Matlab numerical integration involves using algorithms to approximate the integral of a function, enabling users to find the area under curves and solve problems analytically difficult to address.
Here’s a simple code snippet for performing numerical integration using the `integral` function:
f = @(x) x.^2; % Define the function to integrate
result = integral(f, 0, 1); % Compute the integral from 0 to 1
disp(result); % Display the result
Understanding Numerical Integration
What is Numerical Integration?
Numerical integration refers to the techniques of calculating the value of an integral using numerical approximations rather than analytical methods. It is particularly useful when dealing with functions that cannot be integrated analytically or when dealing with empirical data.
While analytical integration yields exact results, numerical integration provides estimates, which are often sufficient for practical applications. For example, numerical integration is commonly used in fields such as physics for calculating areas under curves, in engineering to evaluate load distributions, and in finance to determine the net present value of cash flows.
Why Use MATLAB for Numerical Integration?
Efficiency is a key advantage when using MATLAB for numerical integration. MATLAB offers built-in functions that can perform integration tasks quickly and accurately, thus saving time and reducing the complexity of coding from scratch. The ease of manipulating data within MATLAB’s environment allows users to focus on solving problems rather than dealing with intricate mechanics of numerical methods.
Flexibility is another crucial benefit. MATLAB's capability to handle various types of mathematical functions and problems ensures that users can adapt their approach to different datasets or integration requirements without excessive programming effort.
Finally, visualization in MATLAB aids comprehension and presentation of integration results. The ability to plot functions and their areas of integration enhances understanding and allows for powerful data interpretation.
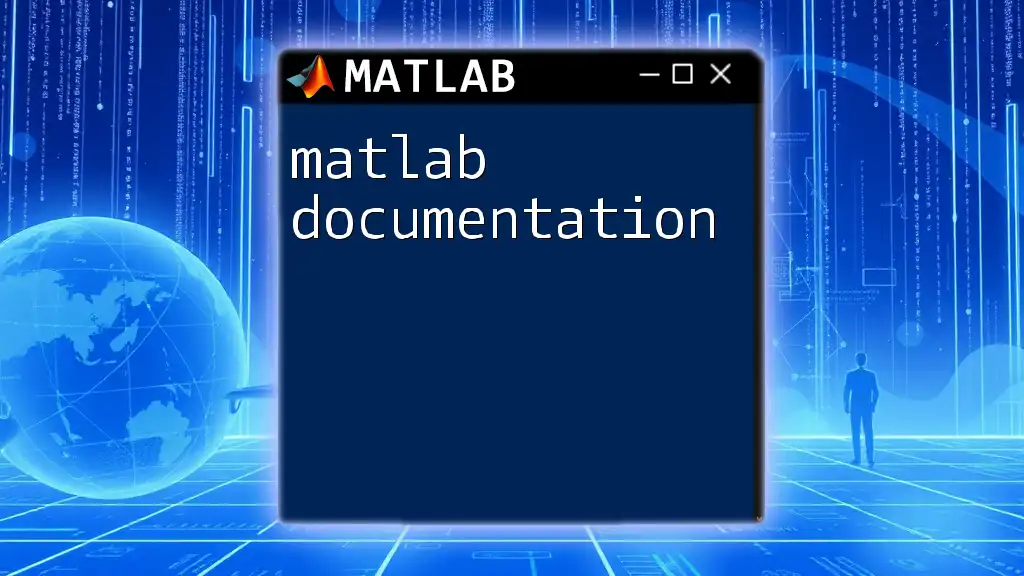
Basic Concepts of Numerical Integration
Types of Integration Techniques
Understanding the types of integration techniques is essential for applying the right approach in MATLAB.
-
Definite vs. Indefinite Integration: Definite integration involves calculating the area under a curve between two specified limits, while indefinite integration seeks to find the general form of the integral without defined boundaries.
-
Approximation Methods: There are several key techniques used in numerical integration:
- Trapezoidal Rule: Approximates the area under a curve by dividing the region into trapezoids.
- Simpson’s Rule: Provides a more accurate approximation by fitting parabolas to segments of the curve.
Understanding Integration Methods in MATLAB
Built-in Functions for Numerical Integration
MATLAB provides a rich set of built-in functions tailored for various integration needs:
- `integral`: A flexible function that can handle inputs ranging from simple functions to more complex ones, including those that exhibit singularities.
Example:
% Example of using integral function
f = @(x) x.^2; % Function to integrate
area = integral(f, 0, 4); % Integrating from 0 to 4
fprintf('Area under the curve: %.4f\n', area);
- `trapz`: Accomplishes numerical integration using the trapezoidal rule; particularly useful for discrete data points.
Example:
% Example of Trapezoidal Rule
x = 0:0.1:10; % define the range
y = sin(x); % function to integrate
area = trapz(x, y); % numerical integration
fprintf('Area under the curve: %.4f\n', area);
- `quad` / `quadgk`: Adaptive quadrature functions that provide high precision by dynamically adjusting step sizes based on the function's behavior.
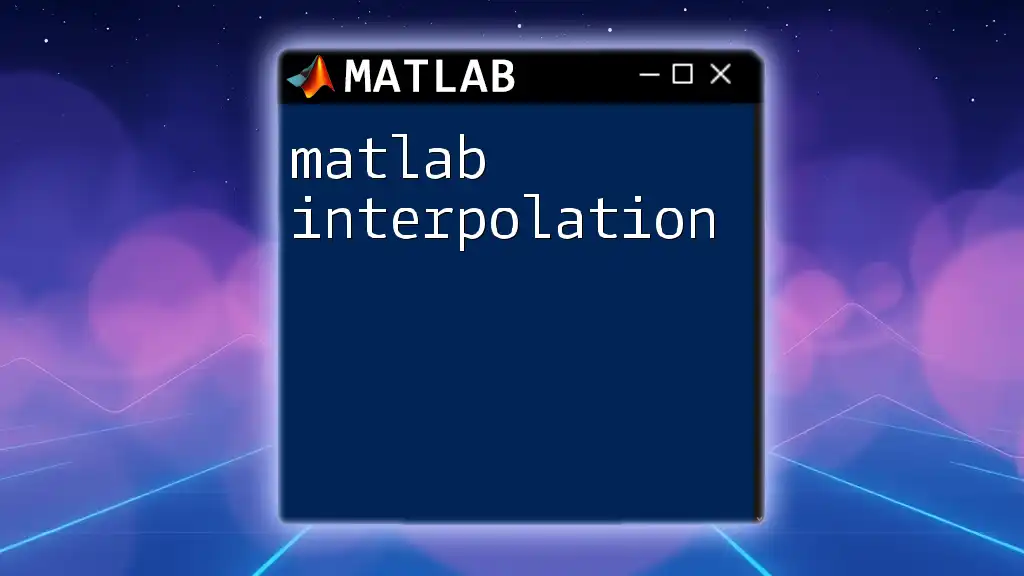
Performing Numerical Integration with MATLAB
Getting Started with MATLAB
Before diving into numerical integration, ensure that you have MATLAB installed along with relevant toolboxes. Familiarizing yourself with the MATLAB environment, including the command window and editor, will streamline your numerical integration tasks.
Step-by-Step Guide to Numerical Integration
Using the Trapezoidal Rule
To effectively perform numerical integration using the trapezoidal rule, one needs to understand the underlying principle that approximates the area under the curve using trapezoid shapes.
Example code for the trapezoidal rule:
% Example of Trapezoidal Rule
x = 0:0.1:10;
y = sin(x);
area = trapz(x, y);
fprintf('Area under the curve: %.4f\n', area);
Using Simpson’s Rule
Simpson’s Rule is another numerical integration method that often provides greater accuracy than the trapezoidal rule, particularly for functions that can be well-approximated by parabolas over small intervals.
Example code for Simpson’s Rule:
% Example of Simpson's Rule
x = 0:0.1:10;
y = sin(x);
area = simpson(y, x); % Assuming 'simpson' is a defined function.
fprintf('Area under the curve using Simpson’s Rule: %.4f\n', area);
Advanced Numerical Integration Techniques
Adaptive Quadrature
For more complex integrations, MATLAB's adaptive functions, such as `quadgk`, automatically adjust to enhance accuracy.
Example code using adaptive quadrature:
% Example of Adaptive Quadrature
f = @(x) exp(-x.^2); % define function
area = quadgk(f, 0, Inf); % integrate from 0 to infinity
fprintf('Area using Adaptive Quadrature: %.4f\n', area);
Multi-dimensional Integration
When dealing with integrals involving more than one variable, `integral2` is MATLAB's go-to function for double integrals, allowing for the integration of functions over rectangular regions.
Example code for double integration:
% Example of Multi-dimensional Integration
f = @(x,y) x.*y; % simple two-variable function
area = integral2(f, 0, 1, 0, 1); % integrating over the unit square
fprintf('Double integral result: %.4f\n', area);
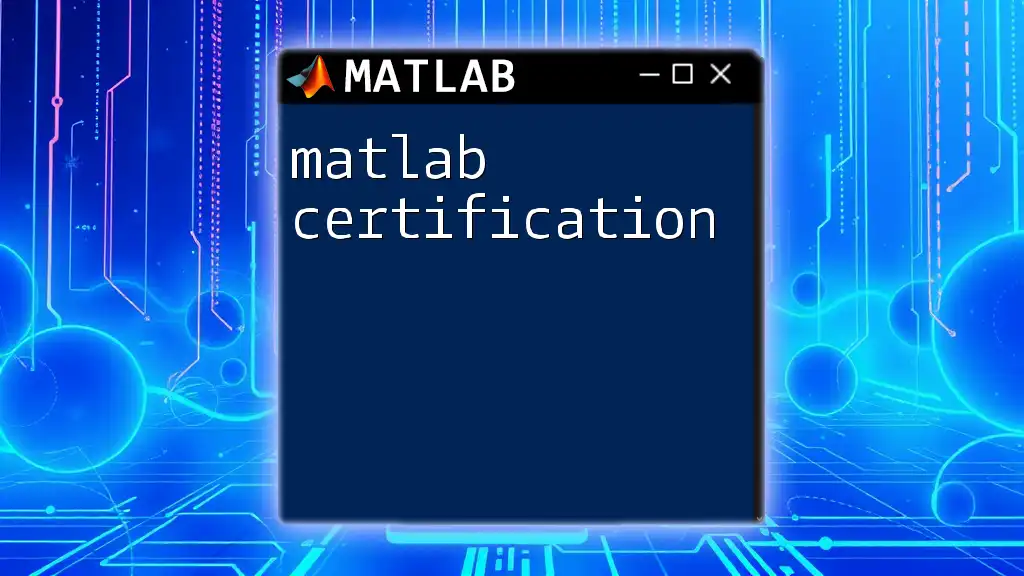
Visualization of Integration Results
Plotting the Function and the Area Under the Curve
Visualization is crucial in communicating the outcomes of numerical integration. MATLAB provides powerful plotting functions that can help you clearly represent results.
Example code for visualizing area under the curve:
% Example of visualizing area under the curve
x = 0:0.1:10;
y = sin(x);
fill(x, [y zeros(1,length(y))], 'g', 'FaceAlpha', 0.5); % fill under curve
plot(x, y, 'b', 'LineWidth', 2);
title('Area under the Sine Curve');
xlabel('x'); ylabel('sin(x)');
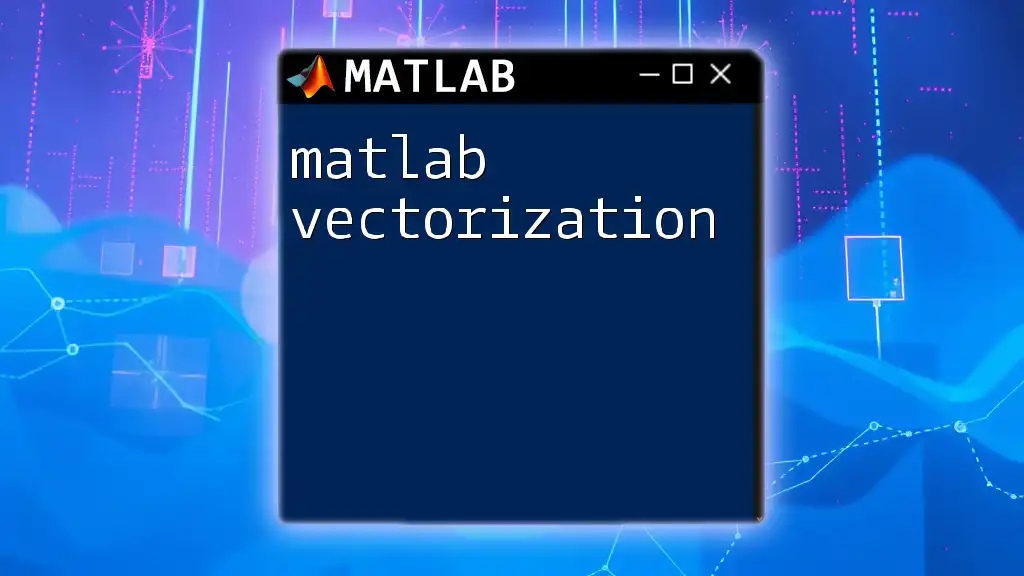
Troubleshooting Common Issues in Numerical Integration
Common Errors and Solutions
While MATLAB simplifies many aspects of numerical integration, users may still encounter challenges. Common errors include numerical instability, which can arise due to poorly chosen limits or highly oscillatory functions. Identifying these problems early and adjusting integration limits or method parameters can alleviate such issues.
Best Practices for Accurate Results
To achieve accurate numerical integrations, consider adjusting the precision settings in MATLAB. This includes refining tolerances in built-in functions and ensuring that the integration segments are adequately sized to minimize error.
Examine the function to identify areas that may require closer approximations, particularly in regions near singularities or discontinuities.
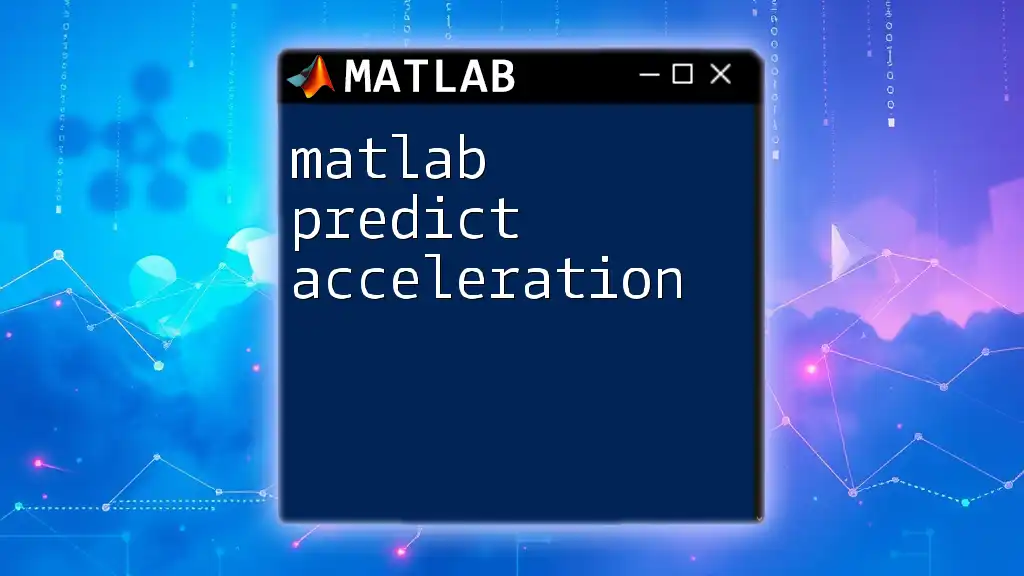
Conclusion
Mastering numerical integration in MATLAB is a valuable skill that empowers users to tackle complex mathematical problems effectively. By understanding the built-in functions, applying the right techniques, and visualizing results, individuals leap towards enhanced computational skills and clearer data interpretation.
To gain a deeper understanding of MATLAB numerical integration, practice with various functions and scenarios, gradually expanding your proficiency. For further guidance, consider exploring additional tutorials and resources to reinforce your learning and application of numerical integration techniques.
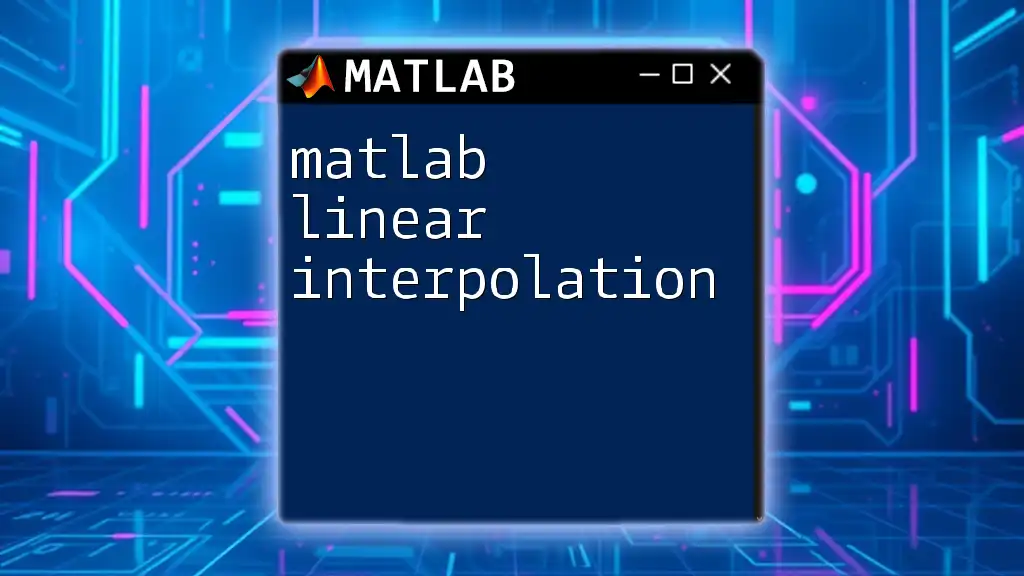
Additional Resources
To enhance your capabilities, visit the official MATLAB documentation on numerical integration functions and consider taking online courses that focus on advanced applications of MATLAB in areas of numerical analysis and engineering.