The `reshape` function in MATLAB allows you to change the dimensions of an array without changing its data, enabling you to reorganize data effortlessly.
A = 1:12; % Create a 1x12 array
B = reshape(A, 3, 4); % Reshape it to a 3x4 matrix
Understanding the Basics of Reshape
What is the `reshape` Function?
In MATLAB, the `reshape` function allows users to change the dimensions of an existing array without altering its data. The command adjusts the organization of the elements, laying them out in the specified dimensions, while preserving the original data order.
Syntax:
B = reshape(A, sz)
Here, `A` represents the input array, and `sz` defines the new dimensions you wish the array to take. It's crucial to remember that the total number of elements in the new dimensions must match that of the original array.
Why Reshape Data?
Reshaping is invaluable in various scenarios, including data preprocessing, reformatting for algorithms, and more.
For example, in machine learning, transforming datasets into the correct shapes for fitting models is essential. Reshaping can also be vital in image processing, where data must be formatted correctly for visual representation or algorithmic processing.
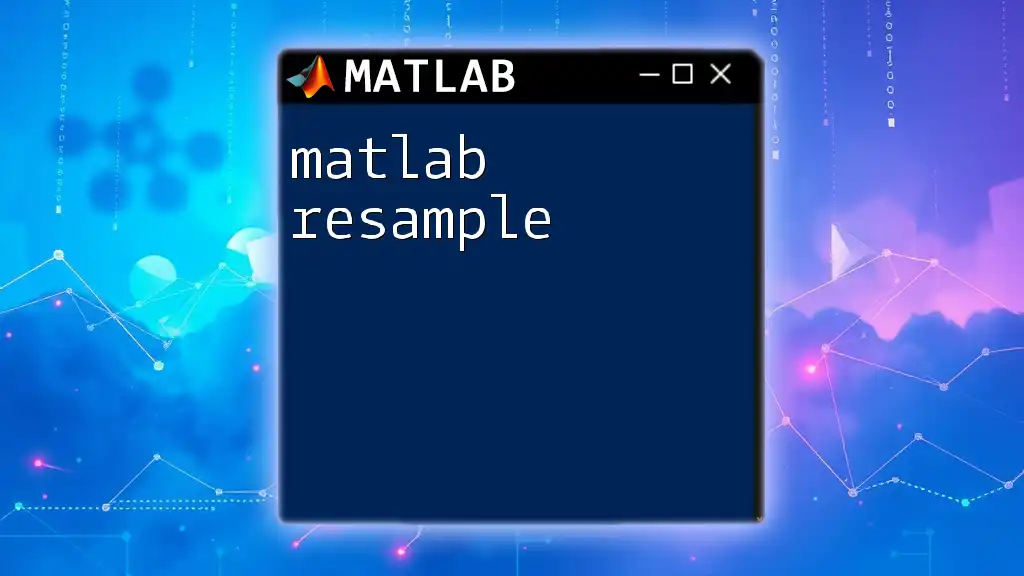
Syntax and Parameters
Basic Syntax
The simplest application of the `reshape` function can be demonstrated with a one-dimensional array that we can reshape into a two-dimensional matrix. For instance:
A = [1, 2, 3, 4, 5, 6];
B = reshape(A, 2, 3); % B will be a 2x3 matrix
In this code, the original vector `A` gets reshaped into a 2-by-3 matrix `B`, where the elements are filled column-wise by default.
Understanding `sz` Parameter
The parameter `sz` is particularly important as it dictates the new shape of the array. When defining `sz`, make sure the product of the specified dimensions equals the number of elements in the original array. If `A` has 6 elements, as in our previous example, `sz` must be a combination like `(2, 3)`, `(3, 2)`, or `(6, 1)`.
Examples of `sz`
Valid examples:
- `reshape(A, 2, 3)` reshapes `A` into a 2-by-3 matrix.
- `reshape(A, 3, 2)` reshapes `A` into a 3-by-2 matrix.
Invalid example:
- `reshape(A, 2, 2)` will produce an error because the new size does not match the total number of elements in `A`.
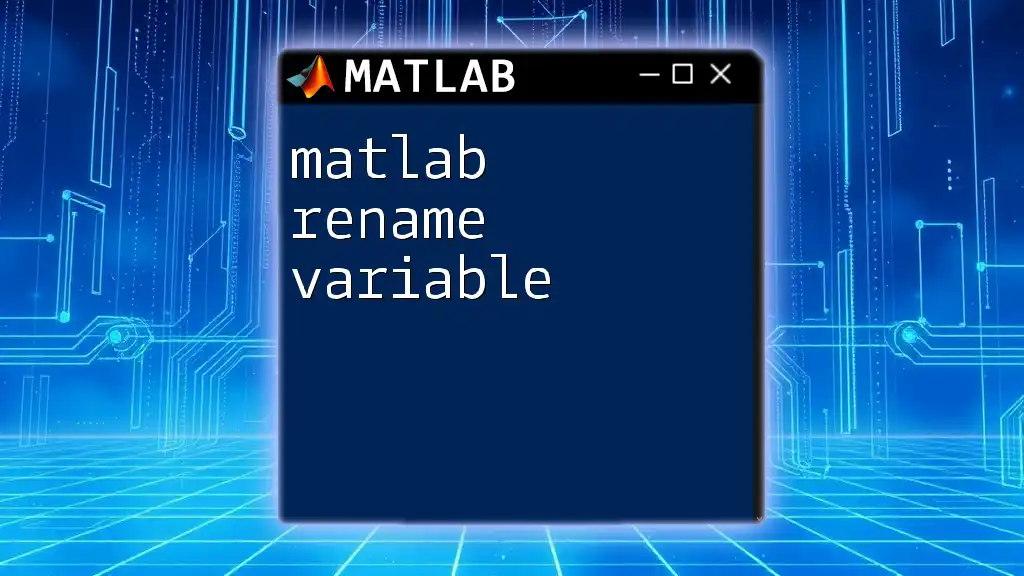
Reshape and Different Data Types
Reshaping Numeric Arrays
`reshape` is most frequently used with numeric arrays. Suppose we have a 2-by-3 matrix:
A_numeric = [1, 2, 3; 4, 5, 6];
B_numeric = reshape(A_numeric, 3, 2);
In this example, `A_numeric` is reshaped into a new matrix `B_numeric`, which is 3-by-2. The order remains column-wise, yielding:
B_numeric = [1, 4;
2, 5;
3, 6]
Reshaping Cell Arrays
MATLAB also allows you to reshape cell arrays. As they can hold varying types of data, reshaping cell arrays is often necessary when organizing mixed datasets. For example:
C = {1, 2; 3, 4; 5, 6};
D = reshape(C, 2, 3);
Here, `C`, a 3-by-2 cell array, gets reshaped into `D`, which is 2-by-3.
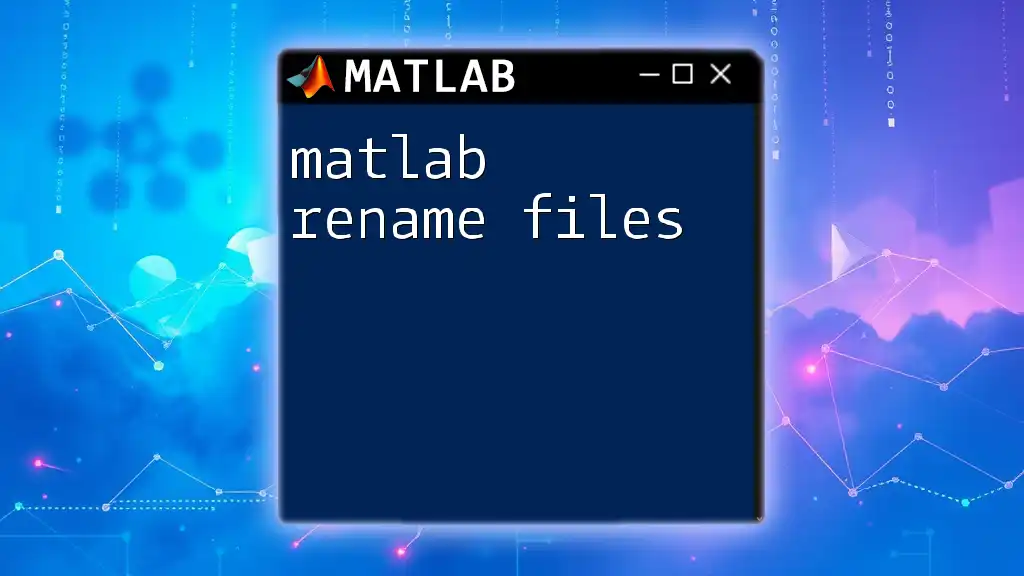
Practical Applications of Reshape
Image Processing
In image processing, an image can be represented as a 3D array (height, width, color channels). Reshaping is frequently needed before applying certain operations, such as flattening an image for machine learning. For instance:
img = imresize(imread('example_image.jpg'), [100, 100]);
reshaped_img = reshape(img, 10000, 3); % Reshaping into a 10,000-by-3 matrix
This transformation enables the pixel data to be prepared in a format suitable for analysis or training algorithms.
Statistical Analysis
When working with statistical models, you may need to transition from a wide format to a long format for analysis. You can easily achieve this through reshaping:
data_wide = [1, 2, 3; 4, 5, 6];
data_long = reshape(data_wide', [], 1); % Convert to long format
Reshaping makes it simpler to apply functions and perform computations across rows or columns.
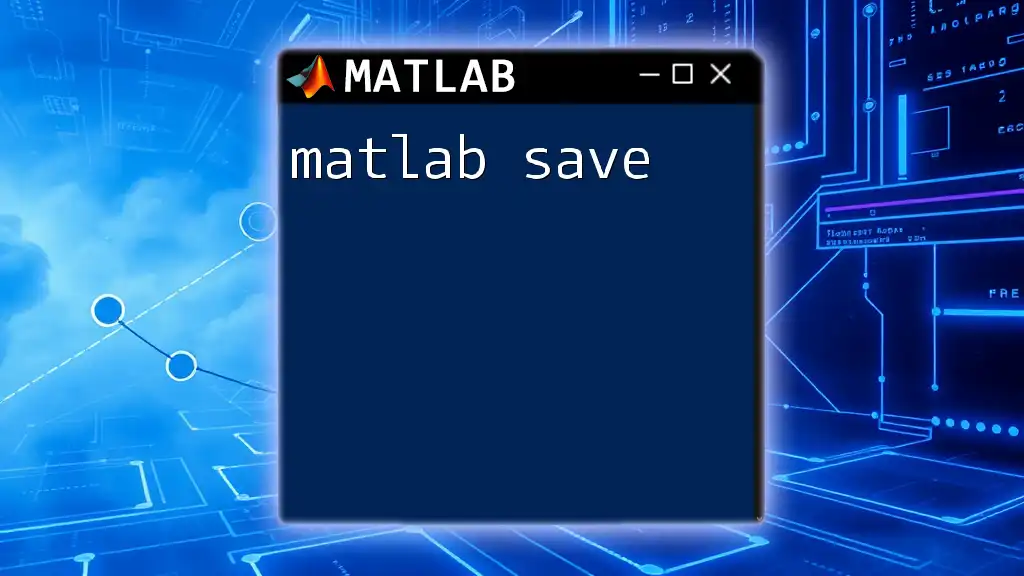
Best Practices for Using Reshape in MATLAB
Ensuring Correct Dimensions
Always verify that the dimensions specified in `sz` align with the total number of elements in the original array. You can check the dimensions using `size(A)`before reshaping to avoid encountering errors.
Performance Considerations
When working with larger datasets, consider memory and computational efficiency. Reshaping very large matrices can be resource-intensive, so ensure that you have optimized your array sizes before performing reshape operations.
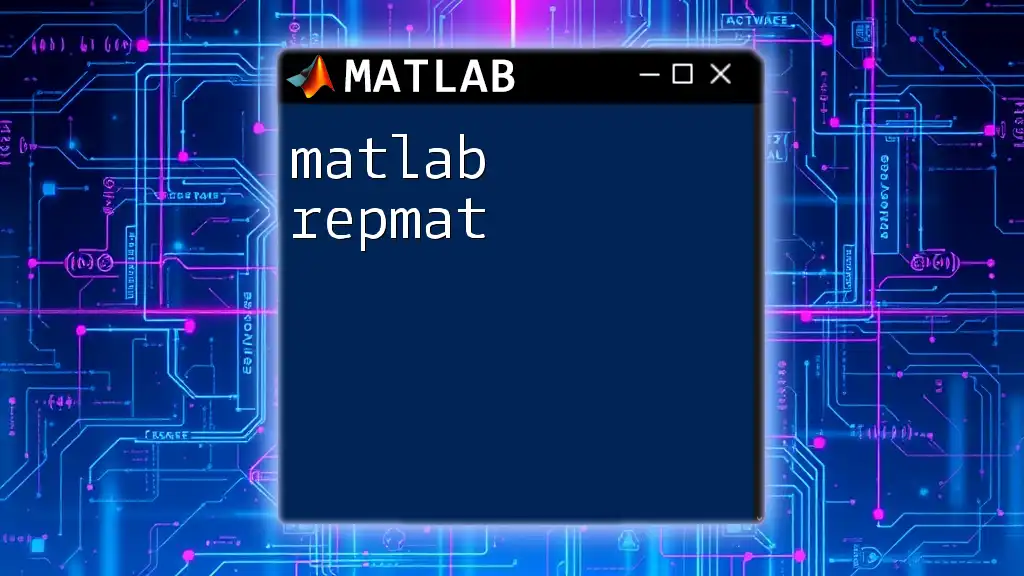
Debugging Common Issues
Identifying Errors with Reshape
Errors often arise from dimension mismatches. If the total number of elements does not match the desired shape, you will see an error message like "Error using reshape: To RESHAPE the number of elements must not change."
Troubleshooting Tips
If you encounter errors, always start by double-checking your `sz` parameter. Use the `numel()` function to ensure it equals the product of your new dimensions. For example:
num_elements = numel(A); % Total elements in A
expected_elements = prod([2, 3]); % Should equal num_elements for reshape to succeed
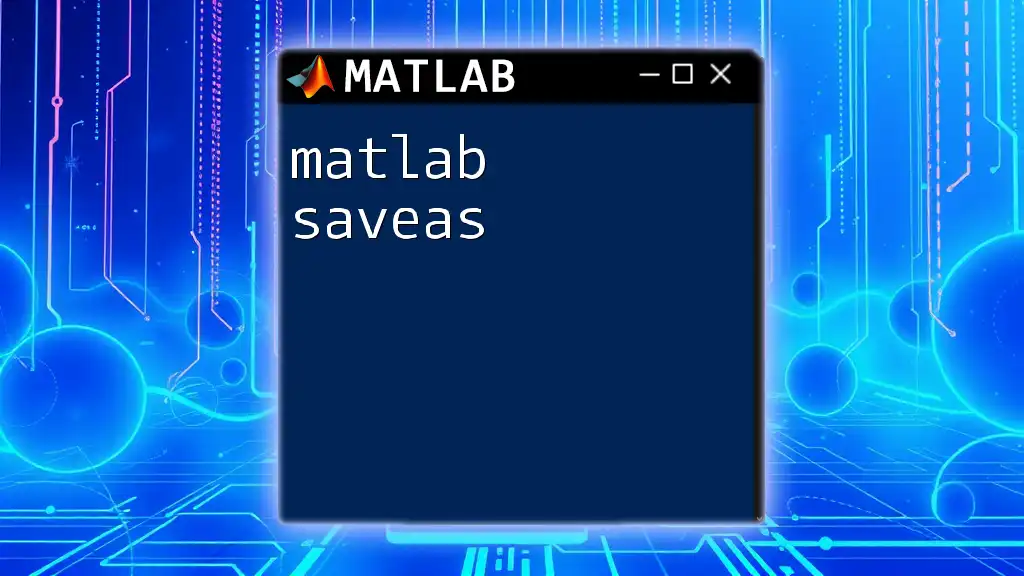
Summary
The `matlab reshape` command is a fundamental tool for manipulating arrays effectively. Remember that reshaping is not about changing data but rather about organizing it in a more functional manner. Practicing with different arrays and reshaping methods will significantly enhance your data manipulation skills in MATLAB.
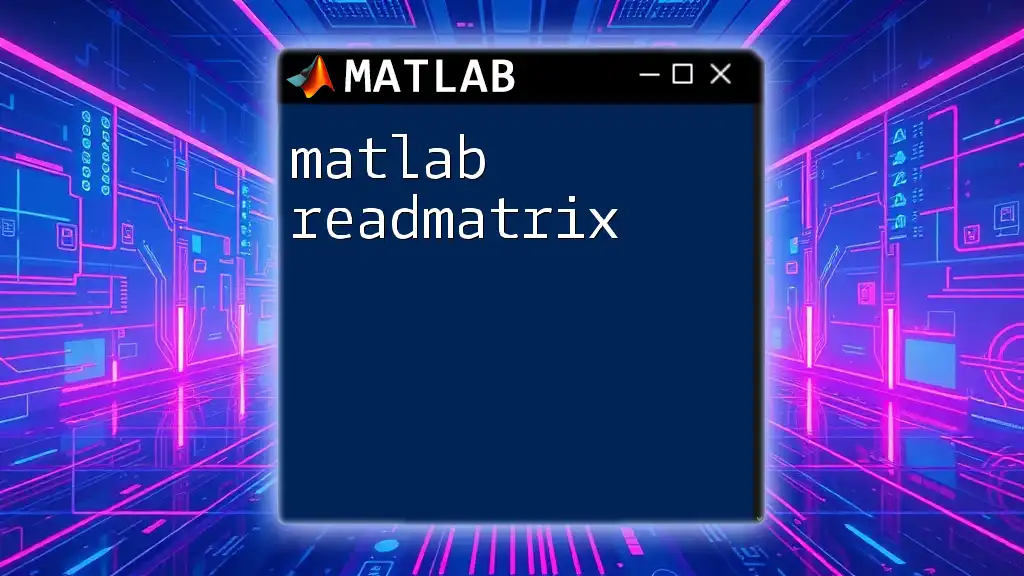
Additional Resources
Reference Documentation
For comprehensive information, consult the official MATLAB documentation on `reshape`. It details not just the syntax but also advanced functionalities and examples.
Tutorials and Further Reading
To deepen your understanding, consider exploring tutorials and online courses that address MATLAB programming, focusing on matrix manipulations and common commands. Books specifically about MATLAB for data analysis can also provide valuable insights.
Community and Support
Engaging with the MATLAB community through forums and discussion groups can provide additional support and inspiration. This networking can amplify your learning experience and present new ways to utilize the `reshape` function effectively.