In MATLAB, you can convert a string to a number using the `str2double` function, which returns a numeric value corresponding to the string representation of the number.
num = str2double('42'); % Converts the string '42' to the number 42
Understanding Strings and Numbers in MATLAB
What is a String?
In MATLAB, a string is a sequence of characters used to represent text. Strings can contain letters, numbers, symbols, and spaces. They are typically enclosed in quotes (single or double). For instance, the statement:
greeting = 'Hello, World!';
creates a string variable `greeting`. Strings are essential in data manipulation, especially for filtering data, formatting outputs, or communicating with users.
What is a Number?
Numeric types in MATLAB represent data in various forms, including integers, decimals, and complex numbers. The most commonly used numeric types are `double` and `single`, which handle floating-point numbers, and `int32`, used for integer values. For example:
count = 10; % Integer
price = 3.99; % Decimal
complexNum = 1 + 2i; % Complex number
Each numeric type has its own precision and range, which is crucial when dealing with large datasets or scientific computations.
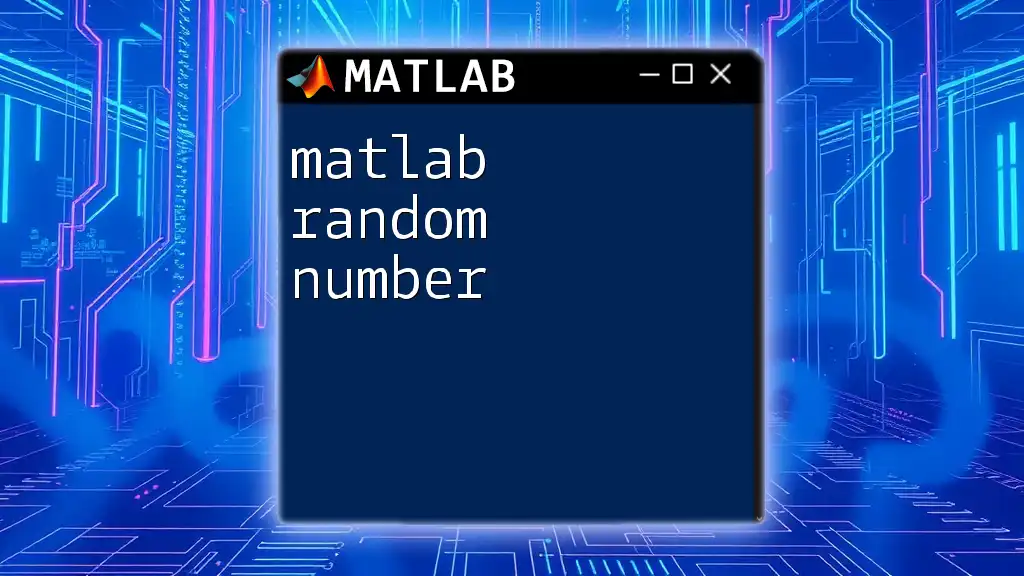
Why Convert Strings to Numbers?
Converting strings to numbers is a critical operation in MATLAB programming. Numeric computations demand numerical data types, so improper formatting can lead to errors or inaccurate results. Common scenarios include:
- Reading data from files, where numerical values may be stored as strings.
- Parsing user input from GUIs where users enter numerical values as text.
- Data analysis, where mathematical operations must be performed on numerical datasets extracted from text files or databases.
Failure to convert strings into usable numeric formats can obstruct data analysis, resulting in computational inefficiencies or erroneous outcomes.
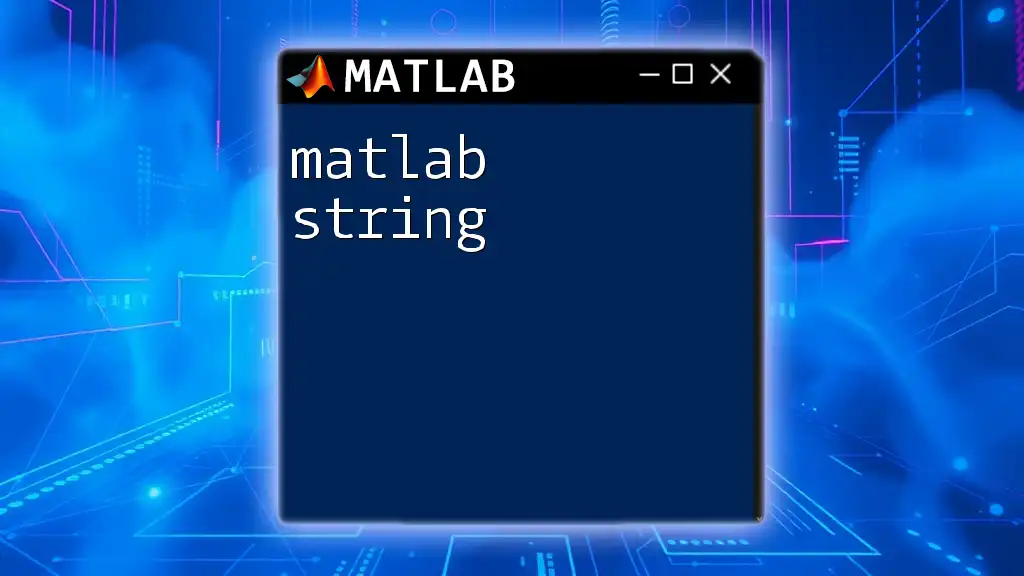
Methods to Convert Strings to Numbers in MATLAB
Using `str2double`
`str2double` is the most straightforward function for converting a string representation of a number into a double-precision number. Its syntax is:
num = str2double(str);
When using `str2double`, valid numeric strings convert seamlessly, while invalid strings return `NaN` (Not a Number). For example:
strValue = '3.14';
numValue = str2double(strValue);
% Output: numValue => 3.14
If you attempt to convert an invalid string:
invalidValue = str2double('not_a_number');
% Output: invalidValue => NaN
This behavior allows you to easily check for conversion errors.
Using `str2num`
While `str2num` also converts strings to numbers, it has unique features. This function evaluates the input string as a MATLAB expression, making it suitable for complex formats but also potentially unsafe if user input is not validated. Its syntax is similar:
array = str2num(str);
For instance, if you want to convert a string that represents an array:
strValue = '[1, 2; 3, 4]';
numArray = str2num(strValue);
% Output: numArray => [1, 2; 3, 4]
However, caution is advised since `str2num` will execute any MATLAB code present in its string input, leading to unwarranted evaluations if not managed carefully.
`sscanf` for String Parsing
For cases where strings follow a specific format, `sscanf` is a robust choice, allowing structured data extraction with format specifiers. Its basic syntax is:
result = sscanf(str, format);
This is particularly useful for extracting numbers from structured strings. For example:
strValue = 'Total: 100 USD';
amount = sscanf(strValue, 'Total: %d');
% Output: amount => 100
Using `sscanf` enhances control over how strings are parsed, but be aware of its limitations compared to `str2double` and `str2num` in more generalized situations.
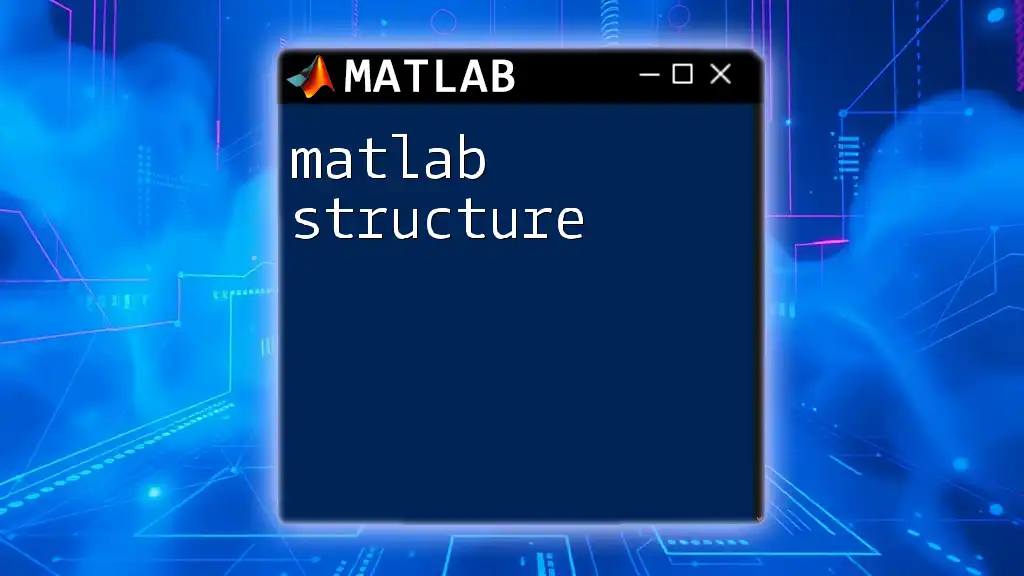
Error Handling during Conversion
Managing Invalid Conversions
Handling invalid conversions is crucial in programming. Common issues include receiving unexpected strings, resulting in `NaN` values. To manage this, consider implementing logical checks:
strArray = {'3.14', 'abc', ''};
numArray = str2double(strArray);
% Use logical indexing to safely convert
validNumbers = numArray(~isnan(numArray));
This technique ensures that users only work with successful conversions, thus avoiding potential computational errors.
Implementing Try-Catch for Robustness
When working with user input or dynamic data, it is prudent to implement error handling using `try-catch` blocks. This approach catches exceptions and prevents program crashes. For example:
try
numValue = str2double('invalid');
catch
disp('Error: The input was not a valid number.');
end
By handling exceptions gracefully, you improve the user experience and the reliability of your MATLAB applications.
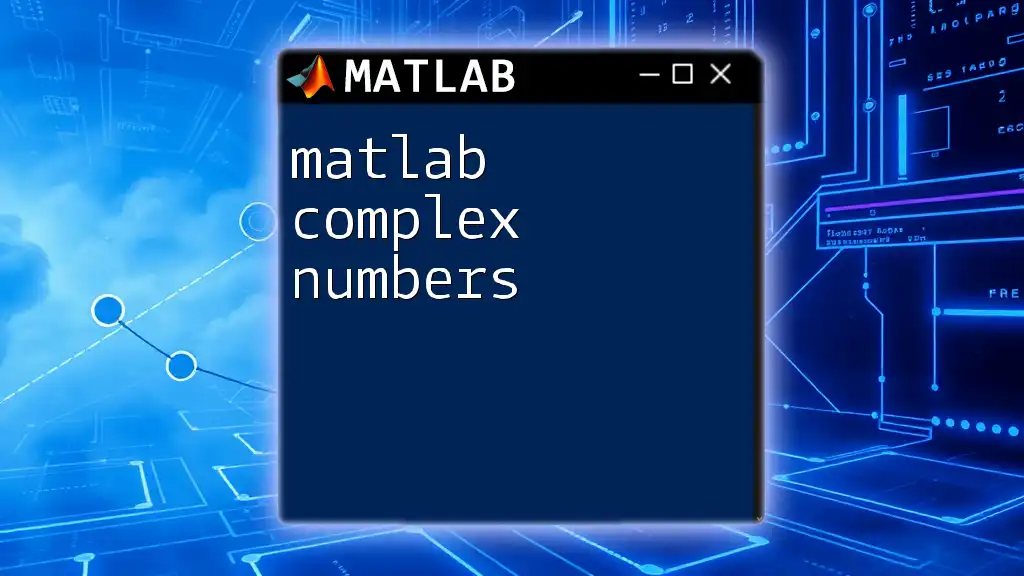
Performance Considerations
When deciding which conversion method to use, performance can play a significant role, especially with larger data sets.
- `str2double` generally performs faster and is safer, making it the preferred choice for converting simple numeric strings.
- `str2num` tends to be slower since it evaluates strings as expressions, which entails additional overhead.
- `sscanf` is typically faster than `str2num` for formatted input, particularly when working with bulk data.
When dealing with high volumes of data or when performance is critical, optimizing your choice based on context is essential.
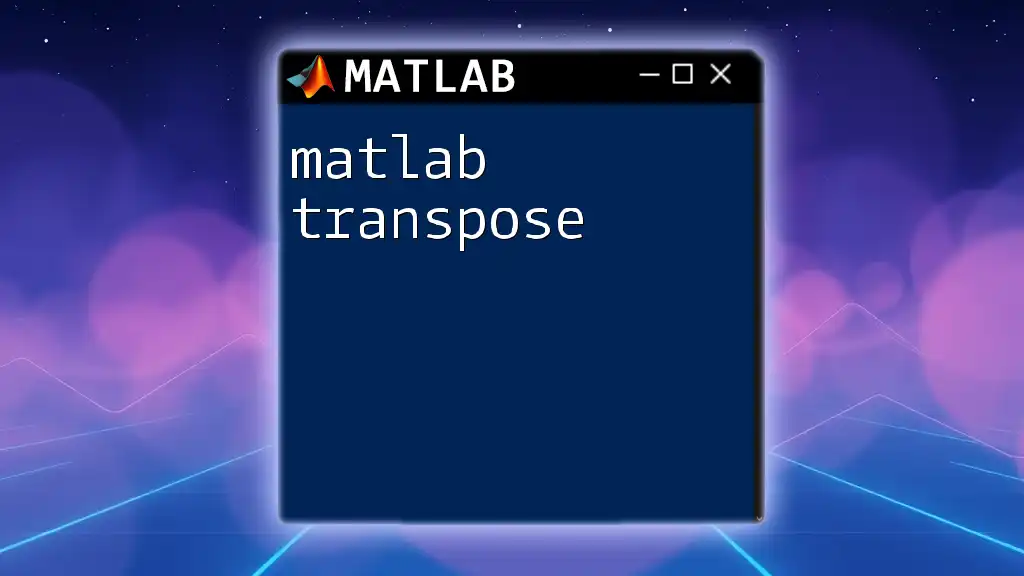
Conclusion
Converting `MATLAB string to number` is a fundamental task in numerical computing, enabling seamless data manipulation and analysis. By understanding the means of conversion—`str2double`, `str2num`, and `sscanf`—and implementing effective error handling, you ensure robust MATLAB programs that manage data efficiently.
Exploring MATLAB’s comprehensive documentation can further enhance your command over these functions, leading you to refined and error-free programming results.
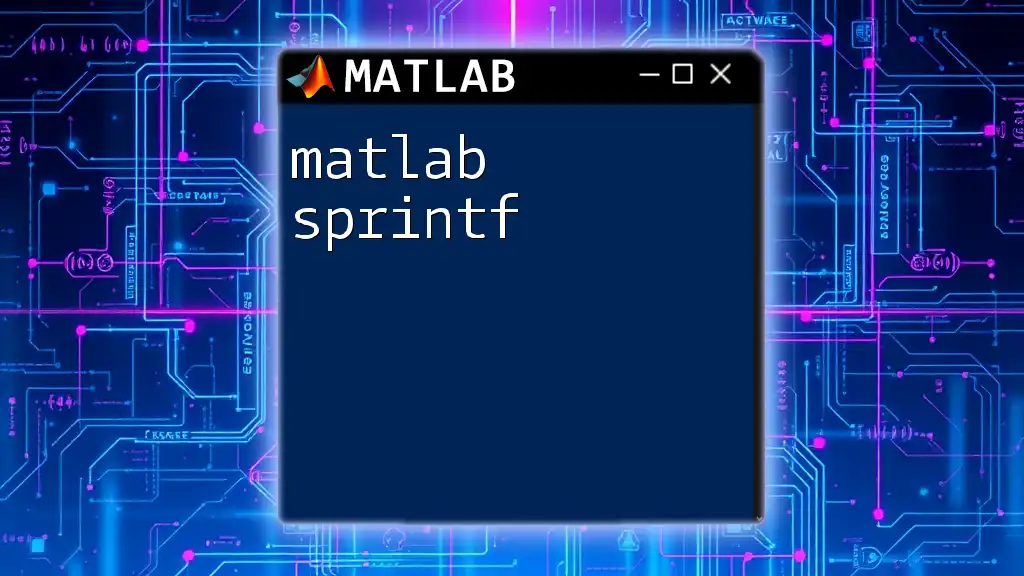
Additional Resources
For those eager to deepen their understanding, I recommend reviewing the official MATLAB documentation on these functions as well as exploring tutorials on string manipulations available through educational platforms and your company's offerings.
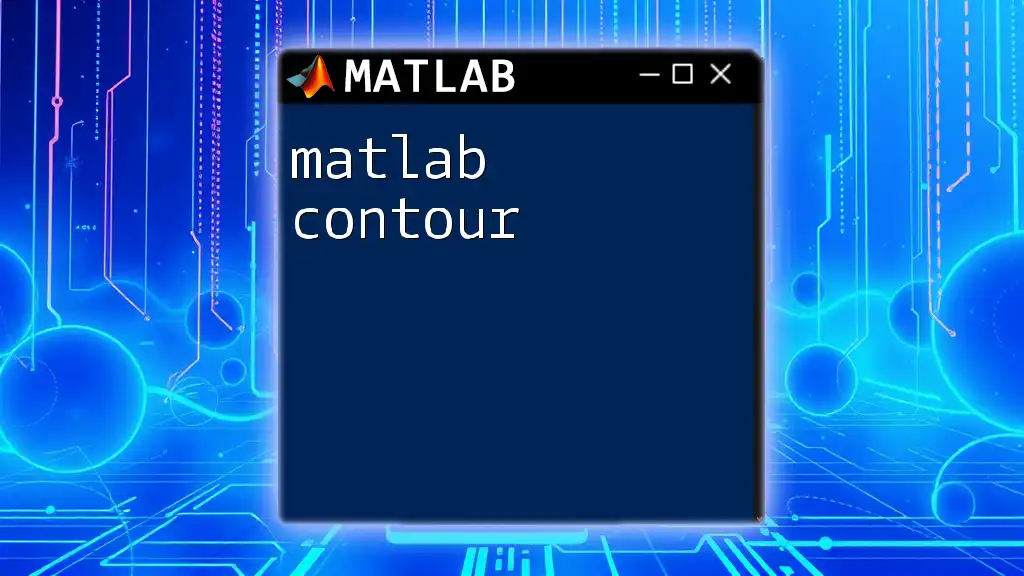
Call to Action
Do you have any experiences or questions regarding the conversion of strings to numbers in MATLAB? Share them in the comments below! If you're interested in more tips and tricks on getting the most out of MATLAB, subscribe for updates on our courses and tutorials.