The `padarray` function in MATLAB expands the size of an array by padding it with specified values, zeros by default, in specified dimensions.
A = [1 2; 3 4];
B = padarray(A, [1 1], 0); % Pads the array A with zeros, creating a 3x4 array
What is `padarray`?
The `padarray` function in MATLAB is designed to add padding to arrays, typically for the purpose of preparing them for various operations in image processing, signal processing, and other numerical computations. Padding involves surrounding an array with additional elements (usually zeros or specified values) that maintain the data's dimensions, making it easier to apply filters, convolutions, or other algorithms that require a full neighborhood of values around each element.
Syntax of `padarray`
The basic syntax for `padarray` is as follows:
B = padarray(A, padsize)
Where:
- A is the input array you want to pad.
- padsize is an array that specifies the size of padding for each dimension of A. For instance, if you want to pad a 2D array both vertically and horizontally, you can specify how many rows and columns to add.
The extended syntax includes additional parameters:
B = padarray(A, padsize, padval, direction)
Where:
- padval is the value used for padding. By default, this is zero, but you can specify any numeric value.
- direction determines how the padding is applied. It can be 'both' (default), 'pre', or 'post', indicating where to add the padding.
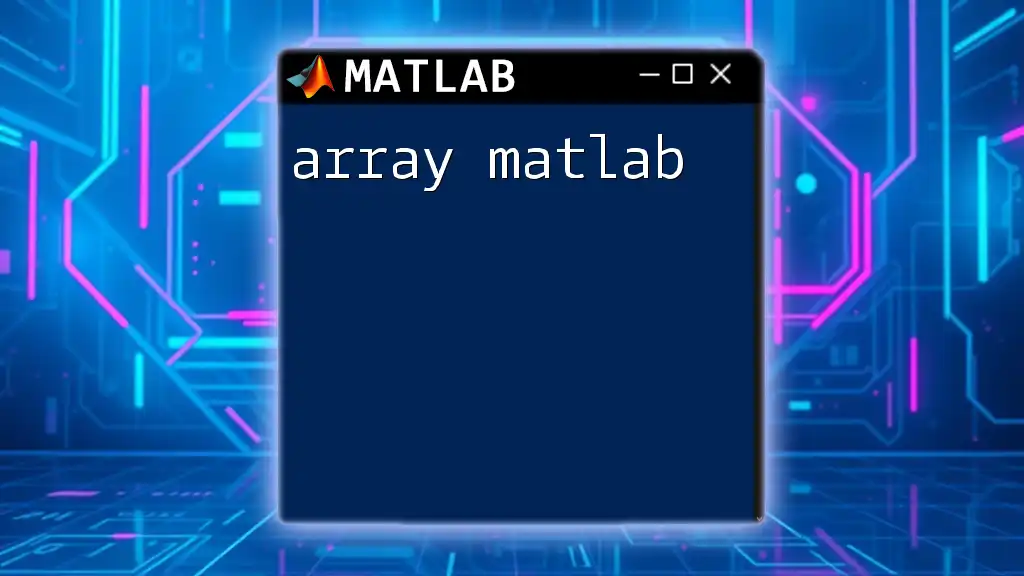
Why Use Padding?
Padding serves several crucial purposes in data processing, particularly in contexts such as image and signal handling:
-
Preventing Information Loss: In operations like convolution or correlation, edge pixels often do not have neighboring pixels surrounding them. Padding helps include those edges in the calculation, ensuring no information is lost.
-
Facilitating Operations: Many mathematical operations, including filtering and resizing, require inputs that are larger than the original array’s dimensions. Padding enables operations to be performed over the entire dataset.
Common Applications of Padded Arrays
- Image Processing: Padding is widely used in filtering or convolution operations to smoothly handle edges without reducing the output quality.
- Signal Processing: When analyzing signals, padding can help in alignment and assessment across varying lengths of signals.
- Machine Learning Data Preprocessing: Neural network inputs often require fixed dimensions. Padding serves to standardize input sizes.
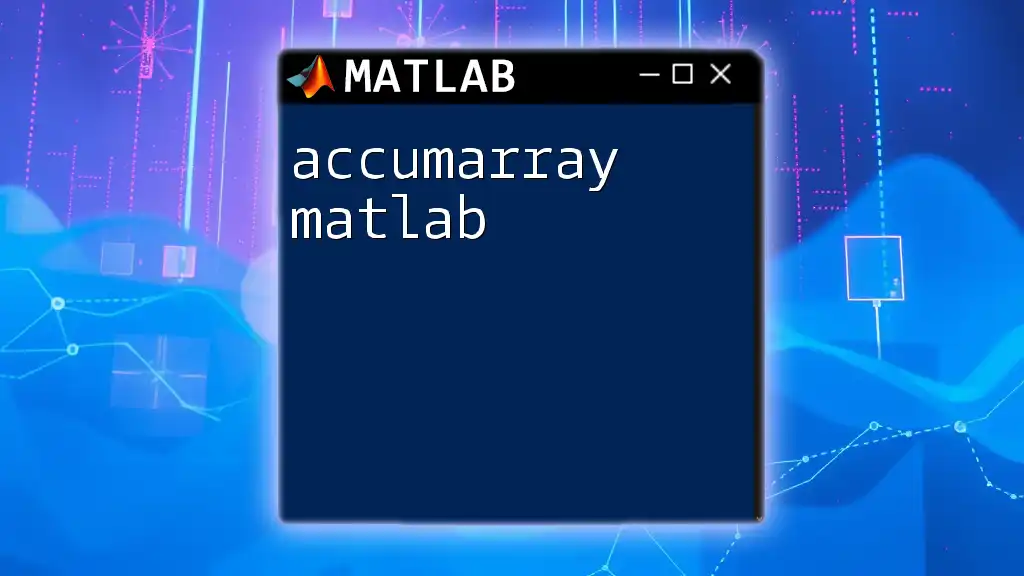
Syntax Breakdown of `padarray`
Basic Syntax
B = padarray(A, padsize)
In this command, if you have a matrix A that you need to pad, you specify how much padding to add through `padsize`:
For example:
A = [1, 2; 3, 4];
B = padarray(A, [1, 1]);
disp(B);
This code creates a 2x2 matrix A and pads it with one row and one column of zeros around the edges, resulting in:
0 0 0
0 1 2
0 3 4
0 0 0
Advanced Syntax
B = padarray(A, padsize, padval, direction)
By introducing `padval`, you get to specify what values are used for padding. The direction parameter provides additional control over where the padding is applied.
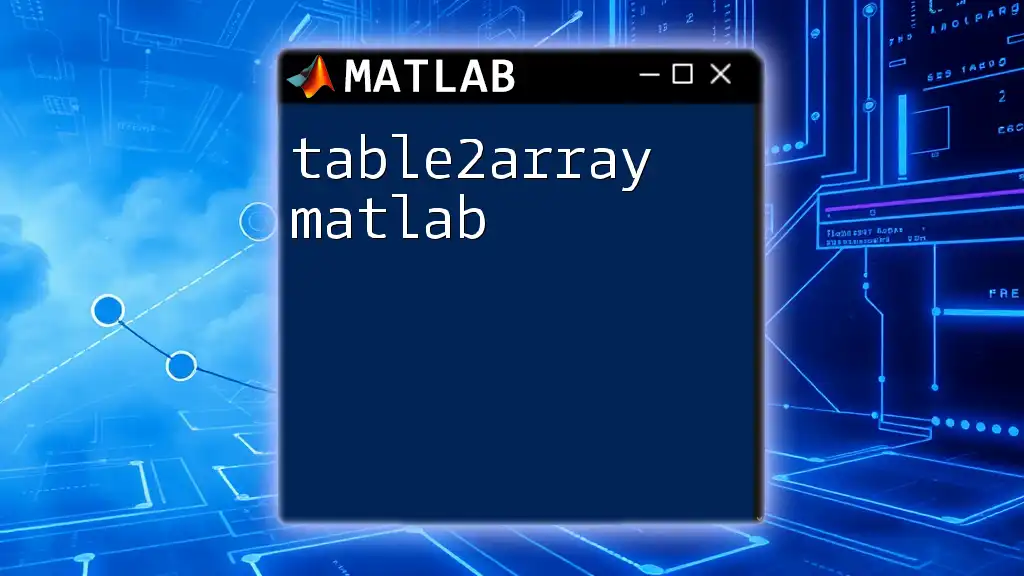
Common Padding Scenarios
Zero Padding
Zero padding is the most common type of padding, where the additional elements added around the original array are zeros. This is especially relevant when working with convolutional layers in neural networks or during filtering operations.
Example Code Snippet
A = [1, 2; 3, 4];
B = padarray(A, [1, 1]);
disp(B);
The output produces additional rows and columns, each filled with zeros.
Symmetric Padding
Symmetric padding mirrors the elements at the boundary. This method is particularly useful for maintaining continuity in your dataset.
Example Code Snippet
B = padarray(A, [1, 1], 'symmetric');
disp(B);
The output would look like:
4 3 4
2 1 2
4 3 4
Reflective Padding
This method replicates the boundary elements rather than adding zeros or symmetry. It is beneficial when you want to maintain certain edge characteristics without introducing more zeros.
Example Code Snippet
B = padarray(A, [1, 1], 'replicate');
disp(B);
The resulting matrix appears as follows:
1 1 2
1 2 4
3 4 4
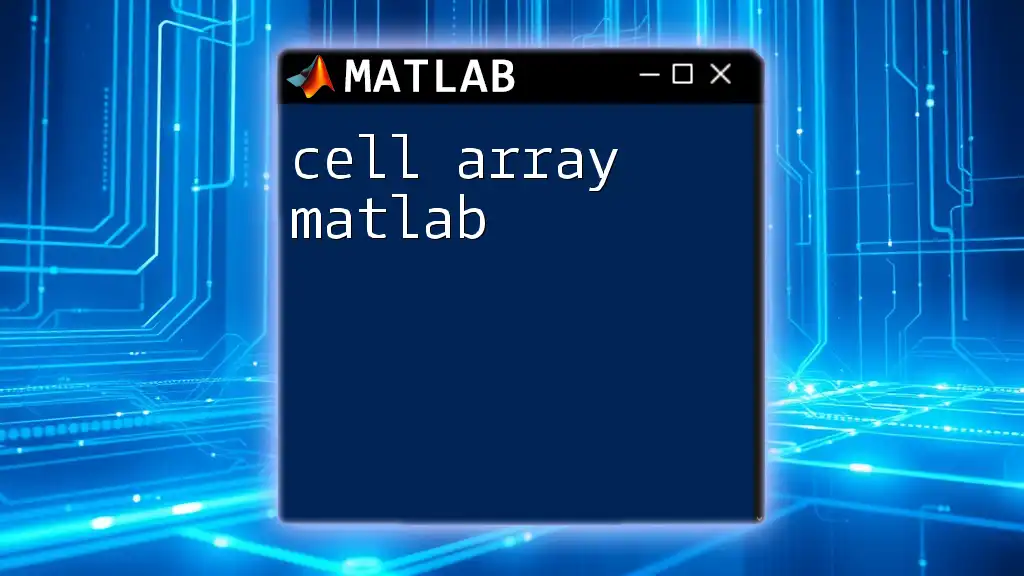
Directions for Padding
Padding Before and After
You can control when and where to apply your padding using the 'pre' and 'post' options.
Example Code Snippet for Both
B_pre = padarray(A, [1, 1], 'pre');
B_post = padarray(A, [1, 1], 'post');
In this case:
- B_pre will add a row and column of zeros before A (top and left)
- B_post will add a row and column of zeros after A (bottom and right)
Padding on All Sides
Using 'both' is the default behavior of `padarray`, where padding is added symmetrically on all sides.
Example Code Snippet
B = padarray(A, [1, 1], 'both');
This command adds padding in all directions, effectively enlarging the matrix with zeros around it.
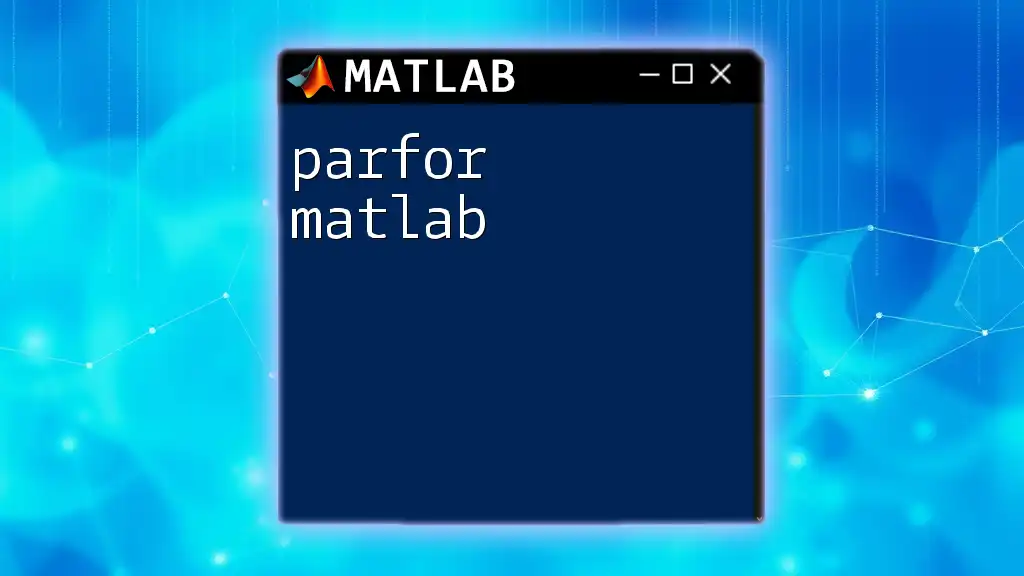
Padding with Custom Values
You can also specify a custom value for padding, giving you flexibility beyond the default zero padding.
Example Code Snippet
B = padarray(A, [1, 1], 5); % Padding with the value 5
disp(B);
The output will look like this:
5 5 5
5 1 2
5 3 4
5 5 5
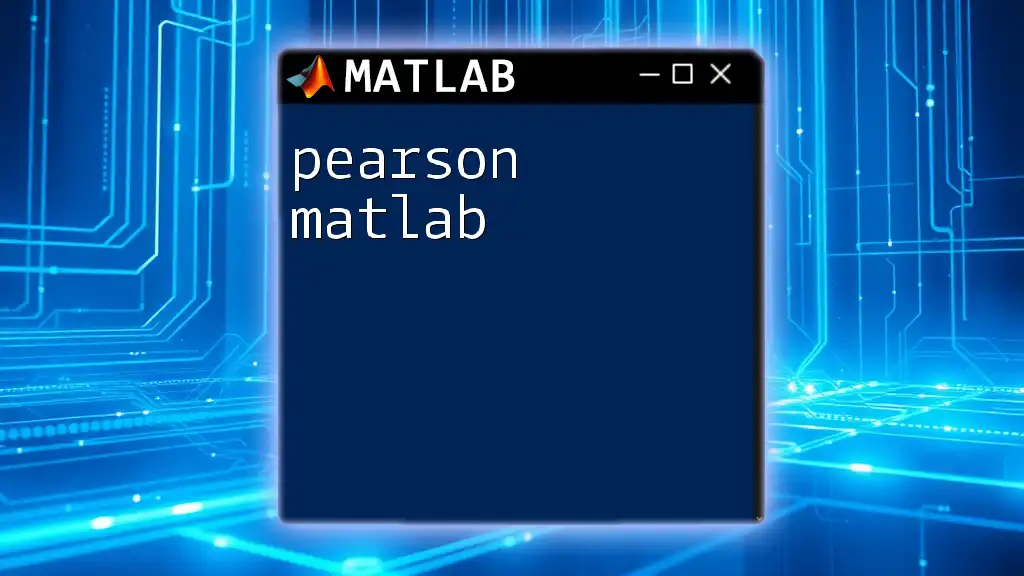
Conclusion
The `padarray` function is a powerful tool within MATLAB for manipulating arrays effectively. By understanding and leveraging different types of padding, users can mitigate information loss, facilitate various data processing operations, and maintain the integrity of edge data. The various padding options offer flexibility, making it easier to adapt pre-existing data structures for advanced algorithm implementations.
FAQs
What does `padarray` return?
`padarray` returns a new array with the specified padding applied, which is crucial for ensuring that array operations work as intended without errors related to dimensions.
Can I use `padarray` on multi-dimensional arrays?
Yes, `padarray` can handle multi-dimensional arrays correctly as long as you specify the appropriate `padsize` for each dimension.
Is there a limit on the padding size?
While there is no fixed limit within MATLAB, it's essential to consider the performance implications and the overall size of data structures, as excessive padding may lead to inefficiencies in memory usage.
Additional Resources
- For detailed documentation, refer to the [MATLAB official documentation on `padarray`](https://www.mathworks.com/help/images/ref/padarray.html).
- Explore additional video tutorials or MATLAB courses for a deeper understanding of array manipulations and functions.
With this guide, you are encouraged to explore the `padarray` function and experiment with different settings to see its effects on your work. Share your experiences and questions in the comments below, and let’s enhance our MATLAB skills together!