The `strrep` function in MATLAB replaces all occurrences of a specified substring within a string with a new substring.
Here’s a code snippet demonstrating its usage:
originalString = 'Hello World';
modifiedString = strrep(originalString, 'World', 'MATLAB');
disp(modifiedString); % Output: 'Hello MATLAB'
What is `strrep`?
The `strrep` function is one of MATLAB's built-in functions, specifically designed for string manipulation. It plays a crucial role in replacing occurrences of a specified substring within a given string. This function is essential for cleaning data, formatting outputs, and various other tasks where string modification is required.
Key Characteristics
One of the key characteristics of `strrep` is its case sensitivity. The function treats uppercase and lowercase letters differently, meaning `"MATLAB"` and `"matlab"` would be considered distinct when using `strrep`. Also, it’s important to note that `strrep` replaces all occurrences of the specified `oldSubstring`, making it a powerful tool for comprehensive string modifications.
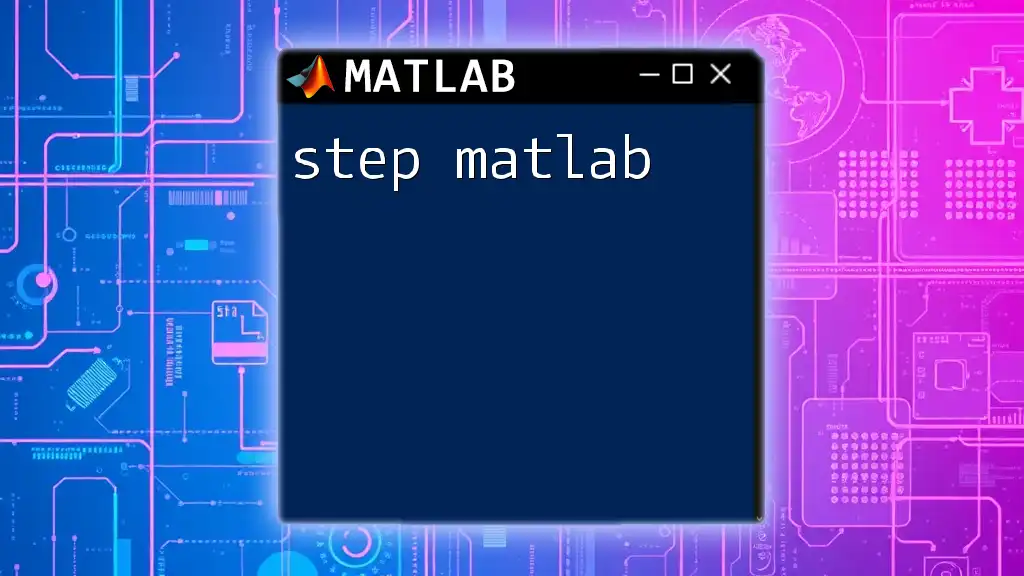
How to Use `strrep`
Basic Syntax
The syntax of the `strrep` function can be expressed as follows:
newString = strrep(originalString, oldSubstring, newSubstring);
Where:
- `originalString` is the string you want to modify.
- `oldSubstring` is the substring you wish to replace.
- `newSubstring` is the substring that will replace `oldSubstring`.
Example 1: Basic String Replacement
To illustrate how `strrep` works, consider the following example:
originalString = 'Hello world!';
newString = strrep(originalString, 'world', 'MATLAB');
disp(newString);
In this case, the output will be:
Hello MATLAB!
Here, the original string `"Hello world!"` has been modified to `"Hello MATLAB!"`, showcasing the straightforward utility of the function.
Example 2: Case Sensitivity
Understanding case sensitivity is vital when using `strrep`. For instance, observe the following code:
originalString = 'Hello World!';
newString = strrep(originalString, 'world', 'MATLAB');
disp(newString);
The output will remain:
Hello World!
In this example, the substring `"world"` in lowercase does not match `"World"` in the original string. Therefore, no replacement occurs, emphasizing the need for attention to detail regarding letter casing in string functions.
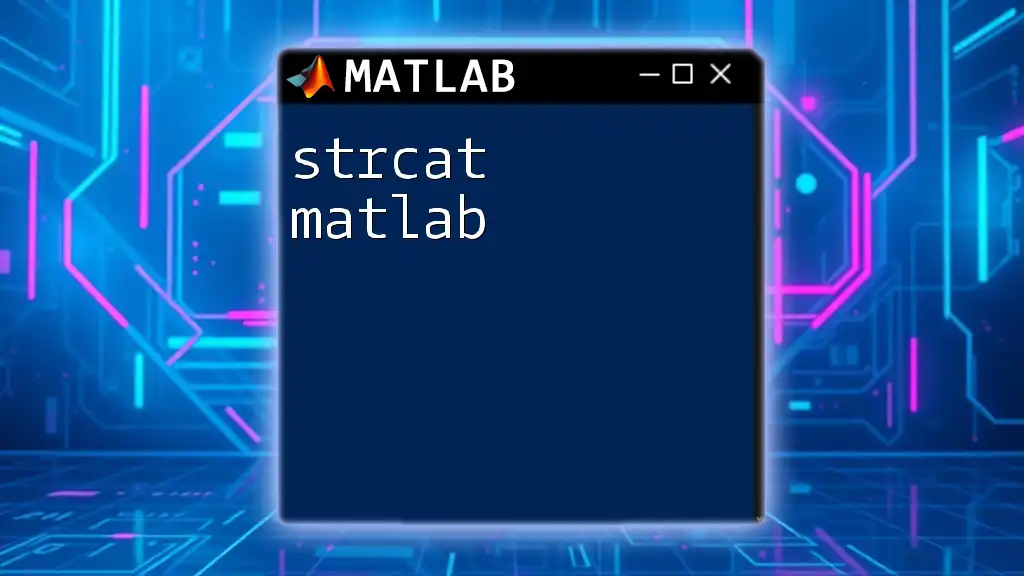
Advanced Uses of `strrep`
Handling Multiple Substrings
One common need in string manipulation is the requirement to replace multiple substrings seamlessly. This can be achieved using a loop to iterate through pairs of substrings. Here’s how it can be done:
originalString = 'The cat sat on the mat.';
replacements = {'cat', 'dog'; 'mat', 'rug'};
for i = 1:size(replacements, 1)
originalString = strrep(originalString, replacements{i, 1}, replacements{i, 2});
end
disp(originalString);
The final output will be:
The dog sat on the rug.
In this code, both `"cat"` and `"mat"` have been replaced with `"dog"` and `"rug"` respectively as specified in the `replacements` matrix.
Working with Cell Arrays of Strings
MATLAB allows for efficient handling of cell arrays, making it possible to apply `strrep` over a collection of strings. Here’s how you can replace substrings in a cell array:
stringArray = {'Hello world!', 'MATLAB is great!'};
newStringArray = cellfun(@(x) strrep(x, 'world', 'MATLAB'), stringArray, 'UniformOutput', false);
disp(newStringArray);
The output will be:
'Hello MATLAB!' 'MATLAB is great!'
By using the `cellfun` function, we apply `strrep` to each string in `stringArray`, demonstrating both versatility and efficiency when manipulating multiple strings at once.
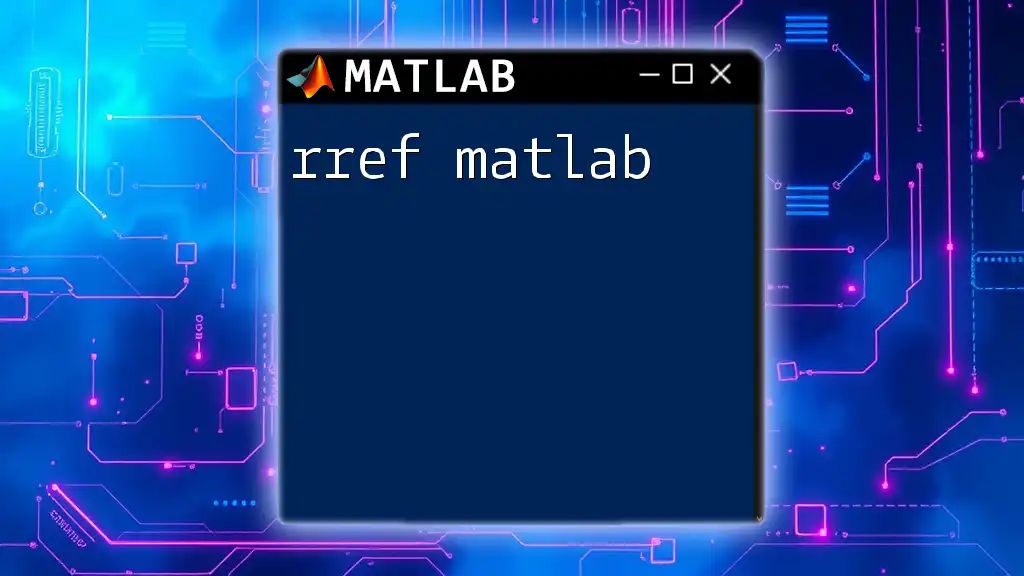
Common Errors and Troubleshooting
Invalid Inputs
Users may encounter some common errors when using `strrep`. These include:
- Providing non-string inputs that lead to unexpected behavior.
- Failing to specify the correct number of arguments, resulting in runtime errors.
To debug these issues, always ensure that your input strings are correctly formatted and that all parameters adhere to the expected data types.
Performance Tips
When working with large datasets or very long strings, the performance of `strrep` may become a consideration. The length of the string and the complexity of the substring can affect processing times. To optimize performance:
- Minimize repetitive calls to `strrep` where possible by batching replacements.
- Consider alternative methods for very large or numerous string modifications.
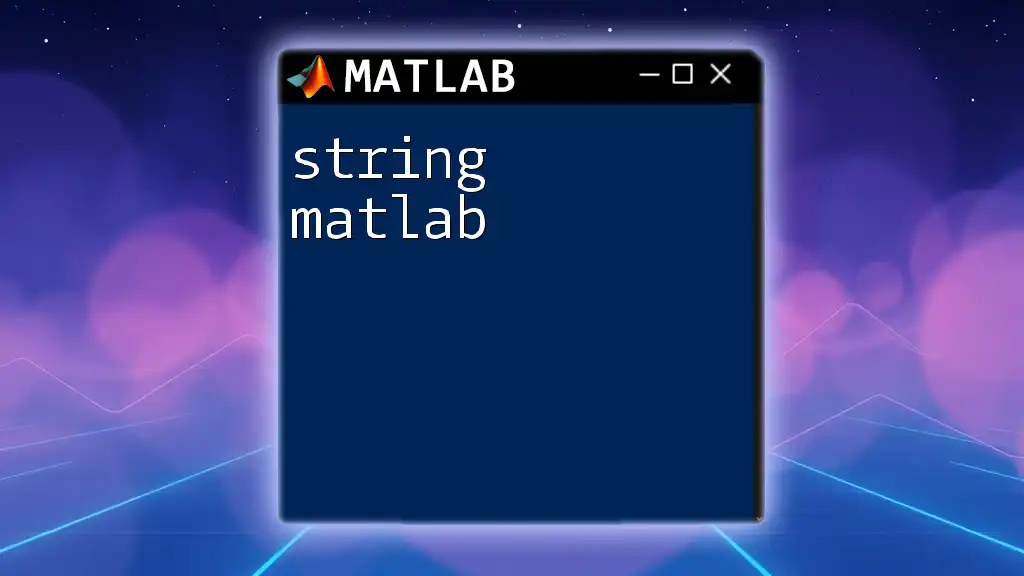
Conclusion
In summary, the `strrep` function in MATLAB is an invaluable tool for string manipulation, essential for tasks that involve searching and replacing substrings within strings. Its ability to handle case sensitivity and replace multiple occurrences makes it versatile for many applications. Practicing with various examples can enhance your understanding and proficiency with `strrep`, paving the way for more advanced string operations.
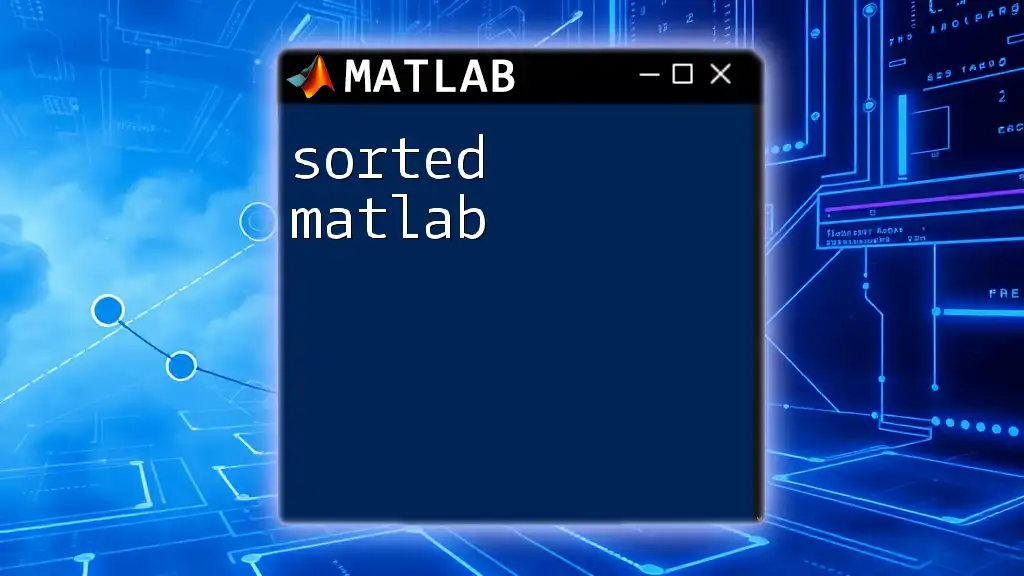
Frequently Asked Questions (FAQs)
What is the difference between `strrep` and `strreplace`?
While `strrep` is specifically for replacing substrings, `strreplace` (if available) may employ different semantic rules or functionality, depending on context. Always check MATLAB documentation for the most accurate details.
Can I use `strrep` for numerical replacements?
`strrep` is primarily designed for string manipulation. If you need to replace numerical values represented as strings, ensure they are formatted appropriately and converted where necessary. Pay attention to the types being used in your MATLAB environment!
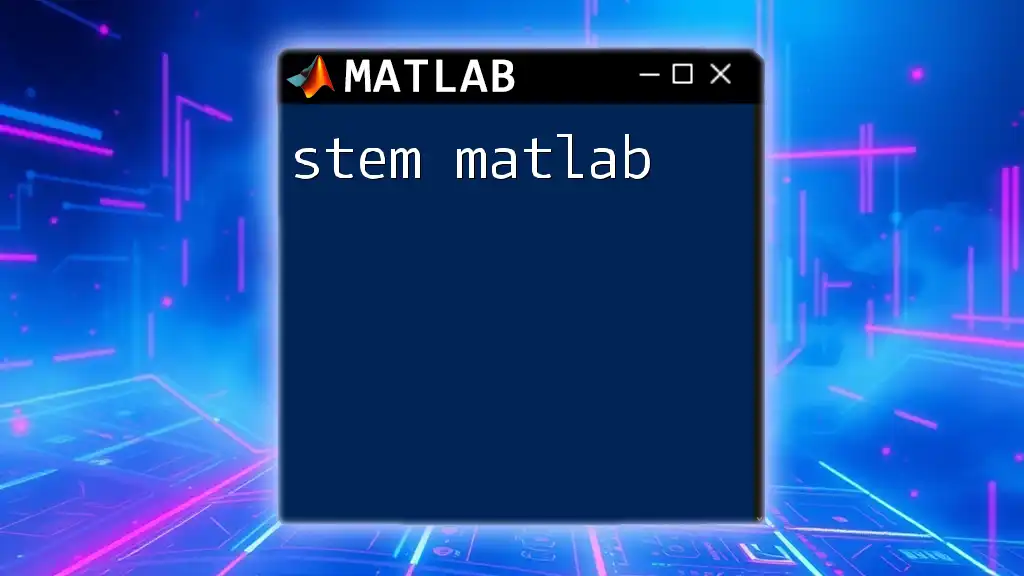
Call to Action
We encourage you to share your own examples or questions regarding string manipulation using `strrep` in the comments section. Explore our other articles for further resources that can help you master MATLAB commands!