In MATLAB, you can clear a graph from the current figure window using the `cla` command, which removes all content from the axes while keeping the axes intact.
cla;
Understanding MATLAB Graphs
What are MATLAB Graphs?
MATLAB graphs are visual representations of data that help users analyze patterns, trends, and relationships within data sets. These graphs serve various purposes, ranging from simple data visualization to complex statistical analysis. Some common types of graphs available in MATLAB include 2D plots, 3D plots, and histograms, each serving its unique function based on the data being analyzed.
Common Uses for Graphs
Graphs in MATLAB find applications in numerous scenarios:
- Data visualization: Presenting data in a way that is easy to interpret.
- Statistical analysis: Helping to describe data distributions and trends.
- Feature exploration: Allowing for the identification of significant features in data sets.
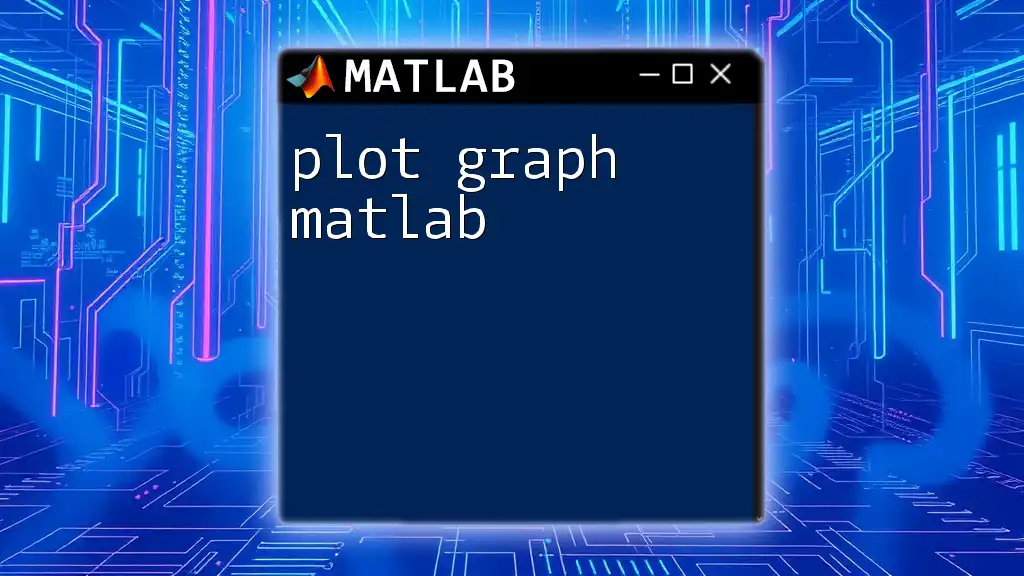
The Importance of Clearing Graphs
Why Clear Graphs Matter
The clarity of a graph significantly impacts the effectiveness of data presentation. Clear graphs lead to better understanding and interpretation of data. If a graph is cluttered with too much information or poorly organized, it may mislead the viewer, leading to erroneous conclusions.
Consequences of Cluttered Graphs
A cluttered graph can have several negative outcomes:
- Overlapping data points may make it difficult to discern individual values.
- Excessive legends and labels can clutter the visual landscape and distract from the main points.
- Inadequate space can lead to confusion, obscuring the actual insights that one should draw from the data.
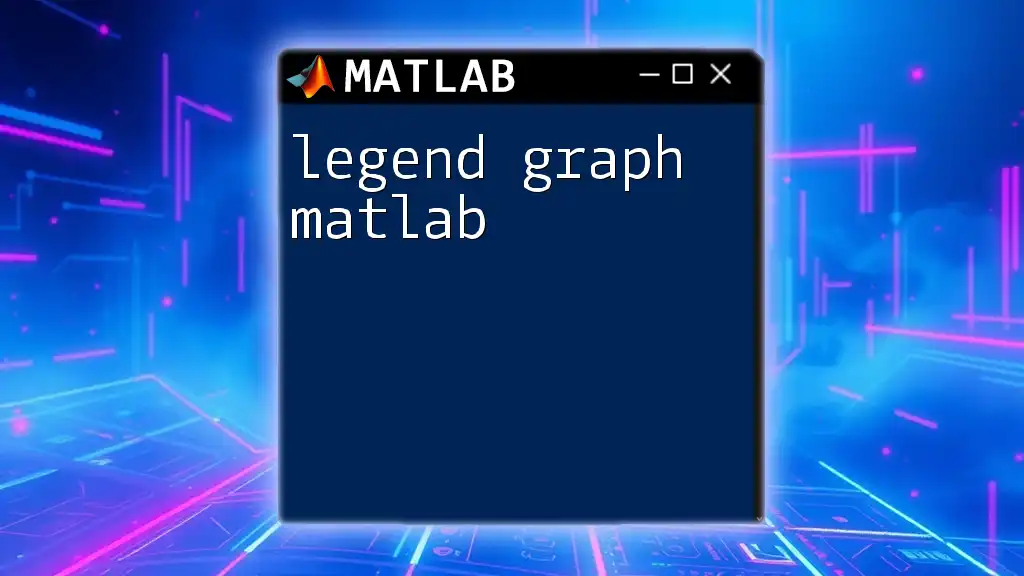
Clearing Graphs in MATLAB
Basic Commands to Clear Graphs
In MATLAB, there are specific commands that allow users to clear graphs and improve their clarity.
-
`clf` Command: This command clears the current figure window, which is useful when creating multiple plots in a single session.
Example:
clf;
This command essentially resets the figure window, allowing you to start fresh without any previous plots clinging to the visual space.
-
`cla` Command: Unlike `clf`, which clears the figure, `cla` clears the current axes without deleting the axes themselves.
Example:
cla;
This can be particularly useful when you want to update the same axes with new data while preserving the axes properties (like limits).
Using `close` Command
Another important command in managing MATLAB figures is the `close` command.
-
`close all` Command: This command closes all open figure windows, which is beneficial for freeing up memory and avoiding confusion when working with numerous figures.
Example:
close all;
Using this command ensures that all previous graphs are closed, providing a clean slate for further analysis.
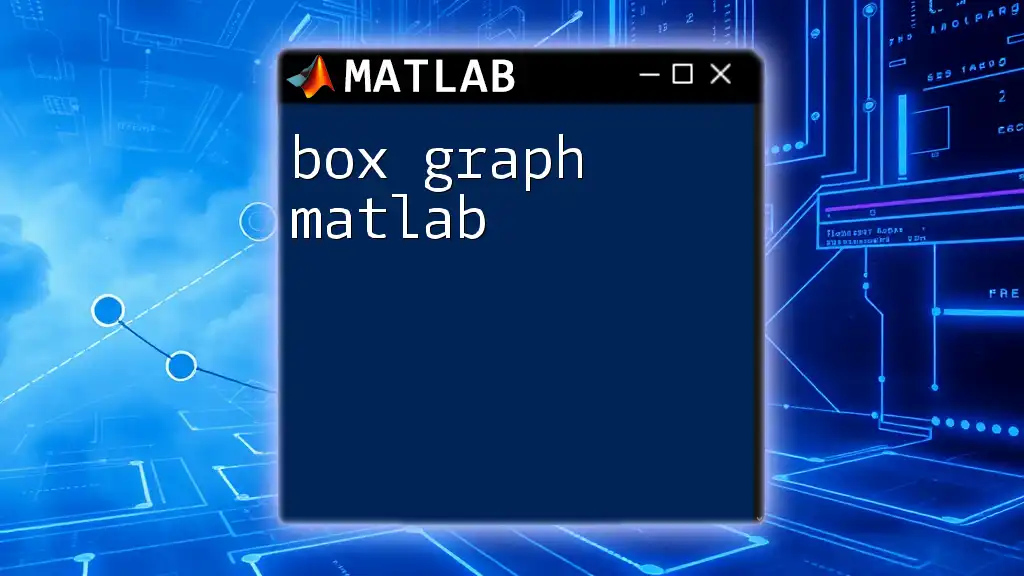
Best Practices for Clear Graphs
Organizing Your Data
Before plotting, organizing your data effectively is crucial. Well-structured data not only enhances visualization but also aids in clearer interpretation. Consider exporting tidy data formats, such as tables in MATLAB, that allow for easy manipulations and plots.
Limiting Graph Complexity
Simplicity is key when it comes to effective data representation. Limiting the complexity of your graphs can significantly enhance their readability. Here are strategies to consider:
- Use fewer data points when revealing clear trends.
- Limit the number of axes and subplots.
- Avoid cluttered annotations and excessive information.
Creating a graph before and after cleaning it up offers a vivid visual comparison of clarity.
Utilizing Colors and Legends Wisely
Choosing appropriate color schemes enhances the graph's clarity. A well-constructed color scheme can not only differentiate data sets but also convey meaning.
Consider these tips:
- Utilize color gradients to represent varying levels or scales.
- Ensure legends are placed strategically to avoid overlapping with data points while remaining informative.
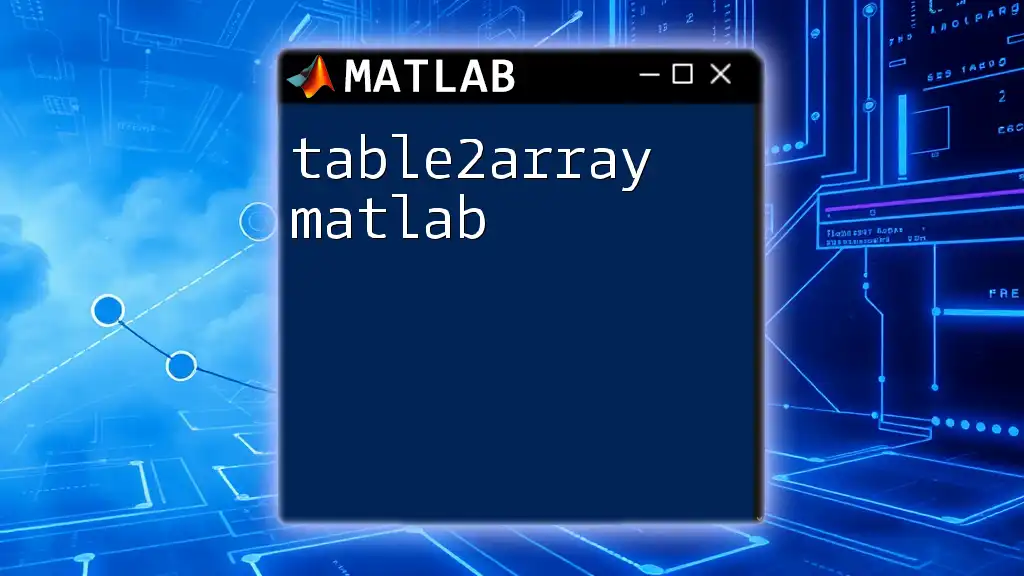
Examples of Clearing Graphs in MATLAB
Example 1: Basic Plot with `clf`
To illustrate the use of the `clf` command, let’s consider the following simple plot:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
clf; % Clear the plot
In this example, the sine wave plot is displayed and then cleared, showcasing how `clf` resets the figure window for new data.
Example 2: Using `cla` with Multiple Plots
Using `cla` permits the updating of current axes with new data while retaining their properties. Here's a demonstration:
x = 0:0.1:10;
plot(x, sin(x));
hold on;
plot(x, cos(x));
pause(2);
cla; % Clear the axes
plot(x, tan(x)); % Plot new data
This example presents sine and cosine functions, holds the axes for a brief period, and then clears it to plot the tangent function without reinitializing the figure.
Example 3: Clearing and Closing Figures
In scenarios where many figures are opened, management is essential. The `close all` command can efficiently streamline this process by closing every open figure:
for i = 1:5
figure;
plot(rand(10,1));
end
close all; % Close all figures
In this loop, multiple figures are created displaying random data, and subsequently, the command cleans up all windows for a fresh start.
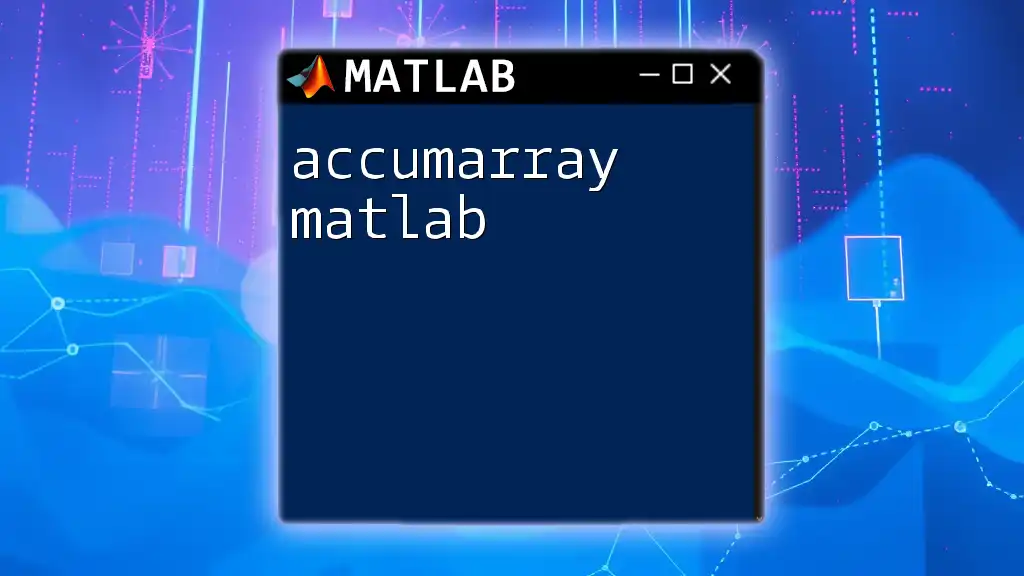
Conclusion
Understanding how to clear graphs in MATLAB is foundational for presenting data effectively. Employing commands like `clf`, `cla`, and `close all` helps maintain clarity in your visualizations. Encourage practice with these commands in your MATLAB projects to enhance your data presentation skills. Share your experiences and tips to engage with the learning community.