MATLAB is a high-level programming language and interactive environment designed for numerical computation, data analysis, and visualization, allowing users to perform complex mathematical calculations efficiently.
Here’s an example of a simple MATLAB command that calculates the sum of an array:
array = [1, 2, 3, 4, 5];
total = sum(array);
disp(total);
Understanding the Basics of MATLAB Language
Structure of MATLAB Language
Variables and Data Types
MATLAB (Matrix Laboratory) is built primarily around arrays and matrices. Understanding how to work with different data types is essential for effective programming in MATLAB. MATLAB supports various data types, including:
- Numeric: The most common type, ideal for mathematical operations.
- Logical: Useful for conditional statements, where values are either true (1) or false (0).
- Character: Used for strings and text data.
To declare and assign variables in MATLAB, follow these examples:
% Example of variable declaration
x = 10; % Numeric variable
y = 'Hello'; % Character variable
Basic Syntax and Commands
Code Structure in MATLAB
MATLAB's syntax is straightforward, but it is essential to adhere to specific rules, like case sensitivity. For example, 'Variable' and 'variable' would be recognized as two separate variables. Understanding how to structure your code properly will enhance both readability and functionality.
Common Commands
In MATLAB, a few commands serve fundamental purposes:
- `disp()`: Displays output on the screen.
- `clc`: Clears the command window.
- `clear`: Removes variables from the workspace.
- `close all`: Closes all open figure windows.
Here’s how these commands work:
clc; % Clear Command Window
clear; % Clear Workspace Variables
close all; % Close All Figures
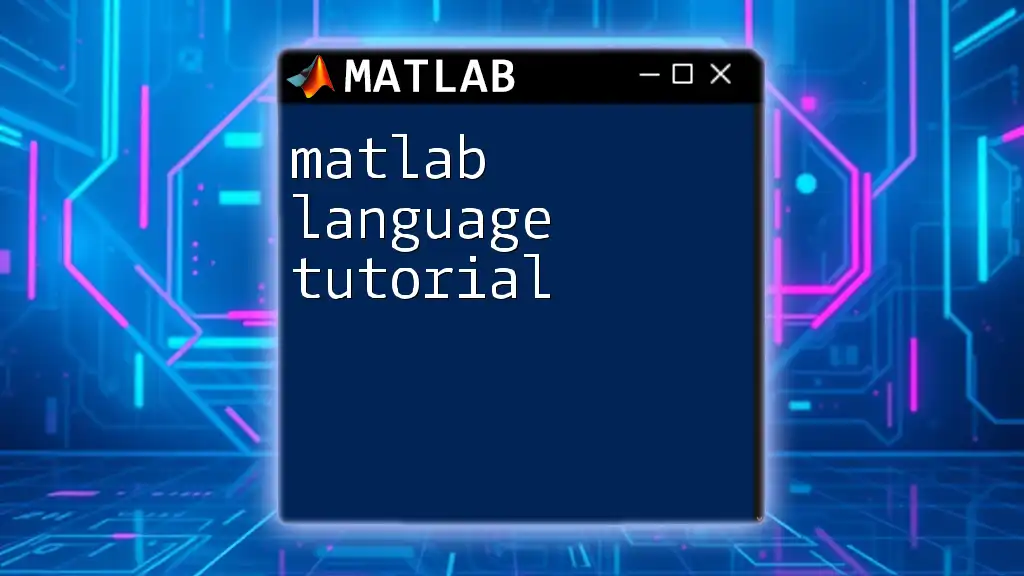
Control Flow in MATLAB
Decision Making
Using `if-else` Statements
Control flow statements such as `if-else` allow you to execute specific code blocks based on certain conditions. Here's the basic structure:
if x > 10
disp('x is greater than 10');
else
disp('x is 10 or less');
end
This code checks the value of `x` and displays a message accordingly.
Loops
For Loops and While Loops
Loops are necessary for repeating tasks. For loops are often used for iterating over a set number of times, while while loops continue to execute as long as a condition is true. Here’s an example of a for loop:
for i = 1:5
disp(i); % Displays numbers from 1 to 5
end
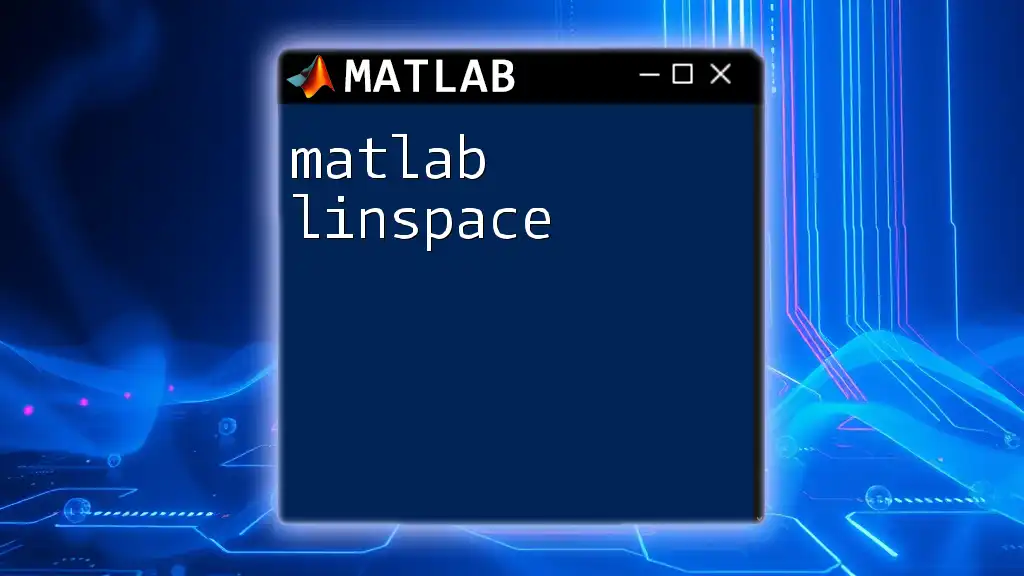
Functions in MATLAB
Creating and Using Functions
Defining Functions
Functions allow you to encapsulate code for reuse. Defining a function in MATLAB involves the `function` keyword followed by the output variable, function name, and input parameters. Here's a sample function that squares an input value:
function output = myFunction(input)
output = input^2; % Squaring the input
end
You can call this function using:
result = myFunction(4); % result will be 16
Calling Functions
When you call a function, you can pass arguments and retrieve outputs directly, which simplifies your code and enhances clarity.
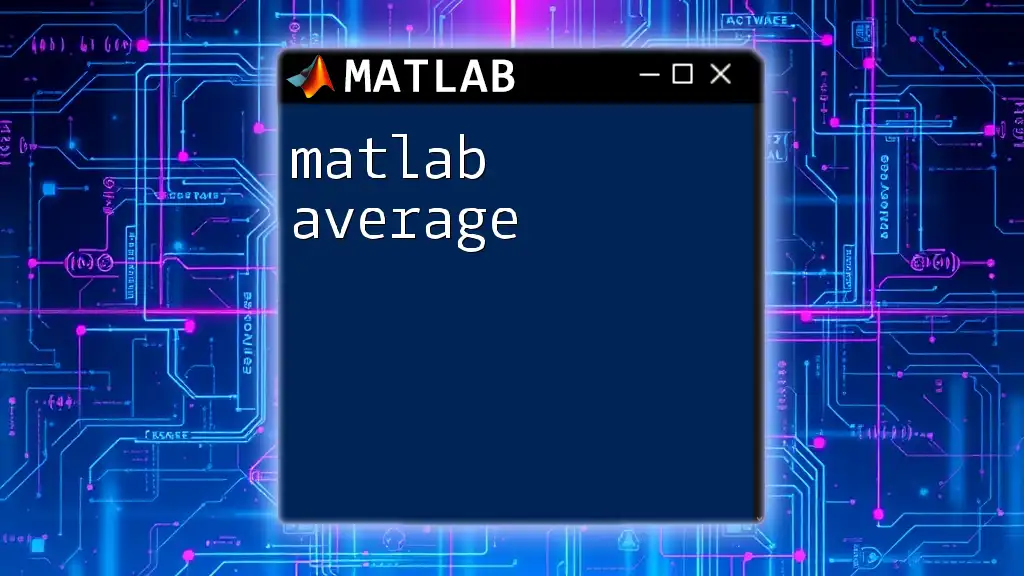
Working with Arrays and Matrices
Matrix Basics
Creating and Manipulating Matrices
Matrices are at the core of MATLAB. Creating a matrix is as simple as using square brackets. Example of a 2x2 matrix:
A = [1, 2; 3, 4]; % 2x2 Matrix
Operations on Arrays
Common Operations
MATLAB makes it simple to perform operations on matrices. Some basic operations include:
- Matrix multiplication using the `*` operator.
- Element-wise operations using `.*`, `./`, and similar operators.
Here’s how to perform these operations:
B = A * A; % Matrix multiplication
C = A + A; % Element-wise addition
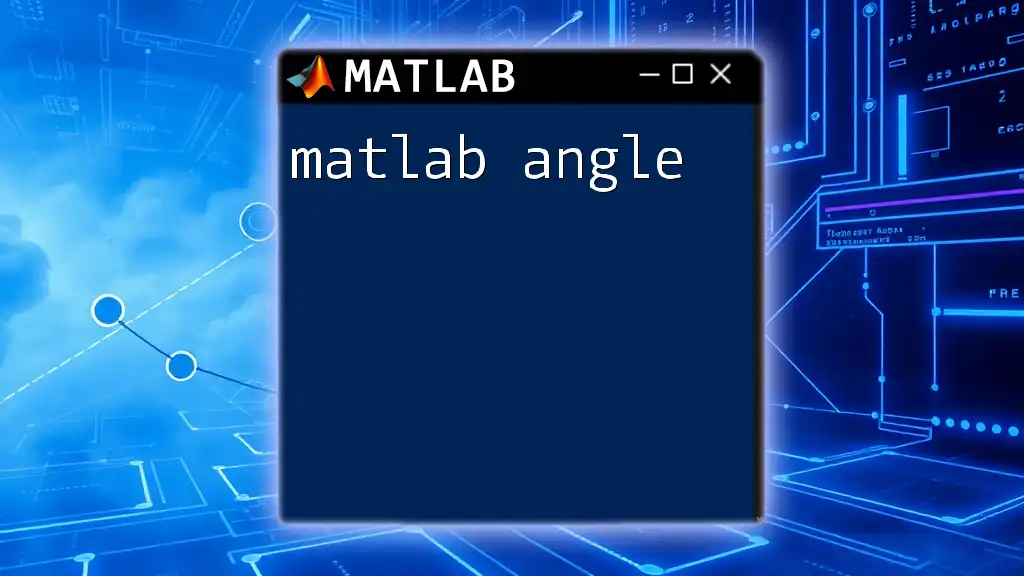
Plotting and Visualization in MATLAB
2D and 3D Plotting
Basic Plotting Commands
Visualization is crucial for data analysis. MATLAB provides built-in functions for plotting data. Below is a simple example of plotting a sine wave:
x = 0:0.1:10; % Range from 0 to 10
y = sin(x);
plot(x, y); % Plotting the sine wave
xlabel('X-axis');
ylabel('Y-axis');
title('Sine Wave');
Customizing Plots
Enhancing Visuals
Enhancing graphical outputs is important for presentations and analysis. You can customize colors, line styles, and labels to suit your needs:
plot(x, y, 'r--'); % Red dashed line
legend('Sine Function');
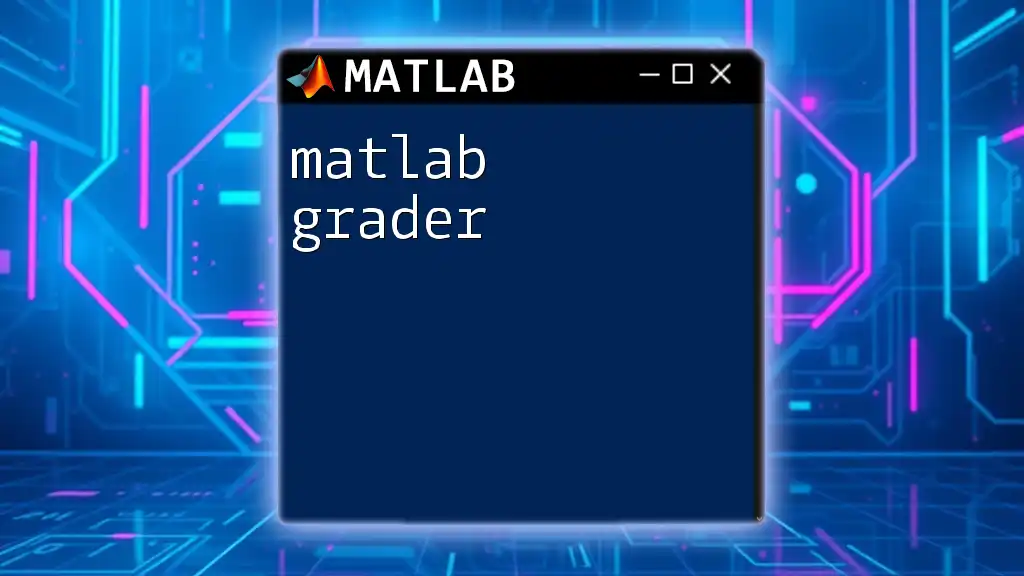
Advanced Topics in MATLAB
Object-Oriented Programming in MATLAB
Creating Classes and Objects
MATLAB supports object-oriented programming, allowing you to define classes that encapsulate data and behavior. You can create properties and methods for reusable code structures.
Working with Toolboxes
Utilizing MATLAB Toolboxes
MATLAB offers various toolboxes tailored for specific applications, such as Image Processing, Statistics, and Machine Learning. Using toolbox functions can expand MATLAB's capabilities significantly. If you're working with images, for instance, you might find the Image Processing Toolbox invaluable.
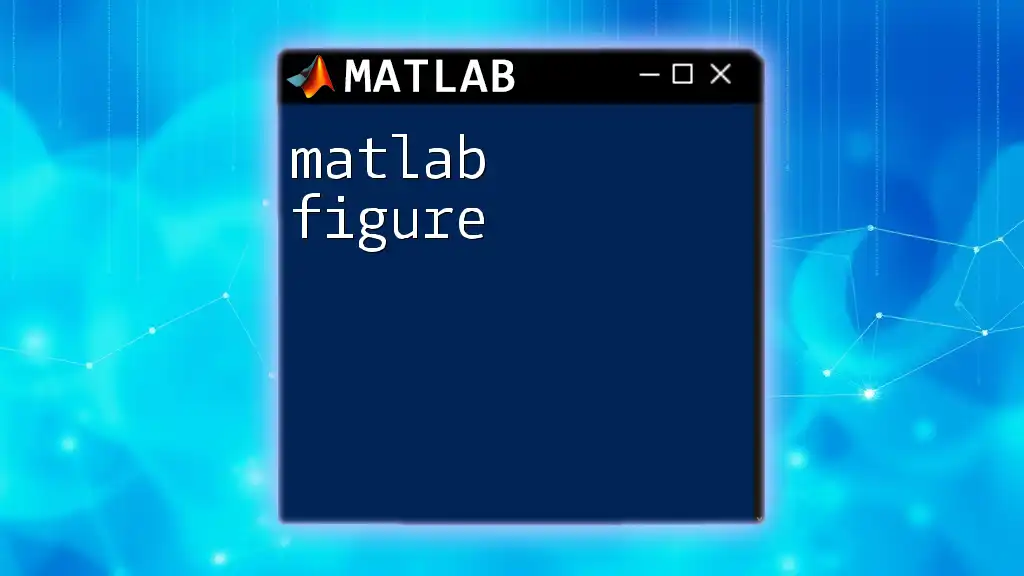
Best Practices for Writing MATLAB Code
Code Optimization Techniques
Writing Efficient Code
Efficient coding practices not only enhance performance but also improve maintainability. Some tips include:
- Avoiding unnecessary loops by leveraging vectorized operations.
- Using built-in functions which are usually optimized for speed.
Debugging and Error Handling
Common Errors and Debugging Techniques
When coding in MATLAB, you will encounter errors. Understanding the error messages is essential for effective debugging. MATLAB offers tools such as breakpoints and the `dbstop` function to help identify issues in your code.
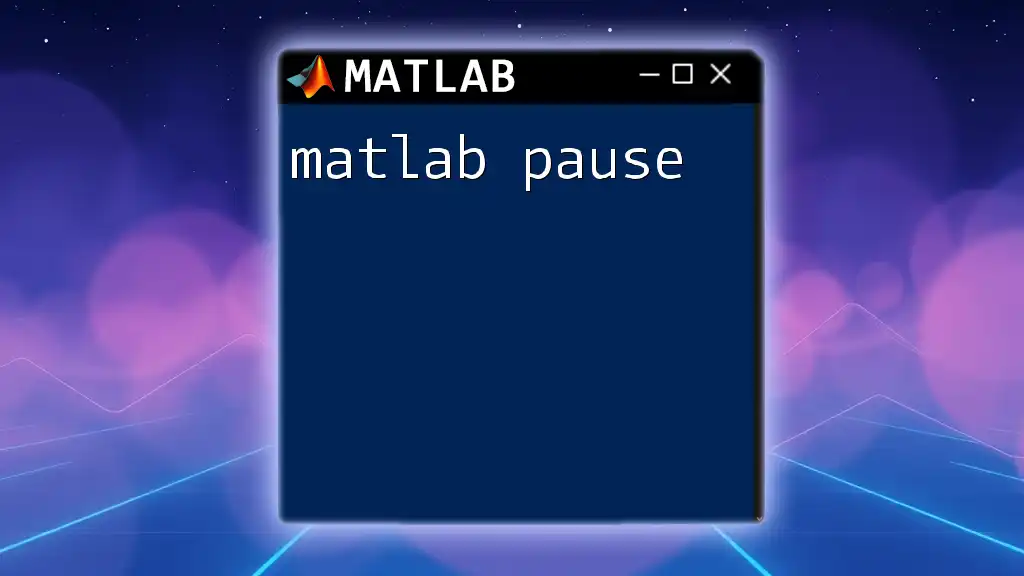
Conclusion
This comprehensive guide provides essential insights into the MATLAB language, from fundamental concepts to more advanced functionalities. Mastering these techniques will significantly aid your MATLAB programming skills, enabling you to tackle a variety of engineering and scientific problems with confidence. Explore further resources for continued learning and mastery of MATLAB to expand your expertise.
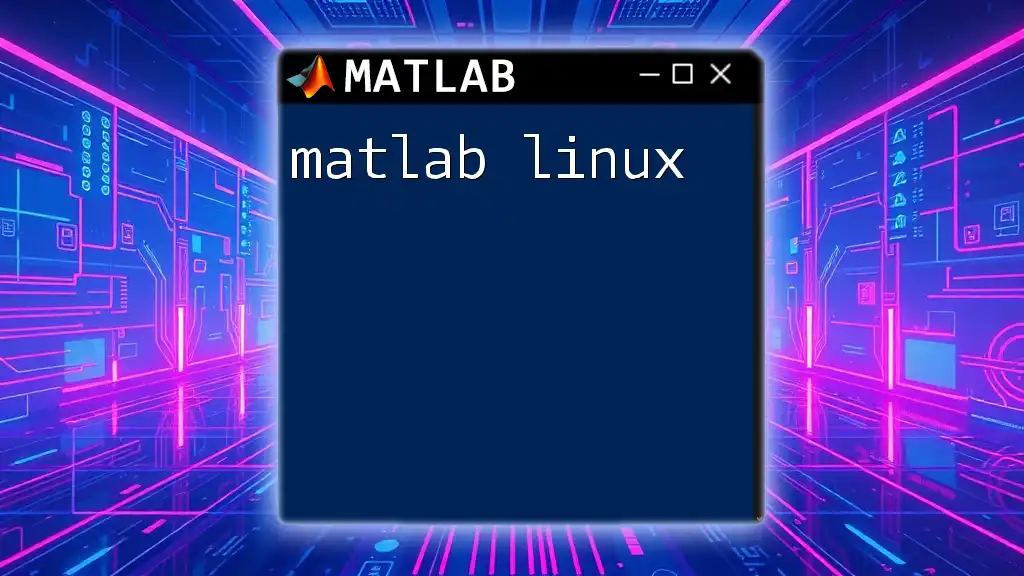
FAQs about MATLAB Language
What is the main purpose of MATLAB?
MATLAB is designed primarily for numerical computing, allowing users to perform complex calculations and data analysis efficiently.
Can I use MATLAB for machine learning?
Yes, MATLAB provides specialized toolboxes for various machine learning applications, making it a powerful tool for data scientists.
Is there a free version of MATLAB available?
While MATLAB itself is a paid software, there are alternatives like GNU Octave that aim to provide similar functionalities and compatibility with MATLAB syntax.