In MATLAB, you can create and visualize a rectangle on a 2D plot using the `rectangle` function, which allows you to specify the position and dimensions of the rectangle.
rectangle('Position', [1, 2, 3, 4], 'EdgeColor', 'r', 'LineWidth', 2);
axis equal; % Set equal scaling on both axes
Understanding Rectangles
What is a Rectangle?
A rectangle is a fundamental shape in geometry defined by four sides with opposite sides that are equal in length, and four right angles (90 degrees). The simplicity of this shape belies its importance in many areas of mathematics and practical applications, including architecture, engineering, and computer graphics.
Applications of Rectangles
Rectangles are utilized extensively across various fields. From defining spaces in engineering designs to serving as the base shapes in graphical interfaces and data visualization, understanding how to manipulate rectangles programmatically (especially using MATLAB) is essential for students, engineers, and scientists alike.
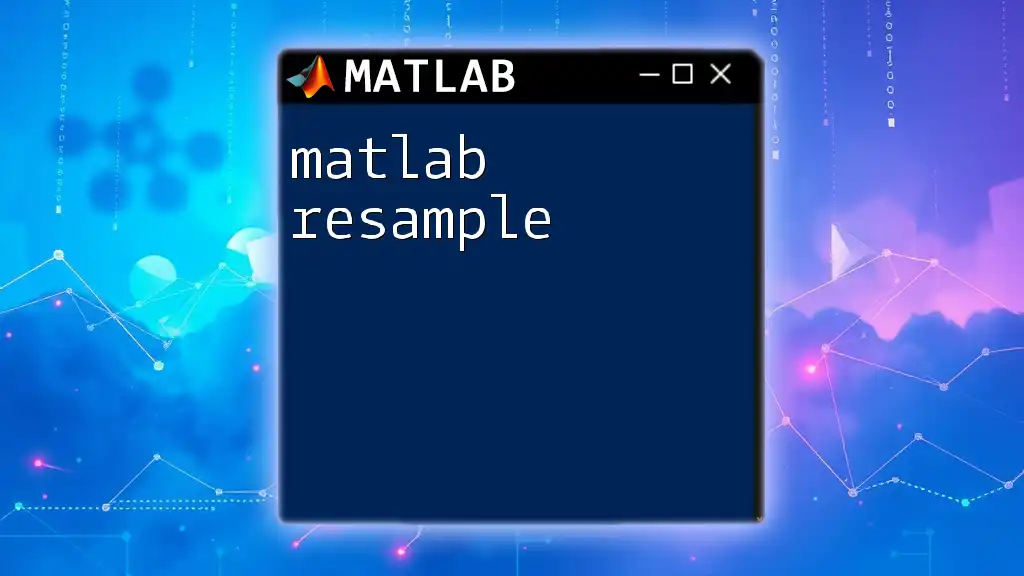
MATLAB Basics for Rectangle Commands
Getting Started with MATLAB
To work effectively with MATLAB, it's crucial to familiarize yourself with its interface and basic functionalities. When you first open MATLAB, you'll be greeted by the desktop environment, comprising of windows such as the Command Window, Workspace, and Editor.
To ensure you can create and visualize rectangles, you can enter simple commands directly in the Command Window or create scripts in the Editor for more complex tasks.
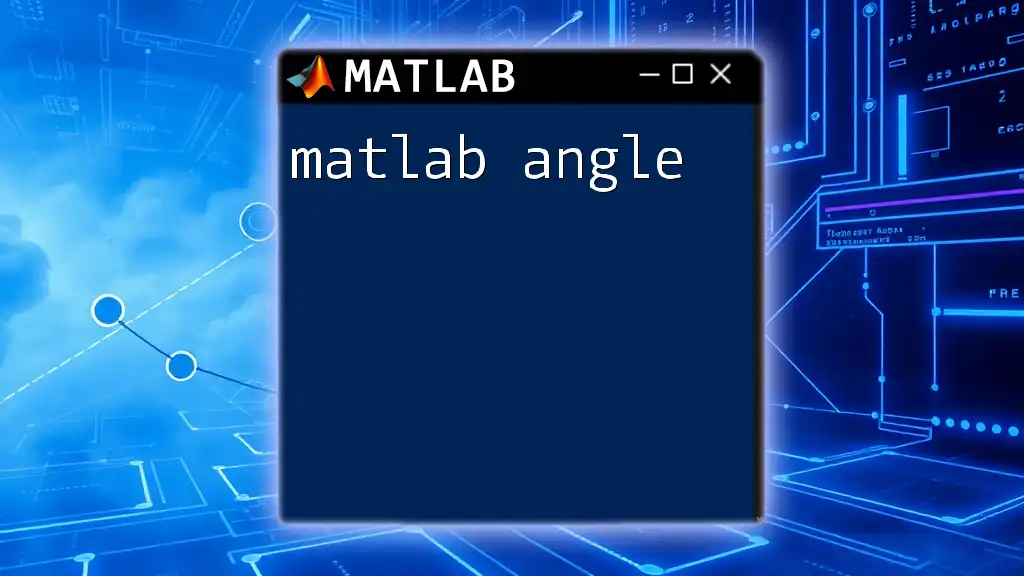
Creating a Rectangle in MATLAB
Using the `rectangle` Function
MATLAB provides a straightforward function called `rectangle` to create rectangles. The basic syntax for this function is:
rectangle('Position', [x y width height])
Here:
- `x` and `y` correspond to the coordinates of the lower-left corner of the rectangle.
- `width` is the horizontal distance from the lower-left corner to the lower-right corner.
- `height` is the vertical distance from the lower-left corner to the upper-left corner.
Example: Creating a Simple Rectangle
Creating a simple rectangle is as easy as executing the following code:
figure;
rectangle('Position', [1, 1, 5, 3]);
axis equal; % Maintain aspect ratio
title('Simple Rectangle');
In this example, a rectangle positioned at (1, 1) with a width of 5 and a height of 3 is generated. The `axis equal` command ensures that the rectangle retains its intended shape by providing equal scaling for both axes.
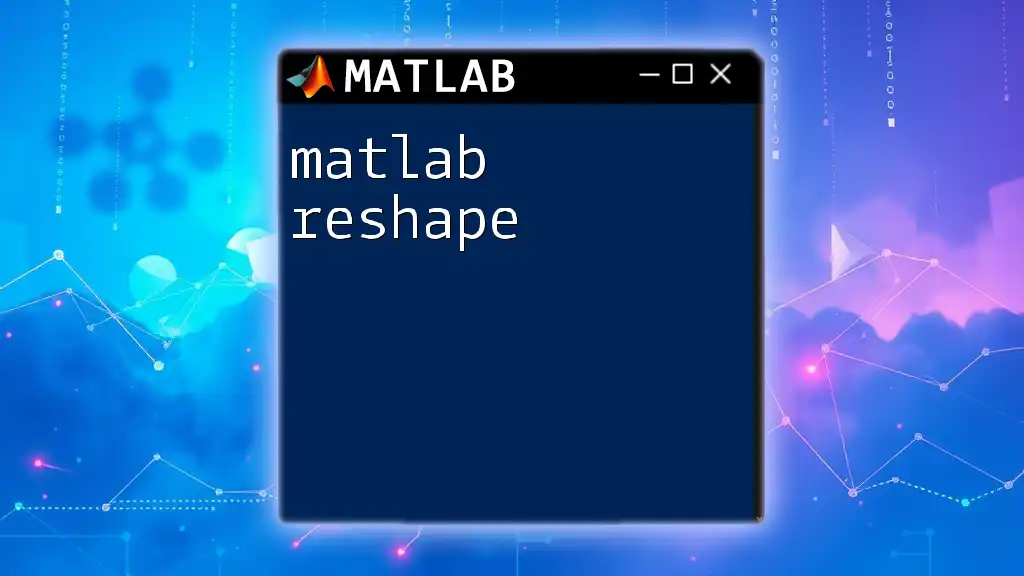
Customizing the Appearance of Rectangles
Changing Colors and Edge Properties
MATLAB allows considerable customization options for rectangles. The `rectangle` function has several attributes you can modify, including the `FaceColor`, `EdgeColor`, and `LineWidth` to enhance visual appeal.
For example, to create a rectangle with specific colors and line width, you can use:
figure;
rectangle('Position', [2, 2, 4, 2], 'FaceColor', 'red', 'EdgeColor', 'blue', 'LineWidth', 2);
axis equal;
title('Colored Rectangle with Custom Edges');
In this case, the rectangle has a red fill and blue border with a line width of 2 pixels. Exploring these options vastly improves the clarity and presentation of graphical outputs.
Adding Annotations and Labels
Enhancing your rectangle with labels can provide additional context. The `text` function allows you to place text anywhere in the figure. Here’s how to add a label within a rectangle:
figure;
rect = rectangle('Position', [2, 2, 4, 2], 'FaceColor', 'yellow');
text(4, 3, 'My Rectangle', 'HorizontalAlignment', 'center');
axis equal;
title('Rectangle with Annotation');
This code places the label "My Rectangle" at the coordinates (4, 3), centered on the rectangle, offering an informative visual cue.
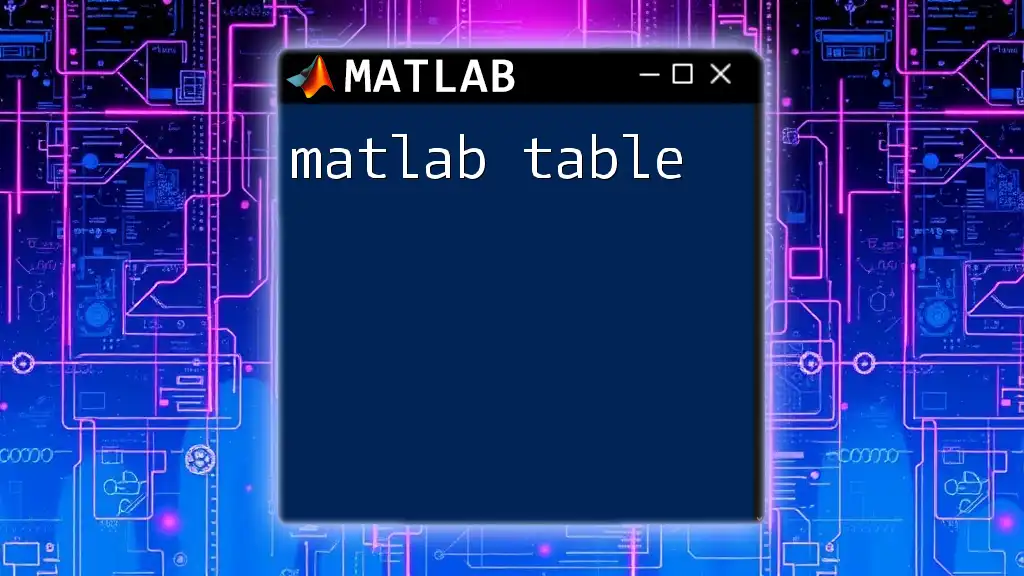
Combining Rectangles with Other Shapes
Creating Complex Figures
Creating complex figures using multiple rectangles can easily be done in MATLAB. By layering different rectangles, you can form intricate designs or composite shapes. The following example illustrates this:
figure;
rectangle('Position', [1, 1, 3, 3], 'FaceColor', 'green');
rectangle('Position', [2, 2, 3, 1], 'FaceColor', 'blue');
axis equal;
title('Complex Shape Using Rectangles');
In this instance, two rectangles overlap to create a composite shape, showcasing the power of layering and visual complexity in MATLAB graphics.
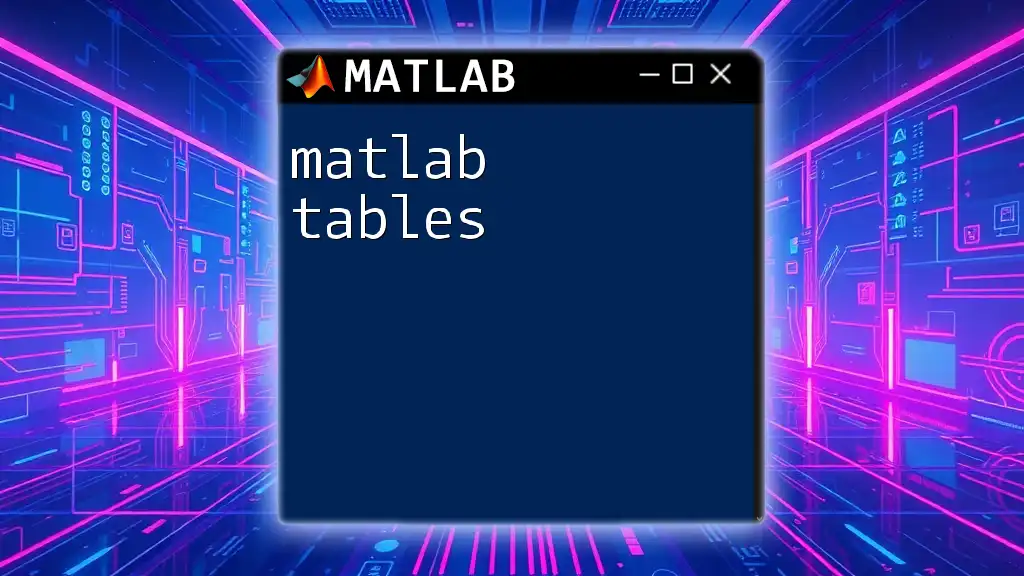
Understanding Rectangle Properties
Accessing and Modifying Rectangle Properties
MATLAB allows you to access and modify rectangle properties programmatically. Using the `get` and `set` functions, you can interact with existing rectangles in your plots.
An example of modifying rectangle properties is shown below:
r = rectangle('Position', [3, 3, 2, 2]);
set(r, 'FaceColor', 'cyan'); % Modify color
Here, a rectangle is created, and its fill color is changed to cyan. Understanding these properties enhances your ability to customize and control the elements in your graphical workspace.
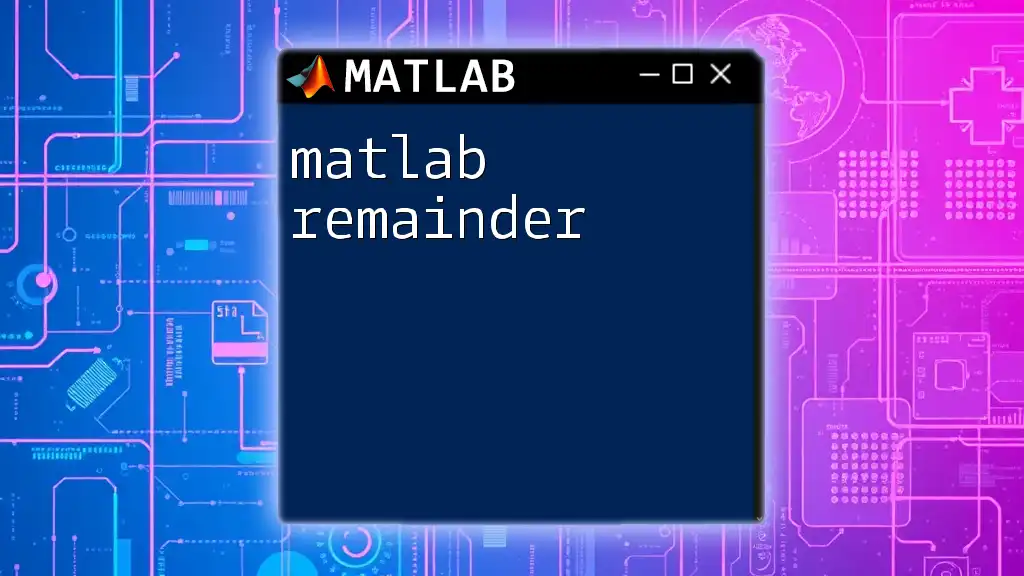
Advanced Uses of Rectangles
Dynamic Rectangles Using Data
One of the powerful capabilities of MATLAB is its handling of data-driven graphics. You can construct rectangles based on data stored in matrices or through user inputs. For example, consider the following code:
data = [1, 1, 2, 3; 2, 3, 3, 4]; % Example data
for i = 1:size(data, 1)
rectangle('Position', data(i,:), 'FaceColor', 'red');
end
axis equal;
title('Dynamic Rectangles from Data');
This script iterates over a data set to create multiple rectangles based on defined parameters, demonstrating how to generate graphics dynamically according to user-defined datasets.
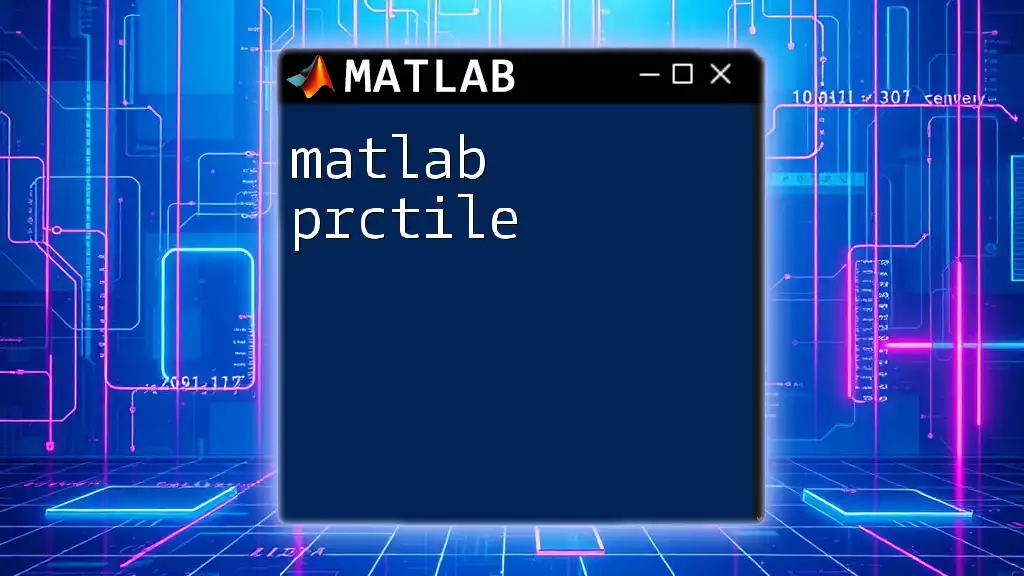
Conclusion
In summary, the variety of functionalities offered for working with MATLAB rectangles makes this temporary shape not only a fundamental geometrical figure but also a crucial building block for various practical applications in science and engineering. By mastering the commands, customization options, and dynamic functionalities of rectangles in MATLAB, you can produce clearer, more informative, and visually appealing graphics.
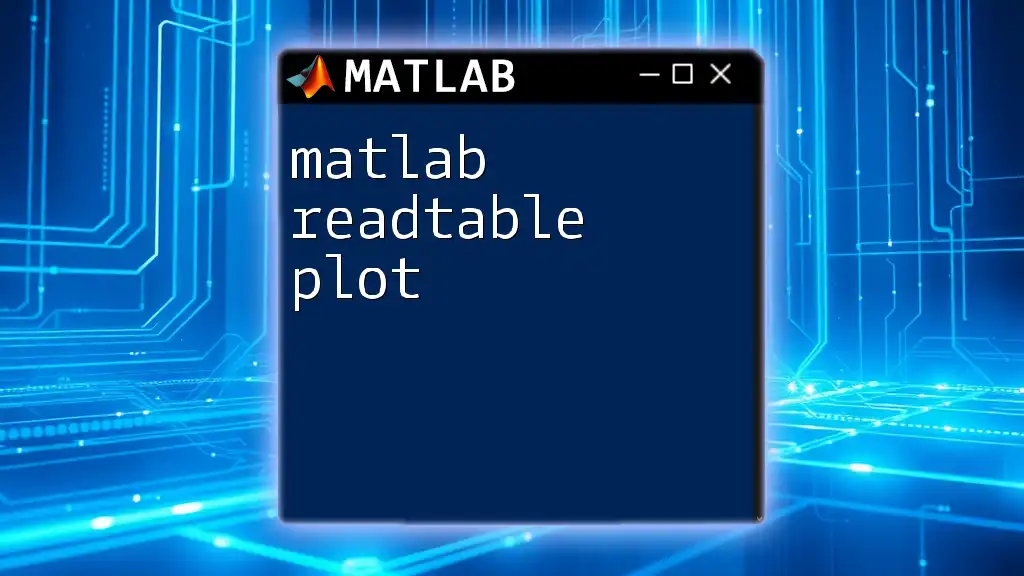
Additional Resources
Recommended MATLAB Documentation
For further reading and in-depth understanding, refer to the [MATLAB documentation](https://www.mathworks.com/help/matlab/) specifically related to graphics and the `rectangle` function.
User Community and Forums
Engaging with the MATLAB community through resources such as [MATLAB Central](https://www.mathworks.com/matlabcentral/) can provide additional insights and solutions, enriching your learning experience.