In MATLAB, a string is a sequence of characters enclosed in single quotes, which can be manipulated and concatenated for various purposes such as displaying messages or storing data.
str = 'Hello, MATLAB!';
disp(str);
What is a String?
A string in programming is a sequence of characters used to represent text data. Understanding strings is crucial for data processing, as they allow user input, text manipulation, and most importantly, the handling of data in a more human-readable format. In MATLAB, there are two main types of strings: character arrays (enclosed in single quotes) and string arrays (enclosed in double quotes). While both serve similar purposes, they differ in functionality.
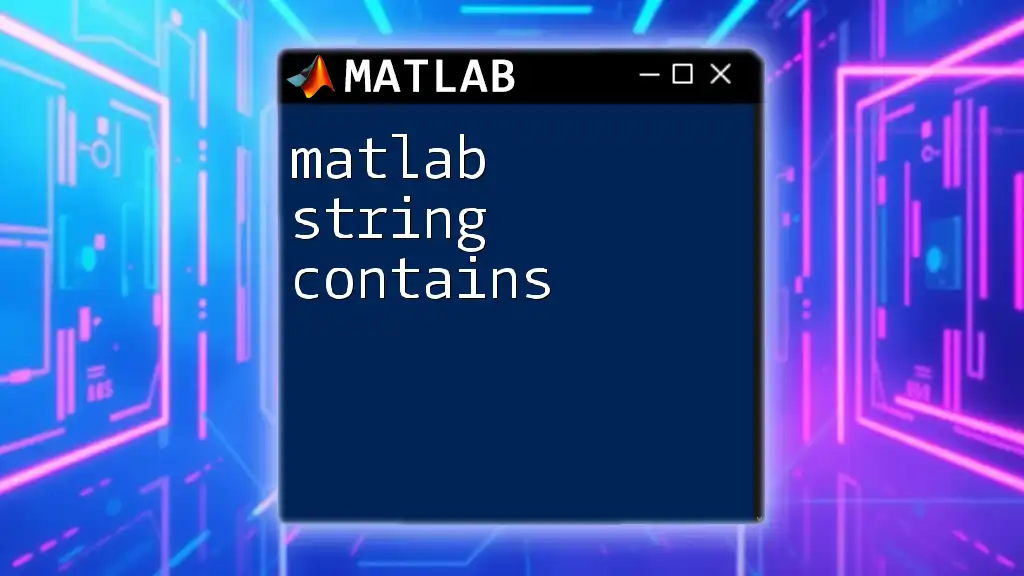
Creating Strings in MATLAB
Creating strings in MATLAB can be done in a straightforward manner. You can denote a string using either double quotes or single quotes, although the functionality differs slightly between the two.
-
Using double quotes: When you create a string with double quotes, you create a string array. This format is more versatile and is recommended for new code.
str1 = "Hello, World!";
-
Using single quotes: This method creates a character array. While it can be used for simpler tasks, it is generally less flexible than using string arrays.
charArray1 = 'Hello, World!';
Understanding these distinctions will help you choose the appropriate format based on your programming needs.
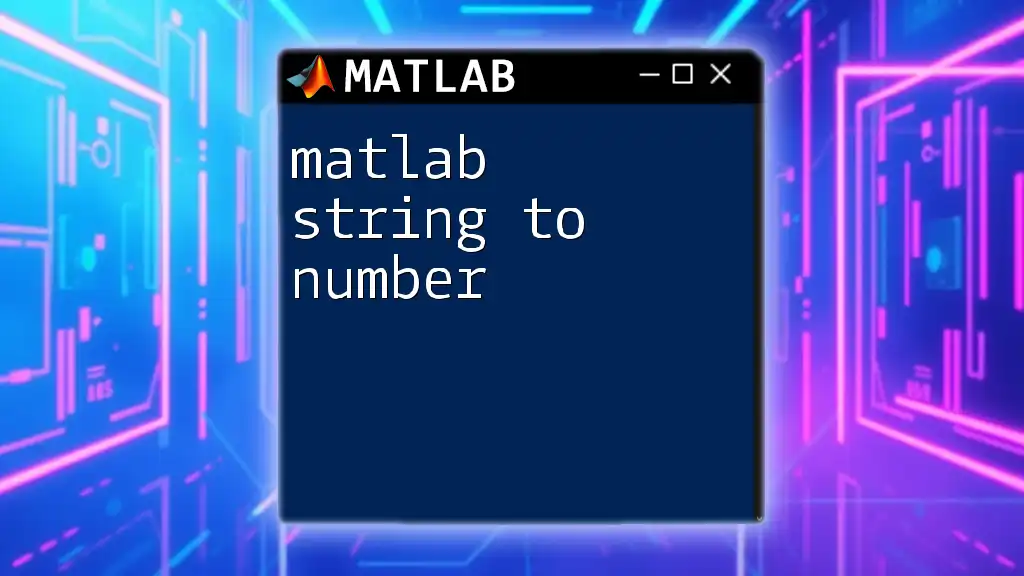
String Manipulation Functions
Concatenation of Strings
One of the most common operations you’ll perform with strings is concatenation, which combines multiple strings into one. MATLAB offers several ways to do this.
-
Using the `+` operator: This operator allows for simple and intuitive string concatenation.
str2 = "Hello," + " World!";
-
Using the `strcat` function: This function provides an alternative method for concatenation, primarily useful for combining string arrays.
concatStr = strcat("Hello,", " World!");
Both methods yield the same result; you can choose according to your preference.
Accessing Characters in Strings
Sometimes you may want to access individual characters in a string. MATLAB utilizes 1-based indexing, meaning the first character is accessed with the index `1`.
-
Example of indexing: To get the first character of `str1`, you can do the following:
firstChar = str1(1); % Returns 'H'
This ability to index directly into strings provides flexibility and control when manipulating string data.
Modifying Strings
Strings in MATLAB can also be modified easily. For instance, if you want to change a specific character within a string, you can directly assign a new character to that index.
-
Changing specific characters:
str1(1) = 'h'; % Changes "Hello" to "hello"
This functionality makes it easy to update strings in various applications.
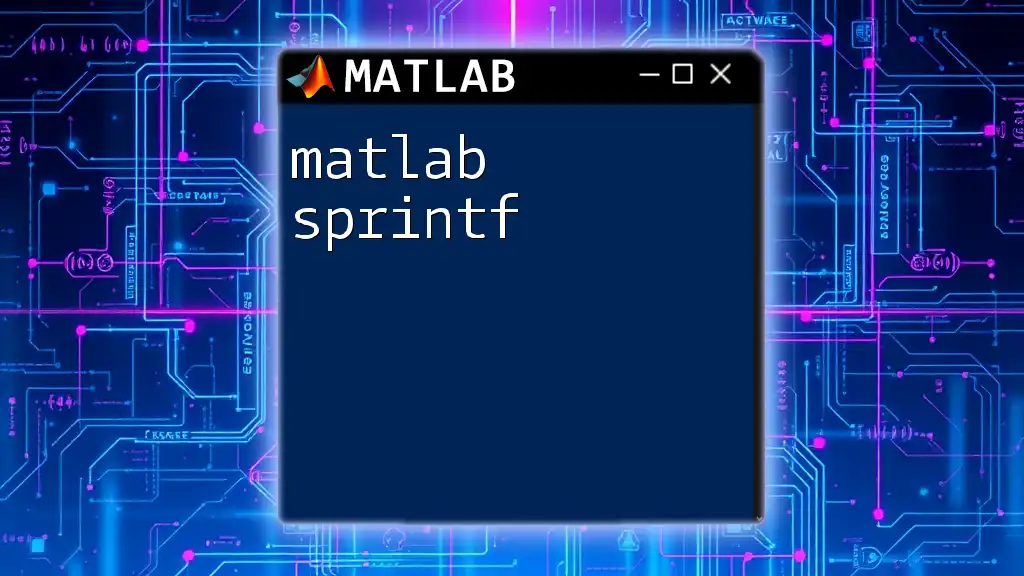
Common String Functions
Finding Length of Strings
Knowing the length of a string can be essential in many cases, such as validating input sizes or processing data.
-
Using the `strlength` function: This function computes the length of a string array.
len = strlength(str1); % Returns the number of characters in str1
This simple function can help inform you about your string data.
Searching and Replacing Substrings
Manipulating substrings can be vital when assessing or reorganizing text data.
-
Using `contains` allows you to check if a substring exists within a string.
hasHello = contains(str1, "Hello"); % Returns true or false
-
Using `replace`: If you need to modify parts of a string, this function is invaluable.
newStr = replace(str1, "World", "MATLAB"); % Changes "World" to "MATLAB"
These functions make string processing tasks straightforward and efficient.
String Comparison
Comparing strings helps you determine if two texts are equivalent, an essential operation in various programming contexts.
-
Using `strcmp`: This function checks if two strings are exactly equal.
isEqual = strcmp(str1, charArray1); % Returns true or false
-
Using `strcmpi`: For case-insensitive comparisons, you can use this function.
isEqualIgnoreCase = strcmpi(str1, "hello, world!"); % Ignores case
Employing string comparisons helps manage and validate your data effectively.
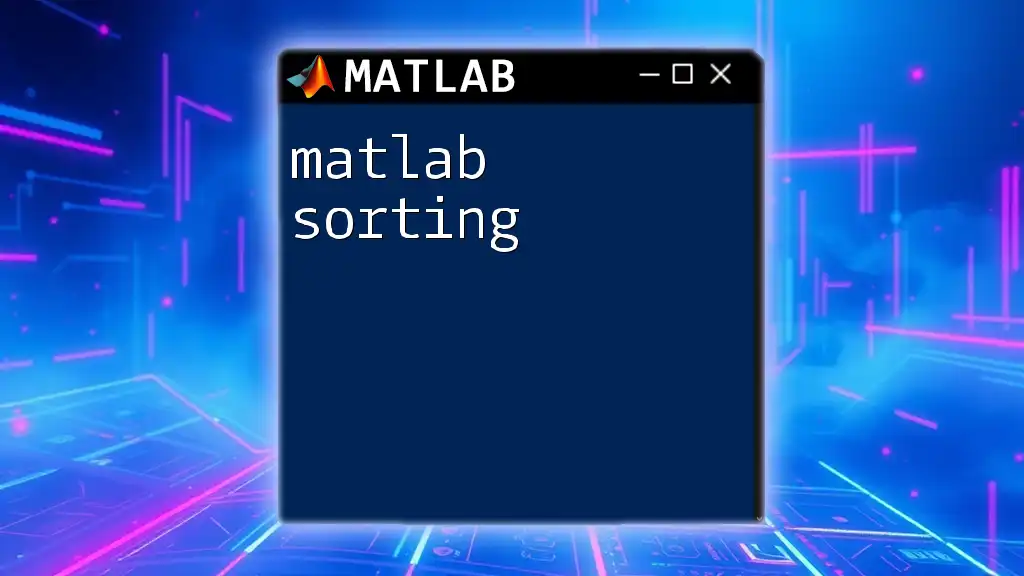
Advanced String Operations
String Formatting
Formatting strings allows you to create more visually appealing and informative outputs in scripts.
-
Using `sprintf`: This function enables you to format strings with variables.
formattedStr = sprintf('The value of pi is approximately %.2f', pi);
This feature is particularly useful for generating reports or presenting results neatly.
Converting Between Types
Sometimes you'll need to convert between strings and numbers for calculations.
-
Converting strings to numbers:
numStr = "123.45"; num = str2double(numStr); % Converts the string to a double
-
Converting numbers to strings:
num = 123.45; strNum = string(num); % Converts the number to a string
Understanding these conversions is crucial for data manipulation and analysis.
Splitting Strings
When you need to break down a string into individual components, splitting can be quite handy.
-
Using `split`: This function divides a string based on specified delimiters.
myString = "MATLAB is great for data analysis"; parts = split(myString); % Splits at spaces
This is useful for parsing user input or file data.
Joining Strings
You might find occasions where you need to consolidate several strings into one. The `join` function allows for this effectively.
-
Using `join`: To concatenate a cell array of strings with a specified delimiter.
stringArray = ["MATLAB", "is", "fun!"]; joinedStr = join(stringArray, " "); % Joins with spaces
This is particularly useful when you have multiple strings stored in arrays.
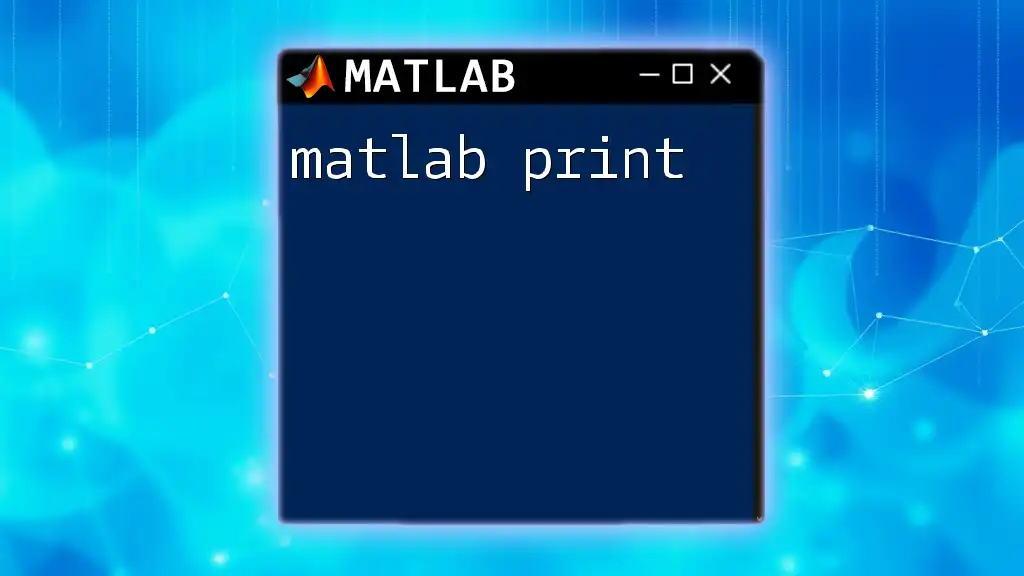
Practical Applications of Strings in MATLAB
Example 1: Formatting Output in Scripts
Utilizing strings effectively can enhance the output of scripts. For instance, by using formatted strings with `fprintf`, you can make your results clearer and more informative.
fprintf('The result of the calculation is: %f\n', result);
Example 2: User Input Handling
When developing applications, you often need to gather user input as strings. The `input` function makes this straightforward.
userStr = input('Please enter your name: ', 's');
This convenience facilitates interaction with users and enhances the overall experience.
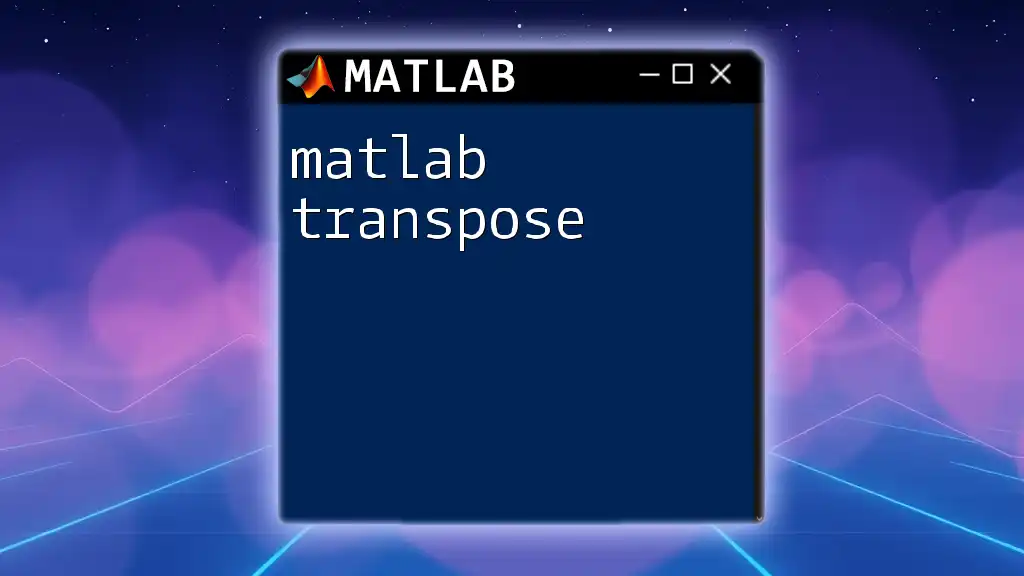
Recap of Key Points
Throughout this guide, we’ve explored the versatility and functionality of MATLAB strings. Understanding how to create, manipulate, and analyze strings opens the door to more dynamic programming.
Further Learning Resources
To deepen your understanding of MATLAB strings, consider exploring official documentation, online communities, and tutorial series focused on programming with MATLAB.
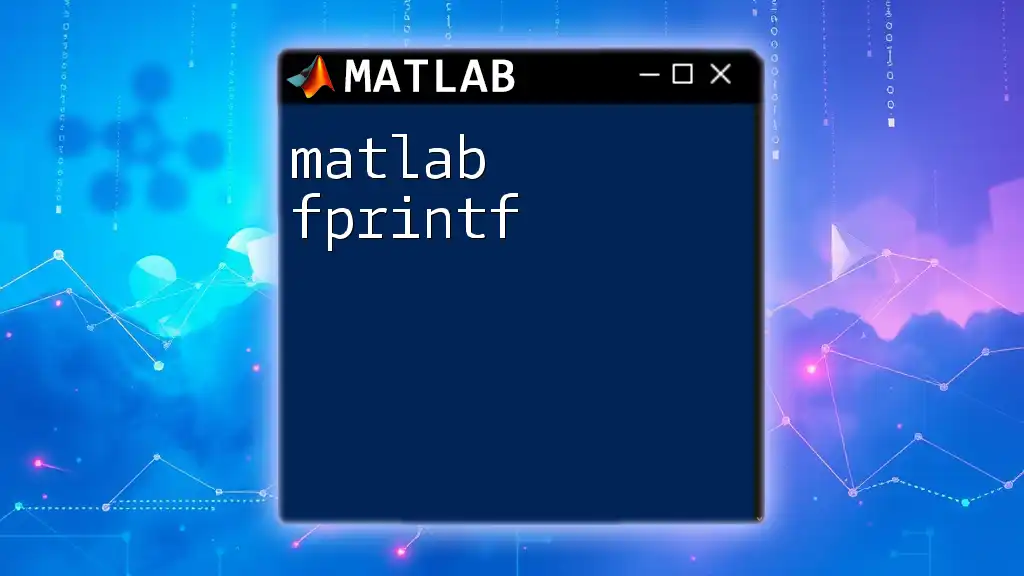
Call to Action
We encourage you to practice these concepts with examples and share your experiences or questions in the comments. Engaging with the MATLAB community can accelerate your learning and offer new insights!