`vertcat` in MATLAB is a function used to concatenate matrices or arrays vertically along the first dimension, stacking them on top of one another.
Here's a quick example:
A = [1, 2; 3, 4];
B = [5, 6];
C = vertcat(A, B); % Result will be a 3x2 matrix
Understanding `vertcat`
What is `vertcat`?
The `vertcat` function in MATLAB is designed for vertical concatenation of arrays. It allows you to stack arrays on top of one another, creating a new array. For instance, when you have multiple datasets, and you need to combine them into a single dataset, `vertcat` enables you to achieve this seamlessly.
Importance of `vertcat` in MATLAB
The versatility of `vertcat` makes it an essential function for data manipulation. It can come in handy in various scenarios, including preparing datasets for analysis or combining multiple outputs from different functions.
When comparing it to other concatenation techniques like `horzcat` (horizontal concatenation), `vertcat` serves a specific purpose: combining arrays vertically. Understanding when to utilize `vertcat` versus other methods can significantly enhance your productivity in MATLAB.
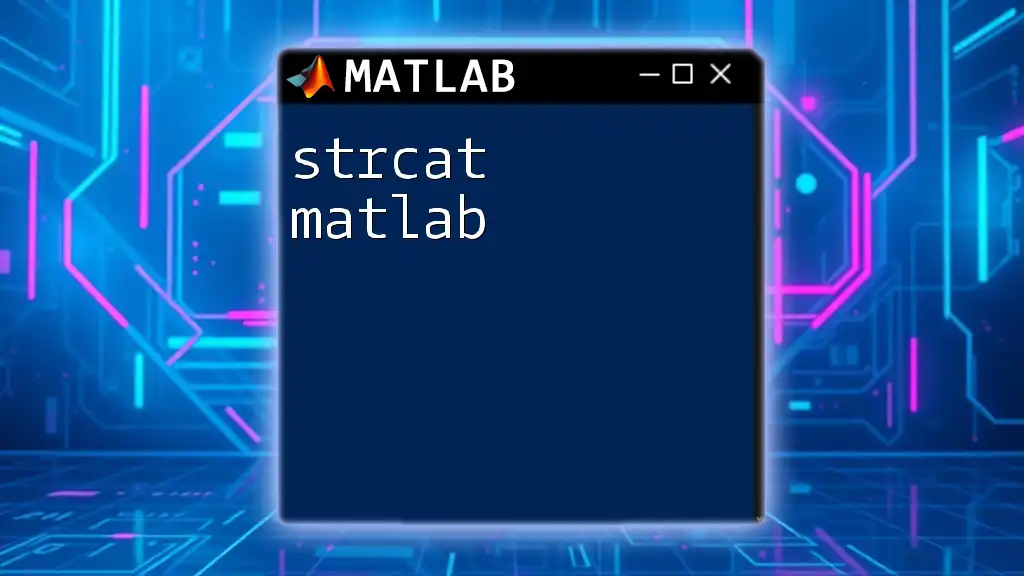
How to Use `vertcat`
Basic Syntax of `vertcat`
The basic syntax of `vertcat` is as follows:
C = vertcat(A1, A2, ..., An)
Here, `A1`, `A2`, ..., `An` are the arrays you want to concatenate vertically. The output, `C`, is a new array formed by stacking `A1`, `A2`, and so on.
Concatenating Different Data Types
Numeric Arrays
Here’s a straightforward example of how you can use `vertcat` to concatenate numeric arrays:
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
C = vertcat(A, B);
In this example, `C` would become:
C =
1 2
3 4
5 6
7 8
This clearly illustrates how two 2x2 matrices can be combined into a single 4x2 matrix.
Cell Arrays
`vertcat` can also be used with cell arrays. For instance:
C1 = {'apple', 'banana'};
C2 = {'cherry', 'date'};
C_combined = vertcat(C1, C2);
In this scenario, `C_combined` would look like:
C_combined =
'apple'
'banana'
'cherry'
'date'
This shows how `vertcat` consolidates different cell arrays into a single column.
Structures
You can utilize `vertcat` with structures, too, but be mindful that the fields must align. Here’s an example:
S1 = struct('field1', {1, 2}, 'field2', {3, 4});
S2 = struct('field1', {5, 6}, 'field2', {7, 8});
S_combined = vertcat(S1, S2);
`S_combined` now contains:
S_combined =
1 3
2 4
5 7
6 8
This functionality allows you to combine results from different observations into a single structured dataset.
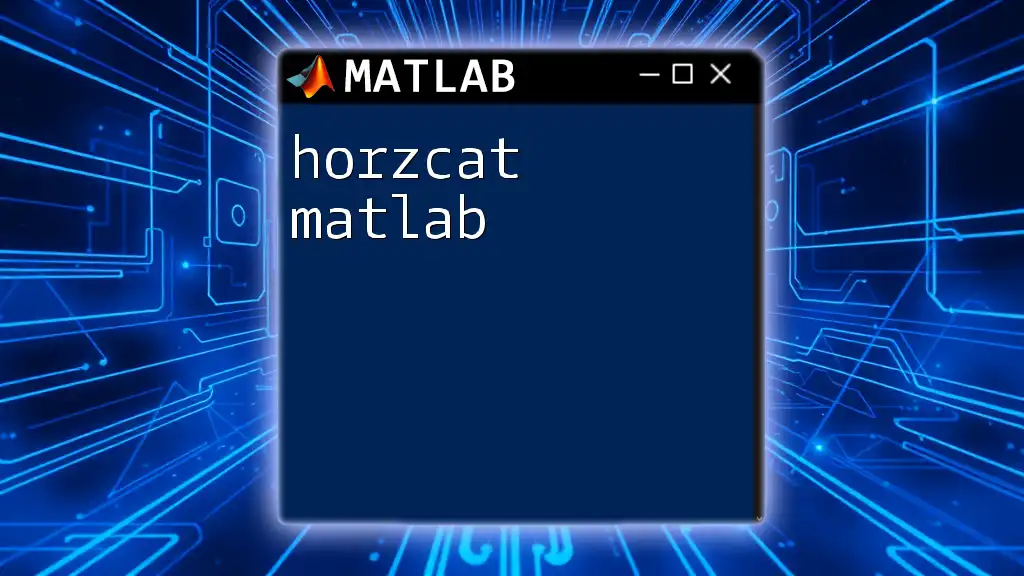
Important Considerations
Dimensionality
When using `vertcat`, one critical aspect is the dimensionality of the arrays. To successfully concatenate arrays, their number of columns must match. If they don’t, MATLAB will throw an error. For example:
A = [1, 2; 3, 4]; % 2x2 matrix
B = [5; 6]; % 2x1 matrix
C = vertcat(A, B); % This will throw an error
In this case, trying to concatenate would fail since `A` has 2 columns while `B` has only 1.
Data Type Consistency
Consistency in data types is vital. If you attempt to concatenate arrays of differing types, MATLAB will generate an error. An example would be trying to combine an array of numbers with an array of strings. Ensuring type consistency before concatenation will help avoid unnecessary troubleshooting.
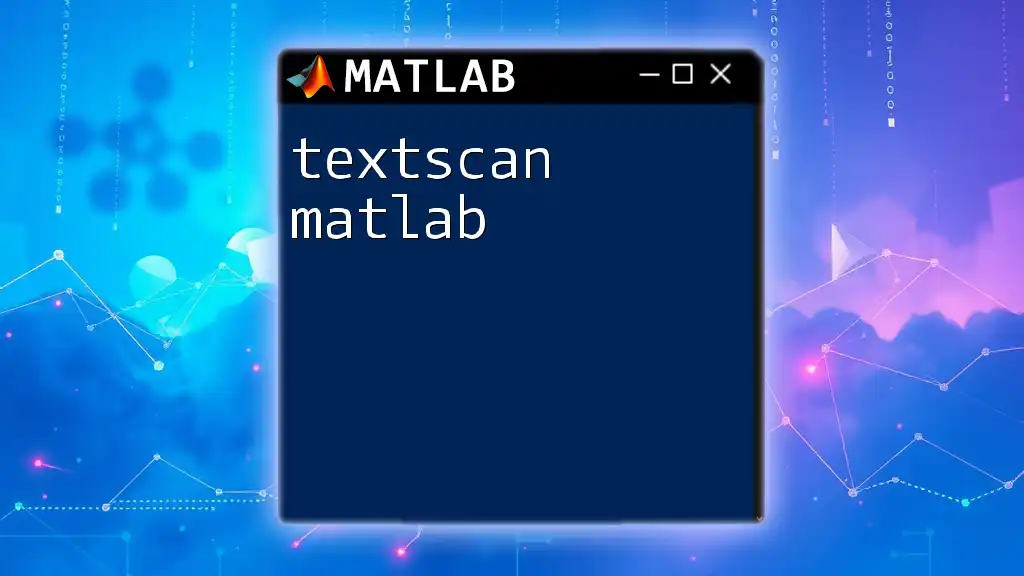
Practical Applications of `vertcat`
Data Preparation in Machine Learning
In machine learning, datasets often need to be prepared for training algorithms. When dealing with multiple feature sets, you can use `vertcat` to stack these datasets, ensuring they fit into the model properly.
For instance, if you have datasets representing different classes or groups, `vertcat` allows you to create a comprehensive dataset readily available for model training.
Merging Results from Different Simulations
When conducting simulations, you might generate multiple output arrays. Using `vertcat` can consolidate these outputs into one cohesive structure. This is particularly useful when comparing results or compiling reports.
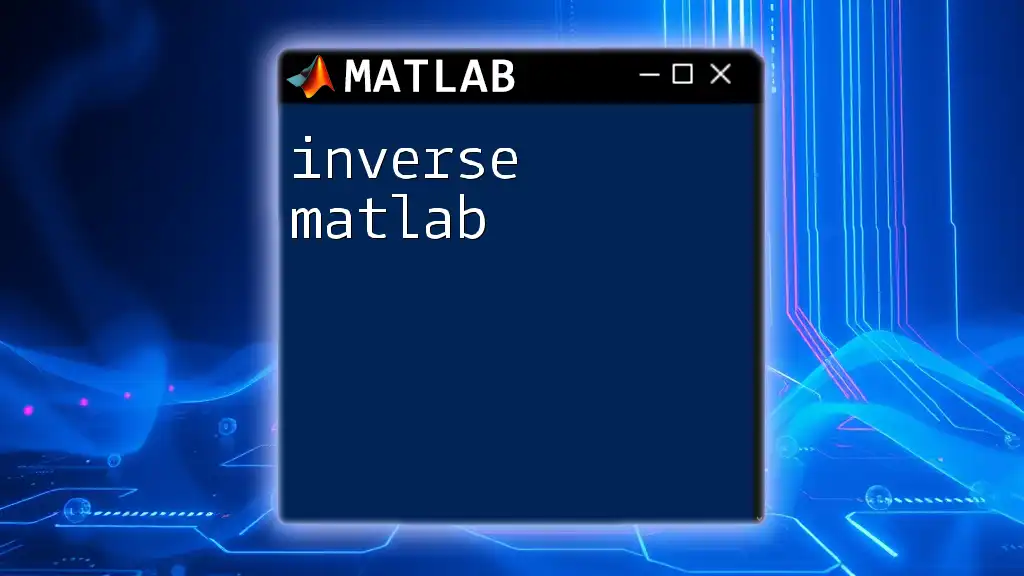
Tips and Best Practices
Efficient Concatenation Techniques
When working with dynamic arrays, consider using cell arrays for concatenation tasks. If you preallocate the size of your matrix before filling it, MATLAB performance will improve, especially with larger datasets.
Common Pitfalls
Be aware of mistakes that can occur frequently with `vertcat`, such as mismatched dimensions or incorrect data types. Always double-check your array dimensions and ensure all inputs are of compatible types before proceeding with concatenation.
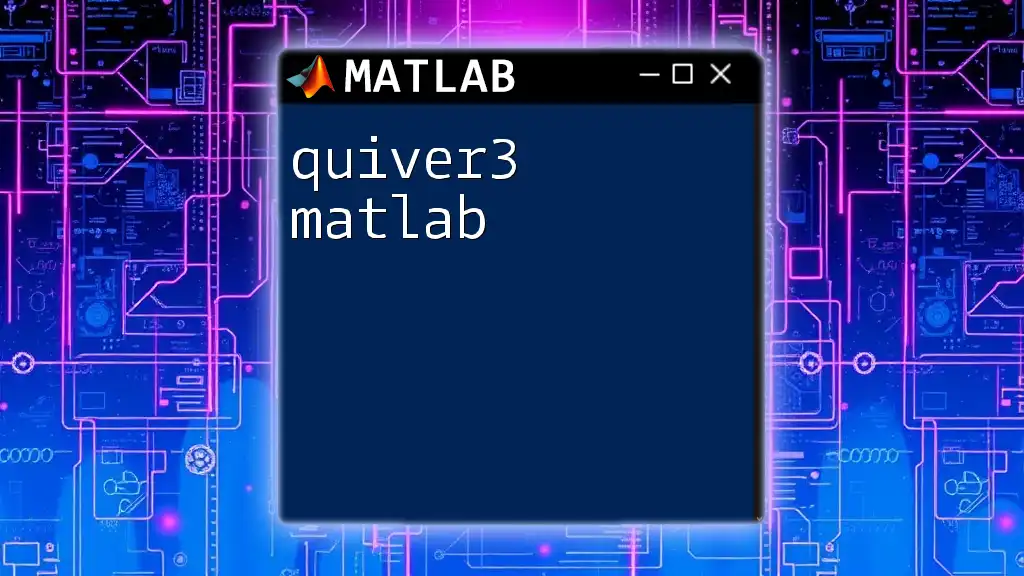
Troubleshooting `vertcat`
Common Error Messages
When a concatenation fails, MATLAB will often provide an error message indicating the nature of the issue. Understanding these common errors can significantly ease the debugging process. For example, if there’s a dimension mismatch, the message will reflect this clearly.
Debugging Tips
If you encounter errors while using `vertcat`, leveraging MATLAB’s debugging tools can be invaluable. Use the command window to inspect array sizes and types before attempting a concatenation, ensuring you're aligned with MATLAB's requirements.
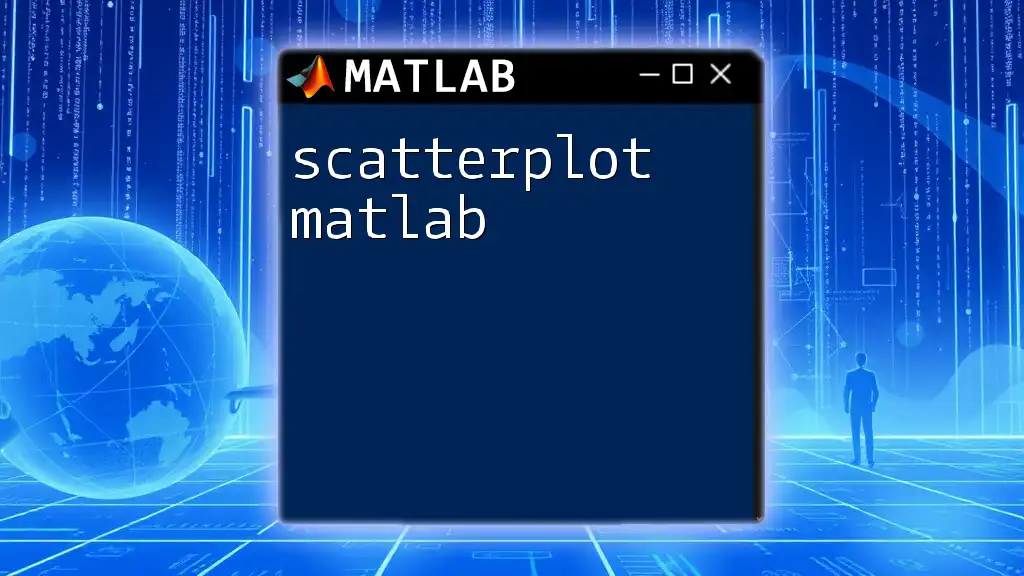
Conclusion
In summary, the `vertcat` function in MATLAB is essential for vertical array concatenation, making it a powerful tool for data analysis and manipulation. By mastering its usage, you can significantly enhance your MATLAB skills and efficiency. For more in-depth usage and exercises, consider exploring MATLAB's documentation and community resources. Engaging with a community can further enhance your learning experience, providing support and knowledge-sharing opportunities as you advance in your MATLAB journey.