In MATLAB, the `sort` function is used to arrange the elements of an array in ascending or descending order, allowing for easy data organization. Here's a simple code snippet demonstrating how to use the `sort` function:
% Example of sorting a numerical array in ascending order
numbers = [5, 3, 8, 1, 4];
sortedNumbers = sort(numbers);
disp(sortedNumbers);
Understanding the `sort` Function
Basic Syntax of `sort`
The `sort` function in MATLAB is a powerful tool used for organizing data in a desired order. The basic syntax of `sort` is as follows:
B = sort(A)
Here, `A` represents the data you want to sort, and `B` is the output containing the sorted data. You can easily apply this function to simple vectors as well as complex matrices.
For example, when given a vector `A`:
A = [3, 1, 4, 1, 5];
sorted_A = sort(A);
The variable `sorted_A` would result in:
sorted_A = [1, 1, 3, 4, 5]
This output showcases how `sort` arranges the elements in ascending order by default.
Sorting Rows and Columns
Sorting a Vector
When you sort a vector, MATLAB organizes the values in ascending order without any additional parameters.
v = [5, 2, 8, 1];
sorted_v = sort(v); % Default sorts in ascending order
The result will be:
sorted_v = [1, 2, 5, 8]
Sorting a Matrix
Sorting a matrix, on the other hand, allows you to specify whether to sort rows or columns.
Sort Rows
You can sort each row individually by specifying `2` as the second argument in the `sort` function.
M = [3, 1, 2; 6, 4, 5];
sorted_rows = sort(M, 2); % Sorts each row
The output will look like this:
sorted_rows = [1, 2, 3; 4, 5, 6]
Sort Columns
To sort the columns of a matrix, simply provide `1` as the second argument.
sorted_columns = sort(M, 1); % Sorts each column
The resulting matrix will be:
sorted_columns = [3, 1, 2; 6, 4, 5]
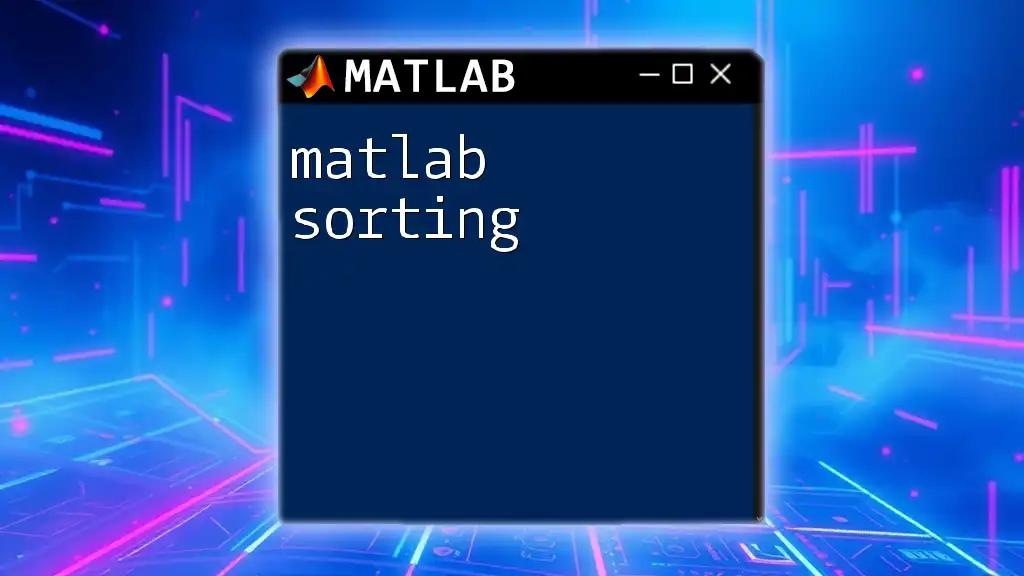
Advanced Sorting Features
Sorting Order and Direction
Next, you can modify the sorting order. By default, the `sort` function arranges values in ascending order. If you want to sort in descending order, specify `'descend'` as the third argument.
descending_A = sort(A, 'descend');
For example, if `A = [3, 1, 4, 1, 5]`, the sorted output will be:
descending_A = [5, 4, 3, 1, 1]
Sorting with Indices
One beneficial feature of `sort` is its ability to return the original indices of the sorted data along with the sorted array.
[sorted_A, indices] = sort(A);
Here, `indices` will give you the position of the elements in the original array. For instance, if `A` is `[3, 1, 4, 1, 5]`, the `indices` will reflect where each element was in the original array:
indices = [2, 4, 1, 3, 5]
Multi-Dimensional Sorting
For sorting in higher dimensions, such as 3D arrays, you can also utilize the `sort` function.
M3D = rand(4, 3, 2); % 3D matrix
sorted_M3D = sort(M3D, 1); % Sorts along the first dimension
This can be particularly useful in specific applications where dimensionality of data is crucial.
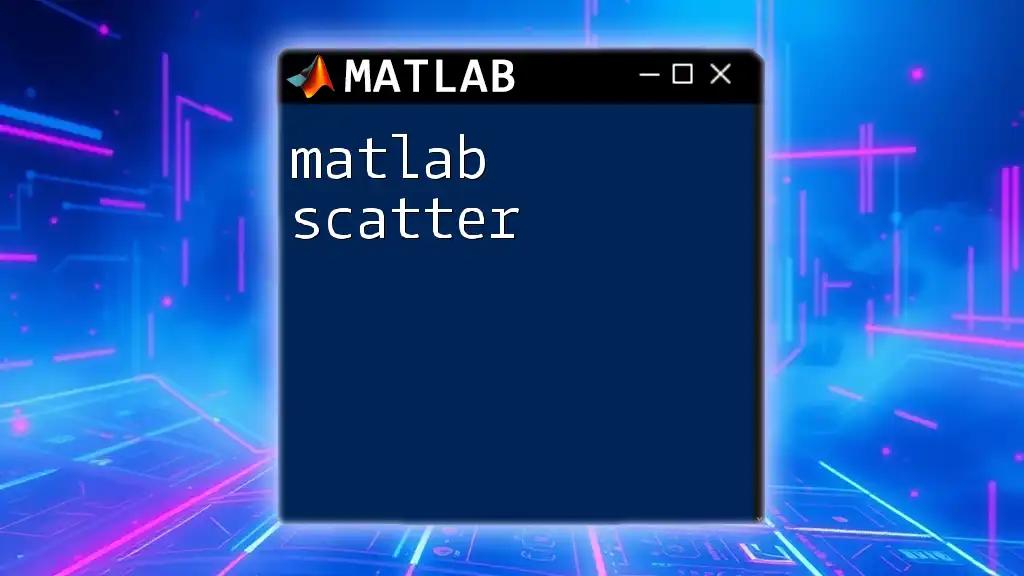
Alternative Sorting Functions
Using `sortrows` for Sorting Tables
When dealing with tables in MATLAB, the `sortrows` function is incredibly helpful for sorting based on one or more columns. The basic syntax is:
B = sortrows(A, column)
Where `column` can be a number or a variable name indicating which column to sort by.
For example:
T = table([1; 2; 3], [6; 5; 4], 'VariableNames', {'A', 'B'});
sorted_T = sortrows(T, 'B');
This will sort the table `T` based on the values in column `B`, providing a structured view of your data.
Using `sortcats` for Categorical Data
MATLAB also provides the `sortcats` function specifically for sorting categorical arrays. This is extremely useful when you need to sort data categorized into discrete groups.
For example:
C = categorical({'apple', 'banana', 'apple'});
sorted_C = sort(C);
You will get a new array sorted in alphabetical order:
sorted_C = ["apple", "apple", "banana"]
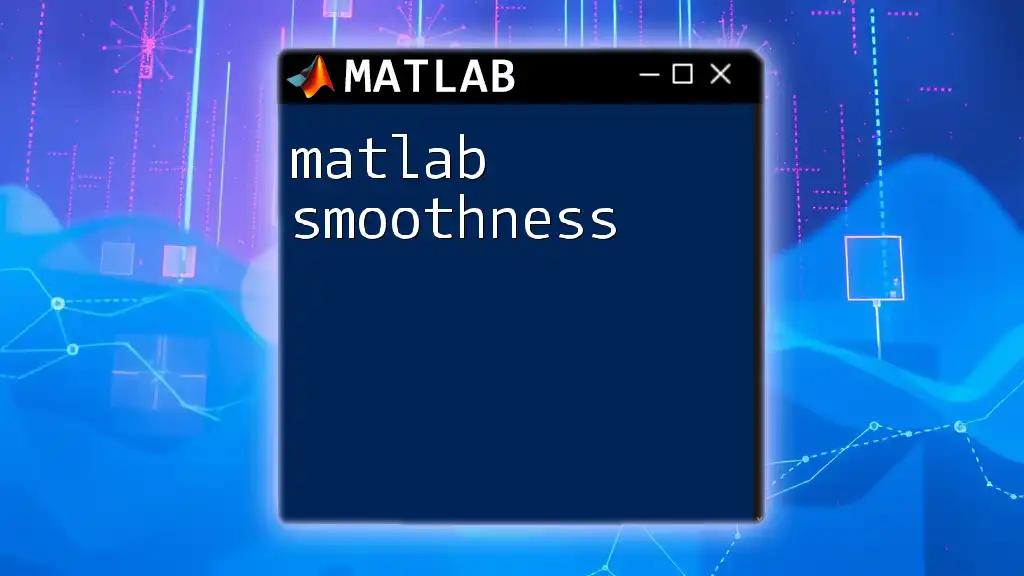
Practical Applications of Sorting
Sorting Data for Visualization
Sorting is often a precursor to data visualization, as it allows for a more intuitive understanding of data trends. A common scenario involves sorting data before plotting a histogram, as shown below:
data = randn(1, 1000);
sorted_data = sort(data);
histogram(sorted_data);
This sorted data ensures that your visualization clearly conveys the distribution of values, making it easier to interpret results.
Sorting and Analyzing Large Datasets
When working with large datasets, it’s critical to manage sorting operations efficiently. MATLAB’s `tall` array functionality can handle large data sets that do not fit into memory, enabling sorting without compromising speed or performance.
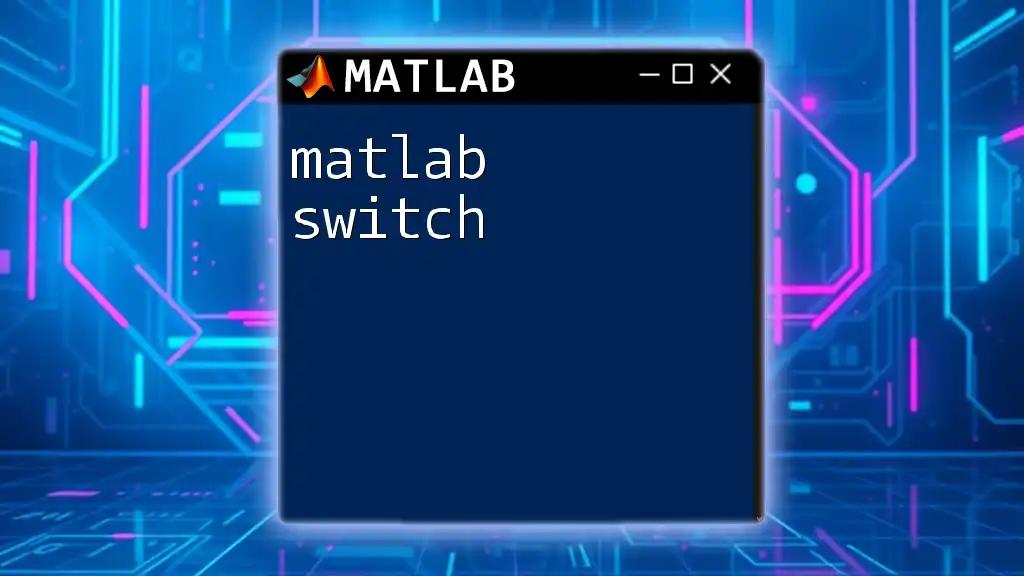
Common Issues and Troubleshooting
Handling Non-Numeric Data
Sorting isn’t limited to numerical values; you can also sort strings and mixed types. For instance, sorting string arrays can be straightforward, as shown below:
strings = ["banana", "apple", "grape"];
sorted_strings = sort(strings);
The output will be arranged in lexicographical order:
sorted_strings = ["apple", "banana", "grape"]
Performance Considerations
It is essential to recognize that sorting algorithms in MATLAB have different time complexities, especially with larger arrays. Understanding your data and choosing the right approach is crucial for optimizing performance.
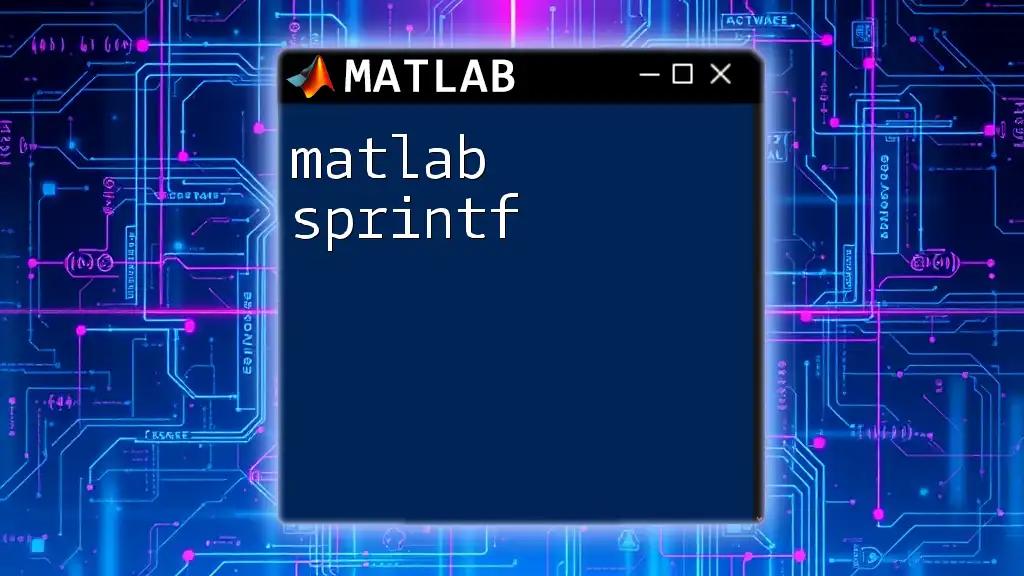
Conclusion
In summary, MATLAB’s sorting capabilities are diverse and robust, offering users multiple ways to order datasets efficiently. By mastering functions like `sort`, `sortrows`, and `sortcats`, and understanding their parameters, you can make data management in MATLAB easier and more effective. Now is the perfect time to explore these functions on various datasets to enhance your proficiency in MATLAB sorting!
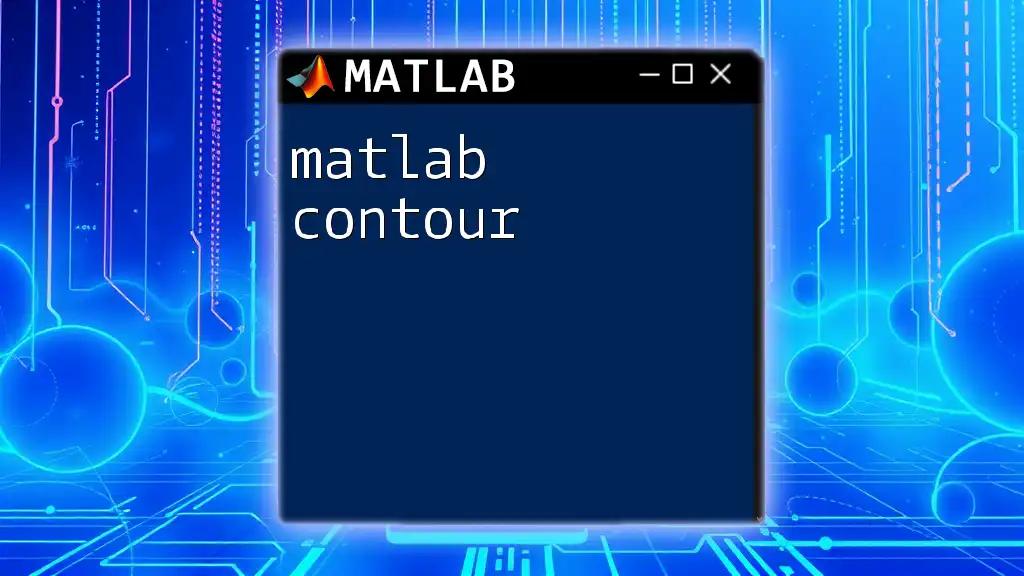
Additional Resources
For further reading, consider referring to the official MATLAB documentation for sorting functions and exploring online tutorials that provide hands-on experience with real-world datasets. These resources can greatly complement your understanding and application of sorting within MATLAB.