Linear interpolation in MATLAB is a method used to estimate values that lie between two known data points, and can be easily implemented using the `interp1` function.
Here's a basic code snippet for linear interpolation in MATLAB:
% Sample data points
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
% Interpolating at new points
xi = 1.5; % Point to interpolate
yi = interp1(x, y, xi); % Perform linear interpolation
What is Linear Interpolation?
Linear interpolation is a method used to estimate unknown values that fall within the range of known data points. It assumes that the change between each pair of known values is linear and connects these points with straight lines. This technique is particularly useful in fields like engineering and data analysis when data points are scarce but we need to estimate intermediate values.
When to Use Linear Interpolation: This method is best applied in various scenarios, such as estimating performance metrics, data visualization, scientific analyses, or any other instances where discrete data points can be represented effectively on a linear scale.
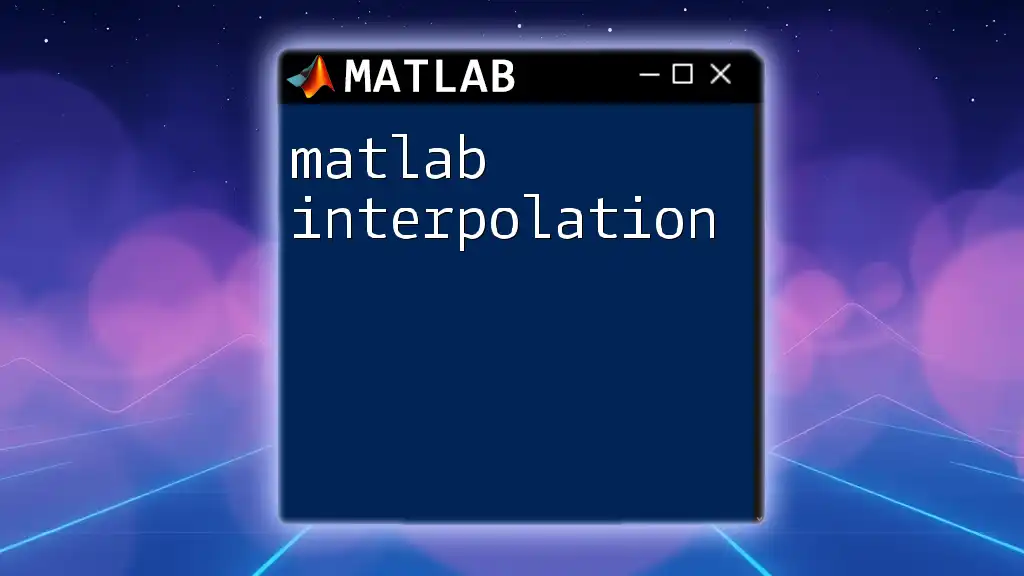
Understanding the Mathematics Behind Linear Interpolation
The formula for linear interpolation is defined as follows:
\[ y = y_0 + \left( \frac{y_1 - y_0}{x_1 - x_0} \right) \cdot (x - x_0) \]
In this formula:
- \( (x_0, y_0) \) and \( (x_1, y_1) \) are the known data points.
- \( x \) is the point where interpolation is performed.
- \( y \) is the interpolated value at point \( x \).
Understanding this formula is crucial, as it lays the foundation for implementing linear interpolation in MATLAB.
Graphical Representation
Visualizing the relationship between points helps solidify the concept of linear interpolation. Imagine a graph where two known data points are plotted. The linear interpolation will result in a straight line connecting these points, allowing for easy estimation of values at any point along the segment.
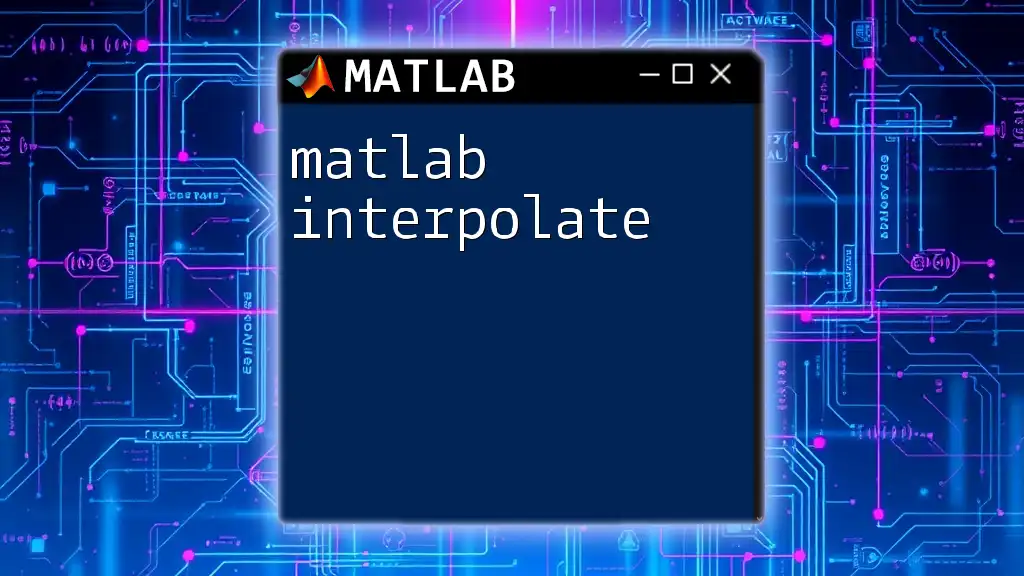
How to Perform Linear Interpolation in MATLAB
Preparing Your Data
Before diving into interpolation, you first need to prepare your data points. In MATLAB, you can represent your known x and y values using arrays. For instance:
x = [1, 2, 3, 4, 5];
y = [2, 4, 1, 3, 5];
Using MATLAB Functions for Interpolation
Interpolation Functions Overview
MATLAB provides several functions for interpolation, with `interp1` and `interp2` being the most commonly used for one-dimensional and two-dimensional data respectively. We're focusing primarily on `interp1` for linear interpolation here.
Using `interp1` for 1-D Linear Interpolation
To perform linear interpolation on a one-dimensional dataset, you will use the `interp1` function. Here's a step-by-step example:
- Define the query point where you want the interpolated value. For example, if you want to estimate the value at \( x = 2.5 \):
xq = 2.5; % Query point
yq = interp1(x, y, xq, 'linear');
disp(yq); % Outputs the interpolated value at xq
This command calculates the value of \( y \) at \( x = 2.5 \) using linear interpolation based on the previously defined arrays \( x \) and \( y \).
Advantages of Vectorized Interpolation
MATLAB excels at vectorized operations, allowing you to interpolate multiple values at once. For example, if you want to compute interpolated values for a range of \( x \):
xq = 2.5:0.1:4.5; % Query points
% Perform Interpolation for multiple query points
yq = interp1(x, y, xq, 'linear');
This flexibility can significantly speed up computations when dealing with large datasets.
Examples of Linear Interpolation Using MATLAB
Example 1: Simple Linear Interpolation
Now let’s look into a complete example illustrating linear interpolation. We will interpolate values between data points while also visualizing the result.
% Known Data Points
x = [1, 2, 3, 4, 5];
y = [2, 4, 1, 3, 5];
% Points for Interpolation
xq = 2.5:0.1:4.5; % Query points
% Perform Interpolation
yq = interp1(x, y, xq, 'linear');
% Plotting the Results
plot(x, y, 'o', xq, yq, '-');
legend('Data Points', 'Interpolated Line');
title('Linear Interpolation in MATLAB');
xlabel('x');
ylabel('y');
In this example, the original data points are displayed as circles, while the interpolated values are connected through a line to showcase the results visually.
Example 2: Interpolating with Different Methods
MATLAB allows you to experiment with various interpolation methods. Here, we will compare linear interpolation with spline interpolation to observe the differences:
yq_spline = interp1(x, y, xq, 'spline'); % Using spline method
plot(x, y, 'o', xq, yq, '-', xq, yq_spline, '--');
legend('Data Points', 'Linear Interpolation', 'Spline Interpolation');
This comparison vividly illustrates how different interpolation techniques affect the resulting estimates.
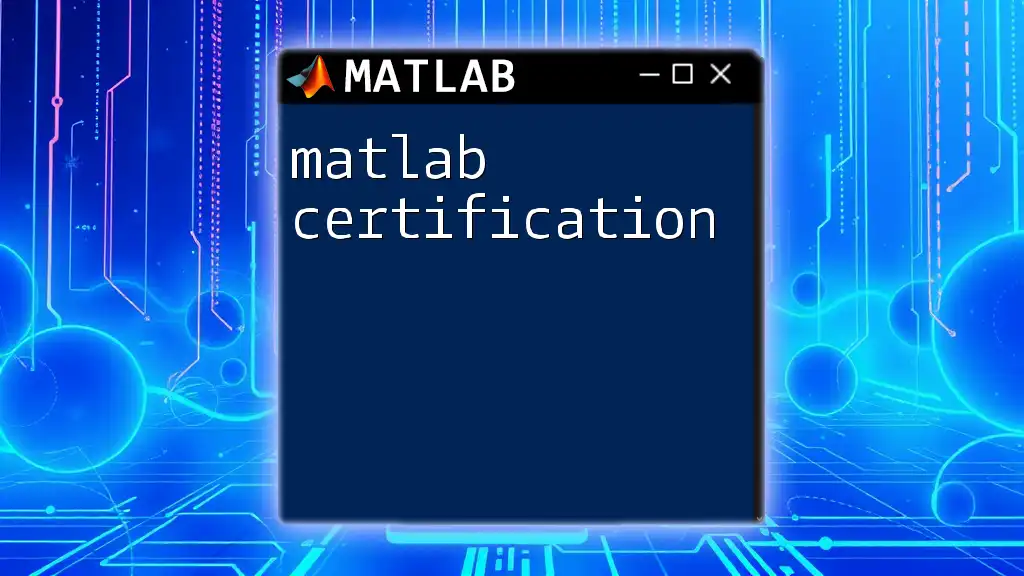
Best Practices for Linear Interpolation
Choosing the Right Input Data
To ensure accurate interpolation, it’s vital to select relevant and appropriately spaced data points. Consider the distribution of your data and the nature of the functions involved. Unevenly spaced points may yield misleading results.
Avoiding Extrapolation
While linear interpolation is effective within the boundaries of your data points, it becomes less reliable when used outside that range. Extrapolation is risky and can produce erroneous results, so be cautious when extending your estimates beyond known data points.
Understanding Error in Interpolation
It's essential to acknowledge that interpolation is an approximation. The interpolated values may not perfectly correspond to actual values, especially in non-linear relationships. Always assess the potential error involved and if possible, validate interpolated results against additional data sources.
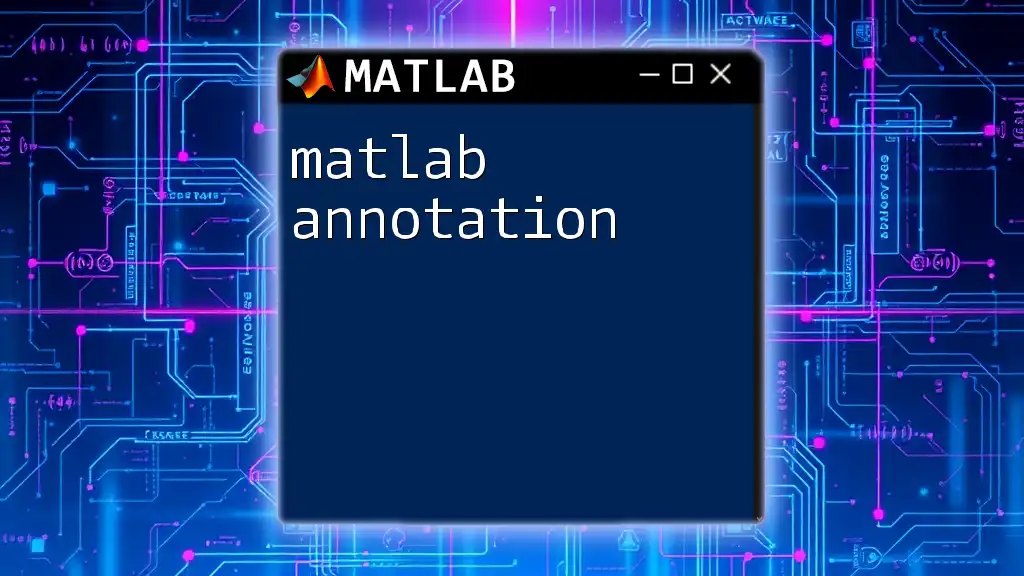
Conclusion
In summary, MATLAB linear interpolation is a powerful and convenient method for estimating unknown values between known data points. By leveraging built-in functions like `interp1`, users can perform efficient one-dimensional interpolation with ease. This guide has provided a comprehensive understanding of the mechanics behind linear interpolation, its MATLAB implementation, and best practices.
Now that you’re equipped with these insights, we encourage you to experiment with your datasets and explore the power of linear interpolation in MATLAB!
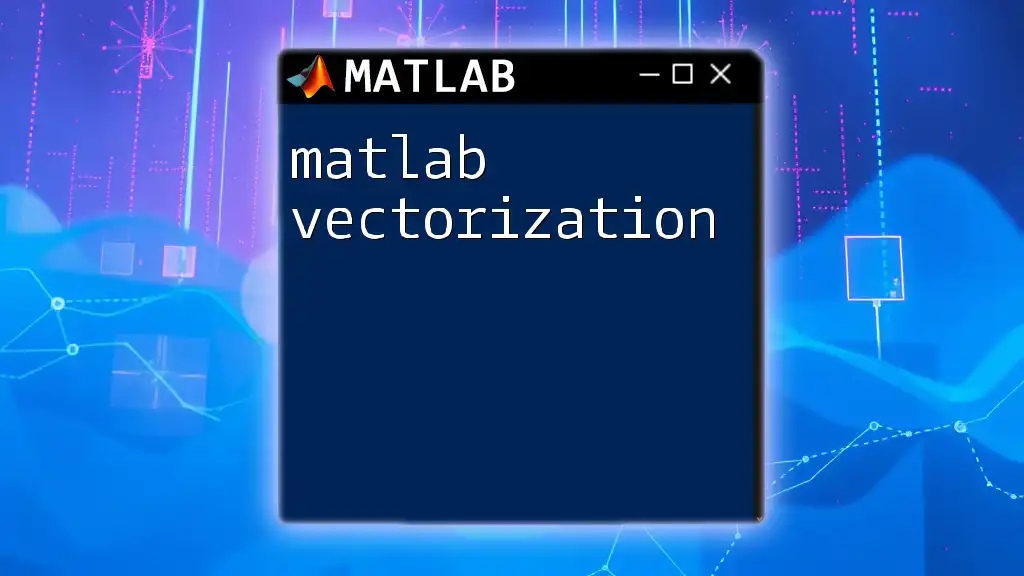
Additional Resources
For further reading on MATLAB linear interpolation and related concepts, look into the following resources:
- Recommended books focusing on numerical methods and MATLAB programming.
- Official MATLAB documentation detailing all functions related to interpolation and data analysis.
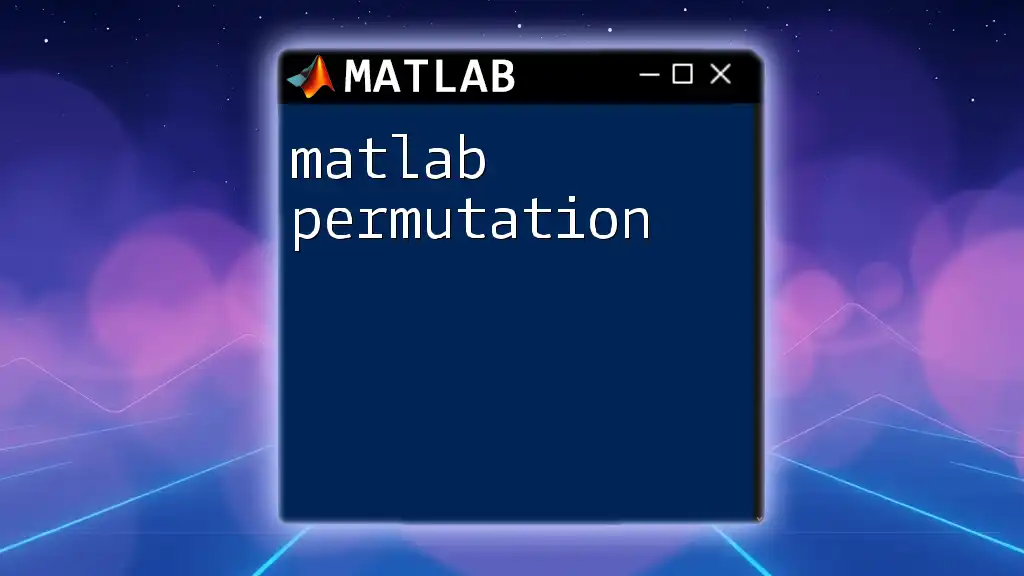
FAQ
What is the difference between linear and non-linear interpolation?
Linear interpolation assumes a straight line between two points, while non-linear methods (like spline interpolation) can create curves, providing potentially more accurate estimates for complex datasets.
When should I use `interp2` instead of `interp1`?
You should utilize `interp2` when working with two-dimensional datasets, such as images or grid data, where both \( x \) and \( y \) values are required for interpolation.
How can I visualize the error in my interpolation?
To visualize errors, you can calculate the difference between actual values and interpolated values and plot this difference. You can also use metrics like root mean square error (RMSE) for quantitative assessments.
This guide will not only enhance your understanding of MATLAB linear interpolation but also prepare you to apply these techniques effectively in your work.