In MATLAB, you can predict acceleration using a simple linear regression model based on time and velocity data to estimate how an object's acceleration changes over time. Here's a code snippet that demonstrates how to perform this prediction:
% Sample data for time (t) and velocity (v)
t = [0, 1, 2, 3, 4, 5]; % time in seconds
v = [0, 2, 5, 9, 14, 20]; % velocity in meters per second
% Calculate acceleration (a) as the derivative of velocity with respect to time
a = gradient(v, t);
% Display the predicted acceleration
disp('Predicted Acceleration:');
disp(a);
Understanding Acceleration
What is Acceleration?
Acceleration is a vector quantity that describes the rate of change of velocity of an object with respect to time. It reflects how quickly an object speeds up, slows down, or changes direction. In a more technical sense, acceleration can be defined through the following key aspects:
- Linear Acceleration refers to the rate of change of velocity in a straight line. It is measured in meters per second squared (m/s²).
- Angular Acceleration describes how quickly an object rotates about an axis, typically measured in radians per second squared (rad/s²).
Fundamentally, acceleration is governed by Newton's Second Law, which states that the force acting on an object is equal to the mass of that object multiplied by its acceleration (F = ma).
Why Predict Acceleration?
Predicting acceleration is crucial across various fields, from automotive engineering to aerospace and robotics. Accurately forecasting how an object will move allows engineers and analysts to design safer and more efficient systems. Consider the following applications:
- In automotive design, understanding acceleration helps with vehicle performance optimization and safety features.
- In aerospace, predicting the acceleration of an aircraft during takeoff and landing enhances operational safety.
- In robotics, predicting acceleration is vital for motion planning and control, ensuring robots operate efficiently and safely.
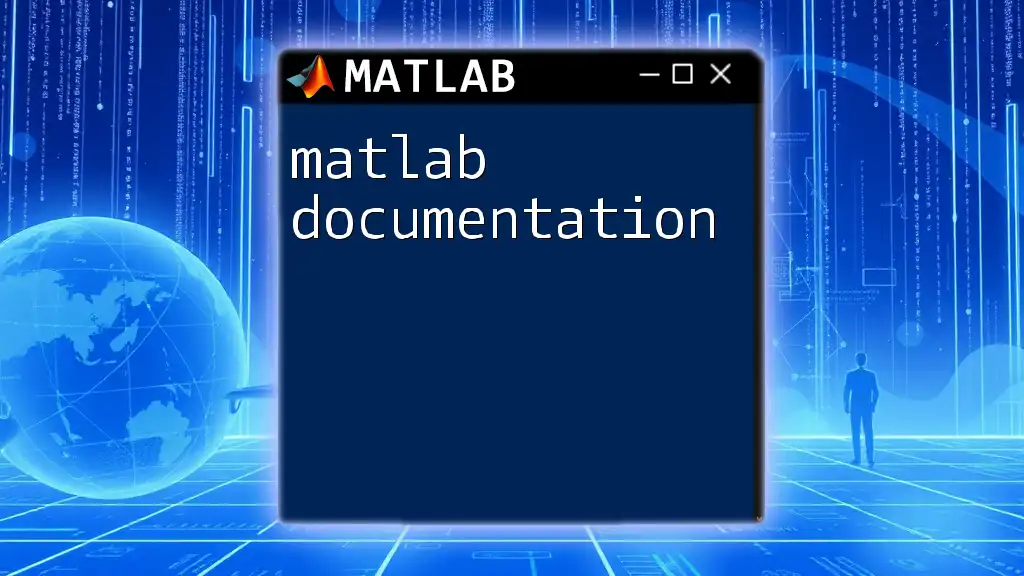
Basics of MATLAB for Predicting Acceleration
Introduction to MATLAB Commands
MATLAB (Matrix Laboratory) is a powerful computing platform utilized extensively for data analysis, mathematical modeling, and simulations. Its user-friendly commands and built-in functions make it a preferred choice among engineers and researchers.
Key MATLAB commands relevant to data analysis, particularly for predicting acceleration, include:
- `plot()`: For visualizing data.
- `diff()`: To compute differences between array elements, essential for calculating derivatives such as velocity and acceleration.
- `polyfit()`: For performing polynomial regression, helpful in forecasting future values based on trends in data.
Setting Up Your Environment
Before predicting acceleration in MATLAB, ensure you have the software installed and set up on your computer. Once the installation is complete, create a new script by navigating to the Home tab and selecting "New Script." This environment will be your workspace for coding and analyzing data.
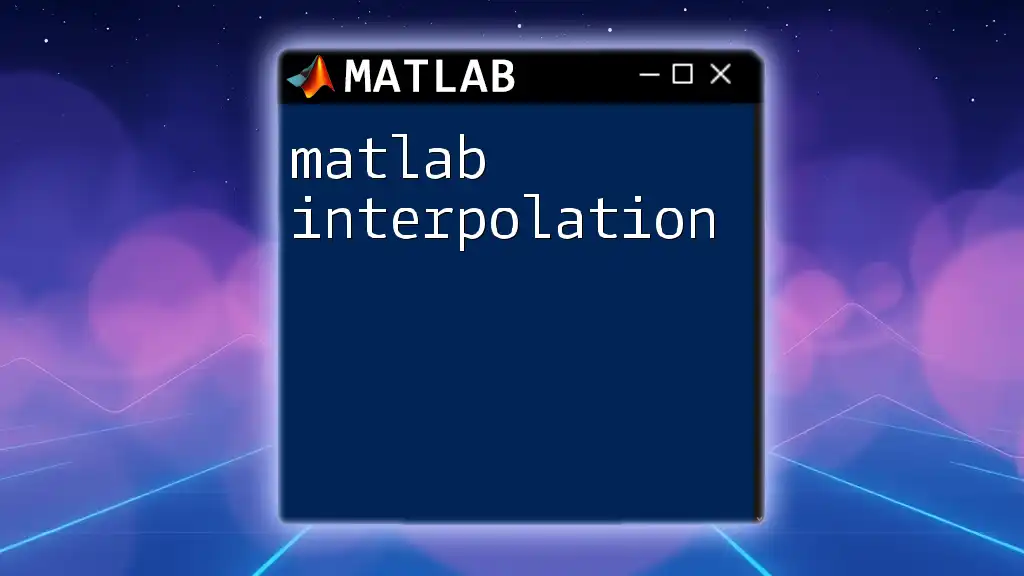
Data Collection for Acceleration Predictions
Importance of Data
Predicting acceleration relies heavily on quality data. The accuracy of your predictions is directly linked to the data you collect. Essential data types for calculating acceleration can include:
- Position: The location of an object over time.
- Time: Accurate timestamps accompanying each position measurement.
- Velocity: Derived from position data, Integer approximations can introduce errors in acceleration predictions.
Simulating Data
Simulated data can be an effective way to practice acceleration predictions when actual data is unavailable. For instance, you can create a simple dataset representing position over time, even simulating some noise to reflect real-world uncertainties. The following MATLAB code snippet demonstrates how to generate such simulated position data:
time = 0:0.1:10; % time vector from 0 to 10 seconds in increments of 0.1 s
position = 5 * time + 0.5 * randn(size(time)); % simulated position with random noise
In this code, `randn(size(time))` introduces randomness, mimicking the unpredictable nature of real-world motion.
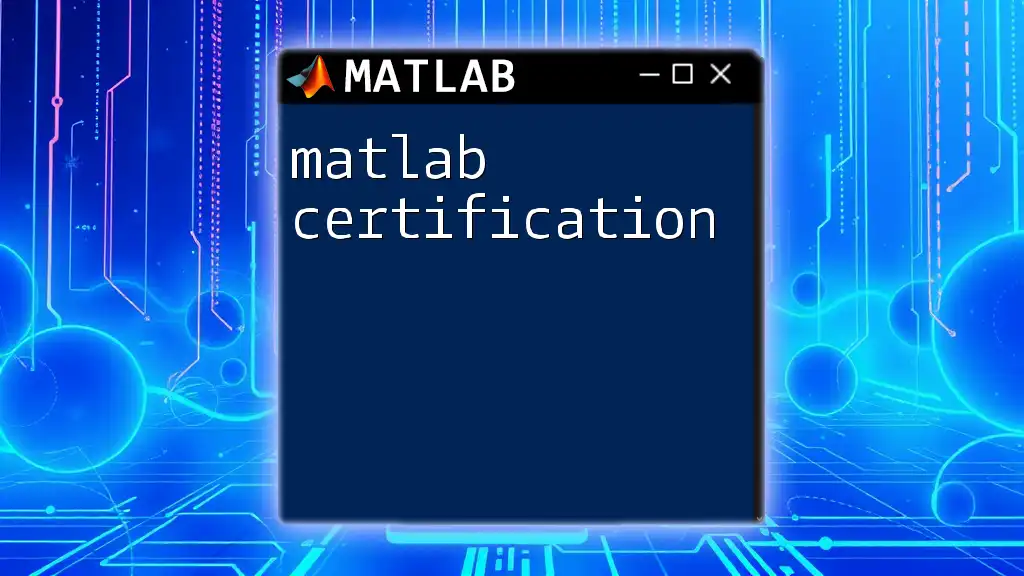
Calculating Acceleration in MATLAB
Basic Formula for Acceleration
The basic formula to calculate acceleration is given by:
\[ a = \frac{dv}{dt} \]
where:
- \( a \) is acceleration,
- \( dv \) is the change in velocity,
- \( dt \) is the change in time.
Deriving Velocity from Position
To find acceleration, we first need to calculate velocity from the position data. The velocity can be determined using the `diff()` function, which computes the difference between successive position measurements, divided by the time intervals. Here’s how you can compute velocity:
velocity = diff(position) ./ diff(time);
% Ensure to adjust the time vector accordingly
time_velocity = time(1:end-1);
Calculating Acceleration
Once you have velocity, you can derive acceleration similarly with respect to time:
acceleration = diff(velocity) ./ diff(time_velocity);
% Adjust time vector for acceleration calculation
time_acceleration = time_velocity(1:end-1);
Example Visualization
After calculating velocity and acceleration, it can be immensely helpful to visualize these values. You can create separate plots to examine how position, velocity, and acceleration change over time.
figure;
subplot(3,1,1);
plot(time, position); title('Position over Time'); xlabel('Time (s)'); ylabel('Position (m)');
subplot(3,1,2);
plot(time_velocity, velocity); title('Velocity over Time'); xlabel('Time (s)'); ylabel('Velocity (m/s)');
subplot(3,1,3);
plot(time_acceleration, acceleration); title('Acceleration over Time'); xlabel('Time (s)'); ylabel('Acceleration (m/s²)');
In each plot, it's essential to discern key trends and understand how position influences velocity, which in turn affects acceleration.
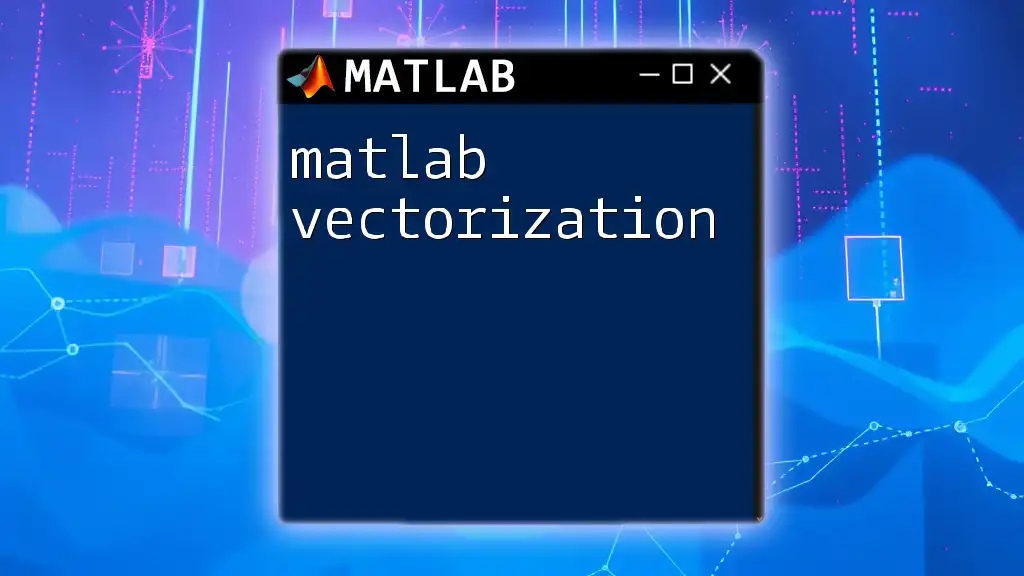
Forecasting Future Acceleration
Using Linear Regression
A significant advancement in predicting acceleration is employing linear regression, which allows you to model acceleration trends from historical data. In MATLAB, you can utilize the `polyfit()` function to perform this regression.
The linear regression can be computed as follows:
p = polyfit(time_acceleration, acceleration, 1); % Performs a linear fit
acceleration_fit = polyval(p, time_acceleration); % Predicts acceleration values
Visualizing Predicted Values
To validate your prediction model, visualize both the actual and predicted values of acceleration. The following code will help you plot these on the same graph:
figure;
plot(time_acceleration, acceleration, 'ro'); % actual values in red circles
hold on;
plot(time_acceleration, acceleration_fit, 'b-'); % predicted values as a blue line
title('Actual vs Predicted Acceleration');
xlabel('Time (s)'); ylabel('Acceleration (m/s²)');
legend('Actual', 'Predicted');
Moving Beyond Linear Models
While linear regression provides a starting point, real-world data can often be non-linear. Consider exploring polynomial regression or more complex predictive models to capture the underlying patterns in your data more effectively. The MATLAB documentation contains extensive resources for diving deeper into these methods.
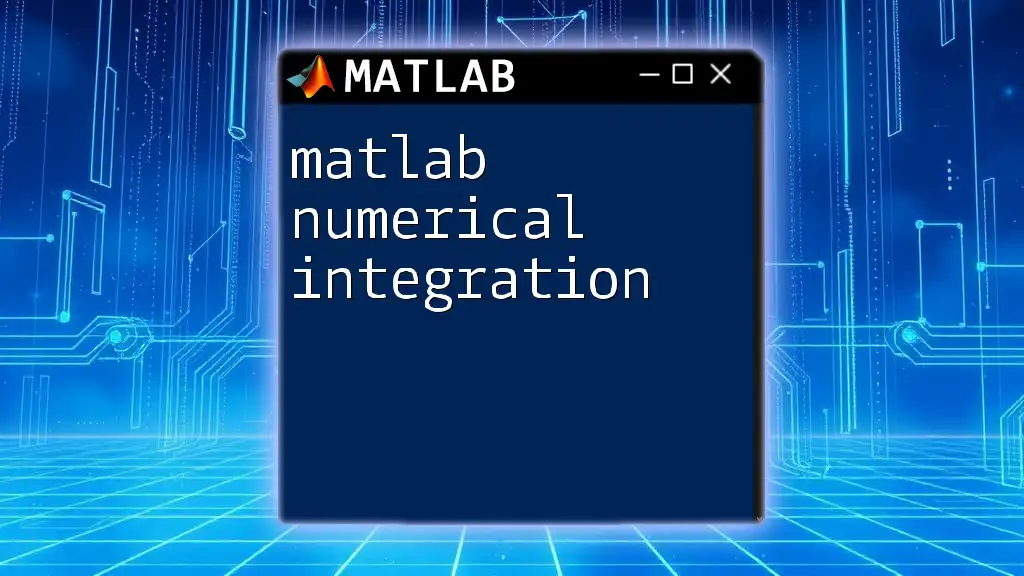
Conclusion
In the journey of learning how to MATLAB predict acceleration, it is essential to recognize the role of quality data, the significance of accurate calculations, and effective visualizations. Through the use of MATLAB’s powerful commands and functions, you can gain deep insights into dynamic motion and enhance your predictive capabilities.
As you develop your skills in predicting acceleration, remember: practice makes perfect. The more you engage with the data and refine your methods, the better equipped you will be to deliver precise predictions across a variety of applications.
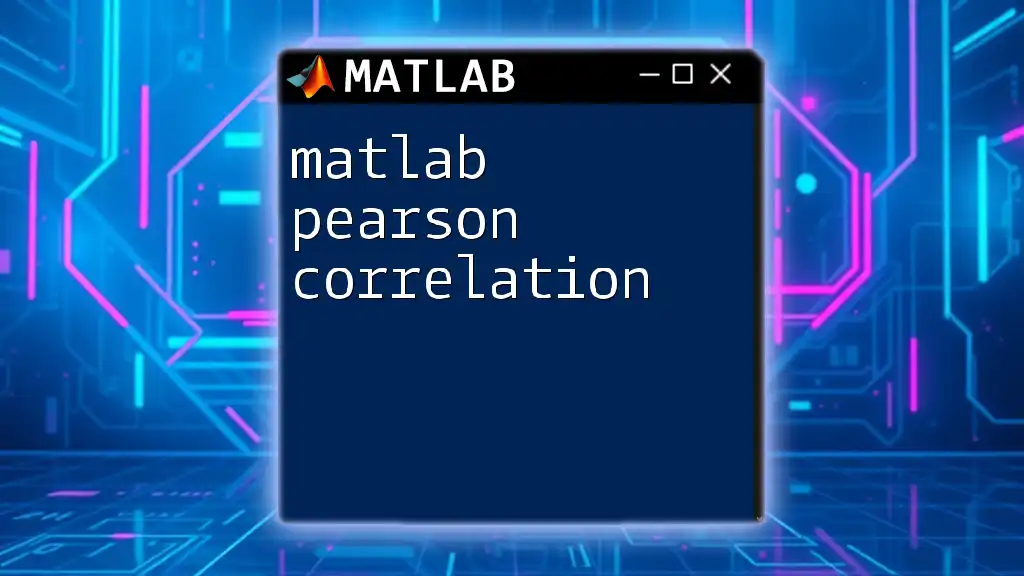
Additional Resources
To enhance your understanding and proficiency, consider diving into official MATLAB documentation, which offers detailed guidance on functions and operations that can aid your learning.
Stay informed by signing up for our newsletters or exploring our courses designed to help you master MATLAB and its applications in fields like acceleration prediction. Feel free to reach out with any questions or comments—you are always welcome in our community!
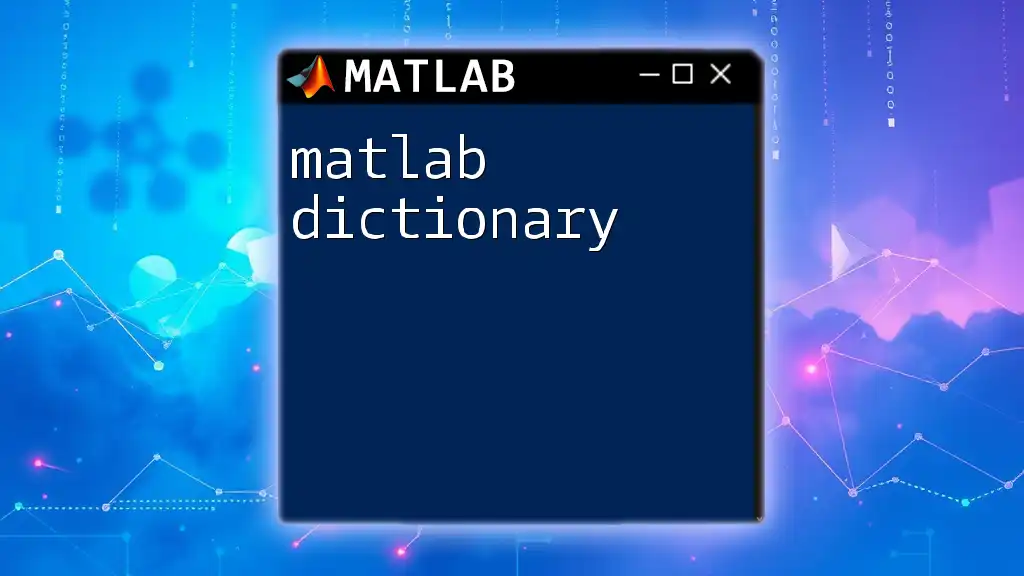
Call to Action
If you found this article valuable, subscribe to our blog for more insights and tutorials. We encourage you to leave comments or inquiries for further help and guidance on your MATLAB journey.