In MATLAB, a cell array is a data type that allows you to store different types of data in one array, where each element can hold a varying type or size of data, making it particularly useful for heterogeneous collections.
Here’s a simple example of creating and accessing a cell array in MATLAB:
% Creating a cell array
C = {1, 'text', [1, 2, 3]; 'another text', pi, true};
% Accessing elements in the cell array
firstElement = C{1, 1}; % Retrieves the number 1
secondElement = C{1, 2}; % Retrieves the string 'text'
thirdElement = C{2, 3}; % Retrieves the boolean true
Understanding Cell Arrays
What are Cell Arrays?
Cell arrays in MATLAB are versatile data containers that allow you to store data of varying types and sizes. Unlike regular arrays, which require all elements to be of the same data type, cell arrays enable you to mix types. This makes them especially useful for handling complex datasets, where you might have numbers, strings, or even other arrays all in one structure.
When to Use Cell Arrays
You should consider using cell arrays when your data includes various types, such as when you need to store strings alongside numbers or arrays. For example, if you are working with a mix of different data types in scientific computing, cell arrays provide the flexibility needed without enforcing type consistency.
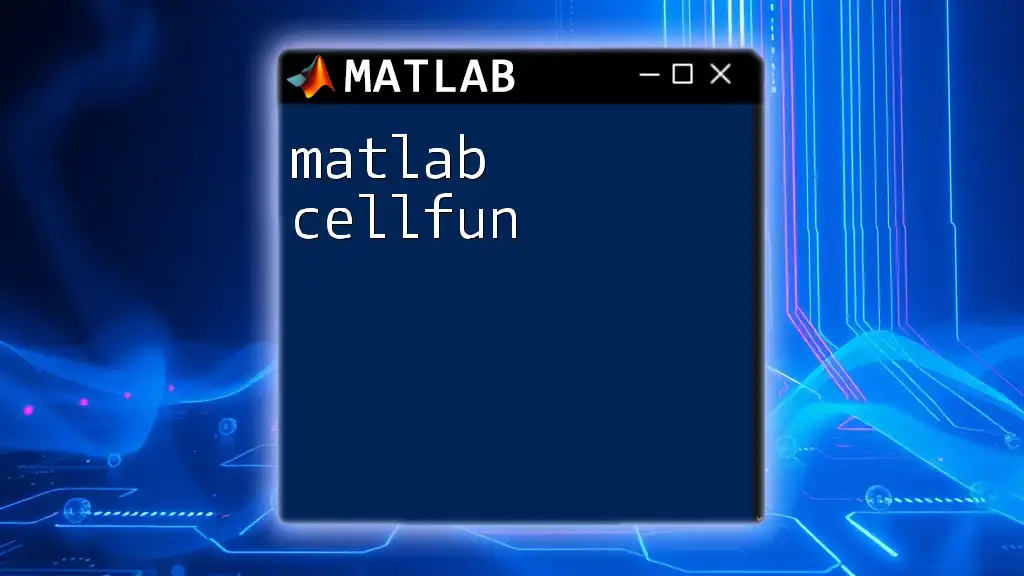
Creating Cell Arrays
Basic Syntax for Creating Cell Arrays
To create a cell array, you can utilize the curly braces `{}` as follows:
C = {1, 2, 3; 'Text', rand(3), {4, 5}};
In this example, `C` is a 2x3 cell array, containing integers, a string, a random 3x3 matrix, and another cell array. You can see how rich and varied the structure of a cell array can be.
Using Functions to Create Cell Arrays
An alternative way to create cell arrays is by using the `cell()` function. This function allows you to specify the size of the array.
C = cell(2,3); % Creates a cell array of size 2x3 filled with empty cells
This initializes all elements to empty, and you can later populate it with whatever data types you need.
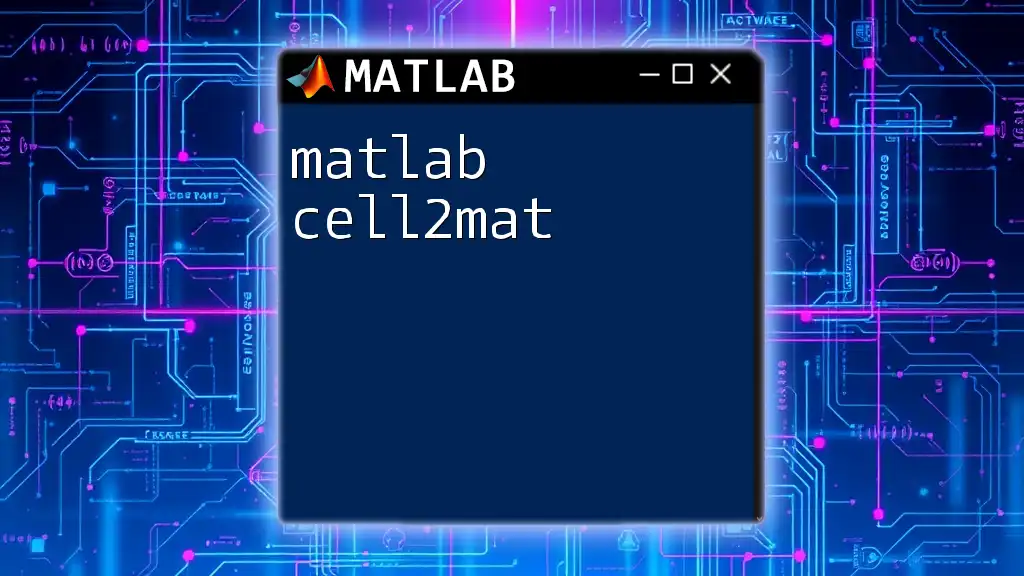
Accessing Data in Cell Arrays
Indexing Cell Arrays
Accessing data within cell arrays requires a clear understanding of indexing. You can retrieve individual elements using curly braces `{}`:
val = C{1,2}; % Accessing the element at row 1, column 2
The use of curly braces `{}` denotes direct access to the content of that specific cell. On the other hand, if you use parentheses `()`, you get back a subcell array.
Extracting Subsets of Cell Arrays
You can access portions of a cell array by specifying ranges. For example, to extract the first two columns, you can use:
subArray = C(:, 1:2); % Accessing the first two columns
This technique is powerful when you want to work with groups of related data.
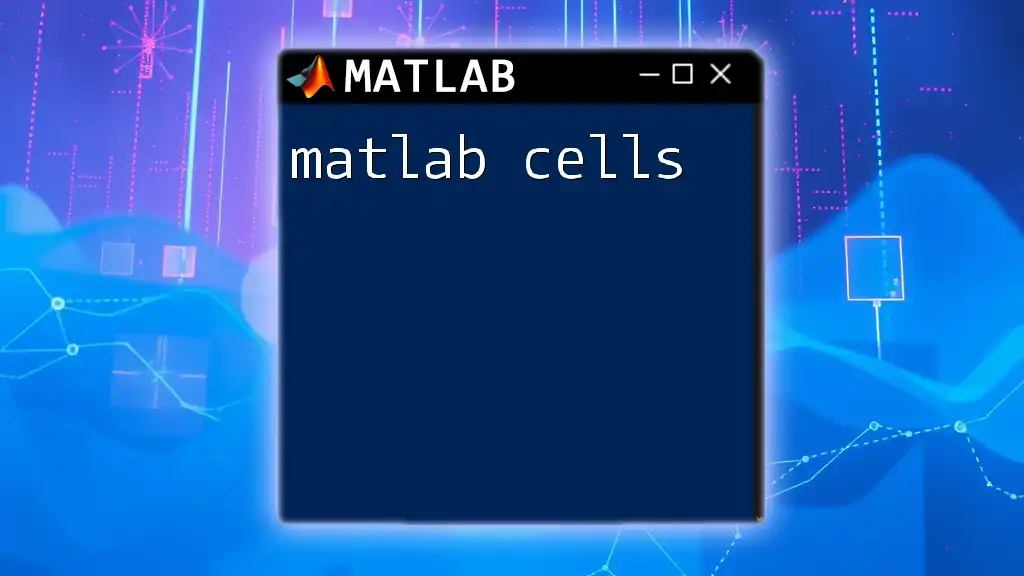
Modifying Cell Arrays
Adding Data to Cell Arrays
You can easily add new elements to a cell array. For example, to append a new item, run:
C{end + 1, 1} = 'New Item'; % Appending a new item in the first column of the next row
Using `end` allows MATLAB to automatically determine the next available index, streamlining the addition process.
Removing Data from Cell Arrays
If you need to remove unwanted elements, you can do so as follows:
C(1,2) = []; % Removing the element at row 1, column 2
This operation will eliminate the specified cell and shift the remaining cells accordingly.
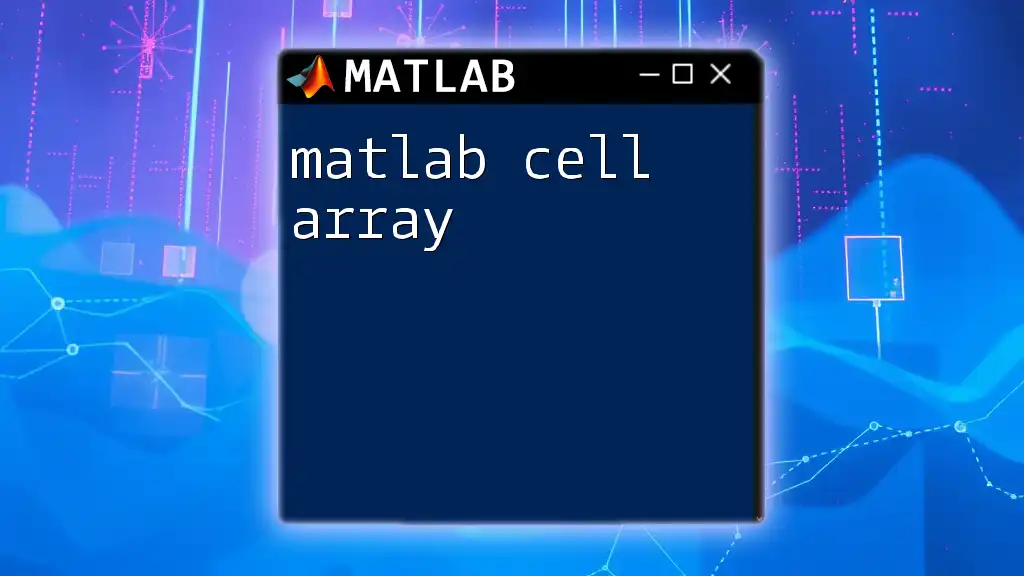
Cell Arrays vs. Other Data Structures
Comparison with Numeric and Character Arrays
Cell arrays differ fundamentally from standard numeric and character arrays. While standard arrays can only contain one type of data, cell arrays provide flexibility to include varying types. This flexibility is crucial when handling complex datasets.
Pros and Cons of Using Cell Arrays
Advantages: Cell arrays can store different data types in one structure, making them highly versatile for exploratory data analysis and when dealing with heterogeneous data.
Disadvantages: They may incur performance drawbacks when working with large datasets due to their inherent flexibility. Standard arrays might be preferred for consistency and efficiency.
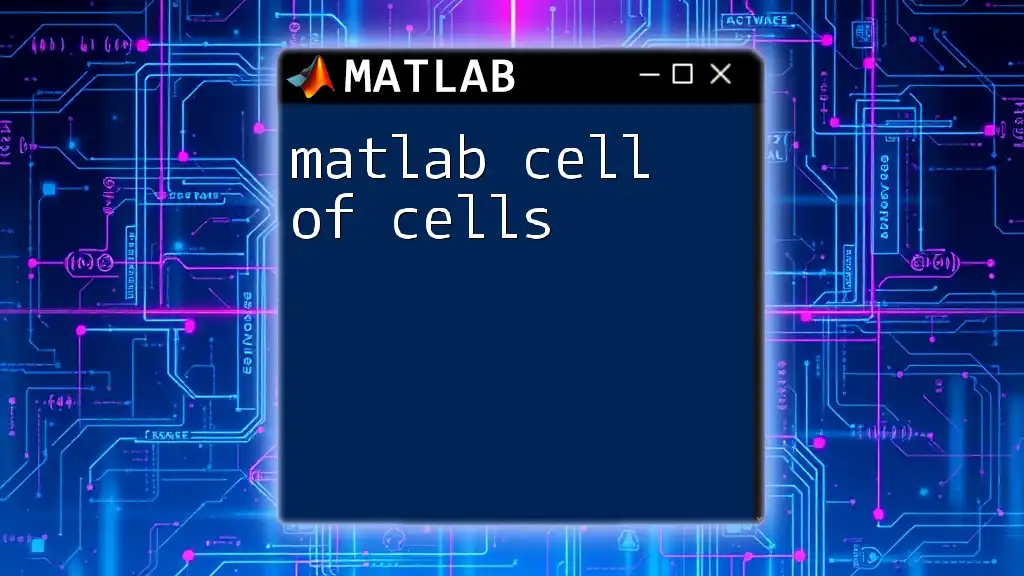
Common Operations with Cell Arrays
Concatenation of Cell Arrays
Concatenating cell arrays is straightforward. Here’s how to perform horizontal concatenation:
C1 = {1, 2, 3};
C2 = {4, 5, 6};
C_combined = [C1, C2]; % Horizontal concatenation
For vertical concatenation, you can simply stack them using a semicolon between the two arrays.
Looping Through Cell Arrays
You may need to process each element of a cell array with a loop. For instance, to display all elements, you could use:
for i = 1:numel(C)
disp(C{i}); % Displaying each element
end
This method is particularly effective for applying functions or performing calculations on each item.
Applying Functions to Cell Arrays
Using the `cellfun()` function allows you to apply a function to each cell. For instance, if you want to find the length of each element, you can execute:
lengths = cellfun(@length, C); % Finding lengths of elements in cell array
This is a compact and efficient way to process entire arrays at once.
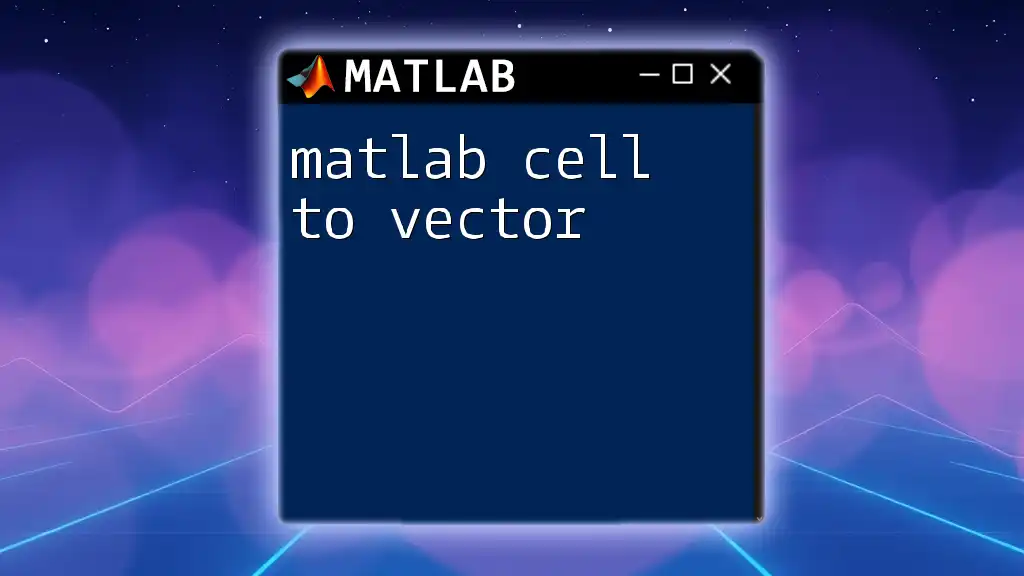
Practical Examples
Storing Mixed Data Types
Creating a cell array that combines strings, numbers, and arrays can be incredibly useful. Here’s an example:
data = {'Name', 'Age'; 'Alice', 30; 'Bob', 25};
In this cell array, you can store related information about individuals, making data retrieval and manipulation intuitive.
Use Case: Simulating Experiment Data
Consider an experiment where you want to store parameters and results. A cell array can structure this data effectively, facilitating easy access and analysis of varied results.
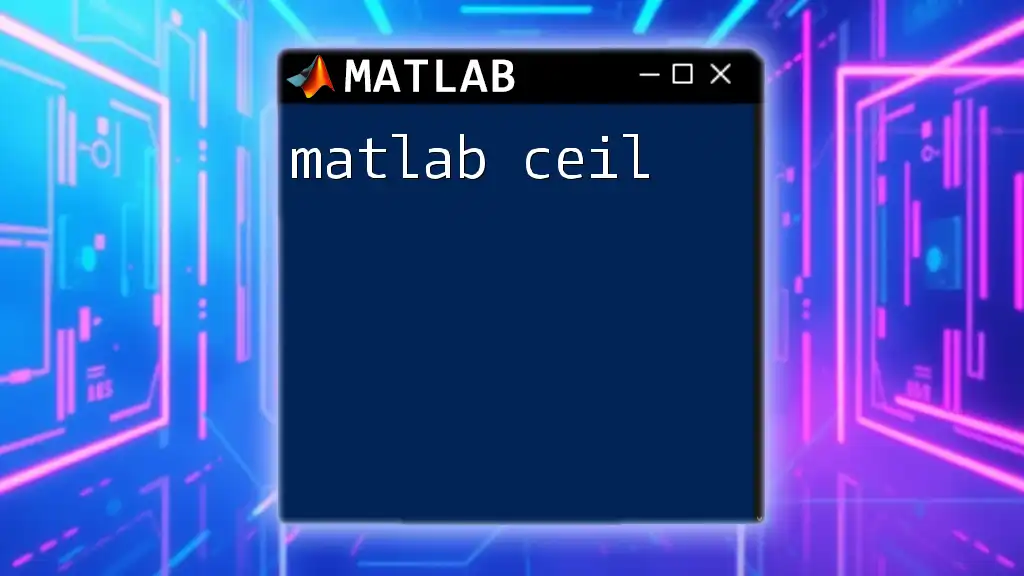
Debugging Common Errors
Common Mistakes with Cell Arrays
A typical mistake is trying to access elements using standard indexing, which can lead to unexpected results or errors. Remember to use curly braces for accessing actual contents of cells.
Using `try-catch` Blocks for Error Handling
Implementing `try-catch` blocks can help manage errors gracefully when accessing cell array elements, ensuring that your program can run smoothly without abrupt failures.
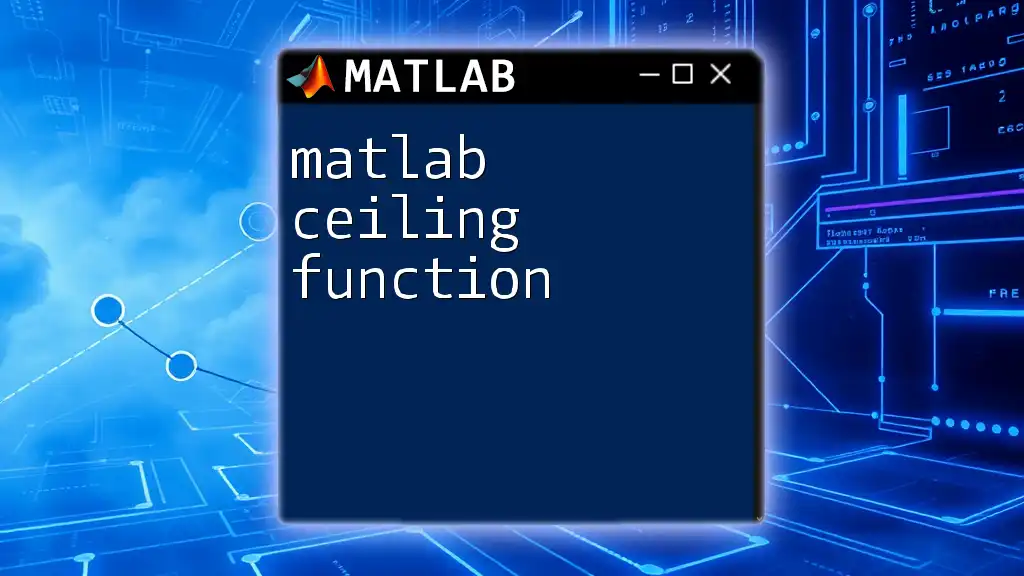
Conclusion
Recap of Cell Array Benefits
MATLAB cell arrays are powerful tools for storing and manipulating data of diverse types, making them indispensable for many applications in data analysis and scientific research.
Encouraging Further Learning
To truly master cell arrays and other data structures in MATLAB, consider immersing yourself in more tutorials and experimentation. The flexibility and functionality of MATLAB can significantly boost your data handling capabilities.
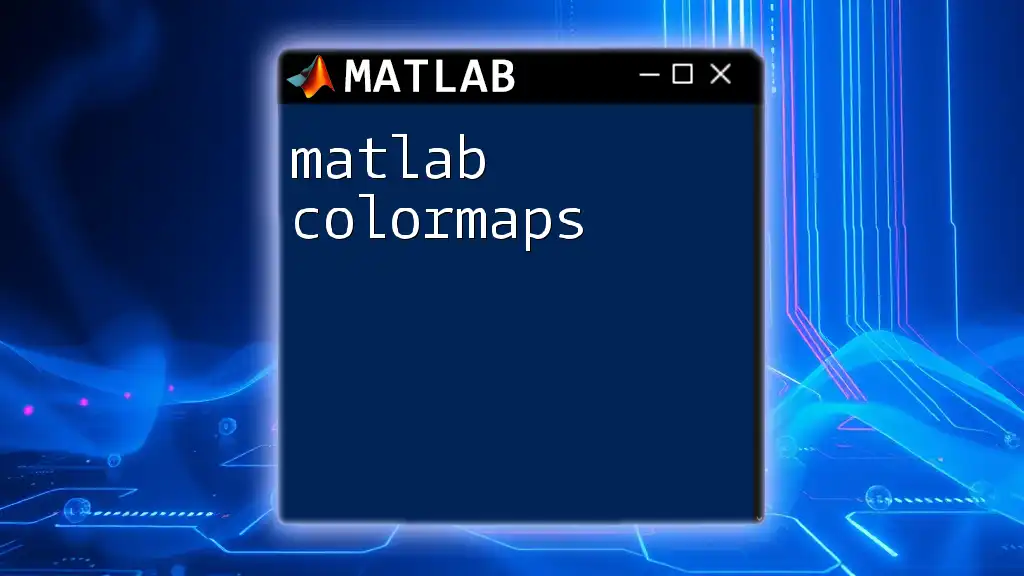
Call to Action
Join Our MATLAB Tutorials
If you found this guide helpful, we invite you to subscribe for more concise MATLAB lessons and tips tailored to enhance your proficiency in using MATLAB effectively.