In MATLAB, you can easily create a simple 2D plot using the `plot` function, which allows you to visualize data by defining the x and y coordinates of your points.
Here's a quick example of how to plot a graph in MATLAB:
x = 0:0.1:10; % Generate x values from 0 to 10 with an increment of 0.1
y = sin(x); % Compute the sine of each x value
plot(x, y); % Plot the graph of y vs. x
title('Sine Wave'); % Add title to the graph
xlabel('x values'); % Label x-axis
ylabel('sin(x)'); % Label y-axis
grid on; % Add grid lines for better readability
Understanding Graphs in MATLAB
What is a Graph?
In the context of MATLAB, a graph is a visual representation of data points that can illustrate relationships, trends, and patterns within datasets. Graphs are essential tools for analyzing and interpreting data intuitively. MATLAB supports various types of graphs, including 2D line plots, 3D surface plots, scatter plots, and even specialized plots like bar graphs and histograms. Each type serves a unique purpose, enabling users to convey different aspects of their data effectively.
Why Use MATLAB for Plotting?
MATLAB is widely regarded as one of the premier platforms for data visualization due to its powerful capabilities and flexibility. Some advantages of using MATLAB to plot graphs include:
- Ease of Use: MATLAB's syntax is straightforward, making it accessible for beginners while still powerful for advanced users.
- Rich Functionality: With built-in functions and toolboxes, users can create a wide range of graphs without the need for extensive coding.
- Interactivity: MATLAB provides options for interactive plots, allowing users to explore data dynamically.
- Customizability: Users can customize nearly every aspect of their graphs—colors, markers, lines, and annotations—tailoring their visualizations to meet specific needs.
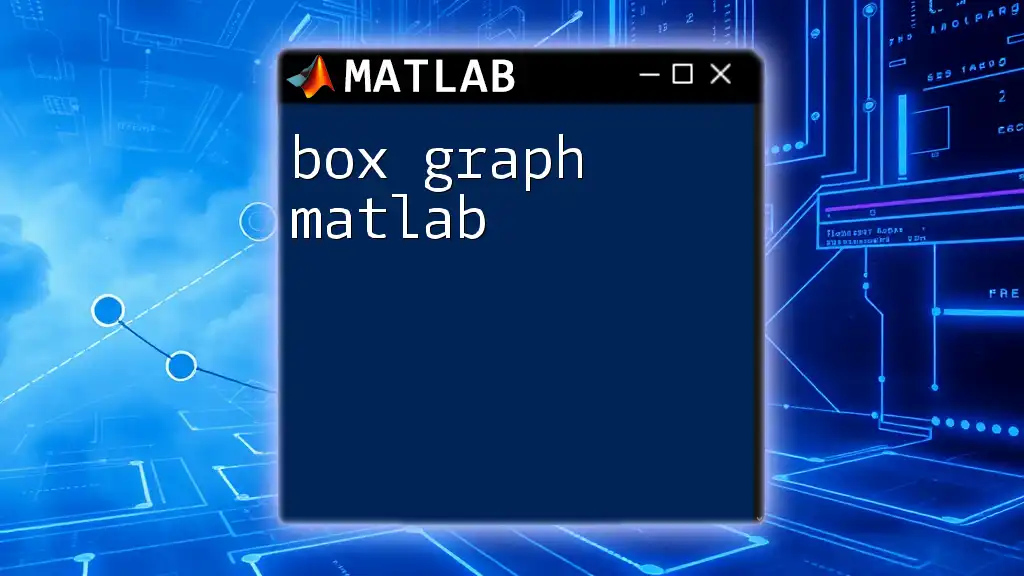
Getting Started with Plotting in MATLAB
Installing MATLAB
Before diving into plotting, you need to have MATLAB installed. The installation process is typically straightforward:
- Visit the MathWorks website and navigate to the MATLAB download section.
- Select the version appropriate for your operating system.
- Follow the instructions to complete the installation.
Ensure you have the necessary toolboxes, such as the MATLAB Graphics Toolbox, to access advanced plotting features.
Basic Syntax for Plotting
The core function for creating plots in MATLAB is the `plot` function. The basic syntax is as follows:
plot(x, y)
Here, `x` and `y` are vectors of data points that you want to plot. It is crucial that both vectors have the same length, as each `x` value corresponds to a `y` value.
Example: Simple 2D Plot
Creating a simple 2D plot is a great way to start. Below is an example that demonstrates how to plot a sine wave:
x = 0:0.1:10;
y = sin(x);
figure;
plot(x, y);
title('Simple Sine Wave');
xlabel('x values');
ylabel('sin(x)');
grid on;
In this example, we generate `x` values from 0 to 10, then compute the sine of these values, producing the `y` vector. The commands `title`, `xlabel`, and `ylabel` add necessary context to the graph, while `grid on` improves readability by displaying grid lines on the plot.
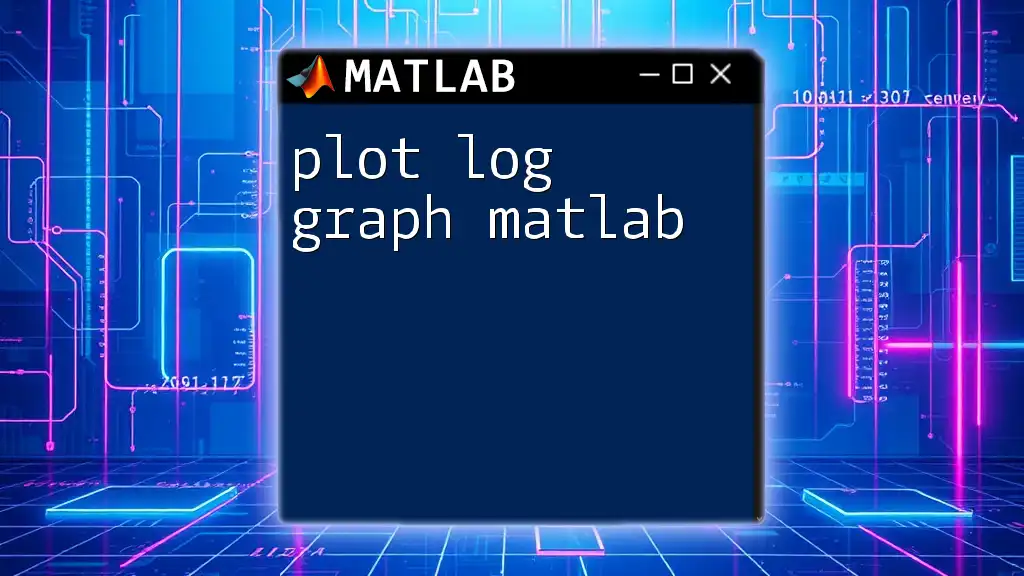
Customizing Your Plots
Adding Titles and Labels
Adding descriptive titles and labels to your plots is vital for clarity:
Plot Titles
To add a title to your graph, use the following command:
title('Your Title Here');
A good title ensures the viewer understands what the graph represents at a glance.
Axis Labels
Labeling the x and y axes is equally important:
xlabel('X Axis Label');
ylabel('Y Axis Label');
Clear labels provide critical context for interpreting the data represented in the plot.
Legends and Annotations
Adding a Legend
When plotting multiple datasets on a single graph, it's essential to include a legend to differentiate between them:
legend('First Dataset', 'Second Dataset');
Legends help viewers understand which line corresponds to which dataset, especially in comparative analyses.
Annotating Points
If you want to highlight specific data points on your graph, you can annotate them with the `text` function:
text(x_value, y_value, 'Annotation Text');
This adds context to critical data points, making your graphs more informative.
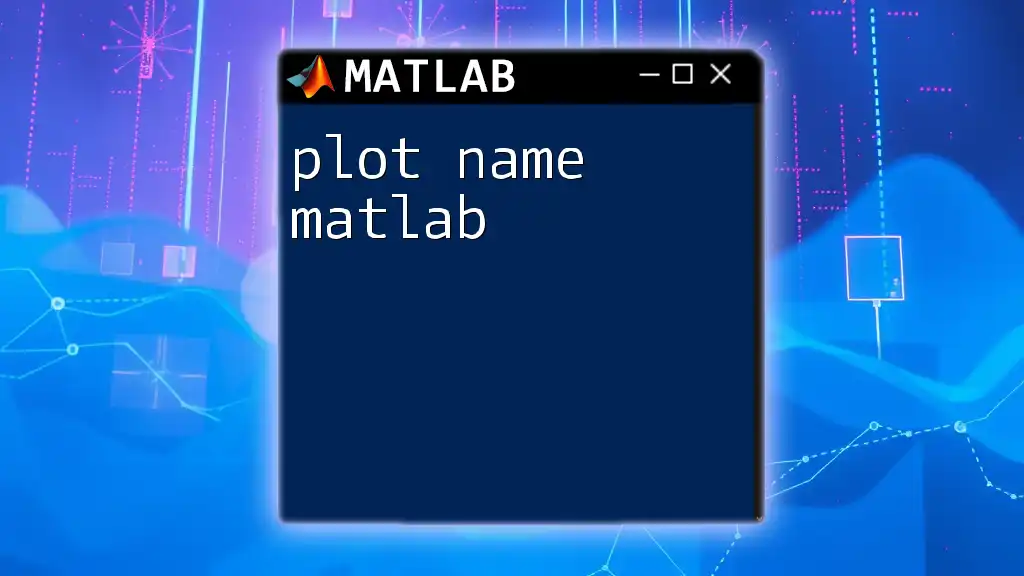
Advanced Plotting Techniques
3D Plots
For more complex data visualizations, MATLAB allows users to plot graphs in three dimensions using the `plot3` function. The syntax looks like this:
plot3(x, y, z);
Example of a 3D Plot
Here’s an example that illustrates how to create a 3D helix:
t = 0:0.1:10;
x = sin(t);
y = cos(t);
z = t;
figure;
plot3(x, y, z);
title('3D Helix');
xlabel('sin(t)');
ylabel('cos(t)');
zlabel('t');
grid on;
This code generates a three-dimensional spiral, effectively showing how the variables are related in a visual space.
Multiple Graphs in One Plot
When you need to display several graphs on one plot, MATLAB offers a straightforward way to achieve this with the `hold on` command:
hold on;
plot(x, y1); % first plot
plot(x, y2); % second plot
hold off;
Using `hold on` allows you to superimpose multiple datasets on the same set of axes, which is valuable for comparative analysis.
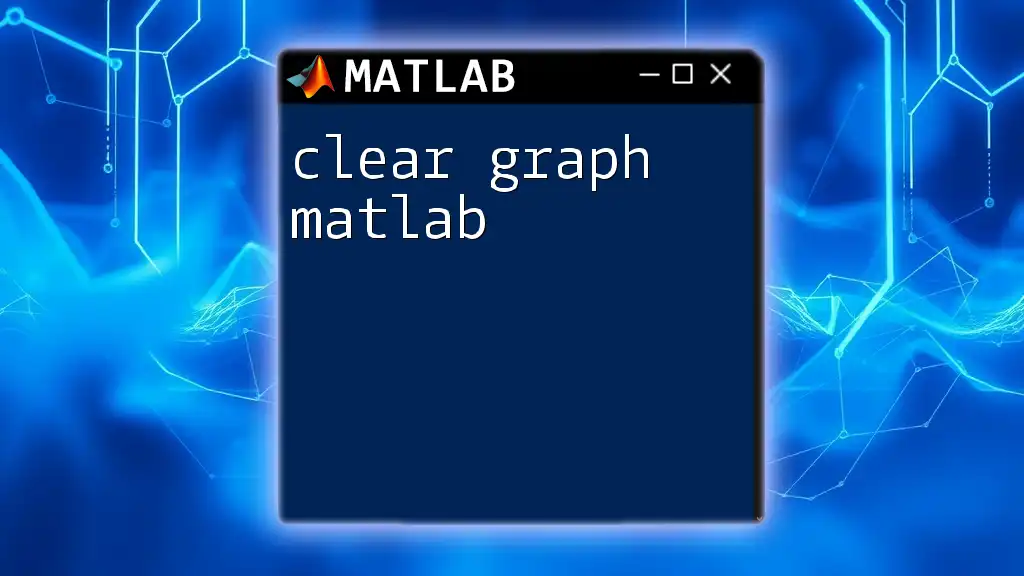
Saving and Exporting Plots
Saving Graphs
Once you have created your plot, you may want to save it for later use. Use the `saveas` function to export your figure in various formats:
saveas(gcf, 'filename.png');
This command saves the current figure (gcf) as a PNG file, but you can choose formats like JPEG, PDF, or EPS by altering the file extension.
Exporting for Publications
For those needing high-quality figures for publications, MATLAB allows you to export graphics in formats that retain resolution and clarity. This is essential to meet the rigorous standards of academic and professional publications.
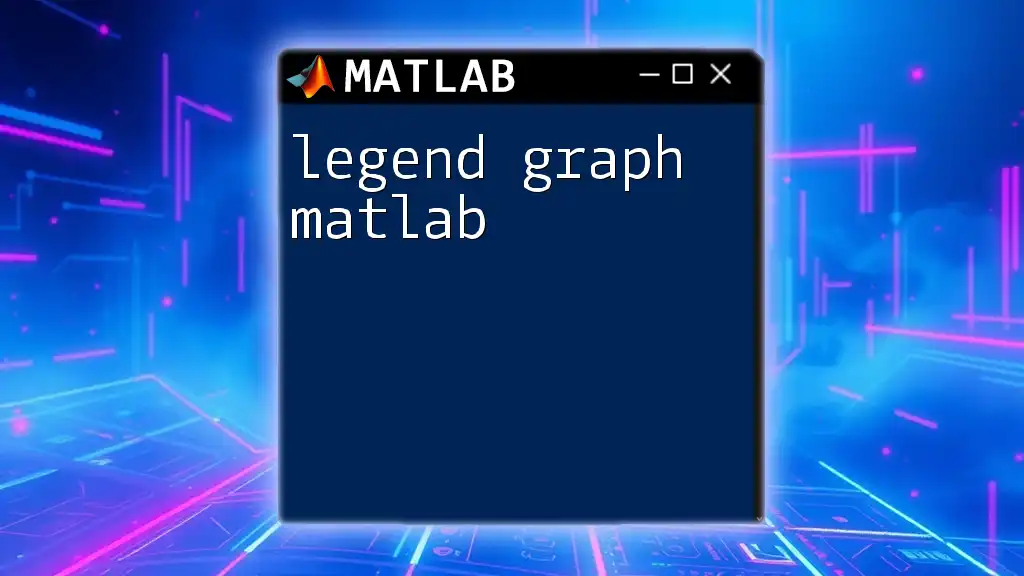
Troubleshooting Common Plotting Issues
Common Errors
While plotting graphs in MATLAB is generally straightforward, users may encounter common errors, such as:
- Mismatched Vector Sizes: Ensure your `x` and `y` data vectors are the same length to avoid errors.
- Figure Not Displaying: Ensure that you call `figure` before plotting, which opens a new window for your plot.
Debugging Tips
When facing issues with your plots, some useful debugging strategies include:
- Check Data Types: Ensure your data is numeric.
- Use `disp()`: Insert `disp(x)` or `disp(y)` commands to check values before plotting.
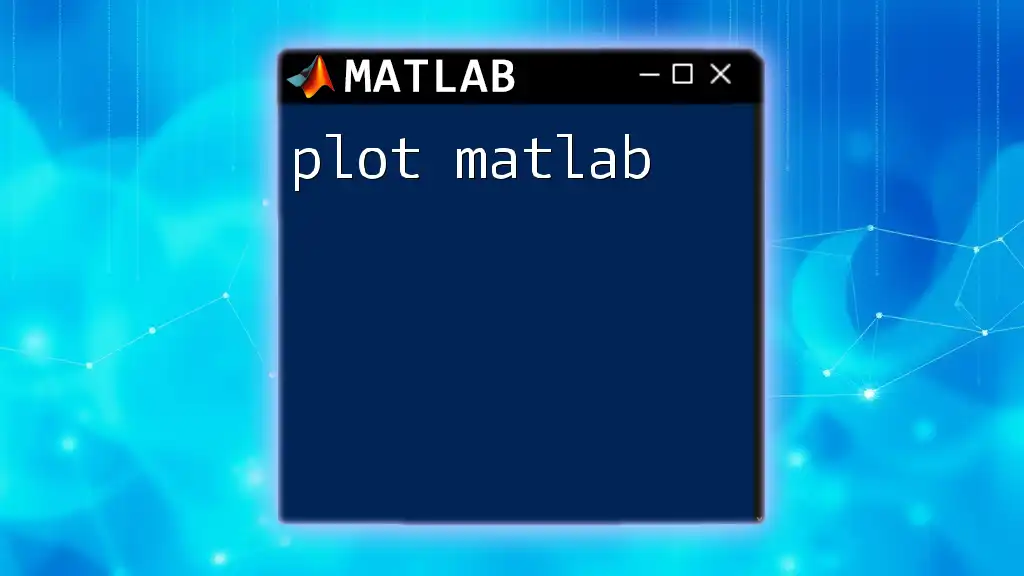
Conclusion
In this comprehensive guide on "plot graph matlab," we explored the essential functions and techniques for creating effective visualizations using MATLAB. By applying these concepts—starting from simple 2D plots to advanced 3D visualizations and customization techniques—you'll be well-equipped to present your data clearly and engagingly. Remember, the key to effective plotting lies in clarity and context, ensuring your audience can easily interpret the information conveyed by your graphs.
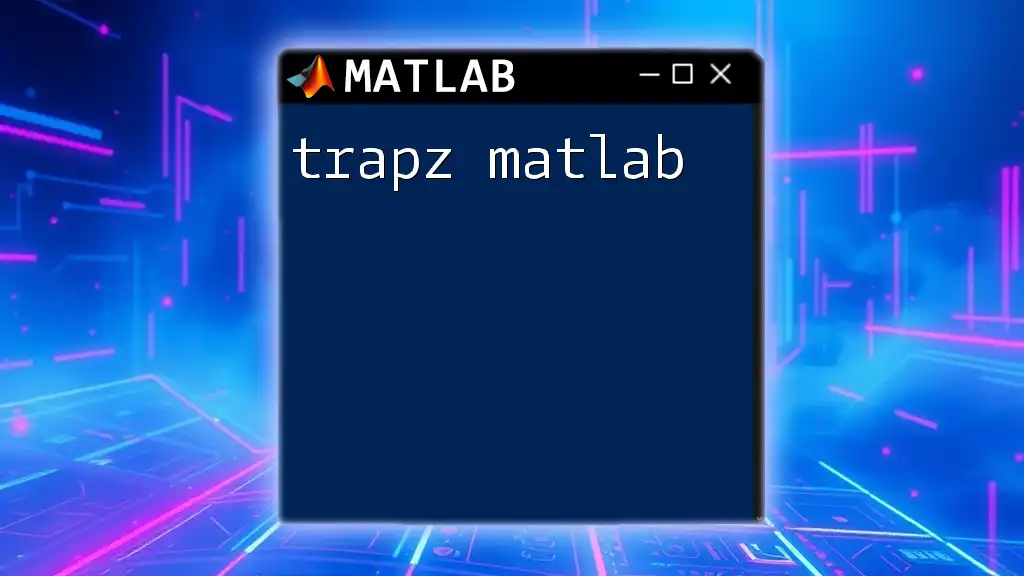
Additional Resources
MATLAB Documentation
The official MATLAB documentation contains detailed information on all plotting functions, providing an excellent resource for further learning.
Community Forums
Joining forums such as Stack Overflow or MATLAB Central can offer additional help and a platform to discuss queries with fellow MATLAB users.
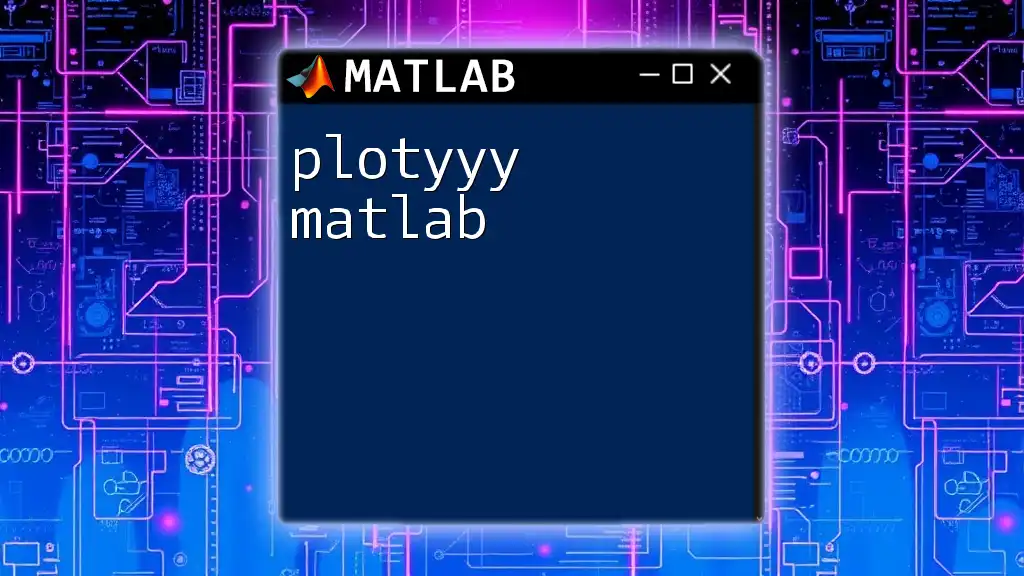
Frequently Asked Questions
Feel free to explore common questions surrounding plotting in MATLAB that can help deepen your understanding and tackle any challenges you may face along your data visualization journey.