A polar graph in MATLAB visualizes data in a radial format, displaying values based on angles and radii, and can be created using the `polarplot` function.
Here’s a simple example of how to create a polar graph in MATLAB:
theta = 0:0.01:2*pi; % Define the angle range from 0 to 2*pi
r = sin(2*theta); % Define the radius based on the sine function
polarplot(theta, r); % Create the polar plot
title('Polar Graph Example'); % Add a title to the plot
Introduction to Polar Graphs
What are Polar Graphs?
Polar graphs represent data in a circular format, where each point on the plane is defined by a distance from the origin (radius, \( r \)) and an angle (θ) from a reference direction. This representation is particularly useful in fields such as physics, engineering, and any discipline that involves wave patterns or circular motion.
Key Differences from Cartesian Coordinates
Unlike traditional Cartesian coordinates, which use \( x \) and \( y \) axes, polar coordinates employ a radial distance and an angular measurement. This means that, instead of plotting a point based on horizontal and vertical distances from the origin, you plot a point based on the angle and the distance from the origin. This can simplify the representation of spiral forms and circular relationships.
Importance of Polar Graphs in MATLAB
Polar graphs are essential for visualizing complex functions and phenomena that naturally fit a circular format. In engineering, they help visualize patterns in signal processing, while in physics, they can represent oscillatory motion. Knowing how to create and manipulate polar graphs in MATLAB is a crucial skill for many professionals.
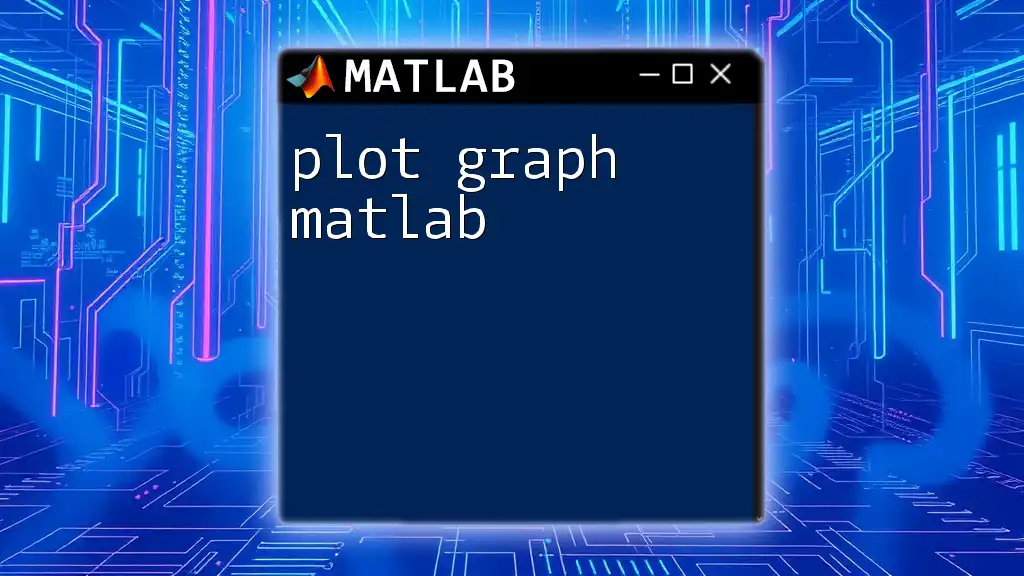
Getting Started with MATLAB
Installing and Setting Up MATLAB
To create a polar graph in MATLAB, ensure you have the software installed on your system. The installation is straightforward—follow the provided instructions on the official MathWorks website. Upon completion, launch MATLAB and familiarize yourself with the basic interface, which includes the Command Window and the Editor.
MATLAB Command Window and Editor
The Command Window is where you can execute single commands, while the Editor is used to write scripts that encompass multiple lines of code. Understanding how to use both efficiently will significantly enhance your productivity.
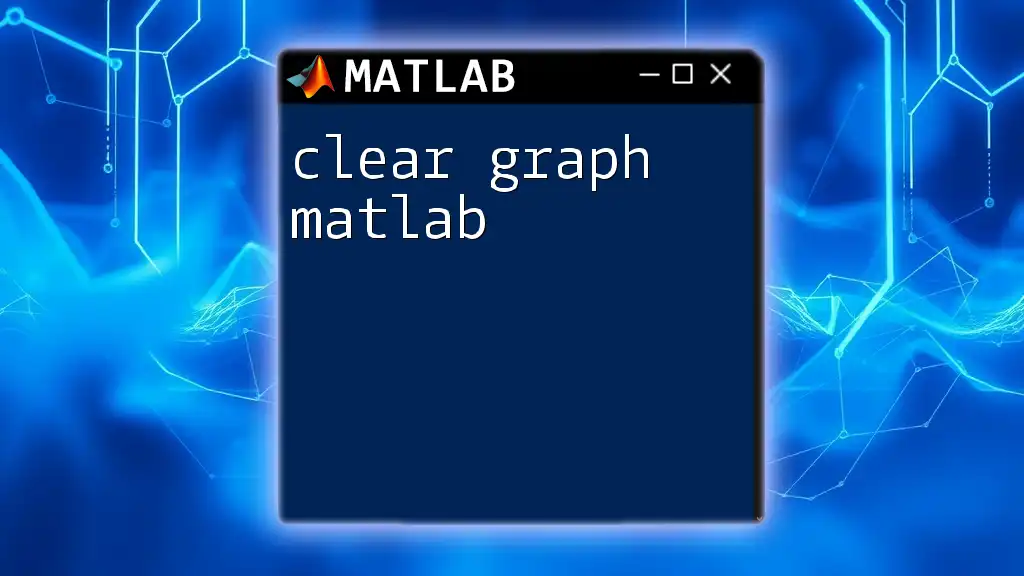
Basics of Polar Coordinates
Understanding Polar Coordinates
In polar coordinates, each point is determined by two unique values: the radius (\( r \)) and the angle (\( θ \)). The radius signifies how far the point is from the origin, while the angle indicates its direction.
How to Convert from Cartesian to Polar
To convert Cartesian coordinates \((x, y)\) to polar coordinates, use the formulas:
- \( r = \sqrt{x^2 + y^2} \)
- \( θ = \tan^{-1}\left(\frac{y}{x}\right) \)
MATLAB Support for Polar Coordinates
MATLAB provides built-in support for polar coordinates, allowing users to plot directly in polar formatting. Familiarizing yourself with polar-specific functions is crucial for effective visualization.
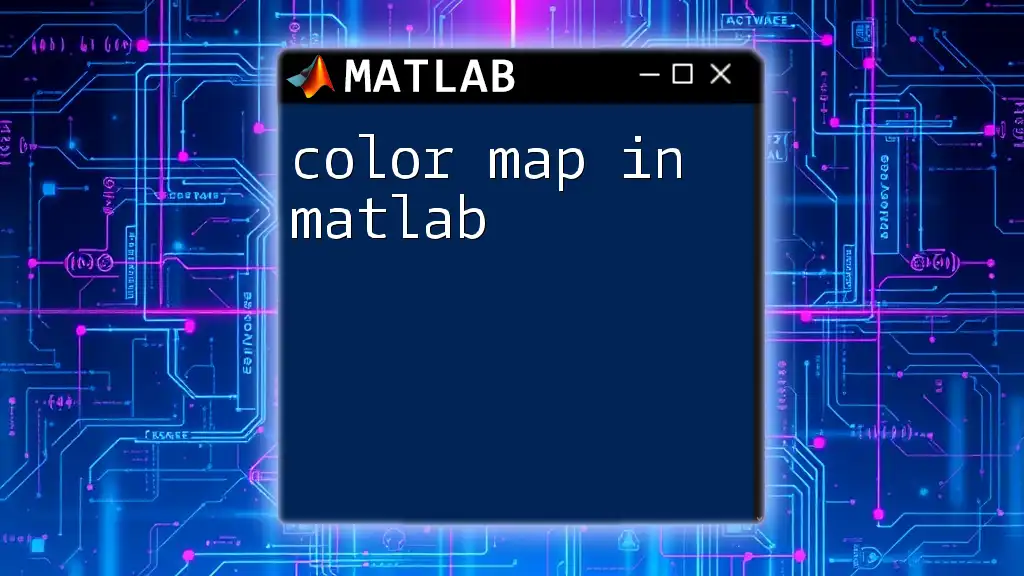
Creating Basic Polar Graphs in MATLAB
Using `polarplot()`
Introduction to the polarplot Function
The `polarplot()` function is the primary method for creating polar graphs in MATLAB. It transforms the data points into a circular plot with the radius and angle defined by your datasets.
Syntax and Basic Usage
The basic syntax to create a polar graph is:
polarplot(theta, r)
This command takes in two vectors: `theta` for angles and `r` for distances.
Example: Simple Polar Plot
Here’s a simple example of how to plot using `polarplot()`:
theta = linspace(0, 2*pi, 100); % Angles
r = sin(2*theta); % Radius
polarplot(theta, r) % Creating Polar Plot
title('Simple Polar Plot of r = sin(2*\theta)')
This example generates a polar plot of the sine function, creating a visually appealing representation of the sine wave.
Customizing Your Polar Plot
Adding Titles and Labels
To provide context to your graphs, titles and labels are essential. Use the following command to add a title:
title('My Polar Plot Example')
For axes labeling, adjust the polar grid using dedicated functions to make your plot more informative.
Changing Line Styles and Colors
Enhancing visual appeal can be achieved by altering line styles and colors within your polar plot. You can customize your plot like so:
polarplot(theta, r, 'r--') % Dashed Red Line
This commands MATLAB to plot a red dashed line, making it distinct and easier to interpret.
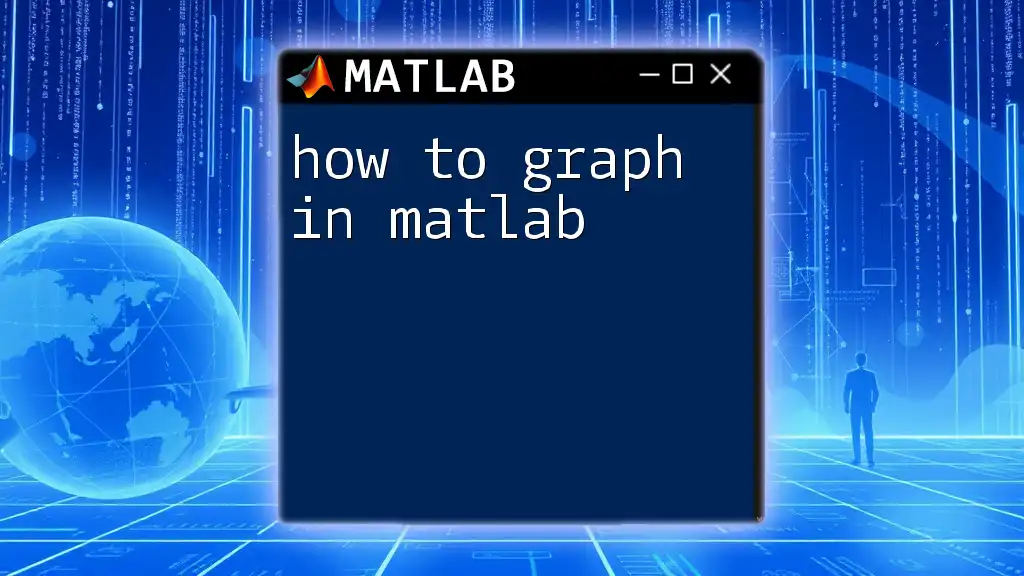
Advanced Polar Graph Techniques
Multiple Polar Curves
Plotting Multiple Functions
An exciting aspect of MATLAB is the ability to overlay multiple functions on the same polar graph. This can help in comparing different datasets or functions effectively.
Example: Overlaying Different Polar Functions
Here’s how you can plot multiple functions:
r1 = sin(theta);
r2 = cos(theta);
polarplot(theta, r1, theta, r2)
legend('r = sin(\theta)', 'r = cos(\theta)')
This code snippet overlays the sine and cosine functions, allowing you to visualize how they interact with each other.
Annotations and Legends
Adding Annotations on Polar Plots
Annotations significantly aid in clarifying specific points on your graph. You can annotate particular data points by using the following commands:
hold on
polarplot(pi/4, 1, 'ko') % Black Circle
text(pi/4, 1, 'Point A', 'VerticalAlignment', 'bottom')
In this example, a black circle marks a specific point on the plot, and a text annotation identifies it.
Working with Polar Grids and Axes
Customizing the Polar Grid
The appearance of the polar grid can also be modified for better readability. You can control grid appearance through commands like so:
polarplot(theta, r)
ax = gca; % Get current axes
ax.ThetaTick = [0 45 90 135 180 225 270 315]; % Custom Theta Ticks
ax.RLim = [0 1]; % Setting R limits
This example customizes the theta ticks and the radius limits, providing users with control over their plot's layout.
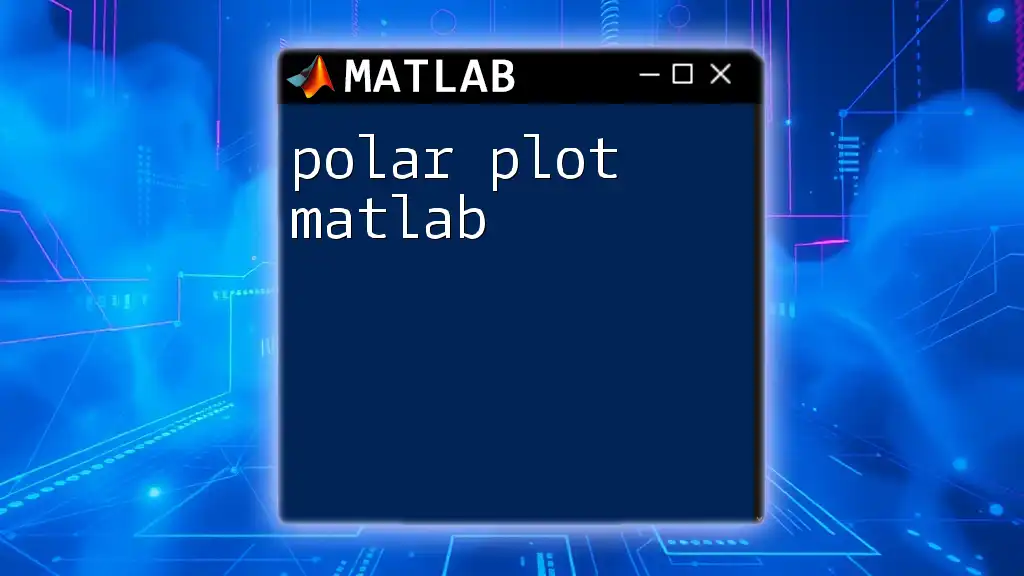
Troubleshooting Common Issues with Polar Graphs
Misalignment of Data Points
It's common to encounter misalignment when the radius or angle vector's lengths are inconsistent. Ensure both `theta` and `r` vectors are the same length to avoid this issue.
Plot Visibility Problems
If a plot doesn't appear as expected, check if the visibility is obstructed by the grid. Using commands to adjust axis limits can help your data stand out more clearly.
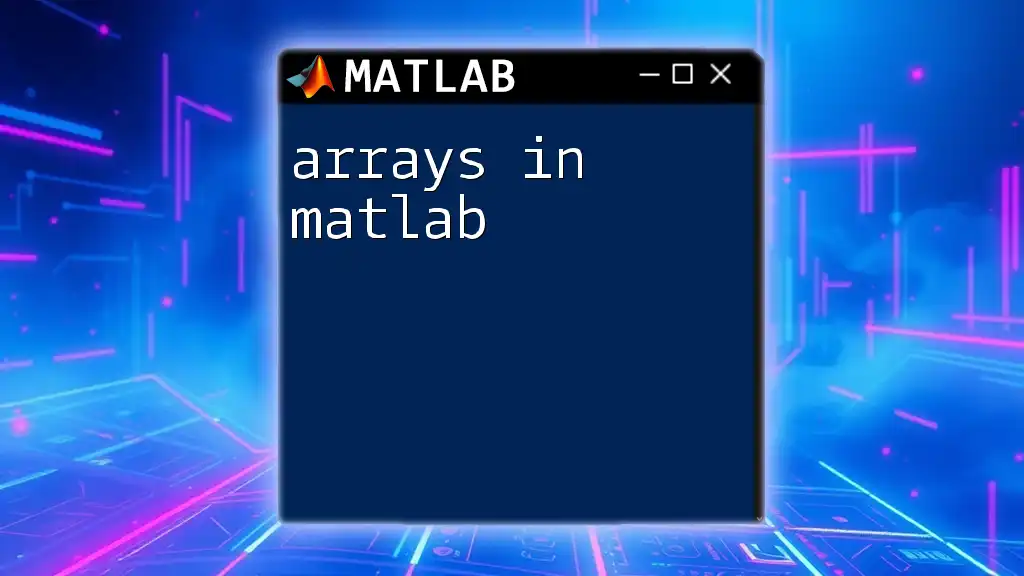
Real-World Examples and Applications
Using Polar Graphs in Data Visualization
Polar graphs are particularly effective in fields like engineering design. For instance, they can visually articulate the strength of signals in wireless broadcast layouts or the structural integrity represented by stress patterns.
Scientific Data Representation with Polar Graphs
In scientific research, polar coordinate systems are invaluable for representing phenomena such as waves. Suppose you are modeling water wave patterns. In that case, a polar graph can simplify the complexity involved in analyzing the interaction between different wave fronts.
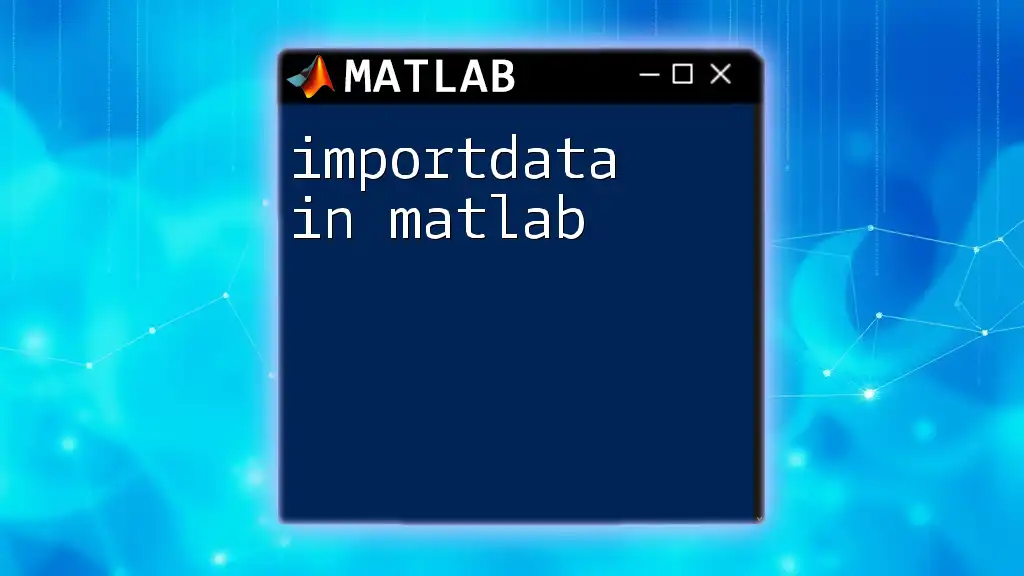
Conclusion
Summary of Key Points
Mastering polar graphs in MATLAB enhances your data visualization capabilities. From basic plots to advanced customizations, the range of features available allows users to create compelling and informative visual representations.
Next Steps for MATLAB Users
Continue exploring MATLAB’s extensive capabilities. Experiment with polar graphs, dive into advanced topics like data fitting and statistical modeling, and leverage additional resources to further your knowledge.
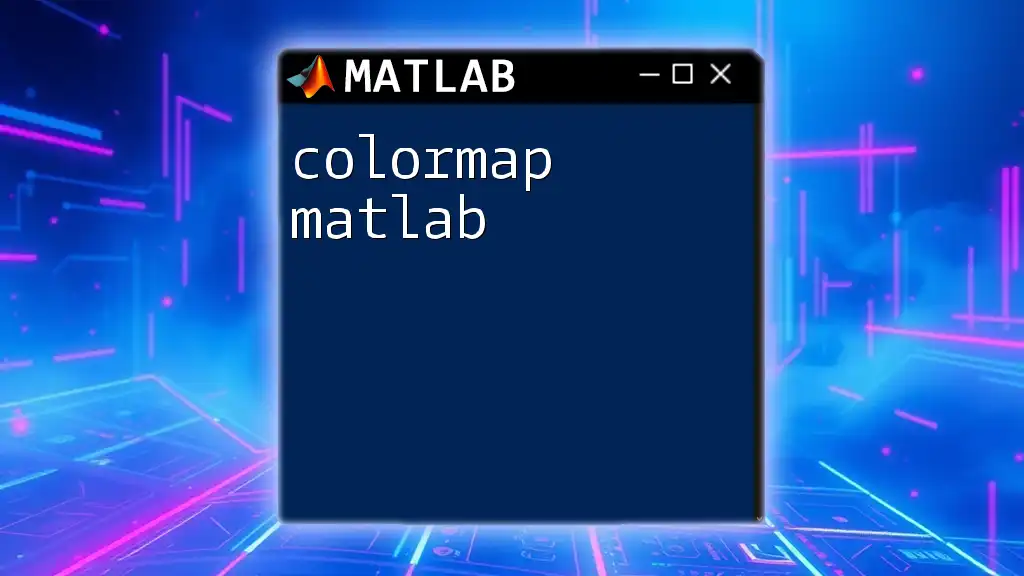
Additional Resources
Links to Documentation and Tutorials
Refer to the MATLAB documentation for detailed explanations of the functions discussed. Online courses and forums dedicated to MATLAB users can provide valuable insights and support.
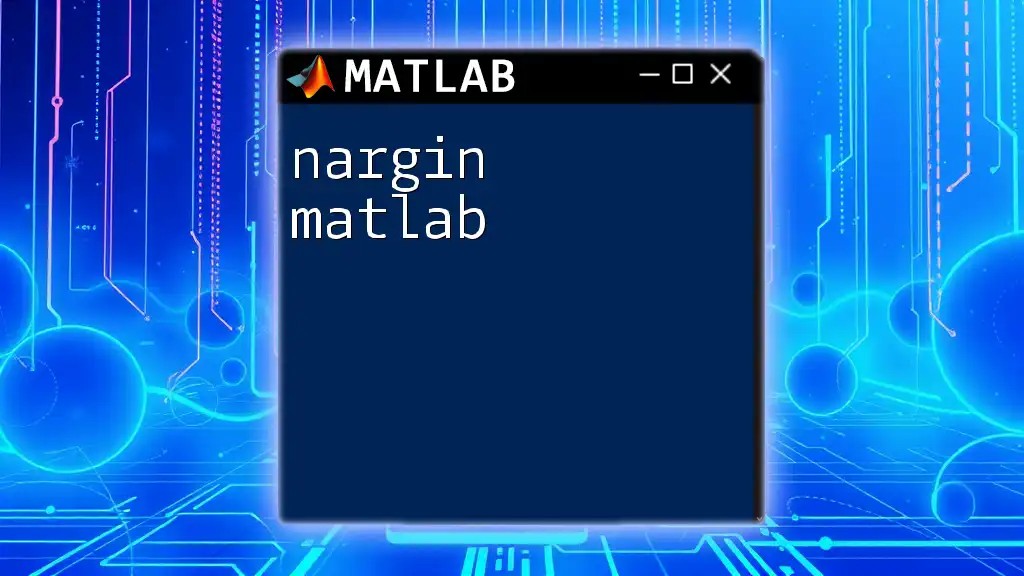
Call to Action
Join Our MATLAB Learning Community
Stay connected and enhance your skills by signing up for our newsletters, online courses, and more resources tailored to help you become proficient in MATLAB!