The moving average in MATLAB is a statistical method used to smooth data by averaging values from a specified number of neighboring points.
Here's a simple example of how to calculate a moving average using MATLAB:
% Define your data
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
% Define the window size for moving average
windowSize = 3;
% Calculate the moving average
movingAvg = movmean(data, windowSize);
% Display the result
disp(movingAvg);
Types of Moving Averages
Simple Moving Average (SMA)
The simple moving average is the most basic form of moving average. It calculates the average of a data set over a specified number of periods (the window size). The formula for SMA is as follows:
\[ SMA_t = \frac{X_{t} + X_{t-1} + \ldots + X_{t-n+1}}{n} \]
Where \(X\) is the value of the data points and \(n\) is the window size. SMA is widely used across various fields including finance, where it's popular for analyzing stock prices to identify trends over time.
Weighted Moving Average (WMA)
Unlike SMA, the weighted moving average assigns different weights to each data point based on its time position. Recent data points typically receive higher weights than older ones, making the WMA more responsive to changes. This is particularly beneficial in situations where recent values are more significant in forecasting future trends.
Exponential Moving Average (EMA)
The exponential moving average builds on the concept of WMA by applying exponentially decreasing weights. This means that the most recent data points have a greater influence on the average than those further back in time. The calculation of EMA relies on a smoothing factor denoted as alpha, which usually ranges between 0 and 1. The smoothing effect allows the EMA to adapt quickly to changes, making it especially useful in volatile markets.
Cumulative Moving Average (CMA)
The cumulative moving average is slightly different from the previous types; it continuously computes the average of all data points up to the current point. This means that the CMA value evolves as new data comes in, which is ideal for real-time data analysis and applications requiring immediate updates.
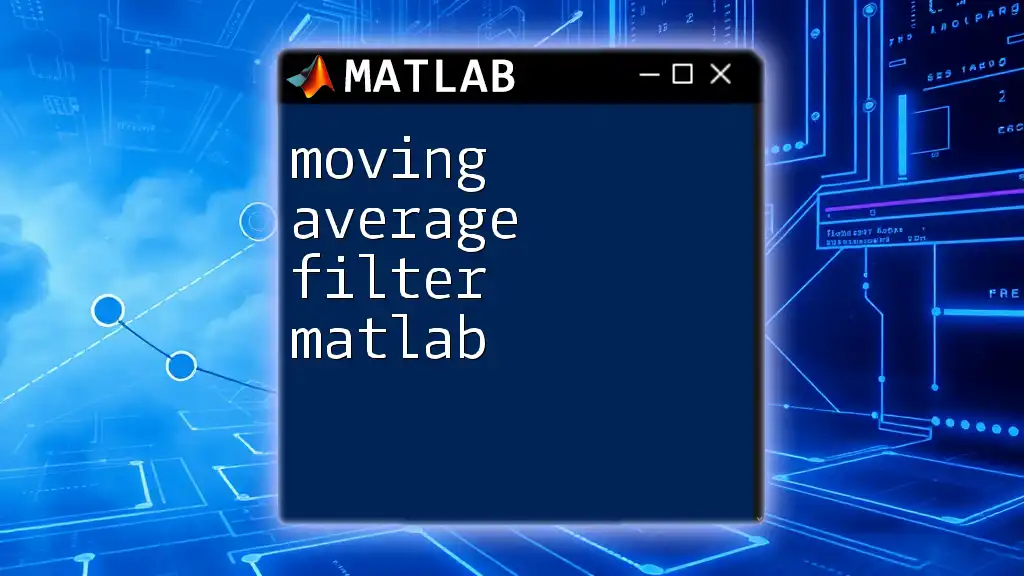
Implementation of Moving Averages in MATLAB
Setting Up MATLAB
To start implementing moving averages in MATLAB, you need to have MATLAB installed on your computer. MATLAB provides a user-friendly environment where you can easily input commands, visualize data, and manipulate matrices.
Simple Moving Average
MATLAB Command for SMA
To calculate a simple moving average in MATLAB, you can use the `movmean()` function. Here’s an example:
data = [1 2 3 4 5 6 7 8 9 10];
windowSize = 3;
sma_values = movmean(data, windowSize);
In this code, we define an array `data`, representing our dataset, along with the `windowSize`, which determines how many past periods are included in the average. The `movmean` function computes the SMA.
Visualizing SMA
To visualize the results and see how the SMA fits with the original data, use MATLAB’s plotting functions:
figure;
plot(data);
hold on;
plot(sma_values);
legend('Original Data','Simple Moving Average');
title('Simple Moving Average in MATLAB');
This code creates a plot that displays both the original data and the SMA, allowing you to quickly understand trends and the smoothing effect of the average.
Weighted Moving Average
MATLAB Command for WMA
To compute a weighted moving average, you can create a custom function that applies weights. Here’s a simple illustration:
weights = [0.1, 0.2, 0.3, 0.4];
wma_values = movmean(data, length(weights), 'Endpoints', 'discard');
In this example, `weights` represents the weights assigned to each corresponding data point in the moving window. Adjusting these values can help you tune how responsive the WMA is to recent changes.
Custom Function for WMA
For a more precise control over how you calculate WMA, consider creating a custom function:
function wma = weightedMovingAverage(data, weights)
wma = conv(data, weights, 'valid');
end
This function uses the convolution operation to compute the weighted moving average and provides a flexible way to experiment with different weights.
Exponential Moving Average
MATLAB Command for EMA
Calculating an exponential moving average in MATLAB requires a bit of manual coding, but it’s straightforward. Below is an example:
alpha = 0.2; % Smoothing factor
ema_values = zeros(size(data));
ema_values(1) = data(1); % Initialize EMA
for i = 2:length(data)
ema_values(i) = alpha * data(i) + (1 - alpha) * ema_values(i-1);
end
In this loop, we initialize the first EMA value to the first data point and apply the formula iteratively for the rest of the data points, ensuring that the EMA captures the influence of more recent data.
Plotting EMA Results
Similar to the SMP, visualize the EMA against the original data:
figure;
plot(data);
hold on;
plot(ema_values);
legend('Original Data','Exponential Moving Average');
title('Exponential Moving Average in MATLAB');
This plot illustrates how the EMA responds more rapidly to recent changes compared to the SMA.
Cumulative Moving Average
MATLAB Command for CMA
To calculate the cumulative moving average, you can take advantage of MATLAB’s built-in functions:
cma_values = cumsum(data) ./ (1:length(data));
This code utilizes `cumsum()` to calculate the cumulative sum of the data, then divides it by a vector that represents the count of values processed so far. The result is an average that continuously updates.
Visualization of CMA
As with the other moving averages, it’s essential to visualize the cumulative moving average alongside the original data:
figure;
plot(data);
hold on;
plot(cma_values);
legend('Original Data','Cumulative Moving Average');
title('Cumulative Moving Average in MATLAB');
This visualization will help you see how the CMA steadies itself as more data points are incorporated.
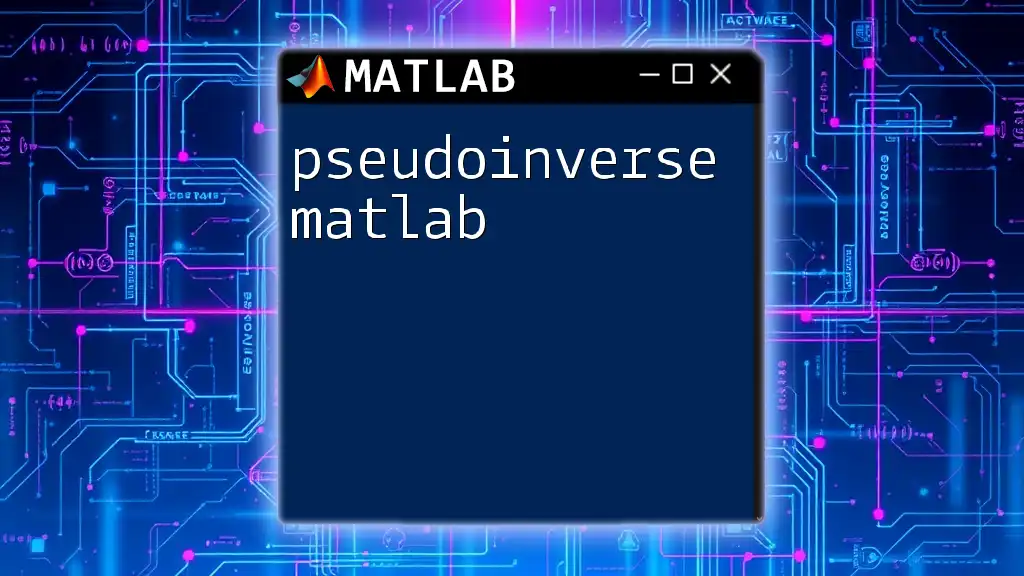
Applications of Moving Averages
Moving averages find extensive use in various domains. In Stock Market Analysis, they help traders identify trends and determine optimal entry and exit points. The crossovers between different moving averages can signal potential buy or sell opportunities, significantly influencing trading decisions.
In Signal Processing, moving averages play a critical role in filtering out noise from data, enabling clearer analysis of the underlying signal. They are often employed for smoothing sensor data or for performing time-series forecasts.
In Forecasting, moving averages assist data scientists and analysts in making predictions about future values based on historical data trends, which is valuable across numerous industries, from finance to manufacturing.
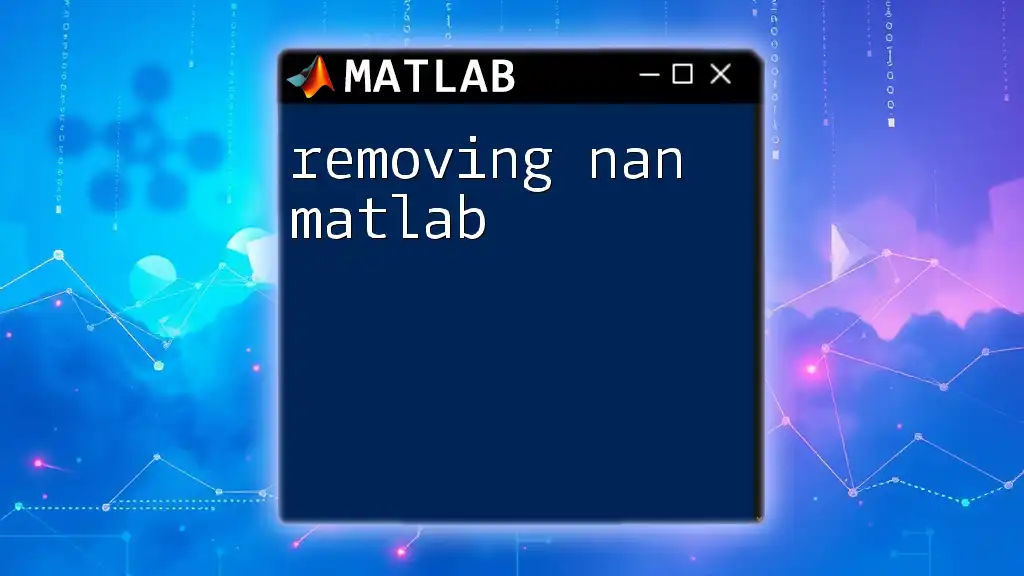
Tips for Using Moving Averages Effectively
When working with moving averages in MATLAB, selecting the right window size is crucial. A small window size can make averages too sensitive, while a large window may smooth out essential fluctuations and trends. Experimenting with various window sizes for your specific application can provide valuable insights.
Avoiding Overfitting is another essential consideration. It’s vital to balance how much sensitivity you want with stability. If the moving average is too reactive, it may produce false signals and lead to potential losses, particularly in financial applications.
Combining moving averages with other analysis techniques, such as trend analysis or momentum indicators, can yield deeper insights. By utilizing moving averages alongside other methods, analysts can enhance the robustness and accuracy of their conclusions.
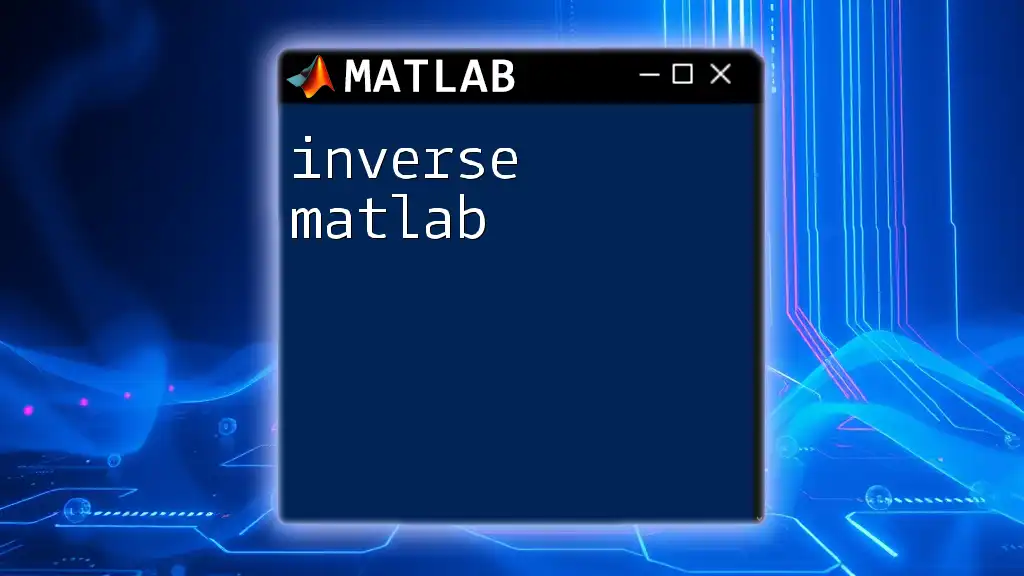
Conclusion
Understanding and implementing moving averages in MATLAB is a powerful skill for anyone involved in data analysis, finance, forecasting, or signal processing. With the practical examples and code snippets provided, you can start applying moving averages directly in your own MATLAB projects. So, go ahead and experiment with these techniques to uncover new trends and insights in your data!
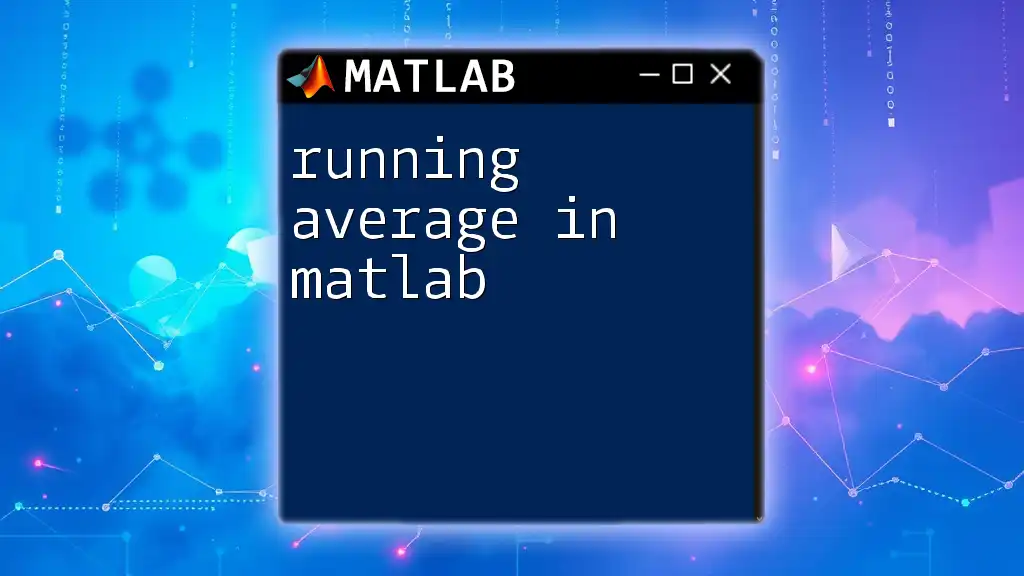
Additional Resources
For further exploration, the official MATLAB documentation offers extensive resources on functions such as `movmean()`, `cumsum()`, and many more that can greatly enhance your understanding and application of moving averages. Additionally, consider seeking out tutorials and courses focusing on time series analysis to expand your knowledge and proficiency in this essential area of data analysis.