The `sprintf` function in MATLAB formats data into a string according to specified formatting rules, making it useful for creating strings with embedded variable values.
str = sprintf('The value of pi is approximately %.2f', pi);
What is `sprintf`?
`matlab sprintf` is a powerful function used for generating formatted strings. It allows you to create text that includes variables neatly incorporated into specific formats, making it easier to communicate complex data visually. This function stands out among string manipulation tools in MATLAB due to its versatility and precision.
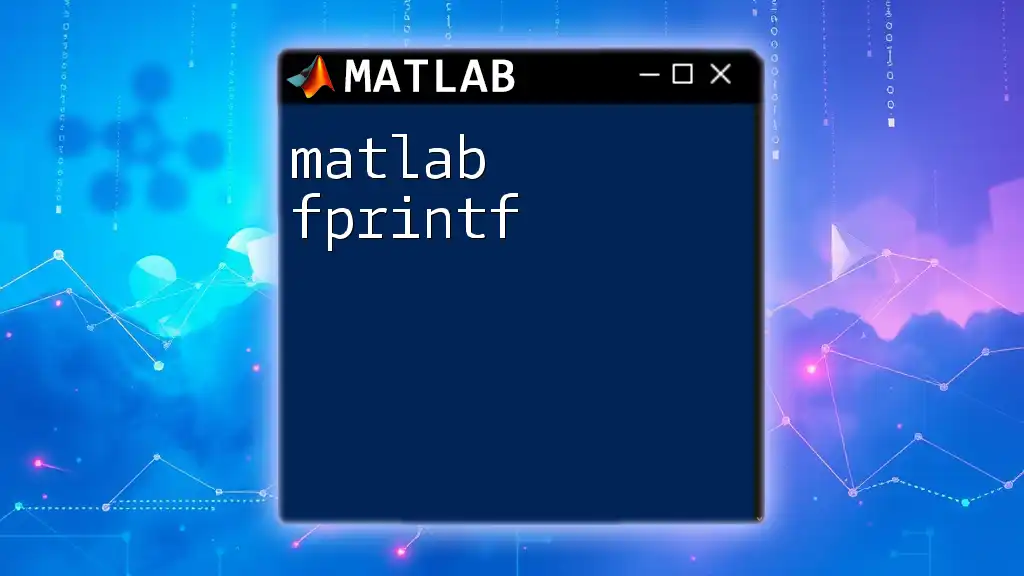
Why Use `sprintf`?
Using `sprintf` provides several advantages over other string functions such as `fprintf`, especially when you need to create strings for display or storage instead of printing directly. Here are a few scenarios where `sprintf` is particularly useful:
- Concise Formatting: You can easily format numbers, strings, and other data types in a single command.
- Improved Readability: By clearly defining how data appears in a string, you can enhance the interpretability of output messages or logs.
- String Manipulation for Outputs: It allows you to generate strings that can be stored in variables rather than displaying them directly, which is beneficial for dynamic outputs.
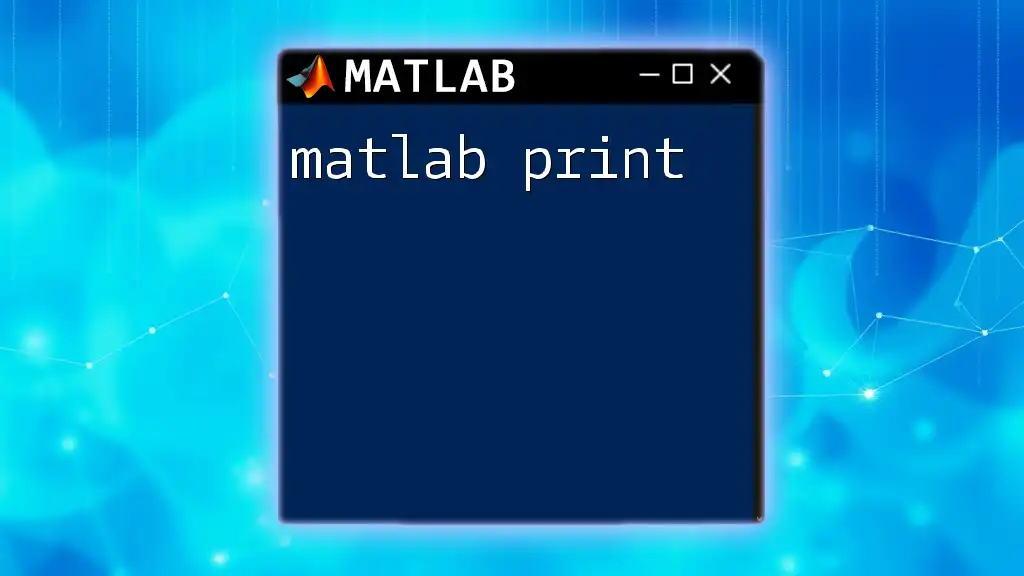
Function Syntax
The basic syntax of `sprintf` is:
sprintf(formatSpec, A1, A2, ..., An)
- `formatSpec`: A string that defines how subsequent arguments are formatted.
- `A1, A2, ..., An`: The data to be formatted according to the specifications in `formatSpec`.
Understanding how to structure these parameters is fundamental for effectively using `sprintf`.
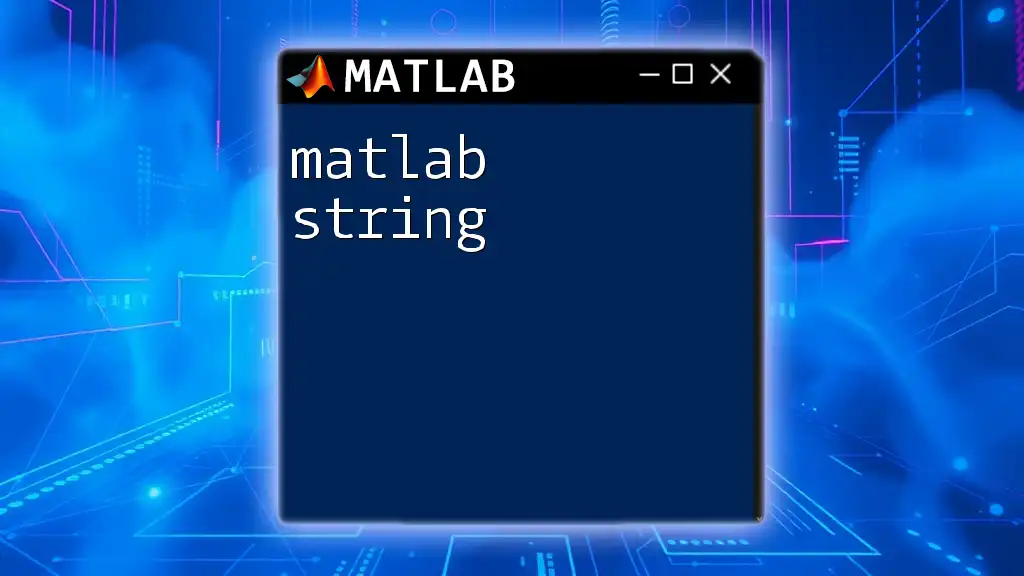
Format Specifiers
Format specifiers instruct MATLAB on how to present data types in the output string. Here are some common specifiers:
- `%d`: Formats an integer.
- `%f`: Formats a floating-point number (you can specify precision, such as `%.2f` for two decimal places).
- `%s`: Formats a string.
As an example, consider the following code snippet, which illustrates using a format specifier for floating-point precision:
str = sprintf('The value of pi is approximately %.2f.', pi);
In this case, `%.2f` formats the value of π to two decimal places, resulting in the string "The value of pi is approximately 3.14."
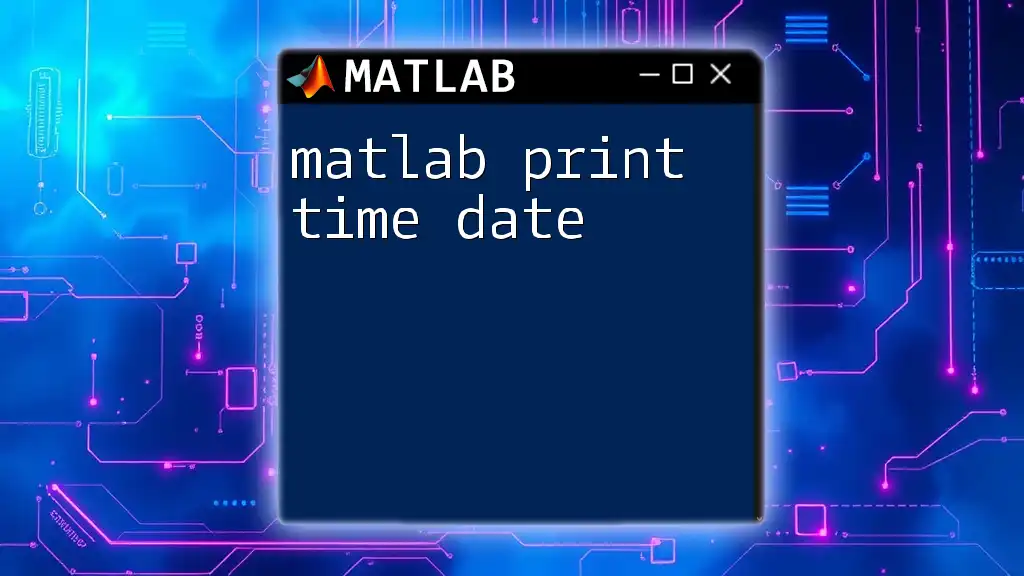
Combining Multiple Variables
One of the strengths of `sprintf` is its ability to handle multiple variables in a single call. Below is an example demonstrating this functionality:
x = 42;
y = 3.14159;
str = sprintf('The values are x: %d and y: %.2f.', x, y);
In this string, `sprintf` combines both an integer and a float, effectively generating the output: "The values are x: 42 and y: 3.14."
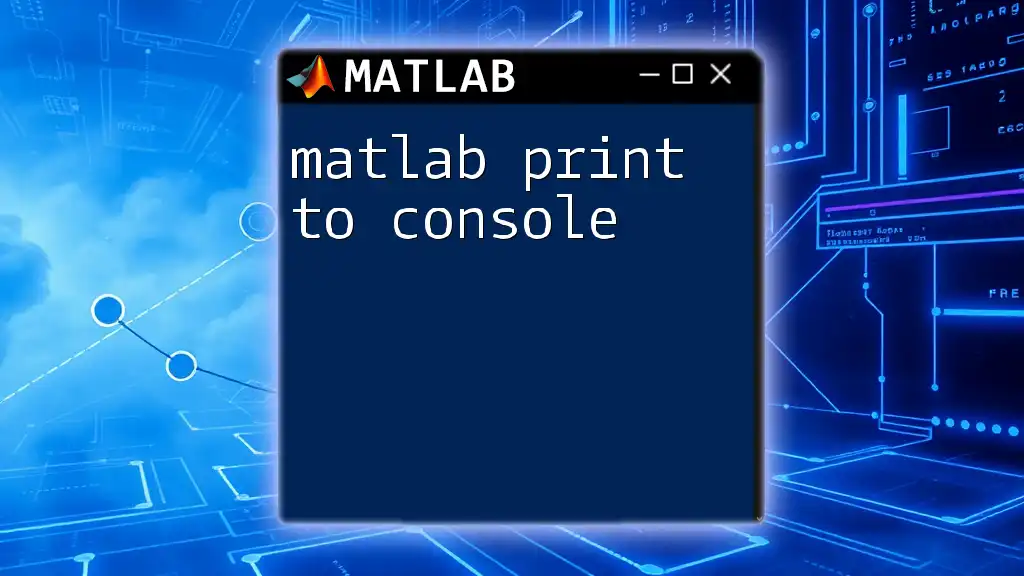
Formatting Arrays and Matrices
You can also utilize `sprintf` to format arrays or matrices. When formatting matrices, remember that `sprintf` does not automatically format multi-line outputs. Here’s how you can handle it:
A = [1, 2; 3, 4];
str = sprintf('Matrix element: %d ', A);
In this example, `sprintf` processes each element of the matrix `A`, returning a string with each matrix element included sequentially, resulting in "Matrix element: 1 2 3 4".
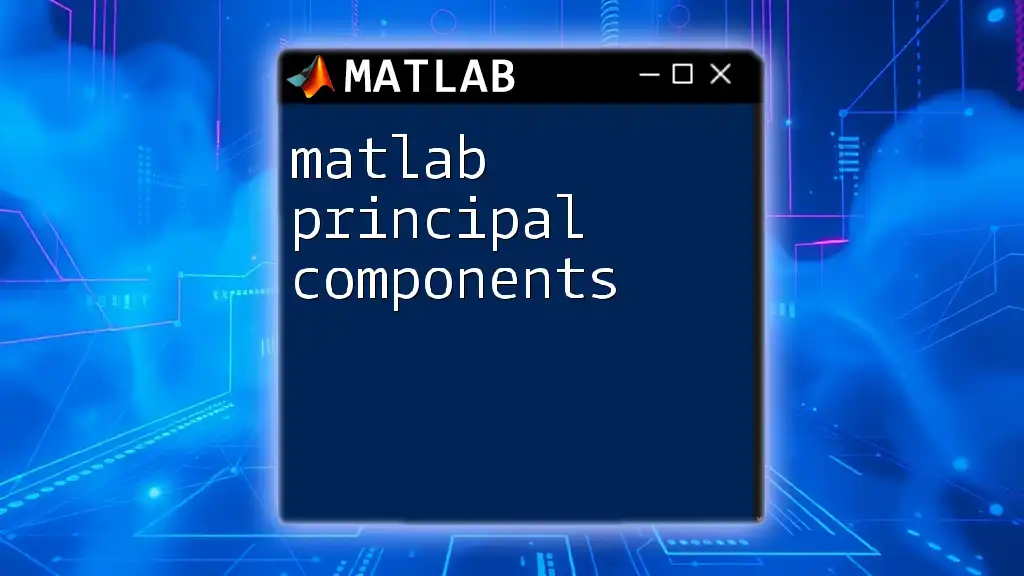
Escape Characters
Incorporating escape characters in your strings allows for added formatting. Common escape sequences include:
- `\n` - New line
- `\t` - Tab character
For instance, you can format a message with both a new line and a tab:
str = sprintf('First line\nSecond line with a tab:\tValue %d.', 10);
This will output:
First line
Second line with a tab: Value 10.
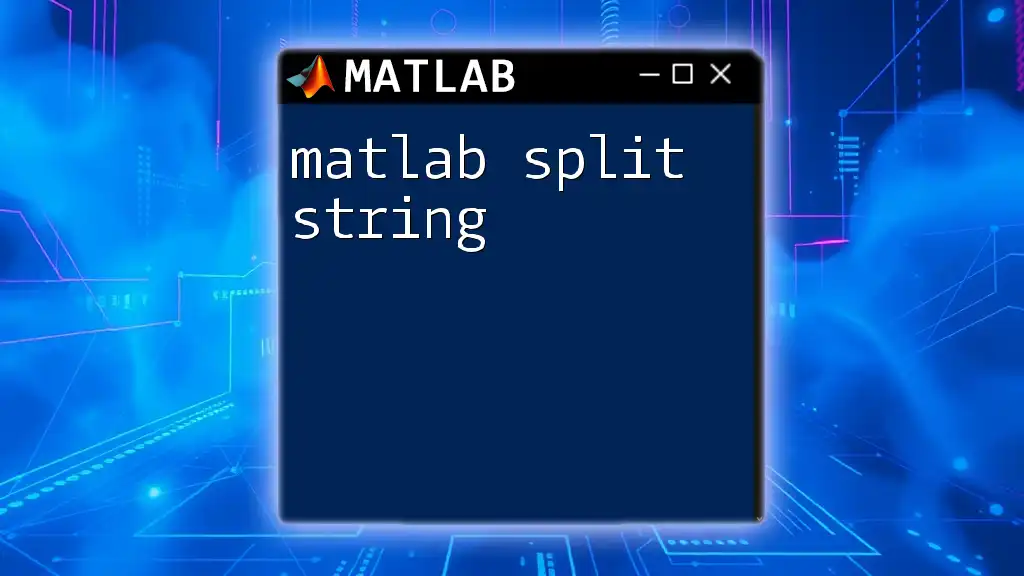
Tips for Effective Use of `sprintf`
Using `sprintf` effectively requires awareness of common pitfalls:
-
Mismatched Format Specifiers: Ensure that your format specifiers align with the data type of the values you’re providing.
For example, using `%f` for an integer will yield a warning or an incorrect format.
-
Array Dimensions: When using `sprintf` with arrays, be aware that it processes elements linearly rather than as a matrix, which can lead to misinterpretation of the output.
Performance Considerations
`matlab sprintf` is efficient for creating formatted strings, especially for reporting and logging purposes. If you're outputting strings to the console, use `fprintf` instead. However, for building strings to be used later or displayed conditionally, `sprintf` is optimal.
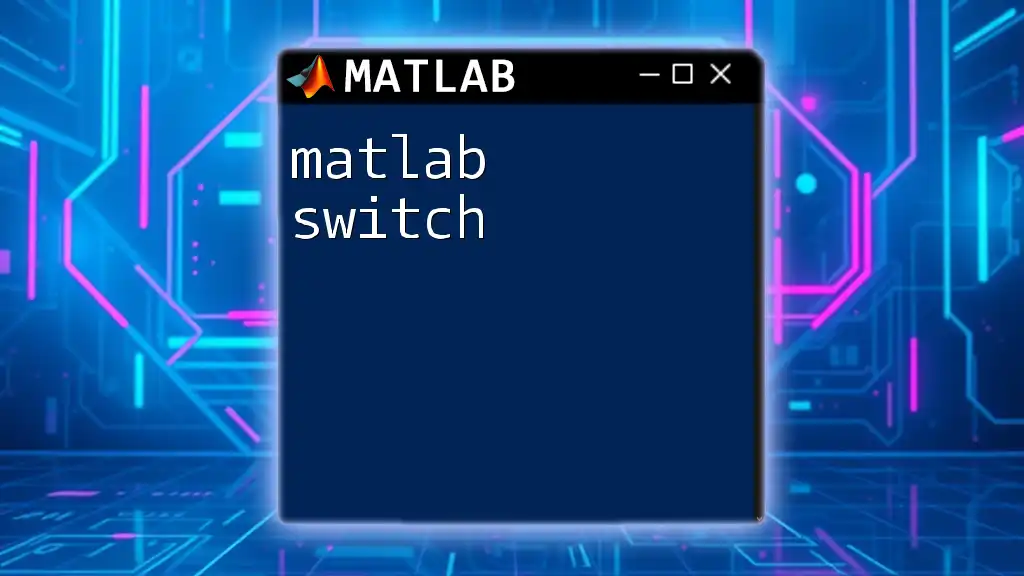
Real-World Applications of `sprintf`
`matlab sprintf` finds practical applications across various areas, including logging and debugging. It can seamlessly combine dates, commands, or error messages into a single string.
For example, to generate a log message in case of an error, you can use:
logMessage = sprintf('Error at %s: %s occurred.', datetime('now'), 'File not found');
In this scenario, you dynamically incorporate the timestamp and error description, producing a clear log entry for troubleshooting.
User Interface Development
In user interface contexts, `sprintf` can help create formatted text for components such as labels or text boxes, ensuring clarity and professionalism in presentation.
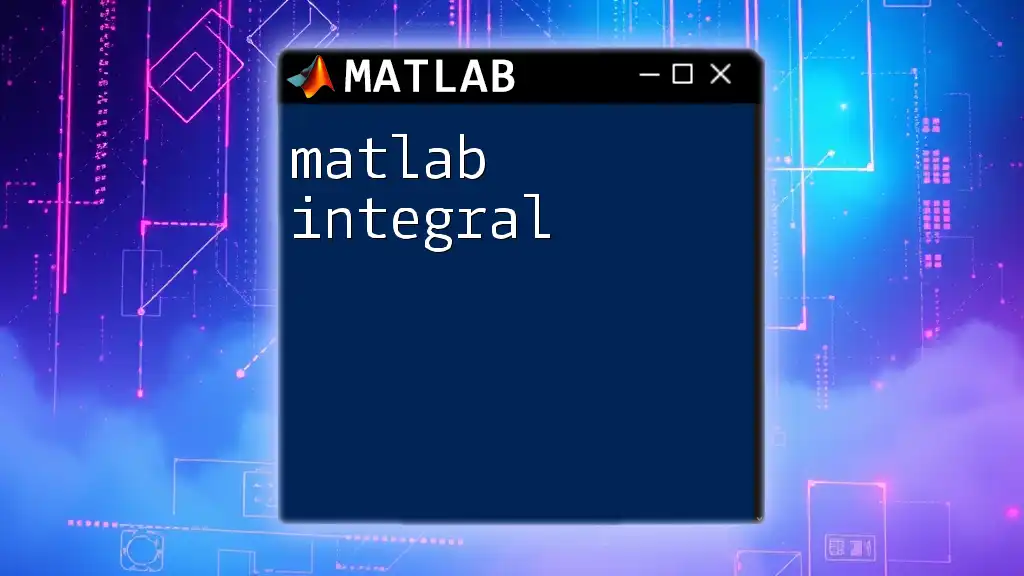
Recap of Key Points
In summary, `matlab sprintf` is essential for creating formatted strings. Knowing how to utilize format specifiers effectively, navigate common pitfalls, and apply it in real-world scenarios greatly enhances your MATLAB programming skills. Utilizing the insights provided in this guide will empower you to take full advantage of this versatile function in your coding endeavors.
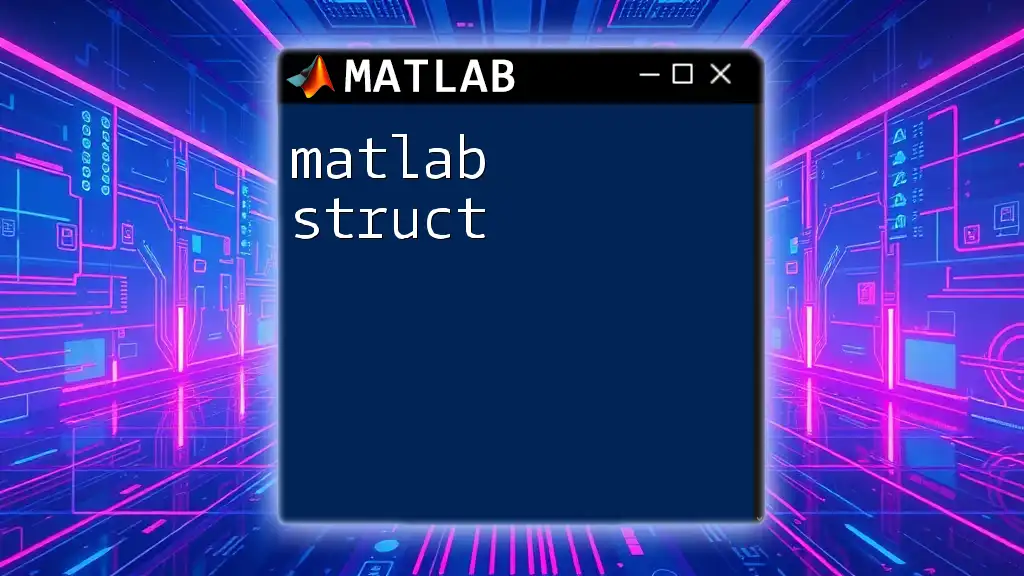
Further Resources
To explore more about `sprintf` and its applications, check MATLAB's official documentation, community forums, and additional tutorials aimed at enhancing your string manipulation techniques. Whether you're a novice or an experienced user, continuing your learning journey ensures you utilize `sprintf` to its fullest potential.