"Umich MATLAB" refers to the MATLAB programming resources and community available at the University of Michigan, which includes tutorials, support, and examples for efficiently utilizing MATLAB commands.
Here's a simple example of how to create a plot in MATLAB:
x = 0:0.1:10; % Create a vector from 0 to 10 with increments of 0.1
y = sin(x); % Calculate the sine of each x value
plot(x, y); % Plot the sine curve
title('Sine Function'); % Add a title to the plot
xlabel('x'); % Label x-axis
ylabel('sin(x)'); % Label y-axis
What is MATLAB?
MATLAB, short for Matrix Laboratory, is a powerful programming environment used for numerical computing. Initially developed for linear algebra and matrix computations, it has evolved into a versatile tool widely utilized in engineering, scientific research, and beyond.
Key features of MATLAB include:
- Extensive mathematical functions and algorithms
- Robust data visualization tools
- Ability to easily interface with other programming languages
- An interactive environment for rapid application development
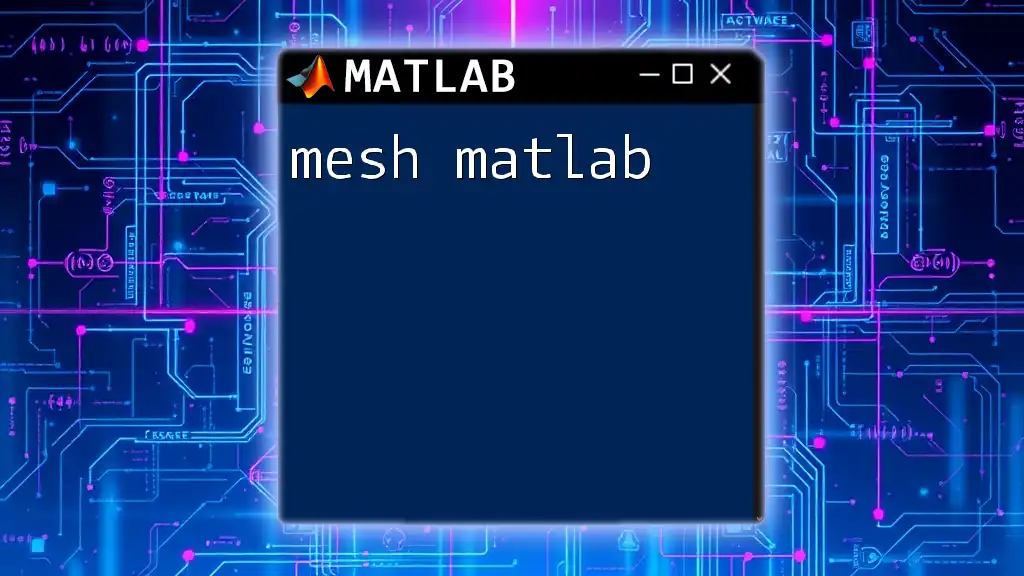
Why Learn MATLAB?
Learning MATLAB is essential for students and professionals alike, especially in technical fields. Here are some compelling reasons:
- Applications Across Disciplines: From engineering to finance, MATLAB is extensively used in simulations, data analysis, and algorithm development.
- Employment Prospects: There is a significant demand for MATLAB skills in the job market. Many companies seek candidates proficient in MATLAB for engineering roles, data science, and computational research.
- Personal Projects: Knowing MATLAB allows you to undertake personal and academic projects with efficiency, leveraging its extensive capabilities for quicker results.
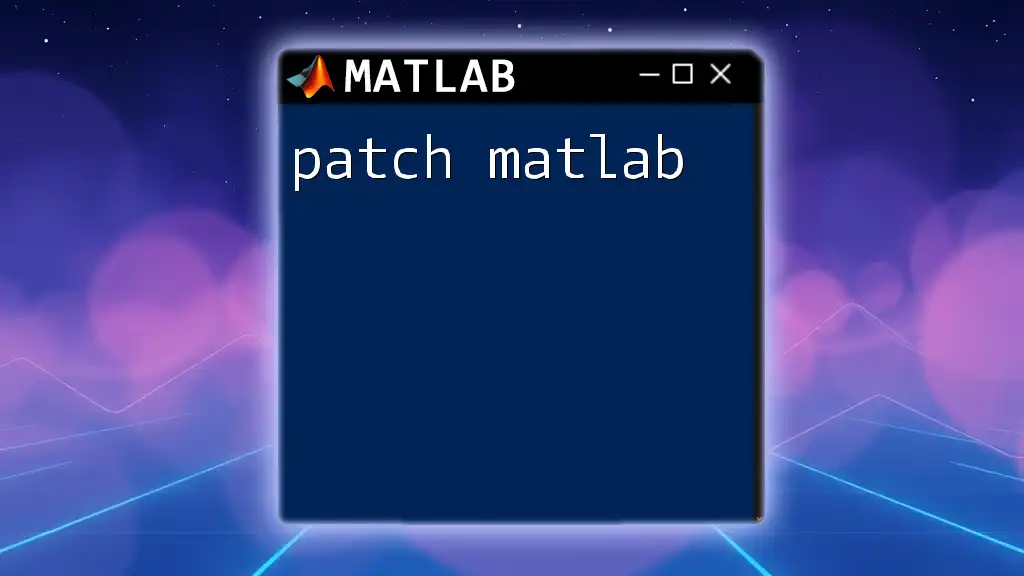
Getting Started with MATLAB in UMich
How to Access MATLAB
At the University of Michigan, students can easily access MATLAB through university licenses. You can download it directly from the MathWorks website using your university credentials.
Creating an Account
To access learning resources and software, create a MathWorks account. This account will grant you access to educational discounts and various tutorials specifically designed for UMich students.
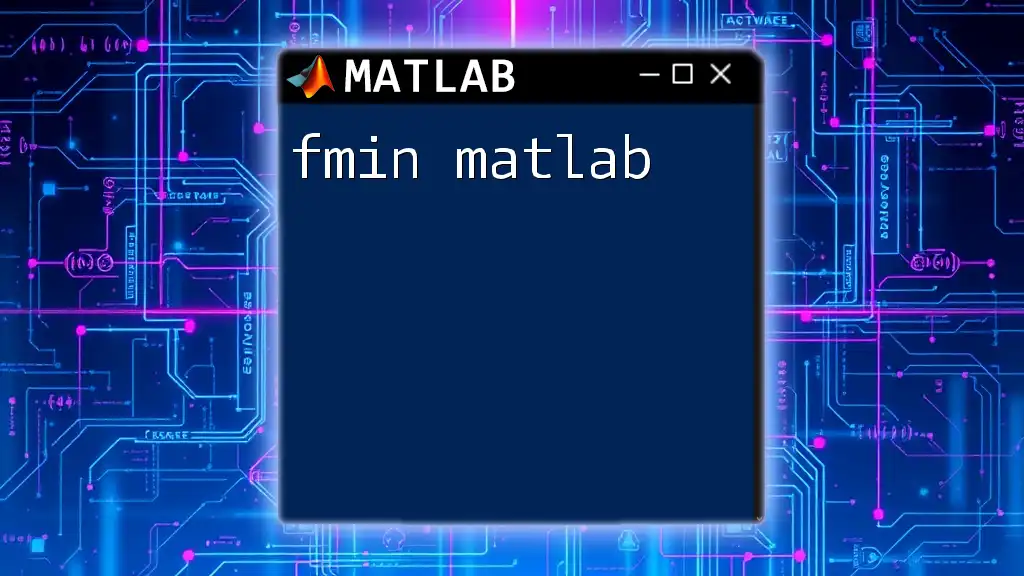
Understanding the MATLAB Interface
Overview of the User Interface
The MATLAB interface is intuitive and consists of several key components:
- Command Window: This is where you enter commands and view outputs in real time.
- Editor: An integrated environment for writing and running scripts and functions.
- Workspace: Displays your variables and their current values, aiding in effective debugging and analysis.
Common Commands and Shortcuts
Familiarizing yourself with common commands and shortcuts is crucial for improving efficiency. For instance, to create a variable, you can simply type:
a = 5; % Creating a numeric variable
In addition, mastering keyboard shortcuts can save you valuable time during coding sessions.
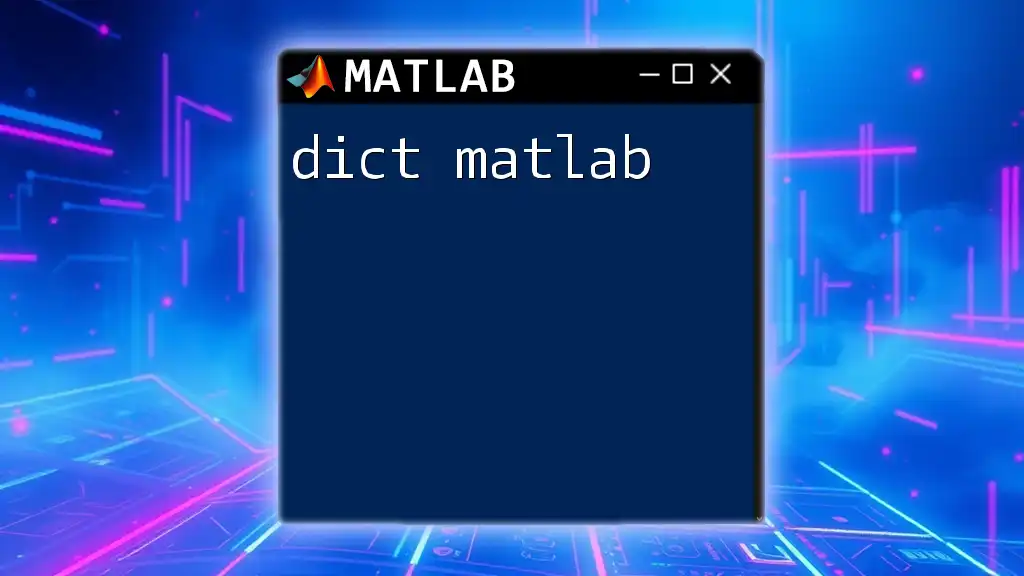
Basic MATLAB Commands
Variables and Data Types
MATLAB primarily deals with arrays and matrices. Understanding variable types is foundational to using MATLAB effectively. You can create various data types, including:
- Numeric: Basic numbers and matrices
- Logical: Boolean values (true or false)
- Cell arrays: To store mixed data types
Here’s how to create an array:
A = [1, 2, 3; 4, 5, 6]; % A 2x3 matrix
Basic Operations
MATLAB allows you to perform a variety of arithmetic operations easily. For example, if you want to add a number to a variable, use:
sum = a + 10; % Adding 10 to variable 'a'
You can perform a range of operations on matrices as well, including addition, subtraction, and multiplication.
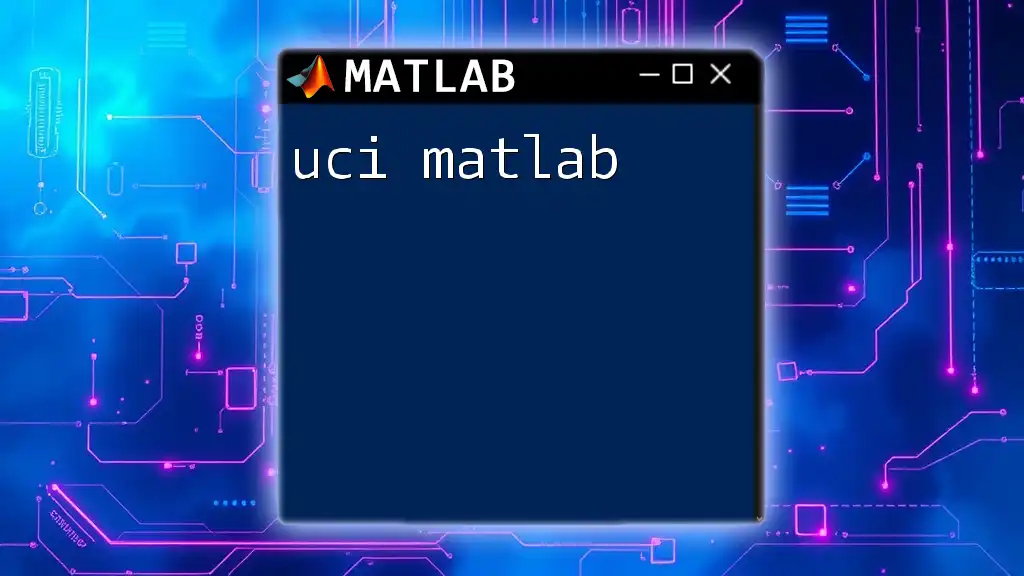
Intermediate MATLAB Commands
Control Structures
Control structures are vital for programming logic. The if-else statement controls the flow of execution:
if a > 10
disp('A is greater than 10');
else
disp('A is not greater than 10');
end
Loops, such as `for` and `while`, allow you to execute code repeatedly. Here’s an example using a `for` loop:
for i = 1:5
disp(i); % Displays numbers from 1 to 5
end
Functions and Scripts
Creating and using functions enhances code reusability. Functions allow you to package MATLAB code into manageable units. Here’s an example of a simple function:
function result = square(x)
result = x^2; % Returns the square of the input
end
Scripts are similar but do not accept inputs or provide outputs in the same structured way.
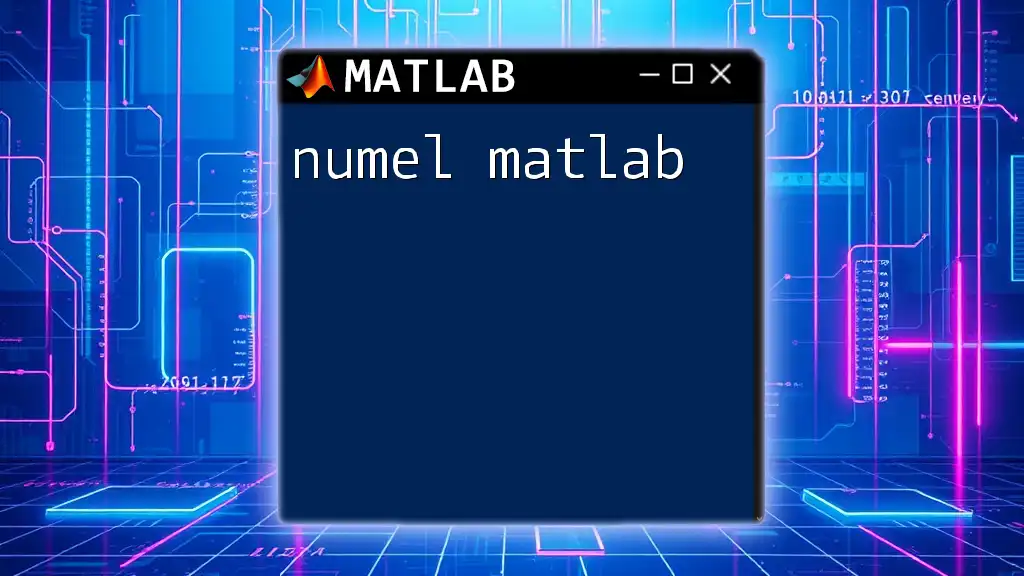
Advanced MATLAB Features
Data Visualization
MATLAB excels at data visualization. Creating plots can be achieved with minimal code. A basic sine wave can be plotted as follows:
x = linspace(0, 2*pi, 100);
y = sin(x);
plot(x, y); % Basic sine wave plot
These graphs can be customized with titles, labels, and legends, making your data visually comprehensible.
Simulink Basics
Simulink, an integral part of MATLAB, is used for modeling dynamic systems through block diagrams. Although it requires a hands-on approach, it empowers users to simulate complex systems without extensive programming.
Creating a Simple Simulink Model
You can begin with a basic model by dragging and dropping blocks into the workspace. Components like Sum, Gain, and Scope can be connected to simulate a simple system.
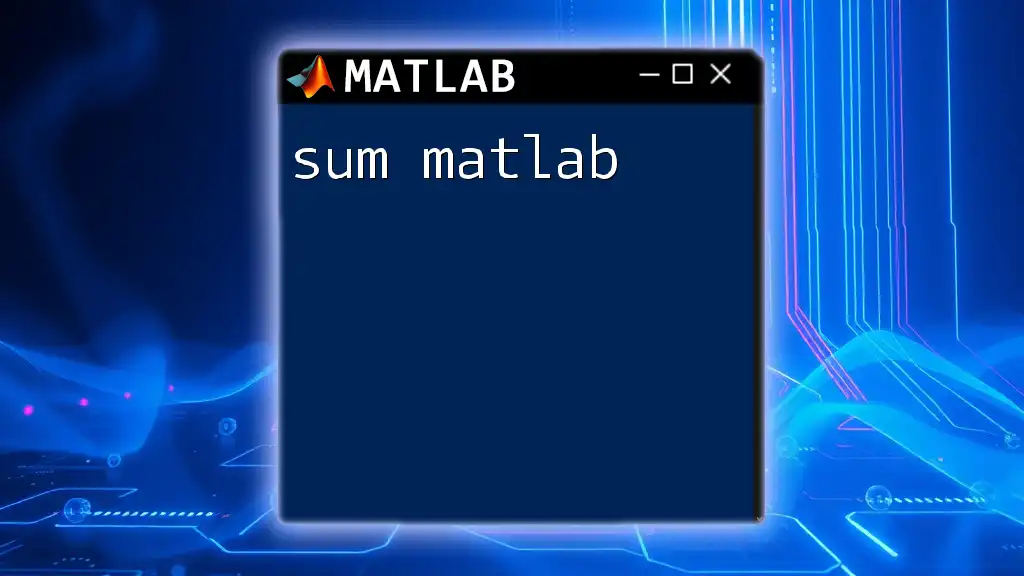
Resources for Learning MATLAB at UMich
Online Tutorials and Workshops
UMich offers various workshops throughout the academic year, tailored to maximize your understanding of MATLAB. Additionally, consider exploring platforms like Coursera and edX for structured online courses.
MATLAB Documentation and User Community
The official MATLAB documentation is a treasure trove of information. Utilize it to find detailed explanations of functions and commands. Engaging with the MATLAB community through forums and user groups can also provide valuable insights and peer support.
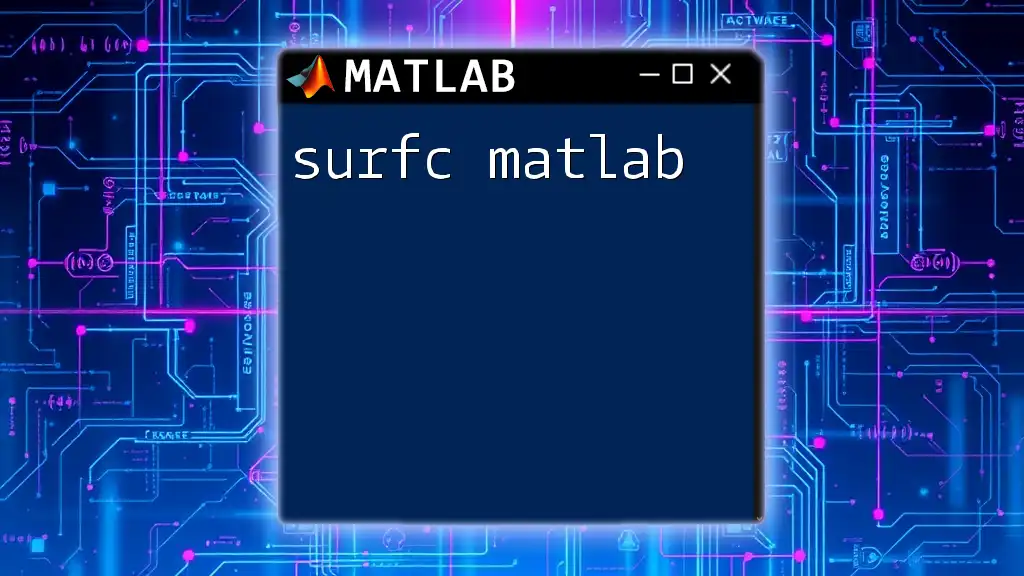
Conclusion
Mastering UMich MATLAB is not just beneficial for academic success; it can serve as a substantial asset in your professional toolkit. With its extensive applications and personable support resources available at UMich, diving into MATLAB will surely amplify your skill set and broaden your horizons.
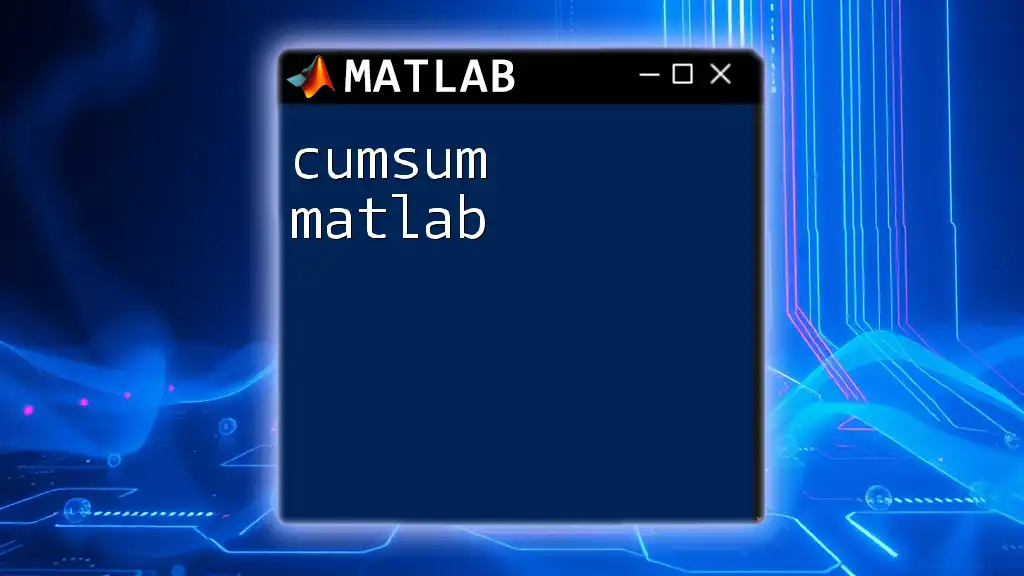
FAQs About UMich MATLAB
-
Is MATLAB free for UMich students?
Yes, students have access to MATLAB licenses provided by the university, making it convenient to download and use the software without additional costs. -
What if I encounter an error while using MATLAB?
MATLAB’s integrated help feature is a reliable source for troubleshooting. Additionally, leverage forums and discussion groups for community support. -
How can I tailor my MATLAB learning experience?
Explore various resources, participate in workshops, and practice coding through personal projects to discover what best suits your learning style.
With these tools and resources at your disposal, you are well on your way to becoming proficient in UMich MATLAB!