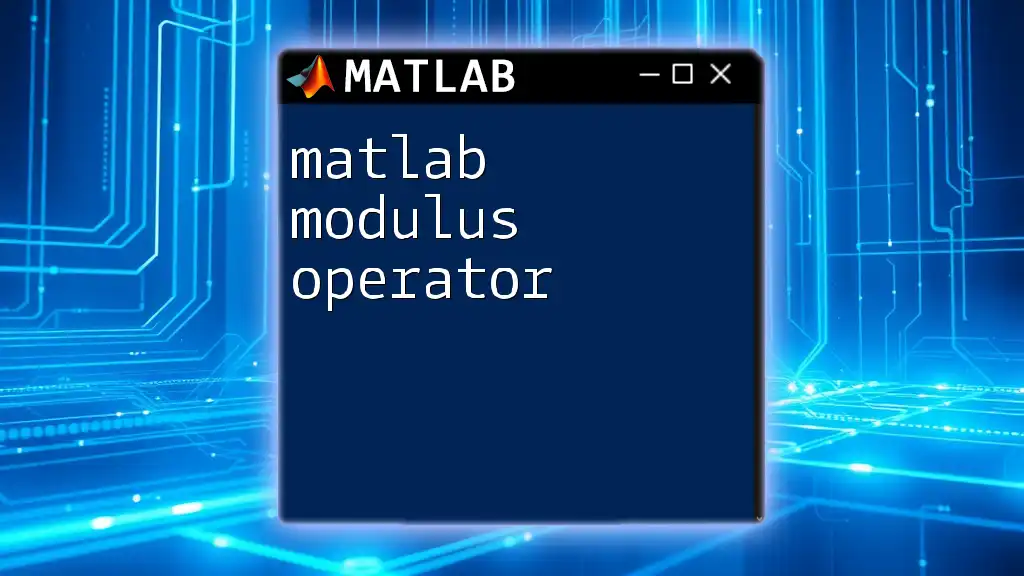
Use Cases of the Colon Operator
Generating Vectors
The colon operator is invaluable for creating both row and column vectors. For instance, you can create a row vector using:
rowVector = 1:5;
If you need a column vector, you simply transpose the row vector:
columnVector = (1:5)';
This distinction is crucial when programming in MATLAB, as it is sensitive to the orientation of matrices.
Slicing Arrays
One of the key applications of the colon operator is slicing arrays. It allows users to directly access subsets of data within matrices.
For example, consider the matrix:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
If you need to access the first two rows of the matrix, you can use:
B = A(1:2, :);
This returns:
1 2 3
4 5 6
In this case, the colon operator selects all columns while specifying the desired row range.
Selecting Specific Elements
Moreover, you can use the colon operator to select entire rows or columns. For instance, to extract the second column from matrix `A`, you would write:
C = A(:, 2);
This command yields:
2
5
8
The colon operator effectively allows the selection of elements at specific intervals and dimensions.
Looping Constructs
The colon operator significantly enhances the efficiency of loop constructions. By using it within a `for` loop, you can iterate through a specified range easily. Here’s a basic loop example:
for i = 1:5
disp(i)
end
In this loop, `i` takes on the values 1 through 5, enabling seamless iteration while reducing the complexity of your code.
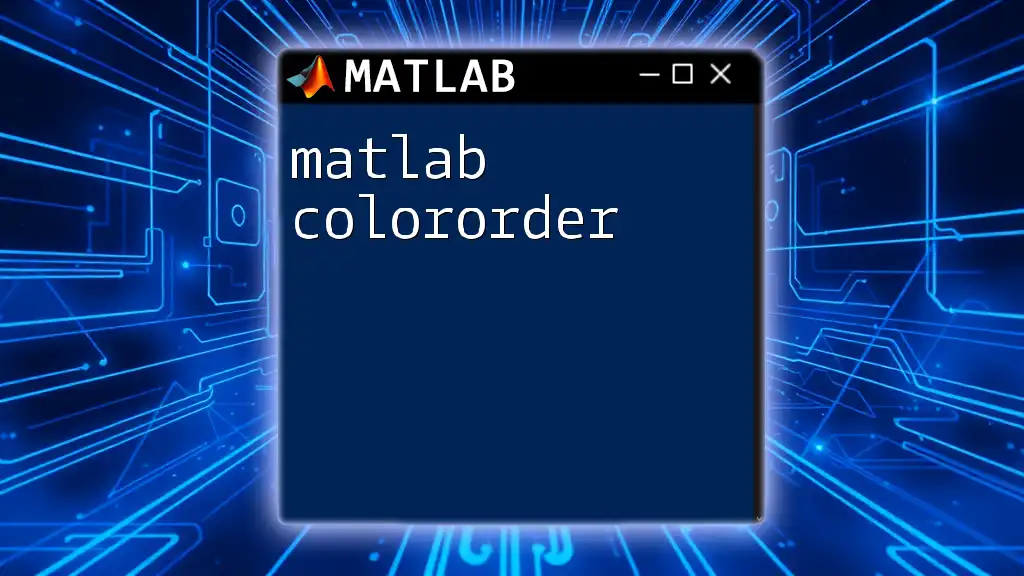
Combining Colon Operator with Other Functions
Using the Colon Operator with `linspace` and `logspace`
While the colon operator allows for straightforward range creation, functions like `linspace` and `logspace` serve similar purposes but with a different approach.
For example, you can use the colon operator to achieve the following:
v1 = 1:0.5:3; % Creates [1, 1.5, 2, 2.5, 3]
On the other hand, `linspace` generates a sequence with equal spacing between elements based on a specified number of points:
v2 = linspace(1, 3, 5); % Creates [1, 1.5, 2, 2.5, 3]
Understanding both methods is essential, as each has its specific use case depending on the desired output or range configuration.
Advanced Applications with Plotting Functions
The colon operator is also instrumental in data visualization tasks. For example, when setting up custom plots, you can generate an x-axis array and corresponding y-values using the sine function:
x = 0:0.1:2*pi;
y = sin(x);
plot(x, y);
title('Sine Wave');
In this context, the colon operator creates a range of `x` values from 0 to \(2\pi\), allowing for smooth curve plotting without manually specifying each point.
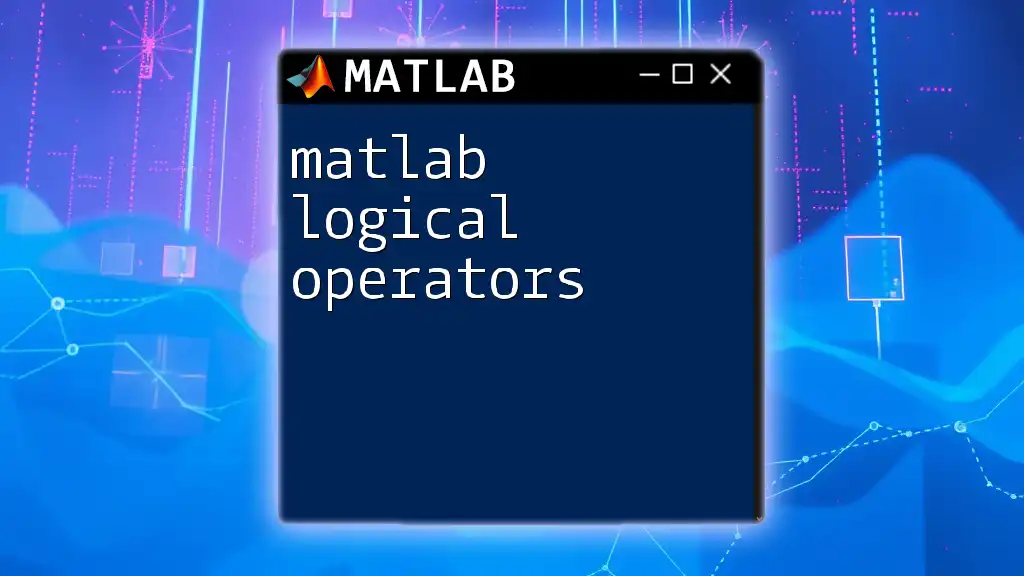
Common Mistakes to Avoid
Syntax Errors
A frequent mistake novices encounter is improperly specifying values in the colon operator. An example of this is using `1:0:10`, which leads to an error since the increment value cannot be zero. Always ensure that the increment correctly separates the values in your range.
Misunderstanding Dimensions
Another common error is misunderstanding the distinction between row and column vectors. MATLAB treats arrays differently based on their orientation, which can lead to unintended dimensional outcomes. Always verify the form of your vectors to avoid issues down the line.
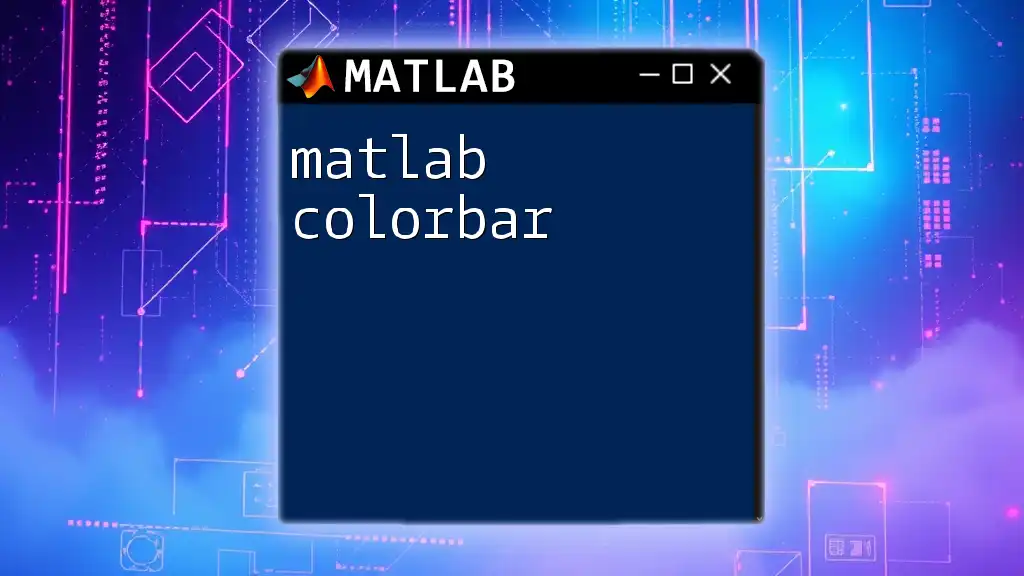
Conclusion
In summary, the MATLAB colon operator is a fundamental aspect of efficient programming within the MATLAB environment. Whether you are generating vectors, slicing arrays, or implementing loops, mastering the colon operator can streamline your workflow and enhance code readability. As you practice and experiment with these concepts, you will find that the colon operator is not just a syntax element but a key to unlocking MATLAB's powerful capabilities.
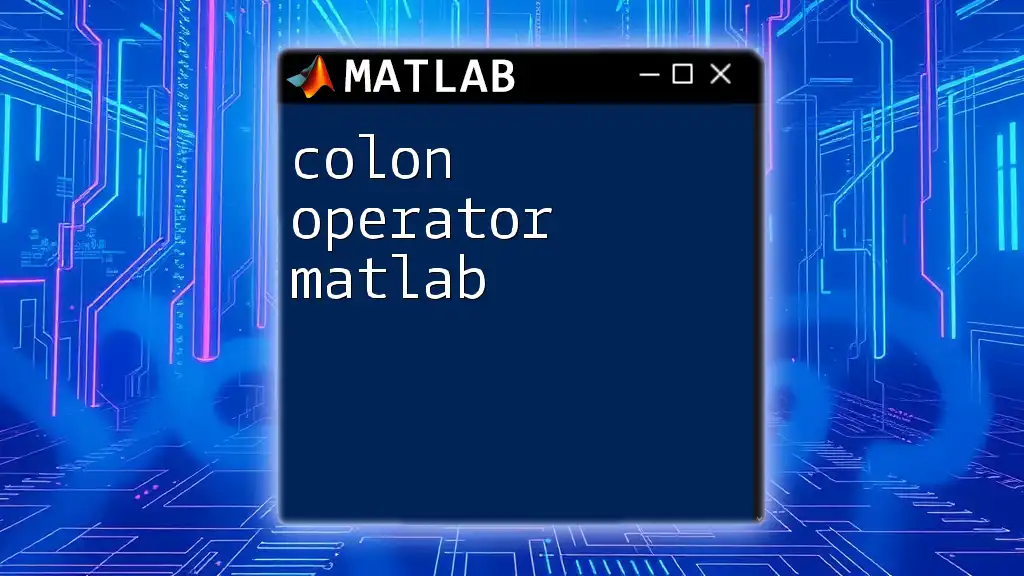
Additional Resources
To further deepen your understanding of the MATLAB colon operator and its applications, consider exploring the official MATLAB documentation, which offers comprehensive explanations and examples. Additionally, pursuing recommended books or online courses can help solidify your foundation in MATLAB programming. Engaging in community forums can also provide valuable insights and support as you embark on your MATLAB learning journey.