The `ttest` function in MATLAB performs a t-test to determine if there is a significant difference between the means of two datasets. Here’s a simple code snippet to illustrate its use:
% Sample data
data1 = [23, 21, 18, 25, 22];
data2 = [20, 19, 21, 24, 18];
% Perform a t-test
[h, p] = ttest2(data1, data2);
% Display results
fprintf('Hypothesis test result: %d\n', h);
fprintf('P-value: %.4f\n', p);
Understanding t-test
What is a t-test?
A t-test is a statistical method used to determine if there is a significant difference between the means of two groups. In the context of hypothesis testing, it helps researchers assess whether their assumptions (hypotheses) hold true or should be rejected based on sample data. The key components of a t-test include:
- Null Hypothesis (\(H_0\)): This typically states that there is no difference between the groups or that a sample mean is equal to a known mean.
- Alternative Hypothesis (\(H_a\)): This posits that there is a significant difference between groups or that the sample mean differs from a known mean.
- Significance Level (\(\alpha\)): Commonly set at 0.05, this threshold determines the excitement of rejecting the null hypothesis based on the p-value.
Types of t-tests
- One-sample t-test: Assesses whether the mean of a single sample differs from a specific known value.
- Independent two-sample t-test: Compares the means of two independent groups.
- Paired t-test: Evaluates means from the same group at different times, suited for before-and-after assessments.
When to Use t-tests?
Understanding when to apply a t-test is crucial. You should consider the type of data and how it was collected. Here are a few scenarios:
- One-sample t-test is useful when comparing the mean of a sample against a known population mean, such as testing a new drug's effectiveness against a standard treatment.
- Independent two-sample t-tests are used when comparing two distinct groups, for example, studying exam scores between two different classes.
- Paired t-tests are ideal for experiments where the same subjects are tested under two different conditions, such as measuring weight loss before and after a diet program.
Additionally, it is vital to check assumptions related to data. Specifically, you should ensure the data is roughly normally distributed and that groups have similar variances when necessary.
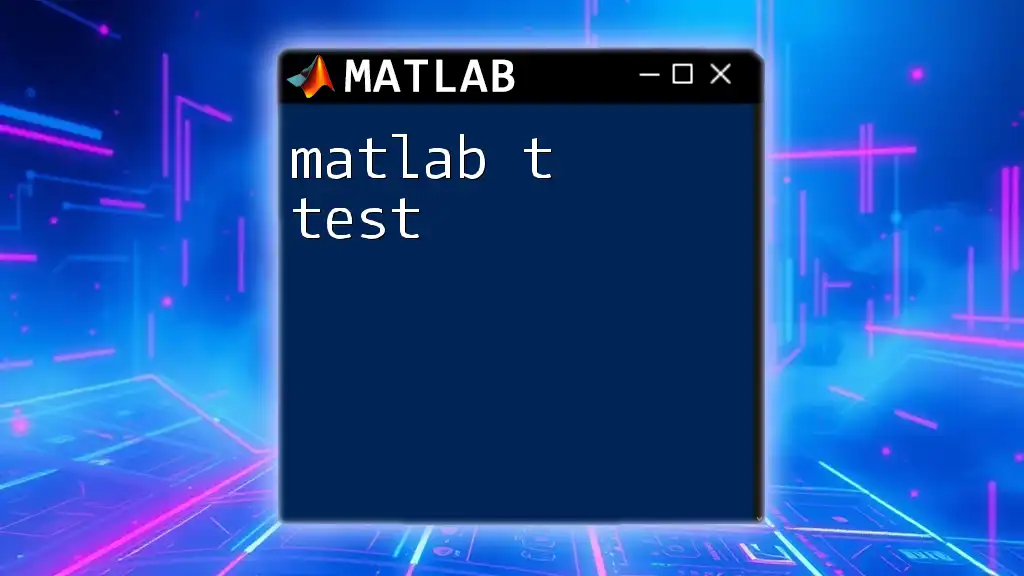
MATLAB's ttest Function
Overview of the ttest Function
The `ttest` command in MATLAB simplifies the t-testing process. The basic syntax is:
h = ttest(x, mu)
Where:
- `x`: your sample data.
- `mu`: the population mean you are comparing against.
Moreover, the function returns `h`, which indicates whether to reject the null hypothesis (1) or fail to reject it (0).
One-Sample t-test
Explanation and Use Case
A one-sample t-test is effective when testing whether a sample mean is significantly different from a known value. For instance, if you have student exam scores, you might want to know if the average score differs from a national average.
Example Code Snippet
data = [1.2, 2.3, 3.4, 2.5, 3.3]; % Sample data
mu = 2; % Population mean
[h, p] = ttest(data, mu);
fprintf('Hypothesis Test Result: %d, p-value: %.4f\n', h, p);
Detailed Explanation of the Example
In this code:
- `data` represents your sample scores.
- `mu` is the population mean for comparison. The `ttest` function evaluates if the mean of `data` significantly differs from `mu` and supplies a p-value (`p`). If `h = 1`, you reject \(H_0\), indicating a significant difference.
Independent Two-Sample t-test
Explanation and Use Case
The independent two-sample t-test compares the means from two distinct groups to determine if they differ. It is useful in experiments where subjects fall into different groups entirely, such as male vs. female heights.
Example Code Snippet
group1 = [1.2, 2.3, 3.4, 2.5, 3.3]; % Sample data from group 1
group2 = [2.3, 3.5, 3.8, 3.7, 4.0]; % Sample data from group 2
[h, p] = ttest2(group1, group2);
fprintf('Hypothesis Test Result: %d, p-value: %.4f\n', h, p);
Detailed Explanation of the Example
The `ttest2` function computes results based on the following:
- `group1` and `group2` represent the two independent datasets.
- A p-value helps gauge whether the difference in means is statistically significant. Should `h = 1`, it suggests a significant discrepancy between the groups.
Paired t-test
Explanation and Use Case
The paired t-test is effective for measuring the same subjects under varied conditions. It’s commonly applied in pre-and post-tests where participants are measured before and after an intervention.
Example Code Snippet
before = [1.2, 2.3, 3.4, 2.5, 3.3]; % Measurements before treatment
after = [2.5, 2.7, 3.9, 2.8, 3.6]; % Measurements after treatment
[h, p] = ttest(before, after);
fprintf('Hypothesis Test Result: %d, p-value: %.4f\n', h, p);
Detailed Explanation of the Example
In this case:
- `before` and `after` are two paired measurements.
- The `ttest` function evaluates whether the differences in measurements before and after treatment are statistically significant. Again, if `h = 1`, the null hypothesis may be rejected.
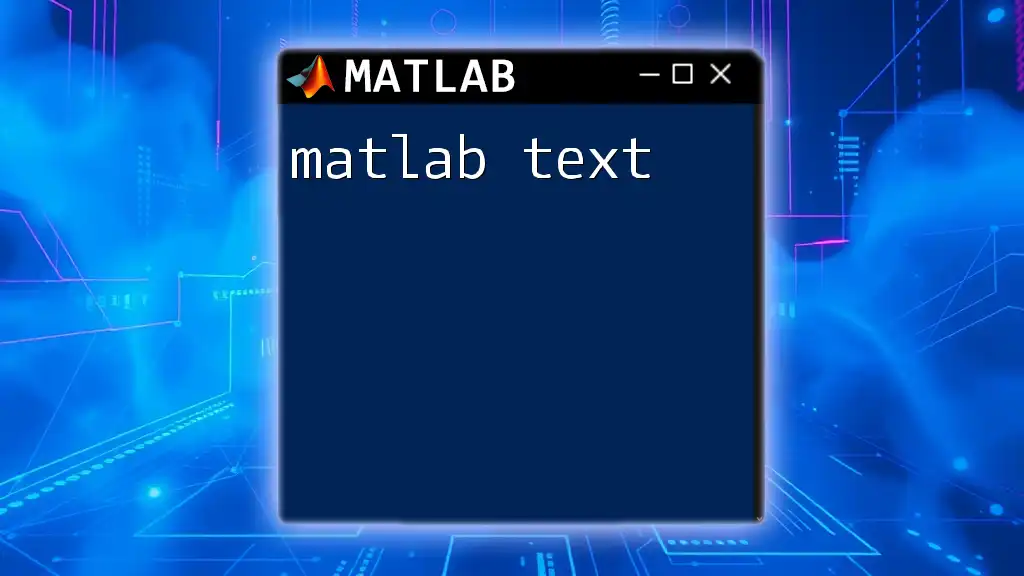
Interpretation of Results
Understanding p-values and Hypothesis Outcomes
The p-value represents the probability of observing the data, or something more extreme, under the assumption that the null hypothesis is correct. In the context of t-tests:
- A low p-value (typically < 0.05) indicates strong evidence against the null hypothesis, leading to its rejection.
- A high p-value suggests a lack of evidence to reject \(H_0\), supporting that any observed difference could be due to random chance.
Practical Considerations
While executing t-tests, avoid common pitfalls such as:
- Non-normality: Assess data with normality tests or visualizations before applying t-tests.
- Unequal Variances: This can be managed by verifying assumptions or using options in `ttest` like 'Vartype', which allows for handling heteroscedastic data.
Visualizing Data
Visual representation of data can enhance interpretation. Creating boxplots or histograms is beneficial for displaying sample distributions and comparing means.
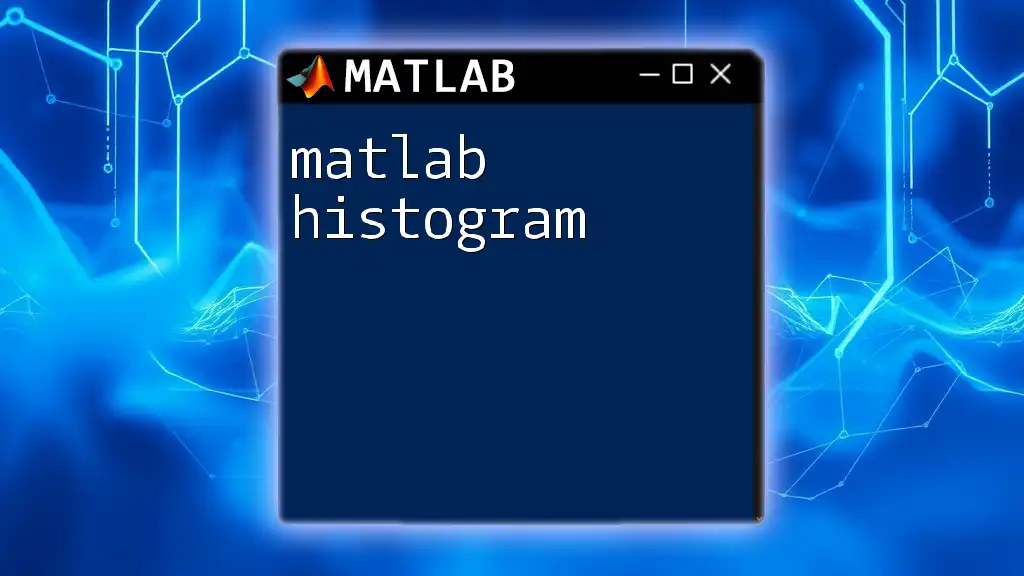
Conclusion
The MATLAB ttest function is a powerful tool for conducting hypothesis tests in various research contexts. By mastering the methodology and applications of one-sample, independent two-sample, and paired t-tests, you can deepen your analytical skills and derive meaningful conclusions from your data.
Embrace the practice opportunities with diverse datasets to solidify your understanding and proficiency with `ttest`. Consider exploring additional resources, including MATLAB documentation and online courses, to further enhance your statistical analysis capabilities.
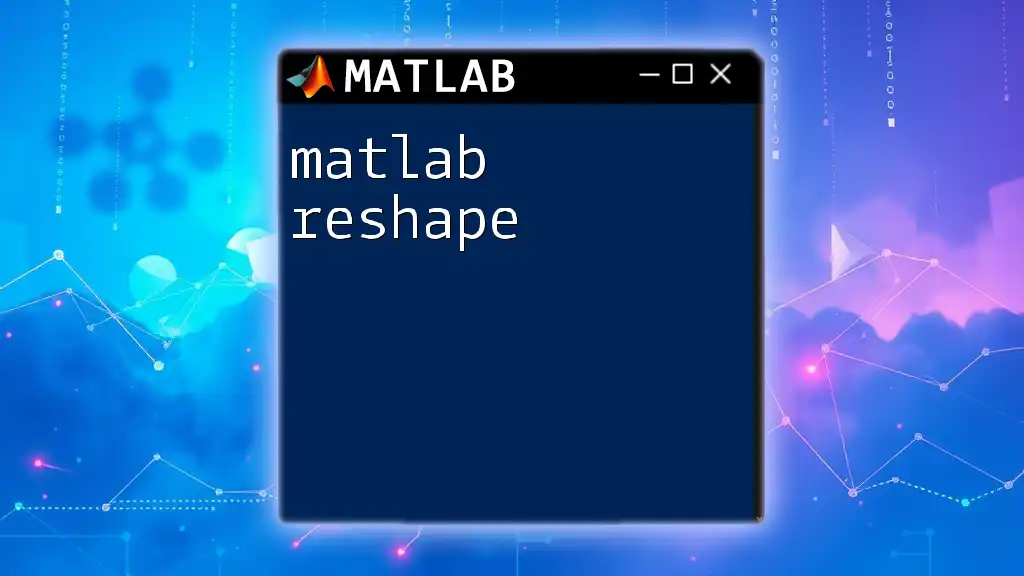
Additional Resources
- Books, articles, and online courses related to statistics and MATLAB functions.
- Direct links to MATLAB’s documentation for `ttest` and related commands.
- Community forums and support groups for MATLAB users seeking assistance or collaboration.
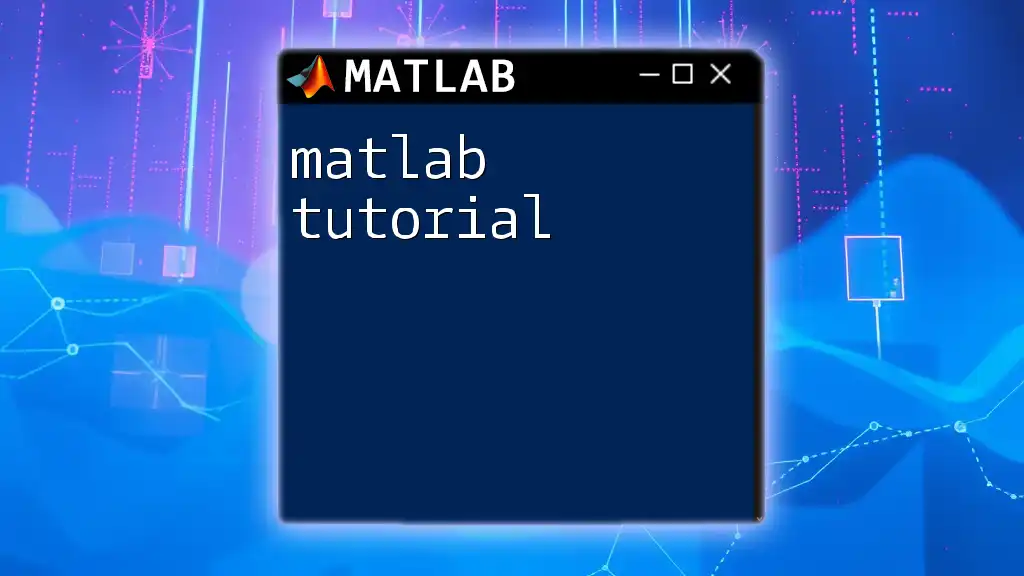
FAQs about MATLAB ttest
Here are some common questions surrounding the MATLAB ttest function and its application in statistical analysis. This section can provide clarity and further support for users navigating the nuances of t-tests in MATLAB.