The `patch` function in MATLAB is used to create filled polygons based on specified vertex coordinates and color options.
Here's a code snippet that demonstrates the usage of the `patch` function:
% Define the vertices of the polygon
x = [1 2 3 1];
y = [1 4 1 1];
% Create a filled polygon using the patch function
figure;
patch(x, y, 'cyan');
title('Filled Polygon using patch');
axis equal;
Understanding the Basics of `patch`
What is `patch`?
The `patch` function in MATLAB is a powerful tool that allows users to create filled polygons in both 2D and 3D graphics. With `patch`, you can visualize complex shapes and data points clearly, making it an essential command for graphical representation in various fields such as engineering, mathematics, and scientific research.
Basic Syntax of `patch`
The general syntax for using the `patch` function is as follows:
patch(X, Y, C)
Here, the parameters are defined as:
- X: Represents the horizontal coordinates of the vertices of the polygon.
- Y: Represents the vertical coordinates of the vertices.
- C: Defines the color of the patch, which can be specified as a color string (e.g., 'r' for red) or an RGB triplet (e.g., [0 1 0] for green).
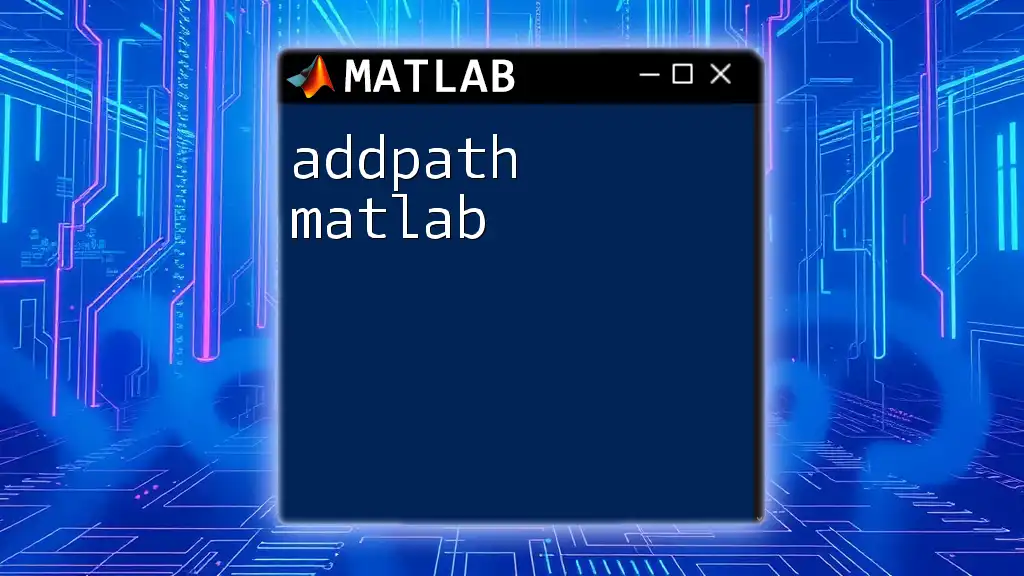
Creating Basic Patches
Example of a Simple Patch
Creating a simple patch is straightforward. Here’s a basic example to illustrate how to use the `patch` command:
X = [1 2 3];
Y = [1 3 2];
C = 'r'; % Fill color red
patch(X, Y, C);
title('Basic Patch Example');
axis equal;
In this example, the code defines three vertices that form a triangle. The color specified is red, so the resulting triangle will be filled with this color. The `axis equal` command ensures that the units are equal on both axes, allowing for a more accurate representation of the shape.
Creating Multiple Patches
To create multiple patches in a single plot, you can use a loop or structured code:
hold on; % Hold the current plot
for k = 1:3
patch(X+k, Y+k, rand(1,3)); % Using random colors
end
hold off;
In this code snippet, the `hold on` command allows subsequent patches to be drawn on the same figure without erasing previous ones. The use of `rand(1,3)` produces different random colors for each patch, offering a vibrant and colorful output.
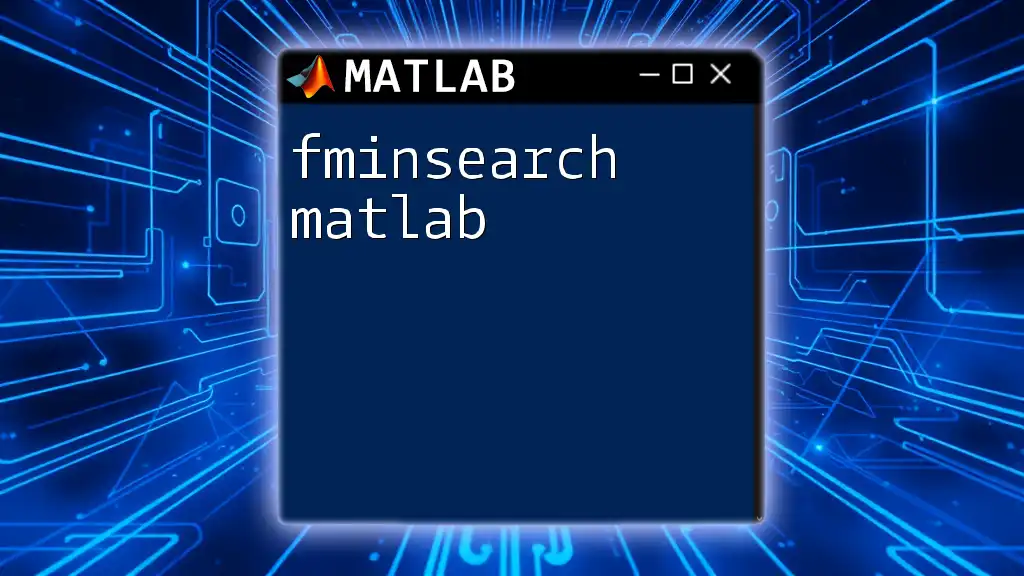
Customizing Patches
Color and Transparency
Customizing the color and transparency of your patches can enhance visual clarity and artistic expression. You can specify colors using RGB values as well:
C = [0 0.5 0; 0.8 0.1 0.1]; % RGB values
alpha = 0.5; % Transparency level
patch(X, Y, C(1,:), 'FaceAlpha', alpha);
In this case, the first patch is filled with a green color defined by its RGB triplet, and the transparency level is set to 0.5, resulting in a semi-transparent patch that allows underlying graphics to be visible.
Edge Properties
The edges of the patches can also be customized to improve the visual distinction between shapes:
patch(X, Y, C(1,:), 'EdgeColor', 'k', 'LineWidth', 2);
Here, the edge color is set to black (`'EdgeColor', 'k'`), and the line width is increased, making it easier to differentiate the boundaries of the patch.
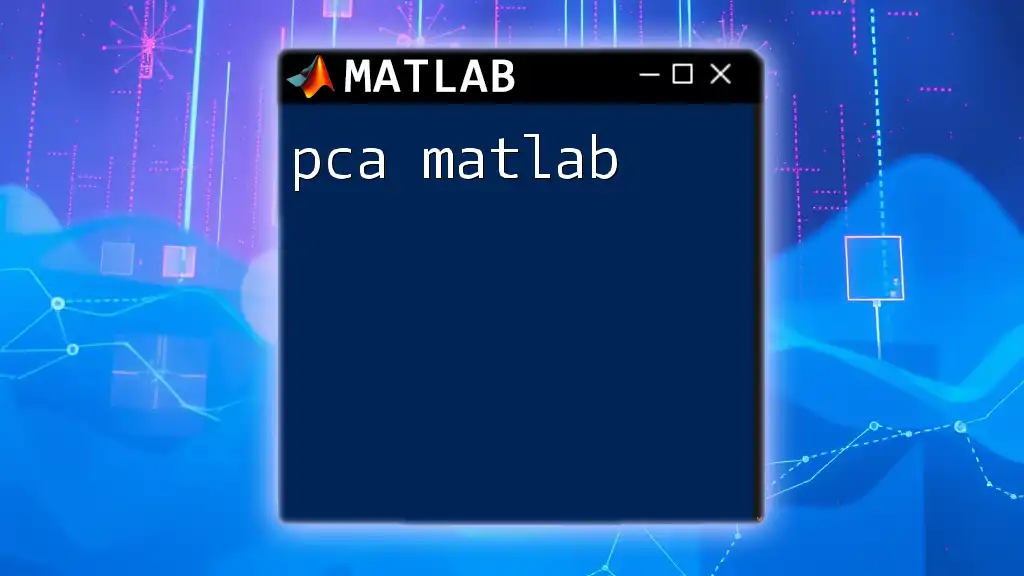
Advanced Usage of `patch`
3D Patches
The `patch` function isn't limited to creating 2D shapes; it can also be used in 3D graphics. This capability is particularly beneficial for visualizing spatial data or objects:
X = [0 1 1 0];
Y = [0 0 1 1];
Z = [1 1 1 1];
patch(X, Y, Z, 'g'); % 3D Patch in green
view(3); % Set a 3D view
In this example, a square patch is drawn in a 3D space. The `view(3)` command arranges the view to provide depth, allowing the viewer to see the shape's three-dimensional structure.
Using `patch` with Coordinates Grids
For creating complex shapes, you can utilize coordinate grids or mesh grids. This allows you to illustrate intricate surfaces effectively, especially when dealing with mathematical equations or physical simulations.
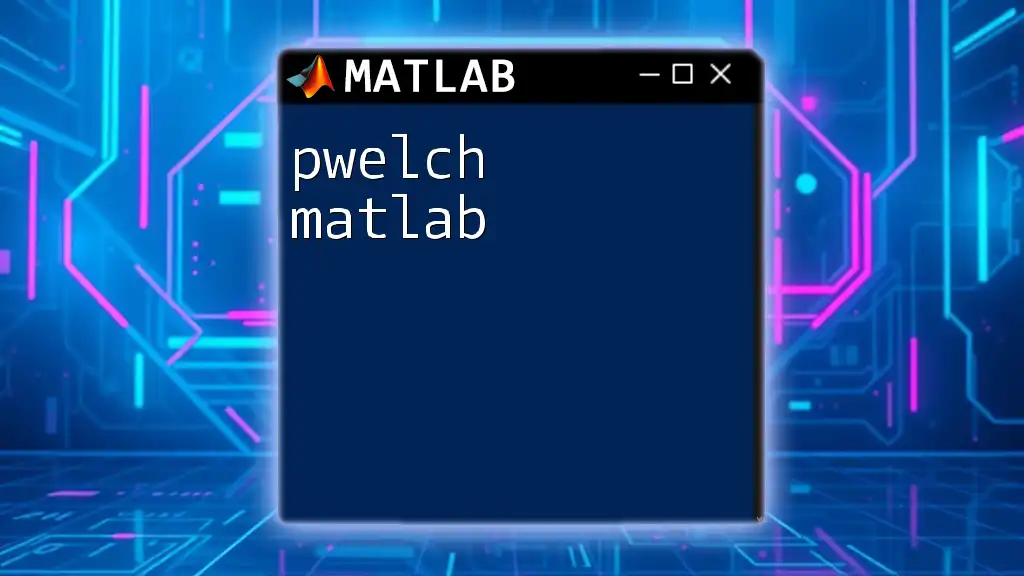
Practical Applications of `patch`
Visualization in Engineering Simulations
In fields like civil or mechanical engineering, the ability to visualize designs and models graphically is paramount. Using the `patch` function, engineers can create models of structures, study stress distribution, and observe fluid flow patterns.
Data Representation
The `patch` function is also effective for representing datasets visually. For instance, it can be utilized to create heat maps or geographical data visualizations, making it easier to interpret large sets of information intuitively.
Interactive Patches
Enhancing user interaction is another remarkable use case. By integrating callbacks and interactive features, users can click on patches to reveal additional data, ensuring an engaging experience when presenting graphical data.
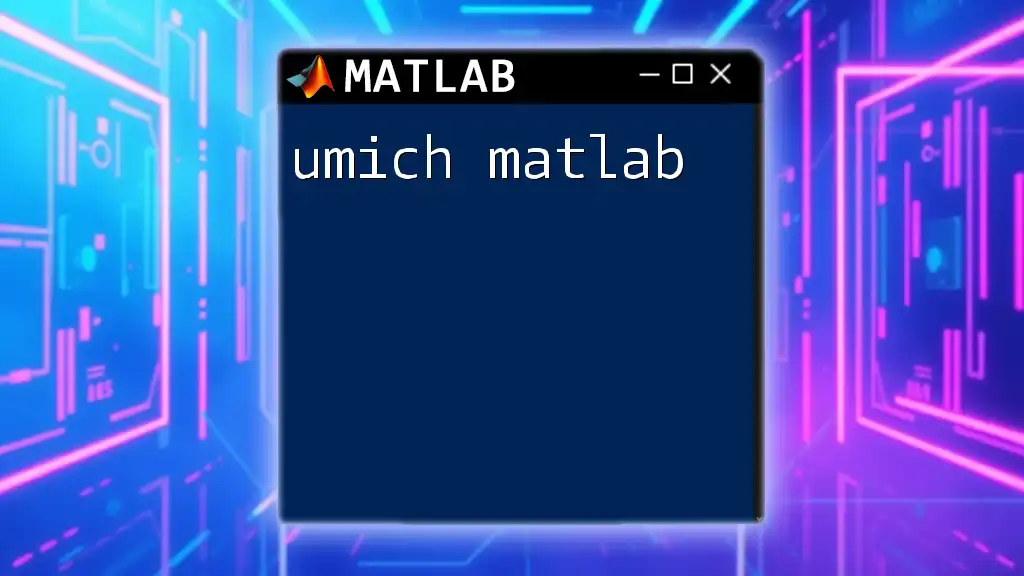
Troubleshooting Common Issues with `patch`
Common Errors and Fixes
While working with the `patch` function, users may encounter some common errors, such as:
-
Building Patches Exceeding Bounds: This often leads to unexpected output. To solve this, make sure that the coordinates you provide are within the bounds of the axes.
-
Color Not Displaying Correctly: If the expected color doesn’t show, verify the format of the color specification. Ensure that RGB values are in the range of [0, 1] and are properly formatted.
Performance Considerations
When visualizing a significant number of patches, performance can degrade, slowing down rendering. To optimize this, consider using fewer, larger patches or grouping related data points into a single patch, reducing rendering overhead while retaining important visual information.
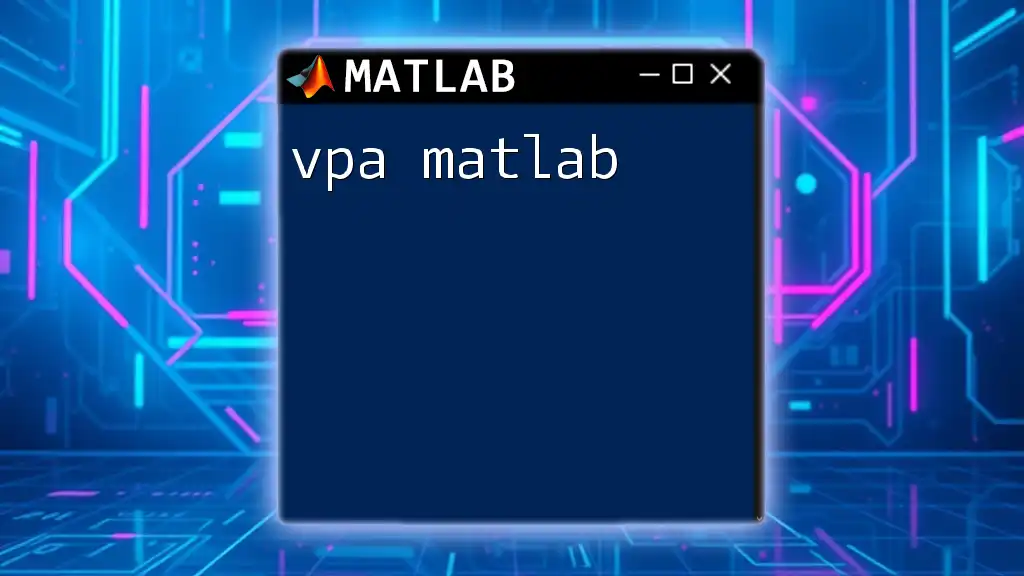
Conclusion
The `patch` function in MATLAB is an indispensable tool for anyone looking to create engaging and informative visualizations. From simple shapes to complex 3D models, mastering this function opens up a world of opportunities in data presentation, simulation, and graphical analysis. Experiment with the diverse features of `patch`, and let your creativity merge with functionality to produce stunning graphics that convey powerful messages in your work.
Feel free to explore additional resources and documentation to enhance your understanding and application of the `patch` function in MATLAB.