In MATLAB, you can calculate the average of an array using the `mean` function, which computes the arithmetic mean of the elements along a specified dimension.
Here’s a simple code snippet to demonstrate this:
% Calculate the average of the array
data = [2, 4, 6, 8, 10];
averageValue = mean(data);
disp(averageValue);
Understanding Averages in MATLAB
What is an Average?
An average is a statistic that summarizes a set of numbers. It represents a central value of a data set and can provide insights into patterns and trends. The most commonly used types of averages include the mean, median, and mode.
- Mean: The sum of all values divided by the number of values.
- Median: The middle value when the numbers are sorted in order.
- Mode: The value that appears most frequently in a data set.
Why Use MATLAB for Calculating Averages?
MATLAB offers powerful tools for performing calculations, making it an ideal environment for statistical analysis. Here are a few reasons why using MATLAB for computing averages is beneficial:
- Efficiency: MATLAB is optimized for handling large datasets, enabling quick calculations.
- Built-in Functions: MATLAB provides a variety of built-in functions that simplify the process of computing averages, reducing the need for custom code.
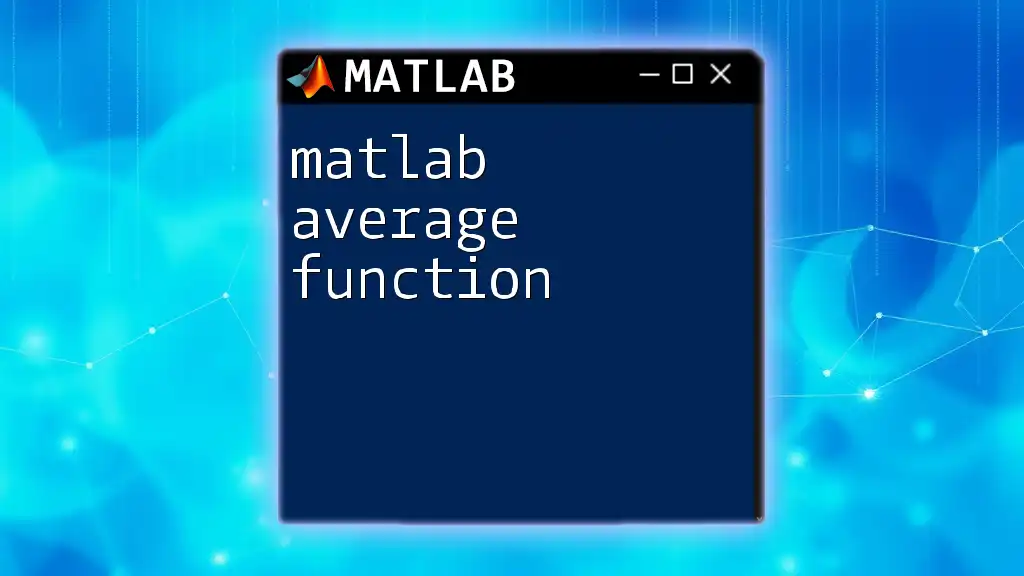
MATLAB Functions for Computing Averages
Mean Calculation
Using the `mean()` Function
The `mean()` function in MATLAB calculates the average of the elements in an array or matrix.
Syntax:
M = mean(X)
Example:
data = [1, 2, 3, 4, 5];
average = mean(data);
disp(average); % Output: 3
In this example, the `mean()` function takes the array `data` and computes the average, which is displayed as 3. This function is particularly useful for quickly summarizing the central tendency of data.
Median Calculation
Using the `median()` Function
The `median()` function finds the middle value of a dataset, which is especially useful when data contains outliers.
Syntax:
M = median(X)
Example:
data = [1, 3, 3, 6, 7, 8, 9];
medianValue = median(data);
disp(medianValue); % Output: 6
In this example, the `median()` function returns 6, which is the middle number in the sorted list. This function is crucial for understanding the distribution of data, especially in cases where mean might be skewed by extreme values.
Mode Calculation
Using the `mode()` Function
The `mode()` function identifies the most frequently occurring value in a dataset.
Syntax:
M = mode(X)
Example:
data = [1, 2, 2, 3, 4];
modeValue = mode(data);
disp(modeValue); % Output: 2
As shown, the mode of the array `data` is 2, the value that appears most often. Knowing the mode can be particularly beneficial when dealing with categorical data or situations where the most common value needs to be highlighted.
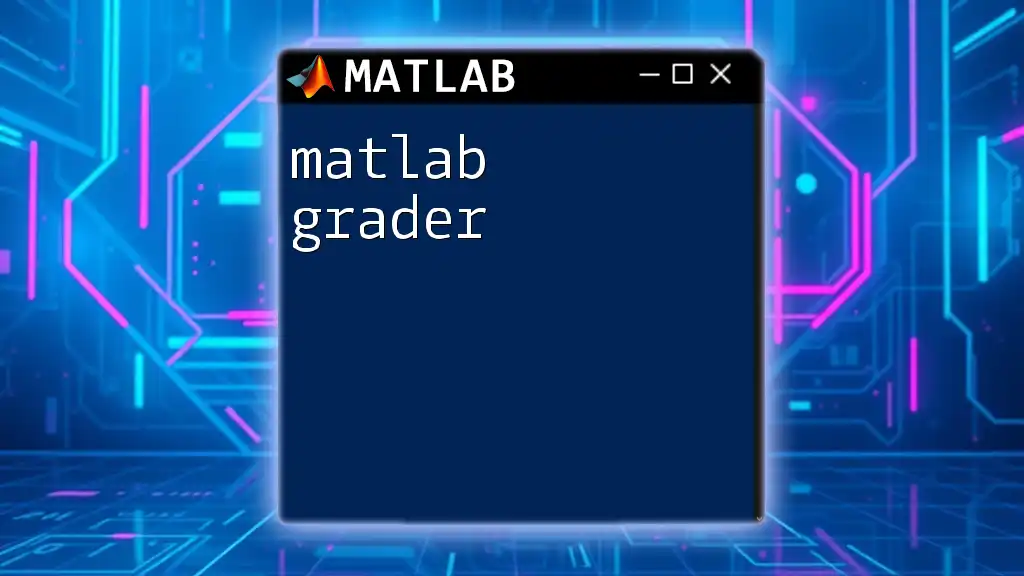
Advanced Average Calculations
Weighted Average
Using Manual Calculation
A weighted average is useful when different data points contribute differently to the final average. The formula for calculating a weighted average is:
\[ \text{Weighted Average} = \frac{\sum (w_i \cdot x_i)}{\sum w_i} \]
Example:
values = [1, 2, 3];
weights = [0.2, 0.5, 0.3];
weighted_avg = sum(values .* weights) / sum(weights);
disp(weighted_avg); % Output: 2.4
In this example, the weighted average is calculated by multiplying each value by its corresponding weight and dividing by the sum of the weights. This approach is particularly helpful in scenarios like calculating grades where different assignments have different importance.
Moving Average
Using `movmean()` Function
The moving average smooths out fluctuations and reveals trends in data over time, often used in time series analysis. The `movmean()` function computes the moving average over a specified window.
Syntax:
M = movmean(X,k)
Example:
data = [1, 2, 3, 4, 5, 6, 7];
moving_avg = movmean(data, 3);
disp(moving_avg); % Output: [2 3 4 5 6 7]
In this example, the moving average with a window of 3 size smooths the data. This function is invaluable in statistical analysis and forecasting as it helps to identify trends over time while filtering out short-term fluctuations.
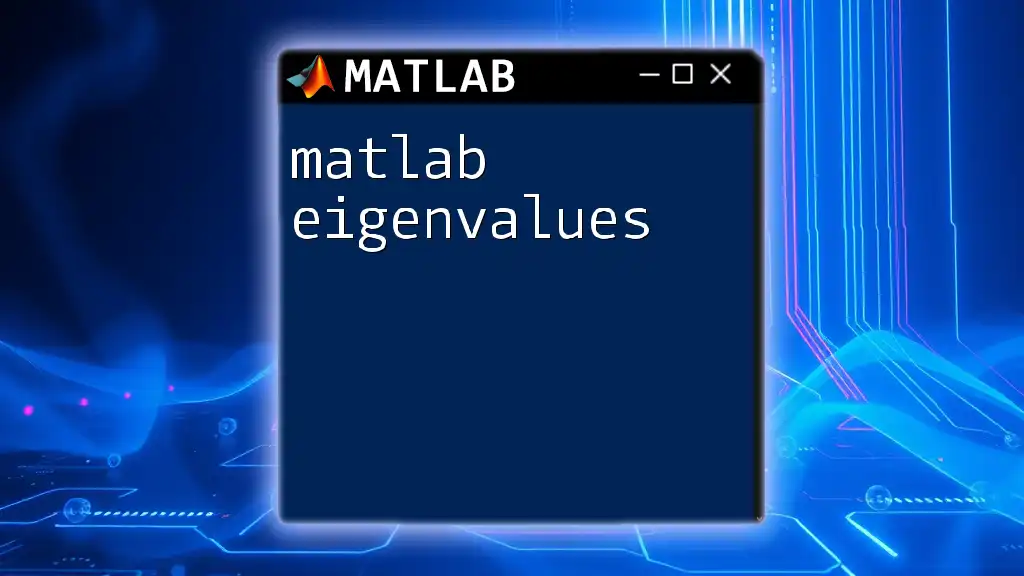
Practical Applications of Averages in MATLAB
Data Analysis
Averages are fundamental in analyzing datasets, particularly in statistics. By summarizing data sets using mean, median, or mode, you can gain insights into the overall distribution and central tendency of data. For instance, calculating the average score of students in a class can inform teaching strategies.
Signal Processing
In signal processing, averages are crucial for filtering signals and removing noise. For example, applying a moving average to a noisy signal helps to produce a cleaner output. This technique is widely used in audio processing, communications, and electronic engineering.
Financial Analytics
Averages play a significant role in financial analysis, particularly in tracking stock market trends. By calculating the average price of a stock over a specific period, traders can identify patterns and make informed trading decisions. Understanding short-term and long-term moving averages helps in forecasting price movements.
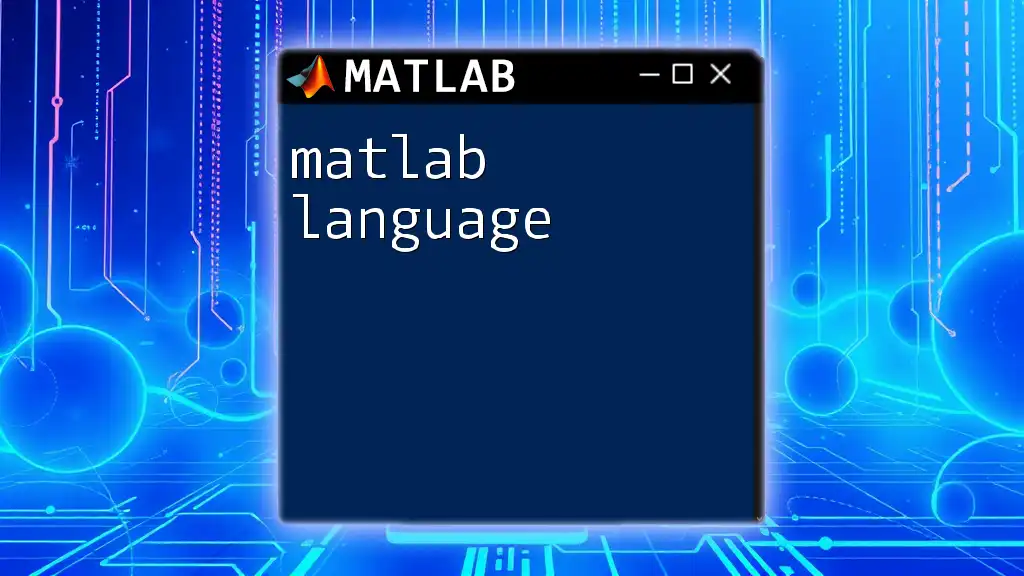
Troubleshooting Common Issues
Handling NaN Values
When calculating averages, encountering NaN (Not a Number) values can lead to misleading results. MATLAB provides a solution with the `nanmean()` function, which calculates the mean while ignoring NaN values.
Example:
data = [1, NaN, 3, 4];
avgWithoutNaN = nanmean(data);
disp(avgWithoutNaN); % Output: 2.6667
This example illustrates how `nanmean()` allows for robust computations without the disruption of missing values, ensuring accurate results in data analysis.
Performance Considerations
When dealing with large datasets, the performance of averaging functions can vary. Optimizing code and choosing the right function can significantly enhance performance. For example, using built-in functions like `mean()` is faster than hand-coding a loop for summation and division.
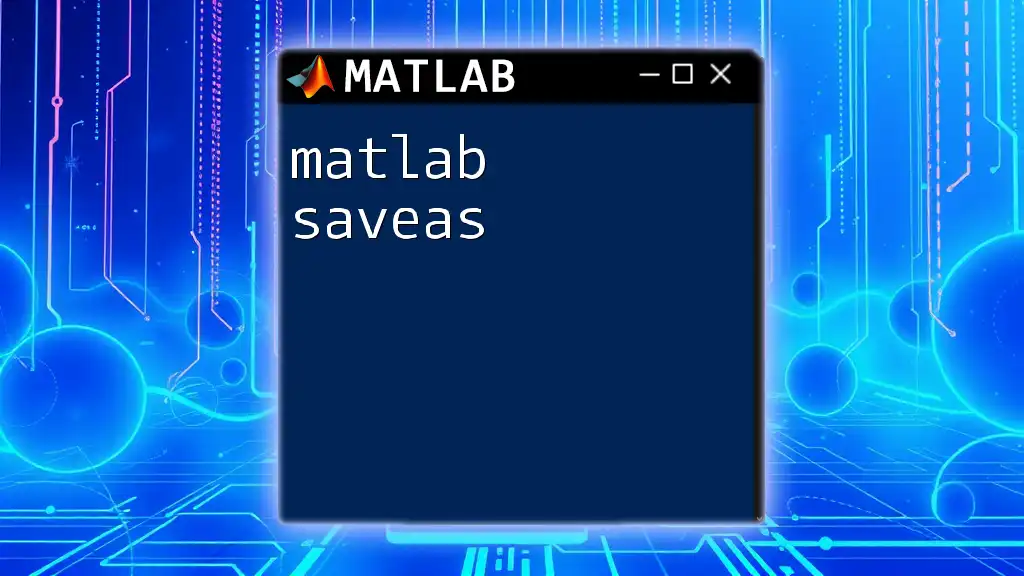
Conclusion
Mastering the computation of averages using MATLAB not only simplifies data analysis but also equips you with essential tools for effective statistical examination. By leveraging functions like `mean()`, `median()`, `mode()`, and their advanced counterparts, you can uncover valuable insights from your data. Begin practicing these techniques today to enhance your proficiency in MATLAB and data analysis.
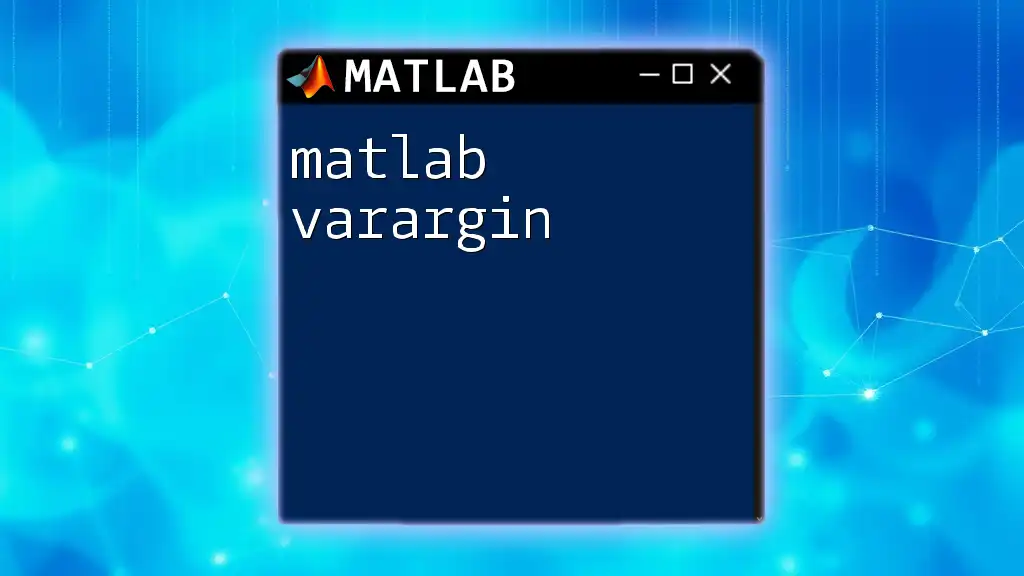
Additional Resources
For further reading, refer to the official MATLAB documentation detailing the usage of statistical functions. Engaging with practical problems and datasets is encouraged to solidify your understanding of these concepts.