In MATLAB, matrix indexing allows you to access and modify elements of a matrix using their row and column indices.
Here's a code snippet to demonstrate basic matrix indexing:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % Create a 3x3 matrix
element = A(2, 3); % Access the element at the second row and third column (result: 6)
A(1, 1) = 10; % Modify the element at the first row and first column to 10
Understanding Matrices in MATLAB
What is a Matrix?
In mathematical terms, a matrix is a rectangular array of numbers, symbols, or expressions, arranged in rows and columns. In MATLAB, matrices are one of the foundational data types, enabling robust numerical computations and data manipulations. For example, consider a simple 3x3 matrix:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
Here, `A` represents a matrix with 3 rows and 3 columns.
Creating Matrices in MATLAB
Creating matrices in MATLAB is straightforward. You can define them directly using square brackets and separating elements with commas (for columns) or semicolons (for rows). For instance:
B = [10, 15, 20; 25, 30, 35; 40, 45, 50];
This creates another 3x3 matrix, `B`. MATLAB also allows various functions for creating special types of matrices, such as zeros, ones, and identity matrices:
C = zeros(2, 2); % 2x2 matrix of zeros
D = ones(3, 3); % 3x3 matrix of ones
E = eye(4); % 4x4 identity matrix
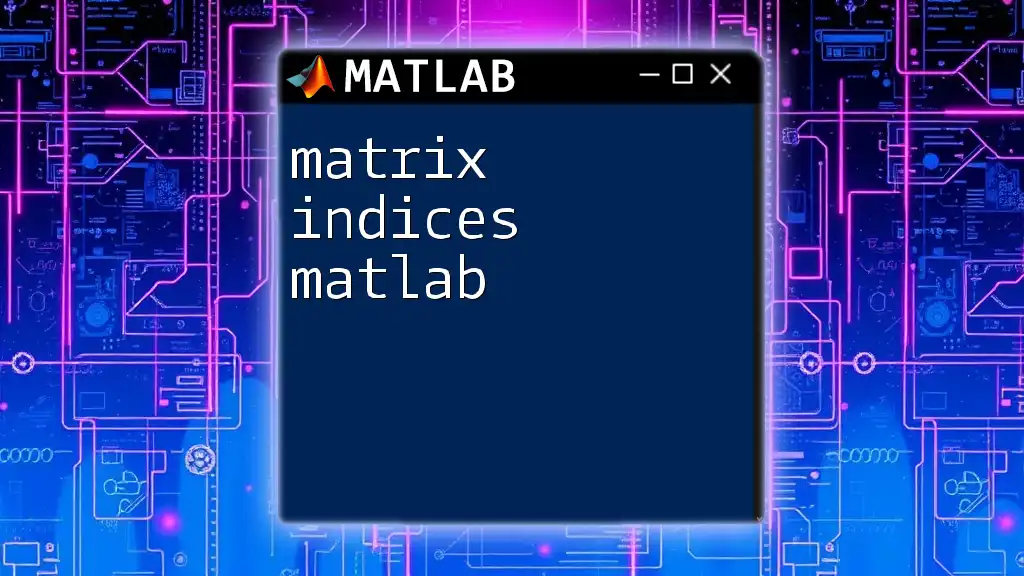
Basics of Indexing
Introduction to Indexing
Indexing is crucial in programming, allowing you to access specific elements in data structures. MATLAB uses 1-based indexing, unlike many other programming languages that typically use 0-based indexing. It means indexing starts from 1 rather than 0.
Accessing Matrix Elements
To access elements in a matrix, you use the syntax `A(row, column)`. For example, to access the element in the 2nd row and 3rd column of matrix `A`:
element = A(2, 3); % element = 6
Key Point: Always remember that the first number refers to the row, and the second number refers to the column.
Accessing Rows and Columns
You can easily retrieve an entire row or column by utilizing the `:` operator. Here’s how to access an entire row or column:
% Access the second row
row = A(2, :); % Returns the second row [4, 5, 6]
% Access the third column
column = A(:, 3); % Returns the third column [3; 6; 9]
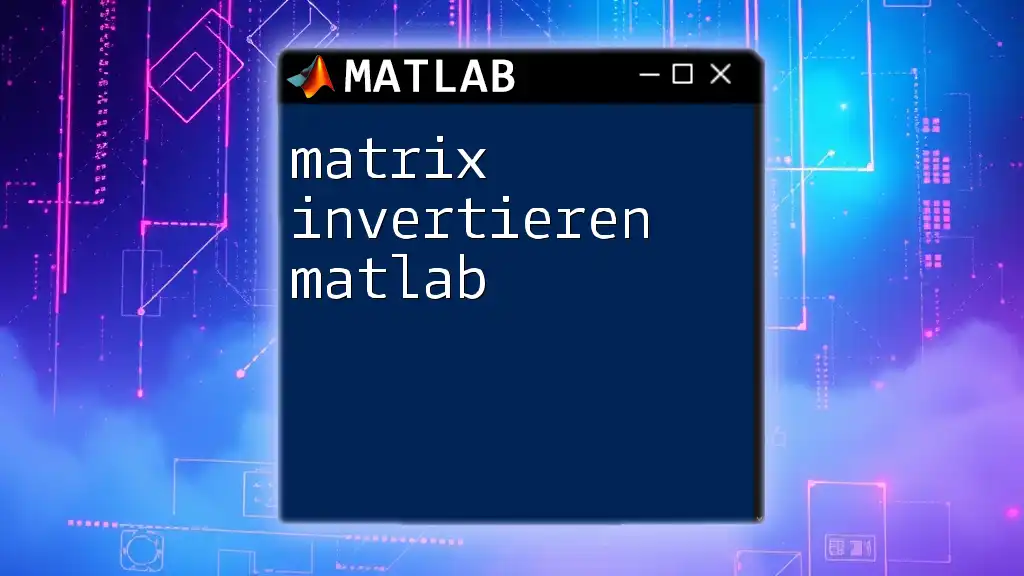
Advanced Indexing Techniques
Logical Indexing
Logical indexing permits you to extract elements using logical conditions rather than specific indices. This technique is invaluable for filtering data. For instance, if you want to extract all even numbers from matrix `A`:
even_elements = A(mod(A, 2) == 0); % Logical array returns even elements
Linear Indexing
In MATLAB, you can also use linear indexing, which treats the matrix as a single column vector. For example, to access the 5th element of matrix `A`:
linear_element = A(5); % Accesses element '5'
This method can be particularly useful when you need to iterate through multi-dimensional arrays linearly.
Using the `find` Function
The `find` function helps you locate the indices of matrix elements that meet a specific condition. For instance, finding all indices of elements greater than 5 can be done as follows:
idx = find(A > 5); % Returns indices of elements greater than 5
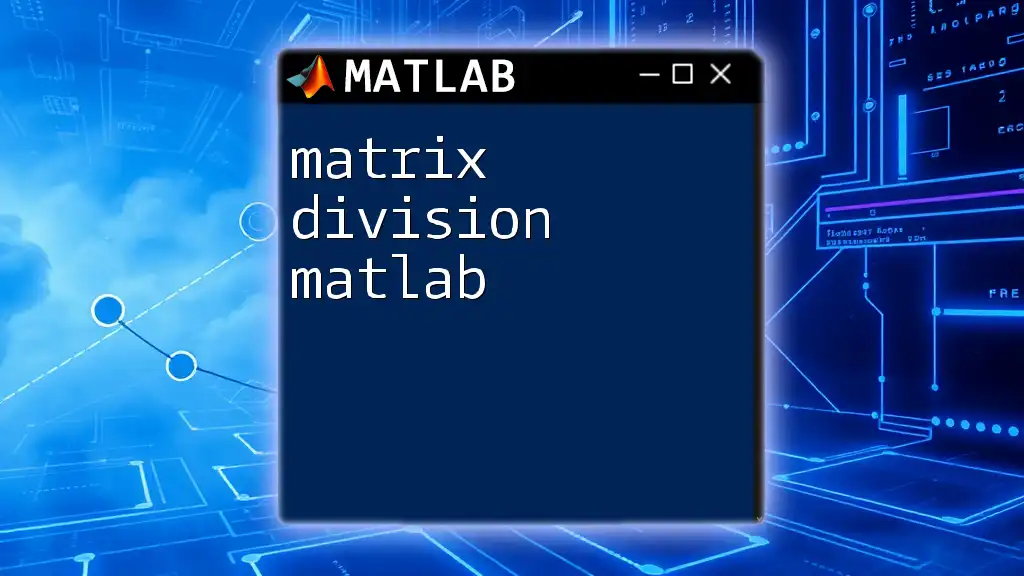
Modifying Matrices Using Indexing
Changing Values in a Matrix
You can easily modify specific matrix elements by referencing their indices. For example, if you wish to change the element at the first row and second column in matrix `A`:
A(1, 2) = 10; % Changes A to [1, 10, 3; 4, 5, 6; 7, 8, 9]
This straightforward syntax provides tremendous flexibility when managing data arrays.
Inserting Rows and Columns
Inserting new rows or columns is also possible through indexing. For example, if you want to append a new row to matrix `A`:
A = [A; 10, 11, 12]; % Append a new row
This operation extends `A` and facilitates dynamic data manipulation.
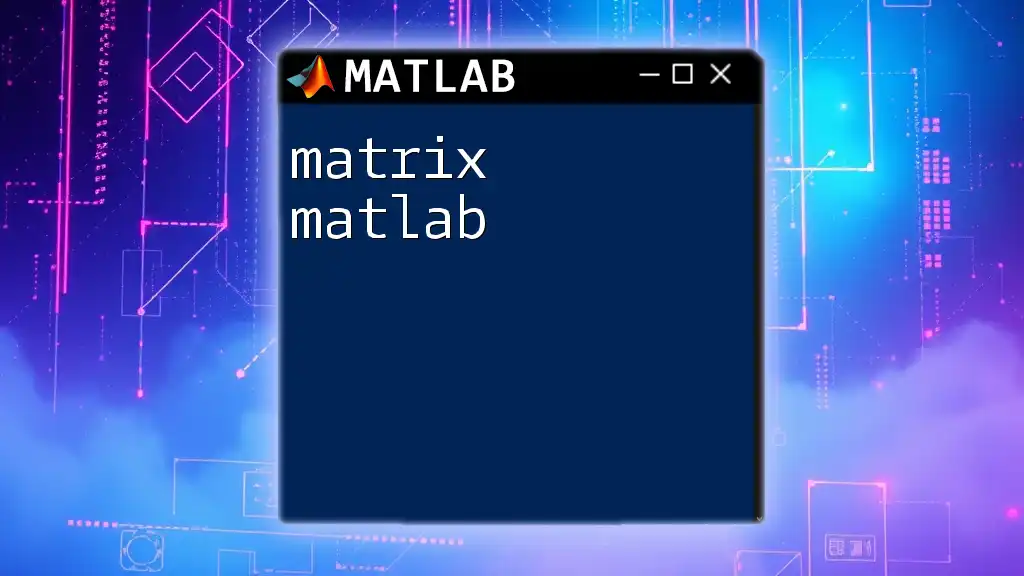
Practical Applications of Matrix Indexing
Data Extraction and Filtering
Indexing is invaluable for extracting submatrices, enabling you to work on smaller data sets without altering the original matrix. For instance, to extract a 2x2 submatrix from `A`:
submatrix = A(1:2, 2:3); % Extracts a 2x2 submatrix
This creates a new matrix containing specific rows and columns, making it easier to analyze subsets of data.
Matrix Reshaping and Reordering
Using indexing techniques, you can reshape and reorder matrices seamlessly. For example, reshaping a matrix can be done as follows:
B = reshape(A, [3, 3]); % Reshapes A into a 3x3 matrix
This allows for versatile data management that fits specific use cases.
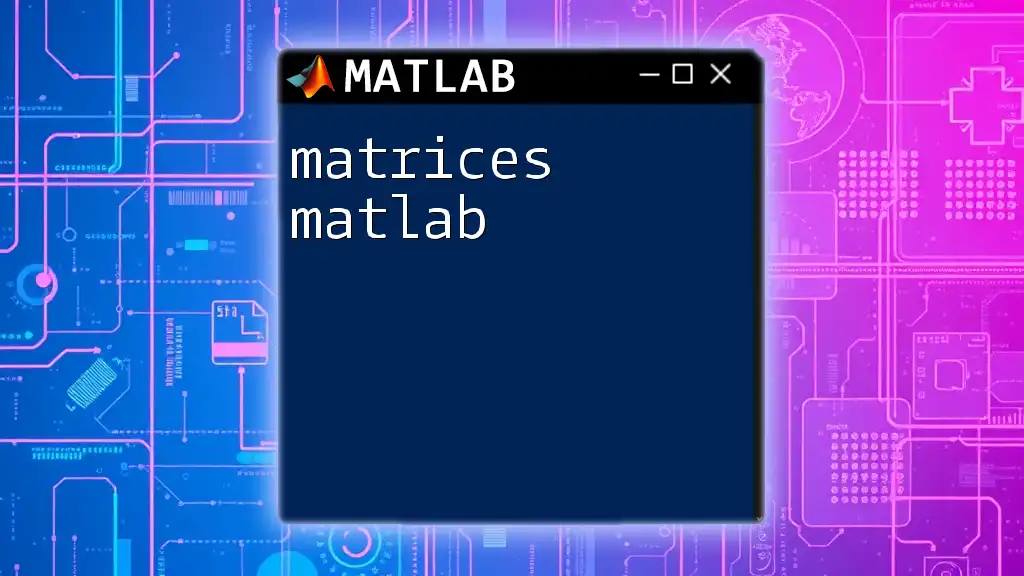
Common Errors and Troubleshooting
Index Out of Bounds
One common error that may occur when working with matrix indexing is the index out of bounds error. This typically happens when attempting to access an element that does not exist in the matrix.
For instance:
% This will result in an error
incorrect_element = A(4, 1); % Error: Index exceeds matrix dimensions.
Always ensure your indices stay within the bounds of the matrix dimensions.
Logical Indexing Errors
Using logical indexing can also lead to errors if the logical array does not correspond to the dimensions of the matrix being indexed.
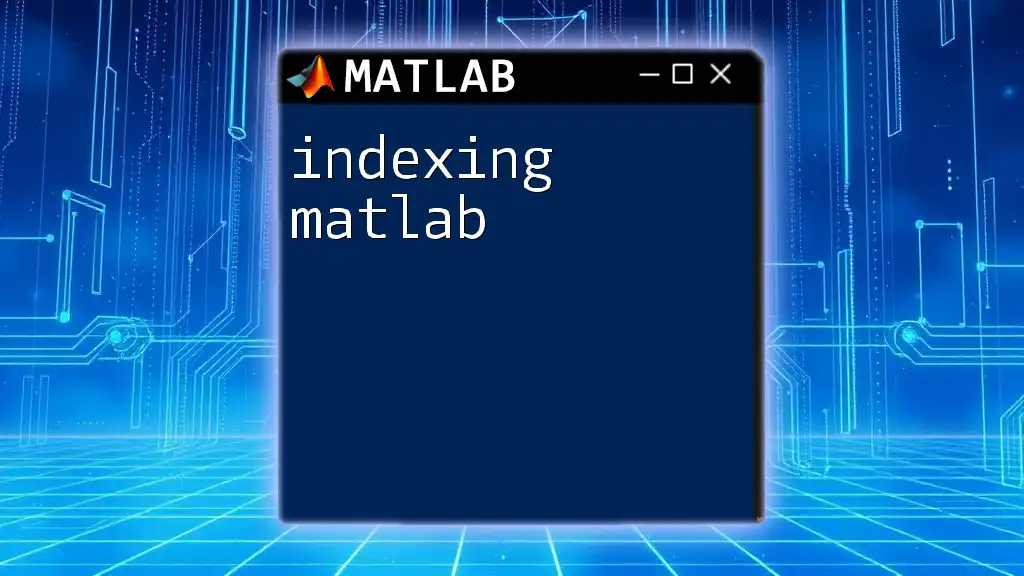
Summary
Mastering matrix index in MATLAB is crucial for enhancing your data manipulation and analysis capabilities. With the knowledge of different indexing methods, you can access, modify, and filter matrix elements effectively, unlocking MATLAB's full potential.
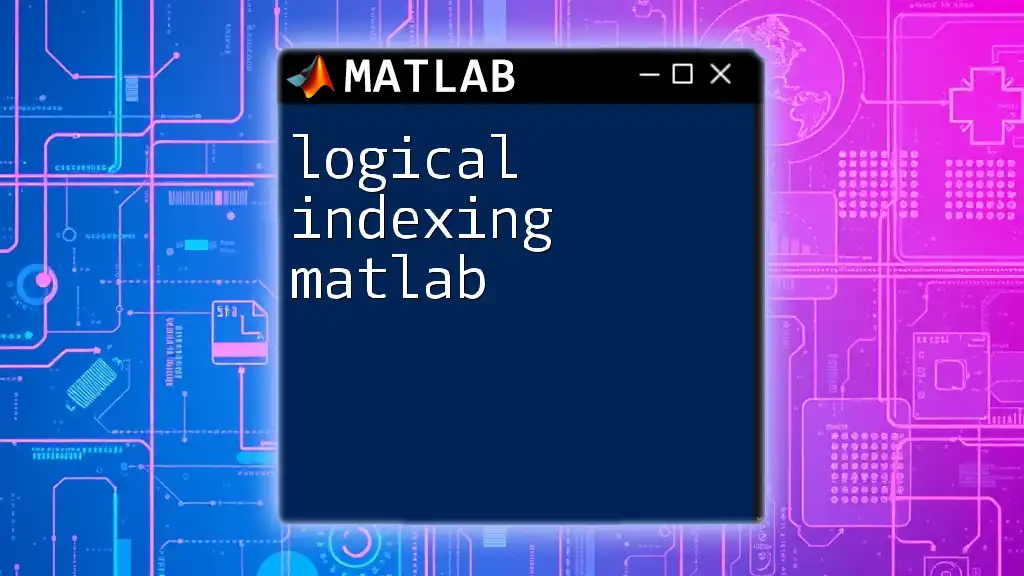
Further Reading and Resources
Consider exploring additional materials such as textbooks, online courses, and the official MATLAB documentation to deepen your understanding of matrix operations and programming techniques.
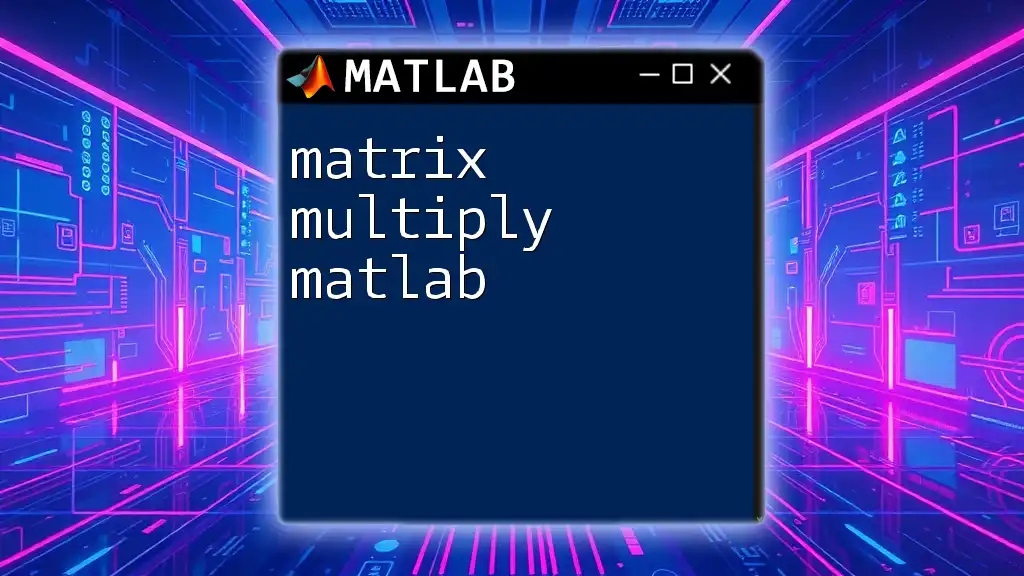
Conclusion
Mastering matrix indexing in MATLAB is not just about learning commands; it's about empowering your data analysis skills and making efficient use of MATLAB's capabilities for mathematical computations. As you practice these techniques, you'll find that they open up a world of possibilities for handling matrices effectively.