The `pwelch` function in MATLAB computes the Power Spectral Density (PSD) of a signal using Welch's method, which averages periodograms to provide a more reliable estimate.
% Example: Compute and plot the Power Spectral Density using pwelch
x = randn(1,1000); % Generate a random signal
[pxx,f] = pwelch(x,[],[],[],fs); % Calculate PSD
plot(f,10*log10(pxx)) % Plot in dB
title('Power Spectral Density using pwelch')
xlabel('Frequency (Hz)')
ylabel('Power/Frequency (dB/Hz)')
What is Power Spectral Density (PSD)?
Power Spectral Density (PSD) is a measure used in signal processing to represent the distribution of power into frequency components of a signal. It provides insight into how the power of a signal is distributed across different frequencies. Understanding PSD is crucial for applications such as telecommunications, audio engineering, vibration analysis, and biomedical signal processing.
PSD estimation helps in identifying dominant frequencies present in a signal, which can be vital for diagnosing issues or optimizing systems based on frequency response.
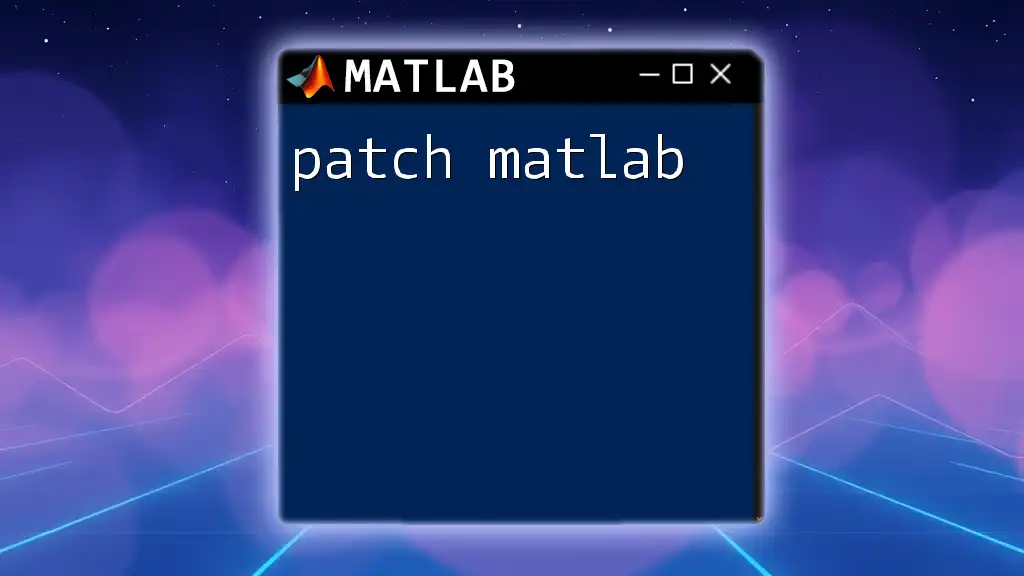
Understanding the `pwelch` Function in MATLAB
Overview of `pwelch`
The `pwelch` function in MATLAB is designed to compute the Power Spectral Density estimate using Welch's method. Welch's method improves upon the traditional periodogram method by averaging the results over overlapping segments of the signal, providing a smoother and more reliable estimate of the PSD.
Syntax of `pwelch`
The basic syntax for the `pwelch` function is as follows:
[Pxx, F] = pwelch(X, window, noverlap, nfft, fs)
Here, `Pxx` represents the PSD estimates, and `F` represents the frequency values corresponding to those estimates.
Input Parameters
X: The Signal
The input signal `X` can be either a vector or a matrix. In the case of a matrix, each column is treated as a separate signal channel.
For example, to create a simple noisy sine wave, you can use:
t = 0:0.001:1; % time vector
x = sin(2*pi*50*t) + randn(size(t)); % signal with noise
window: Window Length
Choosing the correct window length is vital for accurate PSD estimation. The window function reduces spectral leakage. MATLAB offers various options, and you can employ a Hamming or Hanning window for better results. In the `pwelch` function, you can specify the window as follows:
window = hamming(256);
noverlap: Overlap Between Windows
The overlap parameter `noverlap` specifies how many samples are shared between adjacent windows. Increasing overlap can improve the estimate but may require more computational resources and affect resolution. A common value for overlap is half the window length.
nfft: Number of Fourier Coefficients
The `nfft` parameter specifies the number of points used to calculate the Fast Fourier Transform (FFT). Choosing an appropriate value is essential for achieving an adequate frequency resolution. A common practice is to use a power of two, such as 512 or 1024, for computational efficiency.
fs: Sampling Frequency
The sampling frequency `fs` represents how frequently the signal is sampled. It is crucial for interpreting the frequency axis correctly.
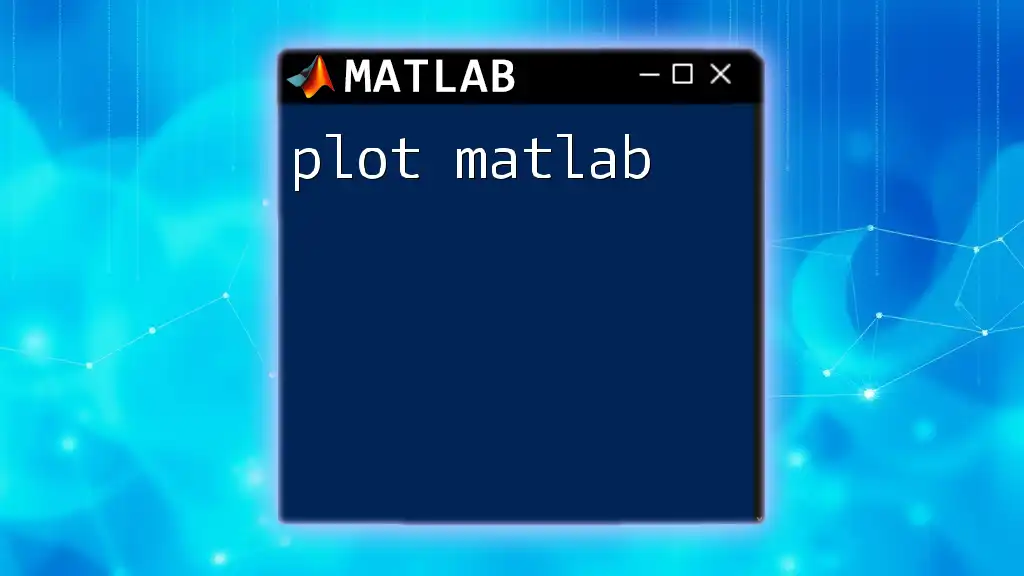
Step-by-Step Example Using `pwelch`
Generating a Signal
To illustrate how to use `pwelch`, we will first generate a signal composed of two sine waves and noise:
fs = 1000; % Sampling Frequency
t = 0:1/fs:1-1/fs; % Time vector
x = cos(2*pi*100*t) + cos(2*pi*200*t) + randn(size(t)); % Noisy signal
Using `pwelch` for PSD Estimation
Next, we apply the `pwelch` function to estimate the Power Spectral Density:
window = hamming(256);
noverlap = 128;
nfft = 512;
[Pxx, F] = pwelch(x, window, noverlap, nfft, fs);
In this example, the `pwelch` command outputs `Pxx`, which contains the PSD estimates, and `F`, which provides the frequency values.
Visualizing the Output
To visualize the estimated PSD, we can create a plot:
figure;
plot(F, 10*log10(Pxx));
title('Power Spectral Density Estimate using pwelch');
xlabel('Frequency (Hz)');
ylabel('Power/Frequency (dB/Hz)');
grid on;
This code snippet generates a graph illustrating how the power of the signal is distributed across different frequencies in dB/Hz.
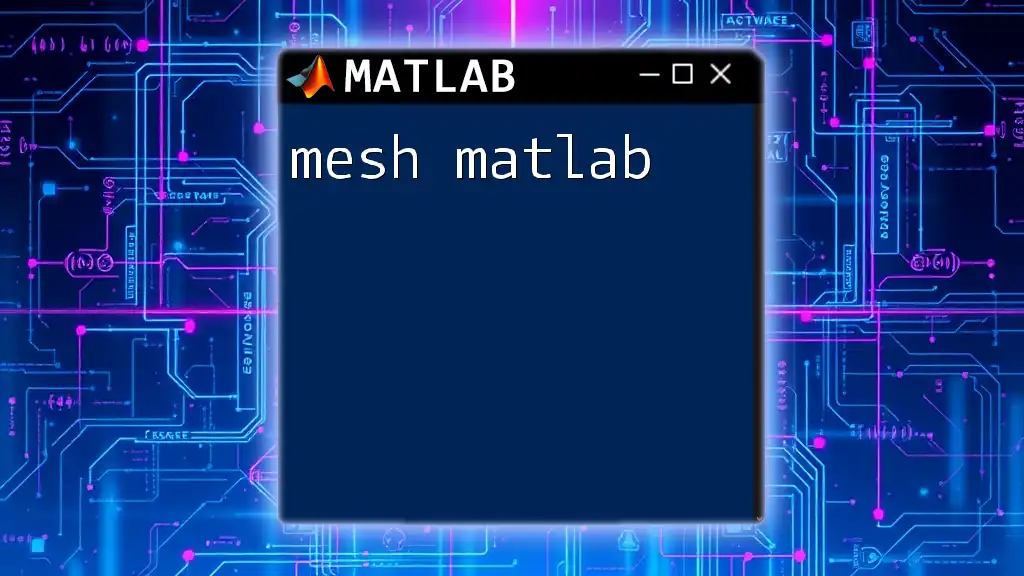
Advanced Features of `pwelch`
Customizing Window Functions
In addition to the Hamming window, MATLAB supports various window functions such as Hanning, Blackman, and others. You can create a window using a specific length and apply custom coefficients to tailor it to your needs.
Multiple Signals
If you have multiple signals, you can utilize `pwelch` to analyze each one separately while using tailored parameters to enhance the analysis. For example:
[Pxx1, F1] = pwelch(signal1, window, noverlap, nfft, fs);
[Pxx2, F2] = pwelch(signal2, window, noverlap, nfft, fs);
This can help you compare the spectral content of different signals side by side.
Scaling and Units
It’s crucial to be aware of how the output of `pwelch` is scaled, especially when converting power to other units such as voltage.
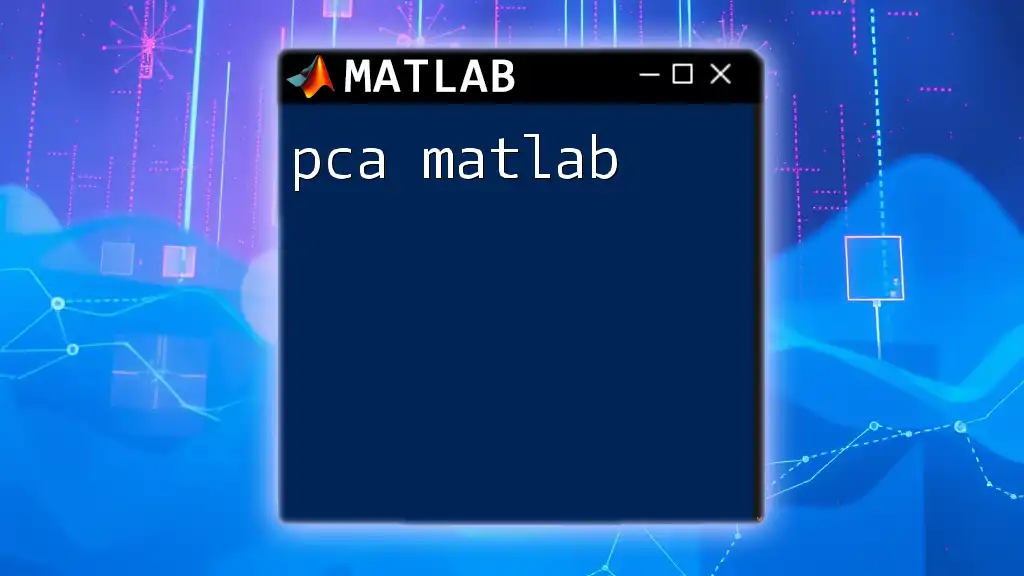
Common Issues and Troubleshooting
Signal Length and Data Input
One common issue encountered is related to the length of the input signal. Ensure that the signal is sufficiently long compared to the window length, overlap, and FFT size. Short signals can lead to unreliable PSD estimates.
Interpretation of Results
Interpreting the results from `pwelch` can be challenging. Familiarize yourself with the characteristics of your signals and examine the PSD plot carefully to discern between real signal components and noise.
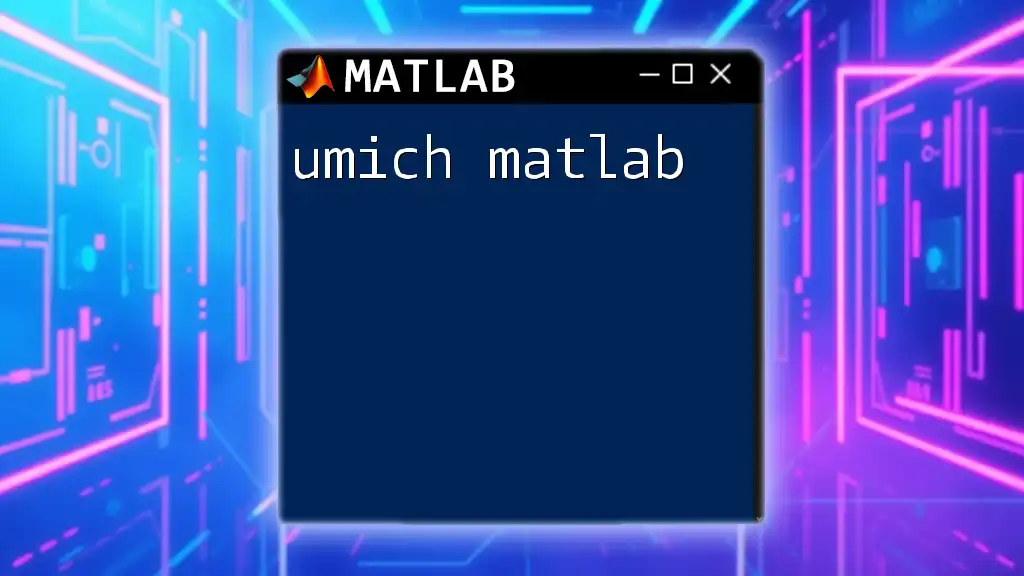
Conclusion
The `pwelch` function in MATLAB is a powerful tool for estimating Power Spectral Density, making it ideal for analyzing signals in various applications. By following the guidelines and examples provided, you can harness the capabilities of `pwelch` to gain valuable insights into the frequency domain characteristics of your signals.
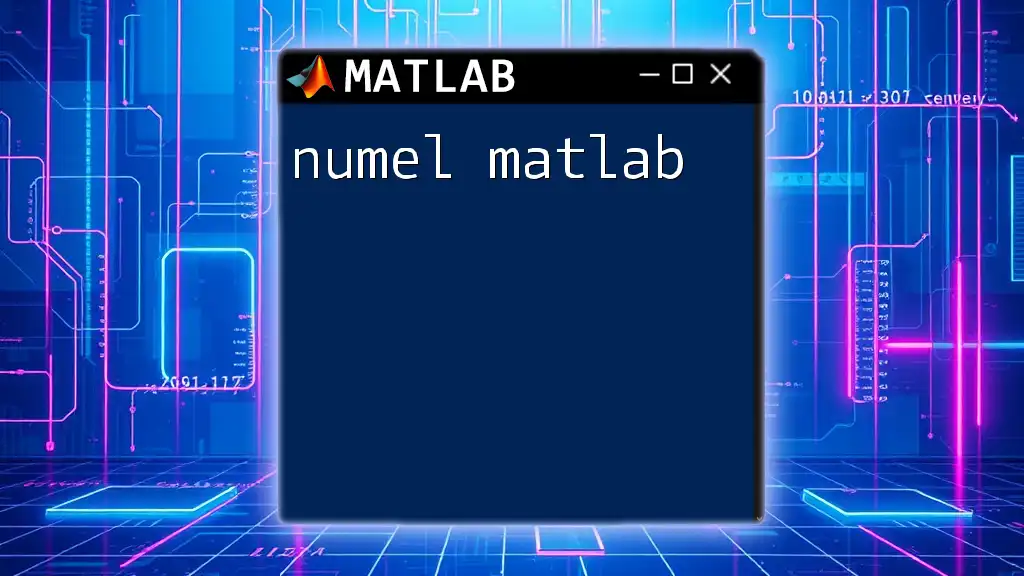
Additional Resources
For further exploration of `pwelch` and related functions, consider reviewing the official MATLAB documentation and engaging with tutorials that provide deeper insights into spectral analysis techniques.
FAQs
-
What are some common applications of `pwelch`? `pwelch` is widely used in audio signal processing, vibration analysis, and telecommunications to analyze the frequency content of various signals.
-
How do I know what parameters to use? Begin with standard values for `window`, `noverlap`, `nfft`, and adjust based on your specific signal characteristics and desired resolution. Experimentation often leads to the best results.
-
Can `pwelch` be used on non-stationary signals? Yes, while `pwelch` is designed for stationary signals, you can apply it to non-stationary signals by changing parameters and analyzing segments of the data separately to observe variations over time.