In MATLAB, a matrix is a two-dimensional array that serves as a fundamental data structure for numerical computations and can be easily manipulated using various built-in functions.
Here’s a simple code snippet to create a 2x2 matrix and perform matrix multiplication:
A = [1, 2; 3, 4]; % Define a 2x2 matrix
B = [5, 6; 7, 8]; % Define another 2x2 matrix
C = A * B; % Perform matrix multiplication
disp(C); % Display the result
Introduction to Matrices in MATLAB
What is a Matrix?
A matrix is a two-dimensional array of numbers arranged in rows and columns. In the mathematical realm and computer programming, matrices play a crucial role in many applications including numerical analysis, data manipulation, and image processing. In MATLAB, matrices are the fundamental data type used for numerical computing, which makes understanding matrix operations pivotal for any MATLAB user.
Why MATLAB for Matrix Operations?
MATLAB, short for "Matrix Laboratory," is specifically designed for numerical computations involving matrices. Compared to other programming languages, MATLAB excels in its straightforward syntax and built-in functions for matrix manipulation, which can significantly simplify complex mathematical operations.
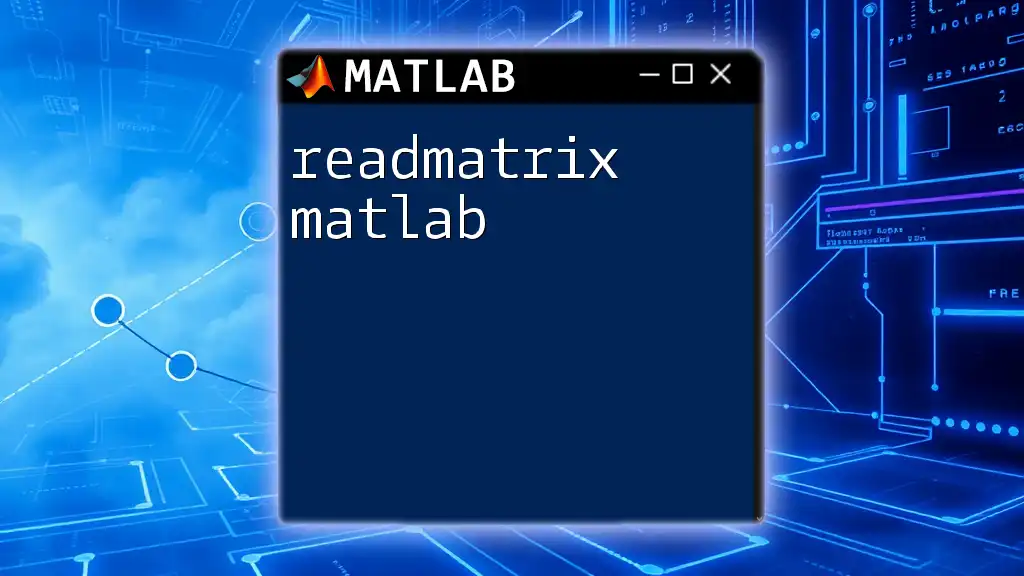
Creating Matrices in MATLAB
Basic Matrix Creation
Creating matrices in MATLAB is incredibly straightforward. You can construct matrices using square brackets. Here is a simple example of defining a 3x3 matrix:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
In this example, `A` is a matrix with three rows and three columns. Each semicolon denotes the start of a new row.
Special Matrices
MATLAB provides functions to create various special types of matrices:
Zero Matrices
A zero matrix is a matrix in which every element is zero. You can create a zero matrix of size 3x3 with the following command:
Z = zeros(3, 3);
Identity Matrices
An identity matrix is a square matrix with ones on the diagonal and zeros elsewhere. Use the following command to generate a 3x3 identity matrix:
I = eye(3);
Random Matrices
To generate matrices with random values, you can use the `rand` function. Here’s an example of creating a 2x4 matrix with random values:
R = rand(2, 4); % 2 rows and 4 columns
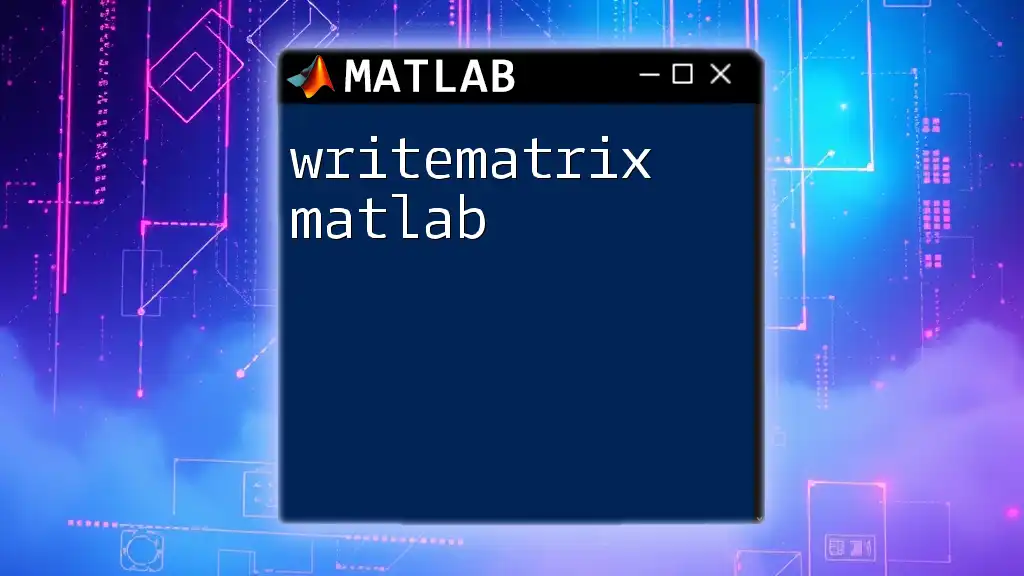
Accessing and Modifying Matrix Elements
Indexing and Slicing
Accessing specific elements in a matrix is done using parentheses. For instance, to access the element located in the second row and the third column of matrix `A`, you can use:
element = A(2, 3); % This retrieves the value '6'
Modifying Elements
Updating matrix components requires referencing their positions as well. For example, to change the element at the first row and second column of matrix `A`, use:
A(1, 2) = 10; % Now, A becomes [1, 10, 3; 4, 5, 6; 7, 8, 9]
Row and Column Operations
You can also manipulate entire rows or columns. To access the second column:
col = A(:, 2); % Retrieves the second column [10; 5; 8]
If you want to modify the first row entirely, you can do so like this:
A(1, :) = [10, 20, 30]; % Updates the first row
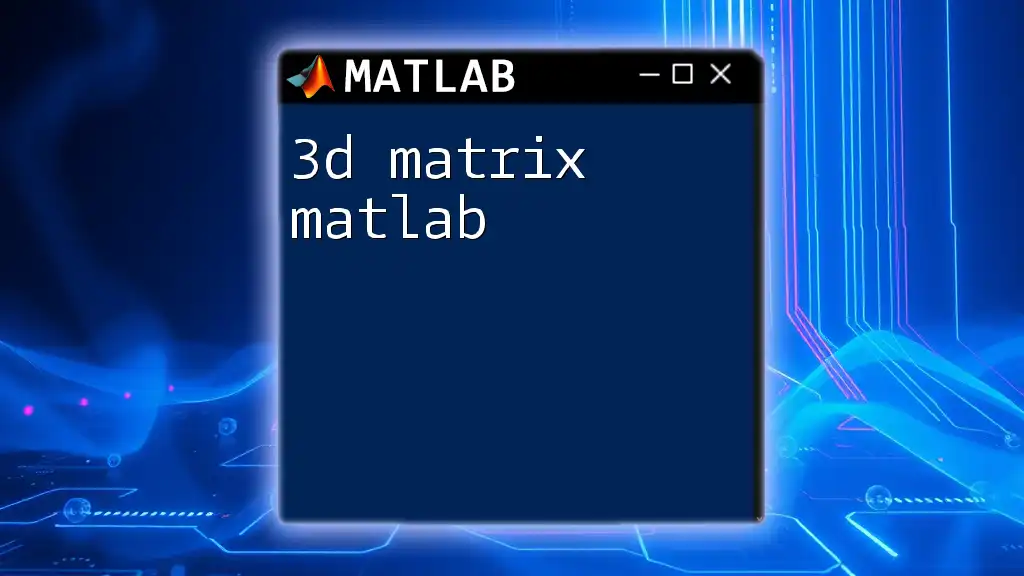
Common Matrix Operations
Matrix Addition and Subtraction
Adding and subtracting matrices in MATLAB is simple but requires that matrices be the same dimensions. Here’s how you can perform those operations:
C = A + B; % Element-wise addition
D = A - B; % Element-wise subtraction
Matrix Multiplication
Matrix multiplication is a fundamental operation in linear algebra. In MATLAB, you achieve this by the asterisk `*`:
E = A * B; % Matrix multiplication requires inner dimensions to match
Element-wise Operations
For operations that apply to each element individually, you use the `.*` operator for multiplication and `./` for division:
F = A .* B; % Element-wise multiplication
G = A ./ B; % Element-wise division
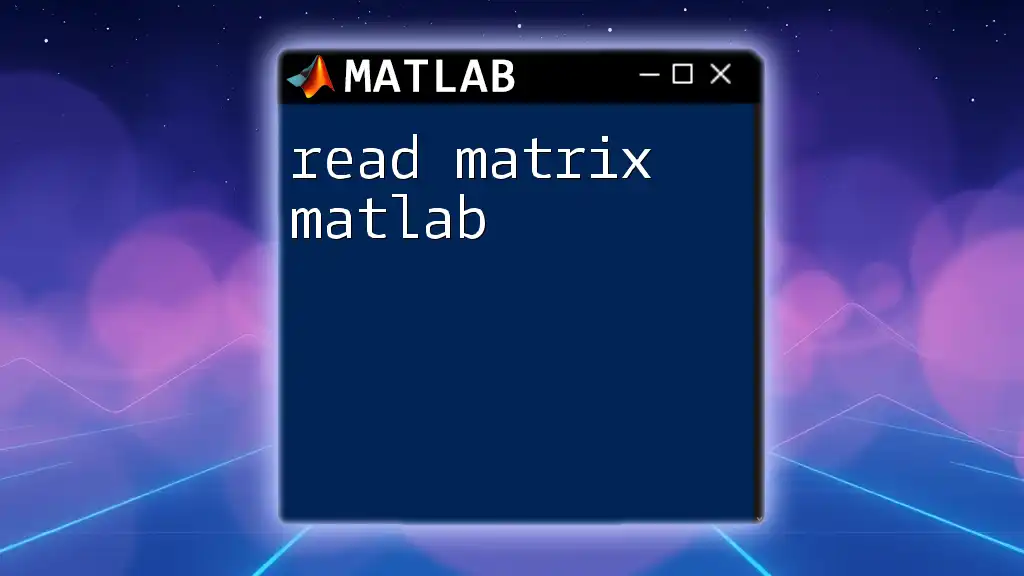
Advanced Matrix Functions
Determinants and Inverses
The determinant of a matrix provides useful information regarding its properties. To calculate the determinant and inverse of a matrix `A`, use:
detA = det(A); % Calculate the determinant
invA = inv(A); % Calculate the inverse
Eigenvalues and Eigenvectors
Finding the eigenvalues and eigenvectors is essential for various applications in physics and engineering. MATLAB simplifies this task with the `eig` function:
[V, D] = eig(A); % V contains eigenvectors, D is a diagonal matrix of eigenvalues
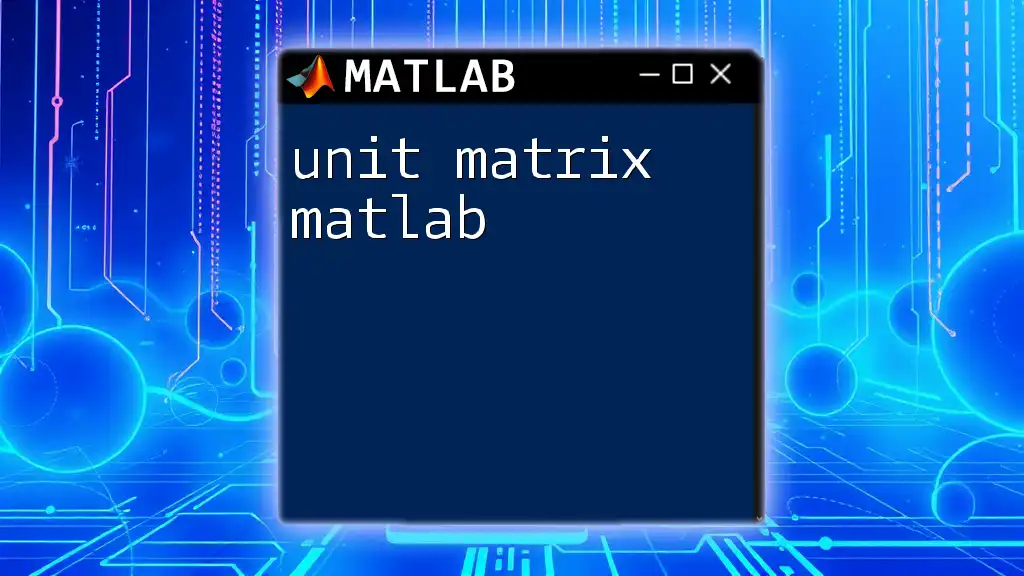
Practical Applications of Matrices
Solving Linear Equations
Matrices can represent systems of linear equations. You can effectively solve these systems using the backslash operator:
x = A\b; % Solve the equation Ax = b
Image Processing with Matrices
Since digital images often consist of pixels, they can be represented as matrices. Each pixel's intensity can be viewed as an element in a matrix, thus leveraging MATLAB's matrix capabilities allows for powerful image processing techniques.
Data Storage and Analysis
Matrices can efficiently store datasets, enabling statistical analysis and machine learning applications, where data manipulation is key to extracting meaningful insights.
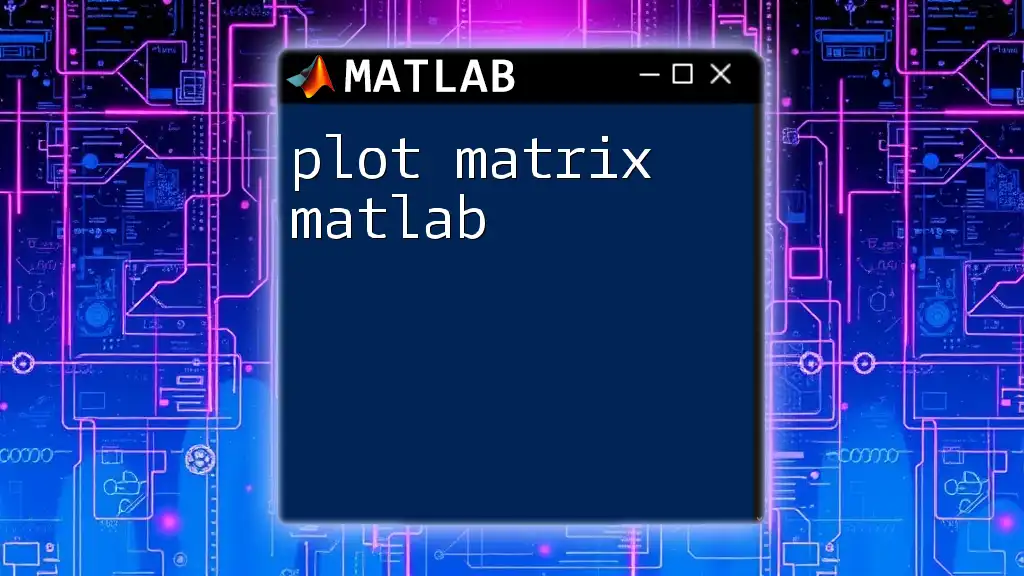
Best Practices for Matrix Manipulation
Avoiding Common Pitfalls
Some common mistakes include forgetting matrix dimensions when performing operations or mistakenly applying element-wise operations when matrix multiplication is required. Always check compatibility before executing operations.
Optimizing Performance
For large datasets or complex operations, consider preallocating matrices and avoiding growing them inside loops. This can drastically improve performance.
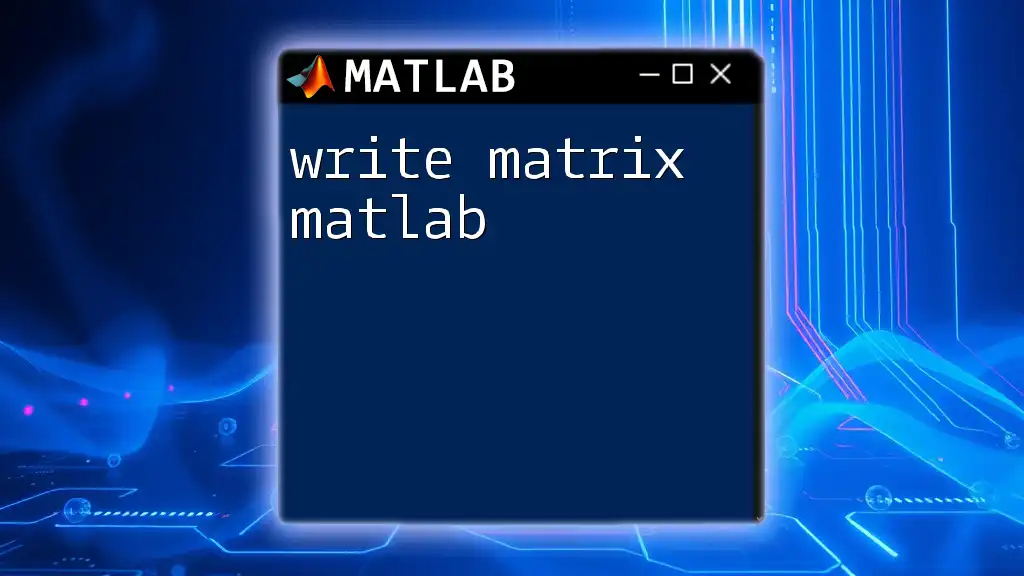
Conclusion
Understanding matrices in MATLAB is fundamental for anyone looking to harness the software's powerful computational capabilities. This guide has covered the essential aspects of matrix manipulation, from creation and access to advanced functions. Exploring these operations will enhance your MATLAB skills and open the door to countless applications. Embrace the vast opportunities that working with matrix MATLAB provides, and continue to explore the wealth of resources available to enrich your knowledge.