The `textscan` function in MATLAB is used to read formatted data from a text file or string into a cell array, allowing for flexible and efficient data handling.
Here's a simple code snippet using `textscan`:
fid = fopen('data.txt', 'r'); % Open the text file for reading
data = textscan(fid, '%s %f %f', 'Delimiter', ','); % Read strings and two floating-point numbers
fclose(fid); % Close the file
Understanding `textscan`
What is `textscan`?
`textscan` is a powerful MATLAB function designed to facilitate the reading of textual data from files. It is primarily used for importing data when typical functions, like `load`, may not suffice due to the complexity of the file format. This function provides a flexible and robust means to parse data, making it suitable for a variety of applications.
Key Features of `textscan`
One of the major advantages of `textscan` is its flexibility. It allows users to easily read data formatted with various delimiters, such as commas, spaces, or custom characters. This capability is particularly useful when dealing with data presented in different formats. Additionally, `textscan` supports reading different data types from the same file, enabling users to import numeric, string, and logical data seamlessly. Importantly, the function can handle white spaces and irregular data layouts commonly found in textual datasets.
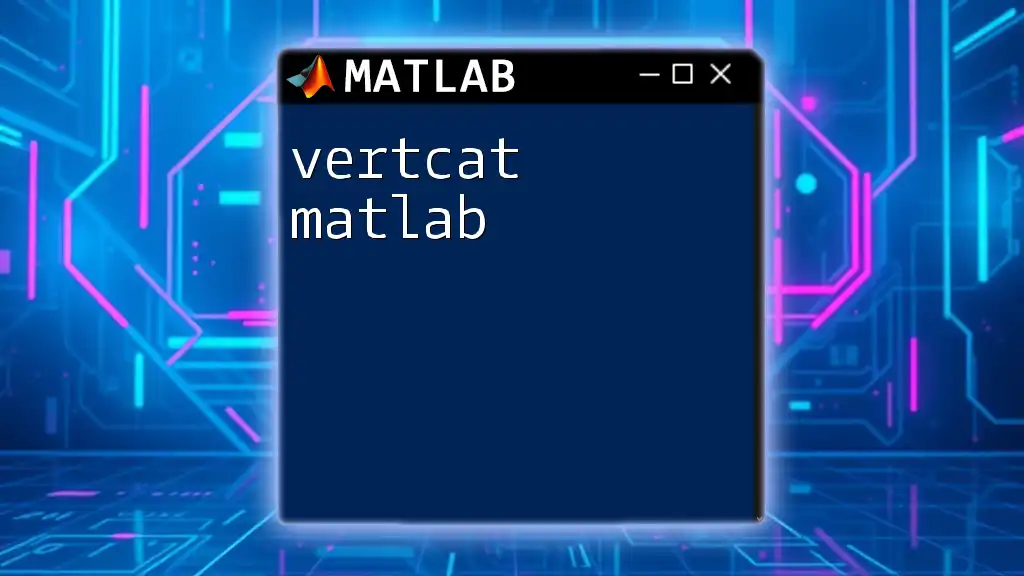
Setting Up the Environment
Preparing Your Files
Before diving into the code, it’s essential to ensure that the text file you’re attempting to read is well-structured. Ideal textual data should be consistent in formatting—for example, if you’re using a comma as a delimiter, each line should follow the same pattern. Here’s a simple example of how a well-structured text file might appear:
1,Apple
2,Banana
3,Cherry
MATLAB Environment
To effectively utilize `textscan`, ensure you are working with a compatible version of MATLAB. Check the MATLAB documentation for specific version requirements if needed. Once your environment is set, you can start writing your scripts.
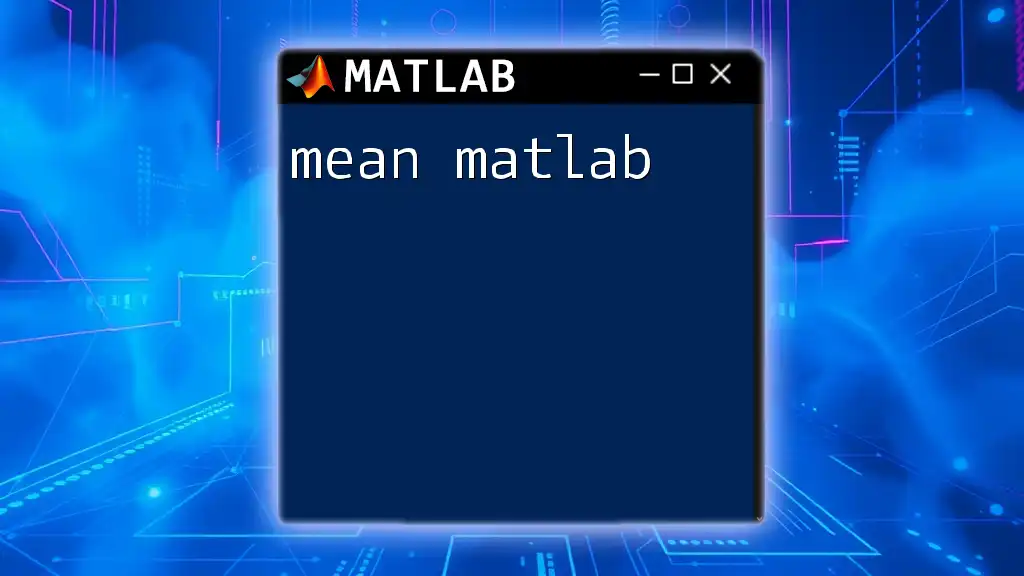
Syntax and Basic Usage
Basic Syntax of `textscan`
The basic syntax of the `textscan` function is as follows:
data = textscan(fileID, formatSpec, 'Name', Value)
In this syntax, `fileID` is the identifier of the file you wish to read, while `formatSpec` defines the expected data types and formats. The `'Name', Value` pairs provide optional parameters to customize the behavior of the function.
Step-by-Step Example
To illustrate the use of `textscan`, let’s take a practical example where we read a simple text file:
fileID = fopen('example.txt', 'r');
data = textscan(fileID, '%f %s', 'Delimiter', ',');
fclose(fileID);
In this code snippet:
- We open a text file named `example.txt`.
- The `textscan` function reads the contents:
- `%f` specifies that the first column contains floating-point numbers.
- `%s` indicates that the second column contains strings.
- The data is then stored in `data`, which will be a cell array.
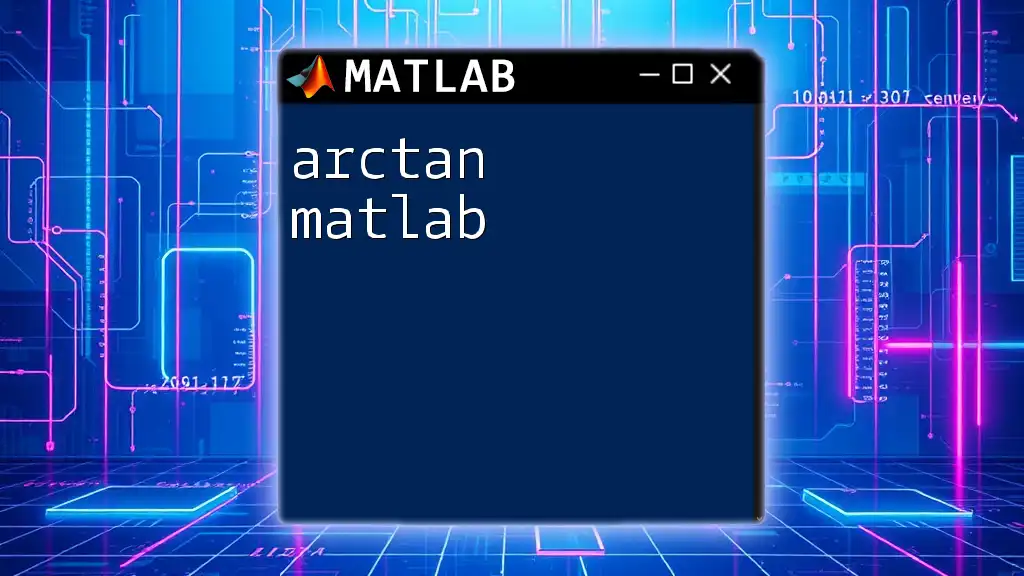
Advanced Features of `textscan`
Specifying Delimiters
Understanding Delimiters
Delimiters are characters used to separate different pieces of data within a file. Common delimiters include commas, tabs, and spaces. The ability to use different delimiters is one of the standout features of `textscan`, allowing it to adapt to various file formats.
Example of Custom Delimiters
To demonstrate, here's how to read data using a semicolon as a delimiter:
data = textscan(fileID, '%f %s', 'Delimiter', ';');
In this example, if your text file uses a semicolon instead of a comma, `textscan` will effectively parse the data accordingly.
Handling Headers and Rows
Skipping Header Lines
When working with data files that contain header lines (descriptive text that identifies the data columns), you can instruct `textscan` to ignore these lines using the `HeaderLines` parameter:
data = textscan(fileID, '%f %s', 'HeaderLines', 1);
This example skips the first line of the file, allowing reading to start from the second line.
Specifying Number of Rows to Read
If you want to limit the number of rows read from a file, you can use the `MaxRows` parameter:
data = textscan(fileID, '%f %s', 'MaxRows', 50);
This will only read the first 50 rows from the file, which can be particularly useful when dealing with large datasets.
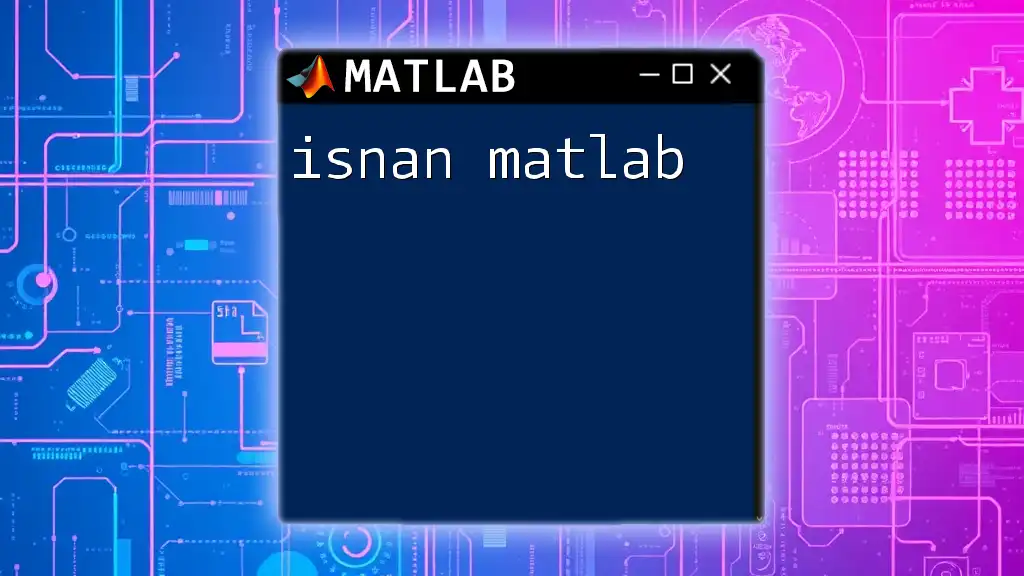
Data Types and Format Specifications
Understanding Format Specifiers
Format specifiers play a crucial role in determining how `textscan` interprets the data. Here are some common specifiers:
- `%f`: For floating-point numbers.
- `%d`: For integers.
- `%s`: For strings.
Example of Mixing Data Types
You can read multiple data types from a single file by specifying different format specifiers:
data = textscan(fileID, '%f %s %d', 'Delimiter', ',');
In this scenario, the first column would be float values, the second would capture strings, and the third would have integer values.
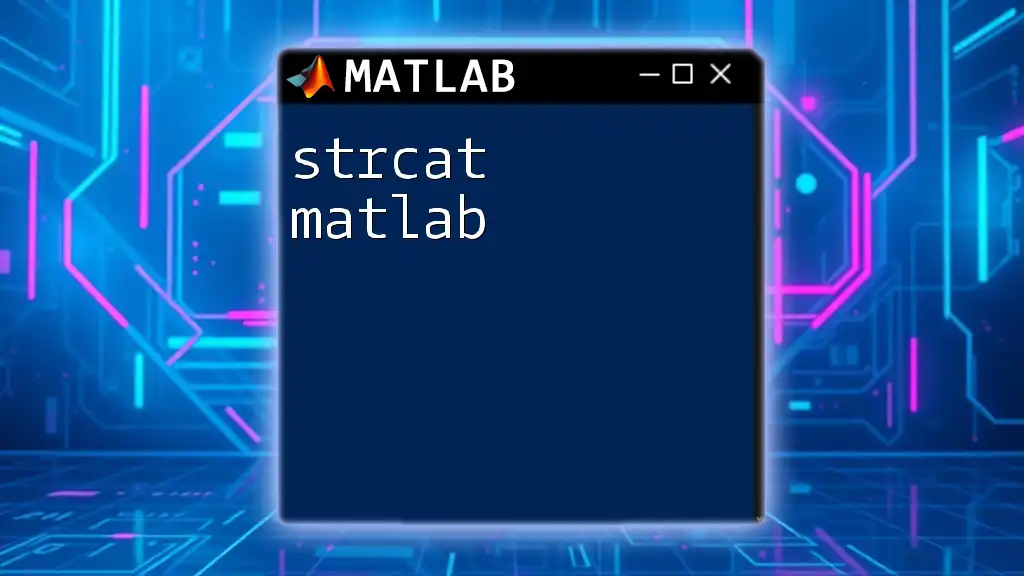
Error Handling and Troubleshooting
Common Errors with `textscan`
While `textscan` is powerful, users may encounter various errors such as mismatched data types or incorrect file format. These errors often arise if the delimiter does not match the actual data structure, or if the expected column type does not correspond to what is in the file.
Best Practices for Avoiding Errors
To minimize potential issues, consider the following best practices:
- Check File Format Before Reading: Always examine the structure of your text file to confirm the delimiters and data types.
- Use Try-Catch Blocks: Implementing a try-catch block can help gracefully handle errors:
try
data = textscan(fileID, '%f %s', 'Delimiter', ',');
catch ME
fprintf('Error reading file: %s\n', ME.message);
end
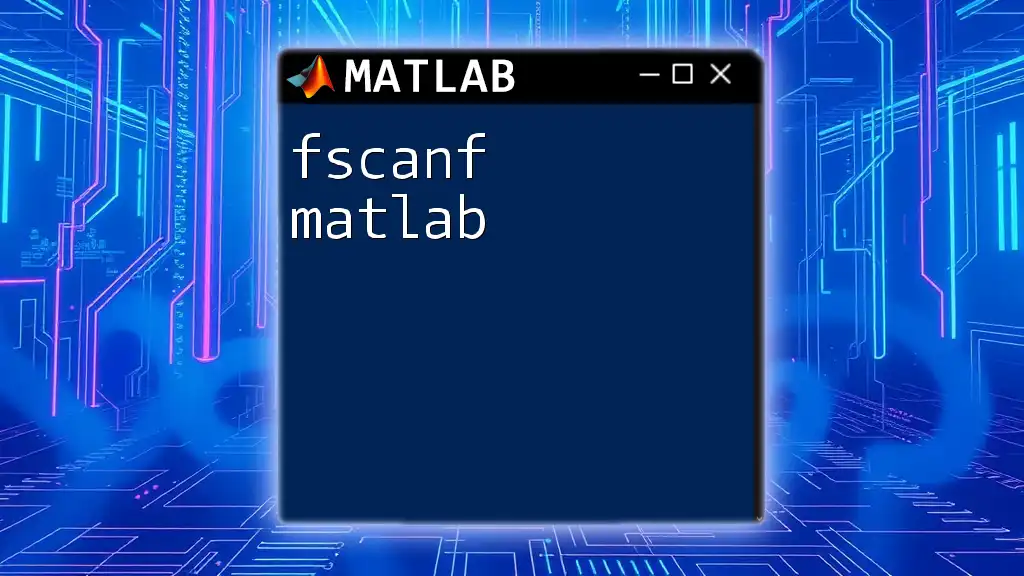
Practical Examples
Example 1: Reading Numeric Data
Consider a text file containing numeric data structured as follows:
1
2
3
4
You can read this data easily with:
fileID = fopen('numbers.txt', 'r');
data = textscan(fileID, '%f');
fclose(fileID);
This will import the numbers into a cell array ready for processing.
Example 2: Reading Mixed Data Types
For a file with both numeric and string data:
1,Apple
2,Banana
3,Cherry
You could use the following:
fileID = fopen('fruits.txt', 'r');
data = textscan(fileID, '%d %s', 'Delimiter', ',');
fclose(fileID);
This will read the integers as the first column and strings as the second.
Example 3: Reading Data with Custom Delimiters
For a data file where entries are separated by semicolons:
1;Apple
2;Banana
3;Cherry
The reading code would be:
fileID = fopen('fruits_semicolon.txt', 'r');
data = textscan(fileID, '%d %s', 'Delimiter', ';');
fclose(fileID);
This versatility allows users to adapt `textscan` to their specific data formats easily.
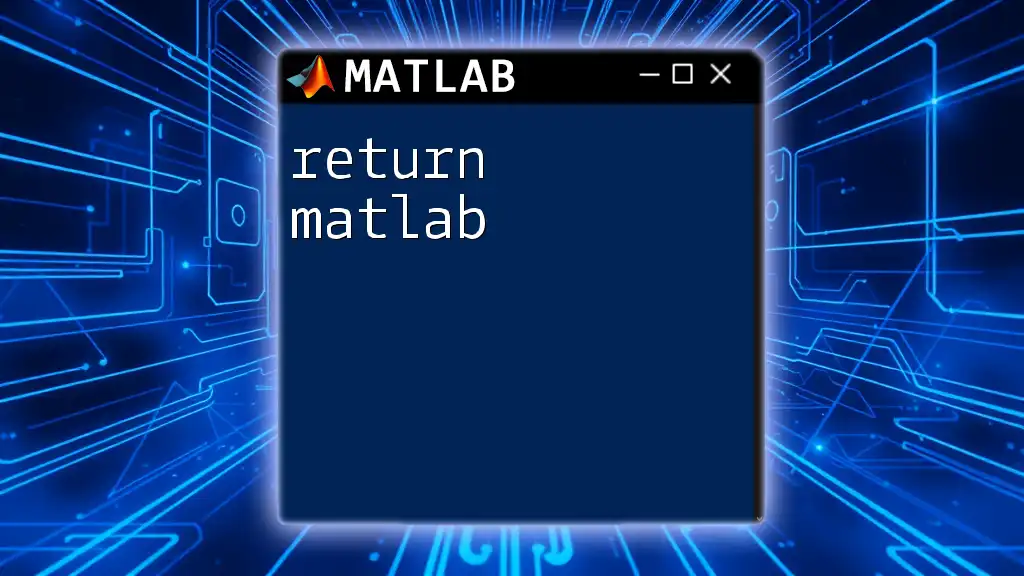
Performance Considerations
Speed and Efficiency
`textscan` is generally efficient for data reading tasks, but keep in mind that for very large files, the read speed might slow down. Always test imports with smaller files first before applying to larger datasets.
Memory Usage
When dealing with large datasets, memory usage can become an issue. To optimize memory consumption, ensure that you are reading only what you need. Use parameters like `MaxRows` to limit data imports to necessary portions of data.
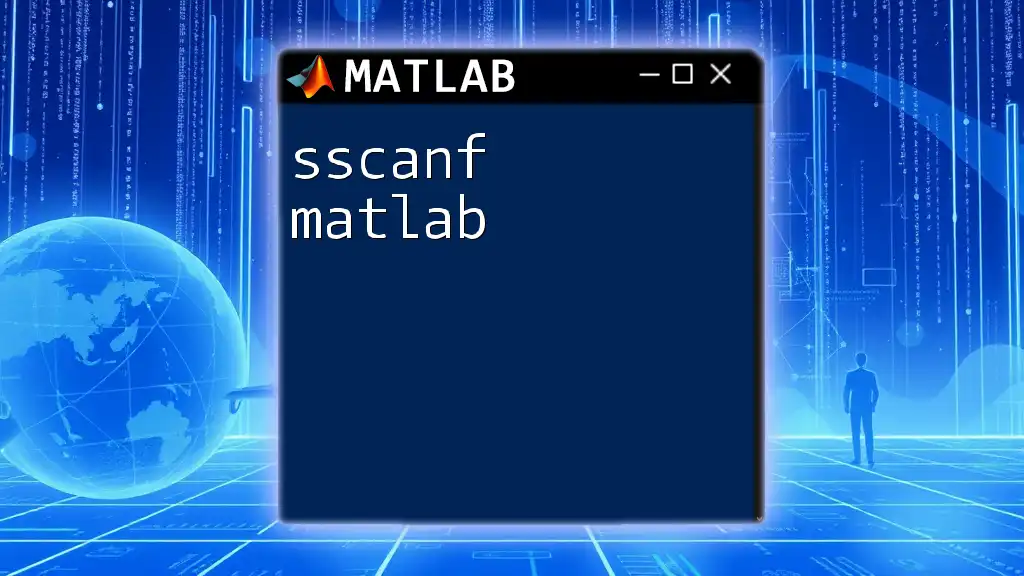
Conclusion
The `textscan` function in MATLAB stands as a versatile solution for users needing to import complex textual data. Understanding its syntax, parameters, and capabilities allows for efficient and effective data manipulation. Experimenting with this function can significantly streamline your workflows and enhance your data processing insights.
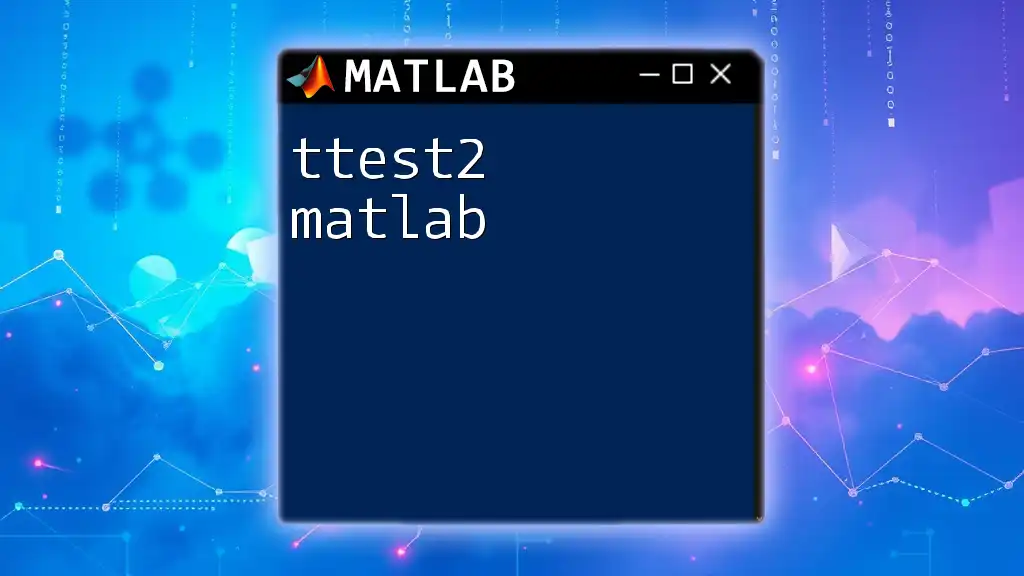
Additional Resources
For further reading on `textscan` and data processing in MATLAB, consult the official MATLAB documentation and consider enrolling in additional online courses focusing on MATLAB programming.
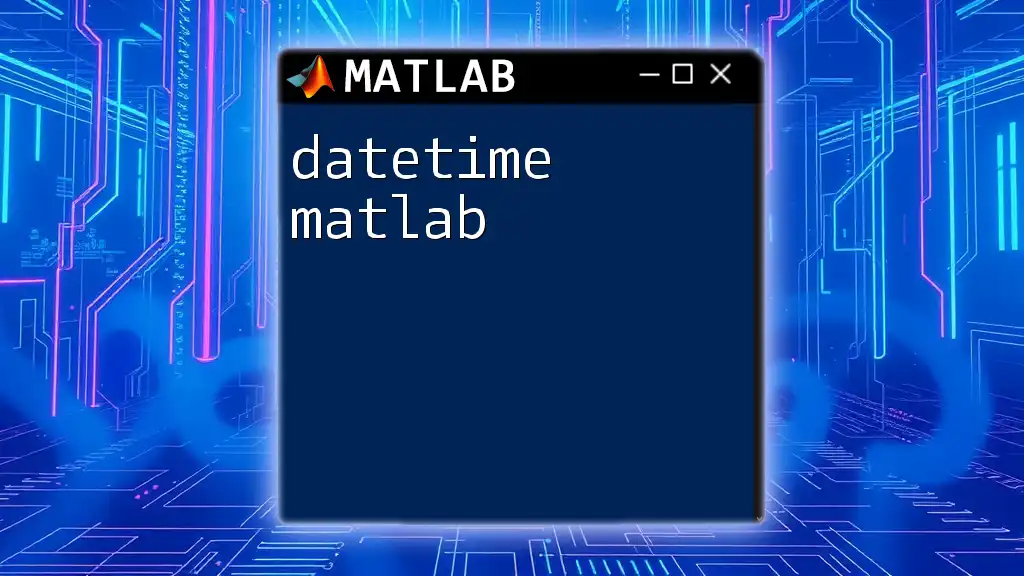
FAQs
Common Questions About `textscan`
-
What if my file has inconsistent column widths?
- Inconsistent data can complicate imports; ensure each line follows a coherent structure.
-
How can I read files with unusual encoding?
- Use the `Encoding` parameter in `textscan` to support various file encodings.
-
Is it possible to read Excel files with `textscan`?
- No, although there are other functions like `readtable` and `xlsread` that can handle Excel files. `textscan` is specifically suited for text files.