In MATLAB, an "if condition" allows you to execute code based on whether a specified condition evaluates to true or false. Here's a simple example demonstrating its syntax:
% Example of an if condition in MATLAB
x = 10;
if x > 5
disp('x is greater than 5');
end
Understanding the Basics of If Condition
What is an If Condition?
An if condition is a fundamental concept in programming that allows a program to make decisions based on certain criteria. It helps execute specific blocks of code only when particular conditions evaluate to true. In MATLAB, if conditions are integral to controlling the flow of execution in scripts and functions.
Syntax of If Condition in MATLAB
The general syntax of an if statement in MATLAB is structured as follows:
if condition
% code to execute if condition is true
end
It's important to emphasize the necessity of the `end` keyword to conclude the if statement, which differentiates it from other programming languages that may use braces or other mechanisms.
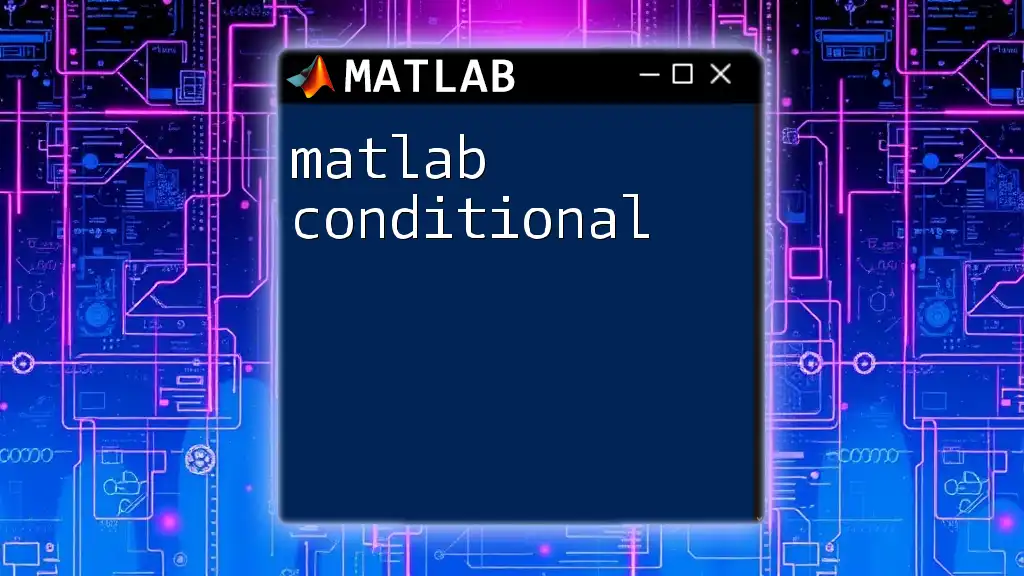
Types of If Conditions in MATLAB
Single If Condition
A single if condition checks a specific condition and executes a block of code only if that condition is true. This is the most basic use of an if statement.
For example:
x = 10;
if x > 5
disp('x is greater than 5');
end
In this case, since `x` is indeed greater than 5, the output will display “x is greater than 5.” If `x` were less than or equal to 5, nothing would be printed.
If-Else Condition
The `if-else` structure allows you to define alternative actions if the initial if condition is false. It's useful for handling multiple outcomes based on conditions.
For instance:
x = 3;
if x > 5
disp('x is greater than 5');
else
disp('x is 5 or less');
end
In this example, because `x` is not greater than 5, the output will be “x is 5 or less.” The if-else statement provides a clear distinction between conditions that require different actions.
If-Else If Condition
When dealing with multiple conditions, the else if statement allows for additional checks after the initial condition. This structure is essential for tiered decision-making.
For example:
x = 7;
if x < 5
disp('x is less than 5');
elseif x < 10
disp('x is between 5 and 10');
else
disp('x is 10 or more');
end
Here, the program checks each condition in sequence. Since `x` is between 5 and 10, it outputs “x is between 5 and 10.” This makes if-else if structures very powerful for evaluating multiple scenarios.
Nested If Condition
Nested if statements occur when an if statement exists within another if statement. This approach is useful when more complex conditional logic is required.
Consider the following example:
x = 10;
if x >= 0
if x == 0
disp('x is zero');
else
disp('x is positive');
end
else
disp('x is negative');
end
In this situation, if `x` is zero, the output will be “x is zero.” If `x` is any positive number, it will output “x is positive.” Only if `x` is negative will it display “x is negative.” Nesting allows for very specific conditions to be managed effectively.
Using Logical Operators in If Conditions
In MATLAB, logical operators like `&&`, `||`, and `~` provide the means to combine multiple conditions in a single if statement. This helps formulate more complex conditions.
For instance:
a = true;
b = false;
if a && ~b
disp('a is true and b is false');
end
In this example, the output will confirm both conditions, indicating that `a` is true and `b` is false. Using logical operators is crucial for handling scenarios where multiple criteria must be met.
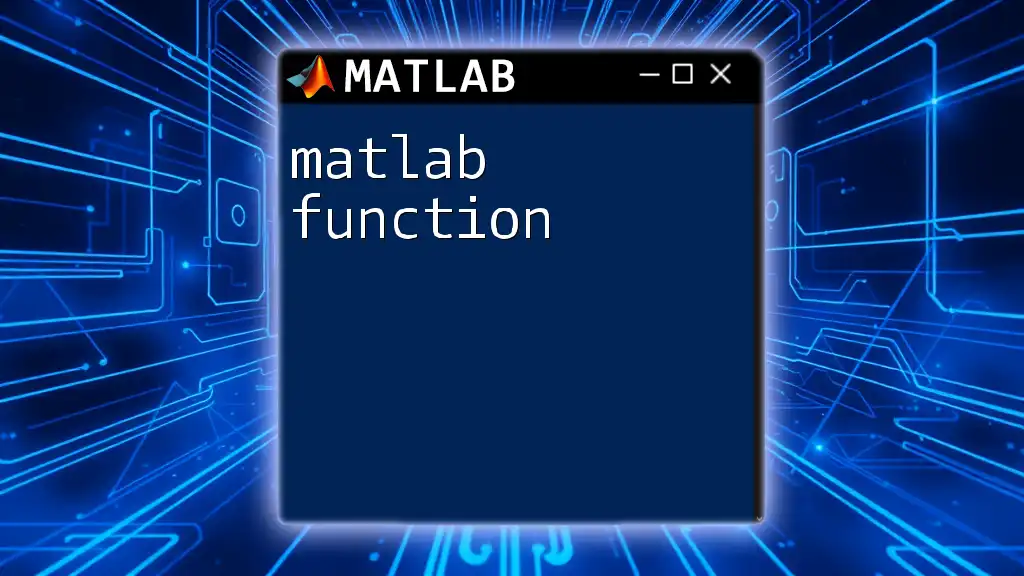
Practical Applications of If Conditions
Data Classification
If conditions are heavily employed in data classification, where specific criteria determine how datasets are categorized. A practical example can illustrate this:
score = 85;
if score >= 90
grade = 'A';
elseif score >= 80
grade = 'B';
else
grade = 'C';
end
disp(['Your grade is: ', grade]);
Based on the score, this code classifies grades accordingly. If a student's score is 85, the output will be “Your grade is: B.” This type of classification is common in educational environments and various algorithm implementations.
Input Validation
One significant application of if conditions is in input validation. Validating user inputs helps ensure that nothing unexpected disrupts the execution of the program.
For example:
input_value = -5;
if input_value < 0
disp('Input must be a non-negative number.');
else
disp('Input is valid.');
end
In this case, the program checks if the provided value is negative. If it is, the output will instruct users that the input is invalid. Effective input validation is critical for maintaining the integrity of data processing.
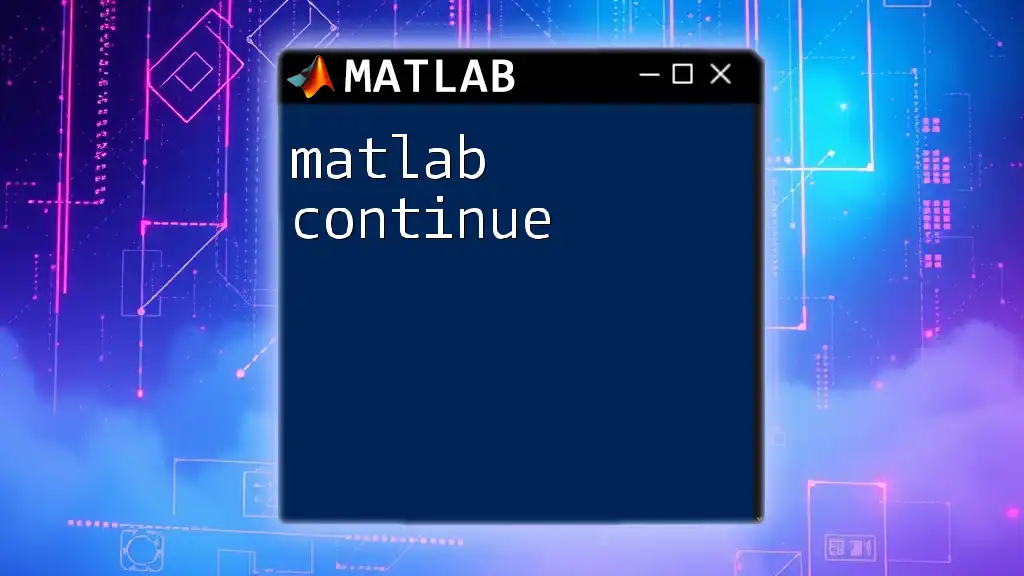
Common Errors and Debugging Tips
Common Mistakes in If Conditions
While working with if conditions, some common pitfalls include:
- Missing the `end` statement, which results in syntax errors.
- Incorrect logical conditions, leading to unintended execution paths.
Debugging Techniques
Debugging is crucial for identifying and resolving issues. Here are some effective techniques:
- Use breakpoints in the MATLAB editor to pause execution and inspect variable values.
- Utilize `disp` statements to output the status of certain conditions. For example:
x = 10;
if x < 5
disp('This will not execute.');
end
This approach can aid in understanding the flow of the program and tracking down where logic may go awry.
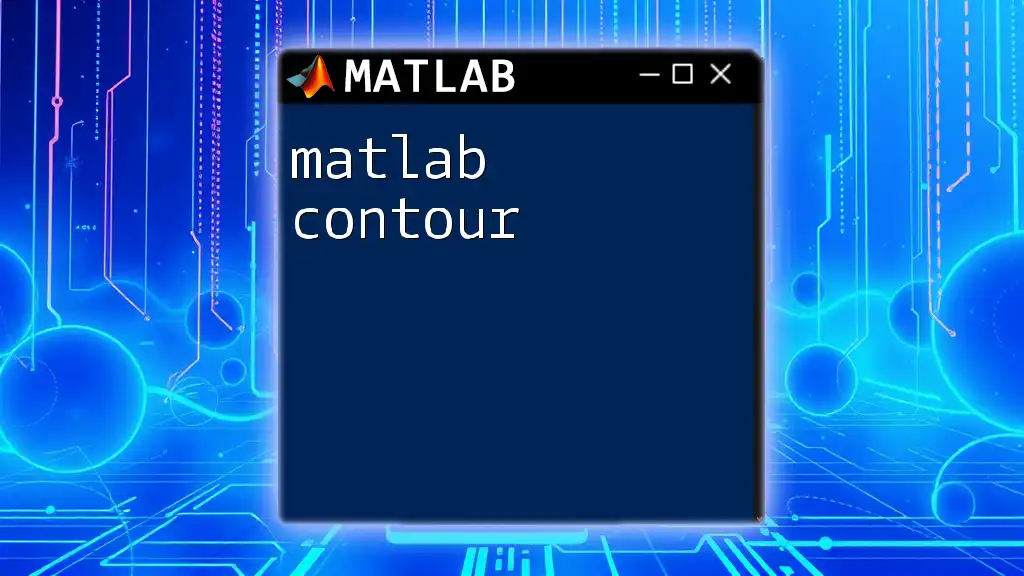
Conclusion
In summary, mastering the MATLAB if condition is essential for effective programming within MATLAB. These conditional statements empower users to create dynamic, responsive scripts that react to various logical scenarios. Understanding the syntax, types, applications, and potential pitfalls allows learners to elevate their MATLAB coding skills significantly.
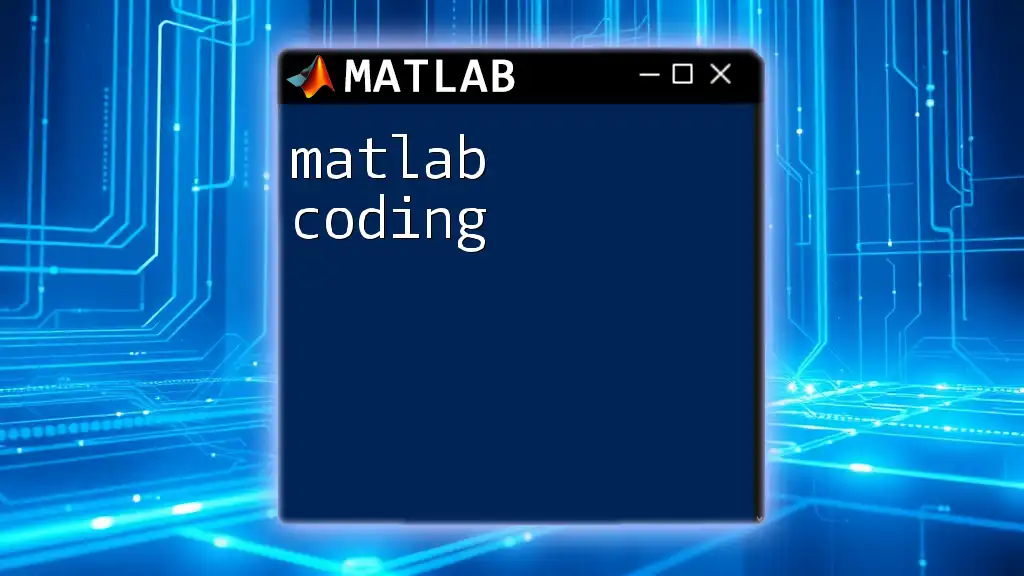
Additional Resources
For further exploration, consider reviewing the official MATLAB documentation on conditional statements and additional resources for programming best practices.
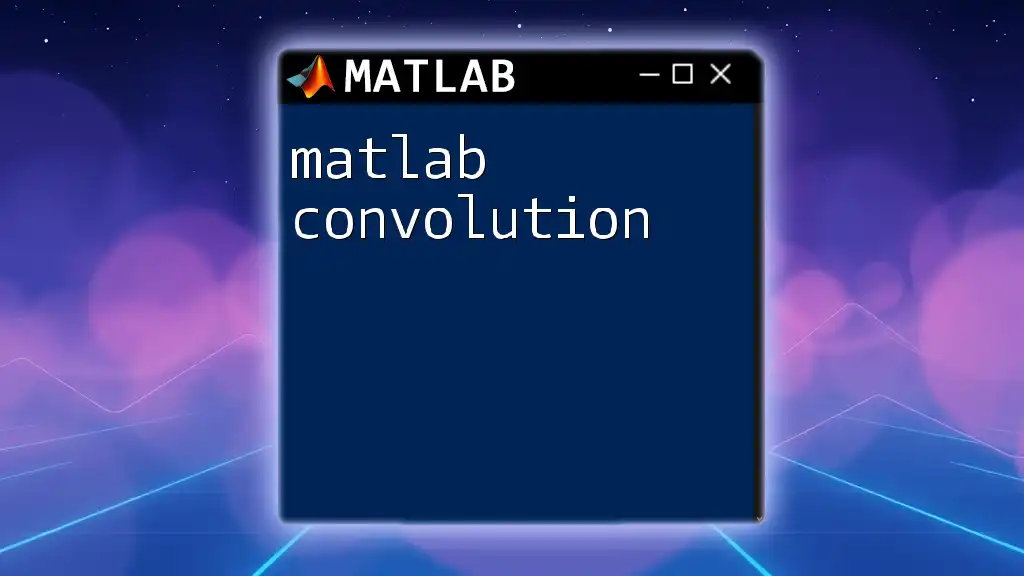
Call to Action
If you're eager to deepen your understanding of MATLAB, consider signing up for our training program. You’ll gain skills for quick and efficient learning that empowers you to tackle even complex programming scenarios with ease.
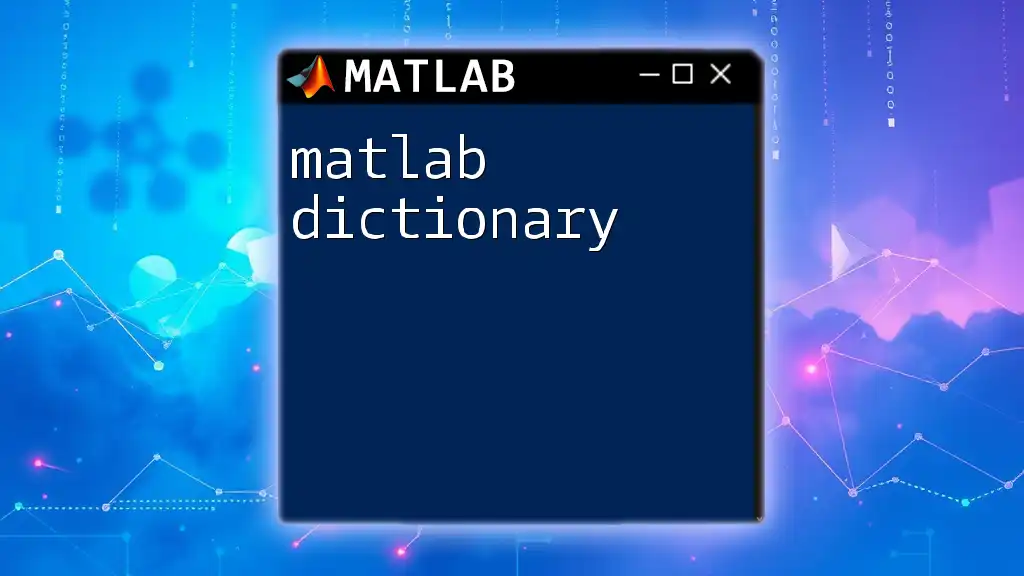
References
For further learning, check out the official MATLAB documentation and tutorials that provide extensive information on `if conditions` and beyond.