A MATLAB vector is a one-dimensional array that can represent a row or a column of numbers, allowing for efficient mathematical operations and data manipulation.
Here’s a simple example of how to create and display a row vector in MATLAB:
% Create a row vector
rowVector = [1, 2, 3, 4, 5];
disp(rowVector);
What is a Vector?
In the context of MATLAB, a vector is a one-dimensional array that can hold multiple values. Vectors are fundamental for mathematical computations and data representation, serving as a primary data structure in MATLAB. You can think of a vector as a list of numbers organized in a specific order.
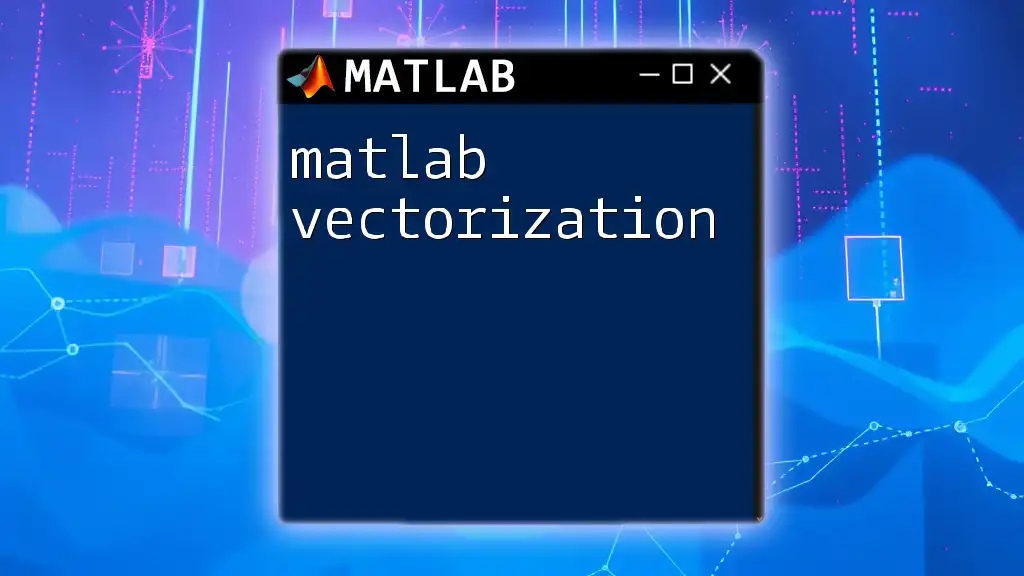
Types of Vectors in MATLAB
Vectors can generally be classified into two main types: row vectors and column vectors.
Row Vectors
A row vector is essentially a single row of data, represented using square brackets with elements separated by spaces or commas. For example, a row vector can be defined as:
rowVector = [1, 2, 3, 4, 5];
Column Vectors
Conversely, a column vector consists of a single column of data. This can be created by using semicolons to separate elements. An example of a column vector is:
colVector = [1; 2; 3; 4; 5];
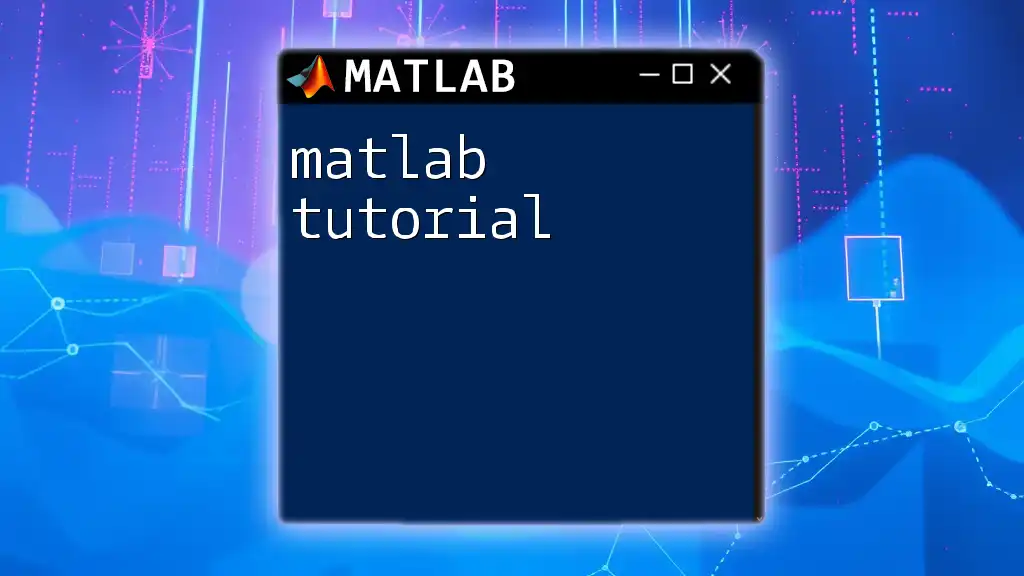
Creating Vectors in MATLAB
Using MATLAB Commands to Create Vectors
You can create vectors in various ways within MATLAB, making it a flexible and powerful tool for data manipulation.
Using the Colon Operator
The colon operator `:` is one of the simplest ways to generate a sequence of numbers. The basic syntax is:
v = start:increment:end;
For example:
v = 1:5;
This will generate the vector `[1, 2, 3, 4, 5]`.
Using the `linspace()` Function
The `linspace()` function is beneficial when you want to create a vector with specified spacing. The syntax is as follows:
v = linspace(start, end, numberOfElements);
For instance, to create a vector containing five equally spaced numbers between 0 and 10:
v = linspace(0, 10, 5);
This generates the output `0 2.5 5 7.5 10`.
Using the `zeros()` and `ones()` Functions
If you require vectors filled with zeros or ones, the `zeros()` and `ones()` functions are invaluable. Here’s how you can create such vectors:
zeroVector = zeros(1, 5); % Creates a row vector of five zeros
oneVector = ones(1, 5); % Creates a row vector of five ones
These functions are often useful for initializing vectors before performing computations or data aggregation.
Modifying Existing Vectors
Changing Elements in a Vector
Once a vector is created, you can modify its elements. Access elements using their indices, like so:
v(2) = 10;
This code changes the second element of the vector `v` to 10. If `v` originally contained `[1, 2, 3, 4, 5]`, it will now be `[1, 10, 3, 4, 5]`.
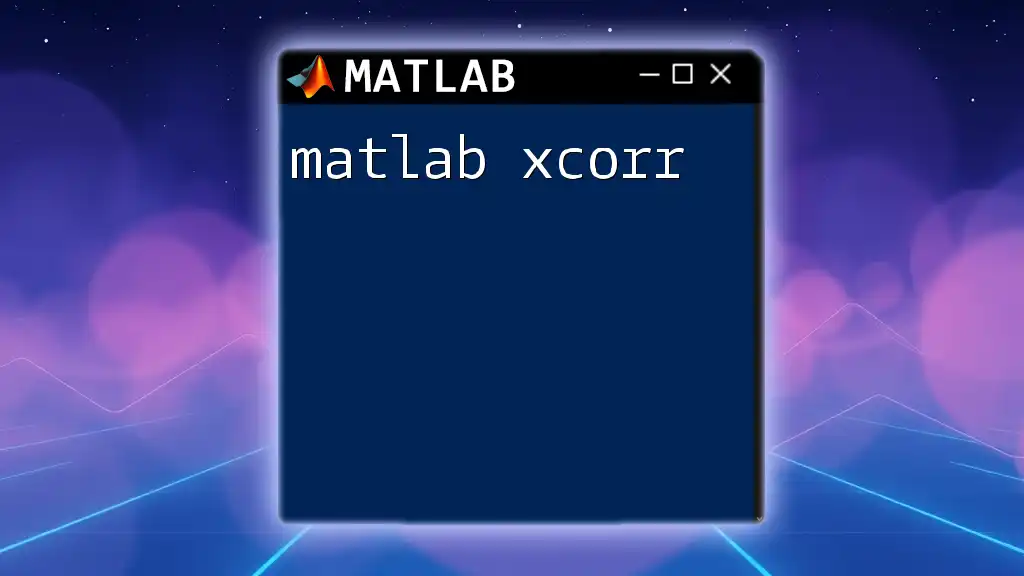
Accessing and Manipulating Vector Elements
Indexing Vectors
MATLAB allows for various ways to access and manipulate vector elements.
Basic Indexing
You can access elements using their position in the vector. For example:
element = v(3);
This code retrieves the third element from vector `v`. You can also access multiple elements:
subset = v(1:3);
This will return the first three elements.
Logical Indexing
Logical indexing enables selection based on conditions. For instance, if you want to extract all elements greater than 2:
filteredElements = v(v > 2);
This command generates a new vector containing only the elements that satisfy the condition.
Vector Operations
MATLAB supports various arithmetic operations on vectors.
Arithmetic Operations on Vectors
You can perform element-wise addition, subtraction, multiplication, and division easily. For example:
A = [1, 2, 3];
B = [4, 5, 6];
sumVec = A + B; % Element-wise addition
diffVec = A - B; % Element-wise subtraction
prodVec = A .* B; % Element-wise multiplication
divVec = A ./ B; % Element-wise division
Dot Product and Cross Product
Vectors can also undergo mathematical operations like dot and cross products. The dot product of two vectors can be computed using the `dot()` function:
dotProduct = dot(A, B);
For cross products, use the `cross()` function:
crossProduct = cross(A, B);
Understanding these operations can help optimize many engineering and mathematical computations.
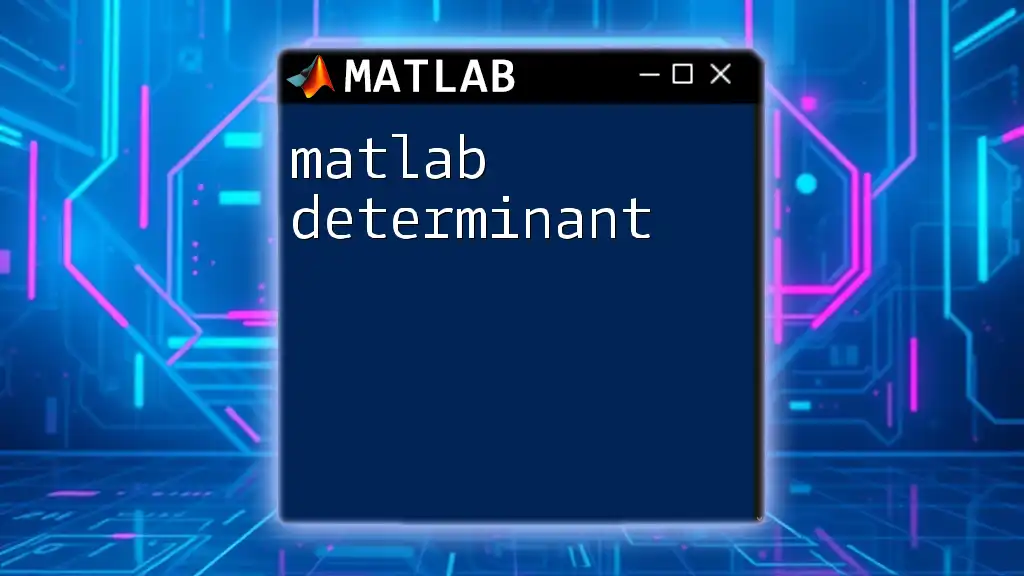
Common Functions for Vectors
Built-in Functions to Manipulate Vectors
MATLAB provides a wide array of built-in functions to help manipulate vectors smoothly.
Size and Length of Vectors
To determine the size and length of a vector, use `length()` and `size()` functions. For instance:
len = length(v); % Returns the number of elements in vector v
sz = size(v); % Returns the dimensions of vector v
Sorting and Finding Maximum/Minimum Values
You can sort the vector or find its maximum and minimum values using the following functions:
sortedVector = sort(v);
maxValue = max(v);
minValue = min(v);
These functions allow for efficient data analysis and quick retrieval of essential statistics.
Advanced Vector Functions
Using `unique()` to Find Unique Values
The `unique()` function is excellent for extracting unique elements from a vector, especially if dealing with repeated values:
uniqueValues = unique(v);
This will return a new vector consisting only of unique elements from `v`.
Using `cumsum()` and `cumprod()`
The `cumsum()` function computes the cumulative sum, while `cumprod()` computes the cumulative product of a vector:
cumsumVector = cumsum(v);
cumprodVector = cumprod(v);
These functions are particularly useful in scenarios needing cumulative data calculations.
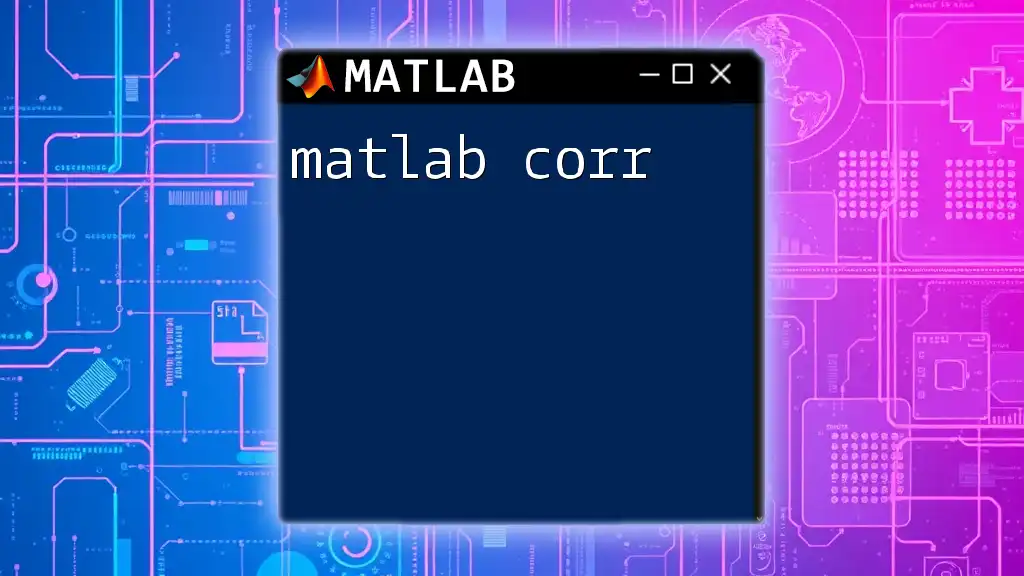
Applications of Vectors in MATLAB
Real-World Applications
Vectors find their footing in various practical applications, including data representation, where they can represent series of measurements or states within scientific computing, engineering simulations, and statistical analyses.
Signal Processing
In signal processing, vectors are pivotal for representing and analyzing signals. They can be used to represent time-domain signals, frequency representations, and more complex data processing tasks.
Visualizing Vectors
MATLAB also allows for robust visualization of vector data using plotting functions. You can create simple plots to visualize your vector data effectively.
For example, crafting a stem plot or a line plot of the vector values can help visualize the data distribution:
stem(v);
title('Stem Plot of Vector v');
xlabel('Index');
ylabel('Value');
This basic visualization technique helps demonstrate data characteristics effectively.
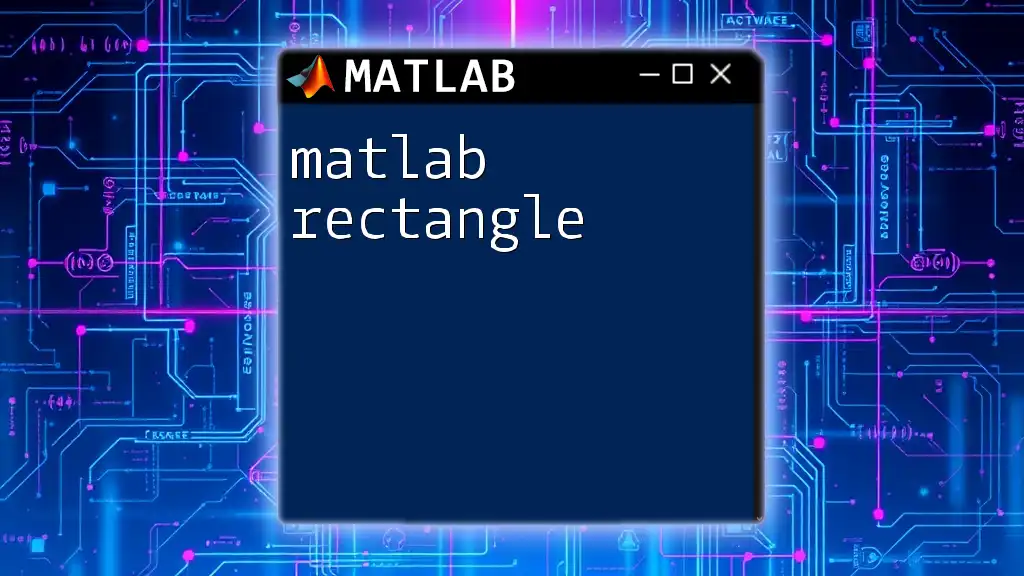
Conclusion
In this comprehensive guide to MATLAB vectors, we explored everything from their fundamentals to advanced manipulations and functions. Understanding vectors is crucial for efficient data analysis and mathematical computations in MATLAB.
By grasping the concepts and functionalities surrounding vectors, you equip yourself with powerful tools that can enhance your capabilities in programming and engineering tasks. Further exploration of sophisticated vector operations and applications will only improve your proficiency in MATLAB as a whole.
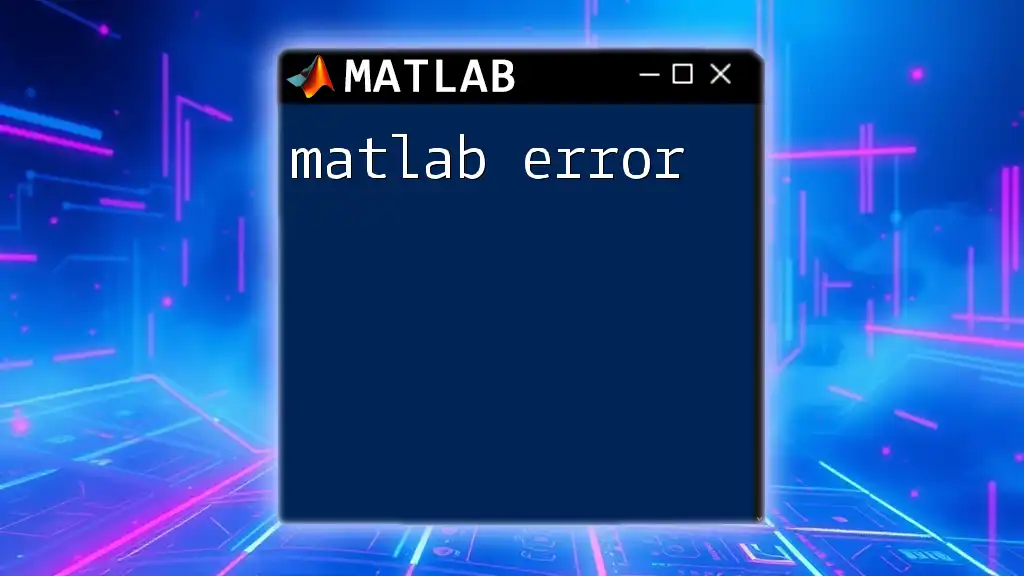
Additional Resources
For anyone looking to deepen their understanding of MATLAB, consider referring to the official MATLAB documentation. You can find various books and online tutorials that offer more detailed insights. Additionally, video resources available on platforms like YouTube can be beneficial for visual learners seeking practical demonstrations.