In MATLAB, a struct (short for structure) is a data type that allows you to group related data using named fields, making it easier to manage complex datasets.
Here’s a simple example of how to create and access a MATLAB struct:
% Create a struct with fields 'name', 'age', and 'height'
person = struct('name', 'John Doe', 'age', 30, 'height', 5.9);
% Access the fields of the struct
personName = person.name;
personAge = person.age;
personHeight = person.height;
disp(['Name: ', personName, ', Age: ', num2str(personAge), ', Height: ', num2str(personHeight)]);
What is a Struct?
In MATLAB, a struct (short for structure) is a versatile data type used for grouping related data. Each struct can contain fields, which are named pieces of data, allowing for organized data management. This is particularly beneficial when dealing with complex data settings, such as in applications involving multiple related parameters.
Advantages of Using Structs
Using structs offers several advantages:
- Flexibility: Structs can hold various data types, making them ideal for heterogeneous data.
- Enhanced organization: They provide a way to bundle related data into a single entity, improving code readability.
- Comparison with other data types: Unlike arrays (which require uniformity), structs allow for different types and sizes for each field, adding to their practicality.
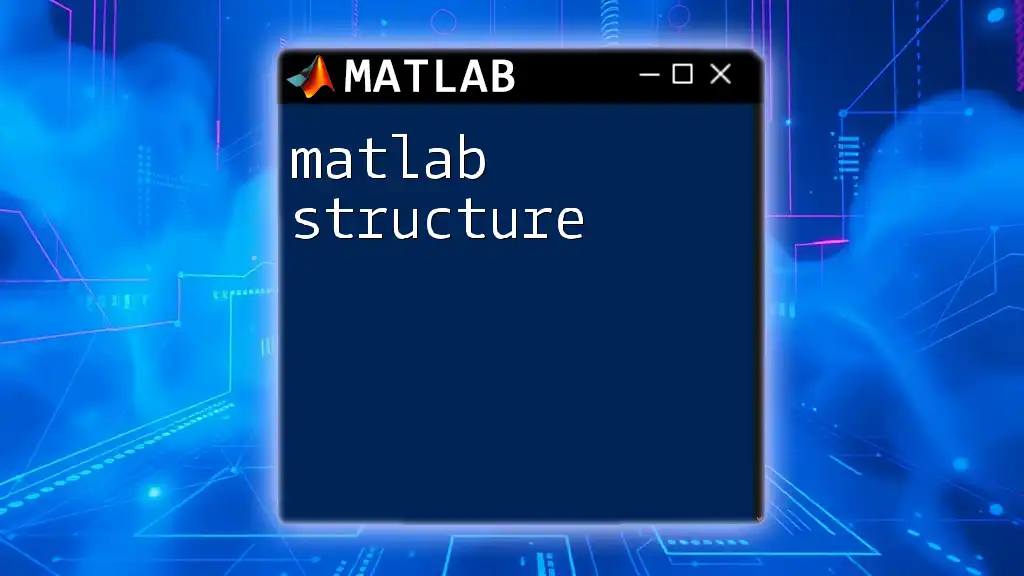
Creating a MATLAB Struct
Basic Struct Creation
Creating a simple struct in MATLAB is straightforward. The syntax involves using a dot notation to assign values to the fields. For example, let's create a struct for a student:
student.name = 'John Doe';
student.age = 21;
student.major = 'Computer Science';
The above code creates a struct named `student` with three fields: `name`, `age`, and `major`, allowing for easy access to student data.
Using the Struct Function
For more complex applications or when handling multiple records, you can use the `struct` function for batch creation. This approach is particularly effective when you need to manage arrays of structs. Here’s how you create a struct array for multiple students:
students = struct('name', {'Jane Doe', 'Alice Smith'}, 'age', {22, 23}, 'major', {'Mathematics', 'Physics'});
In this example, the `students` variable becomes an array of structs, each representing a different student with their respective fields.
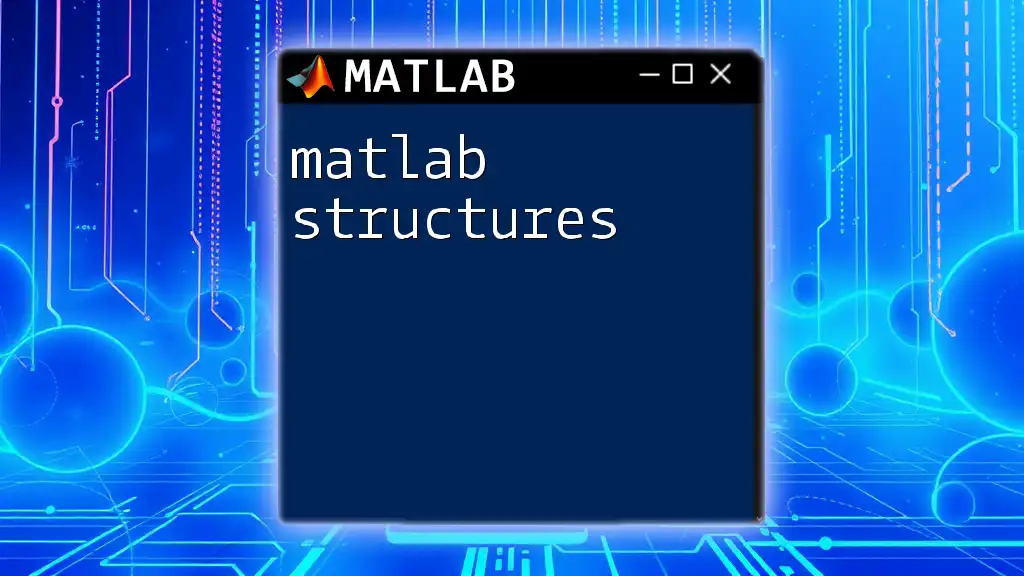
Accessing Struct Fields
Accessing Individual Fields
Accessing fields within a struct is done using the dot notation. For instance, to display John's name, you would use:
disp(student.name);
This command will output the value associated with the `name` field of the `student` struct.
Accessing Multiple Fields
If you need to access and display multiple fields simultaneously, a convenient method is to utilize vectorization or iterating through the struct array. Below is an example that extracts and displays all student names:
disp({students.name});
This will output a cell array containing the names of all students in the `students` struct array.
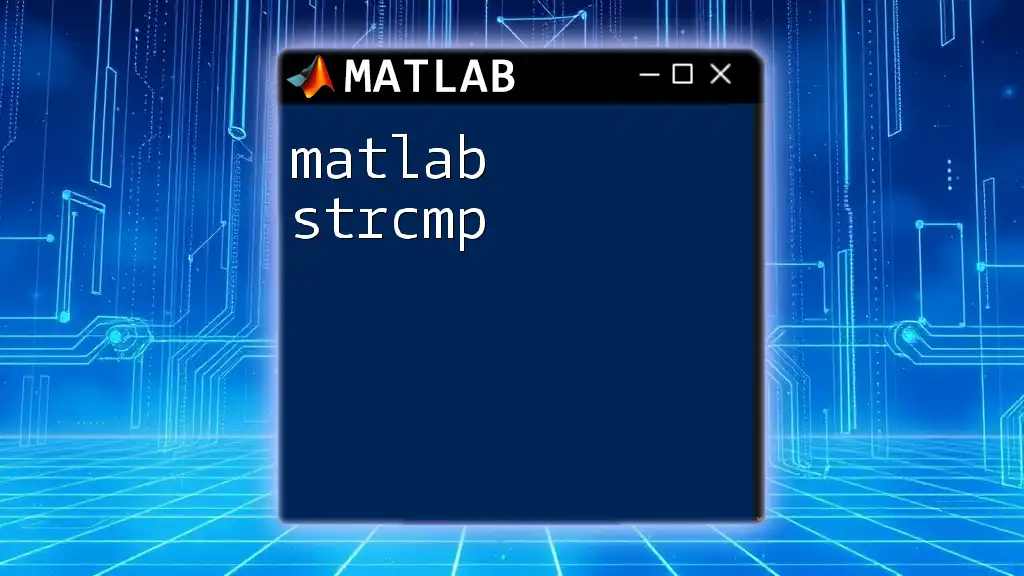
Modifying Structs
Updating Field Values
Modifying the values of existing struct fields is simple. You can directly assign a new value to a specific field. For example, to update the age of our `student`, you would do:
student.age = 22; % Updated age
This command updates the `age` field of the `student` struct to 22.
Adding New Fields
Structs are dynamic, meaning you can add new fields at any time. For instance, if we want to add a GPA field to the `student` struct, we simply assign it:
student.gpa = 3.8;
Now, the `student` struct includes a new field `gpa` without impacting the existing data organization.
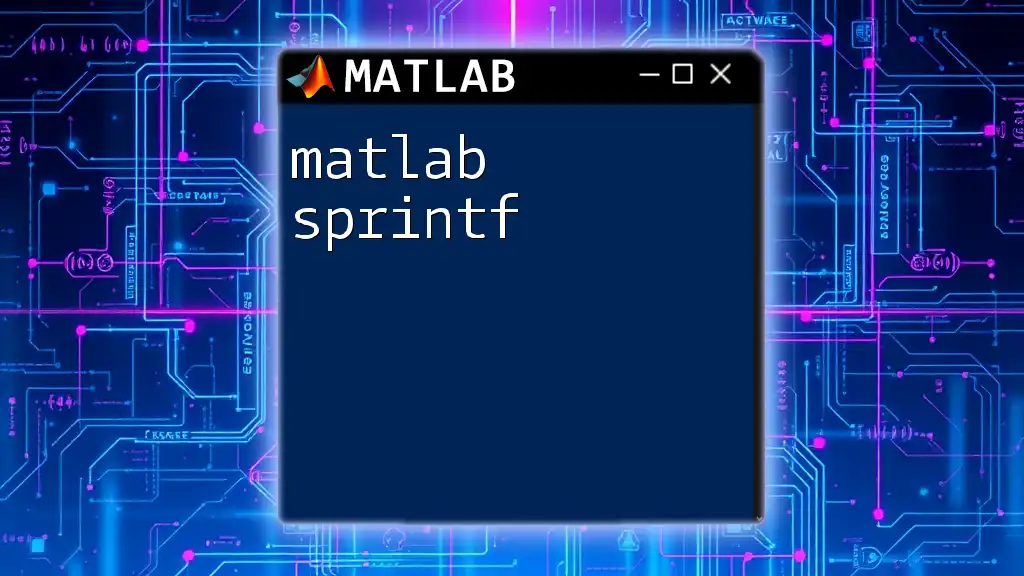
Advanced Struct Techniques
Nested Structs
Nested structs allow you to create more complex data structures by including structs within structs. This is useful for organizing hierarchical data. For example, let's create a struct for a course that contains an instructor struct:
course.name = 'Intro to MATLAB';
course.instructor.name = 'Dr. Smith';
course.instructor.email = 'smith@example.com';
In this case, the `course` struct contains another struct called `instructor`, holding details specific to that instructor.
Struct Arrays
Struct arrays are beneficial when you want to maintain collections of similar data types. Create an array of `student` structs as shown below:
students(1) = struct('name', 'Jane', 'age', 22);
students(2) = struct('name', 'Alice', 'age', 23);
Here, `students` is an array that holds two student records, enabling easy indexing and access.
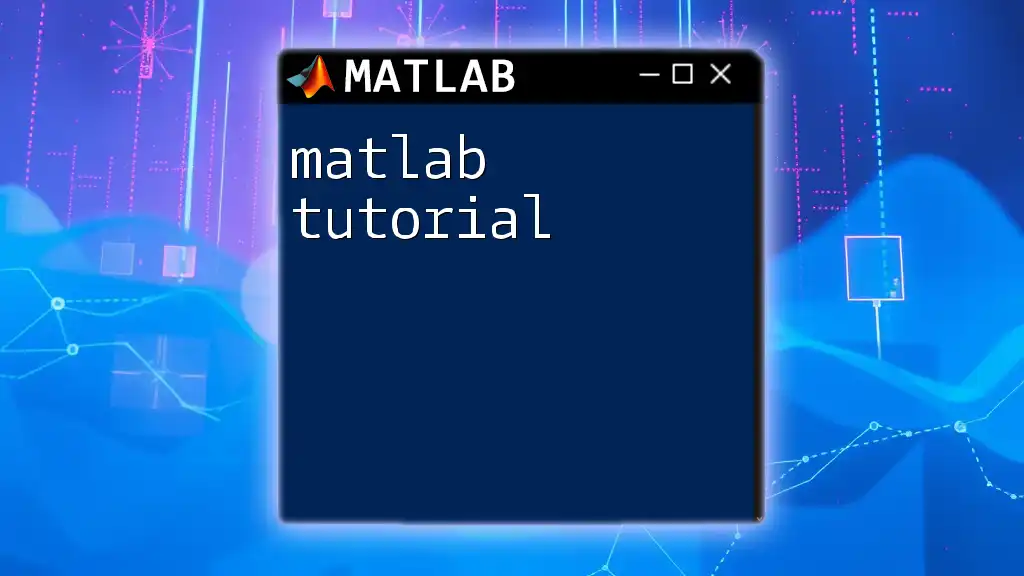
Manipulating Structs
Looping Through Structs
Iterating over struct arrays allows you to perform operations on each struct systematically. For instance, to print the names of all students, you can use a loop:
for i = 1:length(students)
disp(['Student Name: ', students(i).name]);
end
This loop will display the names of each student in the `students` array.
Combining Structs
Sometimes, you may need to merge two structs into one. You can achieve this by using functions like `fieldnames` and `getfield`. Here’s an example:
mergedStudent = cat(1, student1, student2); % Combining two student structs
This combines the `student1` and `student2` structs into a single `mergedStudent` struct array.
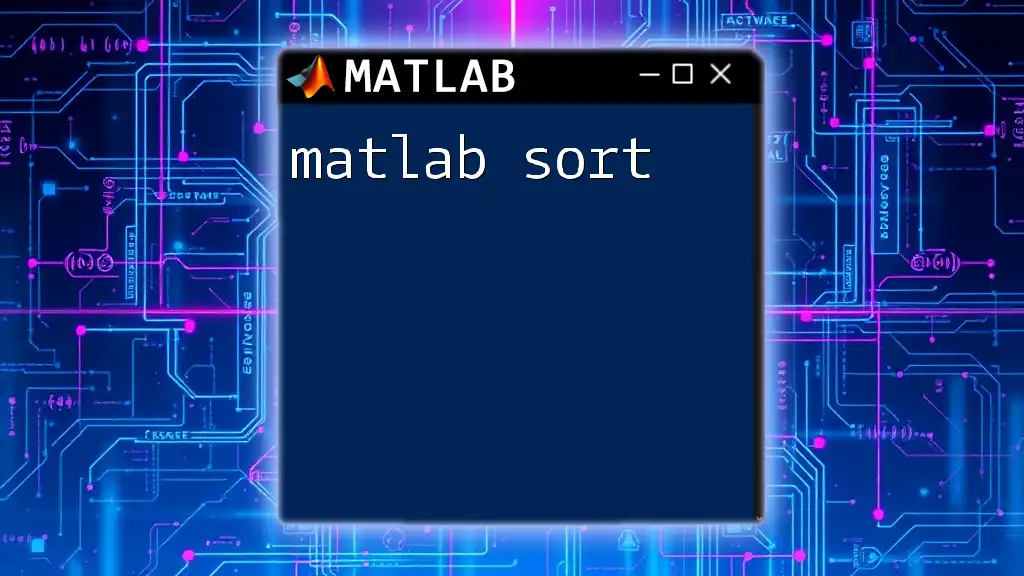
Common Operations on Structs
Sorting Structs
Sorting a struct array based on a specific field can be accomplished easily. For example, if you want to sort students by their age, use the following:
[~, idx] = sort([students.age]);
sortedStudents = students(idx);
This technique sorts the `students` array in ascending order based on their ages.
Filtering Structs
You can filter a struct array based on conditions by leveraging logical indexing. For example, to get students with a GPA greater than 3.5, you would write:
filteredStudents = students([students.gpa] > 3.5);
This creates a new array that includes only those students who meet the specified GPA condition.
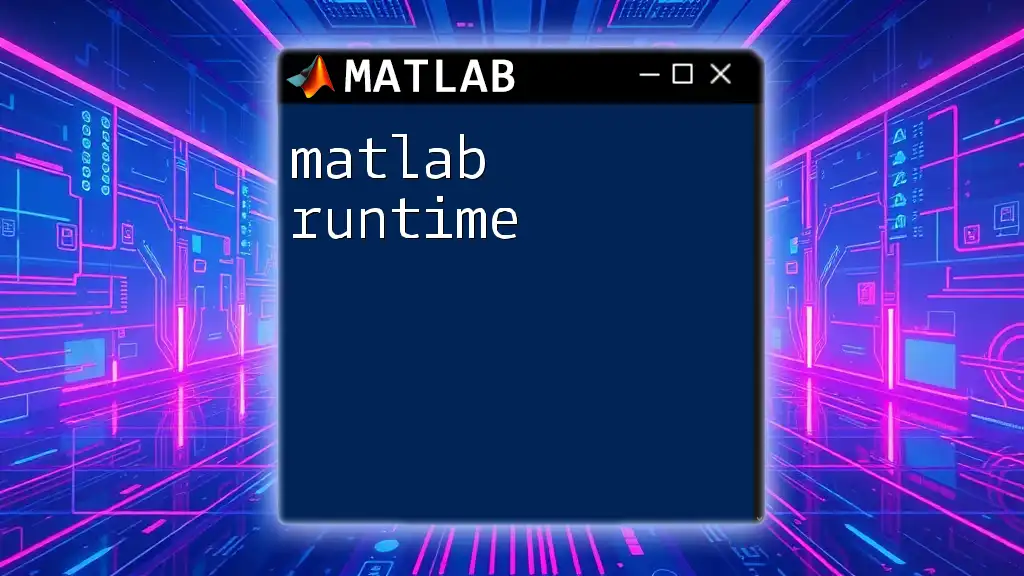
Best Practices with MATLAB Structs
Struct Naming Conventions
When creating structs, maintaining clear and consistent naming conventions is crucial. Use descriptive names for both structs and fields to improve code readability and maintainability. For instance, preferring `studentRecord` over `sr`.
When Not to Use Structs
While structs are powerful, they may not be the best choice in every scenario. If you require fixed-size homogeneous data, consider using arrays or other data types for efficiency. Structs incur some overhead in terms of access speed compared with direct array indexing.
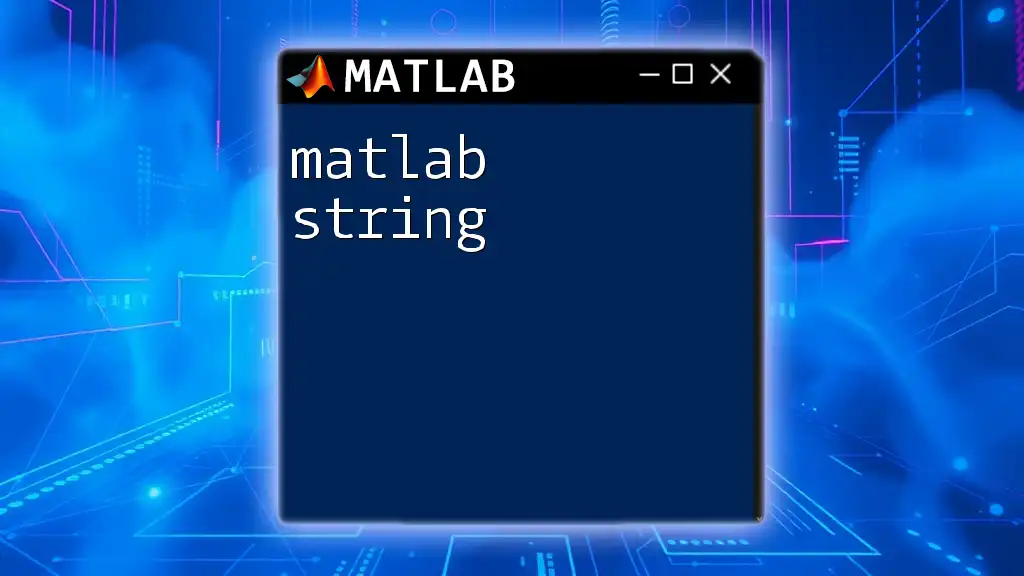
Conclusion
Structs in MATLAB are invaluable for managing and organizing complex data structures. By mastering the use of structs, you equip yourself with a powerful tool for data handling in various applications. Experiment with creating, accessing, modifying, and manipulating structs to solidify your understanding. Keep learning and practicing to maximize your MATLAB skills!
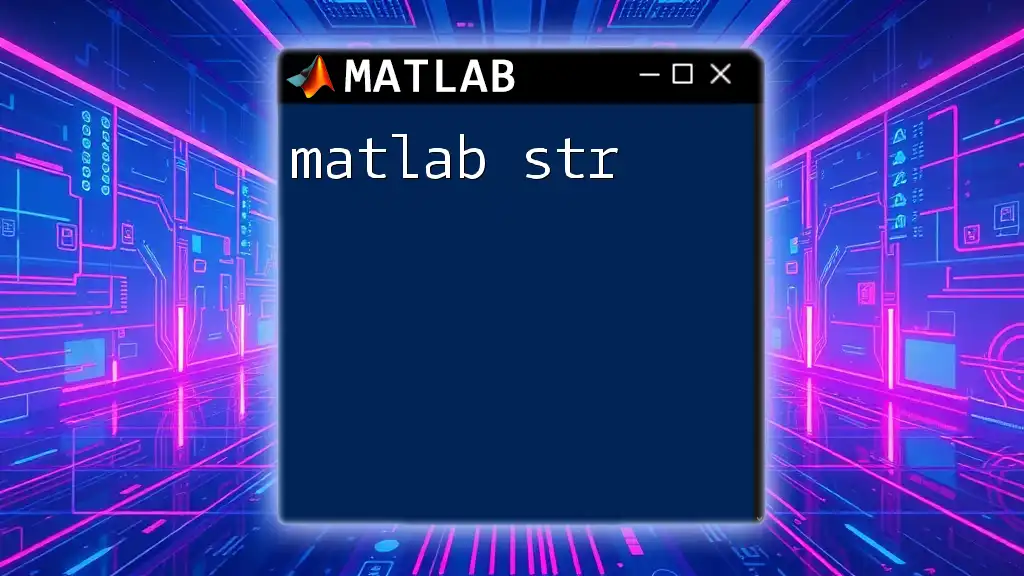
Further Resources
For more in-depth understanding, consult the official MATLAB documentation on structs, and explore multimedia resources like tutorial videos that illustrate various functionalities of MATLAB structs in action.