MATLAB sorting allows you to arrange the elements of an array in ascending or descending order efficiently.
Here’s a basic code snippet to demonstrate sorting in MATLAB:
A = [5, 3, 8, 1, 4];
sortedA = sort(A); % Sorts A in ascending order
Understanding MATLAB Arrays
Types of Arrays in MATLAB
MATLAB provides different types of arrays, each of which is used for specific purposes.
1D Arrays are the simplest form, consisting of a single row or column of elements. They are often used for storing lists of numbers or simple datasets. For instance, creating a numeric array can be done using the following command:
data = [5, 3, 9, 1, 6];
2D Arrays are essentially matrices, and they are commonly used in MATLAB for performing algebraic operations, data representation, and image processing. An example of creating a 2D array is:
matrixData = [4, 2; 3, 5; 1, 4];
Cell Arrays are versatile containers that allow you to store different types of data in an array format, making them useful for managing complex datasets. Example:
cellArray = {1, 'text', [1, 2, 3]};
Creating Arrays
To effectively work with sorting, it’s crucial to know how to create various arrays. The structure and type of an array will often determine which sorting method you can use.
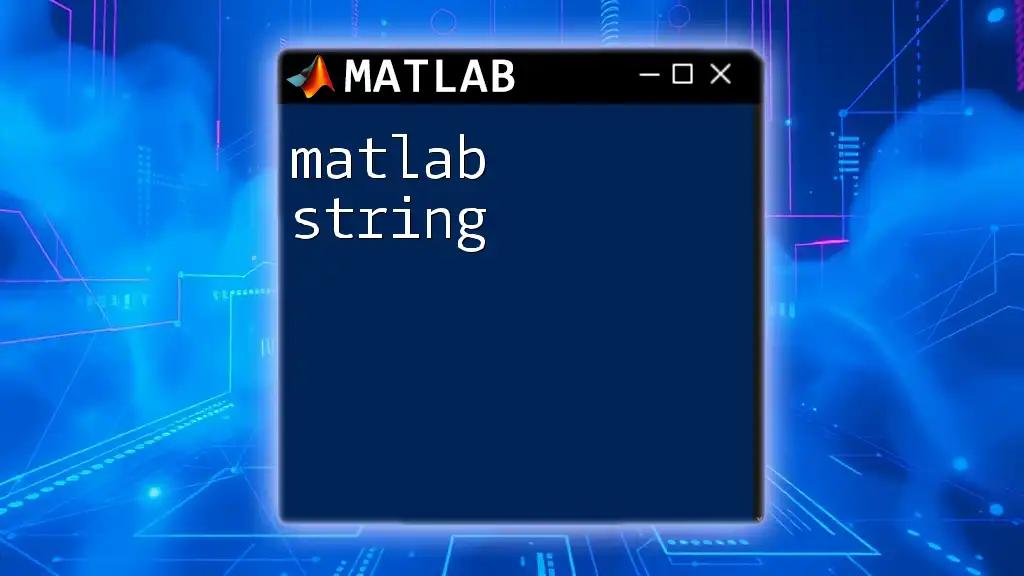
Basic Sorting Functions
sort Function
The primary function for sorting in MATLAB is the `sort` function. This powerful function allows you to sort arrays in ascending or descending order based on the user’s requirement. Its syntax is straightforward:
sortedData = sort(data); % Default is ascending
After executing the above code, the `sortedData` will yield `[1, 3, 5, 6, 9]`. If you want to sort in descending order, you can specify the second argument:
descendingData = sort(data, 'descend');
Here, the output will be `[9, 6, 5, 3, 1]`. Understanding the sort order is essential for data analysis.
sortrows Function
For 2D arrays, the `sortrows` function becomes invaluable. It allows you to sort an entire matrix based on one or more columns. The syntax is as follows:
sortedMatrix = sortrows(matrixData);
If you want to sort based on a specific column, for example, the second column:
sortedMatrix = sortrows(matrixData, 2);
This method is particularly useful when dealing with datasets that include multiple attributes and you need to sort by a specific field.
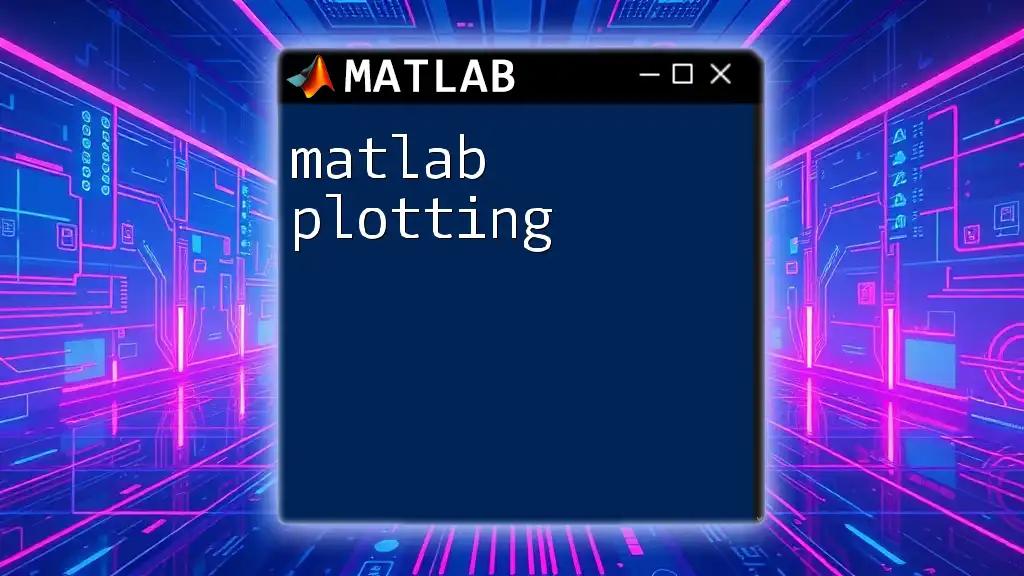
Advanced Sorting Techniques
Custom Sorting with Function Handles
In some cases, the built-in sorting methods may not suffice. MATLAB allows for more advanced control through custom sorting using function handles. Here's how to use it:
dataSorted = sort(data, 'ComparisonMethod', 'Real');
By employing custom comparison methods, you can dictate how the elements should be compared, providing flexibility in sorting beyond the default settings.
Using the 'stable' Option
Another important concept in MATLAB sorting is stability. A sorting algorithm is said to be stable if it maintains the relative order of equal elements. You can enable this in MATLAB using the `'stable'` flag:
stableSortedData = sort(data, 'ComparisonMethod', 'stable');
This ensures that if there are two elements of equal value, their original order remains unchanged in the sorted output, which is valuable for datasets where order is significant.
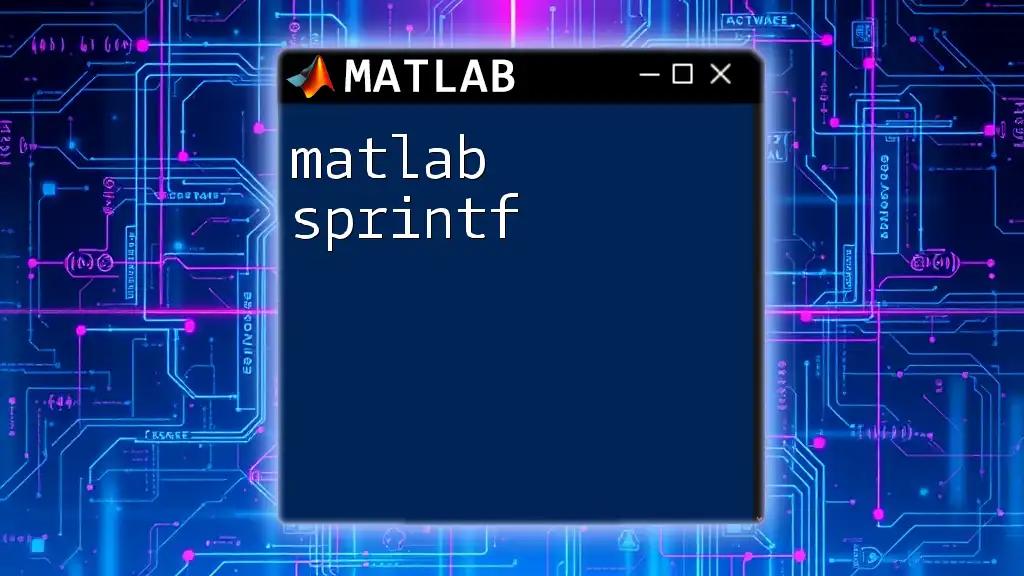
Sorting Structures and Cell Arrays
Sorting Fields in Structures
Sorting arrays of structures is straightforward but requires a custom function. For instance, consider the following array of structures:
structArray = struct('name', {'Alice', 'Bob', 'Clara'}, 'age', {34, 23, 29});
To sort these structures by the field `age`, you would create a sorting function:
function sortedStructs = sort_struct(structArray, field)
[~, idx] = sort([structArray.(field)]);
sortedStructs = structArray(idx);
end
Using this custom function allows for a flexible approach to sort based on desired attributes.
Sorting Cell Arrays
Sorting cell arrays is slightly different as they may contain different data types. MATLAB handles this efficiently. Here's an example on how to sort a cell array of strings:
cellArray = {'Banana', 'Apple', 'Grapes'};
sortedCell = sort(cellArray);
This will return the sorted array `{'Apple', 'Banana', 'Grapes'}`. Sorting cell arrays is useful, particularly when working with categorical data.
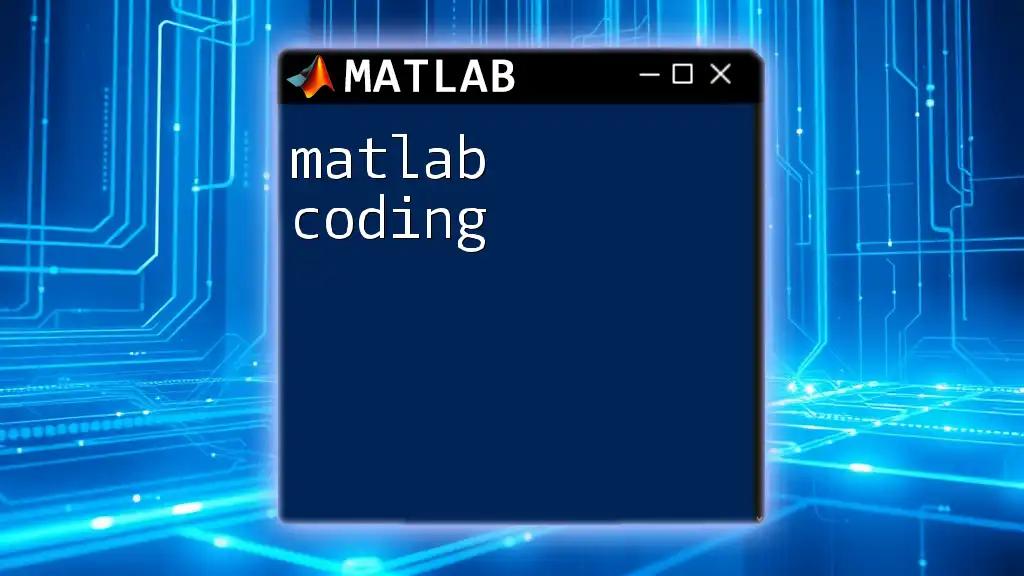
Handling Complex Data Structures
Sorting Tables
As datasets grow more complex, MATLAB tables provide a more structured format for data analysis. When you want to sort a table based on a specific column, the `sortrows` function is still applicable:
T = table({'Alice'; 'Bob'; 'Clara'}, [34; 23; 29], 'VariableNames', {'Name', 'Age'});
sortedTable = sortrows(T, 'Age');
The output will yield a table sorted by age, maintaining readability and ease of access to the data you need.
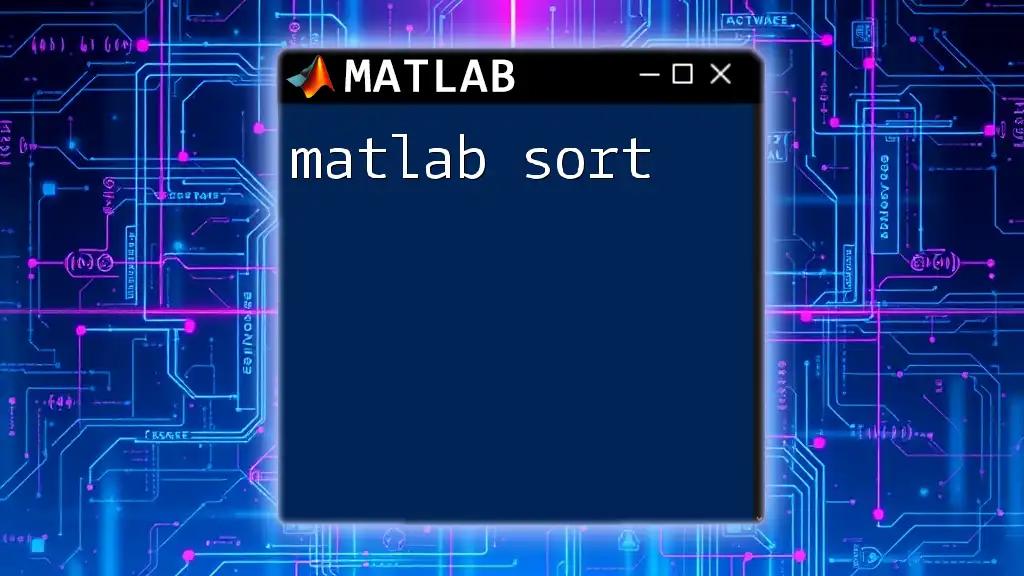
Conclusion
In this comprehensive guide to MATLAB sorting, we have explored the various techniques available to organize and manipulate your data effectively. By understanding the basic and advanced sorting methods, custom sorting functionalities, and handling complex data structures, you can make great strides in your data analysis tasks.
Experimenting with these techniques will not only bolster your programming skills but also enhance your efficiency in data management and analysis in MATLAB. Embrace the power of sorting to unlock new insights from your data and improve your overall productivity.