In MATLAB, the `datetime` function is used to create, manipulate, and display date and time values in a format that is easily understandable and suitable for various applications.
Here’s a quick example of how to create a `datetime` object:
dt = datetime('2023-10-15', 'InputFormat', 'yyyy-MM-dd');
Understanding MATLAB Datetime
What is Datetime?
In MATLAB, a datetime is a data type specifically designed to handle date and time information. Unlike traditional numerical representations of time, the datetime type allows for a more intuitive and user-friendly approach. It encapsulates the complexities of different date formats, time zones, and calendar rules, which are essential for effective date manipulation and analysis.
Creating Datetime Arrays
The foundation of using `matlab datetime` starts with creating datetime arrays. This can be achieved through several methods that give you flexibility depending on your data source:
-
Using the `datetime` Function: This function is straightforward and accepts year, month, and day as arguments. For instance:
dt1 = datetime(2023, 10, 1); % Creates a datetime for October 1, 2023
-
Using String Input: The `datetime` function can also interpret strings that represent dates. For example:
dt2 = datetime('2023-10-01'); % Creates a datetime from a string
-
Specifying Formats with Datetime: You might need to create datetime objects from strings that are not in a default format. MATLAB allows you to specify the format:
dt3 = datetime('01-Oct-2023', 'InputFormat', 'dd-MMM-yyyy');
By utilizing these methods, you can quickly convert different forms of date representations into standardized datetime objects.
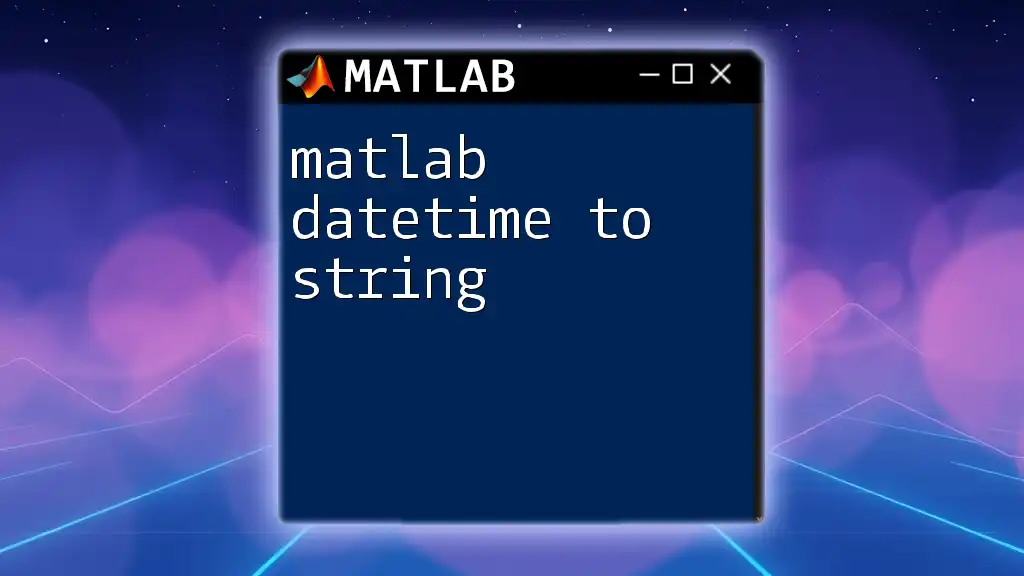
Manipulating Datetime Arrays
Adding and Subtracting Time
One of the most powerful features of the `matlab datetime` functionality is its capability to manipulate dates easily.
-
Addition of Days, Months, and Years: By leveraging MATLAB's built-in functions, adding time becomes straightforward:
futureDate = dt1 + caldays(10); % Adds 10 days to dt1
This allows for easy calculations such as deadlines or estimating future events.
-
Subtraction to Find Duration: You can also subtract datetime objects to calculate the duration between two dates:
duration = dt1 - dt2; % Finds the duration between two datetimes
The resulting duration can be in days, hours, or any other time unit, facilitating various time-based analyses.
Comparing Datetime Values
With `matlab datetime`, comparing dates is as intuitive as you would expect. You can directly use relational operators such as `<`, `>`, and `==` to determine chronological order:
isEarlier = dt1 < dt2; % Checks if dt1 is earlier than dt2
This simplifies tasks such as filtering datasets based on date criteria.
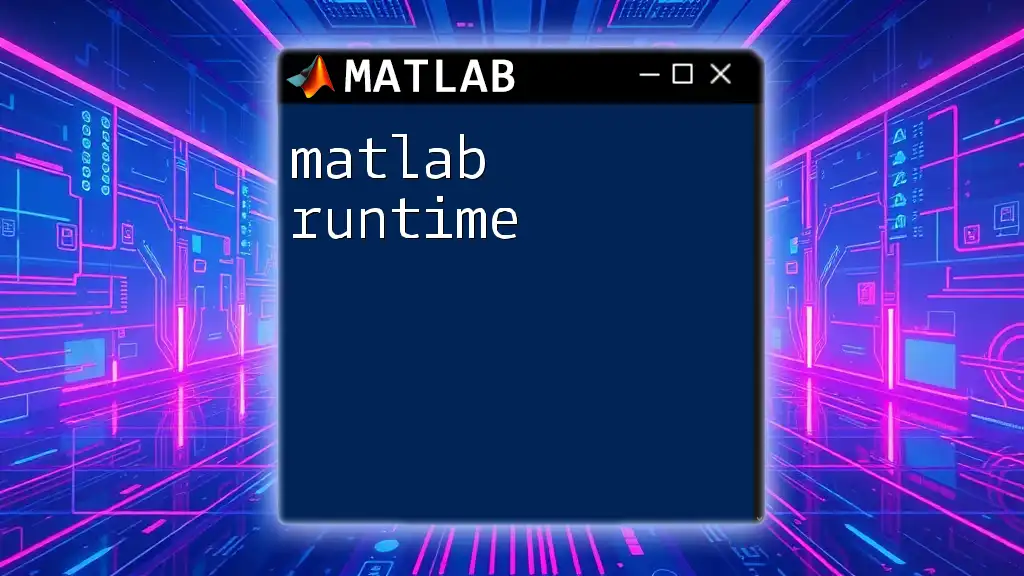
Formatting and Displaying Datetime
Customizing Date and Time Formats
MATLAB provides various options to customize the display format of your datetime objects. This is crucial when presenting data, as the format can dramatically affect readability and understanding.
dt1.Format = 'dd-MM-yyyy HH:mm:ss'; % Changes the format of dt1
Familiarizing yourself with the available formatting options (like 'dd', 'MM', 'yyyy', 'HH') will help you represent dates according to your needs, whether for reports, visualizations, or user interfaces.
Converting Datetime to String
When working with data that may need to be exported or displayed, you often need to convert datetime objects to strings. MATLAB's `datestr` function handles this task:
dateString = datestr(dt1); % Converts dt1 to string format
This conversion is especially useful when integrating MATLAB with other software or report formats that rely on string representations of dates.
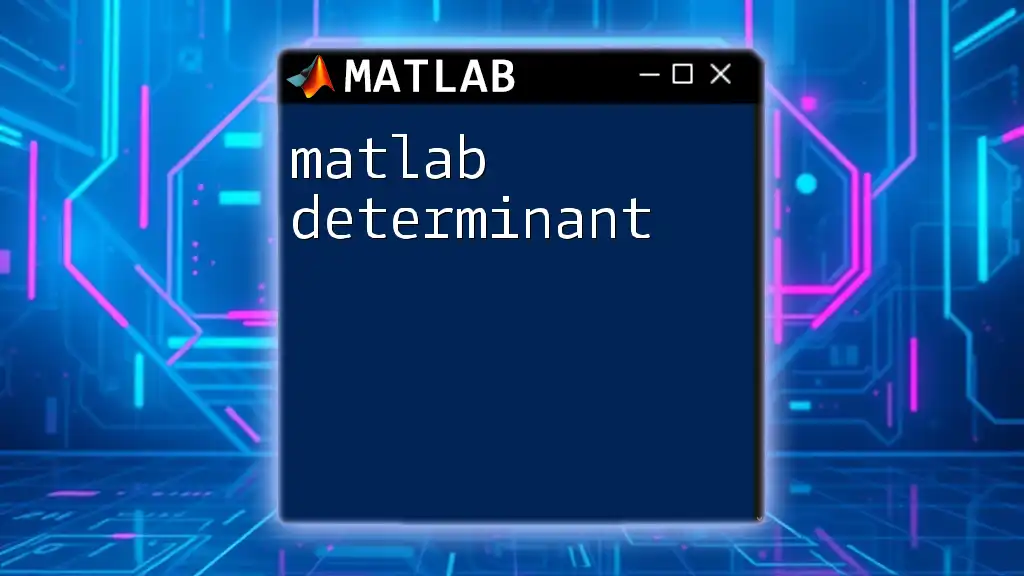
Working with Time Zones
Setting and Converting Time Zones
In today’s interconnected world, often datasets contain information from different time zones. The datetime type in MATLAB can accommodate this complexity.
Understanding Time Zones: Every datetime object can be assigned a time zone, which is crucial for accurate calculations and comparisons.
- Setting Time Zones: By specifying a time zone, you help MATLAB handle date and time correctly. For example:
dt1.TimeZone = 'America/New_York'; % Sets the timezone of dt1
- Converting between Time Zones: If you have a datetime object and need to adjust it to a different time zone, you can do so effortlessly:
dt2 = datetime('2023-10-01', 'TimeZone', 'UTC'); % Creates UTC datetime
dt2.TimeZone = 'America/Los_Angeles'; % Changes the timezone
This capability allows for seamless global data analysis.
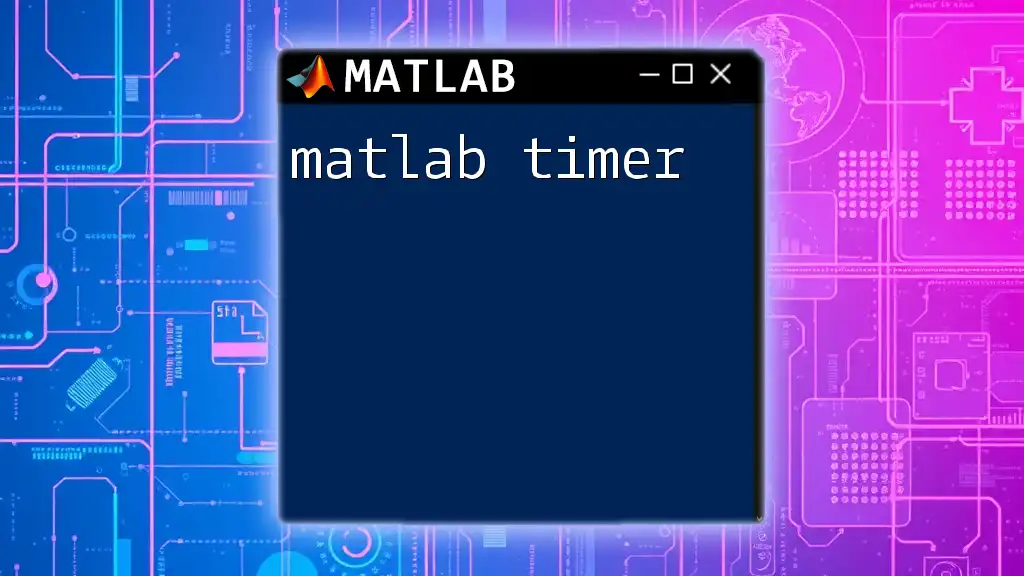
Examples of Practical Applications
Time Series Analysis in MATLAB
When it comes to analyzing time-based datasets, `matlab datetime` allows you to handle time series data with ease. By creating a datetime array, you can visualize trends over time, which can reveal important insights. Here’s a simple example:
t = datetime(2022,1,1:10); % Dates from January 1, 2022, to January 10, 2022
y = rand(1,10); % Random data points
plot(t, y); % Plots the time series
This plot helps illustrate how values change over a specified period.
Scheduling and Timing Tasks
The ability to track and schedule tasks is another advantageous use of MATLAB’s datetime capabilities. For instance, you can implement a simple countdown timer:
startTime = datetime('now'); % Records the current time
pause(5); % Simulates some task
endTime = datetime('now'); % Records the time after the task
fprintf('Task took %s seconds\n', char(endTime - startTime)); % Displays elapsed time
This functionality can be pivotal for performance testing or monitoring intervals between executions.
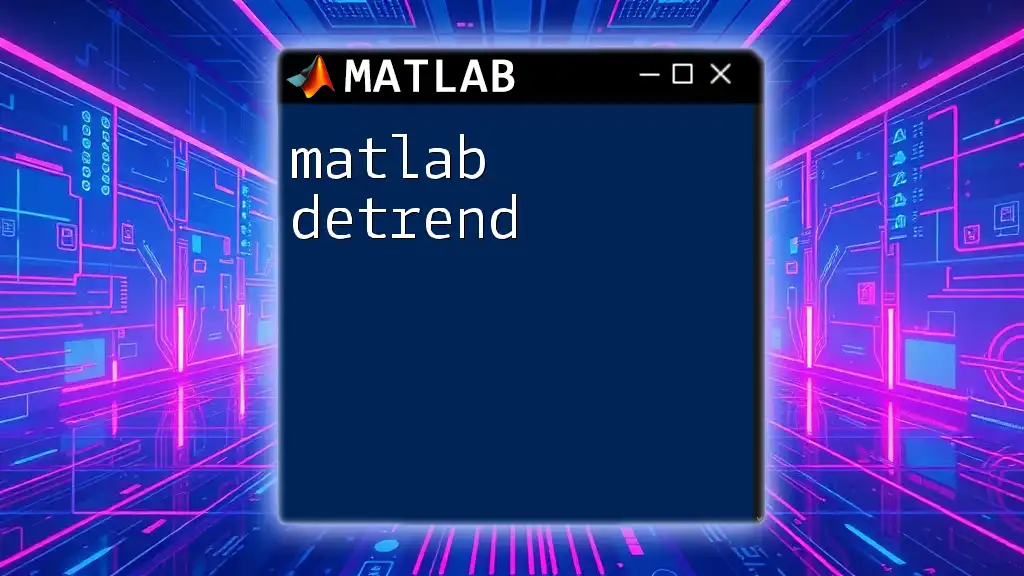
Troubleshooting Common Issues
Common Errors in Datetime Operations
Despite its user-friendliness, working with `matlab datetime` can sometimes lead to errors. One common issue arises from incorrect formats when creating datetime objects. Ensuring that the input format matches the provided string can prevent runtime errors.
Performance Tips for Large Datetime Arrays
If you are dealing with large datasets, performance issues may arise. One way to optimize your performance is by preallocating datetime arrays, which can save time on data manipulation tasks. Instead of growing the array dynamically, initialize it with a fixed size upfront for better performance. This is particularly useful in loops involving many datetime calculations.
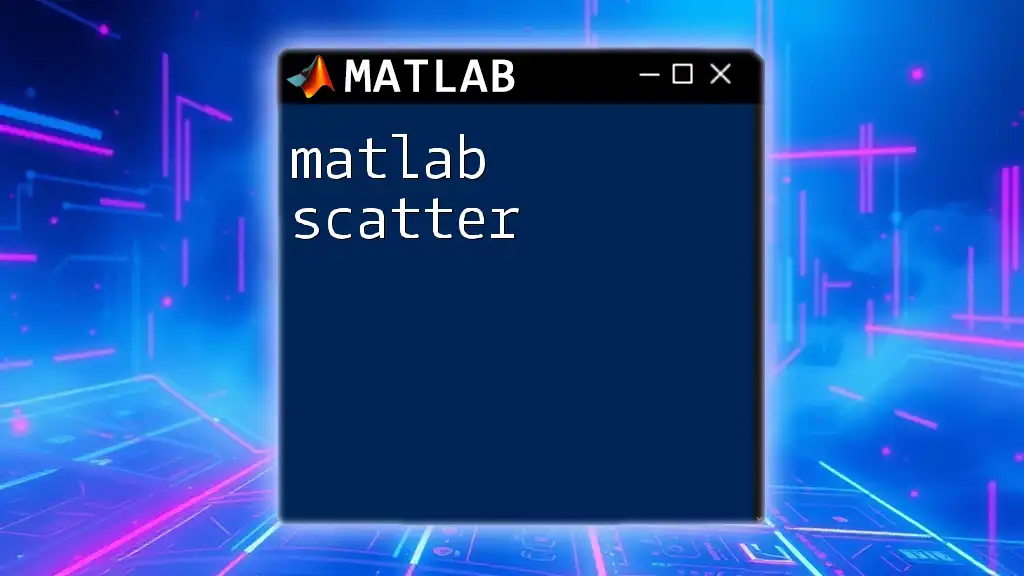
Conclusion
In summary, the `matlab datetime` functionality is a powerful tool for handling date and time data in MATLAB. Understanding how to create, manipulate, and present datetime objects can enhance your programming capabilities significantly. By exploring the various features provided by MATLAB, you can streamline your analysis and gain deeper insights into your data. As you continue your journey, don't hesitate to explore further and master the intricacies of `matlab datetime`.
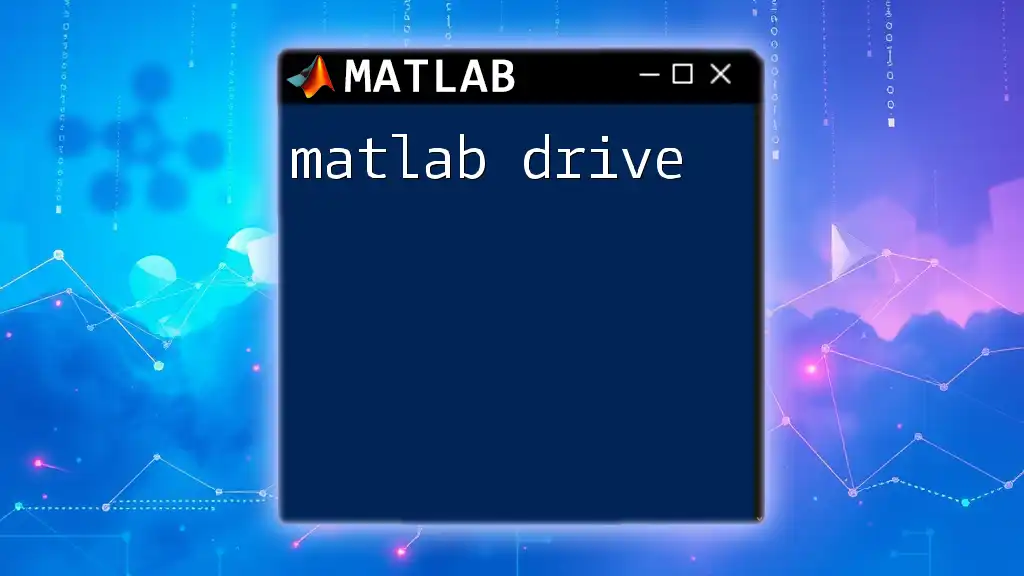
Additional Resources
To deepen your understanding, refer to the official MATLAB documentation and engage with online communities or forums dedicated to MATLAB programming. Exploring referenced books and courses will also enhance your skills and knowledge in using MATLAB effectively.