The `readtable` function in MATLAB allows you to import data from a file into a table format, making it easy to manipulate and analyze structured data.
data = readtable('filename.csv');
Understanding Tables in MATLAB
What are Tables?
Tables in MATLAB are data containers that organize data in rows and columns similar to a spreadsheet or a database table. Each column can contain data of different types, including numeric, text, or categorical data, making tables a versatile option for managing mixed-type data. They are very useful for data analysis and manipulation because they allow you to easily reference columns by name, improving code readability.
Benefits of Using Tables
Using tables over traditional arrays offers several advantages:
- Improved Readability: Tables provide a clear structure for data, allowing easy identification of variables.
- Flexibility: They can hold varying data types, facilitating complex data analysis.
- Enhanced Functionality: Tables come with built-in functions that simplify data operations, such as sorting and filtering.
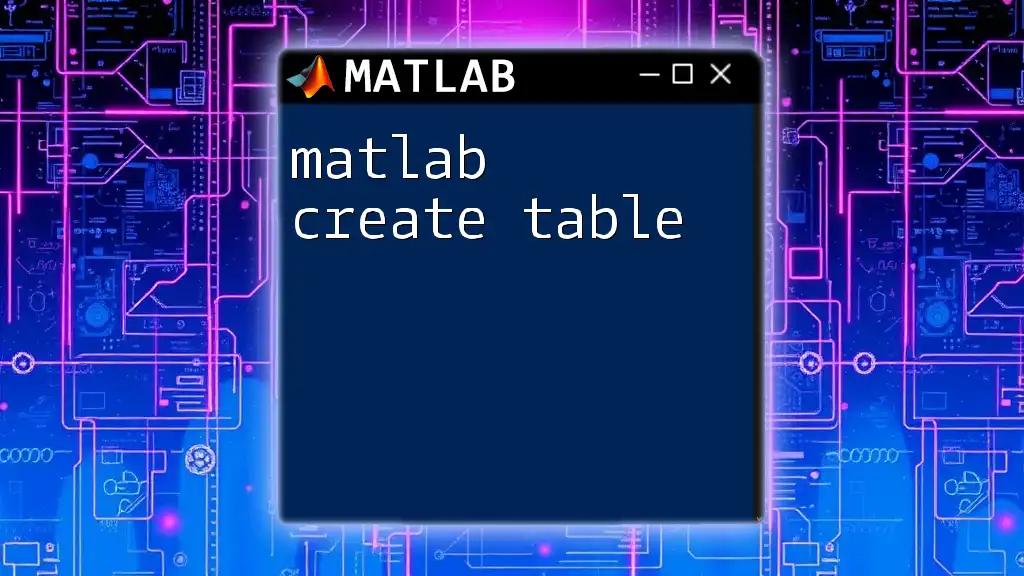
The Purpose of `readtable`
Purpose and Functionality
The `readtable` function is specifically designed to read data from various file formats and load it directly into a MATLAB table. This functionality is crucial for users who need to import large datasets for analysis quickly and efficiently.
File Formats Supported
`readtable` can handle a variety of file formats, including:
- CSV (Comma-Separated Values): Ideal for plain-text data storage with simple structure.
- Excel files: `readtable` can read both .xls and .xlsx formats seamlessly.
- Text files: Any delimited data files, such as tab-separated files, can be read through this function.
- Other delimited data: Files with custom delimiters can also be processed with specific options.
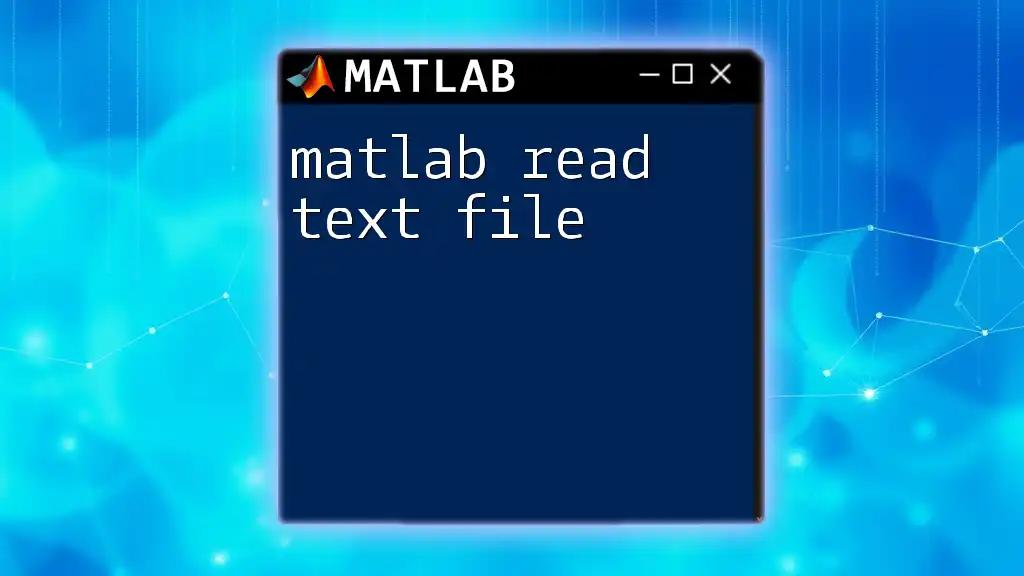
Syntax and Basic Usage of `readtable`
Basic Syntax
The basic syntax of the `readtable` function is as follows:
T = readtable(filename)
In this example, `T` will store the table created from the data in `filename`.
Input Arguments Explained
- `filename`: The path to your data file. This can be a full path or a relative path.
- `opts`: An optional parameter that can be used to specify custom settings for reading the file, such as variable names and data types.
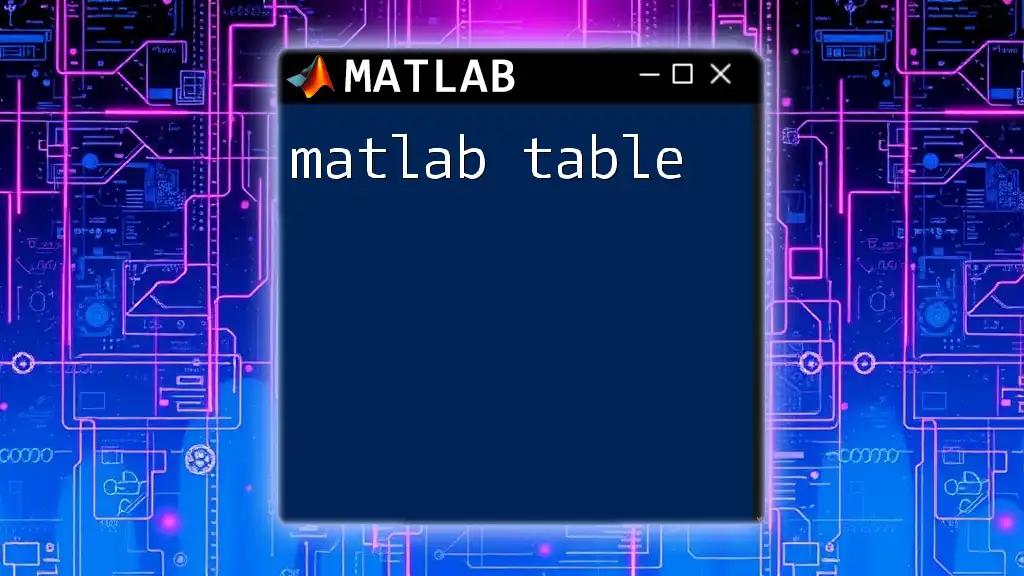
Advanced Usage of `readtable`
Specifying Variable Names and Types
You can manually define variable names and their types while importing data, which is particularly useful for datasets with complex structures. Here’s how to specify variable names when reading a table:
T = readtable(filename, 'VariableNames', {'Var1', 'Var2'}, 'ReadVariableNames', true)
This code will read the data from `filename` and assign `Var1` and `Var2` as the column names, ensuring that you retain meaningful headers for your variables.
Selecting Specific Rows and Columns
If you only need a subset of your data, you can define a specific range of rows and columns to import. For example:
T = readtable(filename, 'Range', 'A1:C10')
This example reads only the first 10 rows and three columns of data from the specified file. This is highly beneficial when dealing with large datasets where you only require a portion of the data.
Handling Missing Data
When dealing with real-world datasets, missing values are common. The `readtable` function allows you to manage these effectively. You can treat specific entries as missing data by using the `TreatAsEmpty` option:
T = readtable(filename, 'TreatAsEmpty', {'', 'NA'})
In this case, both empty strings and the text 'NA' will be interpreted as missing values, enabling MATLAB to represent them correctly within the table structure.
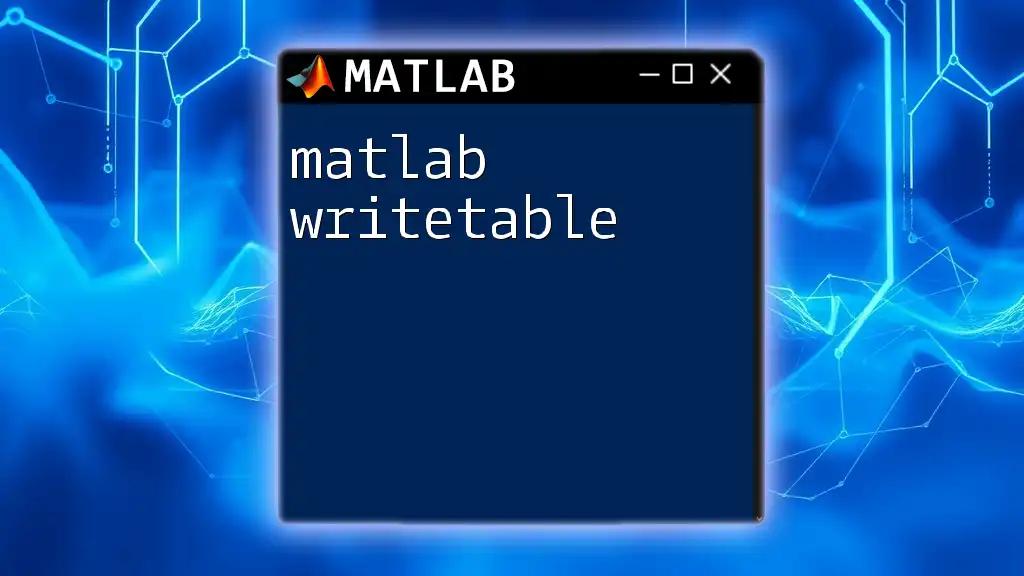
Example Walkthrough
Example Dataset
Suppose you have a CSV file named `sales_data.csv` containing records of sales transactions, with columns for Date, Product, and Revenue. This dataset will serve as an excellent example for demonstrating the use of `readtable`.
Step-by-step Example
To read this dataset into MATLAB using `readtable`, you would execute the following code:
% Read the sales data from the CSV file
T = readtable('sales_data.csv');
disp(T);
This code reads the CSV file and displays the contents of the resulting table, `T`, which now holds all the sales transactions.
Accessing Table Data
Once the data is imported into a table, accessing specific rows and columns becomes straightforward. You can retrieve the first row of the table like this:
firstRow = T(1,:);
To access a particular column, you can refer to it by its variable name:
specificColumn = T.Revenue;
This flexibility makes analyzing and manipulating data much easier.
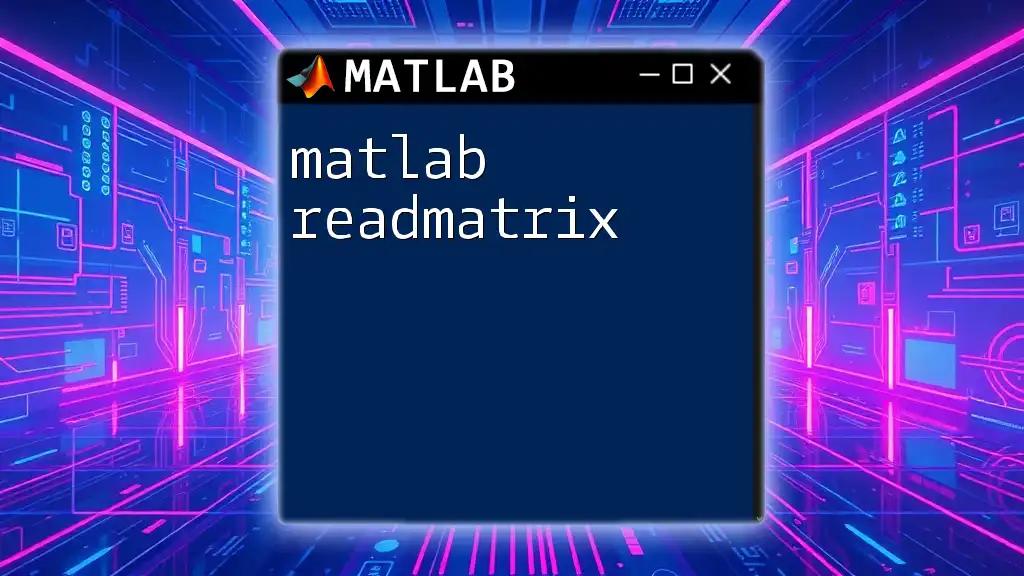
Common Errors and Troubleshooting
Common Issues with `readtable`
When using `readtable`, users may encounter common errors such as:
- File Not Found: Ensure that the file path is correct.
- Inconsistent Delimiters: Make sure that the data file uses a consistent delimiter like commas or tabs.
Debugging Tips
If you run into issues while importing data:
- Check the File Path: Ensure that the filename points to the correct location.
- Inspect File Format: Confirm that the format of your data aligns with the expected format (e.g., CSV, Excel).
- Preview the Data: Use the MATLAB command `type(filename)` in the Command Window to check the first few lines of your dataset.
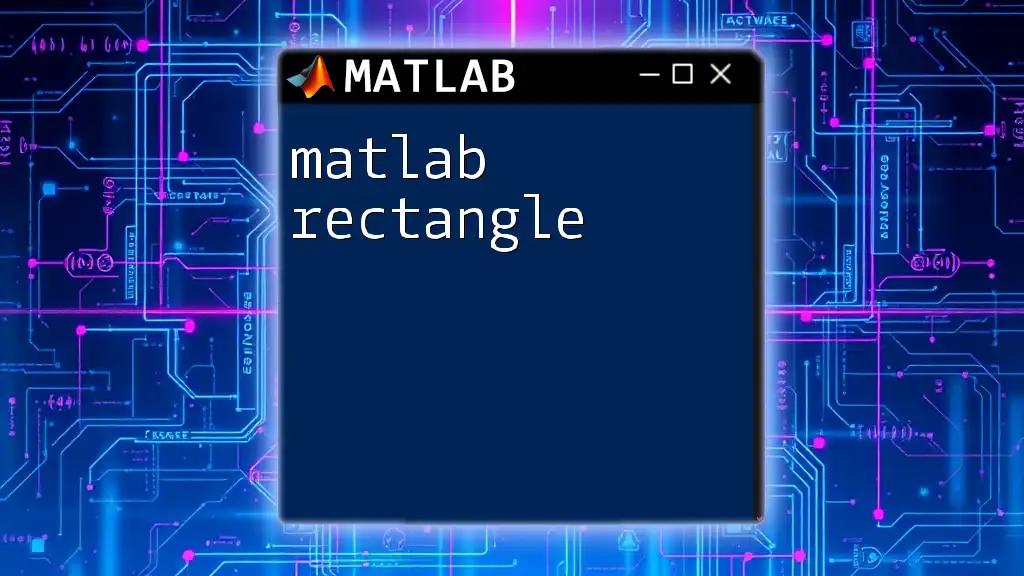
Conclusion
Recap of Key Points
We have explored the `matlab read table` function in depth, demonstrating its functionality and utility in importing and managing data efficiently. We discussed how to handle different file formats, specify variable names, and manage missing data.
Encouragement to Experiment
I encourage you to practice using `readtable` with different datasets to fully grasp its capabilities. Familiarity with this function will significantly enhance your data analysis skills in MATLAB.
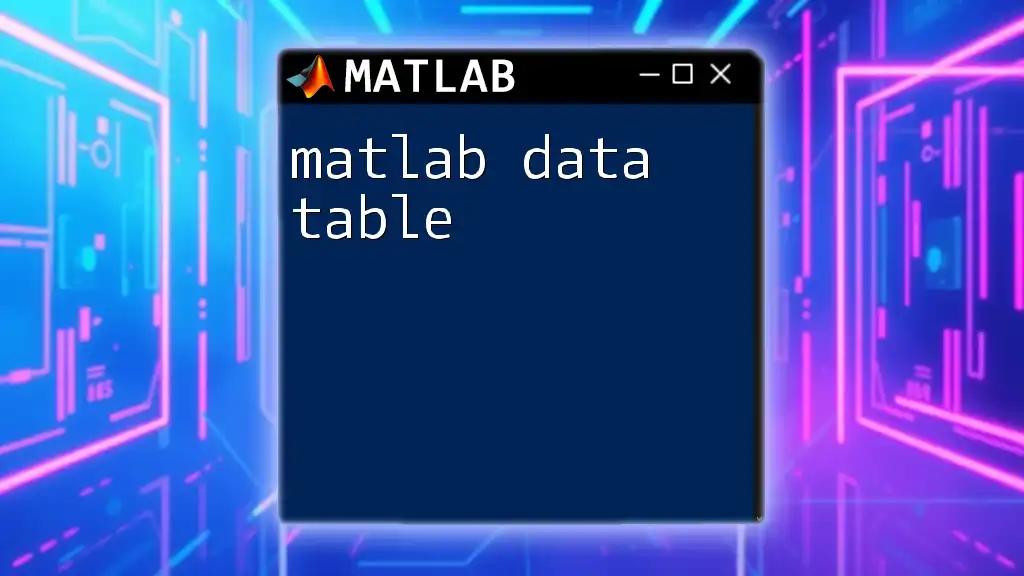
Additional Resources
Official Documentation
For further reading and more advanced options, refer to the official MathWorks documentation on `readtable`.
Video Tutorials and Examples
There are numerous video tutorials available online that can help you visualize the process of using `readtable` in MATLAB.
Community and Forums
Join MATLAB forums and communities such as MATLAB Central for support, discussions, and shared experiences related to the use of `readtable` and other MATLAB features.