`accumarray` in MATLAB is a powerful function that aggregates values based on specified subscripts, allowing for efficient data accumulation and manipulation in an array format.
Here’s a simple example using `accumarray`:
% Example: Using accumarray to sum values based on subscripts
subs = [1; 2; 1; 3; 2; 1]; % Subscripts
vals = [10; 20; 30; 40; 50; 60]; % Values to accumulate
result = accumarray(subs, vals); % Aggregates values according to subs
disp(result); % Displays the output
In this example, `result` will contain the sums of `vals` corresponding to each unique subscript in `subs`.
What is `accumarray`?
`accumarray` is a powerful function in MATLAB designed for accumulating values based on specified indices. This function excels in scenarios where you need to aggregate data, particularly when dealing with large datasets. It allows users to group elements based on their corresponding subscripts, enabling efficient data analysis and manipulation.
In essence, `accumarray` streamlines the process of summarizing data, making it an invaluable tool in data processing applications such as finance, engineering, and scientific research.
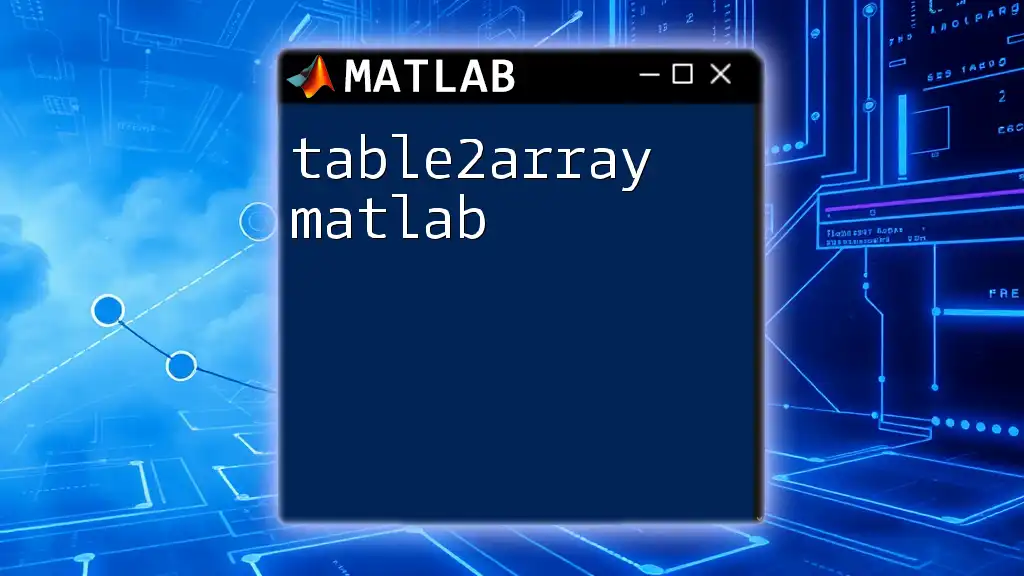
Why Use `accumarray`?
Utilizing `accumarray` comes with multiple advantages over traditional data aggregation functions. It significantly enhances readability of code by concisely expressing logic that might otherwise require multiple loops or additional functions. Furthermore, `accumarray` is optimized for performance, making it suitable for handling large arrays and complex datasets effectively.
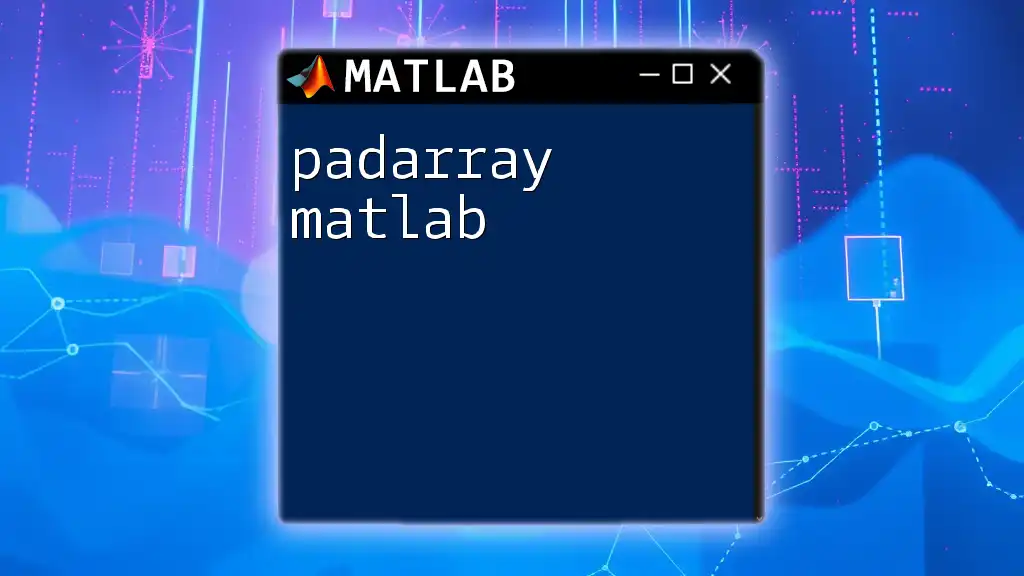
Understanding the Syntax of `accumarray`
Basic Syntax
The fundamental syntax of `accumarray` can be summarized as follows:
B = accumarray(subs, val)
Here, `subs` serves as the subscript indices, while `val` represents the values to be accumulated.
Detailed Explanation of Inputs
`subs`
The `subs` input defines the indices where the values in `val` will be placed in the output array `B`. This parameter can be a column vector of any length and is often used for linear indexing. For example:
subs = [1; 2; 1; 3];
This means we want to aggregate values based on these indices, with multiple entries for the same subscript potentially indicating accumulation.
`val`
The `val` array holds the values corresponding to the subscripts given in `subs`. The dimensions of `val` should match those of `subs`, and it can contain any numeric data type:
val = [10; 20; 30; 40];
Each entry in `val` will be associated with the respective subscript in `subs`.
Optional Parameters
The flexibility of `accumarray` increases with its optional parameters:
-
Initial Value: Specify a starting value for accumulation to avoid the default initialization:
B = accumarray(subs, val, [], [], 0); % starts with 0
-
Function Handle: Use a custom aggregation function to manipulate the accumulated values:
B = accumarray(subs, val, [], @(x) {sum(x) / numel(x)});
-
Size: Define the output array's size, ensuring the result conforms to specific dimensions:
B = accumarray(subs, val, [5, 1]);
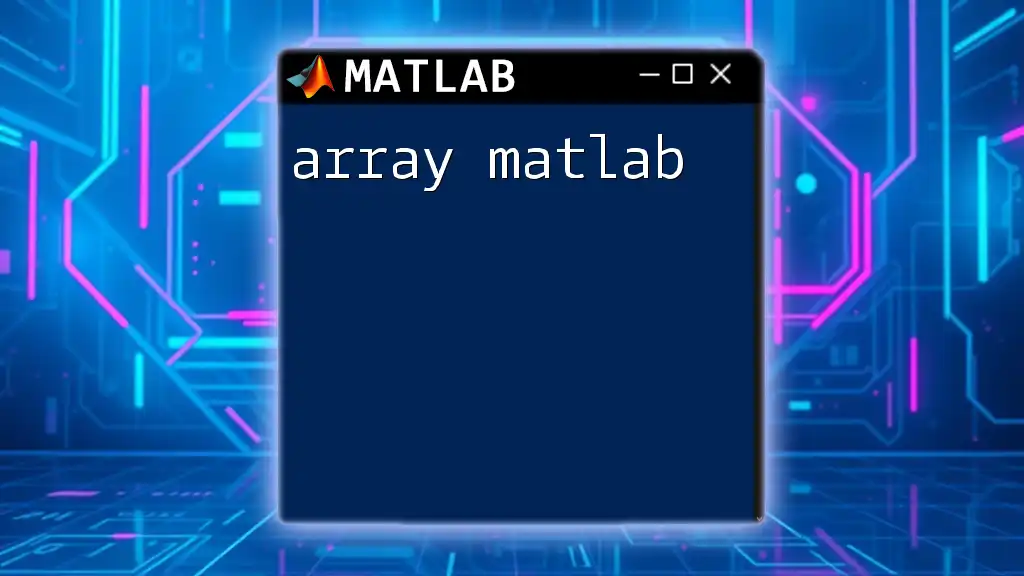
Example Usages of `accumarray`
Example 1: Basic Accumulation
Consider a simple example where we want to sum values based on their indices:
subs = [1; 2; 1; 3];
val = [10; 20; 30; 40];
B = accumarray(subs, val);
In this scenario, the output `B` would yield:
- `B(1) = 10 + 30 = 40`
- `B(2) = 20`
- `B(3) = 40`
Thus, `B` becomes:
B =
40
20
40
Example 2: Using Different Functions
The true strength of `accumarray` shines when different aggregation functions are employed. For instance, if we want to calculate the mean or maximum of grouped values:
subs = [1; 1; 2; 2; 3; 3];
val = [10; 20; 30; 40; 50; 60];
B_mean = accumarray(subs, val, [], @mean);
B_max = accumarray(subs, val, [], @max);
The output for `B_mean` would yield:
B_mean =
15
35
55
Meanwhile, `B_max` would generate:
B_max =
20
40
60
Example 3: Specifying Custom Sizes
When the dimensions of the expected output are known, `accumarray` can enforce these sizes to maintain structure:
subs = [1; 2; 3; 4; 5];
val = [5; 6; 2; 3; 4];
B = accumarray(subs, val, [5, 1]);
The output `B` in this case would maintain the shape defined by `[5, 1]`, neatly fitting the accumulated values based on the provided subscripts.
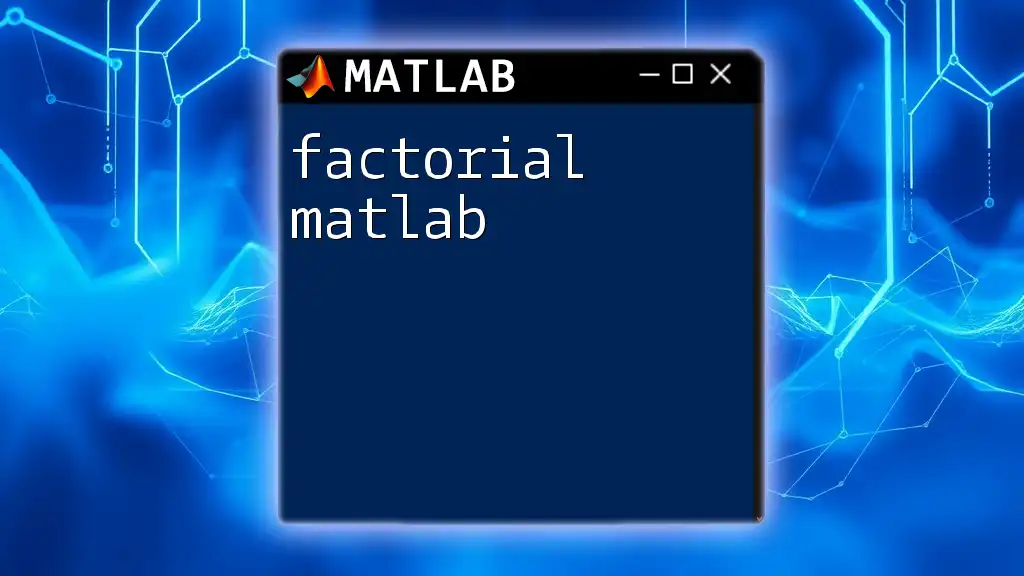
Advanced Features of `accumarray`
Working with Sparse Data
`accumarray` is adept at handling sparse data efficiently, avoiding the unnecessary overhead that can stem from dense representations. For example:
subs = [1; 2; 1; 3];
val = sparse([10; 0; 20; 0]);
B_sparse = accumarray(subs, val);
This effectively allows MATLAB to optimize memory usage, providing significant performance improvements when working with large datasets.
Combining with Other MATLAB Functions
Integrating `accumarray` with functions like `findgroups` can open up sophisticated methods for data operations. Here’s a practical application:
% Using findgroups with accumarray
data = [1 3; 4 5; 1 2; 4 6];
G = findgroups(data(:,1));
B = accumarray(G, data(:,2));
Here, `findgroups` identifies unique groups in `data`, allowing `accumarray` to operate seamlessly for aggregation.
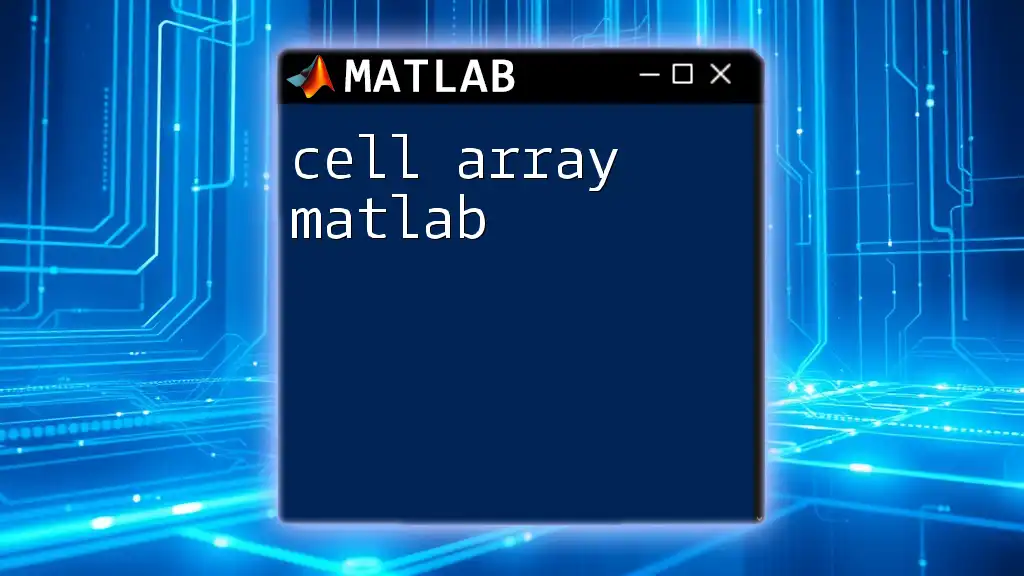
Common Mistakes and Troubleshooting
Incorrect Input Formats
One common pitfall involves mismatched array dimensions. If `subs` and `val` do not align correctly, it will result in an error. Always ensure that these arrays conform before executing `accumarray`.
Performance Considerations
When dealing with extensive datasets, performance can become crucial. To optimize:
- Keep your data types consistent (preferably numeric).
- Use built-in functions rather than custom function handles whenever possible.
- Preallocate space if you know the final size.
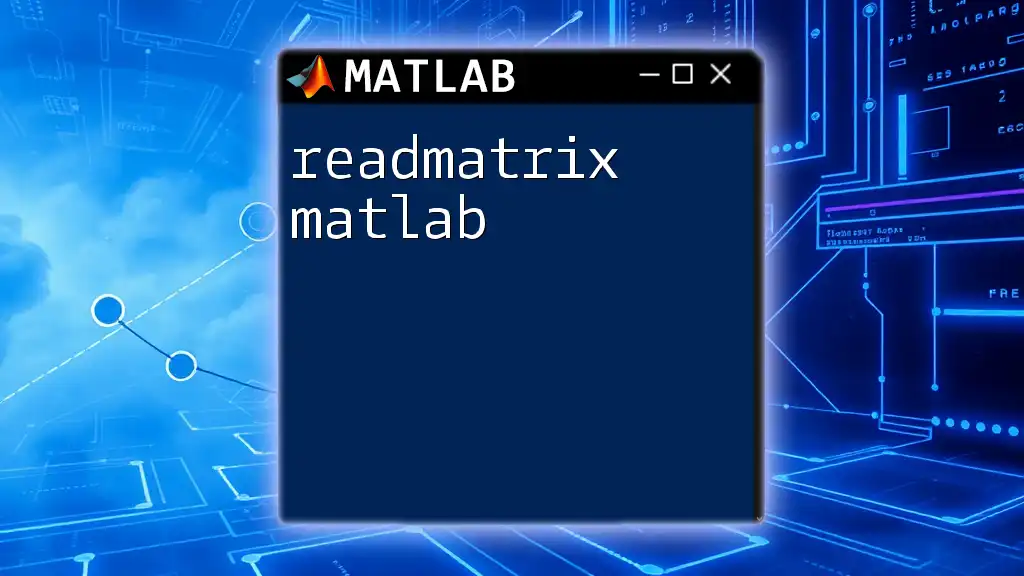
Conclusion
In summary, `accumarray` in MATLAB is a versatile tool that provides a straightforward approach to aggregating values based on indices. From basic summation to advanced custom aggregations, it encourages efficient data handling and processing. Users are encouraged to explore the nuances of `accumarray` further, especially in combination with other powerful MATLAB functions.
Whether you are a beginner or an advanced user, mastering `accumarray` will enhance your ability to manipulate and analyze data proficiently. Dive into your MATLAB environment, test these examples, and begin applying your newfound knowledge to practical scenarios.
Next Steps
To further your understanding, consider exploring additional resources or enrolling in courses that delve deeper into MATLAB functionalities and their applications. Practicing with `accumarray` in your projects will undoubtedly improve your data processing skills.