The standard deviation in MATLAB can be calculated using the `std` function, which computes the square root of the variance, providing a measure of dispersion in your data set.
Here's a code snippet demonstrating how to use it:
data = [5, 7, 3, 9, 4]; % Sample data
std_dev = std(data); % Calculate standard deviation
disp(std_dev); % Display the result
Understanding Standard Deviation
What is Standard Deviation?
The standard deviation is a statistical metric that measures the amount of variation or dispersion in a set of values. A low standard deviation indicates that the data points tend to be close to the mean, while a high standard deviation signifies that they are spread out over a wider range. It serves as a critical tool in understanding data’s reliability and variability.
Importance: Standard deviation is fundamental in various fields, such as finance, where it helps assess the risk of an asset. In research and data analysis, it informs researchers about the reliability of their findings, providing insights into data consistency.
When to Use Standard Deviation
Standard deviation is particularly useful in situations involving uncertainty or variability. It is widely applied in fields such as:
-
Research and Analysis: When conclusions are drawn from datasets, knowing how much the data spreads can guide reliable decision-making.
-
Financial Metrics: Investors look at the standard deviation of stocks to understand volatility, equating lower standard deviations with more stable stocks and higher ones with greater risk.
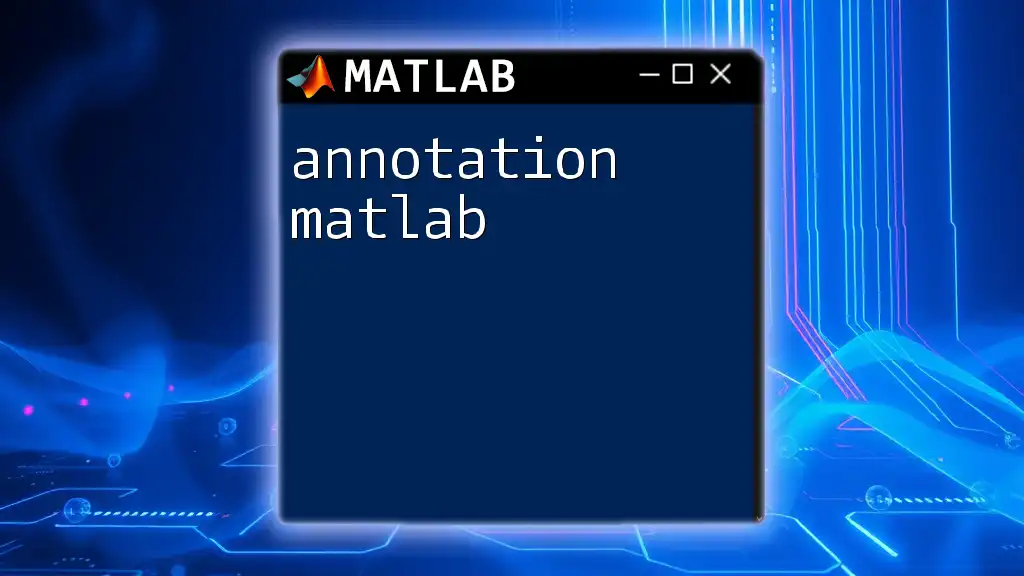
Standard Deviation in MATLAB
Overview of MATLAB and Its Capabilities
MATLAB, a high-level programming language and interactive environment, excels in matrix computations, data analysis, and visualization. Its rich set of built-in functions allows users to perform advanced statistical analysis with ease, making it a preferred choice among engineers and scientists.
Basic Commands to Calculate Standard Deviation
In MATLAB, the `std` function computes the standard deviation of a dataset quickly and efficiently. The basic syntax is as follows:
std(data, 'omitnan')
This syntax will ignore any `NaN` values in the dataset, ensuring more accurate results.
For example, to calculate the standard deviation of a simple dataset, you can execute:
data = [10, 12, 23, 23, 16, 23, 21, 16];
std_dev = std(data);
fprintf('Standard Deviation: %.2f\n', std_dev);
This code snippet will display the standard deviation, providing users an immediate understanding of data dispersion.
Manual Calculation of Standard Deviation
Understanding how standard deviation is calculated manually can deepen your grasp of the concept. The standard deviation is calculated using the following formula:
- Population Standard Deviation: \[ \sigma = \sqrt{\frac{1}{N} \sum_{i=1}^{N} (x_i - \mu)^2} \]
- Sample Standard Deviation: \[ s = \sqrt{\frac{1}{n-1} \sum_{i=1}^{n} (x_i - \bar{x})^2} \]
Here, \( \mu \) and \( \bar{x} \) represent the population and sample means, while \( N \) and \( n \) are the sizes of the population and sample, respectively.
Step-by-Step Example
By breaking this down into simple MATLAB commands, you can visualize every step:
% Data
x = [10, 12, 23, 23, 16, 23, 21, 16];
% Step 1: Mean Calculation
mean_x = mean(x);
% Step 2: Squared Differences
squared_diff = (x - mean_x).^2;
% Step 3: Variance Calculation
variance = sum(squared_diff) / (length(x) - 1); % Sample variance
% Step 4: Standard Deviation
manual_std_dev = sqrt(variance);
fprintf('Manual Standard Deviation: %.2f\n', manual_std_dev);
This example not only calculates the standard deviation manually but also reinforces the concept through calculation.
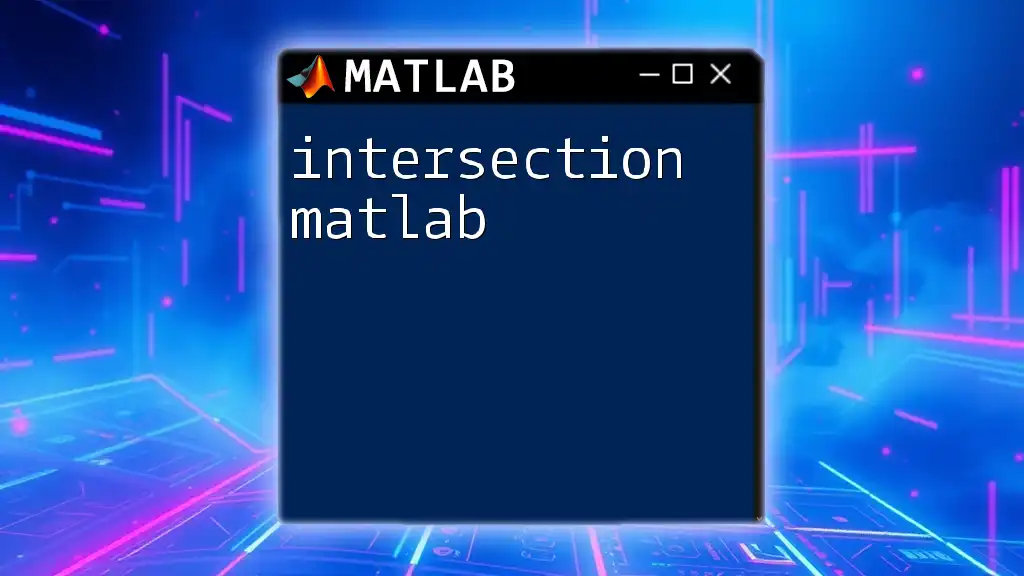
Different Types of Standard Deviation
Population vs. Sample Standard Deviation
When dealing with data analysis, it's essential to understand the distinction between population and sample standard deviation:
- Population Standard Deviation is used when your dataset comprises the entire population you're studying.
- Sample Standard Deviation is utilized when analyzing a subset drawn from a larger population.
The `std` command helps distinguish between the two. By default, it calculates the sample standard deviation unless otherwise specified. To compute the population standard deviation, set the second argument to `0`:
population_std_dev = std(data, 0);
Standard Deviation for Matrices
MATLAB enables users to compute the standard deviation over multiple dimensions, particularly useful for multidimensional arrays. Understanding how to perform these calculations is essential for comprehensive data analysis.
You can compute the standard deviation across different dimensions—rows or columns—using the following:
matrix = [10, 20, 30; 40, 50, 60];
std_dev_rows = std(matrix, 0, 1); % Standard deviation for each column
std_dev_cols = std(matrix, 0, 2); % Standard deviation for each row
Here, `0` indicates that we calculate the sample standard deviation. Specifying `1` or `2` allows flexibility in choosing the dimension along which to compute dispersion.
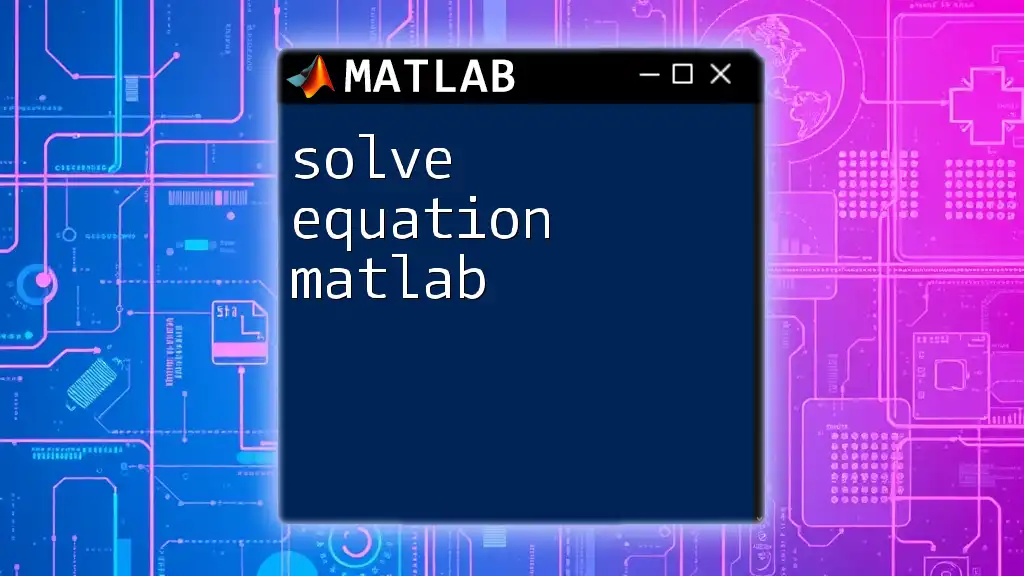
Visualizing Standard Deviation
Using MATLAB for Data Visualization
Data visualization plays a critical role in communication. By representing standard deviation visually, you can easily convey insights. A common method of visualizing standard deviation is through error bars, which give a graphical representation of variability.
Example with Error Bars
Assume you have measurements of a set of data:
% Data
x = [1, 2, 3, 4, 5];
y = [10, 12, 17, 21, 24];
% Standard Deviation
errors = std([10, 12, 17, 21, 24]);
% Plotting with error bars
errorbar(x, y, errors);
title('Error Bars Representing Standard Deviation');
The error bars in this plot represent the calculated standard deviation, visually demonstrating the data's variability and indicating the reliability of each data point.
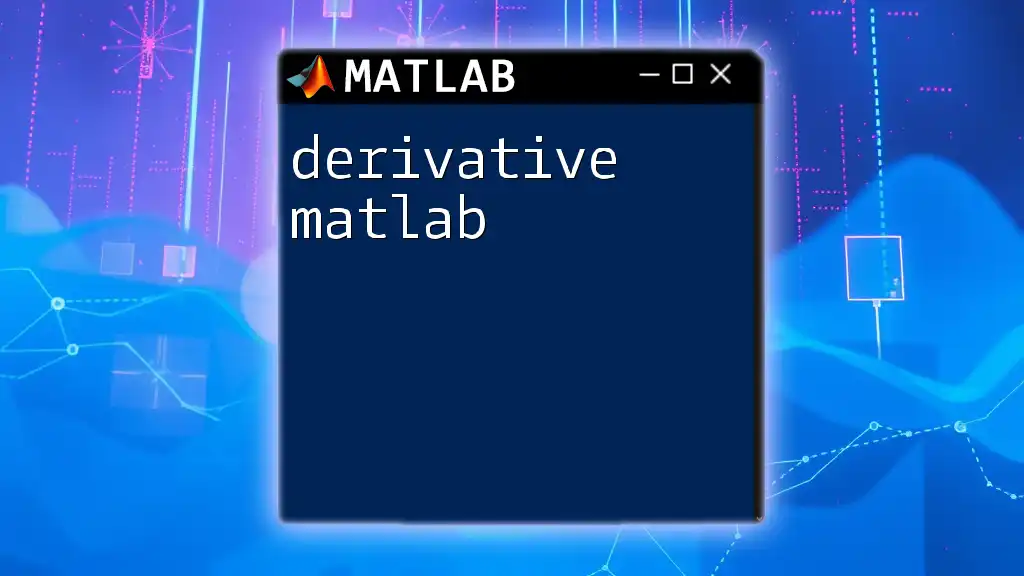
Practical Applications of Standard Deviation in MATLAB
Case Study: Analyzing Data Sets
Standard deviation has practical applications across various industries. For example, in finance, it helps investors measure the volatility of stock prices. A higher standard deviation indicates greater risk, allowing traders to make informed decisions based on their risk tolerance.
In the context of engineering, standard deviation aids in quality control, where manufacturers measure variation in product dimensions or performance to ensure compliance with specifications.
Creating a MATLAB Function for Standard Deviation
To encapsulate the logic of standard deviation calculation, you can create a MATLAB function that computes both standard deviation and variance conveniently. This custom implementation adds flexibility and promotes reusable code:
function [std_dev, variance] = custom_std(data)
mean_val = mean(data);
squared_diff = (data - mean_val).^2;
variance = sum(squared_diff) / (length(data) - 1);
std_dev = sqrt(variance);
end
By calling this function, users can effortlessly calculate the standard deviation and variance of any dataset with minimal effort.
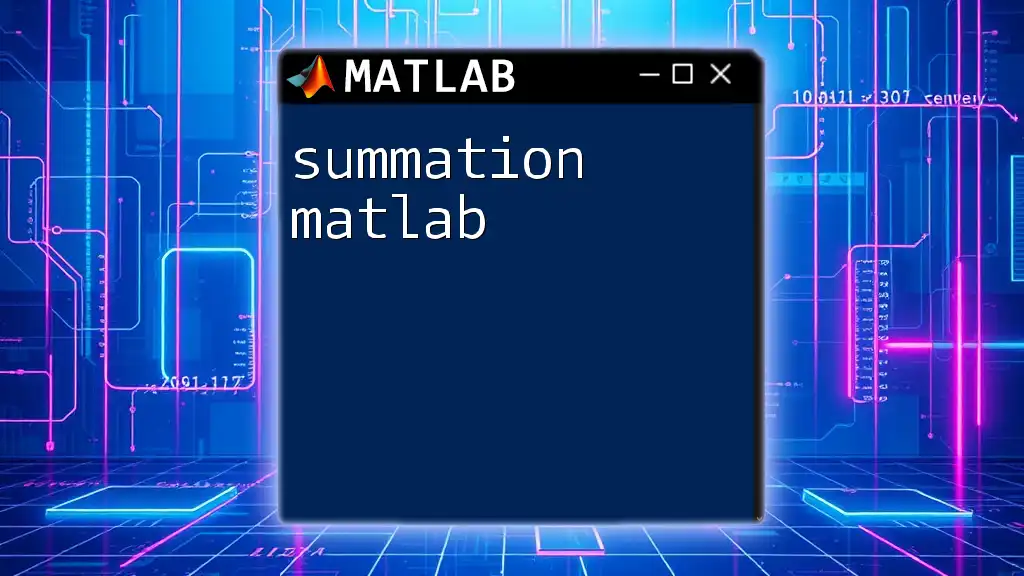
Troubleshooting Common Issues
Common Errors and Solutions
While calculating the standard deviation in MATLAB, users may encounter several issues. One common error arises from invalid input types. If non-numeric values are passed to the `std` command, MATLAB will return an error message, which can be avoided by ensuring your data is processed correctly.
Another significant challenge concerns NaN and Inf values in datasets. To handle these, MATLAB provides the `omitnan` option in the `std` function, ensuring invalid data doesn’t skew your results.
For example:
data_with_nan = [10, 12, NaN, 23, 16];
std_dev_no_nan = std(data_with_nan, 'omitnan');
Using this command, you can compute standard deviation while ignoring `NaN` values, producing a more accurate assessment of your data variability.
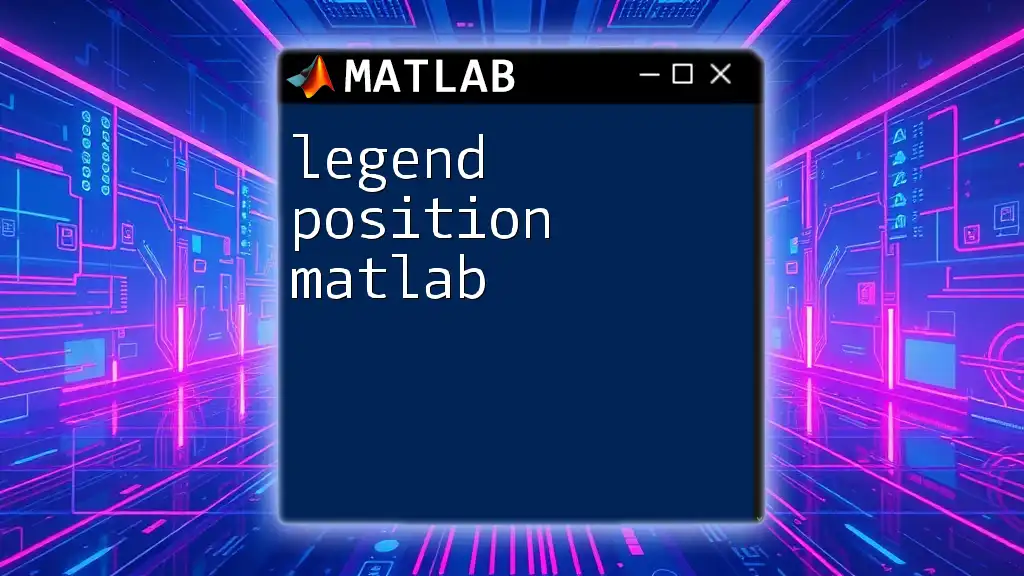
Conclusion
This comprehensive guide has covered the essentials of calculating and interpreting standard deviation using MATLAB. By grasping both the theoretical aspects and practical applications, users can better handle data analysis tasks with ease. Understanding standard deviation in MATLAB is not just about the correct computations; it's about the underlying insights that emerge from the data. Having explored various methodologies, from built-in functions to custom implementations, readers are encouraged to delve deeper into statistical analyses and expand their MATLAB skills.