The `max` function in MATLAB returns the largest element in an array or the largest values along a specified dimension of a matrix.
% Example of using the max function in MATLAB
A = [3, 5, 1; 7, 2, 9; 4, 6, 8];
maxValue = max(A(:)); % Finds the maximum value in the entire array
Introduction to the `max` Function
The `max` function in MATLAB is a powerful tool designed to find the maximum values in arrays, matrices, and even among two or more sets of data. Whether you are performing statistical analysis or processing data, mastering the `max` function can significantly enhance the efficiency of your computations.
What is the `max` Function?
Simply put, the `max` function identifies the largest value in a given set of numerical data. This function is invaluable in various applications, including data analysis, performance evaluations, and algorithm optimization.
Usage Scenarios
You might find the `max` function particularly useful in circumstances such as:
- Statistical Analysis: Finding the highest score in a dataset.
- Data Processing: Retrieving maximum values from signal data.
- Comparative Evaluation: Comparing two datasets to identify more favorable outcomes.
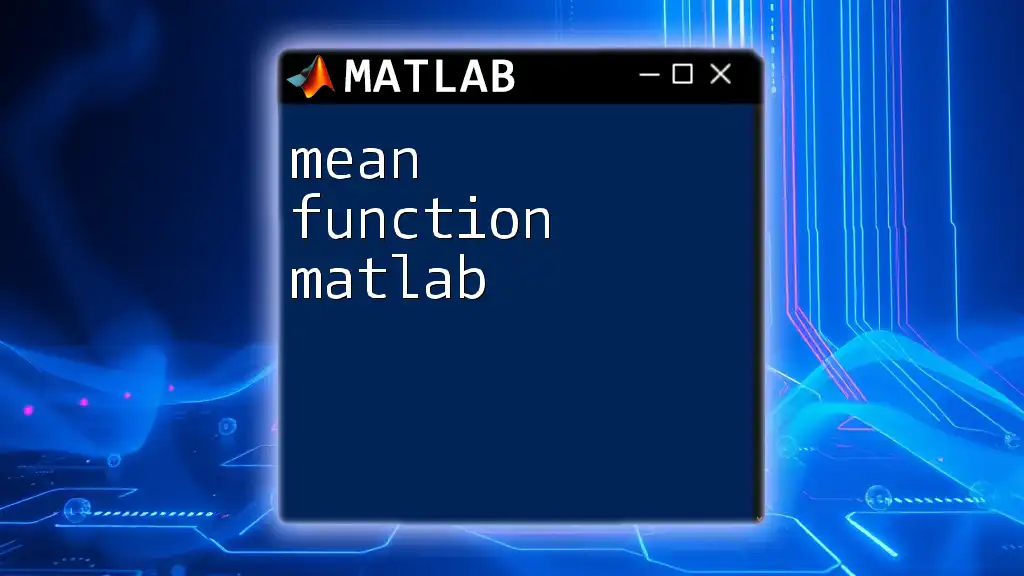
Syntax of the `max` Function
Basic Syntax
The simplest form of the `max` function is:
Y = max(A)
In this syntax, `A` can be a vector, matrix, or multi-dimensional array, and `Y` represents the maximum value derived from `A`.
Two Input Arguments
The function can also compare two arrays or matrices with the following syntax:
Y = max(A, B)
With this command, MATLAB conducts an element-wise comparison, returning the maximum value between corresponding elements of `A` and `B`.
Dimensional Syntax
For working with multi-dimensional arrays, you can specify the dimension with:
Y = max(A, [], dim)
In this case, `dim` is the dimension along which `max` operates (e.g., 1 for columns, 2 for rows).
Multiple Output Variables
Sometimes, you may need both the maximum values and their indices:
[Y, I] = max(A)
In this scenario, `Y` returns the maximum values, while `I` returns the indices of those maximums within `A`.
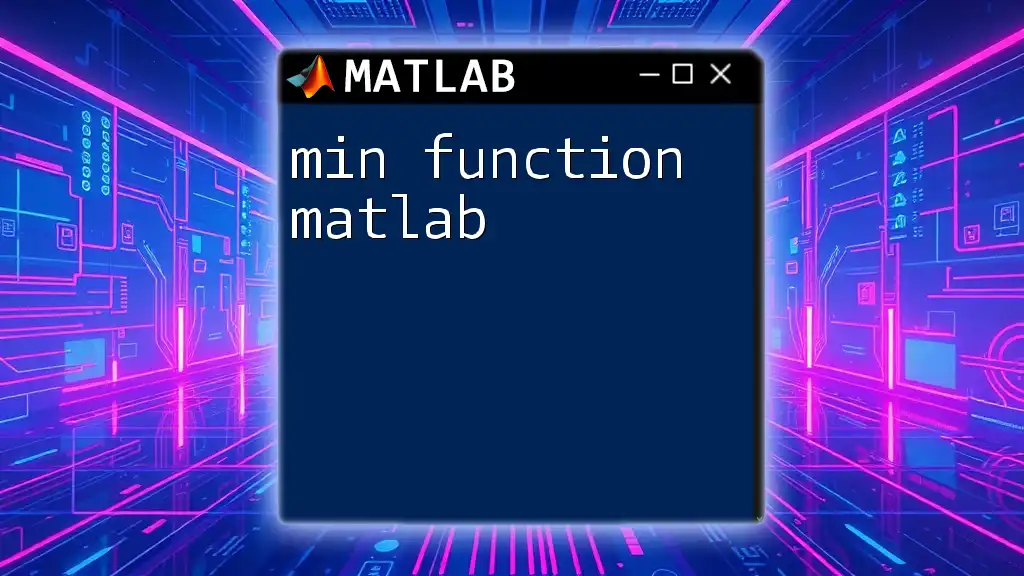
How to Use the `max` Function in Different Scenarios
Finding Maximum in a Vector
Finding the maximum value in a single vector is straightforward. Consider the following code:
vec = [3, 5, 2, 9, 6];
maxValue = max(vec);
In this example, `maxValue` will hold the value `9` since it is the highest number in the vector.
Finding Maximum in a Matrix
You can also calculate maximum values across different dimensions of a matrix. Here's how:
mat = [1, 2, 3; 4, 5, 6; 7, 8, 9];
maxInRows = max(mat, [], 2); % Maximum in each row
maxInCols = max(mat, [], 1); % Maximum in each column
After this execution, `maxInRows` will yield `[3; 6; 9]` and `maxInCols` will return `[7, 8, 9]`. This highlights how `max` can efficiently aggregate data based on your need.
Finding Maximum Between Two Arrays
To compare two arrays, you can also make use of the `max` function as follows:
A = [1, 4, 3];
B = [2, 3, 5];
C = max(A, B);
This will give you `C = [2, 4, 5]`, allowing for easy visual comparison of the elements of the two arrays.
Using the `max` Function with Additional Outputs
The capability to retrieve both maximum values and their indices can be vital when analyzing datasets:
[Y, I] = max(mat);
In this instance, `Y` will return the maximum values in the matrix, while `I` provides their respective indices, facilitating further investigation into data sources.
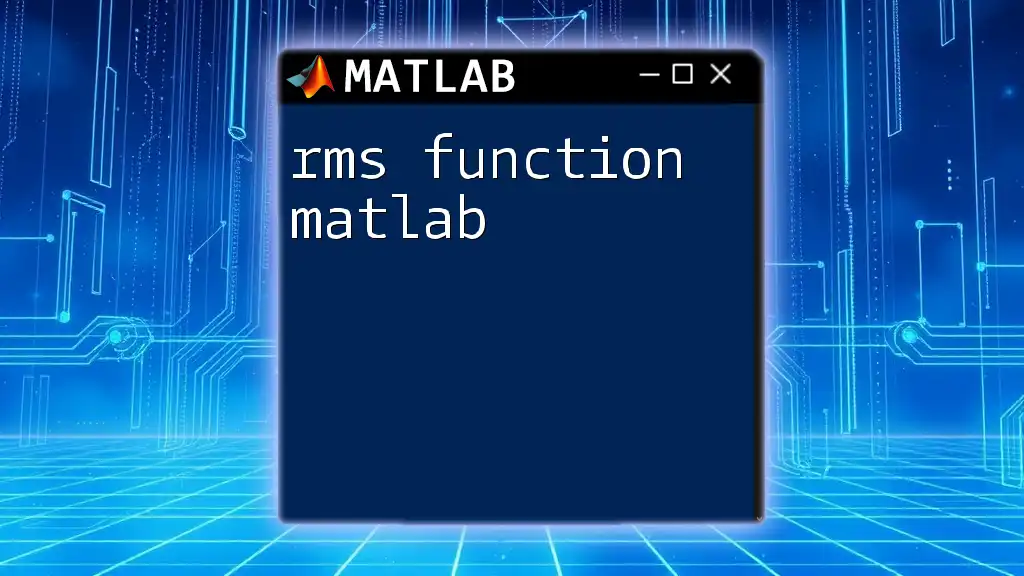
Advanced Usage of the `max` Function
Using `max` with Complex Numbers
MATLAB effectively handles complex numbers when determining maximum values. You may use the `max` function in this context as follows:
complexArray = [3+5i, 2+4i, 1+1i];
maxComplex = max(complexArray);
In this case, MATLAB compares the complex numbers based on their magnitude. The output will reflect the maximum based on absolute value.
Using `max` with NaN Values
Dealing with data that contains `NaN` (Not a Number) values can be tricky. Fortunately, the `max` function in MATLAB allows you to manage this effortlessly:
dataWithNaN = [1, NaN, 3, 4];
maxIgnoringNaN = max(dataWithNaN, [], 'omitnan');
This command will ignore any `NaN` values and return `4` as the maximum value, ensuring that your analysis remains accurate.
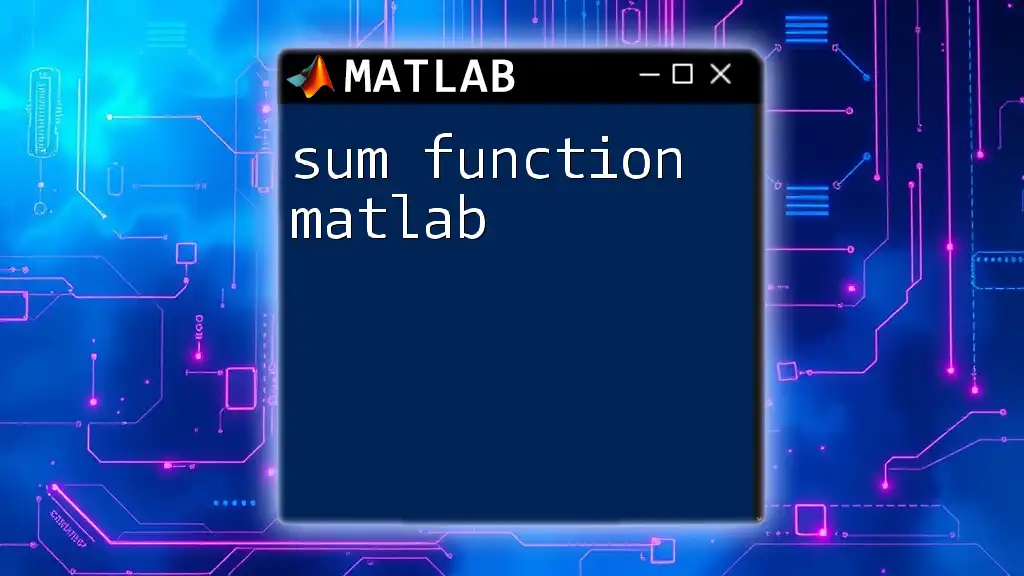
Special Considerations and Common Errors
Understanding Data Types
It is essential to pay attention to the data types you are working with. For instance, comparing integers and floating points could yield unexpected results. MATLAB will implicitly convert data types based on the first operand, which might lead to precision loss if not monitored.
Dimensionality Errors
A common mistake when using the `max` function is operating along the wrong dimension. Ensure you are clear about how your data is arranged before applying dimensional arguments with the `max` function.
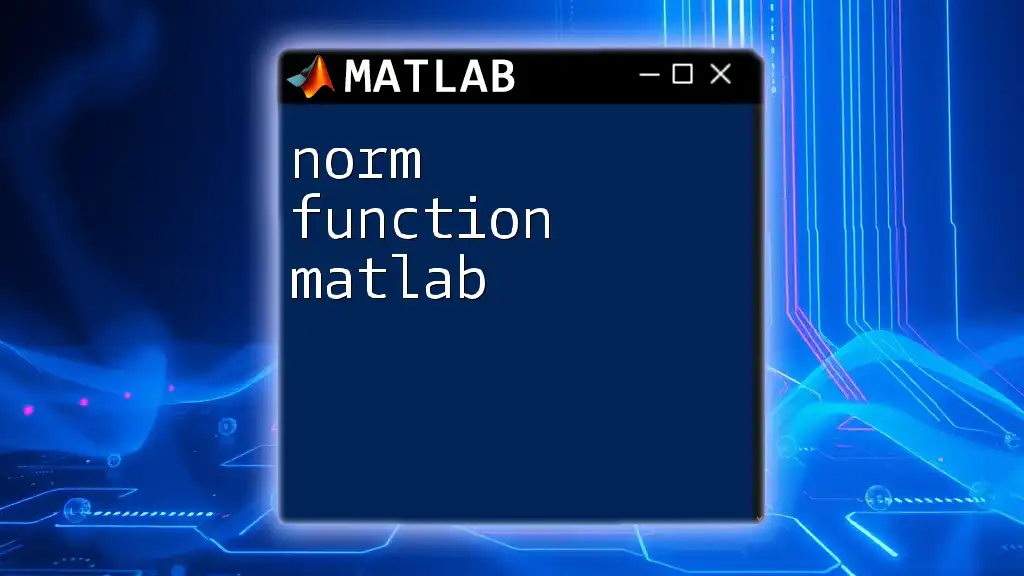
Conclusion
The `max` function in MATLAB is an incredibly versatile tool for identifying maximum values in various datasets. Understanding its syntax and applications can significantly improve your data analysis efficiency. To truly grasp its power, it's recommended to experiment with different examples and utilize this function in real-world scenarios.
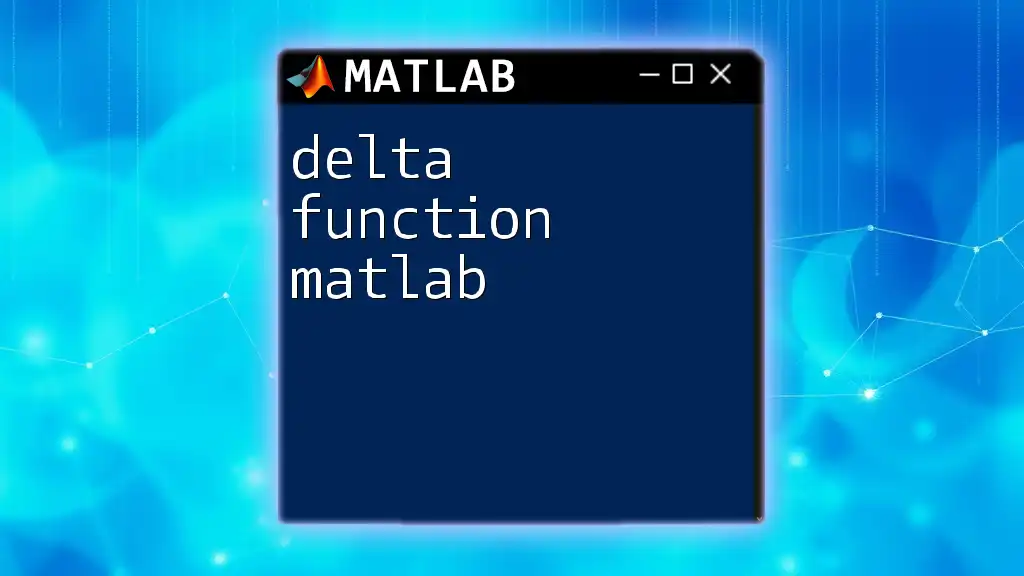
Additional Resources
For more detailed information, you can refer to the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/max.html). Engaging with exercises and projects utilizing the `max` function will further deepen your understanding and expertise in MATLAB programming.
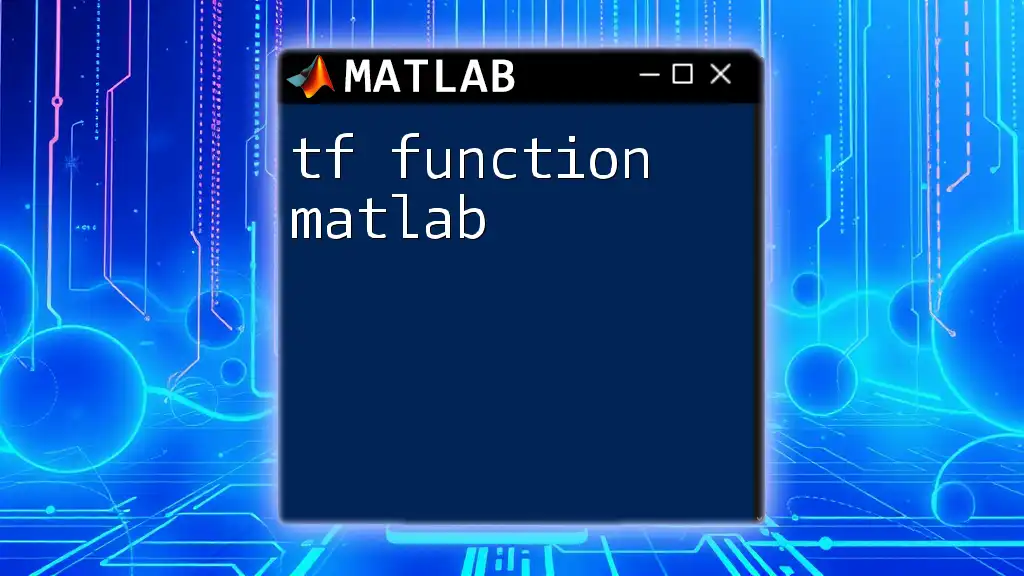
Call to Action
Ready to elevate your MATLAB skills? Join our courses designed to help you master MATLAB commands efficiently and effectively!