The `switch` statement in MATLAB is used to execute different blocks of code based on the value of a variable, allowing for cleaner and more organized conditional logic.
Here’s a simple example:
x = 2;
switch x
case 1
disp('x is one');
case 2
disp('x is two');
case 3
disp('x is three');
otherwise
disp('x is not one, two, or three');
end
Understanding the Syntax of `switch`
Basic Structure
The `switch` statement in MATLAB is a control flow statement that allows the execution of different sections of code based on the value of a specific expression. Its primary advantage is enhancing code readability by simplifying complex decision-making processes.
Syntax:
switch expression
case value1
% Code to execute if expression equals value1
case value2
% Code to execute if expression equals value2
otherwise
% Code to execute if expression does not match any case
end
This structure allows you to execute different code blocks based on the value of `expression`.
Components of a `switch` Statement
Switch Expression: The expression evaluated in the `switch` statement can be a variable or an expression. The value of this expression determines which case will be executed.
Case Labels: Each `case` provides a specific value to compare against the `switch` expression. If a match is found, the corresponding code block runs.
Otherwise Clause: The `otherwise` clause acts like a default case in other programming languages. It runs when none of the `case` labels match the evaluated expression.
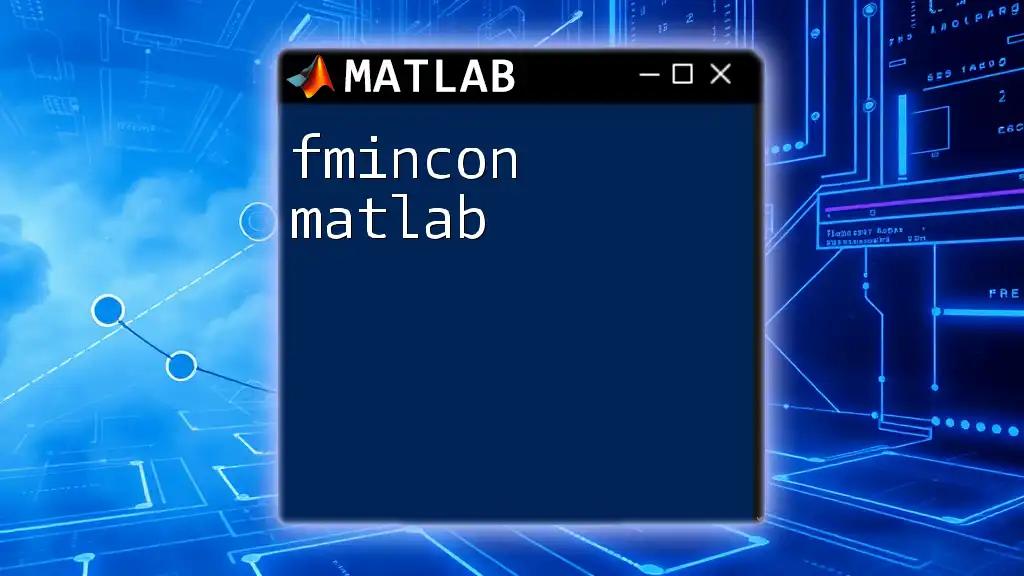
Practical Examples of `switch`
Example 1: Simple `switch` Statement
Here’s a straightforward example that demonstrates the basic use of the `switch` statement. This example evaluates a variable representing a color and displays a corresponding message.
color = 'red';
switch color
case 'red'
disp('Stop');
case 'yellow'
disp('Caution');
case 'green'
disp('Go');
otherwise
disp('Invalid color');
end
In this case, when the value of `color` is 'red', it executes the code under the first case and displays "Stop". Each case checks against the value of `color` and the `otherwise` clause provides a fallback if none matches.
Example 2: Using Numeric Values
The `switch` statement can also work effectively with numeric values. Look at the following example with grades:
grade = 85;
switch true
case grade >= 90
disp('A');
case grade >= 80
disp('B');
case grade >= 70
disp('C');
otherwise
disp('F');
end
In this scenario, rather than comparing a discrete value, we evaluate logical expressions directly in the `case`. Since 85 is greater than or equal to 80, the output will be "B". This illustrates the versatility of the `switch` statement, allowing for more complex logical conditions while still maintaining clarity.
Example 3: Multiple Cases
It's also possible to handle multiple labels within a single `case` clause, making your code more compact. Consider the following example:
day = 'Saturday';
switch day
case {'Saturday', 'Sunday'}
disp('Weekend');
case {'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'}
disp('Weekday');
otherwise
disp('Invalid day');
end
Here, the `case` for the weekend checks if `day` is either 'Saturday' or 'Sunday', allowing both values to lead to the same output without duplicating code. The result will be "Weekend" when `day` is 'Saturday'.
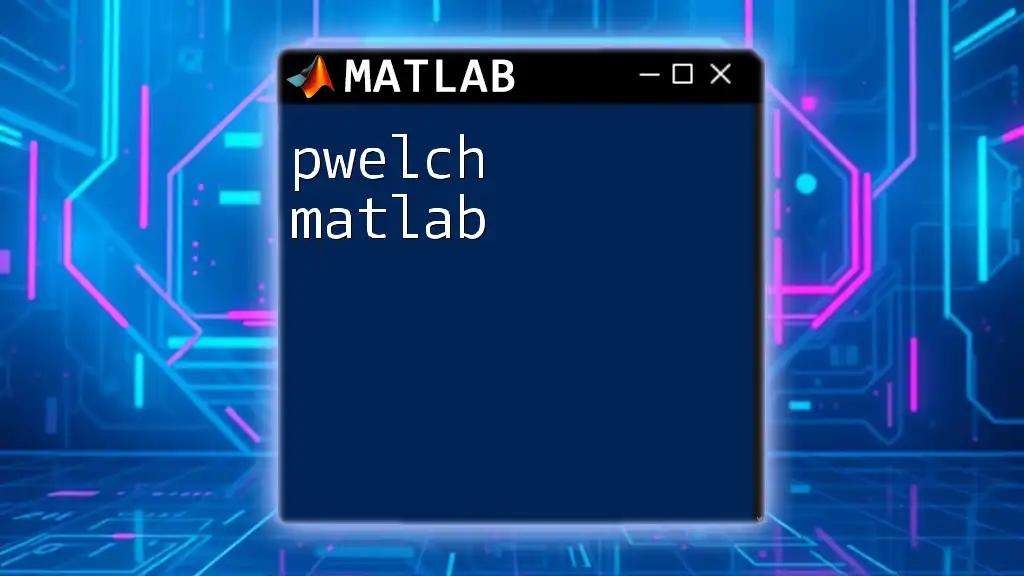
Advantages of Using `switch`
Readability
One of the primary benefits of using the `switch` statement is its ability to enhance code readability. Complex decision-making, particularly with many branches, can be convoluted when using nested `if` statements. The `switch` statement provides a linear structure that's easier to follow and maintain.
Performance
In scenarios where multiple comparisons need to be made, `switch` may offer better performance than `if` statements, as MATLAB can optimize the flow based on the explicit case checks. This is particularly notable when dealing with a large dataset or frequent function calls, where executing numerous `if` checks can slow down processing time.
Flexibility
The `switch` statement is flexible; it allows not just simple value comparisons but also logical and grouped conditions. This adaptability makes it suitable for a wide range of applications across different domains in MATLAB programming.
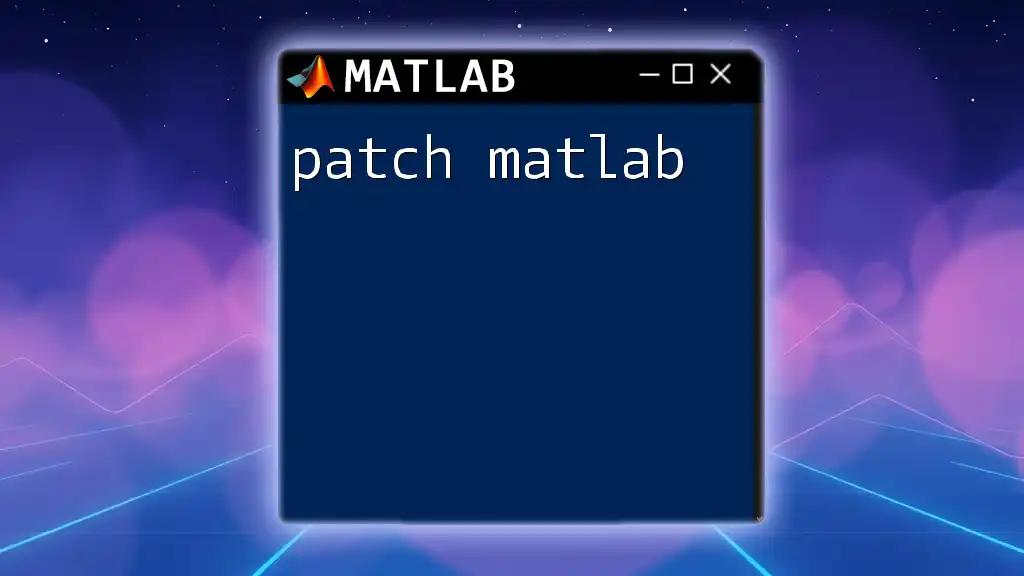
Common Pitfalls and Best Practices
Using Uninitialized Variables
A common mistake is failing to initialize the `switch` expression variable before its use. This can lead to unexpected results or errors since MATLAB will not know what value to compare against. Always ensure that the variable is properly initialized before the `switch` statement.
Overlapping Cases
While it's possible to have overlapping `case` labels, this can lead to confusing behavior. If multiple cases could be true simultaneously, only the first matching `case` will execute, which may not be the intended outcome. To avoid ambiguity, always ensure that your `case` labels are distinct.
Keeping It Clean
Maintaining readability and efficiency in your `switch` statements is crucial. Avoid overly complex expressions or too many cases within a single switch. Instead, consider breaking them into separate functions if the logic becomes convoluted.
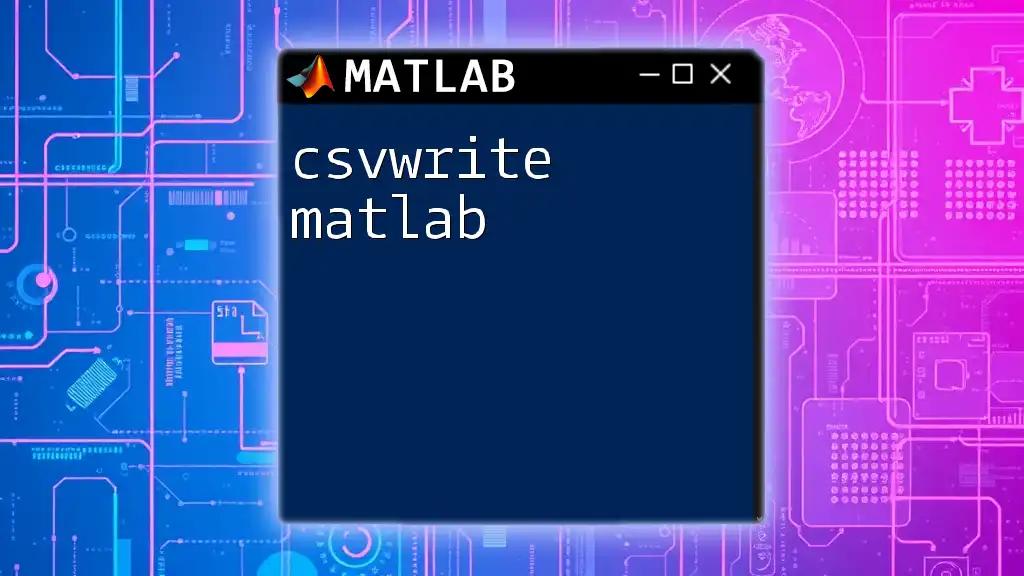
Conclusion
The `switch` statement in MATLAB serves as a powerful tool for managing control flow in your scripts and functions. Its structure not only enhances code clarity but also allows for more efficient performance, particularly when dealing with multiple cases. As you practice using `switch en matlab`, you'll find it beneficial for simplifying your code and improving overall functionality.
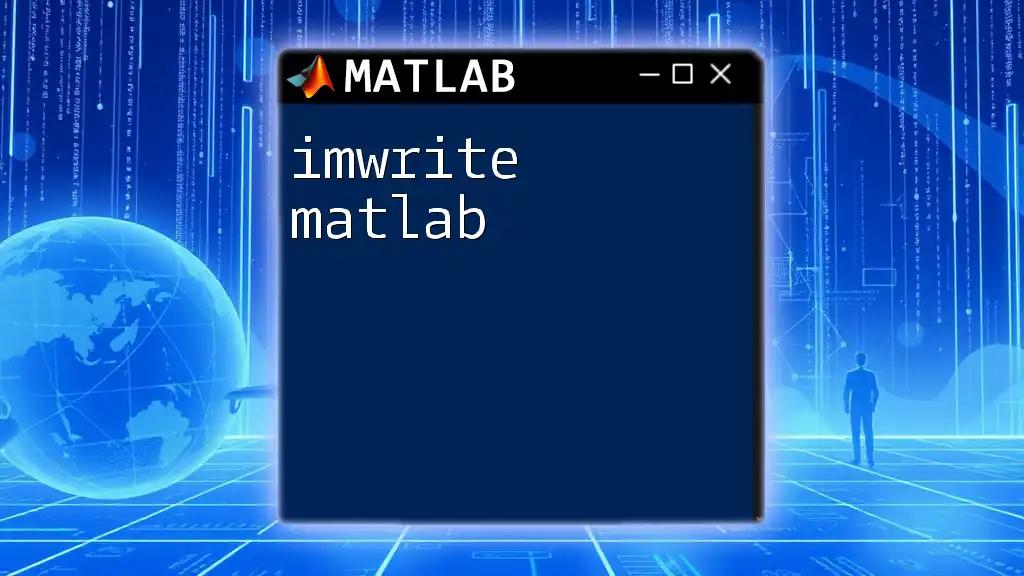
Additional Resources
For further reading and exploration of the `switch` statement, consider visiting MATLAB's [official documentation](https://www.mathworks.com/help/matlab/ref/switch.html). Additionally, look for recommended books or online tutorials that can deepen your understanding of MATLAB programming principles.
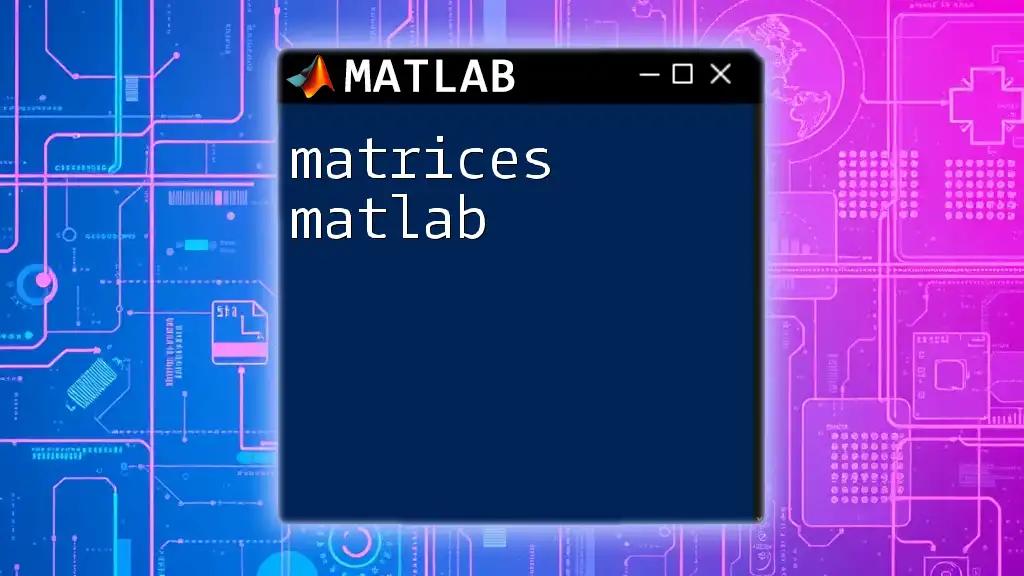
Call to Action
Have you tried using the `switch` statement in MATLAB? Share your experiences or any questions you may have in the comments below! Don’t forget to subscribe for more insightful MATLAB tips and tutorials!