The `readmatrix` function in MATLAB reads data from a file and returns it as a matrix, making it easy to import and manipulate data for analysis.
data = readmatrix('datafile.csv');
What is `readmatrix`?
The `matlab readmatrix` function is a powerful tool that allows users to import data from a variety of file types quickly and efficiently. It is particularly essential for anyone involved in data analysis, as it streamlines the process of reading matrices—structuring data into a format that MATLAB can manipulate and analyze.
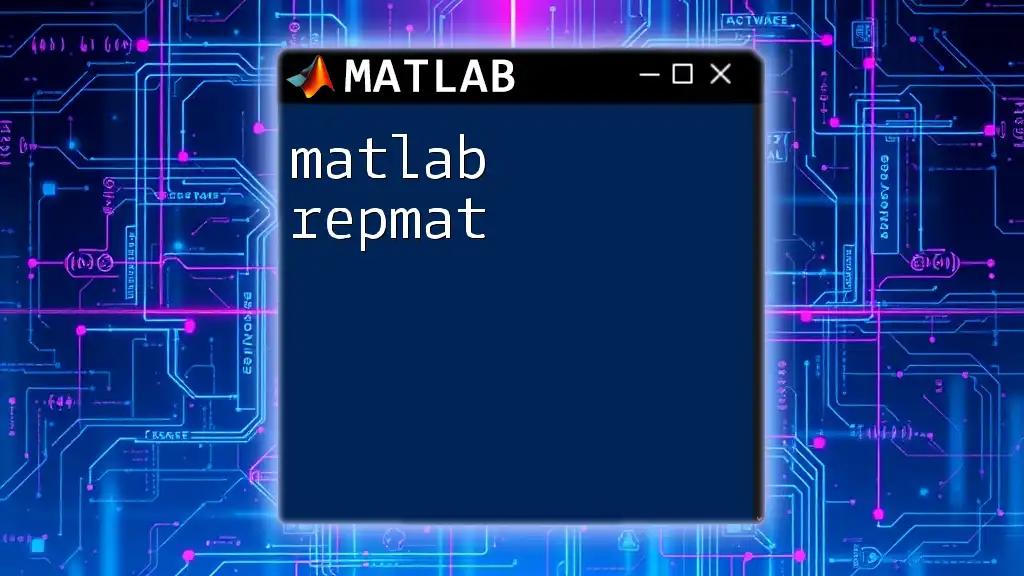
Why Use `readmatrix`?
Using `readmatrix` comes with numerous advantages:
- Versatility: It can handle multiple file formats (e.g., `.csv`, `.xlsx`, `.txt`, etc.), making it suitable for various applications.
- Simplicity: Its straightforward syntax enables quick implementation.
- Performance: Optimized for speed, especially when reading large datasets, which is vital for real-time data analysis and modeling.
Understanding the essence of the `matlab readmatrix` function is key to efficient data handling and analysis.
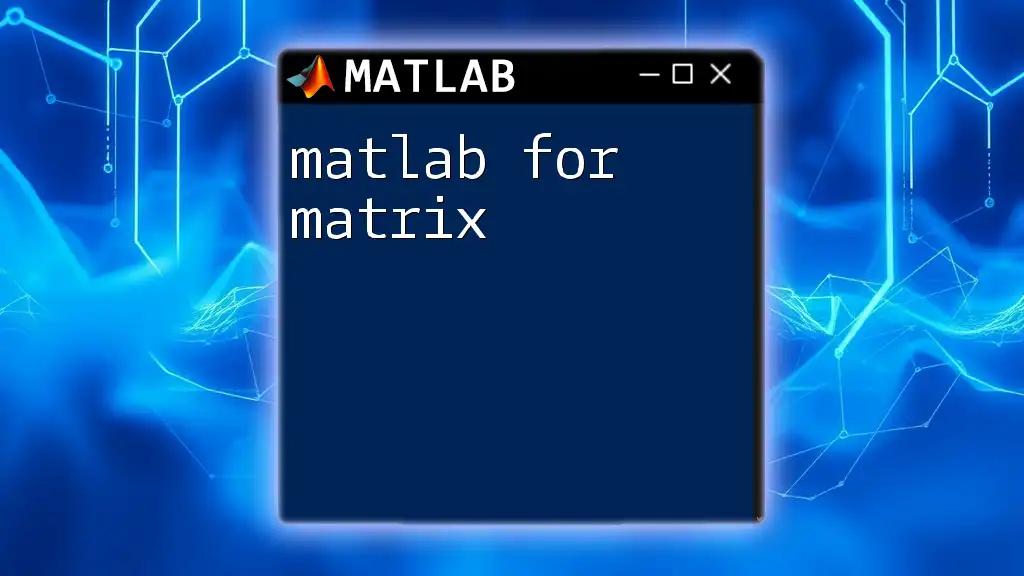
Understanding the Basics of `readmatrix`
Syntax of `readmatrix`
The general syntax for the `readmatrix` function is:
M = readmatrix(filename)
In this syntax:
- M is the matrix that is returned after reading the data from the specified file.
- filename refers to the name of the file being accessed. This can be provided as a string and can include a path if the file is not in the current directory.
Input Arguments
File Name
The filename argument accepts various forms:
- Absolute Path: This includes the entire path to the file, ensuring MATLAB can locate it, regardless of the current working directory.
- Relative Path: If the file is located in the current directory, only the file name and extension are required (e.g., `'data.csv'`).
The `matlab readmatrix` function supports multiple file formats, including:
- Comma-Separated Values (`.csv`)
- Excel Files (`.xlsx`, `.xls`)
- Text Files (`.txt`, `.dat`)
Options Parameter
The Options parameter allows for fine-tuning the importing process. Its common settings include:
- Range: Specifies the subset of the data to read, such as rows and columns.
- Delimiter: Defines how the data is separated (useful for `.txt` files with custom delimiters).
Output
Data Type Returned
The output of `readmatrix` is primarily a numeric matrix; however, if the data contains mixed types, MATLAB will return a cell array. When working with this output, it is essential to understand how MATLAB processes and organizes different types of data during importation, ensuring that analysis is conducted with appropriate data structures.
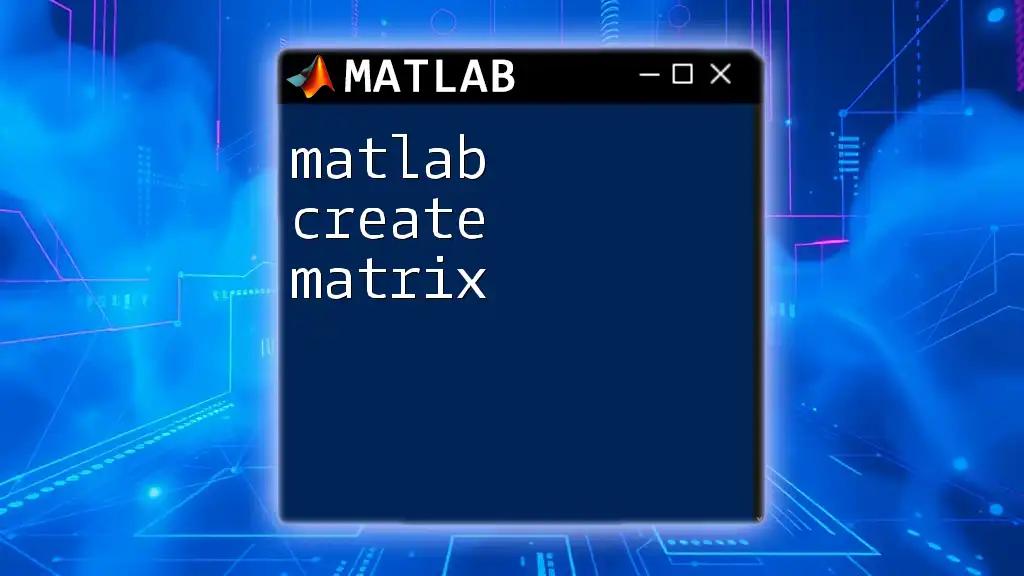
Examples of Using `readmatrix`
Basic Example
A straightforward utilization of `readmatrix` can be demonstrated with a `.csv` file:
data = readmatrix('data.csv');
disp(data);
This example showcases how to read a matrix from a CSV file. The disp function is used to display the data in the command window, facilitating a quick overview of the imported matrix.
Advanced Example with Options
For users needing a more refined approach, here’s an example of importing specific data from an Excel file:
data = readmatrix('data.xlsx', 'Range', 'A1:C10');
disp(data);
In this scenario, the `Range` option limits the import to the first ten rows of columns A to C, making it easier to work with subsets of larger datasets.
Handling Missing Data
Introduction to Missing Data Issues
Missing data is a common issue in real-world data analyses. Efficient handling of these gaps is crucial as they can skew results and lead to inaccurate conclusions.
Using `readmatrix` to Handle Missing Values
You can use `readmatrix` to import a dataset that contains missing values. For instance:
data = readmatrix('data_with_nans.csv');
disp(data);
In this example, any missing entries will be represented as `NaN` (Not a Number) in the matrix. Understanding how MATLAB handles missing data allows for effective preparation and cleaning of datasets prior to analysis.

Additional Features of `readmatrix`
Compatibility with Different Data Formats
The versatility of `matlab readmatrix` is evident in its compatibility with a wide range of formats. This allows users to conveniently import data from various sources without needing to convert files into MATLAB's native format. Whether dealing with `.csv`, `.txt`, or `.xlsx`, `readmatrix` is equipped to handle them all efficiently.
Performance Considerations
When importing large datasets, performance becomes a critical factor. The `readmatrix` function is designed for speed, helping to minimize loading times. To optimize performance:
- Use the Range option to limit the amount of data being imported.
- Familiarize yourself with the structure of the data file to prevent unnecessary processing.
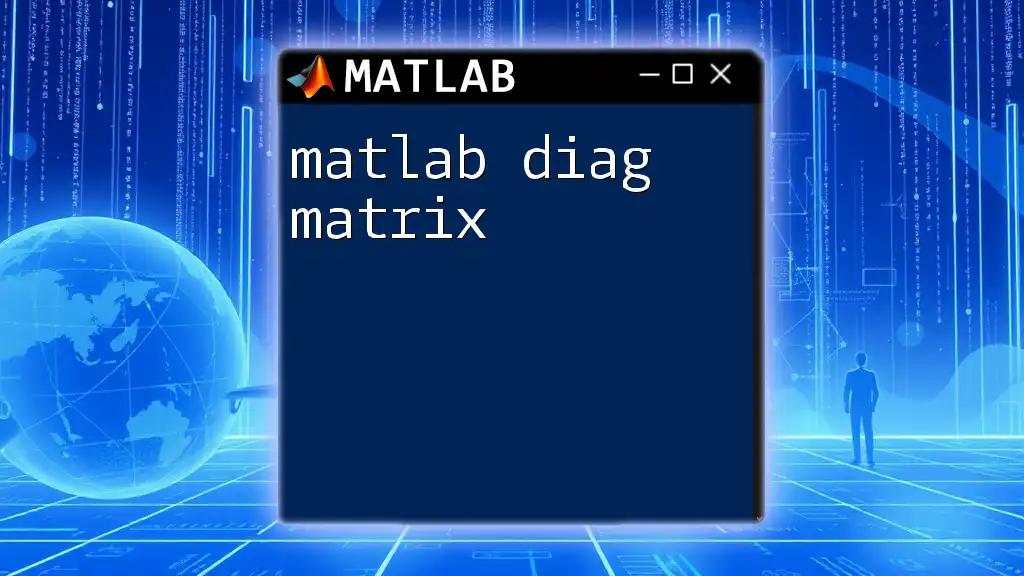
Common Errors and Solutions
Error Handling When Using `readmatrix`
While highly efficient, users may encounter errors such as:
- File Not Found: Ensure that the filename and path are correct.
- Data Mismatch: Verify that the imported data format aligns with expected matrix structures.
Debugging Tips
Implementing robust error handling can prevent runtime issues. Utilizing a `try-catch` block allows you to manage potential errors gracefully:
try
data = readmatrix('data.csv');
catch ME
disp(['Error: ', ME.message]);
end
By incorporating such techniques, users can tackle errors proactively.
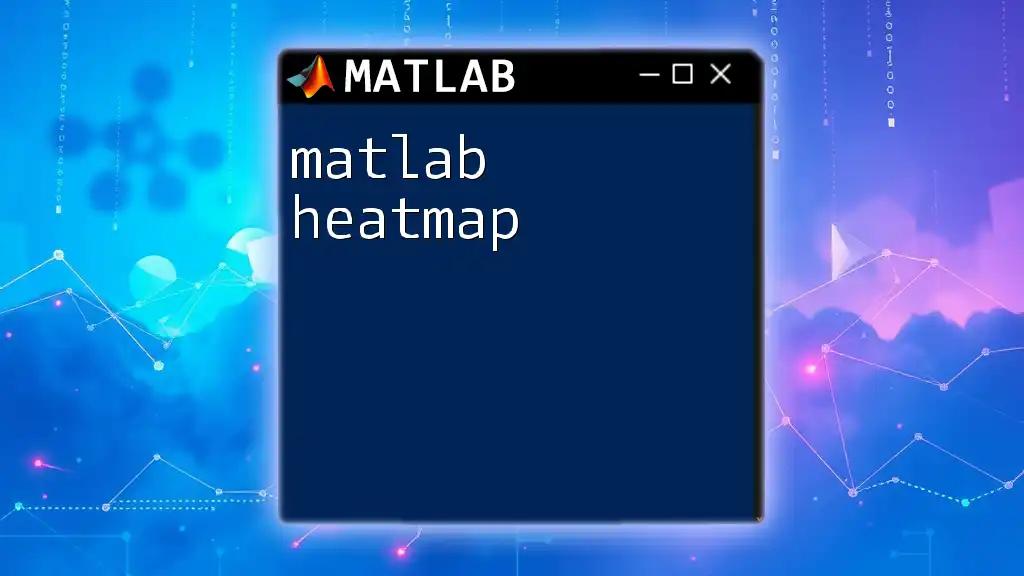
Conclusion
Recap of Key Points
This guide has discussed the utility of the `matlab readmatrix` function, covering its syntax, input arguments, advanced examples, and common pitfalls. Understanding how to effectively use this function empowers users to streamline their data import processes, ensuring better organization and analysis of datasets.
Further Resources
For those looking to deepen their understanding, refer to the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/readmatrix.html) for `readmatrix`. Online tutorials and community forums can also provide additional insights and tips for practical applications.
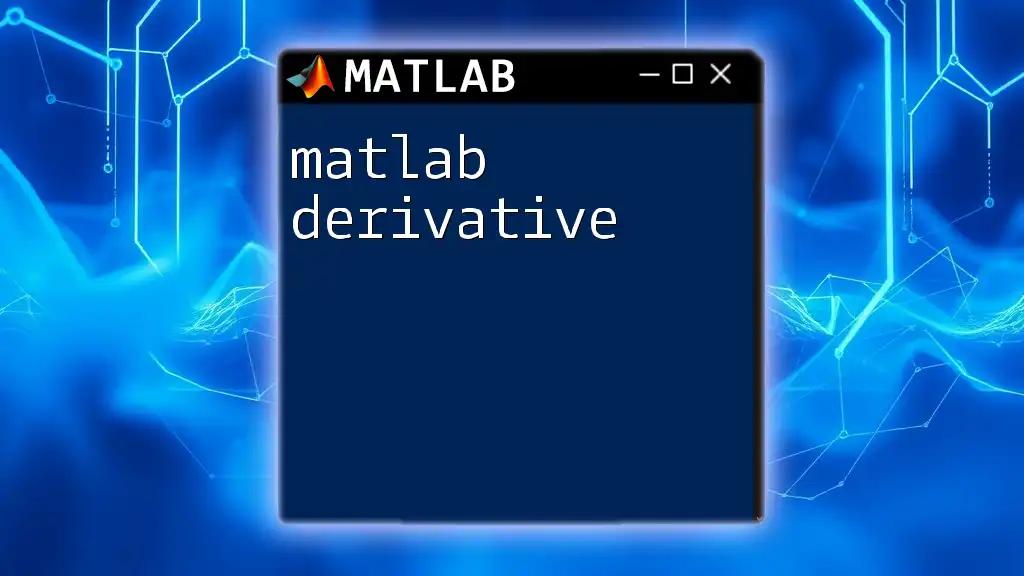
Call to Action
Learn More with Us
If you're eager to enhance your MATLAB skills, consider signing up for our courses designed to teach you how to master commands like `readmatrix`. Stay tuned for our upcoming workshops and seminars, tailored for hands-on experience in using MATLAB effectively!