The `imwrite` function in MATLAB is used to save image data to a file in various formats, such as JPEG, PNG, or TIFF.
Here's a code snippet demonstrating how to use `imwrite` to save an image:
% Create a sample image
img = rand(100, 100, 3); % Generate a random RGB image
% Save the image as a PNG file
imwrite(img, 'sample_image.png');
Understanding Image Formats
When working with imwrite matlab, it's essential to understand the various image formats that it supports. Some of the most common formats include:
- JPEG: Ideal for photographs and images where a balance between quality and file size is necessary.
- PNG: Supports transparency, making it great for web graphics.
- TIFF: Useful for high-quality images, often used in printing and professional photography.
- BMP: A basic format that provides simple storage of pixel data, usually resulting in larger files.
When to Use Each Format
Choosing the correct image format is crucial for achieving desired results. Here are some considerations:
- Quality: JPEG uses lossy compression, which can degrade quality. PNG and TIFF preserve image quality but can result in larger file sizes.
- Transparency: If you need an image with transparent background, prefer PNG.
- File Size: For web images, smaller sizes are preferable; JPEG is often best for this.
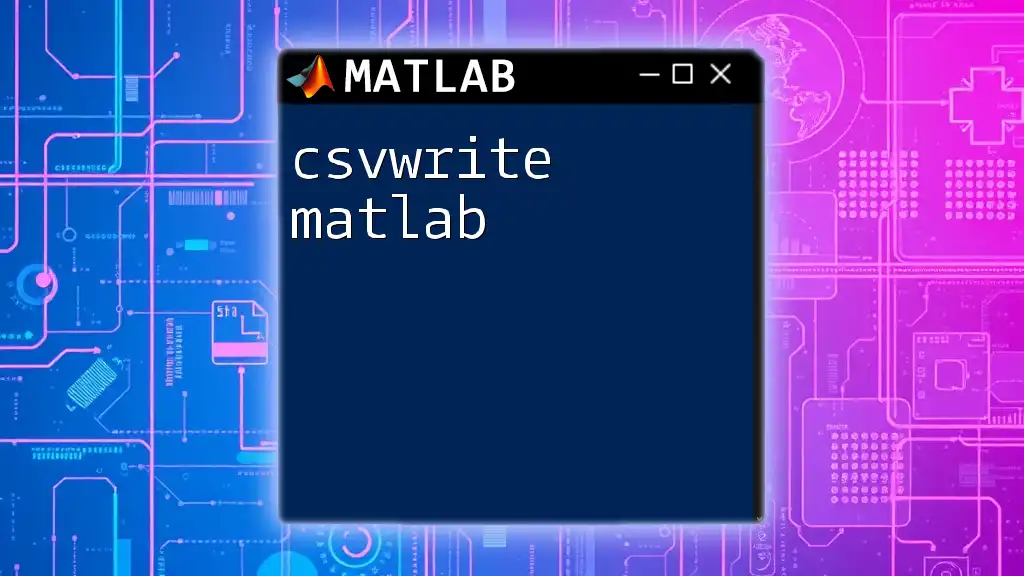
Syntax and Parameters of `imwrite`
The basic syntax for imwrite matlab is straightforward:
imwrite(A, filename)
Key Parameters
- A: This represents the image data as an array. It can be in different formats such as grayscale or RGB.
- filename: The output file name in string format (including file extension, e.g., `.png`, `.jpg`).
- Additional Options: You can control various attributes when saving images. For example, you might want to set the quality in a JPEG file with:
imwrite(A, filename, 'Quality', 90)
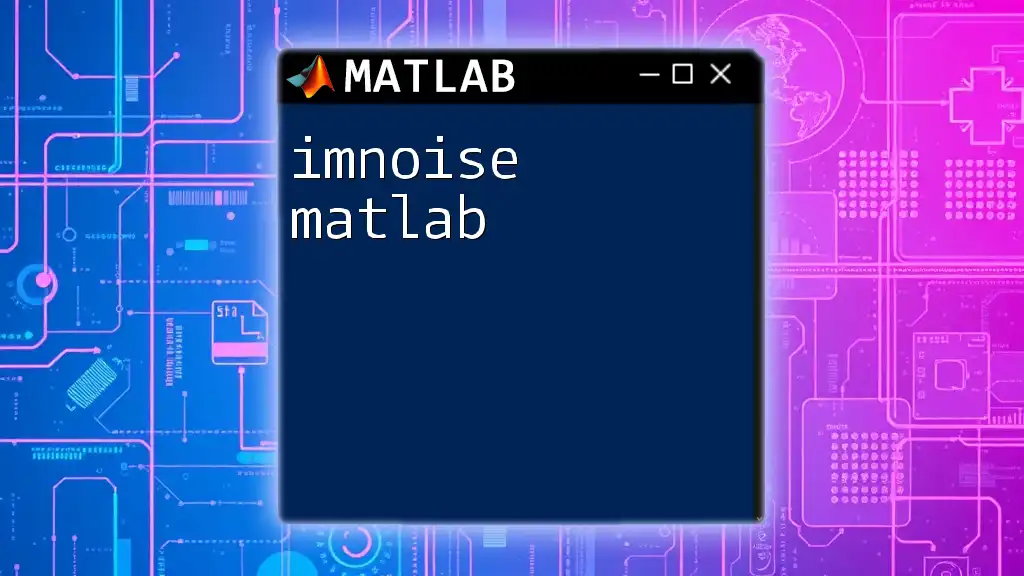
How to Use `imwrite` in Practice
Step-by-Step Instructions
When using imwrite matlab, the first step is to prepare your image data. You can generate sample images using MATLAB functions. For instance:
A = im2uint8(peppers(200));
Now that you have your image data, it’s time to write it to a file. Below is an example of saving this simple image:
imwrite(A, 'peppers.png');
Handling Color Images
If you're working with color images, particularly RGB, imwrite matlab aids in saving them efficiently. Let’s create and save an RGB image:
rgbImage = cat(3, A, A/2, A/3); % Combine layers to create an RGB image
imwrite(rgbImage, 'rgbImage.png');
Handling Grayscale Images
When converting and saving grayscale images, imwrite also proves valuable. You can convert an RGB image to grayscale and save it as follows:
grayImage = rgb2gray(rgbImage);
imwrite(grayImage, 'grayImage.png');
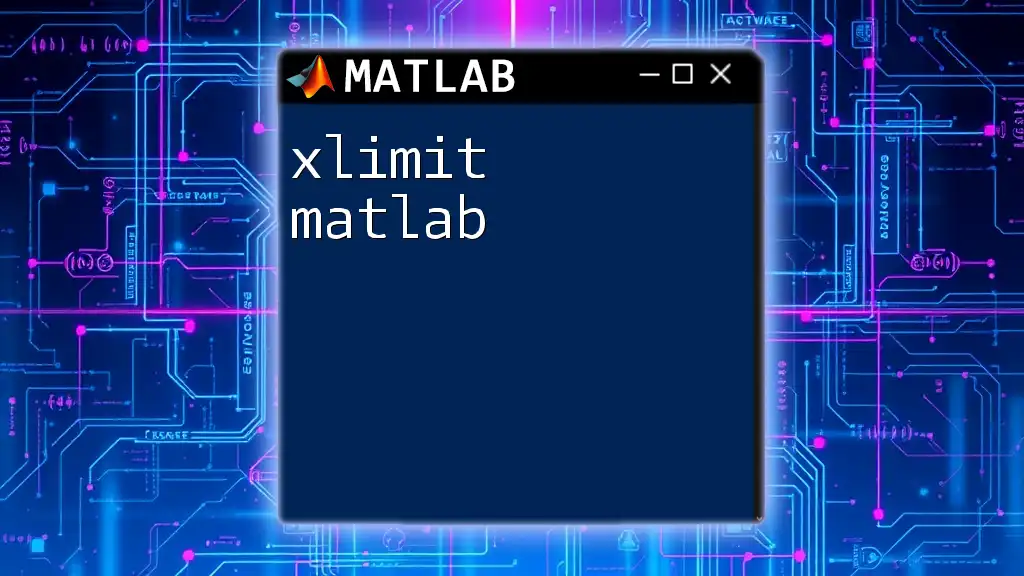
Advanced `imwrite` Features
Writing with Specific Options
You can also control several properties while writing images. For example, if you wish to set the compression and quality of a JPEG image, use:
imwrite(A, 'compressedImage.jpg', 'Quality', 75);
This command illustrates how you can achieve a balance between file size and image fidelity.
Using Transparency with PNG Files
Creating images with transparency is another powerful feature of imwrite matlab. Here’s how you can add an alpha channel and save a PNG file:
alpha = ones(size(A, 1), size(A, 2)); % Creates a full-opacity layer
imwrite(A, 'transparentImage.png', 'Alpha', alpha);
This capability is especially useful when preparing images for web use or graphical applications.
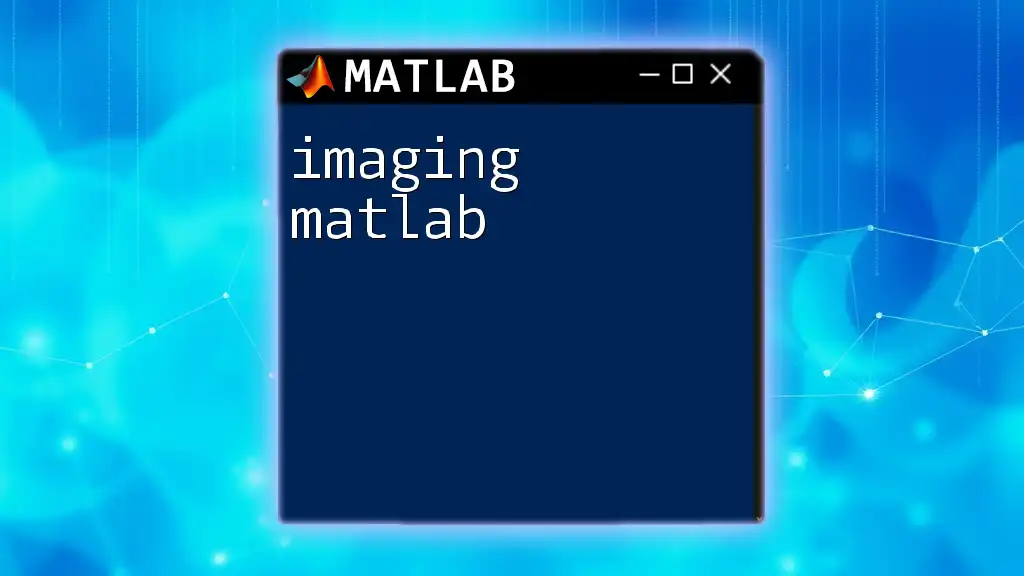
Common Errors and Troubleshooting
When using imwrite in MATLAB, you may encounter several common errors. Issues like permission errors when writing files can arise if the specified path is not writable. Always make sure to check the path specified in your filename.
Another frequent mistake is forgetting to include the file extension. Ensure that your filename ends with a valid image format (e.g., `.png`, `.jpg`).
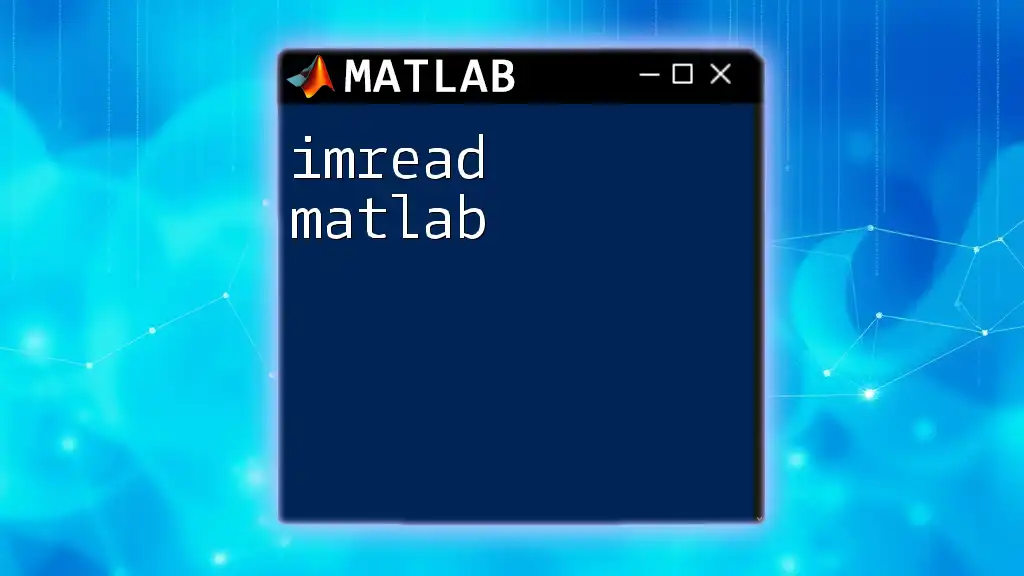
Best Practices for Using `imwrite`
To maximize the effectiveness of imwrite matlab, observe the following best practices:
- Format Selection: Carefully select the image format based on your requirements, whether it’s for web use, printing, or archiving.
- Organizing Output Files: Maintain a structured directory for saving images to streamline retrieval and avoid clutter.
- Efficient Image Data Management: Use appropriate data types for image arrays to optimize performance and memory usage.
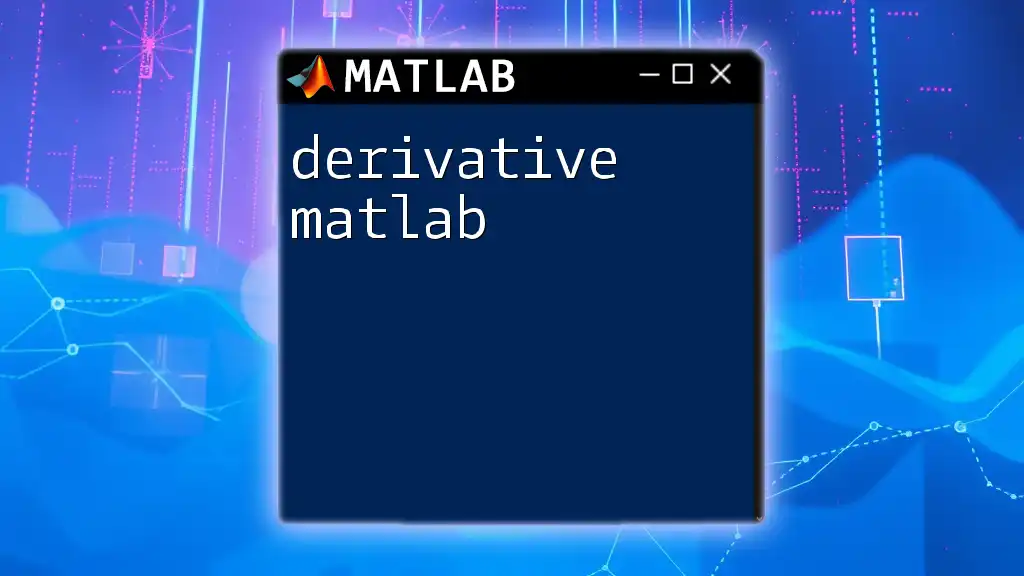
Conclusion
Mastering imwrite matlab opens up a world of possibilities for image processing and manipulation in MATLAB. As you explore its capabilities, practice the examples and variations discussed in this guide to deepen your understanding. Utilize MATLAB's extensive documentation and community resources to further enhance your skills and knowledge in image writing.