The `zeros` function in MATLAB creates an array of zeros with specified dimensions, making it useful for initializing matrices.
A = zeros(3, 4); % Creates a 3-by-4 matrix filled with zeros
What is the `zeros` Function?
The `zeros` function in MATLAB is a fundamental tool that allows users to create arrays filled with zeros. It plays a critical role in initializing matrices and vectors, particularly in computational and mathematical applications. Understanding how to effectively use this function can significantly streamline your coding process.
Understanding the Basics
The syntax for the `zeros` function is straightforward:
Z = zeros(sz)
or
Z = zeros(m, n)
Here, `sz` can be a single value specifying the size of a square matrix, while `m` and `n` define the number of rows and columns, respectively.
Key Features
The `zeros` function supports various data types, with the default being double precision. You can also create single precision arrays by specifying the type:
Z = zeros(3, 'single'); % Returns a 3x3 matrix of single precision zeros
It is essential to understand how `zeros` differs from related functions like `ones` and `rand`. While `zeros` fills an array with zeros, `ones` fills it with ones, and `rand` populates it with random numbers. Each of these functions serves unique purposes in matrix manipulation and mathematical calculations.
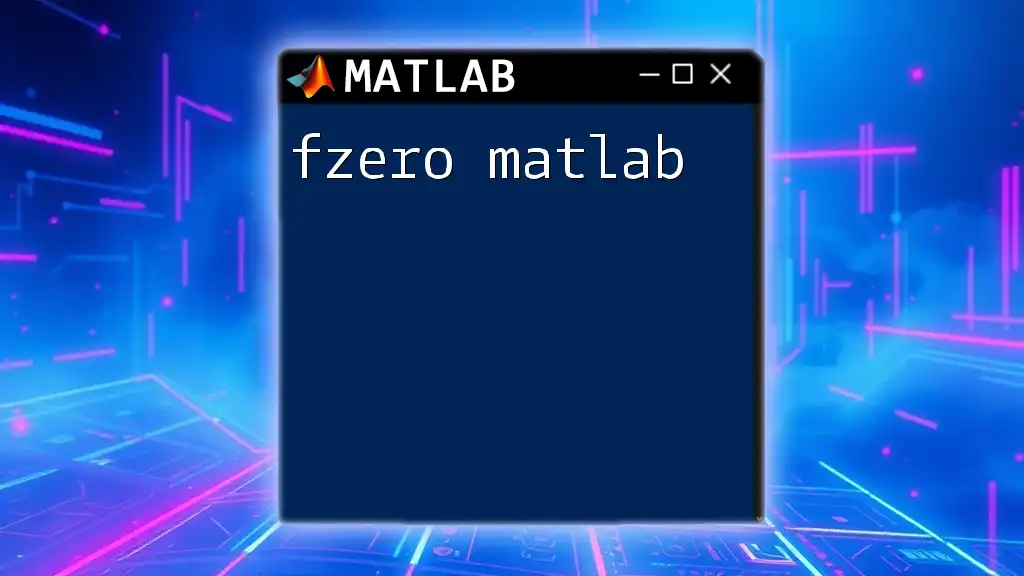
How to Use `zeros` in MATLAB
Creating a Vector of Zeros
Creating a vector of zeros is quite simple with the `zeros` function. You can easily generate both row and column vectors.
For a row vector:
rowVector = zeros(1, 5); % Example of a row vector
This line of code creates a row vector containing five zeros.
For a column vector:
columnVector = zeros(5, 1); % Example of a column vector
This generates a column vector with five zeros.
Explanation: The parameters define the dimensions of the vector. Specifying `1, 5` gives you a single row with five columns, while `5, 1` gives you five rows in a single column.
Creating a Matrix of Zeros
Two-Dimensional Zeros
To create a 3x4 matrix of zeros, you can simply use:
zeroMatrix = zeros(3, 4); % Example of a 3x4 matrix
This generates a matrix with three rows and four columns filled with zeros.
Explanation: Understanding the significance of matrix dimensions is crucial, especially when working on tasks that demand specific matrix sizes for mathematical operations.
Higher-Dimensional Zeros
You can also create higher-dimensional arrays filled with zeros. For example, a 2x3x4 array can be generated using:
zeroArray = zeros(2, 3, 4); % Example of a 2x3x4 array
Discussion: The ability to create multi-dimensional arrays is particularly useful for simulations and data manipulations, where you may need to handle complex data structures like 3D images or multi-channel signals.
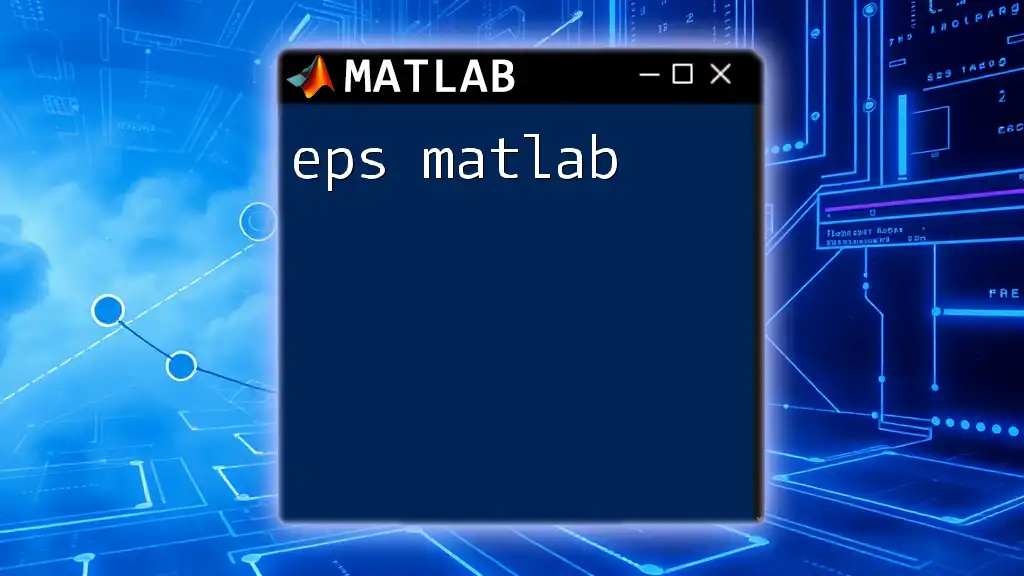
Common Use Cases for `zeros`
Initializing Variables
When writing code, particularly in iterative algorithms, initializing variables is a crucial step. The `zeros` function helps in preparing arrays before algorithms modify their values. For example:
resultArray = zeros(1, 10); % Prepare an array for results
for i = 1:10
resultArray(i) = i^2; % Storing squared values
end
Here, `resultArray` is initialized with zeros to ensure it has a defined structure before storing calculated values.
Placeholder in Algorithms
Using `zeros` as placeholders can aid in scenarios when the final dimensions of an array are unknown during the initial coding phase. Consider the following example:
arraySize = 5;
placeholderArray = zeros(arraySize); % Placeholder for further calculations
This approach is beneficial when working on optimization problems or during simulations where the exact size is determined only after processing initial data.
Integration with Other Functions
The `zeros` function can be seamlessly integrated with other MATLAB functions. For instance, you can use `reshape` to modify the structure of a zeros array:
B = zeros(2, 2);
C = reshape(B, 1, []); % Reshapes to a single row
Explanation: This integration allows you to adapt your data structures dynamically, providing more flexibility in data manipulation.
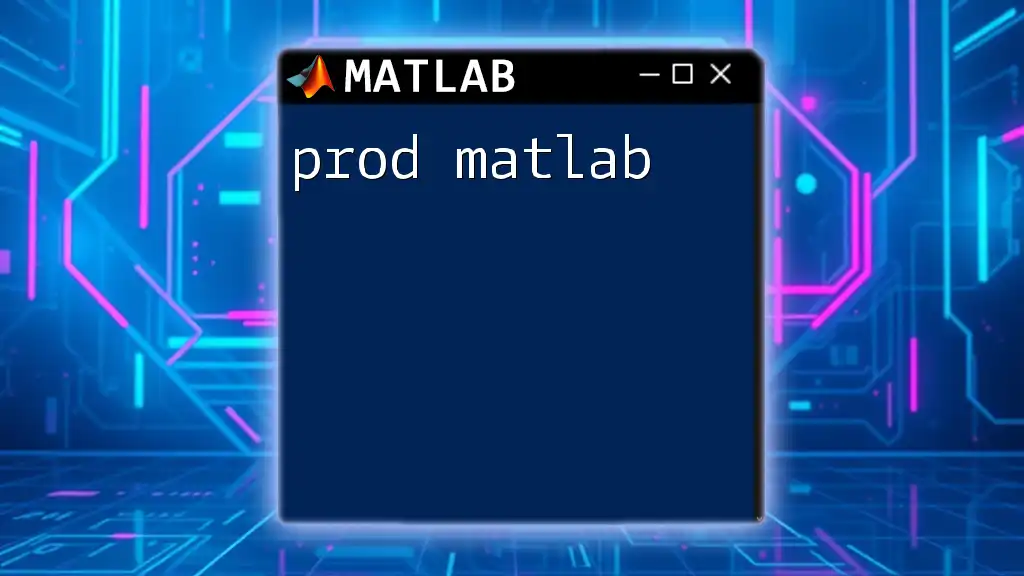
Performance Considerations
Memory Allocation
Efficient memory allocation is one of the greatest strengths of the `zeros` function. MATLAB allocates the necessary memory for zeros arrays in a way that optimizes performance, especially compared to manually populating arrays with loops.
Comparison with Other Methods
Using `zeros` to create arrays is generally more efficient than implementing traditional loops to individually assign zero values to elements:
% Less efficient method
for i = 1:n
for j = 1:m
matrix(i,j) = 0; % Inefficient
end
end
In contrast, using `zeros` is a cleaner and faster approach:
matrix = zeros(m, n); % More efficient, cleaner code
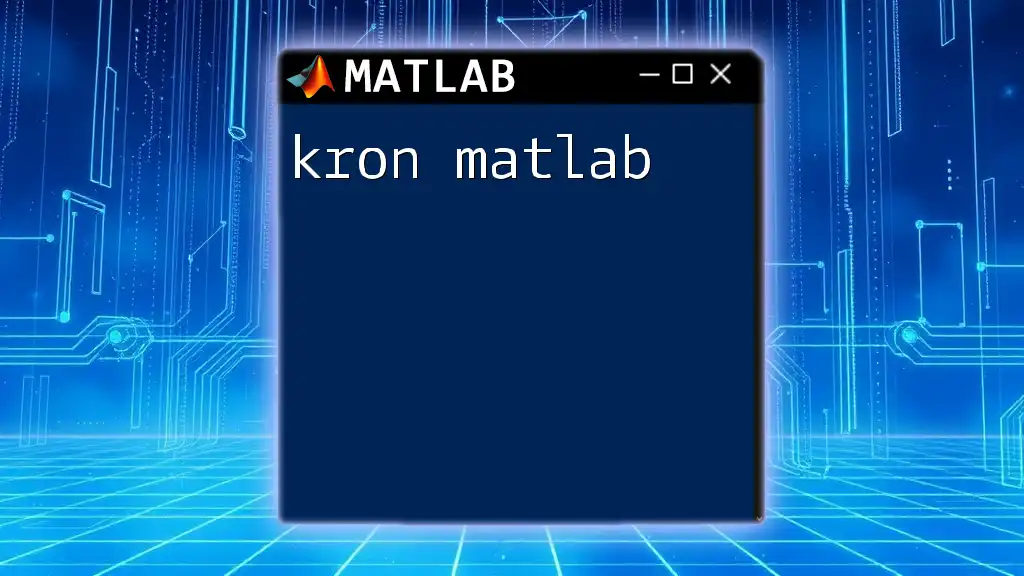
Troubleshooting Common Issues
Dimension Mismatch
One common error users encounter is dimension mismatch, particularly when manipulating arrays after creation. For example, if you attempt to assign values to an array that doesn’t conform to its original dimensions, MATLAB will throw an error.
Type Mismatches
Be mindful of data types; for instance, if you create a zeros array but attempt to assign values that are incompatible (like integers to a floating-point matrix), you may run into issues. It’s crucial to ensure that the types match your data’s requirements during operations.
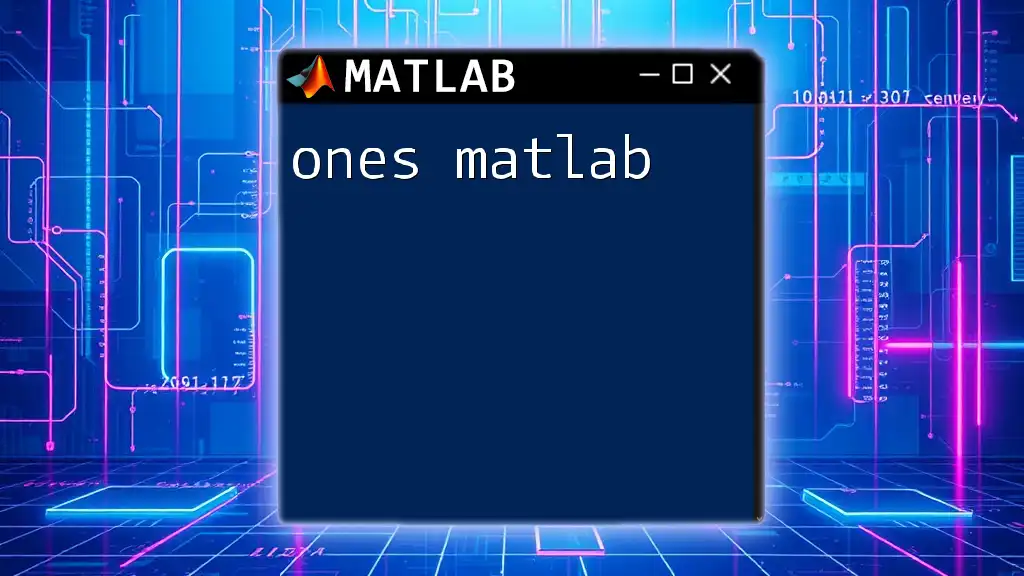
Conclusion
The `zeros` function in MATLAB is not just a simple utility; it is a versatile tool essential for initializing and handling arrays effectively across various applications. Its ability to facilitate the creation of multi-dimensional arrays and its integration with other MATLAB functions make it indispensable for developers and researchers working with complex datasets.
Encouraging practice and experimentation with the `zeros` function will undoubtedly enhance your MATLAB skills, paving the way for effective problem-solving in a multitude of scenarios.
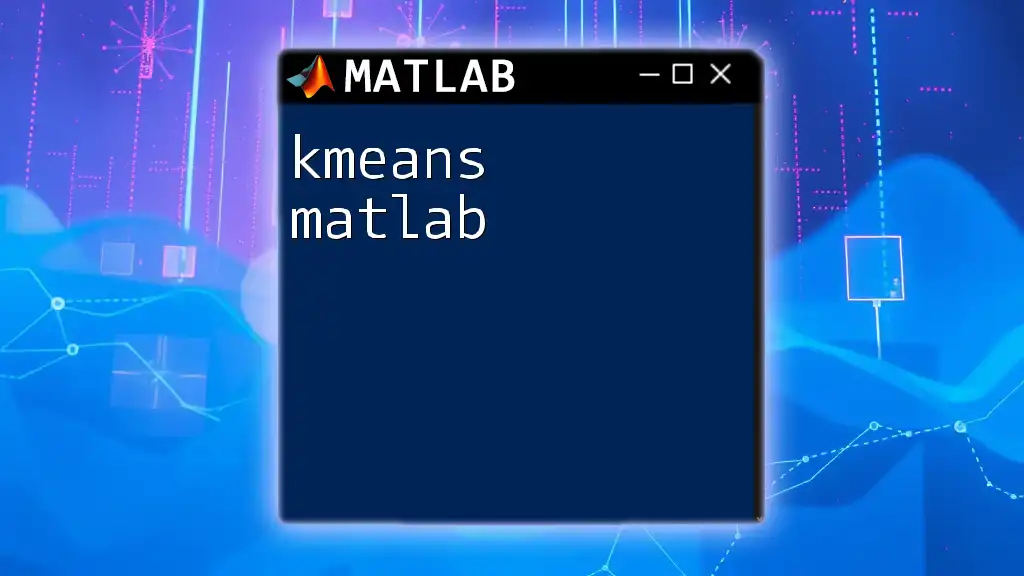
Further Resources
For those looking to deepen their understanding, referring to the official MATLAB documentation is highly recommended. Additional tutorials and courses can also provide invaluable context and examples for mastering MATLAB. Finally, engaging with the MATLAB community can offer support and insights into overcoming obstacles and refining your techniques.