Sure! Here's a concise explanation along with a code snippet:
In MATLAB, matrices are fundamental data structures that allow users to perform complex mathematical operations efficiently.
% Creating a 3x3 matrix and calculating its transpose
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
A_transpose = A'; % Transpose of matrix A
Understanding Matrix Basics
Matrices are defined as rectangular arrays of numbers, symbols, or expressions, arranged in rows and columns. Understanding matrices is crucial in various fields such as engineering, physics, and economics, as they serve as the backbone for representing and solving problems.
Types of Matrices
-
Row Matrix: A matrix that consists of a single row. For example:
R = [1 2 3];
-
Column Matrix: A matrix that consists of a single column. For example:
C = [1; 2; 3];
-
Square Matrix: A matrix where the number of rows is equal to the number of columns. Example:
S = [1 2; 3 4];
-
Zero Matrix: A matrix in which all elements are zero. For instance:
Z = zeros(3, 3); % Creates a 3x3 zero matrix
-
Identity Matrix: A special type of square matrix where all the diagonal elements are 1, and all other elements are 0. Example:
I = eye(3); % Creates a 3x3 identity matrix
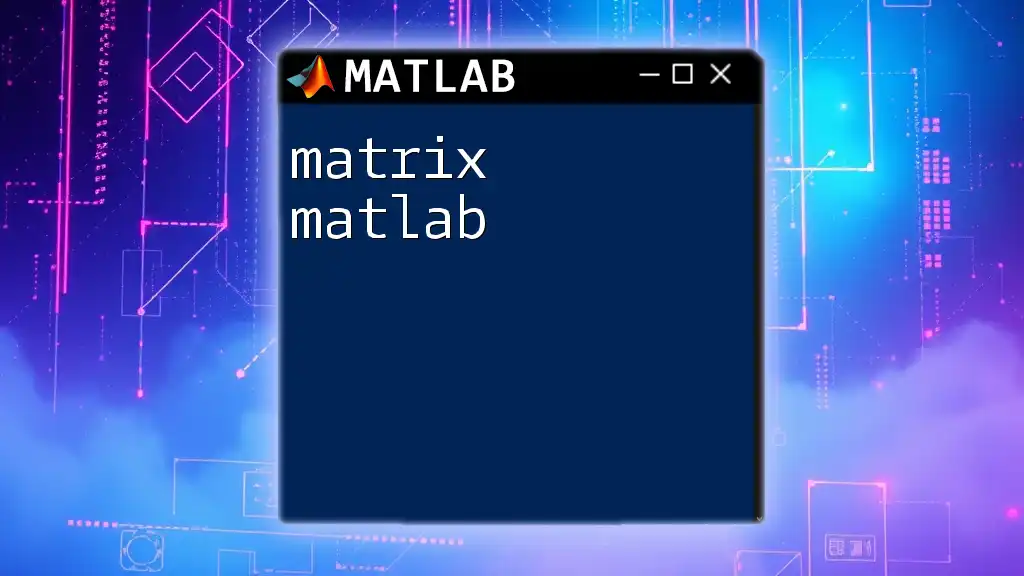
Creating Matrices in MATLAB
Creating matrices in MATLAB can be done using direct input or built-in functions.
Using Direct Input
The simplest way to create a matrix is by defining it with square brackets. Rows are separated by semicolons, and elements within a row are separated by spaces or commas.
For example:
A = [1 2 3; 4 5 6; 7 8 9];
Using Built-in Functions
MATLAB provides several built-in functions that facilitate matrix creation:
-
`zeros()`: This function creates a matrix filled with zeros. Example:
Z = zeros(3, 3); % Creates a 3x3 zero matrix
-
`ones()`: Similar to `zeros()`, this function creates a matrix filled with ones. Example:
O = ones(2, 4); % Creates a 2x4 matrix of ones
-
`eye()`: This function creates an identity matrix. Example:
I = eye(4); % Creates a 4x4 identity matrix
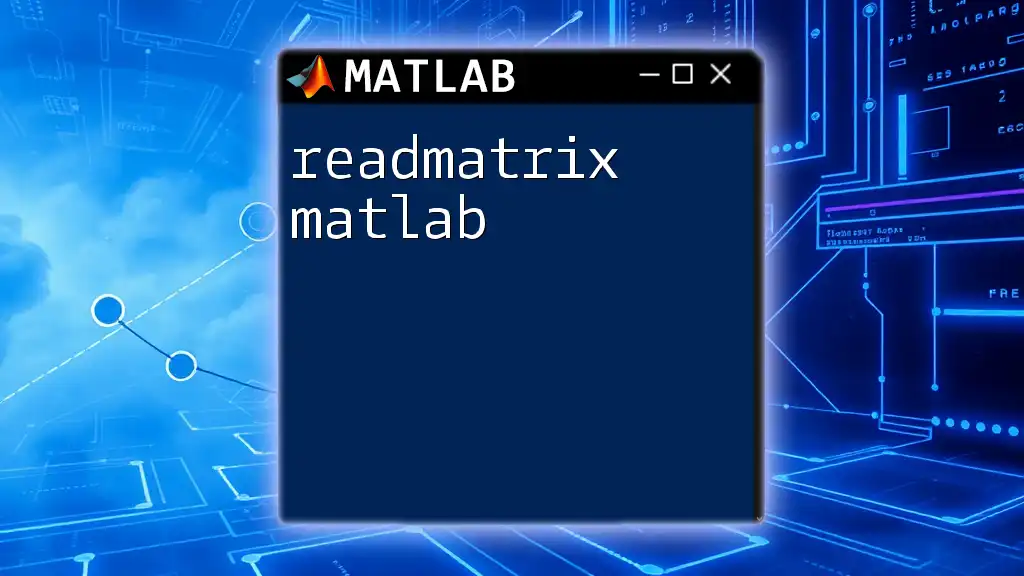
Accessing and Modifying Matrix Elements
Once a matrix is created, users need to access and modify its elements effectively.
Indexing Matrices
To access a specific element of a matrix, the syntax requires specifying the row and column indices. MATLAB uses 1-based indexing.
For instance, to access the element in the second row, third column:
elem = A(2, 3); % Accesses the element at (2,3)
Modifying Elements
Changing a specific element can be easily done using the same indexing technique. For example, changing the element located at the first row, second column to 10:
A(1, 2) = 10; % Updates the element at (1,2) to 10
Extracting Rows and Columns
MATLAB allows for easy extraction of entire rows or columns. The colon operator `:` is handy here. For example, to extract the second row:
secondRow = A(2, :); % Extracts the second row completely
To extract the first column, the syntax would be:
firstColumn = A(:, 1); % Extracts the first column completely
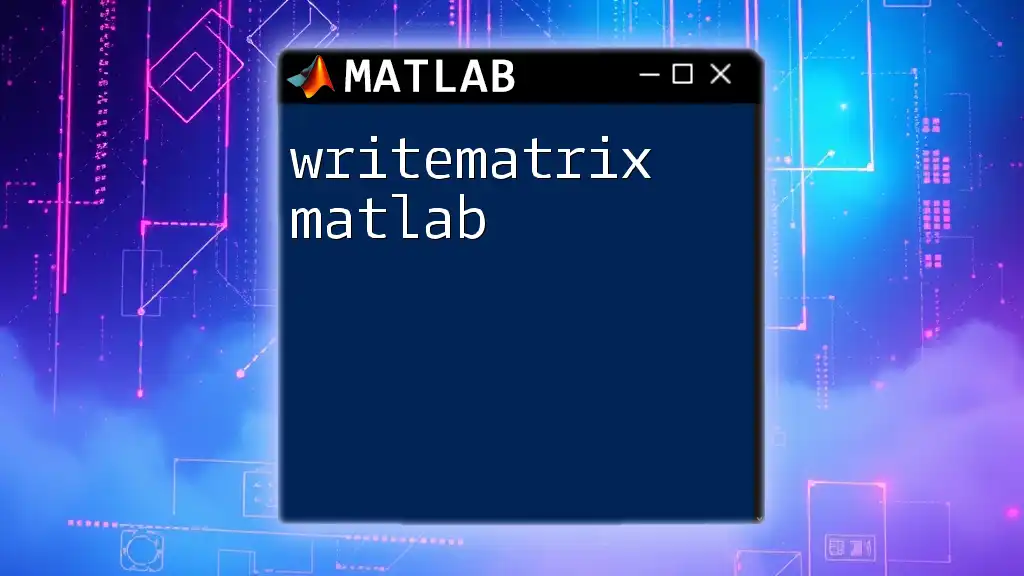
Basic Matrix Operations
Matrix operations are essential for various applications. MATLAB provides straightforward syntax for performing these operations.
Addition and Subtraction
Matrices can be added or subtracted if they have the same dimensions. The operation is performed element-wise.
For example, if matrix B is defined as:
B = [1 1 1; 1 1 1; 1 1 1];
Then the addition of matrices A and B can be done as follows:
C = A + B; % Adds matrices A and B
Matrix Multiplication
Matrix multiplication is a crucial operation that follows specific rules. For matrix A and matrix B to be multiplied, the number of columns in A must equal the number of rows in B.
For example:
D = A * B; % Performs matrix multiplication
Transposition
The transpose of a matrix swaps its rows and columns, and MATLAB uses a single quote (`'`) to denote this operation.
To transpose matrix A, the syntax is:
A_transpose = A'; % Transposes matrix A
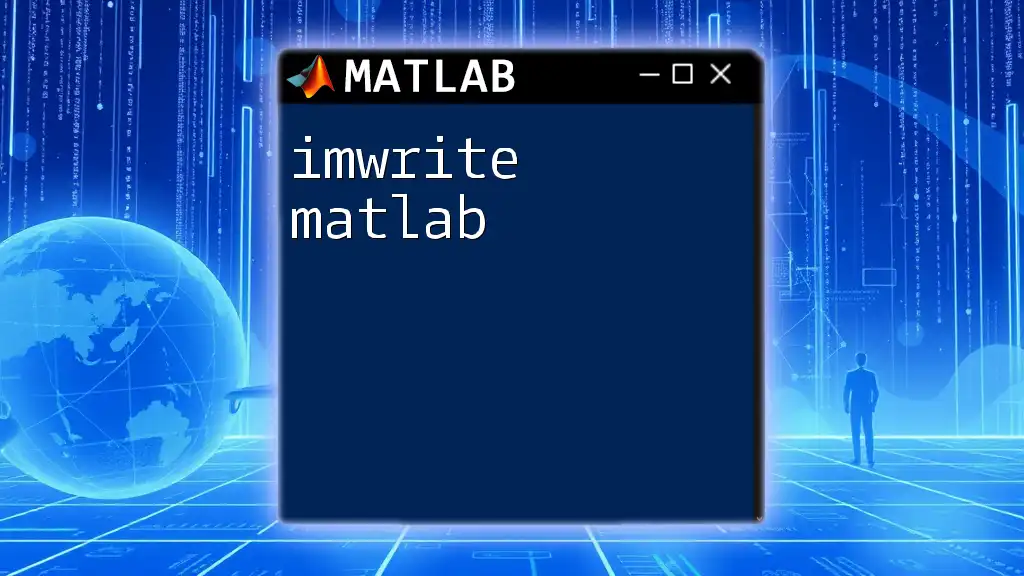
Advanced Matrix Functions
Beyond basic operations, MATLAB offers advanced functions to help users work with matrices effectively.
Determinants and Inverses
The determinant of a matrix provides important information about the matrix, particularly concerning its invertibility. The determinant can be calculated using the `det()` function.
For example:
detA = det(A);
If a matrix is square and has a non-zero determinant, it can be inverted using the `inv()` function:
A_inv = inv(A); % Calculates the inverse of matrix A
Eigenvalues and Eigenvectors
Eigenvalues and eigenvectors are critical concepts in linear algebra, often used to understand linear transformations represented by matrices. The `eig()` function computes the eigenvalues and eigenvectors for a given matrix.
For example:
[V, D] = eig(A); % V are eigenvectors, D are diagonals of eigenvalues
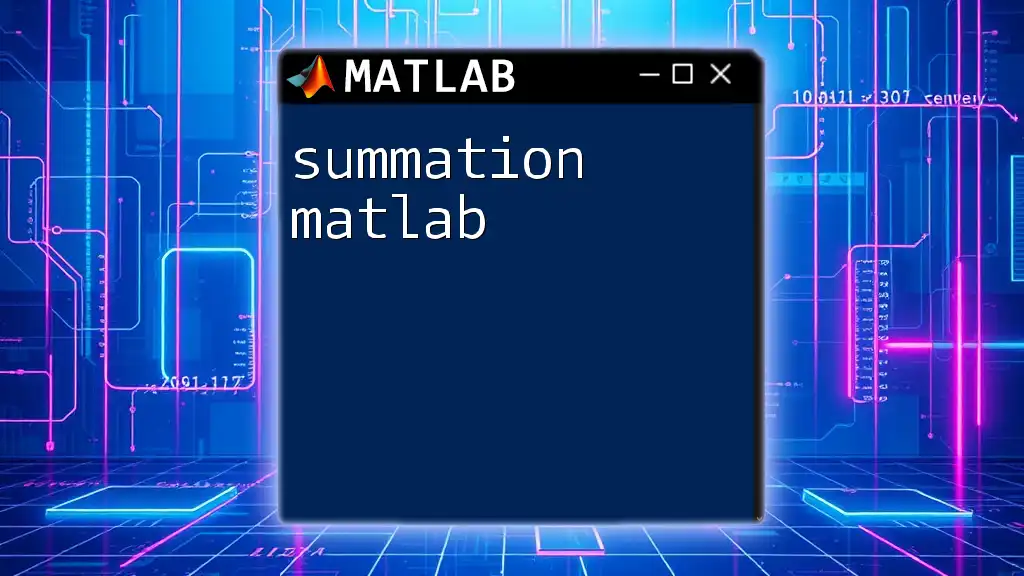
Practical Applications of Matrices
Matrices are integral to solving real-world problems, and MATLAB provides excellent tools for applying matrix operations.
Using Matrices in Linear Algebra
In linear algebra, matrices are used extensively to represent and solve systems of equations. MATLAB's capabilities allow for efficient computation and results interpretation.
Image Processing with Matrices
Images can be represented as matrices, where each pixel’s intensity corresponds to a matrix element. This representation allows for various image processing techniques, such as filtering and transformation, to be easily applied.
Data Representation
Matrices are also vital in data representation, enabling operations like data normalization, transformations, and statistical analysis.
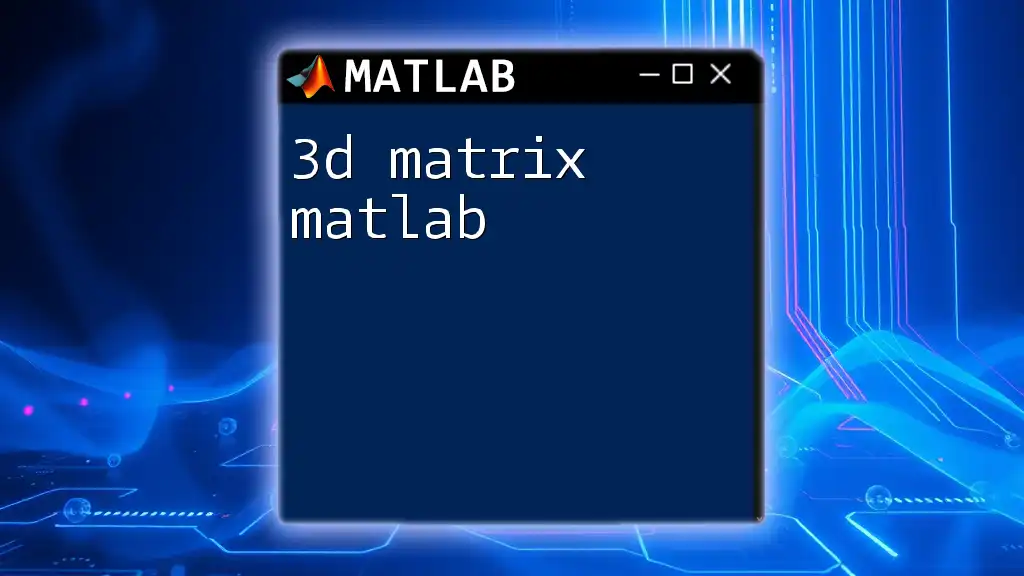
Summary
Understanding matrices in MATLAB is fundamental for anyone looking to excel in computational tasks. From creating and manipulating matrices to performing complex operations, these foundational skills pave the way for more advanced applications in various fields.
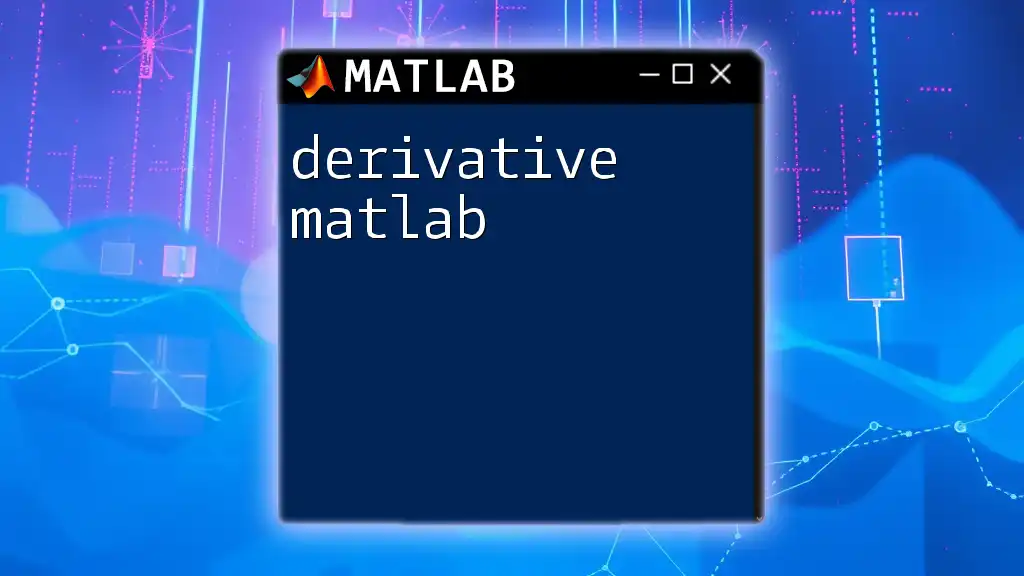
Additional Resources
For further learning, MATLAB’s official documentation provides in-depth explanations and examples regarding matrices. Explore various tutorials that delve into matrix-specific functions to enhance your understanding and proficiency.
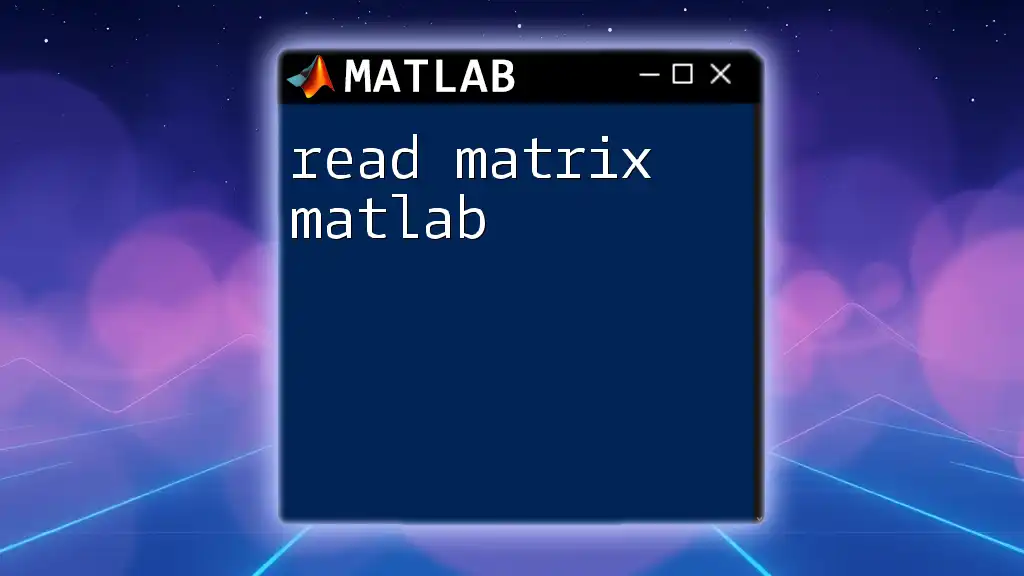
Conclusion
Mastering matrices in MATLAB is essential for tackling a wide range of applications. With practice and exploration, anyone can become proficient in manipulating and utilizing matrices for real-world problem-solving.