The "sorted" function in MATLAB efficiently arranges the elements of an array in ascending order and can return both the sorted array and their original indices.
% Example of using the sorted function in MATLAB
A = [3, 1, 4, 1, 5, 9];
[sorted_A, original_indices] = sort(A);
Understanding Sorting in MATLAB
What is Sorting?
Sorting refers to the process of arranging data in a particular order—either ascending or descending. In programming, sorting is essential as it helps in organizing data, making it easier to analyze or visualize. In MATLAB, the sorted arrangement of data facilitates tasks such as searching, reporting, and preparing datasets for machine learning.
Types of Sorting in MATLAB
MATLAB offers various built-in functions for sorting data, which significantly simplifies the coding process compared to manual sorting techniques. Mastering these sorting functions is essential for effective data manipulation.
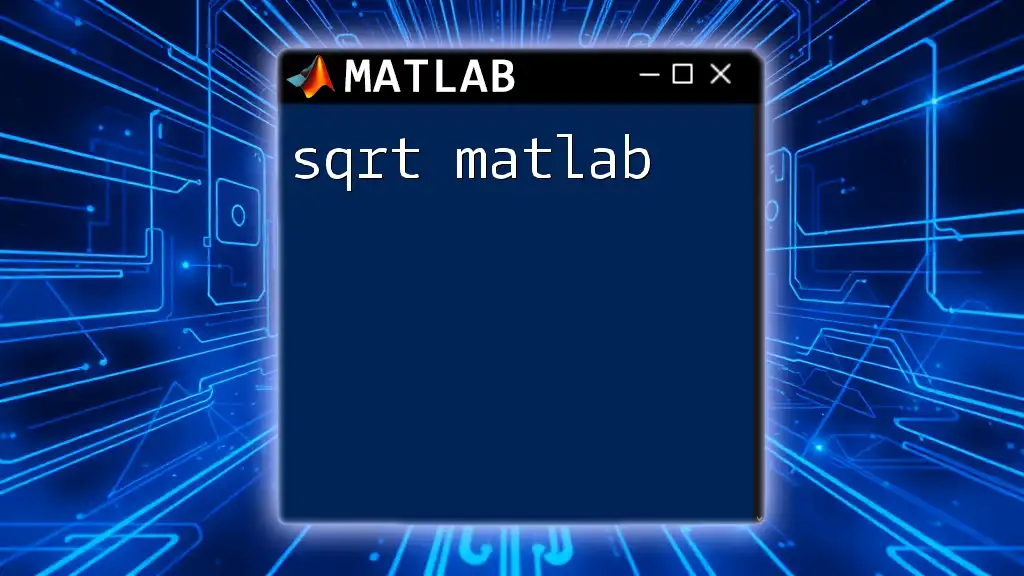
Using the `sort` Function
Introduction to the `sort` Function
The `sort` function is one of the most common ways to sort data in MATLAB. Its primary syntax is straightforward:
B = sort(A)
Here, A represents the input array (which can be of any dimension), and B is the output array that contains the sorted elements.
Parameters of `sort`
Dimension Parameter
When handling multi-dimensional arrays, it's crucial to specify the dimension along which the sorting will occur. By default, MATLAB sorts along the first dimension.
A = [3, 1, 4; 5, 9, 2];
sortedA = sort(A, 2); % Sorts each row
In the above example, the rows of matrix A are sorted individually, resulting in:
sortedA =
1 3 4
2 5 9
Order Parameter
MATLAB allows users to customize the sorting order by using an optional parameter that specifies ascending or descending order.
A = [3, 1, 4, 1, 5, 9];
sortedAscend = sort(A, 'ascend'); % Ascending order
sortedDescend = sort(A, 'descend'); % Descending order
This flexibility allows for efficient data arrangement depending on the analysis needs.
Sorting Strings
Sorting isn't limited to numeric arrays; you can also sort character arrays and string arrays in MATLAB.
strArray = ['apple'; 'orange'; 'banana'];
sortedStr = sort(strArray);
In this case, sortedStr will return:
sortedStr =
'apple '
'banana'
'orange'
The sorting function organizes the strings in alphabetical order, demonstrating the utility of sorting for categorical data.
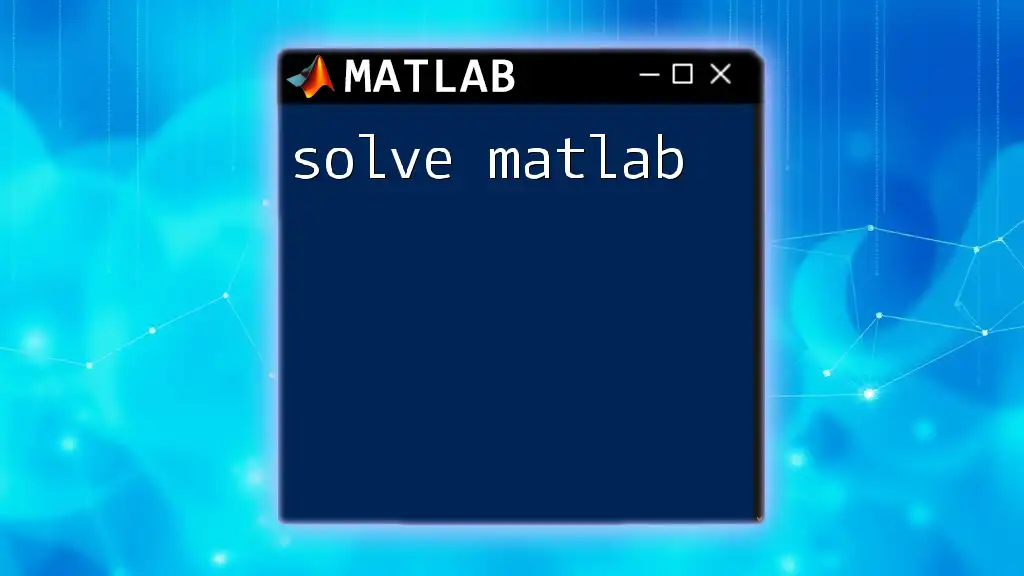
Sorting with Additional Functions
`sortrows` Function
An important tool for sorting matrices or tables is the `sortrows` function, which allows sorting by specific columns.
A = [1, 2; 3, 1; 2, 3];
sortedRows = sortrows(A, 1); % Sorts by the first column
The above code sorts the rows of A based on the values in the first column, providing a comprehensive perspective when dealing with datasets.
`unique` Function
The `unique` function allows you to sort and return unique values from an array, which is particularly useful in data preprocessing.
A = [4, 2, 2, 8, 3, 3];
[C, ia] = unique(A);
sortedUnique = sort(C);
In this example, C contains the unique values of A, and then they are sorted, showcasing an important aspect of data cleaning.
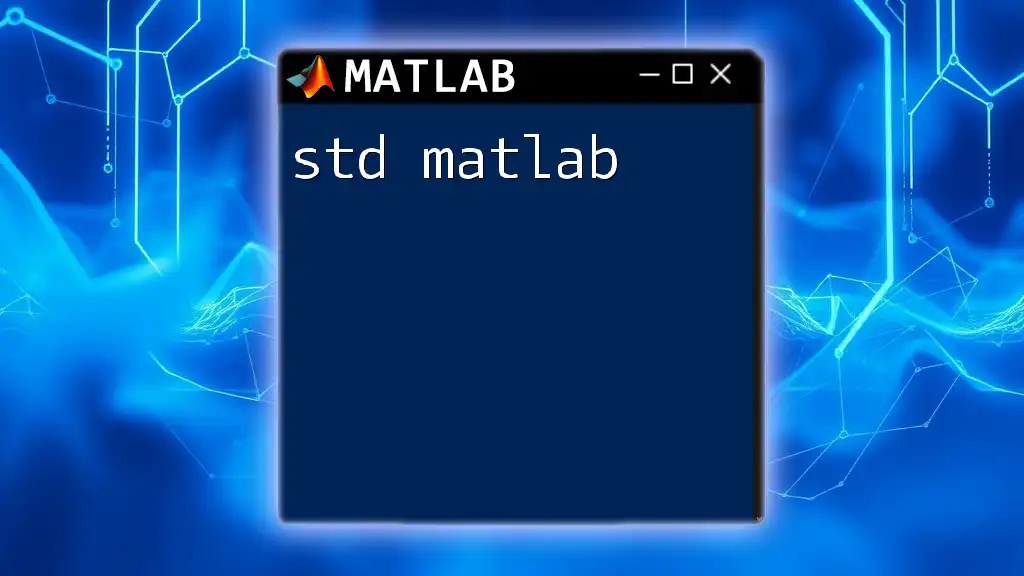
Custom Sorting
Sorting with User-defined Functions
For more specialized sorting needs, you might consider creating a custom sorting function. This enables you to define sorting logic tailored to specific data structure requirements.
function sortedArray = customSort(myArray)
% Custom sorting logic
sortedArray = sort(myArray, 'ascend');
end
This function takes an array as input and returns it sorted in ascending order, demonstrating the simplicity and power of MATLAB functions.
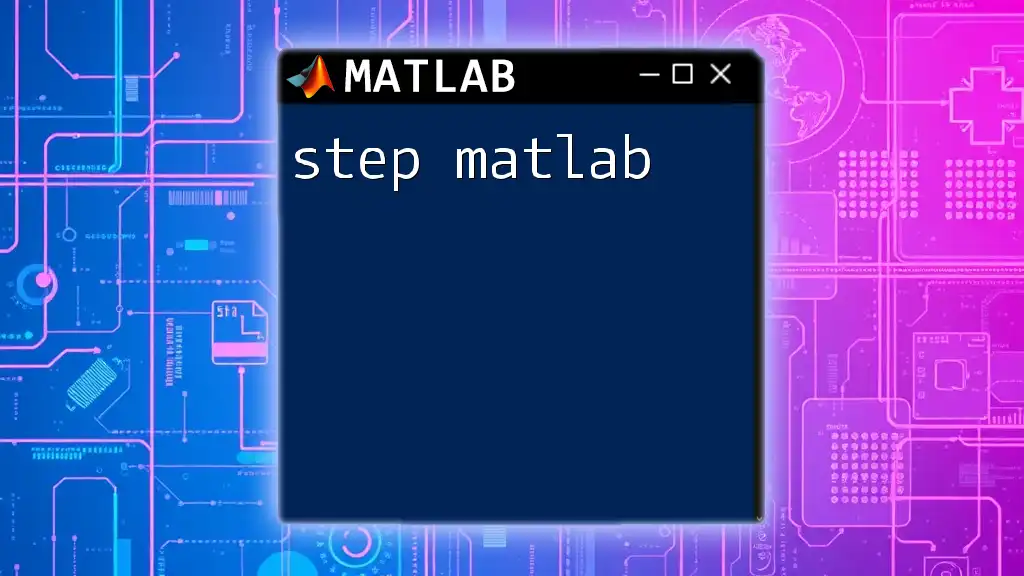
Performance Considerations
Efficiency of Sorting in MATLAB
Sorting can become computationally intensive, especially when dealing with large datasets. MATLAB employs optimized sorting algorithms under the hood, ensuring efficient performance. Understanding these complexities can help you choose the appropriate sorting method based on your dataset size and complexity.
Tips for Improving Sorting Performance
- Ensure array dimensions are consistent to avoid unnecessary computational overhead.
- If applicable, preallocate memory for larger datasets to enhance performance.
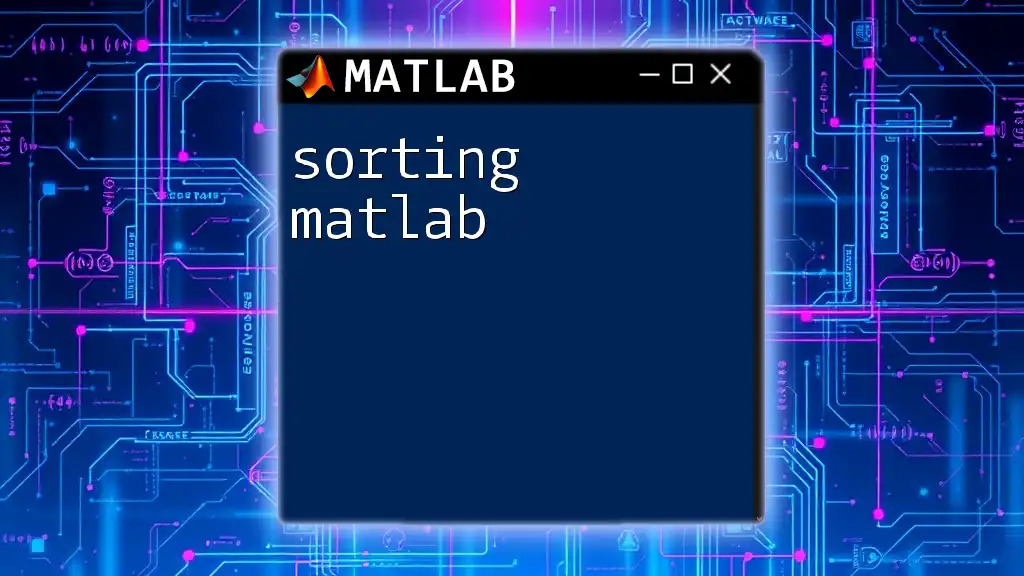
Best Practices for Sorting in MATLAB
General Tips on Sorting Data
When working with sorting operations, keep the following best practices in mind:
- Understand your data structure: Ensure that your data is organized correctly before sorting to avoid unexpected results.
- Choose the right function: Identify which built-in function best suits your sorting needs.
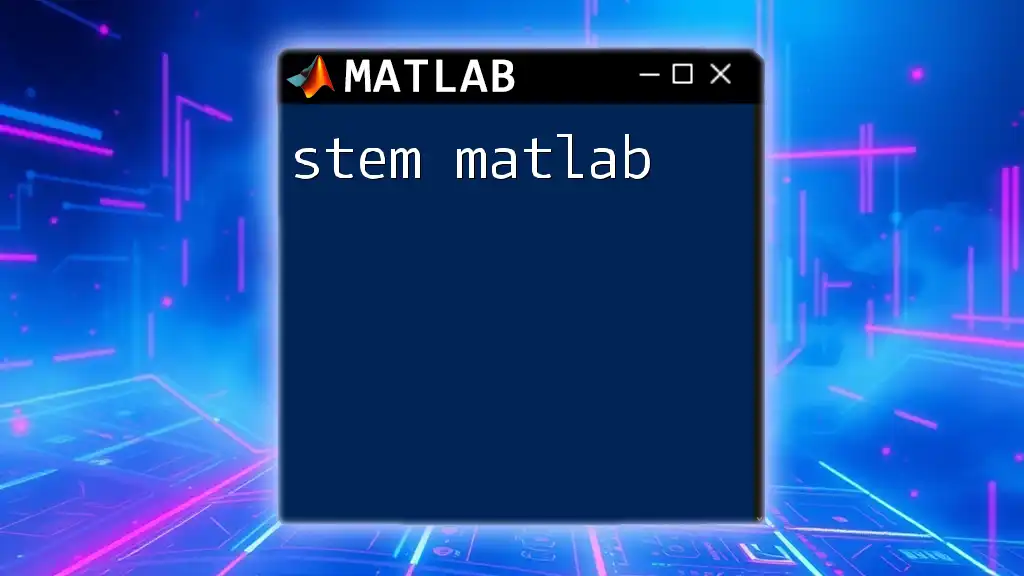
Real-world Applications of Sorted Data
Case Studies
Sorted data plays a crucial role in areas such as data analysis, reporting, and machine learning preprocessing. For instance, pre-sorting dataset features can help in faster model training and better performance evaluation.
Example Scenarios
In visualization tasks, sorted data makes it easier to create graphs and charts, facilitating clearer communication of insights in reports.
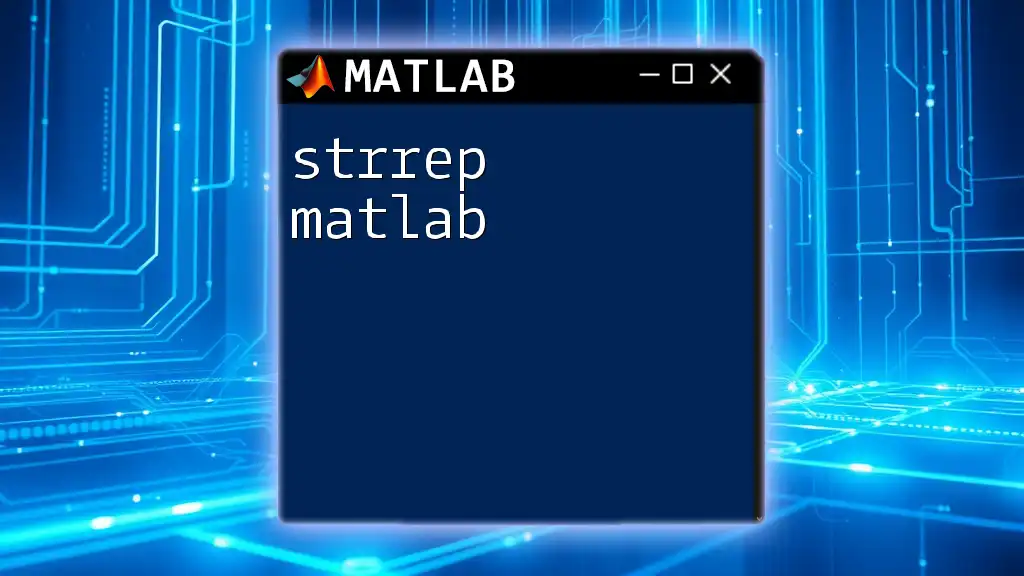
Conclusion
Sorting is a fundamental concept in MATLAB, essential for organizing and analyzing data effectively. Familiarizing yourself with MATLAB's built-in sorting functions and techniques will empower you to handle data more efficiently.
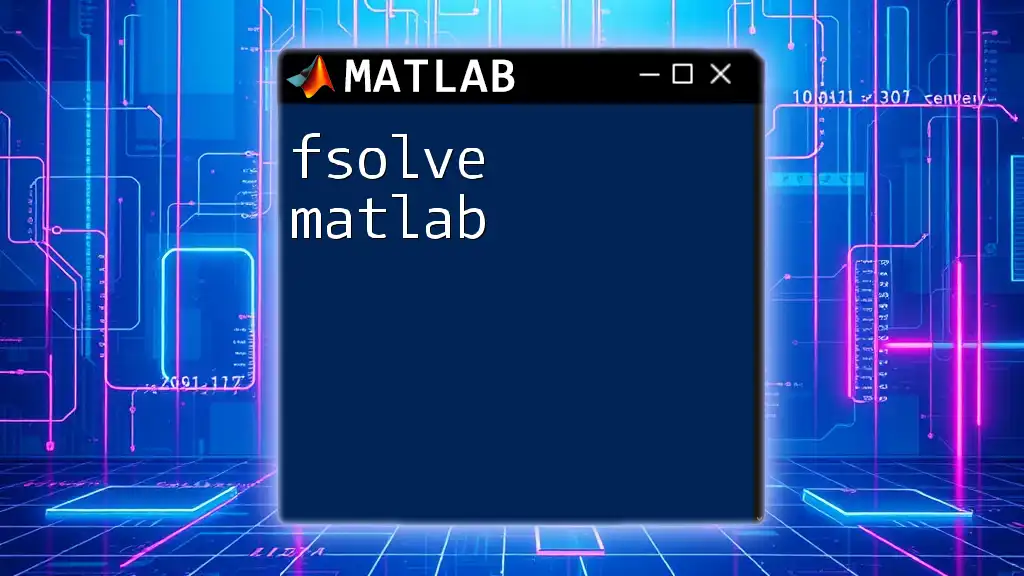
Call to Action
Ready to enhance your MATLAB skills? Consider exploring our courses on sorting techniques and other MATLAB functionalities, making your numerical analysis quicker and more effective. Check out our additional resources for comprehensive learning and community support.