To read data from an Excel file in MATLAB, you can use the `readtable` function, which imports the content of an Excel sheet into a table format for easy manipulation.
data = readtable('filename.xlsx', 'Sheet', 'Sheet1');
Understanding Excel Files
What is an Excel File?
Excel files are spreadsheet documents commonly used for storing, organizing, and manipulating data. They allow users to arrange data in rows and columns, making it easy to analyze and visualize information. The two most prevalent file formats are `.xls` and `.xlsx`. The former is an older format associated with earlier versions of Microsoft Excel, while the latter is a more modern format that supports larger data sets and more complex calculations.
Why Use Excel with MATLAB?
Excel files are beneficial for several reasons:
- User-Friendly: Many users find Excel intuitive for data entry and management.
- Data Presentation: Excel allows for the organized presentation of data in a tabular form, which can be useful for reporting purposes.
- Interoperability: A wide range of software can read and write Excel files, making them a versatile option for data exchange.
Getting Started with MATLAB
Setting Up MATLAB for Excel Operations
Before diving into MATLAB, ensure you have the necessary toolboxes installed, particularly the Spreadsheet Toolbox. To verify that it's available, you can use the command `ver` in the MATLAB command window. Look for "Spreadsheet Toolbox" in the list.
Basic MATLAB Commands for Reading Excel
MATLAB provides several straightforward functions for reading Excel files, including `readtable`, `readmatrix`, and `readcell`.
`readtable`
This function imports data from an Excel file into a table format, well-suited for data manipulation.
Syntax:
T = readtable(filename)
Example: If you want to read a file named `data.xlsx`, you would use:
T = readtable('data.xlsx');
This command imports all the contents of the Excel file into a table `T`, where each column can be accessed using variable names.
`readmatrix`
For users looking to retrieve data in a numeric array format, `readmatrix` is the ideal function.
Syntax:
M = readmatrix(filename)
Example: To read the same Excel file as a matrix, apply:
M = readmatrix('data.xlsx');
This command is particularly useful when the data is numeric.
`readcell`
When dealing with mixed data types, including strings and numbers, `readcell` is the recommended choice.
Syntax:
C = readcell(filename)
Example: To read an Excel file with various data types, you would utilize:
C = readcell('data.xlsx');
This will store all Excel data in a cell array `C`, allowing for flexible data handling.
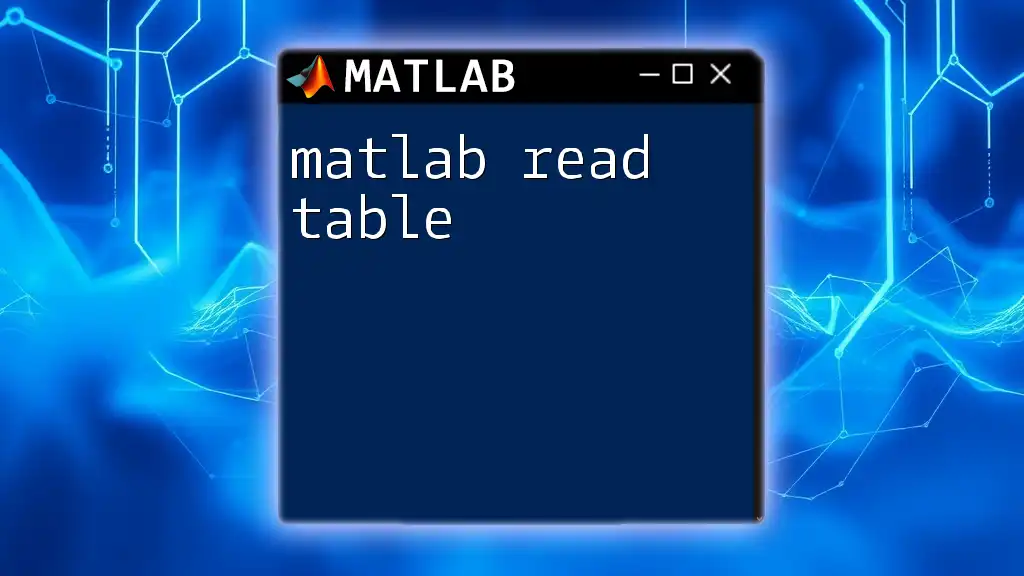
Advanced Options for Reading Excel Files
Specifying Sheet Names and Range
When dealing with complex Excel files, you often need to extract data from specific sheets or ranges.
Using `readtable` with Sheet Name
If your Excel workbook has multiple sheets and you want to target a specific one, you can specify the sheet name as follows:
T = readtable('data.xlsx', 'Sheet', 'Sheet1');
This command reads data only from "Sheet1" of the workbook.
Reading a Specific Range
You can also target a specific data range within a sheet for more focused data retrieval. For example:
T = readtable('data.xlsx', 'Sheet', 'Sheet1', 'Range', 'A1:C10');
This syntax will import only the data from cells A1 to C10 on "Sheet1".
Dealing with Header Rows
Excel files often contain header rows that define the variable names.
Using `readtable` to Skip Rows
Sometimes, it’s necessary to skip a set number of rows to focus on the actual data. You can customize the header reading like this:
T = readtable('data.xlsx', 'ReadVariableNames', true, 'HeaderLines', 3);
In this example, `HeaderLines` tells MATLAB to skip the first three rows, effectively allowing you to set the column headers from the following row.
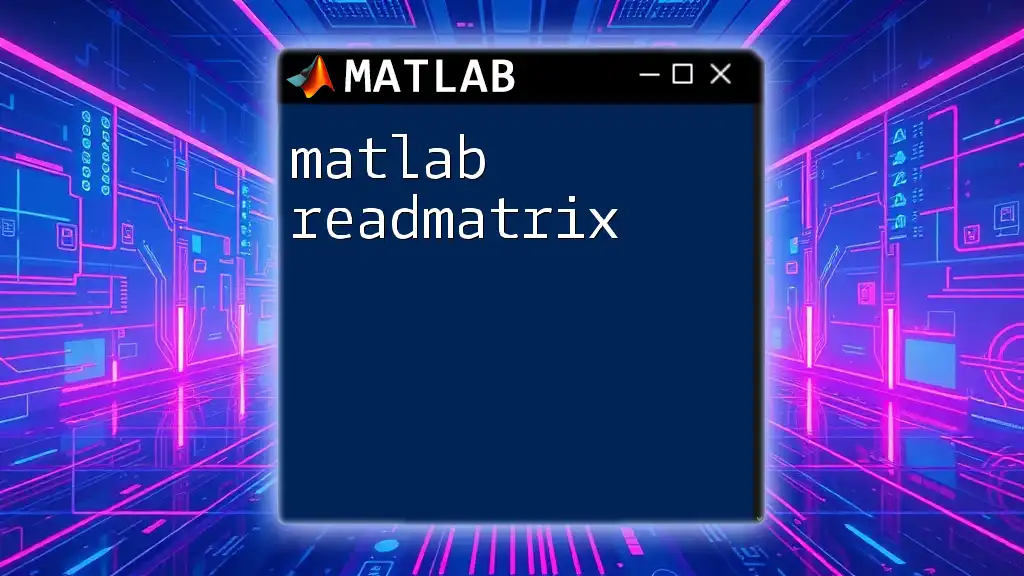
Data Cleaning and Preparation
Quick Data Examination
Once you import your data, it’s crucial to examine it for structure and content. You can use several commands to quickly review your data:
- `head(T)`: Displays the first few rows of the table.
- `summary(T)`: Provides a summary of the data types and descriptive statistics for each variable.
- `size(T)`: Returns the dimensions of the table, which helps you understand its size.
For example:
head(T)
summary(T)
size(T)
Handling Missing Data
Data quality is essential. Missing values can be a common issue in datasets. MATLAB provides several strategies to identify and handle these missing values effectively.
Identifying Missing Values
Use the command `ismissing(T)` to create a logical array that shows the position of missing values in a dataset.
missingData = ismissing(T);
Removing or Replacing Missing Values
You can either remove rows with missing data or replace them with a specific value. For example, to remove all rows containing any missing data, use:
T = rmmissing(T);
This command deletes any rows that contain one or more missing elements.
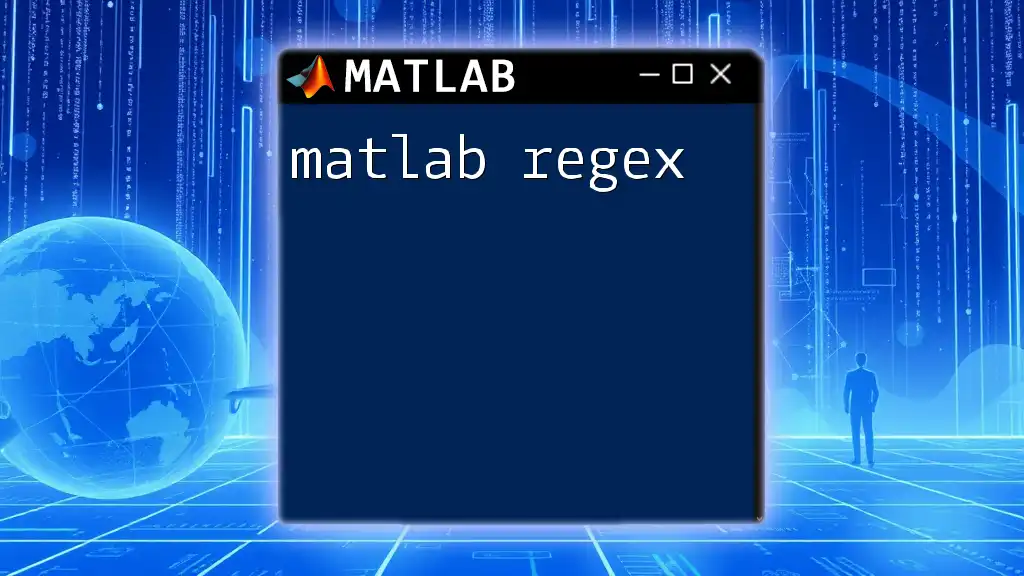
Practical Examples
Example 1: Importing and Analyzing Sales Data
Let’s walk through a practical example where you import a dataset containing sales records.
First, read the data:
salesData = readtable('sales_data.xlsx');
Now that you have imported the data, you can run a quick summary analysis:
summary(salesData)
This will give you insights into the sales figures, count, and data types, allowing you to make informed decisions about further analysis.
Example 2: Time Series Data Analysis
In another scenario, perhaps you're working with time series data to analyze trends.
Start by importing the time series dataset:
timeSeriesData = readtable('time_series.xlsx', 'Sheet', 'Data');
Next, you can visualize this data over time using a simple plot:
plot(timeSeriesData.Date, timeSeriesData.Value);
This command will create a visual representation of your data, helping you observe trends seamlessly.
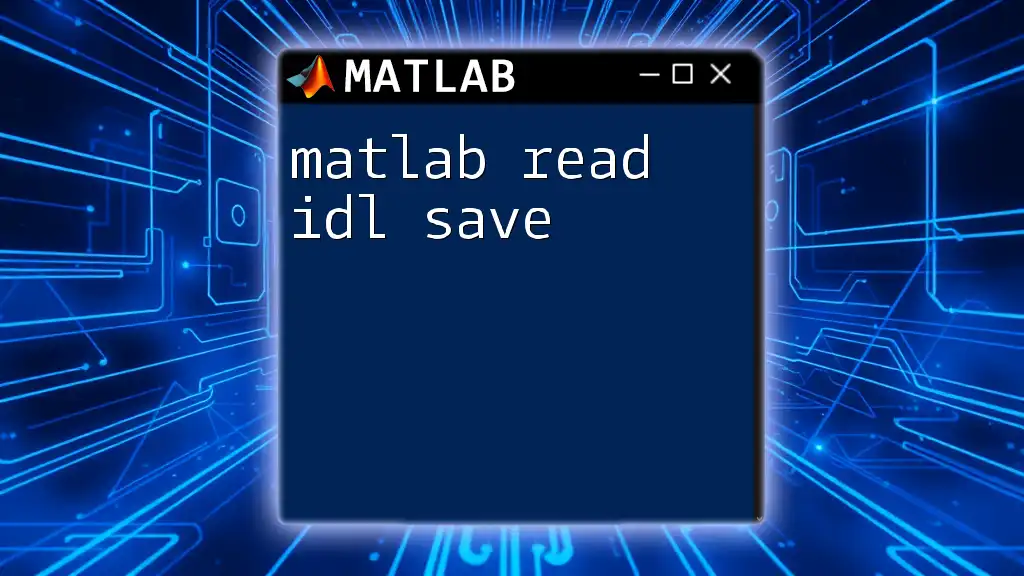
Common Errors and Troubleshooting
Common Errors When Reading Excel Files
While reading Excel files, users might encounter common errors, such as the file not being found, incorrect sheet names, or data type mismatches. Generally, these issues can easily be fixed by double-checking file paths and names.
Troubleshooting Tips
If you face issues, verify your file path using the `exist` function. For instance:
exist('data.xlsx', 'file')
This will return a logical value indicating whether the file is accessible. Always ensure that the Excel file is closed before attempting to read it in MATLAB, as an open file can lead to read errors.
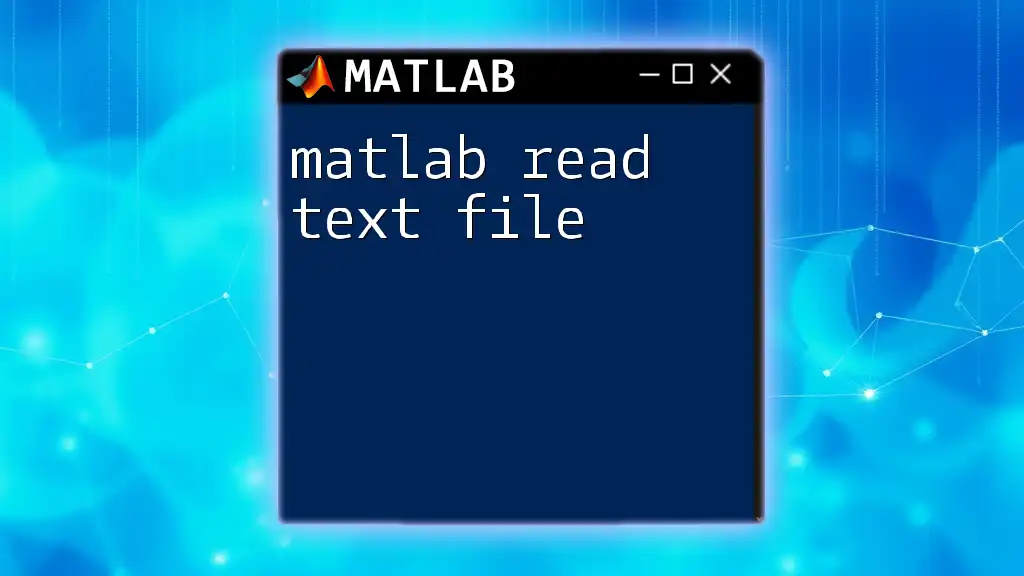
Conclusion
Reading Excel files with MATLAB is a powerful technique that can enhance data analysis capabilities. By leveraging functions like `readtable`, `readmatrix`, and `readcell`, users can efficiently manage and analyze data sets. It's essential to practice the functions outlined in this guide, as hands-on experience will significantly improve your proficiency in MATLAB. Explore additional resources and continuously engage with the MATLAB community for further learning opportunities.