In MATLAB, an array is a fundamental data structure that can hold a collection of numbers, symbols, or strings, and can be created using square brackets to define its elements.
Here's a simple example to create a 1x5 array and display its content:
% Creating a 1x5 array
myArray = [1, 2, 3, 4, 5];
% Displaying the array
disp(myArray);
What are Arrays in MATLAB?
Arrays in MATLAB are fundamental structures that allow you to store and manipulate collections of data. They serve as a cornerstone for data analysis and mathematical computations in the MATLAB environment. Arrays can come in various forms, including row arrays, column arrays, and multidimensional arrays. Understanding how to interact with arrays is essential for efficient programming in MATLAB.

Why Use Arrays?
Using arrays facilitates the handling of large sets of data in a compact and efficient manner. They enable a wide variety of mathematical operations, optimizing both speed and complexity when performing calculations. Additionally, arrays provide a uniform approach to data storage, making it easy to iterate over, modify, and perform operations on datasets.
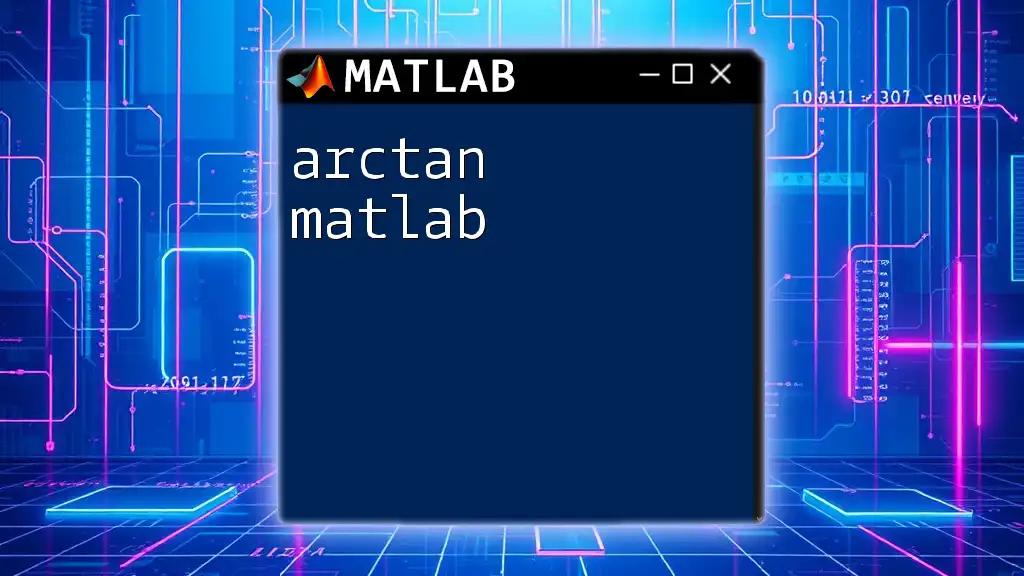
Creating Arrays
MATLAB offers several ways to create arrays, ensuring flexibility depending on your needs.
Using Built-in Functions
MATLAB has convenient functions for generating commonly used types of arrays:
-
Zeros Array: Creates an array filled with zeros.
A = zeros(3, 4); % Creates a 3x4 matrix of zeros
-
Ones Array: Generates an array filled with ones.
B = ones(2, 5); % Creates a 2x5 matrix of ones
-
Random Array: Initializes an array with uniformly distributed random numbers.
C = rand(2, 3); % Creates a 2x3 matrix of random numbers
-
Normally Distributed Random Array: Fills an array with normally distributed random numbers.
D = randn(3, 2); % Creates a 3x2 matrix of normally distributed numbers
Direct Initialization
You can also initialize arrays directly:
E = [1, 2, 3; 4, 5, 6]; % Creates a 2x3 matrix
Using `linspace` and the Colon Operator
-
`linspace`: Generates linearly spaced vectors.
F = linspace(1, 10, 5); % Generates [1, 3.25, 5.5, 7.75, 10]
-
Colon Operator: Provides a straightforward way to create arrays with specific step sizes.
G = 1:2:10; % Generates [1, 3, 5, 7, 9]
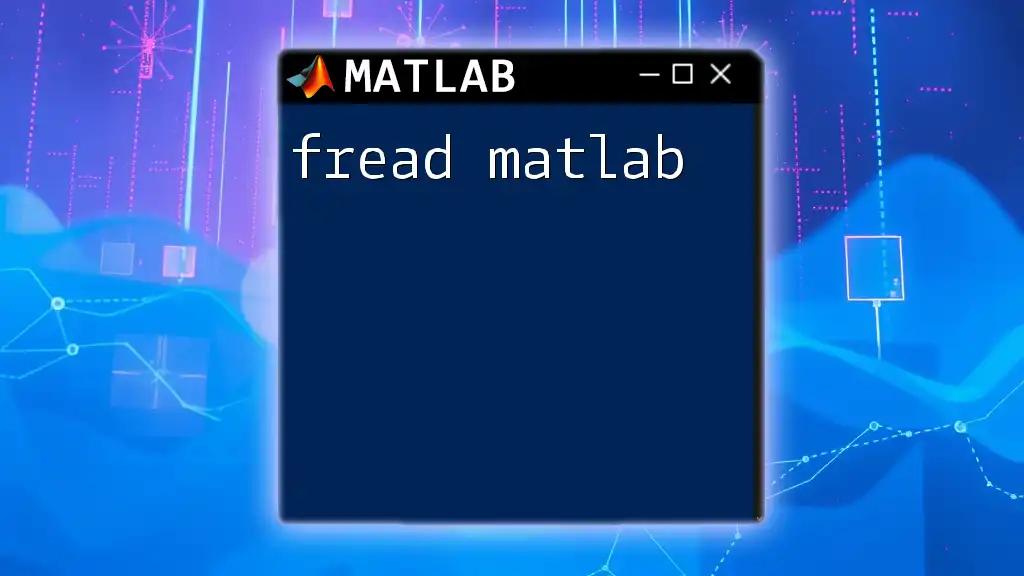
Accessing Elements
Manipulating arrays involves accessing specific elements or groups of elements. MATLAB uses indexing to allow this.
Indexing Techniques
- Accessing Individual Elements:
element = E(1, 2); % Returns the element in the first row and second column
- Column and Row Accessing:
column = E(:, 1); % Returns the entire first column
row = E(2, :); % Returns the entire second row
Modifying Arrays
You can easily change contents or dimensions of arrays.
- Changing Individual Elements:
E(2, 1) = 10; % Updates the element at the second row, first column
- Resizing and Expanding Arrays:
E = [E; 7, 8, 9]; % Appends a new row to the existing array
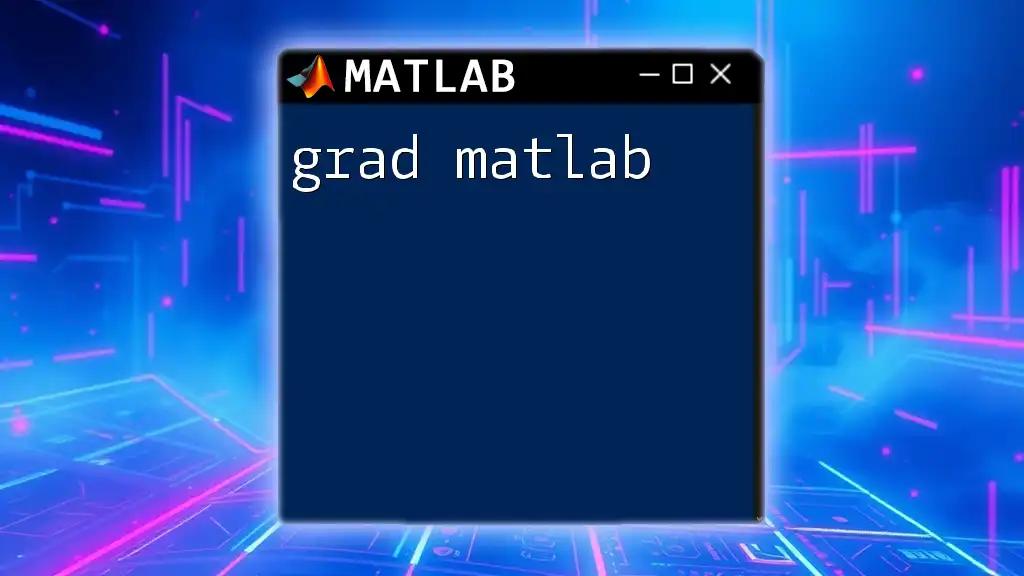
Operations on Arrays
MATLAB provides robust support for both basic and advanced operations on arrays.
Basic Operations
MATLAB supports element-wise operations, allowing you to perform mathematical operations directly on arrays.
H = E + E; % Element-wise addition
J = E .* E; % Element-wise multiplication
Advanced Operations
For more complex mathematical operations:
- Matrix Multiplication:
K = E * E'; % Matrix multiplication (note the transpose of E in this case)
- Applying Functions Across Arrays:
Common functions such as `sum`, `mean`, `max`, and `min` can be applied efficiently:
total = sum(E); % Returns the sum of each column
average = mean(E(:)); % Computes the mean of all elements in E
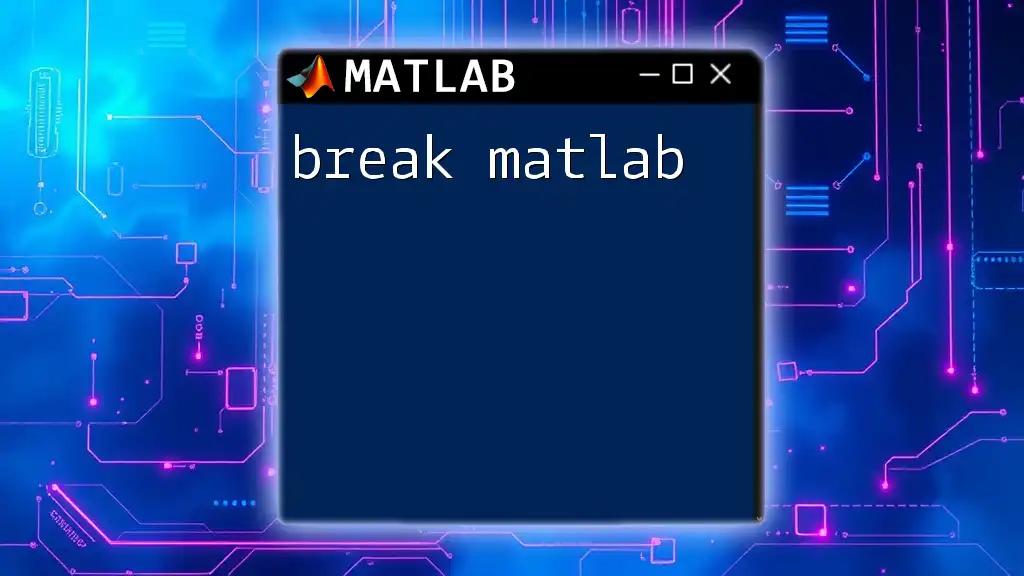
Special Array Types
Cell Arrays
Cell arrays allow you to store different types of data in a single array. This feature is particularly useful when you wish to manipulate heterogeneous datasets.
C = {1, 'hello', [1, 2, 3]};
Struct Arrays
Struct arrays enable you to group related data together using named fields.
S(1).name = 'John';
S(1).age = 25; % Creates a structure array for different attributes
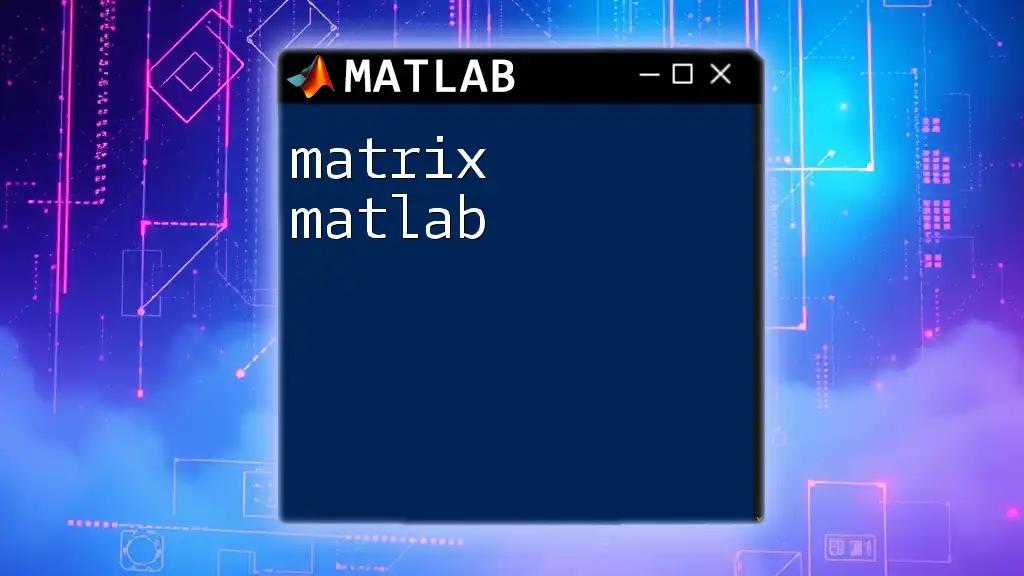
Array Functions and Utilities
A variety of built-in functions enhance your work with arrays, which can be invaluable for efficient programming.
Understanding Array Dimensions
-
`size`: Returns the dimensions of the array.
dimensions = size(E); % E.g., returns [2, 3] for a 2x3 matrix
-
`length`: Provides the length of the largest dimension.
len = length(E); % Returns the length of the largest array dimension
-
`ndims`: Returns the number of dimensions in the array.
numDims = ndims(E); % E.g., returns 2 for a matrix
Reshaping and Permuting
You can reshape and rearrange data within arrays using:
reshapedE = reshape(E, 3, 2); % Reshapes E into a 3x2 matrix
permutedE = permute(E, [2, 1]); % Swaps dimensions of E
Logical Indexing
Logical indexing allows you to filter arrays based on specific conditions, providing a means to extract elements conveniently.
idx = E > 5; % Creates a logical array
filteredE = E(idx); % Extracts elements greater than 5
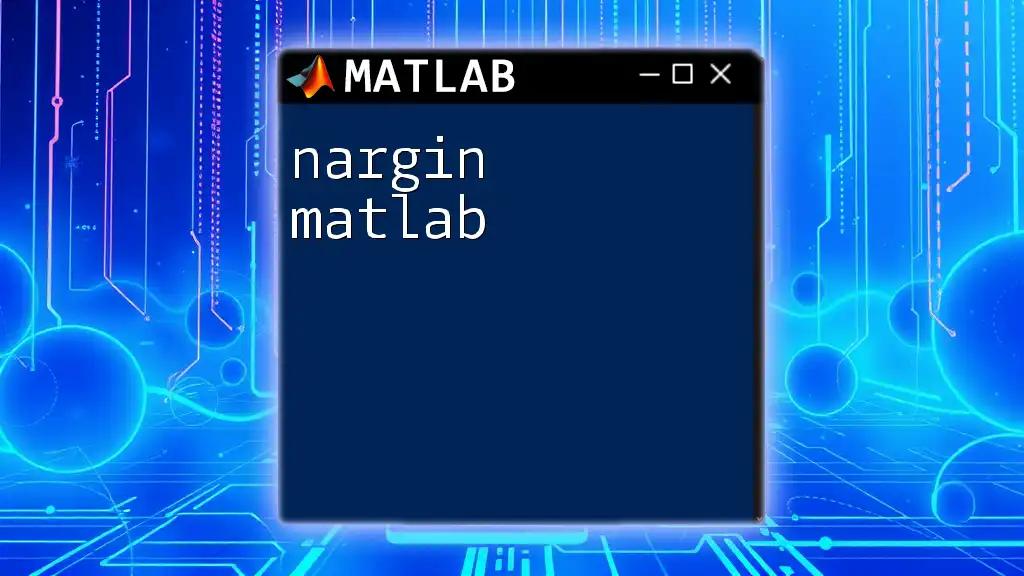
Conclusion
Understanding how to work with arrays in MATLAB is crucial for any programming task. Their versatility across various mathematical operations and data management techniques lays a solid foundation for more advanced analysis in MATLAB. Embrace the power of arrays in MATLAB and explore their capabilities to enhance your programming journey. Practice with different array types and functions to become proficient in your array manipulation skills.
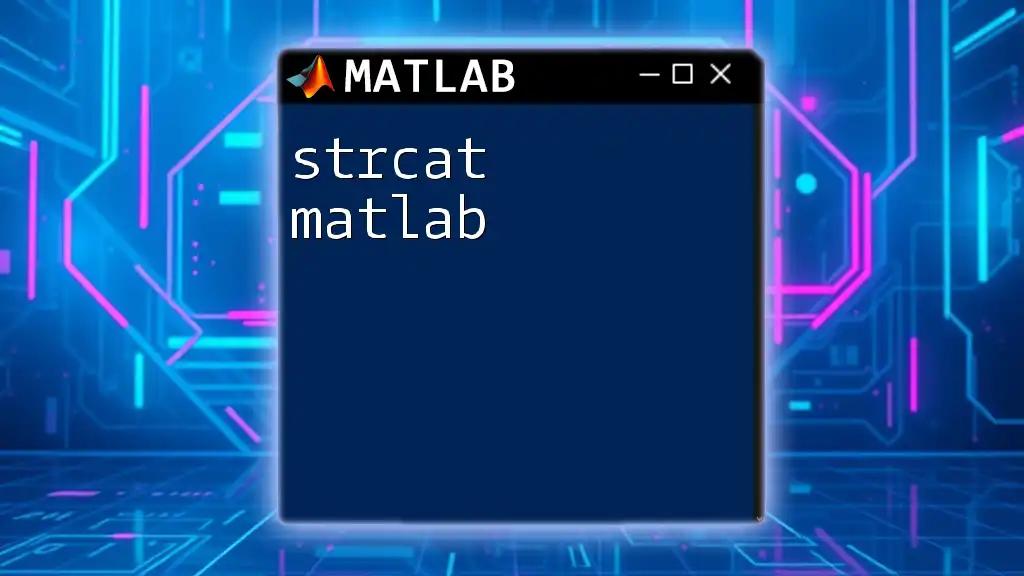
Additional Resources
To further enhance your skills and knowledge of arrays in MATLAB, refer to the official MATLAB documentation. Exploring in-depth tutorials and courses geared toward arrays will deepen your understanding and prepare you for advanced applications in data processing and analysis.