The `length` function in MATLAB returns the number of elements in the largest array dimension, providing a quick way to determine the size of vectors or matrices.
% Example usage of the length function
vec = [1, 2, 3, 4, 5];
len = length(vec); % len will be 5
Understanding the Length Command
What is the Length Command?
The `length` command in MATLAB is a built-in function that returns the length of the largest dimension of an array. This command is particularly useful when you are dealing with arrays or strings, as it provides a quick way to ascertain their sizes.
Basic Syntax
The syntax for using the `length` command is straightforward:
length(array)
Where `array` can be any numerical array, string, or other data types supported by MATLAB.
Types of Arrays in MATLAB
In MATLAB, arrays can take several forms, including row vectors, column vectors, and matrices. Understanding these distinctions is essential for using the `length` command effectively.
Row vs. Column Vectors
- A row vector is a one-dimensional array of elements arranged horizontally, like so:
rowVector = [1, 2, 3, 4, 5]; % This is a row vector
- A column vector, on the other hand, arranges elements vertically:
columnVector = [1; 2; 3; 4; 5]; % This is a column vector
When you apply the `length` command to a row vector, it returns the number of columns, while for a column vector, it returns the number of rows. This makes the command flexible but requires understanding of your data structure.
Matrices and Multi-dimensional Arrays
For matrices or higher-dimensional arrays, the `length` function returns the length of the largest dimension. Consider the following example:
matrix = [1, 2, 3; 4, 5, 6]; % A 2-by-3 matrix
lenMatrix = length(matrix); % This will return 3
In this case, since the largest dimension is the number of columns (3), that’s what is returned.
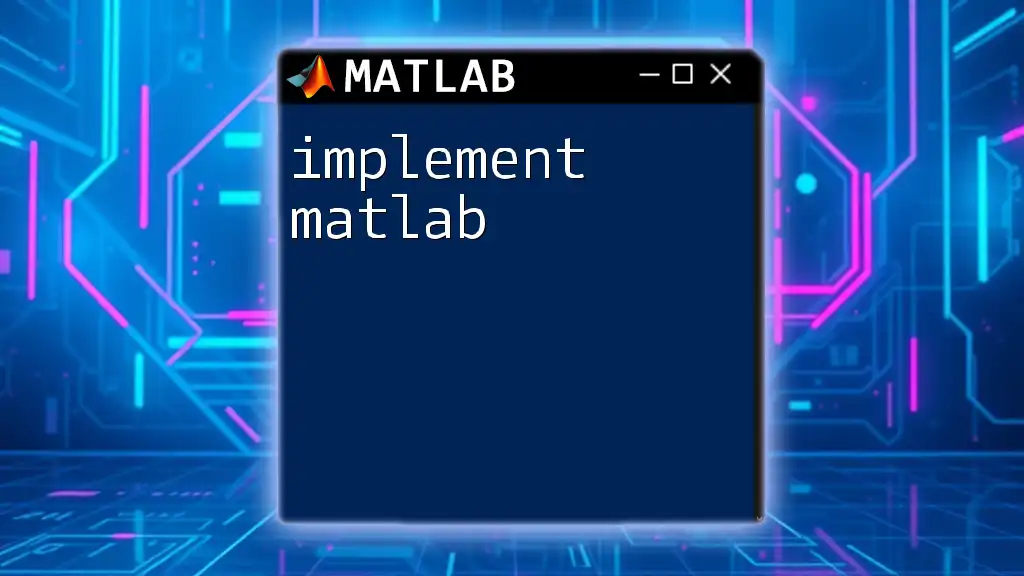
Using the Length Command
Basic Example of Length in Action
To see the `length` command in action, consider the following example where we calculate the length of a vector:
vector = [1, 2, 3, 4, 5];
len = length(vector); % len will be 5
Here, `len` returns 5, which is the number of elements in the vector.
Length with Different Data Types
Length of Numeric Arrays
The `length` command works seamlessly with numeric arrays. Here’s how you would use it:
numericArray = [10.1, 20.2, 30.3, 40.4];
lenNumeric = length(numericArray); % lenNumeric will be 4
Length of Strings
MATLAB also allows you to find the length of strings using the same command:
str = 'Hello, World!';
lenStr = length(str); % lenStr will be 13
In this case, `lenStr` counts all characters in the string, including the space and punctuation.
Length of Cell Arrays
For cell arrays, which can contain different types of data, the `length` function still applies:
cellArray = {1, 'hello', [1, 2, 3]};
lenCell = length(cellArray); % lenCell will be 3
Each cell counts as one element, regardless of the data type contained within.
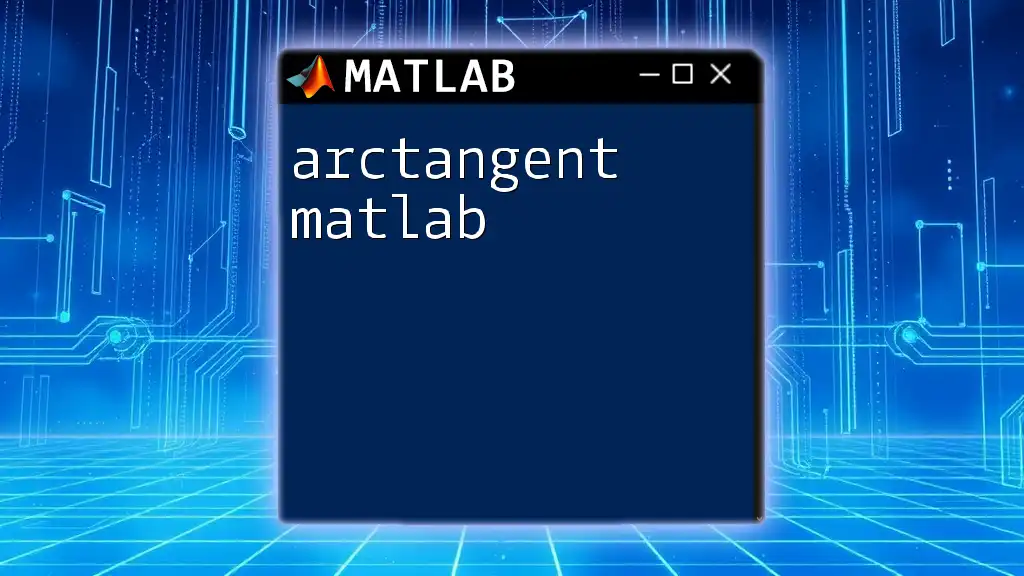
Functions Related to Length
Difference Between Length, Size, and Numel
While the `length` command is useful, it’s essential to understand its relationship with other functions like `size` and `numel`.
- `length()`: Returns the largest dimension of an array.
- `size()`: Provides the dimensions of an array.
- Syntax: `size(array)` returns a two-element vector: `[rows, columns]`.
- `numel()`: Returns the total number of elements in an array.
- Syntax: `numel(array)`
Here’s an example showcasing the differences:
array = [1, 2; 3, 4];
len = length(array); % Returns 2, the largest dimension (rows)
sz = size(array); % Returns [2, 2], showing 2 rows and 2 columns
num = numel(array); % Returns 4, the total number of elements
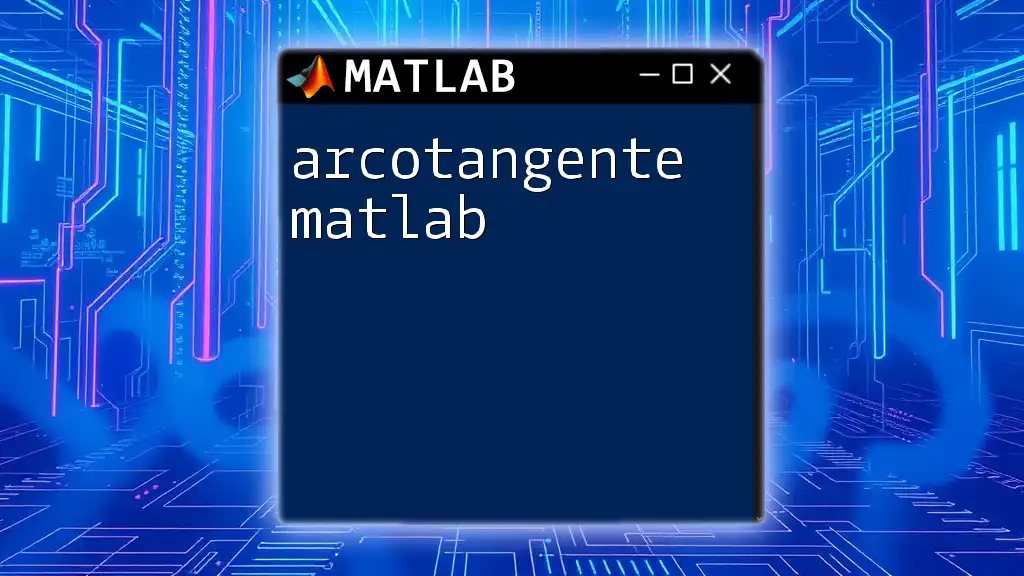
Common Errors and Troubleshooting
Common Mistakes When Using Length
Several common mistakes can arise when using the `length` command. Understanding these can save time and prevent errors in your code.
Example 1: Non-vector Input
Using the `length` command on non-vector inputs may yield unexpected results or errors. Make sure you are applying `length` to valid array types.
Example 2: Working with Empty Arrays
If you apply the `length` command to an empty array, the result will be 0. Here’s an example:
emptyArray = [];
lenEmpty = length(emptyArray); % lenEmpty will be 0
This behavior is important to account for in control structures.
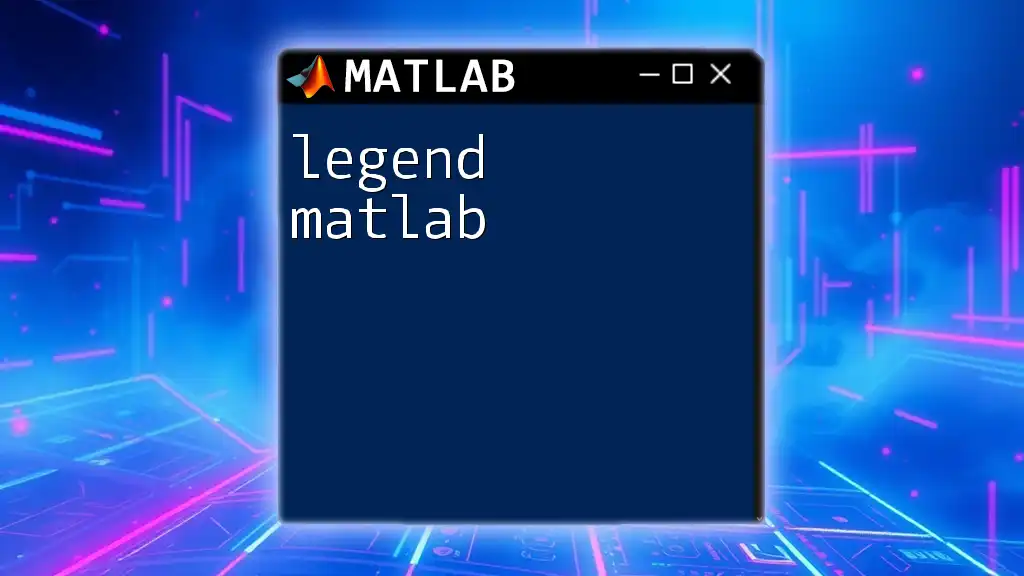
Practical Applications of Length in MATLAB
Leveraging Length in Control Structures
The `length` command can be particularly useful within loops and conditionals. For instance, consider how you might use it in a `for` loop:
for i = 1:length(vector)
disp(vector(i));
end
This loop dynamically adapts to the size of `vector`, displaying each element.
Data Analysis Use Cases
In data analysis, knowing the length of an array can help you adjust algorithms or structure data processing dynamically. For example, if you are plotting data, you can check the length to prevent errors in plotting functions that require consistent array sizes.
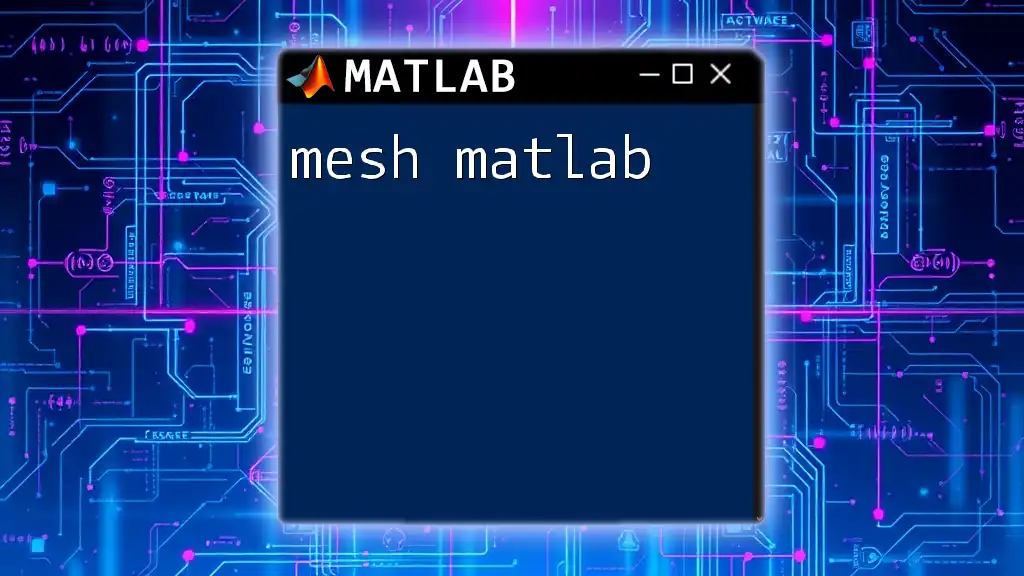
Conclusion
The `length` command in MATLAB is a powerful and versatile tool that enables efficient programming and data handling. By mastering the nuances of this command, including its relation to arrays and other built-in functions, you can enhance your MATLAB skills and optimize your code.
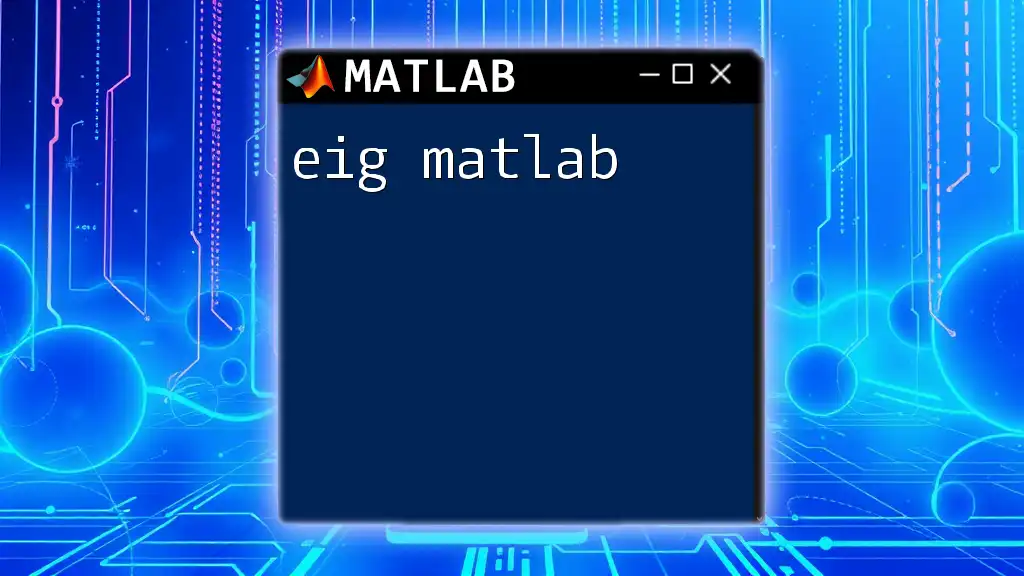
Additional Resources
For further exploration, consider diving into the official MATLAB documentation for more details on the `length` command and its applications. Additionally, various online resources and courses can provide you with in-depth knowledge about MATLAB programming.
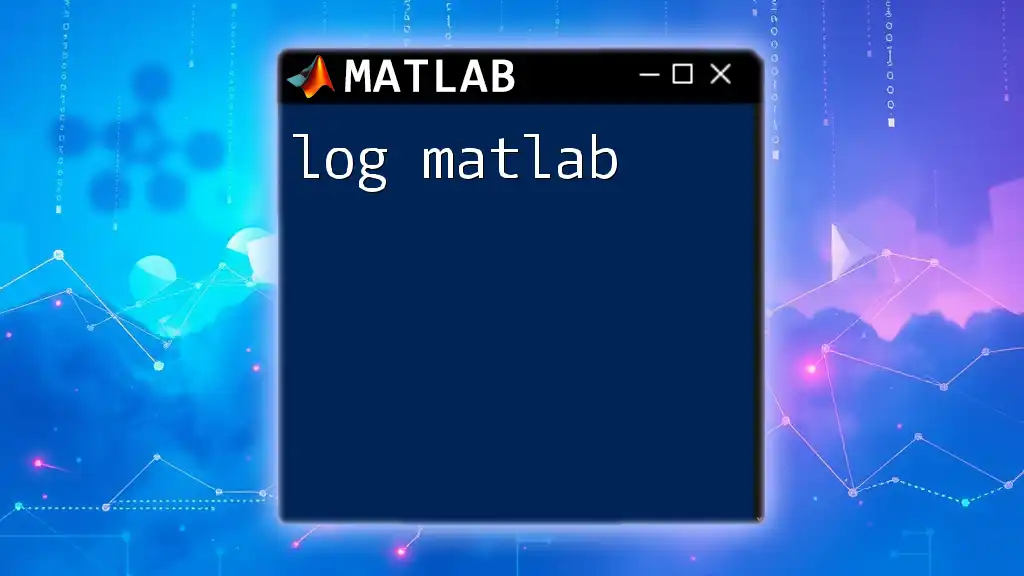
Call to Action
Join our MATLAB community to receive more practical tips, tutorials, and resources that can elevate your programming skills! Don't miss out on the chance to become a more proficient MATLAB user.