Certainly! The `unet3d` function in MATLAB is a three-dimensional U-Net architecture often used for semantic segmentation tasks in volumetric data, providing a robust method to capture spatial hierarchies in three-dimensional images.
Here's a simple code snippet demonstrating how to create a 3D U-Net in MATLAB:
% Define the input size for the 3D U-Net
inputSize = [64 64 64 1]; % [height, width, depth, channels]
% Create a 3D U-Net network
lgraph = unet3dLayers(inputSize, numClasses);
% Display the network architecture
analyzeNetwork(lgraph);
Understanding 3D U-Net
What is U-Net?
U-Net is a seminal neural network architecture primarily used for biomedical image segmentation. Its unique structure consists of an encoder-decoder configuration, which enables the model to learn both the context and the precise localization necessary for effective segmentation tasks. The innovative use of skip connections allows for the merging of features from earlier layers with those from later layers, ensuring that finer details are preserved during the upsampling process.
Evolution of 3D U-Net
The shift from 2D to 3D segmentation is crucial in the medical imaging field, where volumetric data is prevalent. While 2D U-Net configurations are sufficient for planar images, they fall short when it comes to 3D datasets like MRIs or CT scans. The 3D U-Net architecture retains the core principles of its 2D counterpart but adapts them for processing three-dimensional data, allowing for more accurate segmentation across multiple slices of data.
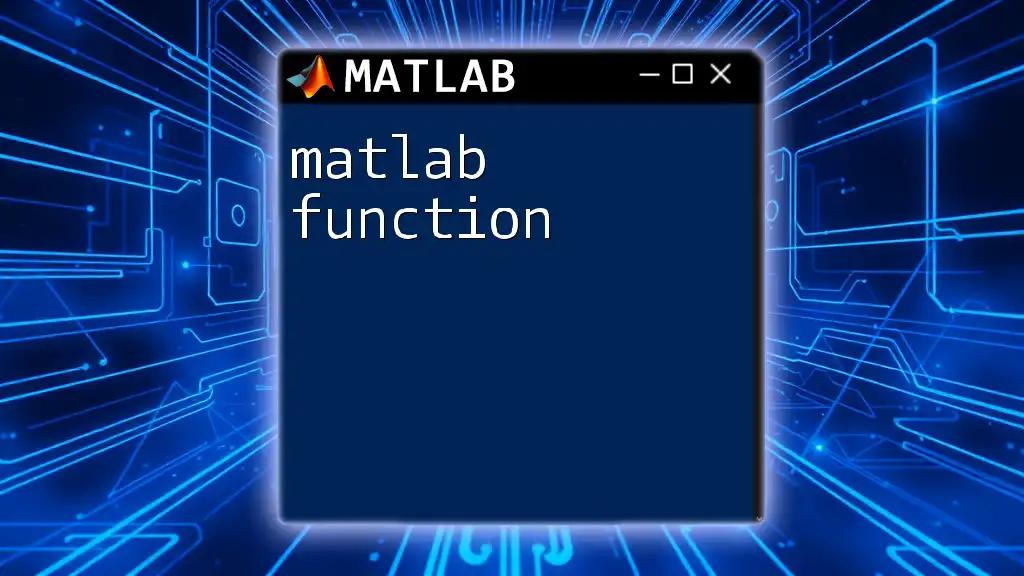
Setting Up Your MATLAB Environment
System Requirements
Before diving into using `matlab unet3d`, it's essential to ensure your system is prepared. The following requirements must be met:
- MATLAB Version: Ensure you are using a version that supports the Deep Learning Toolbox and Image Processing Toolbox.
- Hardware Specifications: A GPU is highly recommended for training deep learning models efficiently, especially for large datasets.
Installing Required Toolboxes
To utilize `matlab unet3d`, you need to verify that the necessary toolboxes are installed. Use the MATLAB Add-On Explorer to check available toolboxes and install any that are missing. Keeping your toolboxes up-to-date is vital for functionality and performance.
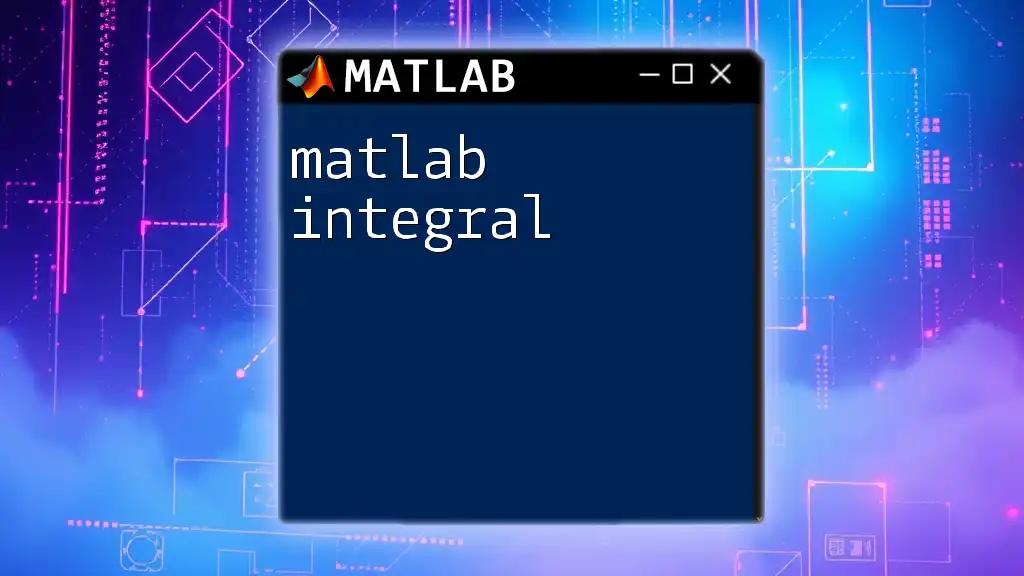
Preparing Your Data
Types of Data Suitable for 3D U-Net
The 3D U-Net architecture works best with volumetric data commonly found in medical imaging. This includes:
- MRI scans
- CT scans
- 3D ultrasound images
For supervised learning, annotated datasets are crucial, as having ground truth labels helps the model learn effectively.
Data Preprocessing Steps
Effective data preprocessing ensures that your input data is clean and standardized. Here are critical steps to follow:
- Resizing Images: Standardizing dimensions across all data prevents dimensional inconsistencies when feeding into the model.
- Normalization: This step helps in stabilizing the training process. Using techniques such as Min-Max scaling can significantly improve convergence.
- Data Augmentation: Augment your dataset artificially by applying transformations such as rotations, flips, and translations, which enhance model robustness.
Example: Code Snippet for Data Preprocessing
% Example code for normalization
image = imread('example_image.nii');
normalized_image = (image - min(image(:))) / (max(image(:)) - min(image(:)));
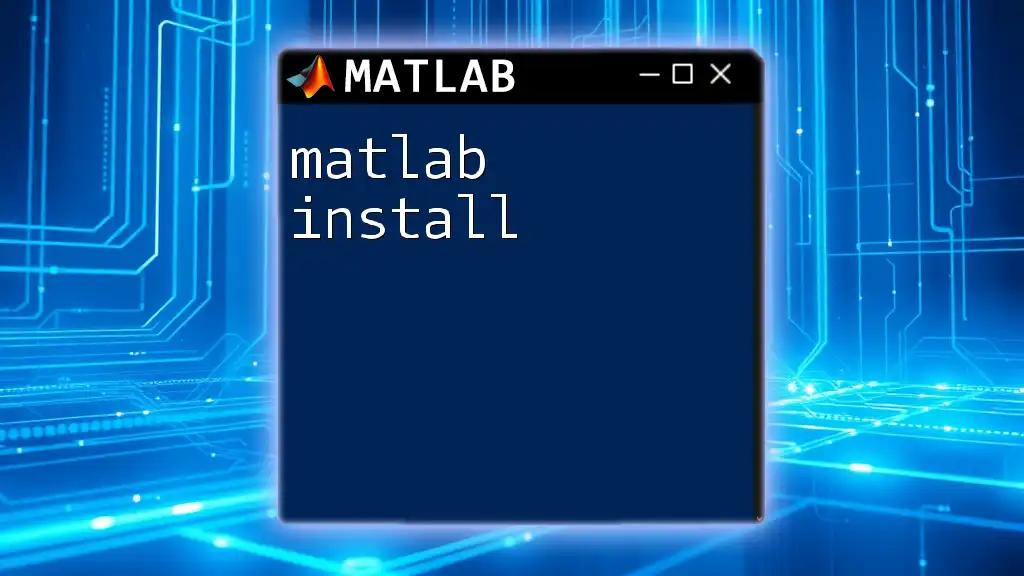
Building the u-net3d Model in MATLAB
Defining the Architecture
Creating a U-Net model in MATLAB involves defining its architecture. The architecture is typically formed by a series of convolutional layers, activation functions, and pooling layers. You can customize the number of filters and the depth of the layers based on the complexity of the data.
Example: Code to Define a 3D U-Net
% Example code to create 3D U-Net architecture
model = unet3d(inputSize, numClasses, 'InputChannels', 1);
Compilation of the Model
Once the architecture is defined, the next crucial step is to compile the model. This involves specifying the following elements:
- Loss Functions: Common choices include binary cross-entropy for binary classes and categorical cross-entropy for multi-class segmentation.
- Metrics: Selecting appropriate metrics like accuracy or Dice coefficient helps evaluate the model’s performance.
- Optimizers: Choose an optimizer such as Adam or Stochastic Gradient Descent (SGD) to enhance the model's learning capability effectively.
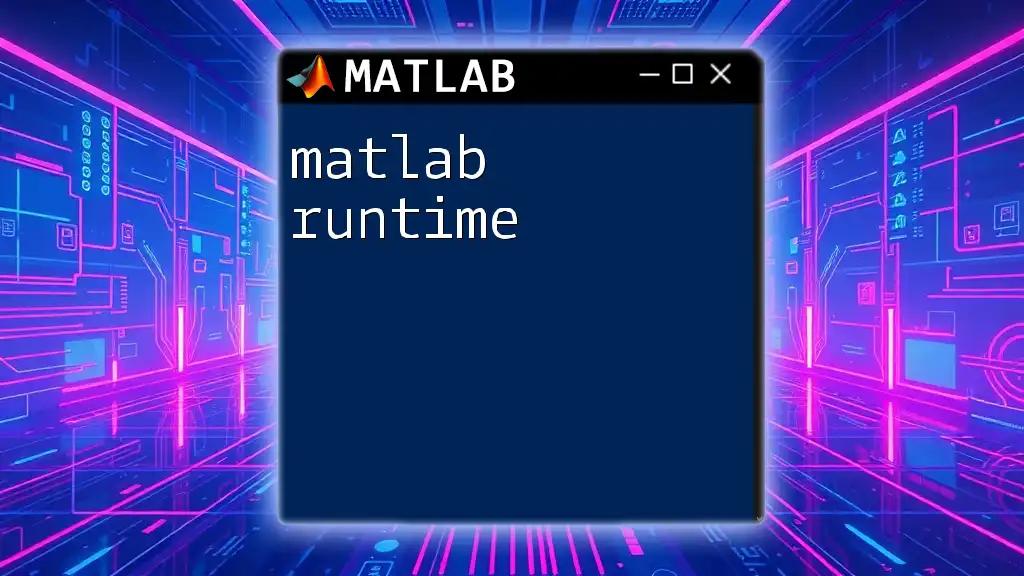
Training the Model
Configuring Training Options
Setting the right training options is vital for successful model training. Important parameters to configure include:
- Batch Size: Determines how many samples your model trains on before updating its weights.
- Number of Epochs: The number of complete passes through the training dataset.
- Learning Rate Schedule: Adjusting the learning rate during training to improve convergence.
Code Snippet: Setting Training Options
% Example code for training options
options = trainingOptions('adam', ...
'MaxEpochs', 50, ...
'MiniBatchSize', 4, ...
'Plots', 'training-progress');
Running the Training Process
Once you have set up your options, initiate the training using the `trainNetwork` function. During this phase, monitoring the training progress through MATLAB’s built-in plots allows you to diagnose potential issues like overfitting or underfitting.

Evaluating the Model Performance
Metrics for Performance Evaluation
After training, evaluating your model's performance is critical. Some key metrics include:
- Accuracy: The fraction of predictions where the model correctly identifies the class.
- Dice Coefficient: A statistical validation metric that gauges the overlap between the predicted and ground truth masks.
- Jaccard Index: Commonly used for segmentation tasks, this metric compares the similarity between sample sets.
Visualization of results is also essential, allowing you to overlay the segmentation mask on the original images to see the effectiveness of the segmentation visually.
Example: Code to Visualize Results
% Example code to visualize segmentation results
overlay = imoverlay(original_image, segmentation_mask, [1 0 0]);
imshow(overlay);
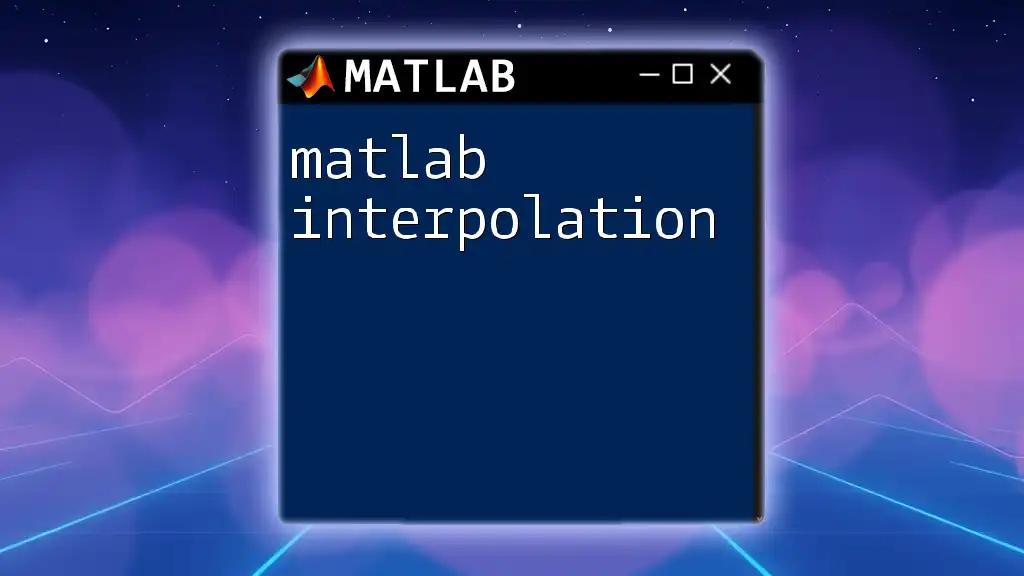
Fine-Tuning and Improving the Model
Tips for Hyperparameter Tuning
Fine-tuning hyperparameters is crucial for optimizing model performance. Experiment with:
- Learning Rates: Adjusting the learning rate can yield better convergence and stability.
- Dropout Rates: Integrating dropout layers helps prevent overfitting by randomly dropping units during training.
- Batch Sizes: Larger batches can improve training speed but may require more memory.
Transfer Learning Strategies
To improve performance further, consider pursuing transfer learning strategies. Pretrained models can serve as excellent starting points, allowing you to fine-tune them while saving on training time and resources. Adjust the model specifically for your target domain to enhance performance.
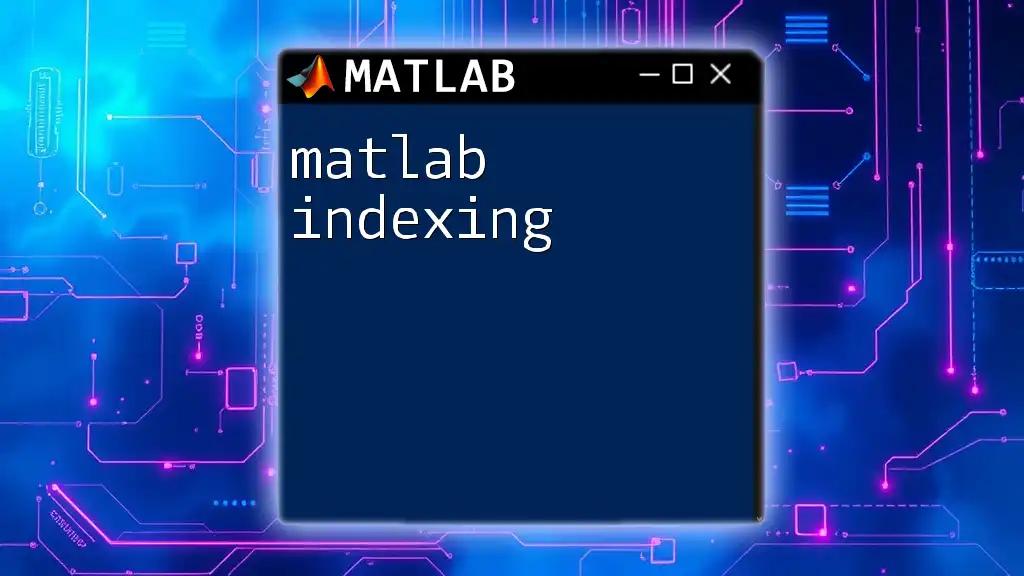
Common Challenges When Using u-net3d
Addressing Overfitting
Overfitting occurs when a model performs well on training data but poorly on unseen data. Techniques for combatting overfitting include:
- Regularization: Implement L1 or L2 regularization to penalize overly complex models.
- Dropout: Use dropout layers to help the model generalize better by reducing reliance on specific neurons.
For best results, practicing cross-validation will help gauge your model's effectiveness better across different subsets of your data.
Dealing with Imbalanced Data
Imbalanced datasets can significantly impact model performance. Addressing this challenge can involve:
- Resampling Techniques: Either oversample the minority classes or undersample the majority classes for balance.
- Adjusting Loss Functions: Use weighted loss functions that give more importance to minority classes, ensuring they influence the model’s learning process effectively.

Conclusion
In this guide, we explored the integral components of utilizing `matlab unet3d` for medical image segmentation. By understanding the architecture's nuances, preprocessing your data correctly, and implementing strategies for training, evaluation, and optimization, you can harness its full potential in your segmentation tasks. Remember, practice with various datasets and configurations will sharpen your skills and expand your understanding of deep learning in MATLAB.
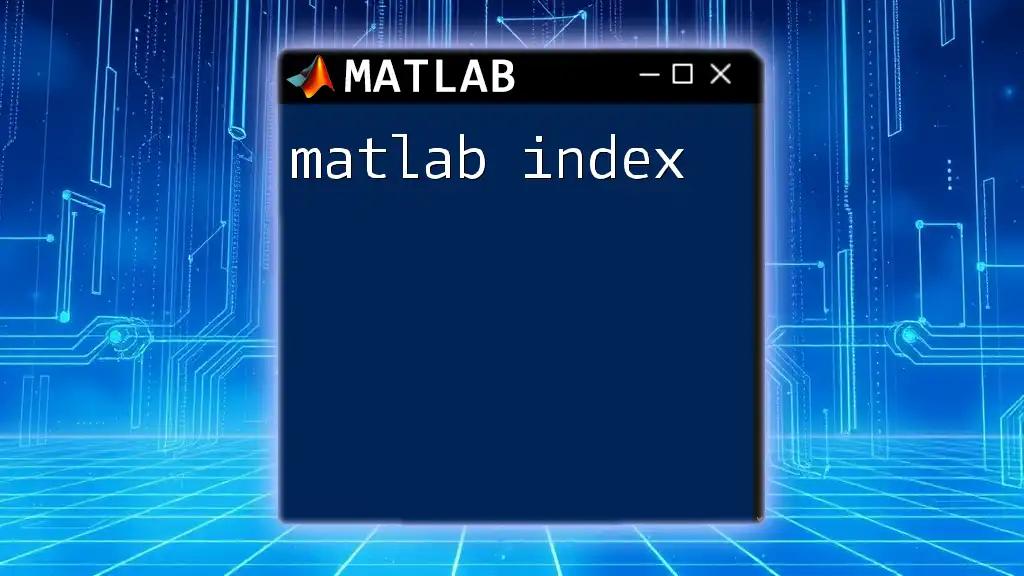
Additional Resources
To continue your learning journey, consider exploring resources such as books on deep learning, online courses, and MATLAB’s official documentation. Engaging with community forums and seeking support from other MATLAB users can significantly enhance your experience and mastery of `matlab unet3d`.