`cellfun` is a MATLAB function that applies a specified function to each cell in a cell array, allowing for efficient batch processing of cell contents.
Here's a code snippet demonstrating its use:
% Example of using cellfun to compute the length of each string in a cell array
strArray = {'hello', 'world', 'matlab'};
lengths = cellfun(@length, strArray);
disp(lengths); % Output: [5 5 6]
Understanding Cell Arrays in MATLAB
What Are Cell Arrays?
Cell arrays in MATLAB are a powerful data structure that allows you to store data of varying types and sizes. Unlike traditional numeric arrays, which can only hold numbers of the same type, cell arrays can contain different data types within a single array. For instance, you can have strings, matrices, and even other cell arrays all in one cell array.
One major difference between cell arrays and regular numerical arrays is that cell arrays are indexed with curly braces `{}` instead of parentheses `()`. This means that when accessing an element in a cell array, you will use:
C{2} % Accesses the second element of the cell array C
Why Use `cellfun`?
The function `cellfun` is specifically designed for applying a function to each element of a cell array efficiently. This capability is especially useful because it eliminates the need for cumbersome loops, leading to cleaner and more readable code. Some key benefits of using `cellfun` include:
- Simplicity: You can apply functions directly without writing iterative code.
- Performance: MATLAB optimizes `cellfun`, making it faster than equivalent loop operations.
- Versatility: Suitable for a wide range of operations, from mathematical functions to logical tests.

Understanding the Basics of `cellfun`
What is `cellfun`?
`cellfun` is a MATLAB function that applies a specified function to each element of a cell array. The syntax of `cellfun` is as follows:
B = cellfun(fun, C)
Where:
- `fun` is the function you want to apply (it can be a built-in function or a user-defined function).
- `C` is the input cell array.
- `B` is the output array, containing the results.
Key Parameters of `cellfun`
In addition to the basic syntax, `cellfun` offers additional parameters for greater control over your function application:
- `UniformOutput`: This parameter determines whether the output is a uniform array. If set to `true`, `cellfun` assumes that the output from `fun` will be a single value for each cell. If the outputs are non-uniform (different sizes or types), set `UniformOutput` to `false` to return a cell array.
For example:
B = cellfun(fun, C, 'UniformOutput', false)
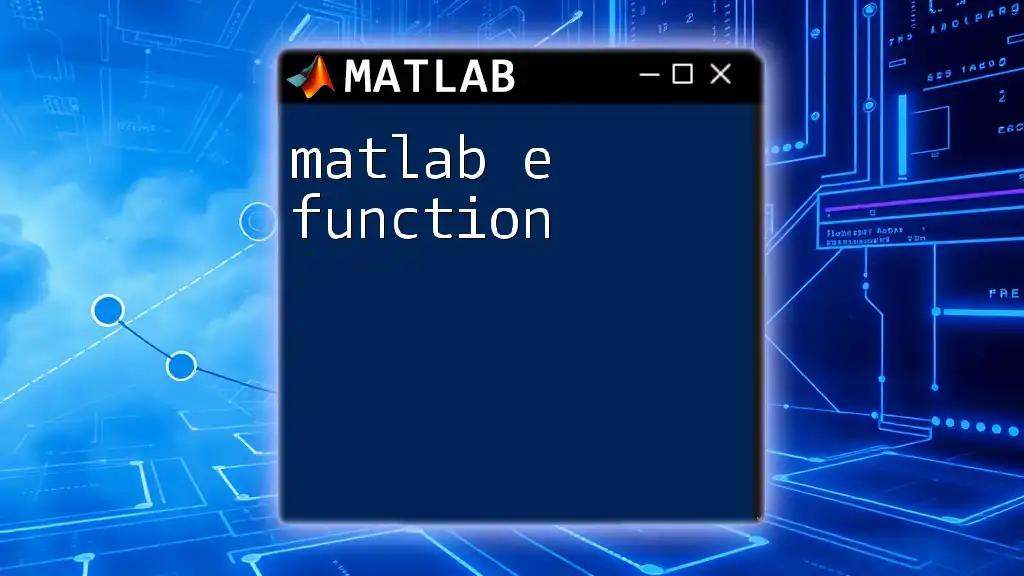
How to Use `cellfun`: Practical Examples
Example 1: Applying a Simple Function to a Cell Array
One of the simplest uses of `cellfun` is to convert strings to numbers. Here's a basic example:
C = {'1', '2', '3'};
numericArray = cellfun(@str2double, C);
In this snippet, `@str2double` is the function applied to each element, converting the string representations of numbers to their numeric form. The resulting `numericArray` will be `[1, 2, 3]`.
Example 2: Using `cellfun` with Custom Functions
Creating a Custom Function
Sometimes, you may need to apply a custom function to your cell array. Here’s how to define a simple custom function that squares a numerical value provided as a string.
function output = squareValue(x)
output = str2double(x)^2;
end
Applying the Custom Function
Now, use this function with `cellfun`:
C = {'1', '2', '3'};
squaredArray = cellfun(@squareValue, C);
In this case, the output `squaredArray` will be `[1, 4, 9]`, showcasing how you can effectively utilize custom logic with `cellfun`.
Example 3: Applying Logical Functions
You can also use `cellfun` to perform logical operations. Let's say you want to check which numbers in a cell array are even:
C = {1, 2, 3; 4, 5, 6};
isEven = cellfun(@(x) mod(x, 2) == 0, C);
In this example, `@(x) mod(x, 2) == 0` is an anonymous function that checks if values are even. The resulting `isEven` variable will be a logical array:
0 1 0
1 0 1
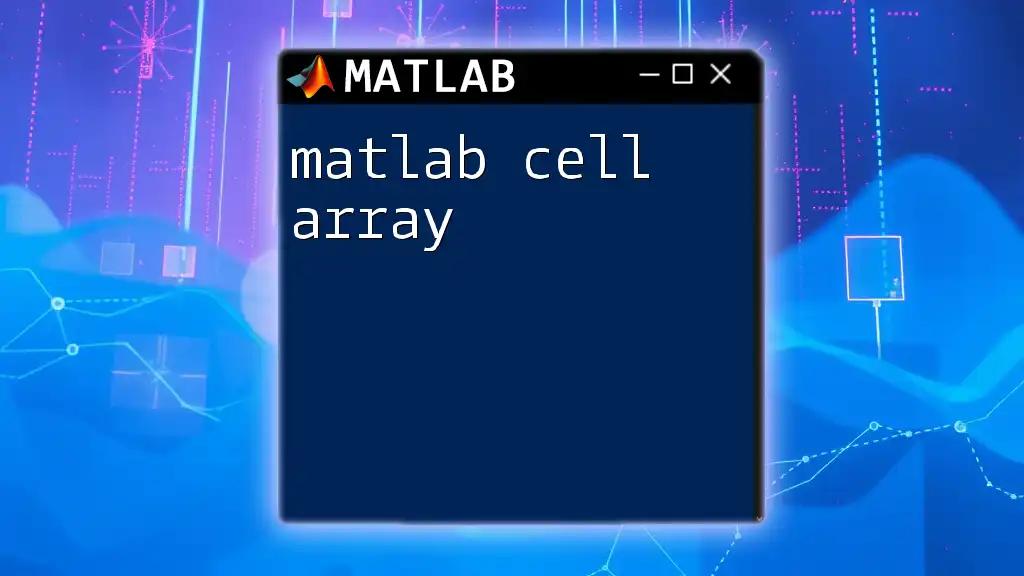
Advanced Techniques with `cellfun`
Combining `cellfun` with Other Functions
`cellfun` can easily be combined with other MATLAB functions to perform more complex operations. For instance, you might want to apply `cellfun` alongside `arrayfun` to process mixed data types or different structures effectively.
Speed and Efficiency Considerations
Using `cellfun` not only optimizes your code but also improves performance. When handling large datasets or complex operations, opt for `cellfun` over traditional loops to save execution time and enhance code clarity.
Dealing with Nested Cell Arrays
When working with nested cell arrays, it may be necessary to apply a function at multiple levels. Use `cellfun` recursively or alongside other functions like `cell2mat` to convert data types as needed. For example:
nestedC = {{1, 2}, {3, 4}};
flattened = cellfun(@(x) cell2mat(x), nestedC, 'UniformOutput', false);
This converts each cell containing arrays into numerical matrices.
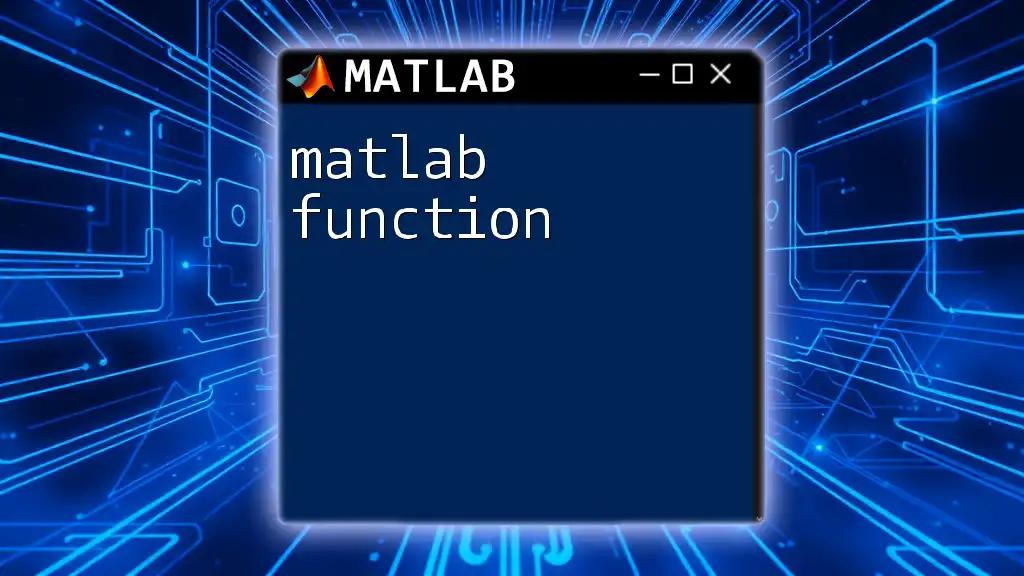
Common Mistakes and Troubleshooting
Handling Errors in `cellfun`
One common issue arises when the function applied via `cellfun` does not return a consistent output format. For example:
C = {'apple', 'banana', 'cherry'};
lengths = cellfun(@length, C);
If `C` contained varying types or sizes, MATLAB would throw an error. Always ensure that your function's output is consistent across the cell array to avoid these pitfalls.
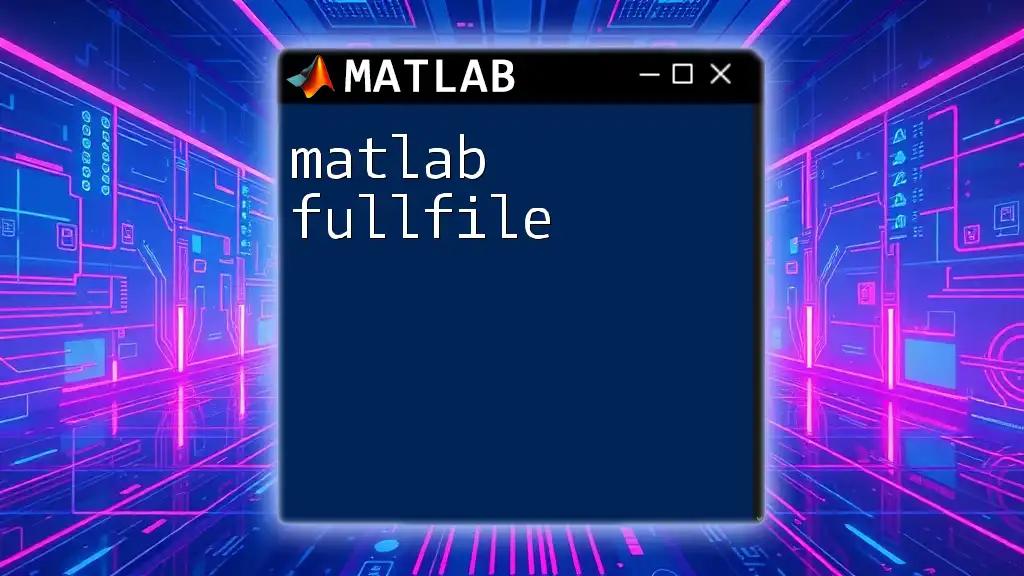
Best Practices for Using `cellfun`
Tips for Writing Readable and Efficient Code
When working with `cellfun`, consider the following best practices:
- Use meaningful function names and comments to clarify complex operations.
- Ensure data types within your cell arrays are compatible with the function being applied.
- When performing operations that might return varied results, set `UniformOutput` to `false`.
When to Opt-Out of `cellfun`
Despite its advantages, `cellfun` may not always be the best option. For highly nested combinations or operations requiring extensive conditional logic, traditional looping structures or vectorization might be clearer.

Conclusion
In summary, `matlab cellfun` is an invaluable tool for efficiently applying functions to cell arrays. By mastering `cellfun`, you unlock a more concise coding style, saving time and enhancing performance in your MATLAB projects. Embrace its potential and explore its capabilities in your own coding practices!
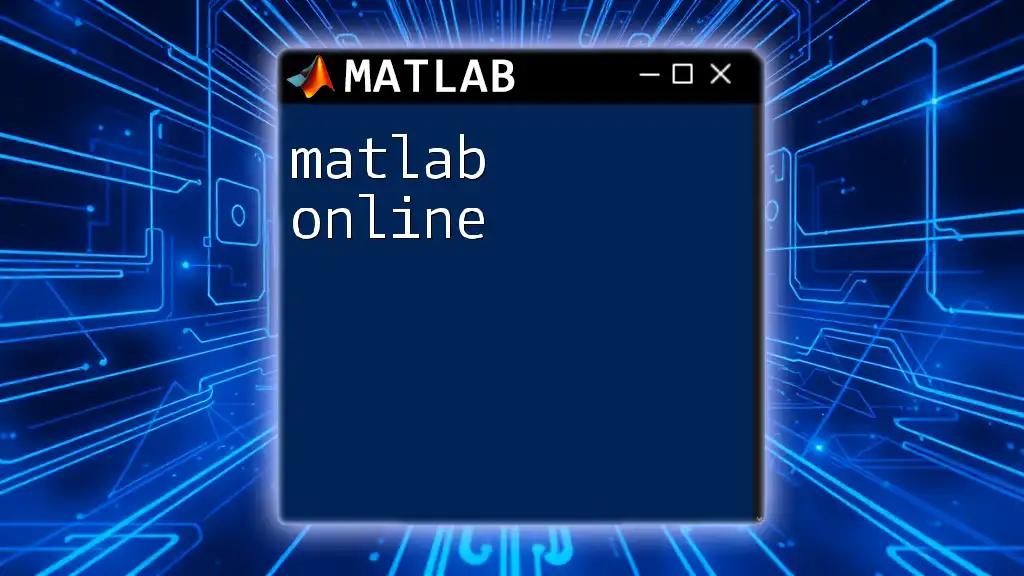
Additional Resources
Recommended Reading and Tutorials
For further exploration, consult the MATLAB documentation related to `cellfun` and cell arrays. Additionally, consider joining MATLAB online forums and communities to deepen your knowledge and skills.
Sample Code Repository
Check for additional downloadable resources or repositories where you can exercise practical examples and further experiment with `cellfun` and other MATLAB features.