The MATLAB t-test is a statistical method used to determine if there is a significant difference between the means of two groups, which can be performed using the `ttest2` function for independent samples.
% Example: Performing a two-sample t-test in MATLAB
group1 = [5.3, 6.1, 5.9, 5.8, 6.3]; % Data for group 1
group2 = [7.1, 7.4, 7.2, 6.9, 7.6]; % Data for group 2
[h, p] = ttest2(group1, group2); % Conducts the t-test
fprintf('Hypothesis test result: %d, p-value: %.4f\n', h, p); % Displays results
Understanding the T-Test
What is a T-Test?
A t-test is a statistical hypothesis test that is commonly used to compare the means of two groups or a group against a known value. This type of test is particularly useful when the sample sizes are small, or when the population standard deviations are unknown. The t-test assesses whether the means of two groups are statistically different from each other.
There are three primary types of t-tests:
- One-sample t-test: Compares the mean of a single group against a known value or hypothesized mean.
- Independent two-sample t-test: Compares the means of two independent groups.
- Paired sample t-test: Compares two related groups, such as measurements taken before and after an intervention.
Understanding when to apply a t-test is crucial, as conducting an inappropriate test can lead to inaccurate conclusions.
When to Use a T-Test
It’s essential to identify situations where a t-test is suitable:
- When comparing sample means: You may want to check if the average score of a test is significantly different from a benchmark.
- Small sample size: If you have a small dataset (typically less than 30), a t-test may be more appropriate than a z-test.
- When the population variance is unknown: The t-test effectively accounts for the added variability when estimating population parameters.
Additionally, t-tests should be conducted under the assumption that:
- The data is approximately normally distributed (especially important for smaller sample sizes).
- The samples being compared have equal variances (for independent t-tests).
Be mindful of other statistical tests as well. When the assumptions for t-tests are not met, consider alternative tests such as the Mann-Whitney U test for independent samples or the Wilcoxon signed-rank test for paired samples.
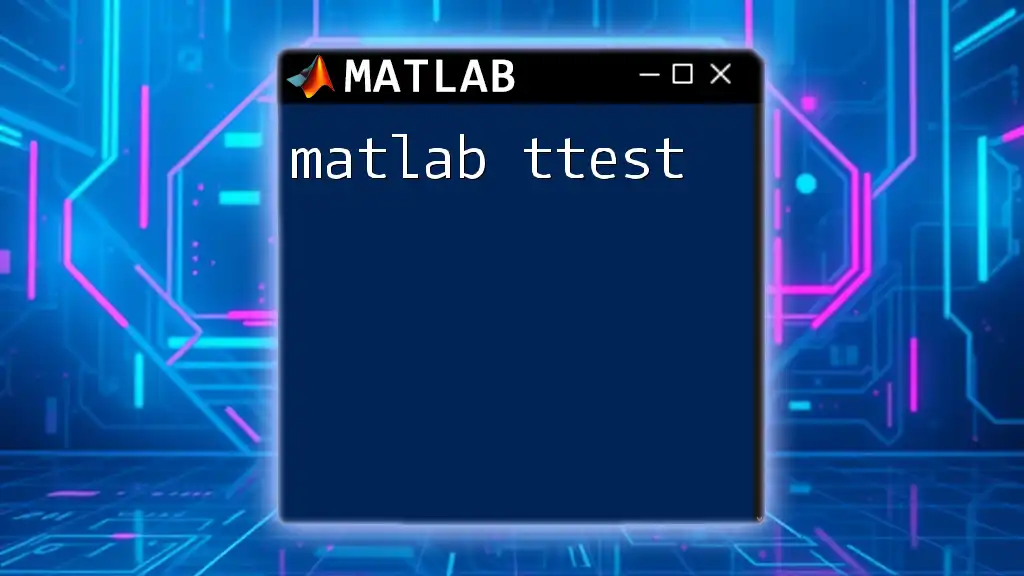
Types of T-Tests in MATLAB
One-Sample T-Test
The one-sample t-test helps determine if the mean of a single group significantly differs from a hypothesized population mean. This is useful in cases like evaluating a new treatment or method against a known standard.
Example: Suppose you have test scores from a class and want to see if they differ significantly from a passing score of 25.
data = [23, 25, 22, 30, 26]; % Sample scores
mu = 25; % Hypothesized mean score
[h, p, ci, stats] = ttest(data, mu);
In this code:
- `h` indicates whether the null hypothesis can be rejected (1 if rejected, 0 otherwise).
- `p` is the p-value, used to determine statistical significance.
- `ci` provides the confidence interval for the mean difference.
- `stats` contains additional statistical information.
Independent Two-Sample T-Test
The independent two-sample t-test is used to compare the means of two groups that are unrelated. For instance, comparing test scores between two different classes.
Example: To compare scores between Class A and Class B, the following code can be employed:
data1 = [23, 25, 22, 30, 26]; % Class A
data2 = [29, 32, 31, 34, 30]; % Class B
[h, p, ci, stats] = ttest2(data1, data2);
Here, the output variables serve the same purpose as described in the one-sample t-test, allowing for a direct comparison between the two groups.
Paired Sample T-Test
The paired sample t-test evaluates the means from the same group at different times. This is especially useful in cases where measurements are taken before and after a treatment.
Example: Imagine measuring blood pressure before and after a treatment.
before = [1.2, 1.4, 1.5, 1.6, 1.3]; % Blood pressure before treatment
after = [1.3, 1.6, 1.7, 1.8, 1.5]; % Blood pressure after treatment
[h, p, ci, stats] = ttest(before, after);
The results here will indicate if the treatment had a significant effect on blood pressure.
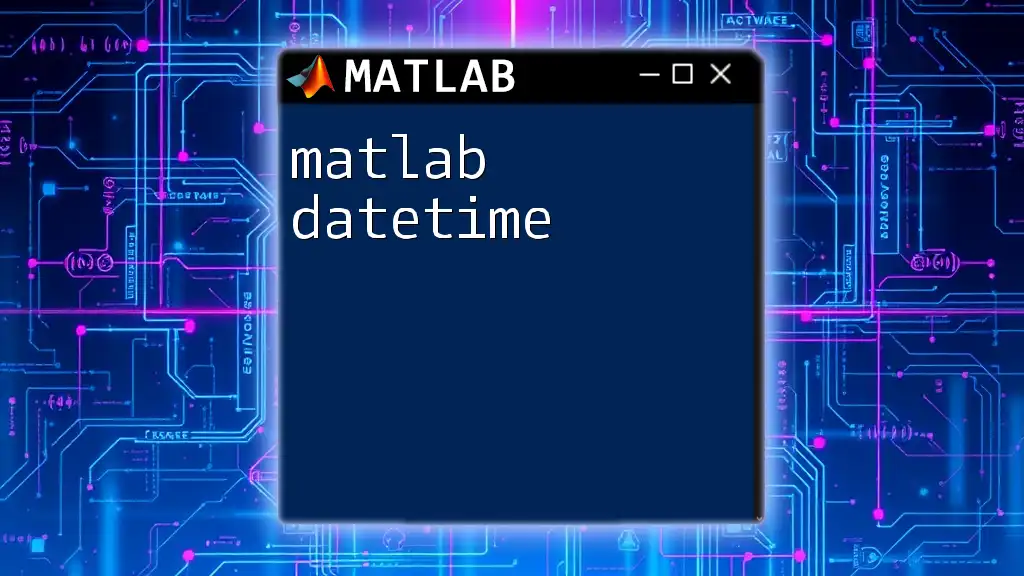
Interpreting T-Test Results
Understanding Output from MATLAB
When conducting a t-test in MATLAB, you receive several important outputs:
- h: A binary result indicating whether to reject the null hypothesis. If `h = 1`, it means there is a significant difference.
- p: The p-value helps in assessing the strength of the evidence against the null hypothesis. A common significance level is 0.05.
- ci: The confidence interval provides a range within which the true mean difference is likely to lie.
- stats: This includes further statistical values like the t-statistic and degrees of freedom.
For interpreting the p-value:
- A low p-value (typically < 0.05) suggests that the means of the groups are significantly different.
- A high p-value indicates no significant difference.
Making Conclusions From T-Test Results
Based on the p-value obtained from the test, you can draw conclusions:
- Reject the null hypothesis if `p < 0.05`, indicating a significant difference between means.
- Fail to reject the null hypothesis if `p >= 0.05`, suggesting no evidence of a difference.
It's crucial to avoid common pitfalls, such as Type I errors (false positives) and Type II errors (false negatives), which can mislead your findings.
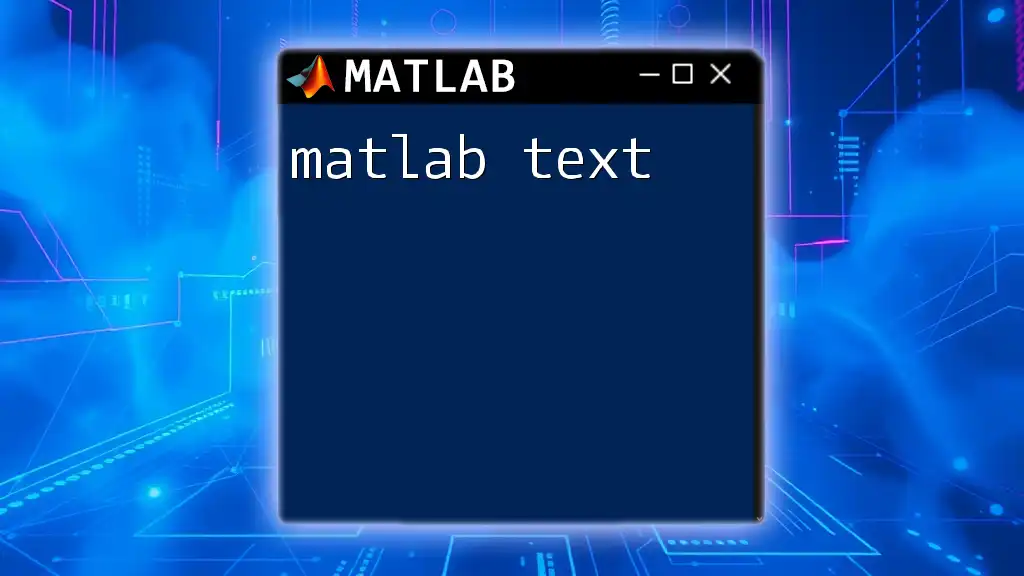
Visualizing T-Test Results
Importance of Visualization
Visualizing statistical results can enhance understanding and convey complex information easily. Graphical representations such as box plots or histograms can illustrate differences between groups effectively.
Creating Visualizations in MATLAB
MATLAB allows for straightforward plot creation. For example, to create a box plot comparing two groups:
data = [data1; data2]';
boxplot(data);
title('Boxplot of Two Sample Data');
xlabel('Groups');
ylabel('Values');
Using visualizations can help in quickly identifying trends, outliers, and distributions, making it easier to convey your findings to others.
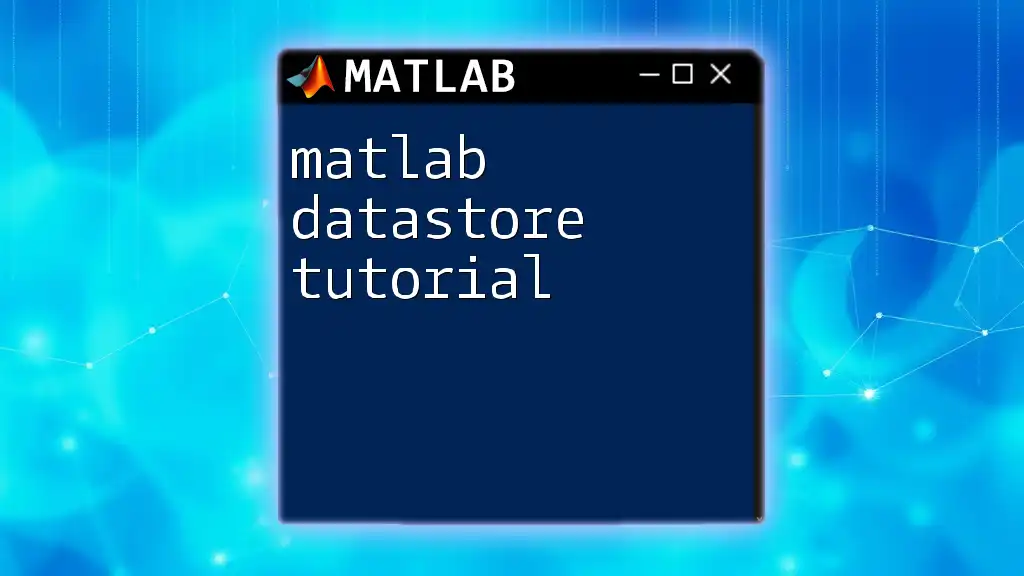
Common Issues and Pitfalls
Violations of T-Test Assumptions
If the assumptions of the t-test are violated, it can lead to incorrect conclusions. Key assumptions include:
- Normality: The data should be approximately normally distributed, especially with small sample sizes. You can test this using the Shapiro-Wilk test.
[h_normality, p_normality] = swtest(data); % Assumes you have the swtest available
If normality is not present, consider data transformation or choose a non-parametric test.
Alternatives to T-Tests
In instances where t-tests are not appropriate due to violations of assumptions, consider alternative statistical tests:
- Mann-Whitney U Test: A non-parametric alternative for independent samples.
- Wilcoxon Signed-Rank Test: A non-parametric alternative for paired samples.
These tests may provide more reliable results when traditional assumptions are not met.
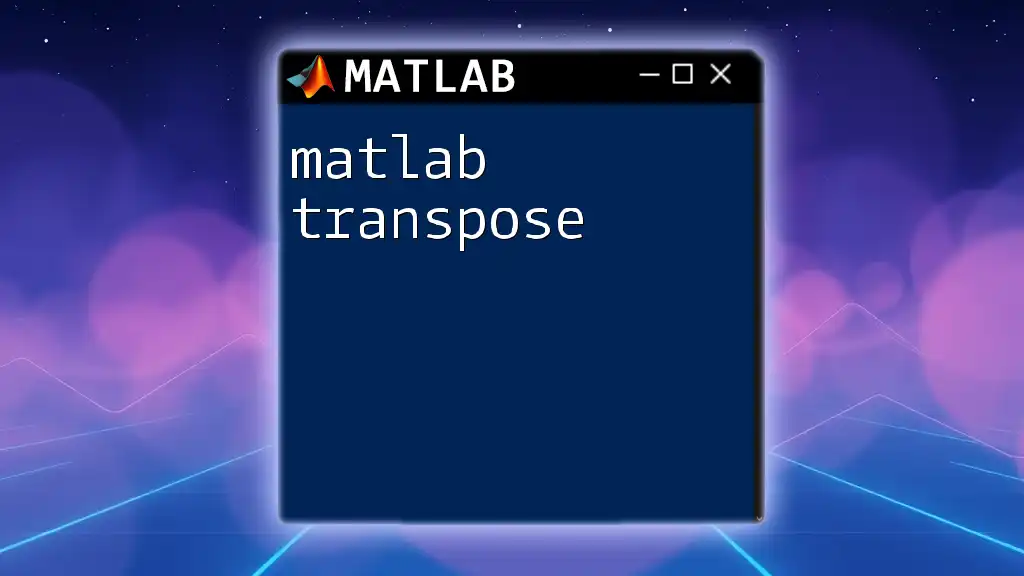
Conclusion
In summary, the MATLAB t-test offers a versatile and powerful method for statistically comparing means across groups. Understanding the different types of t-tests, correctly interpreting their results, and being aware of common pitfalls are critical for accurate analysis. It is advisable to practice with various datasets to strengthen your application of t-tests in different scenarios.